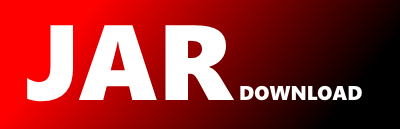
io.baltoro.remote.api.AppServlet Maven / Gradle / Ivy
package io.baltoro.remote.api;
import javax.servlet.http.HttpServlet;
public class AppServlet extends HttpServlet
{
/*
private static final long serialVersionUID = 1L;
static Log log = LogFactory.getLog(AppServlet.class);
@Override
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
//super.service(request, response);
try
{
byte[] bytes = process(request, response);
response.getOutputStream().write(bytes);
}
catch (Exception e)
{
throw new ServletException(e);
}
}
private byte[] process(HttpServletRequest request, HttpServletResponse response) throws Exception
{
System.out.println("**********************************");
System.out.println(request.getMethod());
System.out.println("**********************************");
String url = request.getRequestURL().toString();
String serverName = request.getServerName();
String appName = serverName.substring(0,serverName.indexOf('.'));
String _path = request.getRequestURL().toString();
String path = request.getRequestURL().toString();
int index = path.indexOf("/baltoro-app");
path = path.substring(index+12);
String instanceUuid = null;
Optional cookiesOpt = Optional.ofNullable(request.getCookies());
Cookie[] cookies = cookiesOpt.orElse(new Cookie[0]);
for (int i = 0; i < cookies.length; i++)
{
Cookie cookie = cookies[i];
if(cookie.getName().equals("BLT_INSTANCE_UUID"))
{
instanceUuid = cookie.getValue();
break;
}
}
SessionInstance sessionInstance = WSSessions.getInstance().getSession(appName,instanceUuid);
if(sessionInstance == null)
{
response.addHeader("BALTORO-ERROR", "App Node is not up !! "+path);
return ("App Node ["+appName+"] is not up !! "+path).getBytes();
}
if(instanceUuid==null || !instanceUuid.equals(sessionInstance.getInstanceUuid()))
{
Cookie cookie = new Cookie("BLT_INSTANCE_UUID", sessionInstance.getInstanceUuid());
response.addCookie(cookie);
}
WSTO to = new WSTO();
String uuid = UUID.randomUUID().toString();
to.uuid = uuid;
to.appName = appName;
to.path = path;
RequestContext rc = new RequestContext();
rc.requestParams = request.getParameterMap();
Enumeration headerNames = request.getHeaderNames();
Map headerMap = new HashMap(100);
while (headerNames.hasMoreElements())
{
String headerName = headerNames.nextElement();
String headerValue = request.getHeader(headerName);
headerMap.put(headerName, headerValue);
}
rc.headers = headerMap;
HttpSession httpSession = request.getSession(true);
rc.sessionId = httpSession.getId();
rc.ip = request.getRemoteAddr();
rc.url = request.getRequestURL().toString();
to.requestContext = rc;
try
{
byte[] data = ObjectUtil.toJason(to);
ByteBuffer buffer = ByteBuffer.wrap(data);
//sessionInstance.getSession().getAsyncRemote().sendBinary(buffer);
synchronized (sessionInstance.getInstanceUuid().intern())
{
sessionInstance.getSession().getBasicRemote().sendBinary(buffer);
}
//sessionInstance.getSession().getBasicRemote().sendBinary(buffer);
synchronized (uuid.intern())
{
uuid.intern().wait(10000);
WSTO responseTO = ResponseMap.getInstance().get(uuid);
if(responseTO != null)
{
System.out.println(" ----> found response "+responseTO);
if(responseTO.data == null)
{
return ("response is null for "+uuid).getBytes();
}
return responseTO.data;
}
else
{
System.out.println(" ----> response not found "+uuid);
return ("response not found for uuid "+uuid).getBytes();
}
}
}
catch (Exception e)
{
e.printStackTrace();
new ServiceException(e);
}
return path.getBytes();
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy