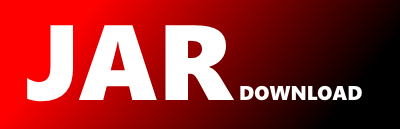
io.baltoro.remote.WSProbe3 Maven / Gradle / Ivy
package io.baltoro.remote;
import java.io.File;
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.logging.Logger;
import javax.servlet.http.HttpSession;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
import org.apache.tomcat.websocket.WsSession;
@ServerEndpoint(value = "/probe3")
public class WSProbe3
{
static Logger log = Logger.getLogger(WSProbe3.class.getSimpleName());
String examUuid;
ByteBuffer notFoundBytes = ByteBuffer.wrap(new byte[]{10,10,10,10});
int lastFrameSend;
String syncKey;
RandomAccessFile examFile;
int skipIndex;
int frames = 0;
byte[] bytes = new byte[1024 * 90];
byte[] lastFrame;
@OnOpen
public void onOpen(Session _session, EndpointConfig config)
{
WsSession session = (WsSession) _session;
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
if(httpSession == null)
{
System.out.println("restart browser ... httpsession is null");
}
examUuid = (String) httpSession.getAttribute("examUuid");
String dir = System.getProperty("user.home")+"/exams";
skipIndex = 0;
//Path path = Paths.get(dir+"/"+examUuid+".dat");
try
{
//bytes = Files.readAllBytes(path);
File f = new File(dir+"/"+examUuid+".dat");
examFile = new RandomAccessFile(f,"r");
}
catch (Exception e)
{
e.printStackTrace();
}
System.out.println("Open Web WS Connection ... "+session);
//WSProbe1.probeWebSession.put(session.getId(), session);
}
@OnClose
public void onClose(Session _session)
{
WsSession session = (WsSession) _session;
System.out.println("Close Web WS Connection ... "+session);
frames = 0;
skipIndex = 0;
try
{
examFile.close();
}
catch (Exception e)
{
e.printStackTrace();
}
//WSProbe1.probeWebSession.remove(session.getId());
}
@OnMessage
public void onMessage(String text, Session _session) throws Exception
{
WsSession session = (WsSession) _session;
WSProbe1 probe = WSProbe1.probeMap.get("WLPBHLA002");
String _examUuid = WSProbe1.examMap.get("WLPBHLA002");
if(_examUuid != null && examUuid.equals(_examUuid))
{
if(lastFrameSend == probe.lastFrame)
{
//System.out.println(" lastframe is same : 1"+lastFrameSend);
String syncKey = examUuid+"-sync";
synchronized(syncKey.intern())
{
long t0 = System.currentTimeMillis();
syncKey.intern().wait(1000);
long t1 = System.currentTimeMillis();
//System.out.println(" ....... wait ("+(t1-t0)+") ");
}
if(lastFrameSend == probe.lastFrame)
{
System.out.println(" recording -> scan lastframe is same : 2 -> "+lastFrameSend);
session.getBasicRemote().sendBinary(notFoundBytes);
return;
}
}
byte[] bytes = probe.lastFrameBytes;
if(bytes != null)
{
System.out.println(" **** >>>>>>>>>>>>>> sending ("+bytes.length+") to brower {"+probe.lastFrame+"}");
session.getBasicRemote().sendBinary(ByteBuffer.wrap(bytes));
}
lastFrameSend = probe.lastFrame;
return;
}
boolean loadMore = true;
if((skipIndex + bytes.length)> examFile.length())
{
skipIndex = (int)(examFile.length() - bytes.length - 10000);
}
examFile.seek(skipIndex);
int byteRead = examFile.read(bytes);
//System.out.println(" >>>>> bytes read : "+byteRead);
if(byteRead == -1)
{
System.out.println("file done ........... ");
session.getBasicRemote().sendBinary(ByteBuffer.wrap(lastFrame));
Thread.sleep(100);
loadMore = false;
//skipIndex = 0;
return;
}
int i = -1;
while(loadMore)
{
i++;
if(bytes[i] == 100 && bytes.length> (i+7) && bytes[i+1] == 100 && bytes[i+2] == 100 && bytes[i+3] == 100
&& bytes[i+4] == 100 && bytes[i+5] == 100 && bytes[i+6] == 100 && bytes[i+7] == 100)
{
frames++;
//System.out.println("-<"+frames+">--, i="+i);
byte[] frame = Arrays.copyOfRange(bytes, 0, i);
//skipIndex = i+8;
skipIndex += 8;
lastFrame = frame;
if(i == 0)
{
frame = notFoundBytes.array();
}
Thread.sleep(70);
session.getBasicRemote().sendBinary(ByteBuffer.wrap(frame));
loadMore = false;
return;
}
skipIndex++;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy