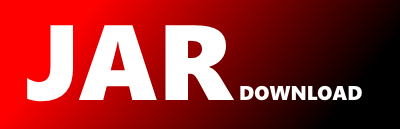
io.baltoro.service.DaoImpl Maven / Gradle / Ivy
package io.baltoro.service;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import io.baltoro.domain.BO;
import io.baltoro.domain.BOBase;
import io.baltoro.domain.BOMD;
import io.baltoro.domain.MetadataMap;
import io.baltoro.domain.ObjectTypeEnum;
import io.baltoro.domain.Permission;
import io.baltoro.exception.CreateNotAllowedException;
import io.baltoro.exception.DAOException;
import io.baltoro.exception.NotFoundException;
import io.baltoro.exception.PermissionException;
import io.baltoro.exception.ReadNotAllowedException;
import io.baltoro.exception.ServiceException;
import io.baltoro.util.ObjectUtil;
import io.baltoro.util.StringUtil;
import io.baltoro.util.UUIDGenerator;
public class DaoImpl implements Dao
{
protected static final Logger log = Logger.getLogger(DaoImpl.class.getName());
DaoImpl()
{
// TODO Auto-generated constructor stub
}
public BO getBOByBaseUuid(String baseUuid)
throws ServiceException,NotFoundException,ReadNotAllowedException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
//PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObject());
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectWithPermission());
stmt.setString(1, baseUuid);
ResultSet rs = stmt.executeQuery();
if (rs.next())
{
BO bo = buildBO(rs);
return bo;
}
else
{
throw new NotFoundException(baseUuid+" not found");
}
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
catch (ServiceException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw e;
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List getBOs(List uuids) throws ServiceException
{
Connection con = null;
List boList = new ArrayList(uuids.size());
Map boMap = new HashMap(uuids.size());
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
String in = ConnUtil.toInClause(uuids);
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectsWithPermission(in));
ResultSet rs = stmt.executeQuery();
while (rs.next())
{
BO bo = null;
try
{
bo = buildBO(rs);
boMap.put(bo.getBaseUuid(), bo);
}
catch (ReadNotAllowedException e)
{
log.info("read not allowed for "+bo.getBaseUuid());
}
}
for (String uuid : uuids)
{
BO bo = boMap.get(uuid);
if(bo != null)
{
boList.add(bo);
}
}
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
return boList;
}
public List findBOByName(String name, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Connection con = null;
List list = new ArrayList(100);
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectWithPermissionByNameTypeContainer());
stmt.setString(1, name);
stmt.setString(2, type.toString());
stmt.setString(3, containerUuid);
ResultSet rs = stmt.executeQuery();
while (rs.next())
{
try
{
BO bo = buildBO(rs);
list.add(bo);
}
catch (ReadNotAllowedException e)
{
log.info("read not allowed");
}
}
return list;
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List find(String name, ObjectTypeEnum type)
throws ServiceException
{
Connection con = null;
List list = new ArrayList(100);
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectWithPermissionByNameType());
stmt.setString(1, name);
stmt.setString(2, type.toString());
ResultSet rs = stmt.executeQuery();
while (rs.next())
{
try
{
BO bo = buildBO(rs);
list.add(bo);
}
catch (ReadNotAllowedException e)
{
log.info("read not allowed");
}
}
return list;
}
catch (Exception e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List findByStateType(String state, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Connection con = null;
List list = new ArrayList(100);
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.OBJECT_BY_STATE_TYPE_SELECT);
stmt.setString(1, state);
stmt.setString(2, type.toString());
stmt.setString(3, containerUuid);
ResultSet rs = stmt.executeQuery();
while (rs.next())
{
try
{
BO bo = buildBO(rs);
list.add(bo);
}
catch (ReadNotAllowedException e)
{
log.info("read not allowed");
}
}
return list;
}
catch (SQLException e)
{
e.printStackTrace();
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public int countByStateType(String state, ObjectTypeEnum type, String containerUuid)
throws ServiceException
{
Connection con = null;
int count = 0;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.COUNT_BY_STATE_TYPE);
stmt.setString(1, state);
stmt.setString(2, type.toString());
stmt.setString(3, containerUuid);
ResultSet rs = stmt.executeQuery();
if(rs.next())
{
count = rs.getInt(1);
}
return count;
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List findBOByMetadata(String colName, String value)
throws ServiceException
{
Connection con = null;
List list = new ArrayList(100);
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
//PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObject());
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectWithPermissionByMetadata(colName));
stmt.setString(1, value);
ResultSet rs = stmt.executeQuery();
while (rs.next())
{
try
{
BO bo = buildBO(rs);
list.add(bo);
}
catch (ReadNotAllowedException e)
{
log.info("read not allowed");
}
}
return list;
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
private T buildBO(ResultSet rs)
throws SQLException, ServiceException
{
String _type = rs.getString("ob.object_type");
Class _class = (Class) ObjectUtil.fromType(_type);
T t = buildBO(rs, _class);
return t;
}
private T buildBO(ResultSet rs, Class type)
throws SQLException, ServiceException
{
/*
base_uuid
name
state
object_type
container_uuid
latest_version_uuid
permission_type
created_by
created_on
*/
String baseUuid = rs.getString("ob.base_uuid");
BO bo = BOCache.instance().get(baseUuid);
if(bo != null)
{
Permission p = bo.getPermission(Ctx.getUserUuid());
if(p == null)
{
p = getPermission(rs, bo.getBaseUuid());
bo.addPermission(Ctx.getUserUuid(), p);
}
if(p instanceof NullPermission || !p.isRead())
{
throw new ReadNotAllowedException(baseUuid, Ctx.getUserUuid());
}
System.out.println("^^^^^^^^^^^^^^^^^^");
System.out.println("^^^^^^^^^^^^^^^^^^");
System.out.println("^^^^^^^["+bo.getBaseUuid()+"] rerturn from cache ^^^^^^^^^^^");
System.out.println("^^^^^^^^^^^^^^^^^^");
return type.cast(bo);
}
String _type = rs.getString("ob.object_type");
if(type != null)
{
bo = (BO)ObjectUtil.initBOByType(type);
}
else
{
bo = ObjectUtil.initBOByType(_type);
}
Permission p = getPermission(rs, baseUuid);
bo.addPermission(Ctx.getUserUuid(), p);
bo.setObjectType(_type);
bo.setBaseUuid(baseUuid);
String name = rs.getString("ob.name");
bo.setName(name);
String state = rs.getString("ob.state");
bo.setState(state);
String containerUuid = rs.getString("ob.container_uuid");
bo.setContainerUuid(containerUuid);
String latestVersionUuid = rs.getString("ob.latest_version_uuid");
bo.setLatestVersionUuid(latestVersionUuid);
bo.setVersionUuid(latestVersionUuid);
String permissionType = rs.getString("ob.permission_type");
bo.setPermissionType(permissionType);
String createdBy = rs.getString("ob.created_by");
bo.setCreatedBy(createdBy);
Timestamp createdOn = rs.getTimestamp("ob.created_on");
bo.setCreatedOn(createdOn);
int versionNumber = rs.getInt("version_number");
bo.setVersionNumber(versionNumber);
loadMetadata(rs, bo);
BOCache.instance().add(bo.getBaseUuid(), bo);
if(p instanceof NullPermission || !p.isRead())
{
throw new ReadNotAllowedException(baseUuid, Ctx.getUserUuid());
}
T t = type.cast(bo);
return t;
}
private Permission getPermission(ResultSet rs, String baseUuid)
throws SQLException, ServiceException
{
String per = rs.getString("op");
if(StringUtil.isNullOrEmpty(per))
{
return new NullPermission();
}
boolean read = per.charAt(0) == '1' ? true : false;
boolean create = per.charAt(1) == '1' ? true : false;
boolean update = per.charAt(2) == '1' ? true : false;
boolean delete = per.charAt(3) == '1' ? true : false;
boolean relation = per.charAt(4) == '1' ? true : false;
boolean share = per.charAt(5) == '1' ? true : false;
boolean grant = per.charAt(6) == '1' ? true : false;
Permission p = new Permission(baseUuid, Ctx.getUserUuid(),
read, create, update, delete, relation, share, grant);
return p;
}
private void loadMetadata(ResultSet rs, BO bo)
throws SQLException, ServiceException
{
BOMD[] bomdArray = bo.getMDDef();
for (BOMD bomd : bomdArray)
{
String colName = bomd.getColType();
if(StringUtil.isNotNullAndNotEmpty(colName))
{
String dbValue = rs.getString(colName);
String mdName = bomd.getName();
bo.addValue(mdName, dbValue);
}
}
}
// @Override
/*
public void saveObjectDef(BODef def)
throws ServiceException, CreateNotAllowedException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.insertObjectDef());
Set mdNames = def.getMDMap().keySet();
for (String mdName : mdNames)
{
MetadataMap md = def.getMetadata(mdName);
stmt.setString(1, def.get);//def_version_uuid
stmt.setString(2, md.getMetadataName());//metadata_name
stmt.setString(4, md.getColumnName());//column_name
stmt.setString(5, md.getDataType());//data_type
stmt.setString(6, md.getDefaultValue());//default_value
stmt.setString(7, md.getEnumValues());//enum_values
stmt.setBoolean(8, md.isRequired());//is_required
stmt.setBoolean(9, md.isEncrypted());//is_encrypted
stmt.setBoolean(10, md.isSearchable());//is_searchable
stmt.setString(11, Context.getUserUuid());//created_by
stmt.addBatch();
}
int[] row = stmt.executeBatch();
log.info("row inserted");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
*/
public void save(BO bo) throws ServiceException,PermissionException
{
BOCache.instance().remove(bo.getBaseUuid());
Connection con = null;
try
{
con = ConnUtil.getConnection();
insertBO(bo, con);
}
catch (Exception e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateState(BO bo, String state) throws ServiceException,PermissionException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.OBJECT_BASE_STATE_UPDATE_QUERY);
stmt.setString(1, state);
stmt.setString(2, bo.getBaseUuid());
int row = stmt.executeUpdate();
bo.setState(state);
BOCache.instance().remove(bo.getBaseUuid());
}
catch (Exception e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
private void updateBO(BOBase bo, Connection con)
throws ServiceException,CreateNotAllowedException
{
try
{
BOCache.instance().remove(bo.getBaseUuid());
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.updateObjectBase());
String versionUuid = UUIDGenerator.uuid(bo.getObjectType());
bo.setVersionUuid(versionUuid);
bo.setLatestVersionUuid(versionUuid);
int versionNumber = bo.getVersionNumber();
bo.setVersionNumber(++versionNumber);
//update object_base set name=?,state=?,latest_version_uuid=?,created_by=? where object_base_uuid=?;
stmt.setString(1, bo.getName());//name
stmt.setString(2, bo.getState());//state
stmt.setString(3, bo.getLatestVersionUuid());//latest_version_uuid
stmt.setString(4, bo.getPermissionType());//permission_type
stmt.setString(5, bo.getCreatedBy());//created_by
stmt.setString(6, bo.getBaseUuid());//object_base_uuid
int row = stmt.executeUpdate();
//log.info("row updated");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
}
private void insertBO(BOBase bo, Connection con)
throws ServiceException,CreateNotAllowedException
{
try
{
//QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.OBJECT_BASE_INSERT_QUERY);
String containerUuid = bo.getContainerUuid();
/*
if(bo.getObjectType().equals(ObjectTypeEnum.USER.toString()))
{
containerUuid = BODefaults.BASE_CONTAINER;
}
*/
stmt.setString(1, bo.getBaseUuid());//base_uuid
stmt.setString(2, bo.getName());//name
stmt.setString(3, bo.getState());//state
stmt.setString(4, bo.getObjectType());//object_type
stmt.setString(5, containerUuid);//container_uuid
stmt.setString(6, bo.getLatestVersionUuid());//latest_version_uuid
stmt.setString(7, bo.getPermissionType());//permission_type
stmt.setString(8, bo.getCreatedBy());//created_by
int row = stmt.executeUpdate();
//log.info("row inserted");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
}
private void insertPermissions(String baseUuid, Set permissionSet, String roleUuid)
throws ServiceException,CreateNotAllowedException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.insertPermission());
for (String permission : permissionSet)
{
stmt.setString(1,baseUuid);//base_uuid
stmt.setString(2, permission);//psermission
stmt.setString(3, roleUuid);//state
stmt.setString(8, Ctx.getUserUuid());//created_by
stmt.addBatch();
}
int[] rows = stmt.executeBatch();
//log.info("rows inserted");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void deleteBO(String baseUuid) throws ServiceException
{
BOCache.instance().remove(baseUuid);
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.DELETE_OBJECT_BASE_BY_UUID_QUERY);
stmt.setString(1,baseUuid);
int row = stmt.executeUpdate();
stmt = con.prepareStatement(QueryBuilder.METADATA_DELETE_QUERY);
stmt.setString(1,baseUuid);
row = stmt.executeUpdate();
stmt = con.prepareStatement(QueryBuilder.PERMISSION_DELETE_QUERY);
stmt.setString(1,baseUuid);
row = stmt.executeUpdate();
stmt = con.prepareStatement(QueryBuilder.RELATIONSHIP_DELETE_P_QUERY);
stmt.setString(1,baseUuid);
row = stmt.executeUpdate();
stmt = con.prepareStatement(QueryBuilder.RELATIONSHIP_DELETE_C_QUERY);
stmt.setString(1,baseUuid);
row = stmt.executeUpdate();
//log.info("rows delete");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void deleteMetadata(BO bo) throws ServiceException,PermissionException
{
BOCache.instance().remove(bo.getBaseUuid());
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.deleteMetadata());
stmt.setString(1,bo.getBaseUuid());//base_uuid
int row = stmt.executeUpdate();
//log.info("rows delete");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateMetadata(BO bo, BOMD[] bomds) throws ServiceException,PermissionException
{
BOCache.instance().remove(bo.getBaseUuid());
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.updateMetadata(bomds));
int colNumber = 1;
for (BOMD bomd : bomds)
{
String value = bo.getMDMap().get(bomd.getName());
//log.info(" >>>>> ********** >>>>>>>>> "+value);
stmt.setString(colNumber,value);//base_uuid
colNumber++;
}
stmt.setString(colNumber, bo.getBaseUuid());
stmt.executeUpdate();
//log.info("rows delete");
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void saveMetadata(BO bo) throws ServiceException,CreateNotAllowedException
{
BOCache.instance().remove(bo.getBaseUuid());
Connection con = null;
try
{
QueryBuilder queryBuilder = new QueryBuilder();
BOMD[] mds = bo.getMDDef();
String query = queryBuilder.getBOMetadataInsertQuery(mds);
//log.info(query);
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(query);
int i=0;
stmt.setString(++i, bo.getVersionUuid());
stmt.setString(++i, bo.getBaseUuid());
stmt.setInt(++i, bo.getVersionNumber());
stmt.setString(++i, Ctx.getUserUuid());
stmt.setString(++i, "uri");
for (BOMD md : mds)
{
++i;
String val = bo.getValue(md.getName());
if(StringUtil.isNullOrEmpty(val))
{
String deault = md.getDefaultValue();
val = deault;
}
//log.info("MD name ("+md.getMetadataName()+"), value="+val);
if(md.getColType().startsWith("str"))
{
stmt.setString(i, val);
}
else if(md.getColType().startsWith("int"))
{
if(StringUtil.isNullOrEmpty(val))
{
stmt.setNull(i, java.sql.Types.INTEGER);
}
else
{
int _val = Integer.parseInt(val);
stmt.setInt(i, _val);
}
}
else if(md.getColType().startsWith("bol"))
{
if(StringUtil.isNullOrEmpty(val))
{
stmt.setNull(i, java.sql.Types.BOOLEAN);
}
else if(val.equals("1"))
{
stmt.setBoolean(i, true);
}
else
{
stmt.setBoolean(i, false);
}
}
else if(md.getColType().startsWith("dts"))
{
if(StringUtil.isNullOrEmpty(val))
{
stmt.setNull(i, java.sql.Types.TIMESTAMP);
}
else
{
//Date date = Date.parse(val);
Timestamp _val = new Timestamp(System.currentTimeMillis());
stmt.setTimestamp(i, _val);
}
}
}
int row = stmt.executeUpdate();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
/*
private void copyMetadataMap(BO bo)
throws ServiceException,CreateNotAllowedException
{
String type = bo.getObjectType();
if(type.equals(ObjectTypeEnum.BCNT.toString())
|| type.equals(ObjectTypeEnum.BUSR.toString())
|| type.equals(ObjectTypeEnum.CUST.toString()))
{
return;
}
String copyFromContainerUuid = null;
String copyFromtypeVersionUuid = null;
if(type.equals(ObjectTypeEnum.CONT.toString()))
{
copyFromContainerUuid = Context.BASE_CONTAINER;
copyFromtypeVersionUuid = Context.BASE_CONTAINER;
}
else if(type.equals(ObjectTypeEnum.DOMN.toString()))
{
copyFromContainerUuid = Context.BASE_CONTAINER;
copyFromtypeVersionUuid = Context.BASE_DOMAIN;
}
else if(type.equals(ObjectTypeEnum.ROLE.toString()))
{
copyFromContainerUuid = Context.BASE_CONTAINER;
copyFromtypeVersionUuid = Context.BASE_ROLE;
}
else if(type.equals(ObjectTypeEnum.USER.toString()))
{
copyFromContainerUuid = Context.BASE_CONTAINER;
copyFromtypeVersionUuid = Context.BASE_USER;
}
Connection con;
try
{
con = getTestConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.getMetadataMapCopyQuery());
stmt.setString(1, bo.getContainerUuid());//container_uuid
stmt.setString(2, bo.getVersionUuid());//object_type_version_uuid
stmt.setString(3, Context.getUserUuid());//created_by
stmt.setString(4, copyFromContainerUuid);//copy_from
stmt.setString(5, copyFromtypeVersionUuid);//copy_from_object_type_uuid
int row = stmt.executeUpdate();
log.info("Metadata map inserted rows ("+row+") for "+bo.getBaseUuid());
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
//con.close();
}
}
*/
public boolean objectExists(String name, String containerUuid, ObjectTypeEnum type)
throws ServiceException
{
boolean found = false;
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectBaseByNameType());
stmt.setString(1, name);//name
stmt.setString(2, containerUuid);//conatinerUuid
stmt.setString(3, type.toString());//bo type
ResultSet rs = stmt.executeQuery();
if(rs.next())
{
found = true;
}
rs.close();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
return found;
}
/*
public BODef getDefByBaseUuid(String baseUuid)
throws ServiceException, NotFoundException, ReadNotAllowedException
{
if (!baseUuid.startsWith("D"))
{
throw new ServiceException("baseuuid not a valid BO definition uuid");
}
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectObjectBaseByUUD());
stmt.setString(1, baseUuid);
ResultSet rs = stmt.executeQuery();
boolean found = false;
BODef def = new BODef();
while (rs.next())
{
found = true;
def.setBaseUuid(baseUuid);
String name = rs.getString(2);
def.setName(name);
String state = rs.getString(3);
def.setState(state);
String type = rs.getString(4);
def.setObjectType(type);
String defBaseUuid = rs.getString(5);
def.setDefBaseUuid(defBaseUuid);
String containerUuid = rs.getString(6);
def.setContainerUuid(containerUuid);
String latestVersionUuid = rs.getString(7);
def.setLatestVersionUuid(latestVersionUuid);
def.setVersionUuid(latestVersionUuid);
String permissionType = rs.getString(8);
def.setPermissionType(permissionType);
String createdBy = rs.getString(9);
def.setCreatedBy(createdBy);
Timestamp createdOn = rs.getTimestamp(10);
def.setCreatedOn(createdOn);
}
rs.close();
stmt.close();
if(!found)
{
throw new NotFoundException(baseUuid+" not found");
}
Map mdMap = getObjectDefCoumnMap(def.getVersionUuid());
def.setMdMap(mdMap);
return def;
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
throw new DAOException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
*/
private Map getObjectDefCoumnMap(String defVersionUuid)
throws ServiceException, SQLException
{
//String containerUuid = bo.getContainerUuid();
//String versionTypeUuid = bo.getVersionUuid();
Map cols = new HashMap(50);
Connection con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.getObjectDefSelectQuery());
stmt.setString(1, defVersionUuid);
ResultSet rs = stmt.executeQuery();
while(rs.next())
{
MetadataMap md = new MetadataMap();
md.setDefVersionUuid(rs.getString(1));
md.setMetadataName(rs.getString(2));
md.setDefBaseUuid(rs.getString(3));
md.setColumnName(rs.getString(4));
md.setDataType(rs.getString(5));
md.setDefaultValue(rs.getString(6));
md.setEnumValues(rs.getString(7));
md.setEncrypted(rs.getBoolean(8));
md.setSearchable(rs.getBoolean(9));
cols.put(md.getMetadataName(),md);
}
rs.close();
stmt.close();
//con.close();
return cols;
}
/*
public void savePermissions(BOBase base, String sysBaseUuid, PermissionTypeEnum permission)
throws ServiceException
{
Connection con;
try
{
con = ConnUtil.getTestConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.insertPermission());
String userUuid = Context.getUserUuid();
stmt.setString(1, base.getBaseUuid());//base_uuid
stmt.setString(2, permission.toString());//permission
stmt.setString(3, sysBaseUuid);//role_uuid
stmt.setString(4, userUuid);//userUuid
for (String permission : permissions)
{
//base_uuid,permission,role_uuid,created_by
stmt.setString(1, base.getBaseUuid());//base_uuid
stmt.setString(2, permission);//permission
stmt.setString(3, sysBaseUuid);//role_uuid
stmt.setString(4, userUuid);//userUuid
stmt.addBatch();
}
int row = stmt.executeUpdate();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
//con.close();
}
}
*/
public void savePermissions(Permission p)
throws ServiceException
{
BOCache.instance().remove(p.getBaseUuid());
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.insertPermission());
String userUuid = Ctx.getUserUuid();
stmt.setString(1, p.getBaseUuid());//base_uuid
stmt.setString(2, p.getSysBaseUuid());//sys_base_uuid
stmt.setBoolean(3, p.isRead());//read
stmt.setBoolean(4, p.isCreate());//create
stmt.setBoolean(5, p.isUpdate());//update
stmt.setBoolean(6, p.isDelete());//delete
stmt.setBoolean(7, p.isRelation());//create_relation
stmt.setBoolean(8, p.isShare());//share
stmt.setBoolean(9, p.isGrant());//grant
stmt.setString(10, userUuid);//created_by
int row = stmt.executeUpdate();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public Map getPermissions(Set baseUuids)
throws ServiceException
{
Map pMap = new HashMap(200);
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectPermission(baseUuids));
ResultSet rs = stmt.executeQuery();
while(rs.next())
{
String baseUuid = rs.getString(1);
Permission p = getPermission(rs, baseUuid);
pMap.put(baseUuid, p);
}
rs.close();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
return pMap;
}
public Permission getPermission(String baseUuid)
throws ServiceException
{
Set set = new HashSet();
set.add(baseUuid);
Map pMap = getPermissions(set);
if(pMap == null || pMap.isEmpty())
{
return new NullPermission();
}
else
{
return pMap.get(baseUuid);
}
}
public void saveRelationship(String pBaseUuid, String cBaseUuid)
throws ServiceException
{
saveRelationship(pBaseUuid, cBaseUuid, 0);
}
public void saveRelationship(String pBaseUuid, String cBaseUuid, int sortOrder)
throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.RELATIONSHIP_INSERT_QUERY);
String userUuid = Ctx.getUserUuid();
stmt.setString(1, pBaseUuid);//p_base_uuid
stmt.setString(2, cBaseUuid);//c_base_uuid
stmt.setInt(3, sortOrder); //sort_order
stmt.setString(4, userUuid);//created_by
stmt.executeUpdate();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List findChildrenByType(String baseUuid, ObjectTypeEnum type)
throws ServiceException
{
List uuids = new ArrayList(2000);
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement(QueryBuilder.RELATIONSHIP_SELECT_TYPE_P_QUERY);
stmt.setString(1, baseUuid);
stmt.setString(2, type.toString());
ResultSet rs = stmt.executeQuery();
while(rs.next())
{
String cBaseUuid = rs.getString(2);
uuids.add(cBaseUuid);
}
rs.close();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
List bos = null;
if(uuids.size() > 0)
{
bos = getBOs(uuids);
}
else
{
bos = new ArrayList<>();
}
return bos;
}
public List getParentByType(String baseUuid, ObjectTypeEnum type)
throws ServiceException
{
List list = new ArrayList(200);
Connection con = null;
try
{
con = ConnUtil.getConnection();
QueryBuilder queryBuilder = new QueryBuilder();
PreparedStatement stmt = con.prepareStatement(queryBuilder.selectTypeRelationshipByC());
stmt.setString(1, baseUuid);
stmt.setString(2, type.toString());
ResultSet rs = stmt.executeQuery();
while(rs.next())
{
String cBaseUuid = rs.getString(1);
list.add(cBaseUuid);
}
rs.close();
stmt.close();
}
catch (SQLException e)
{
log.log(Level.SEVERE,e.getMessage(), e);
}
finally
{
ConnUtil.closeConnection(con);
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy