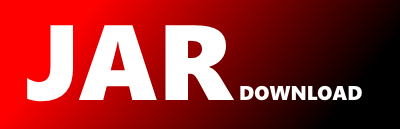
io.baltoro.service.ConnUtil Maven / Gradle / Ivy
package io.baltoro.service;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.Collection;
import java.util.Iterator;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.sql.DataSource;
import org.apache.commons.dbcp2.cpdsadapter.DriverAdapterCPDS;
import org.apache.commons.dbcp2.datasources.SharedPoolDataSource;
import io.baltoro.domain.BO;
import io.baltoro.exception.ServiceException;
public class ConnUtil
{
//static Connection tempConn = null;
static private SharedPoolDataSource localPool;
private static Connection getServerConnection()
throws ServiceException
{
try
{
Context initContext = new InitialContext();
Context envContext = (Context)initContext.lookup("java:comp/env");
DataSource datasource = (DataSource)envContext.lookup("jdbc/baltoro");
Connection con = datasource.getConnection();
return con;
}
catch(Exception e)
{
throw new ServiceException(e);
}
}
public static void closeConnection(Connection con)
{
String runType = System.getProperty("run-type");
if(runType != null)
{
return;
}
try
{
if(con != null)
{
con.close();
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static void close(PreparedStatement stmt)
{
try
{
if(stmt != null)
{
stmt.close();
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static void close(ResultSet rs)
{
try
{
if(rs != null)
{
rs.close();
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static Connection getConnection()
throws ServiceException
{
String runType = System.getProperty("catalina.home");
if(runType != null)
{
return getServerConnection();
}
else
{
return getLocalConnection();
}
}
private static Connection getLocalConnection()
throws ServiceException
{
try
{
if(localPool != null)
{
return localPool.getConnection();
}
DriverAdapterCPDS cpds = new DriverAdapterCPDS();
//cpds.setDriver("org.gjt.mm.mysql.Driver");
cpds.setDriver("com.mysql.jdbc.Driver");
cpds.setUrl("jdbc:mysql://db.baltoro.io:3306/baltoro?useSSL=false");
cpds.setUser("baltoro");
cpds.setPassword("baltoro7");
localPool = new SharedPoolDataSource();
localPool.setConnectionPoolDataSource(cpds);
localPool.setMaxTotal(10);
localPool.setDefaultMaxWaitMillis(50);
return localPool.getConnection();
//Driver driver = (Driver) Class.forName("com.mysql.jdbc.Driver").newInstance();
//tempConn = DriverManager.getConnection("jdbc:mysql://baltoro-db:3306/baltoro?user=baltoro&password=baltoro7");
}
catch (Exception e)
{
throw new ServiceException(e);
}
}
static String toInClause(String[] array)
{
StringBuilder buffer = new StringBuilder(array.length * 10);
for (String val : array)
{
buffer.append("'"+val+"',");
}
buffer.deleteCharAt(buffer.length()-1);
return buffer.toString();
}
static String toInClause(Collection col)
{
if(col==null || col.isEmpty())
{
return "";
}
StringBuilder buffer = new StringBuilder(col.size() * 10);
Iterator it = col.iterator();
while(it.hasNext())
{
String val = it.next();
buffer.append("'"+val+"',");
}
buffer.deleteCharAt(buffer.length()-1);
return buffer.toString();
}
static String toInClauseFromBO(Collection col)
{
if(col==null || col.isEmpty())
{
return "";
}
StringBuilder buffer = new StringBuilder(col.size() * 10);
Iterator it = col.iterator();
while(it.hasNext())
{
BO val = it.next();
buffer.append("'"+val.getBaseUuid()+"',");
}
buffer.deleteCharAt(buffer.length()-1);
return buffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy