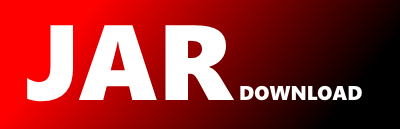
io.baltoro.util.StreamUtil Maven / Gradle / Ivy
package io.baltoro.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.io.ObjectOutputStream;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
import io.baltoro.exception.ServiceException;
public class StreamUtil
{
public static byte[] toBytes(InputStream in) throws ServiceException
{
BufferedInputStream bis = new BufferedInputStream(in);
ByteArrayOutputStream out = new ByteArrayOutputStream();
BufferedOutputStream bos = new BufferedOutputStream(out);
try
{
while(bis.available()>0)
{
byte[] data = new byte[1024 * 10];
int bytesRead=0;
while( (bytesRead = bis.read(data)) != -1)
{
bos.write(bytesRead);
}
}
}
catch (Exception e)
{
throw new ServiceException(e);
}
finally
{
try
{
bis.close();
bos.close();
out.close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
return out.toByteArray();
}
public static byte[] toBytes(Object obj) throws ServiceException
{
try
{
ByteArrayOutputStream out = new ByteArrayOutputStream();
ObjectOutputStream os = new ObjectOutputStream(out);
os.writeObject(obj);
return out.toByteArray();
}
catch (Exception e)
{
throw new ServiceException(e);
}
}
public static byte[] toBytes(int i)
{
byte[] result = new byte[4];
result[0] = (byte) (i >> 24);
result[1] = (byte) (i >> 16);
result[2] = (byte) (i >> 8);
result[3] = (byte) (i /*>> 0*/);
return result;
}
public static byte[] compress(byte[] data)
{
try
{
ByteArrayOutputStream bos = new ByteArrayOutputStream(data.length);
GZIPOutputStream gzip = new GZIPOutputStream(bos);
gzip.write(data);
gzip.close();
byte[] compressed = bos.toByteArray();
bos.close();
return compressed;
}
catch (Exception e)
{
e.printStackTrace();
throw new RuntimeException(e);
}
}
public static byte[] decompress(byte[] compressed)
{
try
{
ByteArrayInputStream bis = new ByteArrayInputStream(compressed);
GZIPInputStream gis = new GZIPInputStream(bis);
ByteArrayOutputStream out = new ByteArrayOutputStream();
BufferedOutputStream bos = new BufferedOutputStream(out);
while(gis.available()>0)
{
byte[] data = new byte[1024 * 10];
int bytesRead=0;
while( (bytesRead = gis.read(data)) != -1)
{
bos.write(bytesRead);
}
}
bos.close();
out.close();
gis.close();
bis.close();
return out.toByteArray();
}
catch (Exception e)
{
e.printStackTrace();
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy