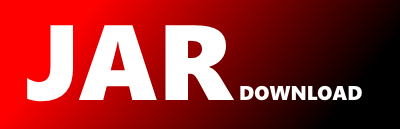
io.baltoro.remote.WSServerWeb Maven / Gradle / Ivy
package io.baltoro.remote;
import java.nio.ByteBuffer;
import java.sql.Timestamp;
import java.util.Map;
import java.util.logging.Logger;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpSession;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
import org.apache.tomcat.websocket.WsSession;
import io.baltoro.domain.App;
import io.baltoro.domain.AppUserSession;
import io.baltoro.domain.BaltoroAppAPI;
import io.baltoro.domain.ObjectTypeEnum;
import io.baltoro.service.Service;
import io.baltoro.service.ServiceFactory;
import io.baltoro.to.WSTO;
import io.baltoro.to.WebSocketContext;
@ServerEndpoint(value = "/websocketXYZ")
public class WSServerWeb
{
private Timestamp startedOn;
private String appName;
private App app;
private String path;
private SessionInstance sessionInstance;
private String eToken;
private AppUserSession appUserSession;
private BaltoroAppAPI api;
private WSTO to;
Map headerMap;
Map requestParams;
Cookie[] cookies;
String url;
static Logger log = Logger.getLogger(WSServerWeb.class.getSimpleName());
@OnOpen
public void onOpen(Session _session, EndpointConfig config)
{
System.out.println(" >>>>>>>>>>>>>>>>>>> Open Client WS Connection ... "+_session);
startedOn = new Timestamp(System.currentTimeMillis());
WsSession session = (WsSession) _session;
Service service = ServiceFactory.getInstance();
HttpSession httpSession = HTTPSessions.getSession(session.getHttpSessionId());
appName = (String) httpSession.getAttribute("BLT_APP_NAME");
app = (App) service.getByName(appName, ObjectTypeEnum.APPW);
if(app == null)
{
throw new RuntimeException("No app with name : "+appName);
}
path = (String) httpSession.getAttribute("BLT_METHOD_PATH");
eToken = (String) httpSession.getAttribute("BLT_TOKEN");
headerMap = (Map) httpSession.getAttribute("WEB_HEADER_MAP");
requestParams = (Map)httpSession.getAttribute("WEB_PARAM_MAP");
cookies = (Cookie[]) httpSession.getAttribute("WEB_COOKIE");
url = (String) httpSession.getAttribute("WEB_URL");
WSSessionsWeb.get().addSession(app, session);
sessionInstance = WSSessions.get().getSession(app.getBaseUuid(),null);
if(sessionInstance == null)
{
throw new RuntimeException("App Node is not up !! "+path);
}
appUserSession = BLTRequest.getAppUserSession(sessionInstance, cookies, httpSession);
CachePathMap pathCache = CachePathMap.get();
String openPath = path + "/onopen";
api = pathCache.getBaltoroAppPath(app.getBaseUuid(), openPath);
if(api == null)
{
throw new RuntimeException("resource not found "+api);
}
try
{
String wsSessionId = app.getBaseUuid()+"-"+session.getId();
to = BLTRequest.sendWebSocketRequest(sessionInstance, appUserSession, api, appName, openPath, requestParams, headerMap, wsSessionId);
String uuid = to.uuid;
synchronized (uuid.intern())
{
uuid.wait(5000);
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
@OnClose
public void onClose(Session session)
{
System.out.println(" >>>>>>>>>>>>>>>>>>>> Close Client WS Connection ... "+session);
WSSessionsWeb.get().removeSession(app.getBaseUuid(),session.getId());
String closePath = path + "/onclose";
try
{
String wsSessionId = app.getBaseUuid()+"-"+session.getId();
WebSocketContext ctx = new WebSocketContext();
ctx.setInitRequestUuid(to.uuid);
ctx.setApiPath(closePath);
ctx.setWsSessionUuid(wsSessionId);
to.webSocketContext = ctx;
WSSessions.get().send(to);
}
catch (Exception e)
{
e.printStackTrace();
}
}
@OnMessage
public void onMessage(ByteBuffer buffer, Session _session)
{
WsSession session = (WsSession) _session;
byte[] data = buffer.array();
String wsSessionId = app.getBaseUuid()+"-"+session.getId();
String key = wsSessionId+"-ws-push";
synchronized (key.intern())
{
WSTO mto = new WSTO();
mto.appName = to.appName;
mto.appUuid = to.appUuid;
mto.instanceUuid = to.instanceUuid;
mto.uuid = to.uuid;
WebSocketContext ctx = new WebSocketContext();
ctx.setInitRequestUuid(to.uuid);
ctx.setApiPath(path+"/onmessage");
ctx.setData(data);
ctx.setWsSessionUuid(wsSessionId);
mto.webSocketContext = ctx;
WSSessions.get().send(mto);
}
}
@OnMessage
public void onMessage(String msg, Session _session)
{
WsSession session = (WsSession) _session;
String wsSessionId = app.getBaseUuid()+"-"+session.getId();
String key = wsSessionId+"-ws-push";
synchronized (key.intern())
{
WSTO mto = new WSTO();
mto.appName = to.appName;
mto.appUuid = to.appUuid;
mto.instanceUuid = to.instanceUuid;
mto.uuid = to.uuid;
WebSocketContext ctx = new WebSocketContext();
ctx.setInitRequestUuid(to.uuid);
ctx.setApiPath(path+"/onmessage");
ctx.setMessage(msg);
ctx.setWsSessionUuid(wsSessionId);
mto.webSocketContext = ctx;
WSSessions.get().send(mto);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy