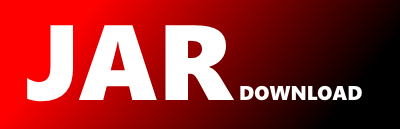
com.fivefaces.cloud.chat.AzureMeetingService Maven / Gradle / Ivy
package com.fivefaces.cloud.chat;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.web.client.RestTemplateBuilder;
import org.springframework.context.annotation.Profile;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.util.UriComponentsBuilder;
import java.util.Map;
@Profile("CHAT_AZURE")
@Slf4j
@Service
public class AzureMeetingService implements MeetingService {
private final RestTemplateBuilder restTemplateBuilder;
private RestTemplate restTemplate;
public AzureMeetingService(RestTemplateBuilder restTemplateBuilder) {
this.restTemplateBuilder = restTemplateBuilder;
}
@Override
public String getToken() {
UriComponentsBuilder builder = UriComponentsBuilder.fromHttpUrl("https://login.microsoftonline.com/db22c75a-5289-4193-9327-70732230ff28/oauth2/token");
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
MultiValueMap map = new LinkedMultiValueMap();
map.add("grant_type", "client_credentials");
map.add("client_id", "4ee3d788-2170-4872-b331-081fe95dafda");
map.add("client_secret", "cXW7Q~K.KQJt9ECiD5Zq1GfOAugXyctOcVzkC");
map.add("resource", "https://graph.microsoft.com/");
HttpEntity> request = new HttpEntity>(map, headers);
ResponseEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy