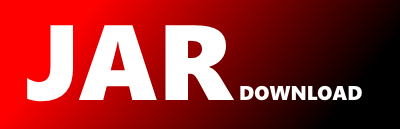
com.caucho.v5.http.protocol2.ConnectionHttp2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of baratine Show documentation
Show all versions of baratine Show documentation
A reactive Java web server.
/*
* Copyright (c) 1998-2015 Caucho Technology -- all rights reserved
*
* This file is part of Baratine(TM)
*
* Each copy or derived work must preserve the copyright notice and this
* notice unmodified.
*
* Baratine is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* Baratine is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE, or any warranty
* of NON-INFRINGEMENT. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License
* along with Baratine; if not, write to the
*
* Free Software Foundation, Inc.
* 59 Temple Place, Suite 330
* Boston, MA 02111-1307 USA
*
* @author Scott Ferguson
*/
package com.caucho.v5.http.protocol2;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicInteger;
import com.caucho.v5.io.ReadBuffer;
import com.caucho.v5.io.WriteBuffer;
/**
* InputStreamHttp reads a single HTTP frame.
*/
public class ConnectionHttp2
{
private final InHttp _inHttp;
private final OutHttp _outHttp;
private final PeerHttp _peer;
private ChannelFlowHttp2 _channel0;
private ConcurrentHashMap _channelMap
= new ConcurrentHashMap<>();
private AtomicInteger _channelCount = new AtomicInteger();
private InHttpHandler _inHandler;
public ConnectionHttp2(InHttpHandler handler, PeerHttp peer)
{
_inHandler = handler;
_inHttp = new InHttp(this, handler);
_outHttp = new OutHttp(this, peer);
_channel0 = new ChannelFlowHttp2();
_peer = peer;
}
/**
* Constructor for testing.
*/
public ConnectionHttp2(PeerHttp peer)
{
this(new DummyHandler(), peer);
}
public PeerHttp getPeer()
{
return _peer;
}
public InHttp getInHttp()
{
return _inHttp;
}
public OutHttp getOutHttp()
{
return _outHttp;
}
public void init(ReadBuffer is, WriteBuffer os)
{
getOutHttp().init(os);
getInHttp().init(is);
_channel0.init();
_channelCount.set(1);
}
public void init(ReadBuffer is)
{
getInHttp().init(is);
_channel0.init();
_channelCount.set(1);
}
public void init(WriteBuffer os)
{
getOutHttp().init(os);
_channel0.init();
_channelCount.set(1);
}
public ChannelFlowHttp2 getChannelZero()
{
return _channel0;
}
public ChannelHttp2 getChannel(int streamId)
{
return _channelMap.get(streamId);
}
public void putChannel(int streamId, ChannelHttp2 channel)
{
if (_channelCount.get() == 0) {
throw new IllegalStateException();
}
_channelMap.put(streamId, channel);
if (_channelCount.get() == 0) {
throw new IllegalStateException();
}
_channelCount.incrementAndGet();
}
public void onStreamOutClose()
{
InHttp inHttp = _inHttp;
if (inHttp != null) {
if (inHttp.onCloseStream() <= 0) {
_outHttp.close();
}
}
}
void onReadGoAway()
{
_channelCount.decrementAndGet();
if (isClosed()) {
_outHttp.close();
//_inHandler.onGoAway();
}
}
void onWriteGoAway()
{
_inHandler.onGoAway();
}
void closeStream(int streamId)
{
_channelMap.remove(streamId);
_channelCount.decrementAndGet();
if (isClosed()) {
_outHttp.close();
//_inHandler.onGoAway();
}
}
public boolean isClosed()
{
return _channelCount.get() == 0;
}
/**
* Called when the socket read is complete.
*/
public void closeRead()
{
}
public void closeWrite()
{
}
/**
* Dummy handler for QA.
*/
private static class DummyHandler implements InHttpHandler {
@Override
public InRequest newRequest()
{
return null;
}
@Override
public void onGoAway()
{
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy