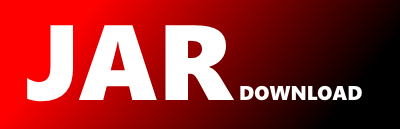
io.bdrc.auth.model.BudaUserInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bdrc-auth-lib Show documentation
Show all versions of bdrc-auth-lib Show documentation
BDRC authorization library based on auth0
package io.bdrc.auth.model;
import java.util.HashMap;
import org.apache.jena.rdf.model.Model;
import org.apache.jena.rdf.model.ModelFactory;
import org.apache.jena.rdf.model.Property;
import org.apache.jena.rdf.model.ResIterator;
import org.apache.jena.rdf.model.Resource;
import org.apache.jena.rdf.model.ResourceFactory;
import org.apache.jena.rdfconnection.RDFConnection;
import org.apache.jena.rdfconnection.RDFConnectionRemote;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import io.bdrc.auth.AuthProps;
public class BudaUserInfo {
public final static String SKOS_PREF_LABEL = "http://www.w3.org/2004/02/skos/core#prefLabel";
public static final String BDOU_PFX = "http://purl.bdrc.io/ontology/ext/user/";
private static HashMap budaUserByAuth0Id;
public final static Logger log = LoggerFactory.getLogger(BudaUserInfo.class.getName());
private static Model loadModel() {
String fusekiUrl = AuthProps.getProperty("fusekiAuthData");
Model infos = ModelFactory.createDefaultModel();
String query = "construct { " + "?s <" + BDOU_PFX + "hasUserProfile> ?pr. " + "?s <" + SKOS_PREF_LABEL + "> ?label. } " + "where { " + "{ "
+ "?s ?p ?o. ?s a <" + BDOU_PFX + "User>. " + "?s <" + BDOU_PFX + "hasUserProfile> ?pr. " + "?s <" + SKOS_PREF_LABEL + "> ?label. "
+ "}" + "}";
RDFConnection conn = RDFConnectionRemote.create().destination(fusekiUrl).build();
infos = conn.queryConstruct(query);
conn.close();
return infos;
}
public static HashMap getBudaRdfUsers() {
if (budaUserByAuth0Id == null) {
Model m = loadModel();
budaUserByAuth0Id = new HashMap<>();
ResIterator it = m.listSubjects();
Property authId = ResourceFactory.createProperty(BDOU_PFX + "hasUserProfile");
Property lab = ResourceFactory.createProperty(SKOS_PREF_LABEL);
while (it.hasNext()) {
Resource rs = it.next();
String auth0Id = rs.getPropertyResourceValue(authId).getURI();
String key = auth0Id.substring(auth0Id.lastIndexOf("/") + 1);
String label=rs.getProperty(lab).getObject().toString();
if(label.indexOf("/")>0) {
label=label.substring(label.lastIndexOf("/")+1);
}else {
label = rs.getProperty(lab).getObject().asLiteral().getString();
}
budaUserByAuth0Id.put(key, new BudaRdfUser(rs.getURI(), auth0Id, label));
}
}
return budaUserByAuth0Id;
}
public static BudaRdfUser getBudaRdfInfo(String auth0Id) {
return getBudaRdfUsers().get(auth0Id);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy