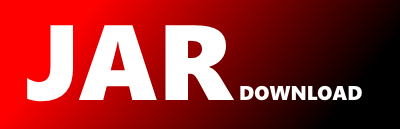
io.bdeploy.common.TaskSynchronizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Public API including dependencies, ready to be used for integrations and plugins.
package io.bdeploy.common;
import java.util.Map;
import java.util.TreeMap;
import java.util.concurrent.Callable;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Performs tasks. When *another* Thread wants to perform the *same* operation, it instead waits for the first Thread to complete
* the task.
*/
public class TaskSynchronizer {
private static final Logger log = LoggerFactory.getLogger(TaskSynchronizer.class);
@SuppressWarnings("unchecked")
private final Map, Future>> tasks = new TreeMap<>((a, b) -> {
if (a == null && b == null) {
return 0;
}
if (a == null || b == null) {
return a == null ? 1 : -1;
}
String clsAName = a.getClass().getName();
String clsBName = b.getClass().getName();
int x = clsAName.compareTo(clsBName);
if (x != 0) {
return x;
}
// only if SAME classes.
return ((Comparable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy