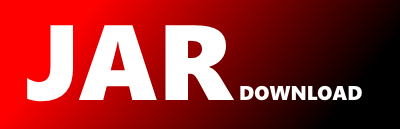
io.bdrc.auth.UserProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bdrc-auth-lib Show documentation
Show all versions of bdrc-auth-lib Show documentation
BDRC authorization library based on auth0
package io.bdrc.auth;
import java.util.ArrayList;
import com.auth0.jwt.interfaces.Claim;
import com.auth0.jwt.interfaces.DecodedJWT;
import io.bdrc.auth.model.User;
import io.bdrc.auth.rdf.RdfAuthModel;
/*******************************************************************************
* Copyright (c) 2018 Buddhist Digital Resource Center (BDRC)
*
* If this file is a derivation of another work the license header will appear
* below; otherwise, this work is licensed under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with the
* License.
*
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
public class UserProfile {
ArrayList groups;
ArrayList roles;
ArrayList permissions;
String name;
User user;
public UserProfile(final DecodedJWT decodedJwt) {
final String id = getId(decodedJwt);
user = RdfAuthModel.getUser(id);
if (user != null) {
this.groups = RdfAuthModel.getUser(id).getGroups();
this.roles = RdfAuthModel.getUser(id).getRoles();
this.permissions = RdfAuthModel.getPermissions(roles, groups);
this.name = user.getName();
} else {
this.user = new User();
this.groups = new ArrayList<>();
this.roles = new ArrayList<>();
this.permissions = new ArrayList<>();
// by default email and name are the same
// auth0 requires email for registration, both in dashboard and on register page
this.user.setName(getName(decodedJwt));
this.user.setEmail(getName(decodedJwt));
this.user.setUserId(getId(decodedJwt));
this.user.setAuthId(getAuth0Id(decodedJwt));
}
}
public UserProfile() {
this.groups = new ArrayList<>();
this.roles = new ArrayList<>();
this.permissions = new ArrayList<>();
this.name = "";
this.user = new User();
}
public ArrayList getGroups() {
return groups;
}
public ArrayList getRoles() {
return roles;
}
String getName(final DecodedJWT decodedJwt) {
final Claim claim = decodedJwt.getClaims().get("name");
if (claim != null) {
return claim.asString();
}
return null;
}
String getId(final DecodedJWT decodedJwt) {
final Claim claim = decodedJwt.getClaims().get("sub");
if (claim != null) {
final String id = claim.asString();
if (!id.endsWith("@clients")) {
return id.substring(id.indexOf("|") + 1);
}
}
return "";
}
String getAuth0Id(final DecodedJWT decodedJwt) {
final Claim claim = decodedJwt.getClaims().get("sub");
if (claim != null) {
final String id = claim.asString();
if (!id.endsWith("@clients")) {
return id;
}
}
return "";
}
public ArrayList getPermissions() {
return permissions;
}
public boolean isInGroup(String group) {
return groups.contains(group);
}
public boolean hasRole(String role) {
return roles.contains(role);
}
public boolean hasPermission(String permission) {
return permissions.contains(permission);
}
public User getUser() {
return user;
}
@Override
public String toString() {
return "User [groups=" + groups + ", roles=" + roles + ", permissions=" + permissions + ", name=" + name + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy