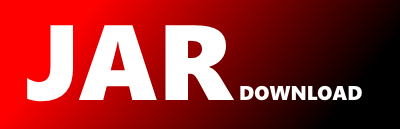
com.fivefaces.cloud.file_storage.AzureBlobFileService Maven / Gradle / Ivy
package com.fivefaces.cloud.file_storage;
import com.azure.storage.blob.BlobClientBuilder;
import com.fivefaces.cloud.Utils;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.NotImplementedException;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Service;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.util.UUID;
import static com.fivefaces.common.cloud.CloudConstants.getCannotSaveFile;
@Profile("FILE_STORAGE_AZURE_BLOB")
@Service
@Slf4j
@RequiredArgsConstructor
public class AzureBlobFileService implements FileService {
private final BlobClientBuilder client = new BlobClientBuilder();
private final Utils utils;
@Override
public String saveFile(String destination, String filename, byte[] data) {
if(data.length>0){
InputStream inputStream = new ByteArrayInputStream(data);
try{
String fullFilePath = UUID.randomUUID() + "/" + filename;
client.connectionString(utils.getAzureConnectionString())
.containerName(utils.getAzureContainerName())
.blobName(fullFilePath)
.buildClient()
.upload(inputStream,inputStream.available());
return fullFilePath;
} catch (Exception exception){
log.error(getCannotSaveFile(exception));
}
}
throw new NotImplementedException();
}
@Override
public String getFileUrl(String destination, String fileKey) {
throw new NotImplementedException();
}
@Override
public void delete(String destination, String fileKey) {
throw new NotImplementedException();
}
public byte[] getFile(String fileName) {
return client.connectionString(utils.getAzureConnectionString())
.containerName(utils.getAzureContainerName())
.blobName(fileName)
.buildClient()
.downloadContent()
.toBytes();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy