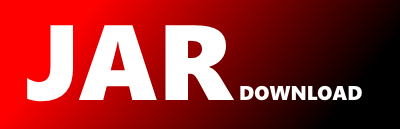
com.fivefaces.cloud.email.JavaEmailService Maven / Gradle / Ivy
package com.fivefaces.cloud.email;
import lombok.AllArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.context.annotation.Profile;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
@Profile("EMAIL_JAVA")
@Slf4j
@AllArgsConstructor
@Service
public class JavaEmailService implements EmailService {
private final JavaMailSender mailSender;
private final boolean PLAIN = Boolean.FALSE;
private final boolean HTML = Boolean.TRUE;
@Override
public void sendEmail(final String fromAddress, final String toAddress, final String subject, final String htmlBody, final String plainBody) throws MessagingException {
doSendEmail(fromAddress, toAddress, subject, htmlBody, plainBody);
}
private void doSendEmail(final String fromAddress, final String toAddress, final String subject, final String htmlBody, final String plainBody) throws MessagingException {
MimeMessage mimeMessage = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, "utf-8");
helper.setTo(toAddress);
helper.setFrom(fromAddress);
helper.setSubject(subject);
if (StringUtils.isNotEmpty(plainBody)) {
helper.setText(plainBody, PLAIN);
} else {
helper.setText(htmlBody, HTML);
}
mailSender.send(mimeMessage);
log.info("Send email to " + toAddress);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy