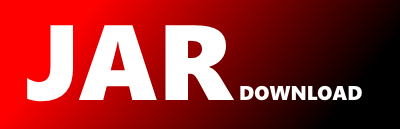
io.baltoro.util.StringUtil Maven / Gradle / Ivy
package io.baltoro.util;
import java.util.Base64;
import java.util.Collection;
import io.baltoro.exception.ServiceException;
public class StringUtil
{
public static boolean isNullOrEmpty(String str)
{
if(str != null && str.length() > 0)
{
return false;
}
return true;
}
public static boolean isNullOrEmpty(Collection> col)
{
if(col != null && col.size() > 0)
{
return false;
}
return true;
}
public static boolean isNotNullAndNotEmpty(String str)
{
if(str != null && str.length() > 0)
{
return true;
}
return false;
}
public static String stripPhoneNumber(String phoneNumber)
{
StringBuffer str = new StringBuffer();
char[] chars = phoneNumber.toCharArray();
for (int i = 0; i < chars.length; i++)
{
char c = chars[i];
if(c >= '0' && c <= '9')
{
str.append(chars[i]);
}
}
return str.toString();
}
public static String getDomainFromEmail(String email)
throws ServiceException
{
int i = email.indexOf('@');
if(i == -1)
{
throw new ServiceException("not a valid email");
}
String domain = email.substring(i);
return domain;
}
public static String encode(byte[] bytes)
{
return Base64.getEncoder().encodeToString(bytes);
}
public static byte[] decode(String str)
{
return Base64.getDecoder().decode(str);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy