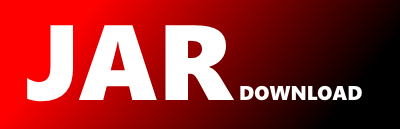
com.caucho.v5.amp.stream.StreamBuilderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of baratine Show documentation
Show all versions of baratine Show documentation
A reactive Java web server.
/*
* Copyright (c) 1998-2015 Caucho Technology -- all rights reserved
*
* This file is part of Baratine(TM)
*
* Each copy or derived work must preserve the copyright notice and this
* notice unmodified.
*
* Baratine is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* Baratine is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE, or any warranty
* of NON-INFRINGEMENT. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License
* along with Baratine; if not, write to the
*
* Free Software Foundation, Inc.
* 59 Temple Place, Suite 330
* Boston, MA 02111-1307 USA
*
* @author Scott Ferguson
*/
package com.caucho.v5.amp.stream;
import io.baratine.function.BiConsumerAsync;
import io.baratine.function.BiConsumerSync;
import io.baratine.function.BiFunctionAsync;
import io.baratine.function.BiFunctionSync;
import io.baratine.function.BinaryOperatorAsync;
import io.baratine.function.BinaryOperatorSync;
import io.baratine.function.ConsumerAsync;
import io.baratine.function.ConsumerSync;
import io.baratine.function.FunctionAsync;
import io.baratine.function.FunctionSync;
import io.baratine.function.PredicateAsync;
import io.baratine.function.PredicateSync;
import io.baratine.function.SupplierSync;
import io.baratine.service.Cancel;
import io.baratine.service.Result;
import io.baratine.service.ServiceExceptionCancelled;
import io.baratine.service.ServiceRef;
import io.baratine.stream.ResultStream;
import io.baratine.stream.ResultStreamBuilderSync;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Objects;
import java.util.concurrent.TimeUnit;
import java.util.stream.Stream;
import com.caucho.v5.amp.ServiceRefAmp;
import com.caucho.v5.amp.message.HeadersNull;
import com.caucho.v5.amp.message.StreamCallMessage;
import com.caucho.v5.amp.spi.HeadersAmp;
import com.caucho.v5.amp.spi.OutboxAmp;
import com.caucho.v5.amp.stub.MethodAmp;
@SuppressWarnings("serial")
public class StreamBuilderImpl implements ResultStreamBuilderSync, Serializable
{
private transient ServiceRefAmp _serviceRef;
private transient MethodAmp _method;
private Object[] _args;
// private SupplierSync> _init;
// private StreamBuilderFilter _filter;
// private Object _value;
public StreamBuilderImpl(ServiceRefAmp serviceRef,
MethodAmp method,
Object []args)
{
Objects.requireNonNull(serviceRef);
Objects.requireNonNull(method);
_serviceRef = serviceRef;
_method = method;
_args = args;
// this(serviceRef, method, args, StreamBuilderFilter.identity());
}
/*
private StreamBuilderImpl(ServiceRefAmp serviceRef,
MethodAmp method,
Object []args,
StreamBuilderFilter filter)
{
Objects.requireNonNull(serviceRef);
Objects.requireNonNull(method);
Objects.requireNonNull(filter);
_serviceRef = serviceRef;
_method = method;
_args = args;
_filter = filter;
}
*/
private StreamBuilderImpl(StreamBuilderImpl,U> next)
{
Objects.requireNonNull(next);
_serviceRef = next._serviceRef;
_method = next._method;
_args = next._args;
// _filter = StreamBuilderFilter.identity();
}
//
// builder
//
protected ResultStream build(ResultStream super T> next)
{
return (ResultStream) next;
}
//
// terminals
//
@Override
public Cancel exec()
{
ResultStreamExecute resultStream = new ResultStreamExecute<>(Result.ignore());
offer(resultStream);
return resultStream;
}
@Override
public void result(Result super T> result)
{
ResultStream resultStream = new ResultStreamExecute(result);
offer(resultStream);
// return resultStream;
}
@Override
public void to(ResultStream super T> result)
{
Objects.requireNonNull(result);
local().offer(result);
}
@Override
public T result()
{
/*
ResultFuture future = new ResultFuture<>();
return _serviceRef.getManager().run(future, 60, TimeUnit.SECONDS,
()->result(future));
*/
//System.err.println("CURR: " + OutboxAmp.getCurrent());
return _serviceRef.manager().run(60, TimeUnit.SECONDS,
r->result(r));
}
@Override
public StreamBuilderImpl forEach(ConsumerSync super T> consumer)
{
return new ForEachSync<>(this, consumer);
}
@Override
public StreamBuilderImpl forEach(ConsumerAsync super T> consumer)
{
return new ForEachAsync<>(this, consumer);
}
@Override
public StreamBuilderImpl first()
{
return new First(this);
}
@Override
public StreamBuilderImpl ignore()
{
return new Ignore(this);
}
//
// reduce
//
/**
* Reduce with a binary function
*
*
* s = init;
* s = op(s, t1);
* s = op(s, t2);
* result = s;
*
*/
@Override
public StreamBuilderImpl reduce(T init,
BinaryOperatorSync op)
{
return new ReduceOpInitSync<>(this, init, op);
}
/**
* reduce((x,y)->op) with a sync binary function
*
*
* s = t1;
* s = op(s, t2);
* s = op(s, t3);
* result = s;
*
*/
@Override
public StreamBuilderImpl reduce(BinaryOperatorSync op)
{
return new ReduceOpSync<>(this, op);
}
/**
* Reduce with an accumulator and combiner
*/
@Override
public StreamBuilderImpl
reduce(R identity,
BiFunctionSync accumulator,
BinaryOperatorSync combiner)
{
return new ReduceAccumSync<>(this, identity, accumulator, combiner);
}
/**
* Async Reduce with combiner
*/
/*
@Override
public StreamBuilderImpl
reduce(R identity,
BiFunctionAsync accumulator,
BinaryOperatorAsync combiner)
{
return new ReduceAccumAsync<>(this, identity, accumulator, combiner);
}
*/
@Override
public StreamBuilderImpl
collect(SupplierSync init,
BiConsumerSync accum,
BiConsumerSync combiner)
{
return new CollectSync<>(this, init, accum, combiner);
}
/*
@Override
public StreamBuilderImpl
collect(SupplierSync init,
BiConsumerAsync accum,
BiConsumerAsync combiner)
{
return new CollectAsync<>(this, init, accum, combiner);
}
*/
//
// map
//
@Override
public StreamBuilderImpl map(FunctionSync super T,? extends R> map)
{
return new MapSync(this, map);
}
@Override
public StreamBuilderImpl map(FunctionAsync super T,? extends R> map)
{
return new MapAsync(this, map);
}
/**
* chain(s->builder) - builds a ResultStream chain with custom builder
*/
@Override
public StreamBuilderImpl
custom(FunctionSync,ResultStream> builder)
{
return new Chain(this, builder);
}
/**
* peek(consumer) - accepts a copy of the item and passes it on.
*/
@Override
public StreamBuilderImpl peek(ConsumerSync super T> consumer)
{
return new Peek(this, consumer);
}
/**
* local() - switch to local (caller) methods from service (remote) methods.
*/
@Override
public StreamBuilderImpl local()
{
return new Local(this);
}
/**
* prefetch() - limit the flow prefetch credit
*/
// @Override
public StreamBuilderImpl prefetch(int prefetch)
{
return new Prefetch(this, prefetch);
}
//
// filter
//
/**
* filter() sync
*/
@Override
public StreamBuilderImpl filter(PredicateSync super T> test)
{
return new FilterSync(this, test);
}
/**
* filter() async
*/
@Override
public StreamBuilderImpl filter(PredicateAsync super T> test)
{
return new FilterAsync(this, test);
}
//
// blocking versions
//
/**
* reduce(init, (x,y)->op) sync
*/
/*
@Override
public T reduce(T init,
BinaryOperatorSync op)
{
ResultFuture future = new ResultFuture<>();
return doFuture(future,
()->reduce(init, op, future));
}
*/
/*
@Override
public R collect(SupplierSync init,
BiConsumerSync accum,
BiConsumerSync combiner)
{
ResultFuture future = new ResultFuture<>();
return doFuture(future,
()->collect(init, accum, combiner, future));
}
*/
@Override
public StreamBuilderImpl,U> iter()
{
return new Iter<>(this);
/*
ResultFuture> future = new ResultFuture<>();
ResultStreamIter resultIter = new ResultStreamIter<>(future);
return doFuture(future, ()->offer(resultIter));
*/
}
@Override
public StreamBuilderImpl,U> stream()
{
return new StreamFactory<>(this);
/*
ResultFuture> future = new ResultFuture<>();
ResultStreamIter resultIter = new ResultStreamIter<>(future);
return doFuture(future, ()->offer(resultIter));
*/
}
/*
@Override
public V reduce(V identity,
BiFunctionSync accumulator,
BinaryOperatorSync combiner)
{
ResultFuture future = new ResultFuture<>();
return doFuture(future,
()->reduce(identity, accumulator, combiner, future));
}
*/
/*
private R doFuture(ResultFuture future, Runnable task)
{
return _serviceRef.getManager().run(future, 60, TimeUnit.SECONDS, task);
}
*/
/*
private R doFuture(Consumer> task)
{
return _serviceRef.getManager().run(60, TimeUnit.SECONDS, task);
}
*/
private void offer(ResultStream super T> resultStream)
{
long expires = 600 * 1000L;
try (OutboxAmp outbox = OutboxAmp.currentOrCreate(_serviceRef.manager())) {
HeadersAmp headers = HeadersNull.NULL;
ResultStream stream = build(resultStream);
StreamCallMessage msg;
msg = new StreamCallMessage(outbox, outbox.inbox(), headers,
_serviceRef, _method,
stream,
expires, _args);
long timeout = 10000;
msg.offer(timeout);
}
}
@Override
public String toString()
{
return getClass().getSimpleName() + "[" + _method + "]";
}
//
// inner classes
//
abstract private static class Next extends StreamBuilderImpl {
private StreamBuilderImpl _next;
Next(StreamBuilderImpl next)
{
super(next);
Objects.requireNonNull(next);
_next = next;
}
protected StreamBuilderImpl getNext()
{
return _next;
}
}
//
// chain
//
/**
* chain() builder
*/
private static class Chain extends Next {
private FunctionSync,ResultStream> _builder;
Chain(StreamBuilderImpl next,
FunctionSync,ResultStream> builder)
{
super(next);
Objects.requireNonNull(builder);
_builder = builder;
}
@Override
public ResultStream build(ResultStream super S> result)
{
ResultStream chainResult = new ResultStreamChain<>(result, _builder);
return getNext().build(chainResult);
}
}
/**
* chain() ResultStream
*/
private static class ResultStreamChain extends ResultStream.Wrapper
{
private ResultStream super S> _prev;
private FunctionSync,ResultStream> _builder;
ResultStreamChain(ResultStream super S> prev,
FunctionSync,ResultStream> builder)
{
super(builder.apply((ResultStream) prev));
_prev = prev;
_builder = builder;
}
@Override
public void accept(T value)
{
next().accept(value);
}
@Override
public ResultStream> createJoin()
{
ResultStream> reduce = _prev.createJoin();
return reduce;
}
@Override
public ResultStream createFork(ResultStream