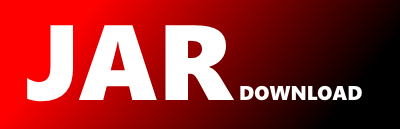
io.baltoro.remote.WSServer Maven / Gradle / Ivy
package io.baltoro.remote;
import java.net.InetAddress;
import java.nio.ByteBuffer;
import java.sql.Timestamp;
import java.util.List;
import java.util.Optional;
import java.util.logging.Logger;
import javax.servlet.http.HttpSession;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnError;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
import org.apache.tomcat.websocket.WsSession;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.baltoro.domain.App;
import io.baltoro.domain.BODefaults;
import io.baltoro.domain.BaltoroAppAPI;
import io.baltoro.domain.BaltoroInstance;
import io.baltoro.service.InstanceService;
import io.baltoro.service.ServiceFactory;
import io.baltoro.to.MgntContext;
import io.baltoro.to.ReplicationContext;
import io.baltoro.to.UserSessionContext;
import io.baltoro.to.WSTO;
import io.baltoro.util.StringUtil;
@ServerEndpoint(value = "/ws", configurator = WSSessionConfig.class)
public class WSServer
{
private App app;
private String instanceUuid;
private String eToken;
private Timestamp startedOn;
static Logger log = Logger.getLogger(WSServer.class.getSimpleName());
static ObjectMapper objectMapper = new ObjectMapper();
@OnOpen
public void onOpen(Session _session, EndpointConfig config)
{
startedOn = new Timestamp(System.currentTimeMillis());
WsSession session = (WsSession) _session;
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
app = (App) httpSession.getAttribute("BLT_APP");
instanceUuid = (String) httpSession.getAttribute("BLT_INSTANCE_UUID");
eToken = (String) httpSession.getAttribute("BLT_TOKEN");
System.out.println("=========> aap name = "+app.getName()+" --- "+instanceUuid);
SessionInstance instance = new SessionInstance(app.getBaseUuid(), app.getName(), instanceUuid, session);
WSSessions.get().addSession(instance);
System.out.println("Open Connection ..."+instance);
}
@OnClose
public void onClose(Session session)
{
System.out.println("Close Connection ... "+this.app.getName()+"-"+this.instanceUuid+"-"+session.getId());
WSSessions.get().removeSession(this.app.getBaseUuid(), this.instanceUuid, session.getId());
}
@OnMessage
public void onMessage(ByteBuffer buffer, Session _session)
{
WsSession session = (WsSession) _session;
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
if(httpSession != null)
{
String sesssionId = httpSession.getId();
}
WSTO to = null;
try
{
to = objectMapper.readValue(buffer.array(), WSTO.class);
}
catch (Exception e)
{
e.printStackTrace();
}
//if(to != null && to.uuid != null && to.webSocketContext == null)
if(to != null && to.uuid != null)
{
log.info(">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> uuid-->"+to.uuid+", data --- >"+to.responseContext.getData());
String uuid = to.uuid;
ResponseMap.getInstance().add(uuid, to);
synchronized (uuid.intern())
{
uuid.intern().notify();
}
}
else if(to != null && to.mgntContext != null)
{
//System.out.println(".................. received mgnt call ");
handleInstanceCall(to, session);
}
else if(to != null && to.replicationContext != null)
{
//System.out.println(".................. received replication call ");
handleReplicationCall(to, session);
}
else if(to != null && to.userSessionContext != null)
{
//System.out.println(".................. received user call ");
handleUserSession(to, session);
}
/*
else if(to != null && to.webSocketContext != null)
{
String uuid = to.uuid;
if(to.webSocketContext.getApiPath().endsWith("onopen"))
{
synchronized (uuid.intern())
{
uuid.intern().notify();
}
}
if(to.webSocketContext.getData() == null && to.webSocketContext.getMessage() == null)
{
return;
}
WsSession wsSession = WSSessionsWeb.get().getSession(to.webSocketContext.getWsSessionUuid());
if(wsSession != null)
{
//synchronized (to.webSocketContext.getWsSessionUuid().intern())
{
if(to.webSocketContext.getData() != null && to.webSocketContext.getData().length > 0)
{
ByteBuffer byteBuffer = ByteBuffer.wrap(to.webSocketContext.getData());
wsSession.getAsyncRemote().sendBinary(byteBuffer);
}
if(StringUtil.isNotNullAndNotEmpty(to.webSocketContext.getMessage()))
{
wsSession.getAsyncRemote().sendText(to.webSocketContext.getMessage());
}
}
}
}
*/
}
@OnError
public void onError(Throwable e)
{
e.printStackTrace();
}
private void handleUserSession(WSTO to, Session session)
{
UserSessionContext uctx = to.userSessionContext;
InstanceService service = ServiceFactory.get(InstanceService.class);
if(uctx.isInvalidateSession())
{
service.closeAppUserSession(uctx.getSessionUuid());
SessionCache.get().remove(uctx.getSessionUuid());
}
else
{
service.updateAppUserSessionAtt(uctx.getSessionUuid(), uctx.getPrincipalName(), uctx.getAttJson());
}
}
private void handleReplicationCall(WSTO to, Session session)
{
ReplicationContext ctx = to.replicationContext;
InstanceService service = ServiceFactory.get(InstanceService.class);
service.insert(to.appUuid, to.instanceUuid, ctx);
}
private void handleInstanceCall(WSTO to, Session session)
{
MgntContext ctx = to.mgntContext;
//log.info(" receving monitoring data ..."+ctx.getHeartBeatCount());
InstanceService service = ServiceFactory.get(InstanceService.class);
try
{
BaltoroInstance instance = service.get(to.instanceUuid);
if(instance == null)
{
instance = new BaltoroInstance();
instance.setAppUuid(to.appUuid);
instance.setUuid(to.instanceUuid);
instance.setStartedOn(new Timestamp(System.currentTimeMillis()));
instance.setThreadCount(ctx.getThreadCount());
instance.setCreatedBy(BODefaults.BASE_USER);
String localName = Optional.ofNullable(InetAddress.getLocalHost().getHostName()).orElse(InetAddress.getLocalHost().getHostAddress());
instance.setHostAddress(localName);
instance.setRemoteAddress("remote");
instance.setClusterPath(ctx.getClusterPath());
service.insert(instance);
}
if(startedOn != null && (instance.getStartedOn().getTime() < startedOn.getTime()))
{
service.updateInstanceStartedOn(to.instanceUuid, instance.getClusterPath(), "remote", ctx.getThreadCount());
}
service.update(to.instanceUuid, "LIVE", ctx.getCpuPercent(), ctx.getMemoryGB());
//session.getBasicRemote().sendText(""+ctx.getHeartBeatCount());
if(ctx.getPathTOs() != null)
{
List list = service.registerAppAPI(to.instanceUuid, to.appUuid, ctx.getPathTOs());
CachePathMap cache = CachePathMap.get();
for (BaltoroAppAPI baltoroAppAPI : list)
{
cache.addPathUuid(app.getBaseUuid(), baltoroAppAPI);
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy