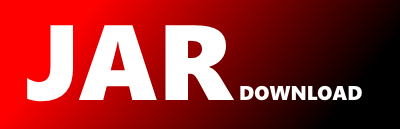
io.baltoro.service.InstanceDAOImpl Maven / Gradle / Ivy
package io.baltoro.service;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import io.baltoro.domain.AppUserSession;
import io.baltoro.domain.BODefaults;
import io.baltoro.domain.BaltoroAppAPI;
import io.baltoro.domain.BaltoroInstance;
import io.baltoro.domain.BaltoroInstanceRequest;
import io.baltoro.domain.StateTypeEnum;
import io.baltoro.exception.ServiceException;
import io.baltoro.to.ReplicationContext;
import io.baltoro.to.ReplicationTO;
import io.baltoro.util.StringUtil;
import io.baltoro.util.UUIDGenerator;
public class InstanceDAOImpl implements InstanceDAO
{
static Log log = LogFactory.getLog(InstanceDAOImpl.class);
InstanceDAOImpl()
{
// TODO Auto-generated constructor stub
}
public BaltoroInstance getInstance(String uuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("select * from baltoro_instance where uuid = ?");
stmt.setString(1, uuid);
ResultSet rs = stmt.executeQuery();
if (rs.next())
{
BaltoroInstance obj = new BaltoroInstance();
obj.setUuid(uuid);
obj.setAppUuid(rs.getString("app_uuid"));
obj.setState(rs.getString("state"));
obj.setHostAddress(rs.getString("host_address"));
obj.setRemoteAddress(rs.getString("remote_address"));
obj.setThreadCount(rs.getInt("thread_count"));
obj.setCpuPercent(rs.getInt("cpu_percent"));
obj.setMemoryGB(rs.getInt("memory_gb"));
obj.setLastHeartBeatOn(rs.getTimestamp("last_hb_on"));
obj.setStartedOn(rs.getTimestamp("started_on"));
obj.setCreatedBy(rs.getString("created_by"));
obj.setCreatedOn(rs.getTimestamp("created_on"));
obj.setClusterPath(rs.getString("cluster_path"));
return obj;
}
else
{
return null;
}
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List find(String appUuid) throws ServiceException
{
Connection con = null;
try
{
List list = new ArrayList<>();
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("select * from baltoro_instance where app_uuid = ? order by cluster_path");
stmt.setString(1, appUuid);
ResultSet rs = stmt.executeQuery();
while(rs.next())
{
BaltoroInstance obj = new BaltoroInstance();
obj.setUuid(rs.getString("uuid"));
obj.setAppUuid(rs.getString("app_uuid"));
obj.setState(rs.getString("state"));
obj.setHostAddress(rs.getString("host_address"));
obj.setRemoteAddress(rs.getString("remote_address"));
obj.setThreadCount(rs.getInt("thread_count"));
obj.setCpuPercent(rs.getInt("cpu_percent"));
obj.setMemoryGB(rs.getInt("memory_gb"));
obj.setLastHeartBeatOn(rs.getTimestamp("last_hb_on"));
obj.setStartedOn(rs.getTimestamp("started_on"));
obj.setCreatedBy(rs.getString("created_by"));
obj.setCreatedOn(rs.getTimestamp("created_on"));
obj.setClusterPath(rs.getString("cluster_path"));
list.add(obj);
}
return list;
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public BaltoroInstance insertInstance(BaltoroInstance obj) throws ServiceException
{
if(StringUtil.isNullOrEmpty(obj.getUuid()))
{
throw new ServiceException("uuid cannot be null");
}
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("insert into "
+ "baltoro_instance(uuid, app_uuid,state, cluster_path, host_address, remote_address, thread_count, created_by, started_on, created_on ) "
+ "values (?,?,?,?,?,?,?,?,?,?)");
obj.setState(StateTypeEnum.LIVE.name());
obj.setStartedOn(new Timestamp(System.currentTimeMillis()));
stmt.setString(1, obj.getUuid());
stmt.setString(2, obj.getAppUuid());
stmt.setString(3, StateTypeEnum.LIVE.name());
stmt.setString(4, obj.getClusterPath());
stmt.setString(5, obj.getHostAddress());
stmt.setString(6, obj.getRemoteAddress());
stmt.setInt(7, obj.getThreadCount());
stmt.setString(8, obj.getCreatedBy());
stmt.setTimestamp(9, obj.getStartedOn());
stmt.setTimestamp(10, obj.getCreatedOn());
int a = stmt.executeUpdate();
return obj;
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateInstanceStartedOn(String uuid, String clusterPath, String remoteAddress, int threads) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update baltoro_instance set started_on=?, cluster_path=?, remote_address=?, thread_count=? where uuid=? ");
stmt.setTimestamp(1, new Timestamp(System.currentTimeMillis()));
stmt.setString(2, clusterPath);
stmt.setString(3, remoteAddress);
stmt.setInt(4, threads);
stmt.setString(5, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateInstance(String uuid, String state, int cpuPercent, int memGB) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update baltoro_instance set state=?, cpu_percent=?, memory_gb=?, last_hb_on=? where uuid=? ");
stmt.setString(1, state);
stmt.setInt(2, cpuPercent);
stmt.setInt(3, memGB);
stmt.setTimestamp(4, new Timestamp(System.currentTimeMillis()));
stmt.setString(5, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void closeDeadInstances() throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update baltoro_instance set state='DOWN' where last_hb_on < ? and state='LIVE' ");
long t0 = System.currentTimeMillis()-45000;
stmt.setTimestamp(1, new Timestamp(t0));
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void deleteInstances(String uuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("delete from baltoro_instance where uuid=? ");
stmt.setString(1, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public BaltoroAppAPI insert(BaltoroAppAPI obj) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("insert into baltoro_app_api"
+ "(uuid, app_uuid,path, state, auth_flag, timeout_sec, discoverable_flag,roles, props_json, created_by, created_on ) "
+ "values (?,?,?,?,?,?,?,?,?,?,?)");
String uuid = UUIDGenerator.uuid("APTH");
int auth = obj.isAuthRequired() ? 1 : 0;
int discoverable = obj.isDiscoverable() ? 1 : 0;
obj.setCreatedOn(new Timestamp(System.currentTimeMillis()));
stmt.setString(1, uuid);
stmt.setString(2, obj.getAppUuid());
stmt.setString(3, obj.getPath());
stmt.setString(4, obj.getState());
stmt.setInt(5, auth);
stmt.setInt(6, obj.getTimeoutSec());
stmt.setInt(7, discoverable);
stmt.setString(8, obj.getRoles());
stmt.setString(9, obj.getPropsJson());
stmt.setString(10, obj.getCreatedBy());
stmt.setTimestamp(11, obj.getCreatedOn());
int a = stmt.executeUpdate();
obj.setAppUuid(uuid);
return obj;
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List getAppAPI(String appUuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("select * from baltoro_app_api where app_uuid = ?");
stmt.setString(1, appUuid);
ResultSet rs = stmt.executeQuery();
List list = new ArrayList<>(200);
while (rs.next())
{
BaltoroAppAPI obj = new BaltoroAppAPI();
obj.setUuid(rs.getString("uuid"));
obj.setAppUuid(appUuid);
obj.setPath(rs.getString("path"));
obj.setAuthRequired(rs.getInt("auth_flag") == 1 ? true : false);
obj.setState(rs.getString("state"));
obj.setRoles(rs.getString("roles"));
obj.setDiscoverable(rs.getInt("discoverable_flag") == 1 ? true : false);
obj.setPropsJson(rs.getString("props_json"));
obj.setCreatedOn(rs.getTimestamp("created_on"));
obj.setCreatedBy(rs.getString("created_by"));
obj.setTimeoutSec(rs.getInt("timeout_sec"));
list.add(obj);
}
return list;
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void update(String uuid, BaltoroAppAPI obj) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update baltoro_app_api set state=?, auth_flag=?, discoverable_flag=?, roles=?, props_json=?, created_by=?, created_on=?, timeout_sec=? where uuid=? ");
stmt.setString(1, obj.getState());
stmt.setInt(2, obj.isAuthRequired() ? 1 : 0);
stmt.setInt(3, obj.isDiscoverable() ? 1 : 0);
stmt.setString(4, obj.getRoles());
stmt.setString(5, obj.getPropsJson());
stmt.setString(6, obj.getCreatedBy());
stmt.setTimestamp(7, obj.getCreatedOn());
stmt.setInt(8, obj.getTimeoutSec());
stmt.setString(9, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public BaltoroInstanceRequest insert(BaltoroInstanceRequest obj) throws ServiceException
{
Connection con = null;
try
{
/*
* `uuid` varchar(42) COLLATE utf8_bin NOT NULL,
`instance_uuid` varchar(42) COLLATE utf8_bin NOT NULL,
`api_uuid` varchar(42) COLLATE utf8_bin NOT NULL,
`add_path` varchar(256) COLLATE utf8_bin DEFAULT NULL,
`started_on` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`millis_taken` int(8) DEFAULT NULL,
`size_kb` int(10) DEFAULT NULL,
`error` varchar(256) COLLATE utf8_bin DEFAULT NULL,
`created_by` varchar(42) COLLATE utf8_bin NOT NULL DEFAULT '',
*/
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("insert into baltoro_instance_request"
+ "(uuid, instance_uuid,api_uuid, add_path, started_on, millis_taken, size_kb, error, created_by ) "
+ "values (?,?,?,?,?,?,?,?,?)");
stmt.setString(1, obj.getUuid());
stmt.setString(2, obj.getInstanceUuid());
stmt.setString(3, obj.getAPIUuid());
stmt.setString(4, obj.getAddPath());
stmt.setTimestamp(5, obj.getStartedOn());
stmt.setInt(6, obj.getMillisTaken());
stmt.setInt(7, obj.getSizeKB());
stmt.setString(8, obj.getError());
stmt.setString(9, obj.getCreatedBy());
int a = stmt.executeUpdate();
return obj;
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateRequest(String uuid, int millis, int sizeKB, String error) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update baltoro_instance_request set millis_taken=?, size_kb=?, error=? where uuid=? ");
stmt.setInt(1, millis);
stmt.setInt(2, sizeKB);
stmt.setString(3, error);
stmt.setString(4, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void createUserSession(AppUserSession obj) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("insert into app_user_session"
+ "(uuid, app_uuid,instance_uuid,user_name, state, timeout_min, created_on, last_activity_on, created_by, att_json ) "
+ "values (?,?,?,?,?,?,?,?,?,?)");
stmt.setString(1, obj.getUuid());
stmt.setString(2, obj.getAppUuid());
stmt.setString(3, obj.getInstanceUuid());
stmt.setString(4, obj.getUserName());
stmt.setString(5, "LIVE");
stmt.setInt(6, obj.getTimeoutMin());
stmt.setTimestamp(7, obj.getCreatedOn());
stmt.setTimestamp(8, obj.getLastActivityOn());
stmt.setString(9, obj.getCreatedBy());
stmt.setString(10, obj.getAttJson());
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void touchAppUserSession(String uuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update app_user_session set last_activity_on=? where uuid=? ");
stmt.setTimestamp(1, new Timestamp(System.currentTimeMillis()));
stmt.setString(2, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void updateAppUserSessionAtt(String uuid, String userName, String json) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update app_user_session set user_name=?, att_json=? where uuid=? ");
stmt.setString(1, userName);
stmt.setString(2, json);
stmt.setString(3, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void closeAppUserSession(String uuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("update app_user_session set state='DEAD' where uuid=? ");
stmt.closeOnCompletion();
stmt.setString(1, uuid);
int a = stmt.executeUpdate();
}
catch (Exception e)
{
log.error(e);
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List getUserSessionByAppUuid(String appUuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("select * from app_user_session where app_uuid = ?");
stmt.setString(1, appUuid);
ResultSet rs = stmt.executeQuery();
List list = new ArrayList<>(200);
while (rs.next())
{
AppUserSession obj = new AppUserSession();
obj.setUuid(rs.getString("uuid"));
obj.setAppUuid(appUuid);
obj.setInstanceUuid(rs.getString("instance_uuid"));
obj.setUserName(rs.getString("user_name"));
obj.setState(rs.getString("state"));
obj.setTimeoutMin(rs.getInt("timeout_min"));
obj.setLastActivityOn(rs.getTimestamp("last_activity_on"));
obj.setCreatedOn(rs.getTimestamp("created_on"));
obj.setCreatedBy(rs.getString("created_by"));
obj.setAttJson(rs.getString("att_json"));
list.add(obj);
}
rs.close();
stmt.close();
return list;
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public AppUserSession getUserSessionByUuid(String uuid) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
PreparedStatement stmt = con.prepareStatement("select * from app_user_session where uuid = ?");
stmt.setString(1, uuid);
ResultSet rs = stmt.executeQuery();
if (rs.next())
{
AppUserSession obj = new AppUserSession();
obj.setUuid(rs.getString("uuid"));
obj.setAppUuid(rs.getString("app_uuid"));
obj.setInstanceUuid(rs.getString("instance_uuid"));
obj.setUserName(rs.getString("user_name"));
obj.setState(rs.getString("state"));
obj.setTimeoutMin(rs.getInt("timeout_min"));
obj.setLastActivityOn(rs.getTimestamp("last_activity_on"));
obj.setCreatedOn(rs.getTimestamp("created_on"));
obj.setCreatedBy(rs.getString("created_by"));
obj.setAttJson(rs.getString("att_json"));
return obj;
}
rs.close();
stmt.close();
return null;
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public void insert(String appUuuid, String instUuid, ReplicationContext ctx) throws ServiceException
{
Connection con = null;
try
{
con = ConnUtil.getConnection();
String query = "insert into baltoro_replication"
+ "(uuid, app_uuid,source_app_uuid,inst_uuid,millis, cmd,created_by ) "
+ "values (?,?,?,?,?,?,?)";
PreparedStatement stmt = con.prepareStatement(query);
String uuid = UUIDGenerator.uuid("REPC");
stmt.setString(1, uuid);
stmt.setString(2, appUuuid);
stmt.setString(3, "");
stmt.setString(4, instUuid);
stmt.setLong(5, System.currentTimeMillis());
stmt.setString(6, ctx.getCmd());
stmt.setString(7, BODefaults.BASE_USER);
int a = stmt.executeUpdate();
stmt.close();
String[] apps = ctx.getApps();
if(apps != null)
{
stmt = con.prepareStatement(query);
for (String srcAppUuid : apps)
{
uuid = UUIDGenerator.uuid("REPC");
stmt.setString(1, uuid);
stmt.setString(2, srcAppUuid);
stmt.setString(3, appUuuid);
stmt.setString(4, instUuid);
stmt.setLong(5, System.currentTimeMillis());
stmt.setString(6, ctx.getCmd());
stmt.setString(7, BODefaults.BASE_USER);
stmt.addBatch();
}
stmt.executeBatch();
stmt.close();
}
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
public List getReplication(ReplicationTO to) throws ServiceException
{
Connection con = null;
try
{
PreparedStatement stmt = null;
ResultSet rs = null;
con = ConnUtil.getConnection();
if(!to.reset)
{
stmt = con.prepareStatement("select count(1) from baltoro_replication where app_uuid = ? and inst_uuid != ? and millis > ?");
stmt.setString(1, to.appUuid);
stmt.setString(2, to.instUuid);
stmt.setLong(3, to.lcpMillis);
rs = stmt.executeQuery();
int totalCount = -1;
if(rs.next())
{
totalCount = rs.getInt(1);
to.totalCount = totalCount;
}
rs.close();
stmt.close();
if(totalCount == 0)
{
return null;
}
stmt = con.prepareStatement("select * from baltoro_replication where app_uuid = ? and inst_uuid != ? and millis > ? order by millis limit 1000");
stmt.setString(1, to.appUuid);
stmt.setString(2, to.instUuid);
stmt.setLong(3, to.lcpMillis);
}
else
{
stmt = con.prepareStatement("select count(1) from baltoro_replication where app_uuid = ? and millis > ?");
stmt.setString(1, to.appUuid);
stmt.setLong(2, to.lcpMillis);
rs = stmt.executeQuery();
int totalCount = -1;
if(rs.next())
{
totalCount = rs.getInt(1);
to.totalCount = totalCount;
}
rs.close();
stmt.close();
if(totalCount == 0)
{
return null;
}
stmt = con.prepareStatement("select * from baltoro_replication where app_uuid = ? and millis > ? order by millis limit 1000");
stmt.setString(1, to.appUuid);
stmt.setLong(2, to.lcpMillis);
}
rs = stmt.executeQuery();
List list = new ArrayList<>(2000);
while (rs.next())
{
ReplicationContext ctx = new ReplicationContext();
ctx.setUuid(rs.getString("uuid"));
ctx.setMillis(rs.getLong("millis"));
ctx.setCmd(rs.getString("cmd"));
ctx.setSourceAppUuid(rs.getString("source_app_uuid"));
list.add(ctx);
}
rs.close();
stmt.close();
if(list.size() >999)
{
to.moreAvailable = true;
}
return list;
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
ConnUtil.closeConnection(con);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy