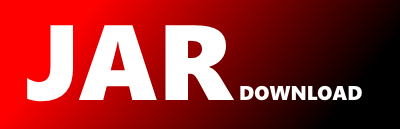
com.caucho.v5.h3.ser.SerializerFactoryH3Impl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of baratine Show documentation
Show all versions of baratine Show documentation
A reactive Java web server.
/*
* Copyright (c) 2001-2016 Caucho Technology, Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* @author Scott Ferguson
*/
package com.caucho.v5.h3.ser;
import java.lang.reflect.Modifier;
import java.lang.reflect.Type;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import com.caucho.v5.h3.SerializerH3;
import com.caucho.v5.h3.context.ContextH3;
import com.caucho.v5.h3.io.ConstH3;
/**
* H3 typed serializer.
*/
public class SerializerFactoryH3Impl implements SerializerFactoryH3
{
private static final SerializerH3Amp> MAP;
private static final SerializerH3Amp> LIST;
private static final HashMap,SerializerH3Amp>> _serMap
= new HashMap<>();
private static final ArrayList> _serArray
= new ArrayList<>();
@Override
public void initSerializers(ArrayList> serArray)
{
serArray.clear();
serArray.addAll(_serArray);
}
@Override
public SerializerH3Amp serializer(Class type, ContextH3 context)
{
SerializerH3Amp> ser = _serMap.get(type);
if (ser != null) {
return (SerializerH3Amp) ser;
}
else if (Map.class.isAssignableFrom(type)) {
return (SerializerH3Amp) MAP;
}
else if (Enum.class.isAssignableFrom(type)) {
return new SerializerH3Enum(type);
}
else if (type.isArray()) {
throw new UnsupportedOperationException(String.valueOf(type));
}
else if (Modifier.isAbstract(type.getModifiers())) {
throw new UnsupportedOperationException(String.valueOf(type));
}
else {
return new SerializerH3Java<>(type, context);
}
}
@Override
public SerializerH3 get(Type type)
{
throw new UnsupportedOperationException(String.valueOf(type));
}
private static void ser(Class> type, SerializerH3Amp> ser)
{
_serMap.put(type, ser);
while (_serArray.size() <= ser.typeSequence()) {
_serArray.add(null);
}
_serArray.set(ser.typeSequence(), ser);
}
static {
MAP = new SerializerH3MapPredef(HashMap.class, ConstH3.DEF_MAP);
LIST = new SerializerH3ListPredef(ArrayList.class, ConstH3.DEF_LIST);
ser(Boolean.class, new SerializerH3Boolean());
ser(String.class, new SerializerH3String());
ser(Byte.class, new SerializerH3Byte());
ser(Character.class, new SerializerH3Character());
ser(Short.class, new SerializerH3Short());
ser(Integer.class, new SerializerH3Integer());
ser(Long.class, new SerializerH3Long());
ser(Float.class, new SerializerH3Float());
ser(Double.class, new SerializerH3Double());
ser(HashMap.class, MAP);
ser(ArrayList.class, LIST);
ser(byte[].class, new SerializerH3ArrayByte());
ser(char[].class, new SerializerH3ArrayChar());
ser(Object[].class, new SerializerH3ArrayObject());
ser(String[].class, new SerializerH3ArrayString());
//time
ser(Instant.class, new SerializerH3Instant());
ser(LocalDate.class, new SerializerH3LocalDate());
ser(LocalTime.class, new SerializerH3LocalTime());
ser(LocalDateTime.class, new SerializerH3LocalDateTime());
ser(ZonedDateTime.class, new SerializerH3ZonedDateTime());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy