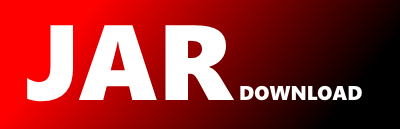
io.baltoro.remote.api.APIBO Maven / Gradle / Ivy
package io.baltoro.remote.api;
import java.io.IOException;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import org.apache.juli.logging.Log;
import org.apache.juli.logging.LogFactory;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import io.baltoro.domain.App;
import io.baltoro.domain.BO;
import io.baltoro.domain.Container;
import io.baltoro.domain.ObjectTypeEnum;
import io.baltoro.domain.User;
import io.baltoro.exception.ServiceException;
import io.baltoro.service.Ctx;
import io.baltoro.service.Service;
import io.baltoro.service.ServiceFactory;
import io.baltoro.service.TOFactory;
import io.baltoro.to.APIError;
import io.baltoro.to.AppTO;
import io.baltoro.to.BaseTO;
import io.baltoro.to.ContainerTO;
import io.baltoro.to.UserTO;
import io.baltoro.util.ObjectUtil;
import io.baltoro.util.StringUtil;
@Path("/bo")
public class APIBO extends APIBase
{
static Log log = LogFactory.getLog(APIBO.class);
@Override
void init()
{
}
@Path("/getContainer")
@GET
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.TEXT_PLAIN)
public ContainerTO getContainer(@QueryParam("uuid") String uuid)
throws ServiceException
{
Service service = ServiceFactory.getInstance();
Container bo = service.get(uuid, Container.class);
ContainerTO to = TOFactory.getTO(bo, ContainerTO.class);
return to;
}
@Path("/get")
@GET
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.TEXT_PLAIN)
public BaseTO get(@QueryParam("base-uuid") String baseUuid)
throws ServiceException
{
if(StringUtil.isNullOrEmpty(baseUuid))
{
log.info("=============================== base - uuid is null =============== ");
log.info("=============================== base - uuid is null =============== ");
log.info("=============================== base - uuid is null =============== ");
throw new APIError("base-uuid is null");
}
Service service = ServiceFactory.getInstance();
BO bo = service.get(baseUuid);
Class _class = (Class) ObjectUtil.fromUuidTO(baseUuid);
T to = TOFactory.getTO(bo, _class);
return to;
}
@Path("/getUserByUuid")
@GET
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.TEXT_PLAIN)
public UserTO getUserByUuid(@QueryParam("uuid") String baseUuid)
throws ServiceException
{
Service service = ServiceFactory.getInstance();
User bo = service.get(baseUuid, User.class);
UserTO to = TOFactory.getTO(bo, UserTO.class);
return to;
}
@Path("/getUserByEmail")
@GET
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.TEXT_PLAIN)
public UserTO getUserByEmail(@QueryParam("email") String email)
throws ServiceException
{
Service service = ServiceFactory.getInstance();
User user = (User) service.getByName(email, ObjectTypeEnum.USER);
UserTO to = TOFactory.getTO(user, UserTO.class);
return to;
}
@Path("/create")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_JSON)
public BaseTO create(BaseTO in)
throws ServiceException, JsonParseException, JsonMappingException, IOException
{
if(StringUtil.isNullOrEmpty(in.name))
{
throw new ServiceException("Name can't be null");
}
Service service = ServiceFactory.getInstance();
BO bo = service.createBO(in.name, ObjectTypeEnum.valueOf(in.type));
Class _class = (Class) ObjectUtil.fromType(bo.getObjectType());
BaseTO to = TOFactory.getTO(bo, _class);
return to;
}
@Path("/createUser")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public UserTO createUser(@FormParam("email") String email, @FormParam("password") String password, @Context HttpServletRequest request)
throws APIError, ServiceException,JsonParseException, JsonMappingException, IOException
{
Service service = ServiceFactory.getInstance();
User user = service.createUser(email, password);
HttpSession session = request.getSession(true);
session.setAttribute("auth-user", user);
UserTO to = TOFactory.getTO(user, UserTO.class);
return to;
}
@Path("/createContainer")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public ContainerTO createContainer(@FormParam("name") String name, @Context HttpServletRequest request)
throws ServiceException, JsonParseException, JsonMappingException, IOException
{
Service service = ServiceFactory.getInstance();
Container container = service.createContainer(name);
ContainerTO to = TOFactory.getTO(container, ContainerTO.class);
HttpSession session = request.getSession();
session.setAttribute("container-uuid", to.uuid);
return to;
}
@Path("/createApp")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public AppTO createApp(@FormParam("name") String name, @Context HttpServletRequest request)
throws ServiceException, JsonParseException, JsonMappingException, IOException
{
Service service = ServiceFactory.getInstance();
App app = service.createApp(name);
AppTO to = TOFactory.getTO(app, AppTO.class);
return to;
}
@Path("/getMyApps")
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public List getMyApps(@Context HttpServletRequest request)
throws ServiceException, JsonParseException, JsonMappingException, IOException
{
Service service = ServiceFactory.getInstance();
User user = Ctx.getUser();
List apps = service.findChildrenByType(user.getBaseUuid(), ObjectTypeEnum.APPW);
List> list = TOFactory.getTO(apps);
return (List) list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy