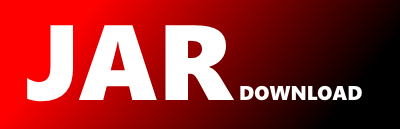
io.baltoro.remote.WSProbe2 Maven / Gradle / Ivy
package io.baltoro.remote;
import java.nio.ByteBuffer;
import java.util.logging.Logger;
import javax.servlet.http.HttpSession;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
import org.apache.tomcat.websocket.WsSession;
@ServerEndpoint(value = "/probe2")
public class WSProbe2
{
static Logger log = Logger.getLogger(WSProbe2.class.getSimpleName());
String examUuid;
ByteBuffer notFoundBytes = ByteBuffer.wrap(new byte[]{10,10,10,10});
int lastFrameSend;
String syncKey;
@OnOpen
public void onOpen(Session _session, EndpointConfig config)
{
WsSession session = (WsSession) _session;
System.out.println("Open Web WS Connection ... "+session);
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
if(httpSession == null)
{
System.out.println("restart browser ... httpsession is null");
}
examUuid = (String) httpSession.getAttribute("examUuid");
WSProbe1.examMap.put("WLPBHLA002", examUuid);
}
@OnClose
public void onClose(Session _session)
{
WsSession session = (WsSession) _session;
System.out.println("Close Web WS2 Connection ... "+session);
WSProbe1.examMap.remove("WLPBHLA002");
WSProbe1 probe = WSProbe1.probeMap.get("WLPBHLA002");
probe.sendMessage("stop");
}
@OnMessage
public void onMessage(String text, Session session) throws Exception
{
System.out.println(" >>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> income : "+text);
WSProbe1 probe = WSProbe1.probeMap.get("WLPBHLA002");
if(probe == null)
{
System.out.println(" can't find probe data .... may be not started ");
session.getBasicRemote().sendBinary(notFoundBytes);
return;
}
if(text.equals("stop"))
{
WSProbe1.examMap.remove("WLPBHLA002");
probe.sendMessage(text);
}
else
{
this.examUuid = text;
WSProbe1.examMap.put("WLPBHLA002", examUuid);
}
if(probe.lastFrame == -1)
{
try
{
Thread.sleep(500);
System.out.println("nothing to send >> ......... "+probe);
session.getBasicRemote().sendBinary(notFoundBytes);
return;
}
catch (Exception e)
{
e.printStackTrace();
}
}
if(lastFrameSend == probe.lastFrame)
{
//System.out.println(" lastframe is same : 1"+lastFrameSend);
String syncKey = examUuid+"-sync";
synchronized(syncKey.intern())
{
long t0 = System.currentTimeMillis();
syncKey.intern().wait(1000);
long t1 = System.currentTimeMillis();
//System.out.println(" ....... wait ("+(t1-t0)+") ");
}
if(lastFrameSend == probe.lastFrame)
{
System.out.println("live -> scan lastframe is same : 2 -> "+lastFrameSend);
session.getBasicRemote().sendBinary(notFoundBytes);
return;
}
}
byte[] bytes = probe.lastFrameBytes;
if(bytes != null)
{
System.out.println(" **** >>>>>>>>>>>>>> sending ("+bytes.length+") to brower {"+probe.lastFrame+"}");
session.getBasicRemote().sendBinary(ByteBuffer.wrap(bytes));
}
lastFrameSend = probe.lastFrame;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy