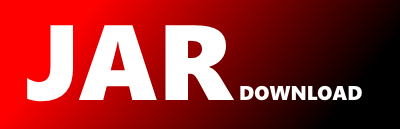
io.baltoro.service.InstanceServiceImpl Maven / Gradle / Ivy
package io.baltoro.service;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import io.baltoro.domain.AppUserSession;
import io.baltoro.domain.BaltoroAppAPI;
import io.baltoro.domain.BaltoroInstance;
import io.baltoro.domain.BaltoroInstanceRequest;
import io.baltoro.domain.StateTypeEnum;
import io.baltoro.exception.ServiceException;
import io.baltoro.to.PathTO;
import io.baltoro.to.ReplicationContext;
import io.baltoro.to.ReplicationTO;
public class InstanceServiceImpl implements InstanceService
{
static Log log = LogFactory.getLog(InstanceServiceImpl.class);
public BaltoroInstance get(String uuid) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
BaltoroInstance bi = dao.getInstance(uuid);
return bi;
}
public List find(String appUuid)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
List list = null;
try
{
list = dao.find(appUuid);
}
catch (ServiceException e)
{
e.printStackTrace();
}
return list;
}
public BaltoroInstance insert(BaltoroInstance obj) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
BaltoroInstance bi = dao.insertInstance(obj);
return bi;
}
public BaltoroInstance update(String uuid, String state, int cpuPercent, int memGB) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
dao.updateInstance(uuid, state, cpuPercent, memGB);
BaltoroInstance bi = dao.getInstance(uuid);
return bi;
}
public void updateInstanceStartedOn(String uuid, String clusterPath, String remoteAddress, int threads) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
dao.updateInstanceStartedOn(uuid, clusterPath, remoteAddress, threads);
}
public void closeDeadInstances()
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.closeDeadInstances();
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public void deleteInstance(String uuid)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.deleteInstances(uuid);
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public List getAppAPI(String appUuid)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
List currentPathList = null;
try
{
currentPathList = dao.getAppAPI(appUuid);
}
catch (ServiceException e)
{
e.printStackTrace();
}
return currentPathList;
}
public List registerAppAPI(String instanceUuid, String appUuid, List paths) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
List currentPathList = dao.getAppAPI(appUuid);
List resolvedList = dao.getAppAPI(appUuid);
Map oldPathMap = new HashMap<>(200);
for (BaltoroAppAPI baltoroAppPath : currentPathList)
{
oldPathMap.put(baltoroAppPath.getPath(), baltoroAppPath);
}
Set newPathSet = new HashSet<>(200);
for (PathTO to : paths)
{
newPathSet.add(to.path);
BaltoroAppAPI oldPath = oldPathMap.get(to.path);
if(oldPath != null)
{
boolean changed = false;
if(oldPath.isAuthRequired() != to.authRequired)
{
oldPath.setAuthRequired(to.authRequired);
changed = true;
}
if(oldPath.isDiscoverable() != to.discoverable)
{
oldPath.setDiscoverable(to.discoverable);
changed = true;
}
if(oldPath.getPropsJson() != to.propsJson)
{
oldPath.setPropsJson(to.propsJson);
changed = true;
}
if(oldPath.getTimeoutSec() != to.timeoutSec)
{
oldPath.setTimeoutSec(to.timeoutSec);
changed = true;
}
if((to.roles != null || oldPath.getRoles()!=null) && !oldPath.getRoles().equals(to.roles))
{
oldPath.setRoles(to.roles);
changed = true;
}
if(!oldPath.getState().equals("LIVE"))
{
oldPath.setState(StateTypeEnum.LIVE.name());
changed = true;
}
if(!changed)
{
oldPathMap.remove(to.path);
}
}
else
{
BaltoroAppAPI obj = new BaltoroAppAPI();
obj.setAppUuid(appUuid);
obj.setPath(to.path);
obj.setState(StateTypeEnum.LIVE.toString());
obj.setAuthRequired(to.authRequired);
obj.setRoles(to.roles);
obj.setCreatedBy(instanceUuid);
obj.setDiscoverable(to.discoverable);
obj.setPropsJson(to.propsJson);
obj = dao.insert(obj);
resolvedList.add(obj);
}
}
for (BaltoroAppAPI oldPtah : oldPathMap.values())
{
if(!newPathSet.contains(oldPtah.getPath()))
{
oldPtah.setState("DEAD");
}
dao.update(oldPtah.getUuid(), oldPtah);
resolvedList.add(oldPtah);
}
return resolvedList;
}
public BaltoroInstanceRequest insert(BaltoroInstanceRequest obj) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
obj = dao.insert(obj);
return obj;
}
public void updateRequestTime(String uuid, int millis, int sizeKB, String error) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
dao.updateRequest(uuid, millis, sizeKB, error);
}
public void createUserSession(AppUserSession session)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.createUserSession(session);
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public void touchAppUserSession(String uuid) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
dao.touchAppUserSession(uuid);
}
public void updateAppUserSessionAtt(String uuid, String userName, String json)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.updateAppUserSessionAtt(uuid, userName, json);
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public void closeAppUserSession(String uuid)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.closeAppUserSession(uuid);
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public AppUserSession getUserSessionByUuid(String uuid)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
return dao.getUserSessionByUuid(uuid);
}
catch (ServiceException e)
{
e.printStackTrace();
}
return null;
}
public List getUserSessionByAppUuid(String appUuid) throws ServiceException
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
return dao.getUserSessionByAppUuid(appUuid);
}
public void insert(String appUuuid, String instUuid, ReplicationContext ctx)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
try
{
dao.insert(appUuuid, instUuid, ctx);
}
catch (ServiceException e)
{
e.printStackTrace();
}
}
public List getReplication(ReplicationTO to)
{
InstanceDAO dao = DaoFactory.get(InstanceDAO.class);
List list = null;
try
{
list = dao.getReplication(to);
}
catch (ServiceException e)
{
e.printStackTrace();
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy