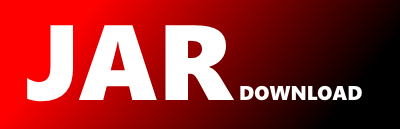
com.fivefaces.cloud.file_storage.FileSystemFileService Maven / Gradle / Ivy
package com.fivefaces.cloud.file_storage;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.NotImplementedException;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Service;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.OutputStream;
import static com.fivefaces.common.cloud.CloudConstants.getCannotSaveFile;
@Profile("FILE_STORAGE_FILE")
@Slf4j
@Service
public class FileSystemFileService implements FileService {
@Override
public String saveFile(String destination, String filename, byte[] data) {
try {
File file = new File(destination + filename);
OutputStream out = new FileOutputStream(file);
out.write(data);
out.close();
return file.getAbsolutePath();
} catch (Exception e) {
log.error(e.getMessage());
return String.format("Could not save file %s", e.getMessage());
}
}
@Override
public String getFileUrl(String destination, String fileKey) {
try {
File file = new File(destination + fileKey);
if (file.exists()) {
return file.getAbsolutePath();
} else {
return String.format("Could not find specified file. ");
}
} catch (Exception e) {
log.error(e.getMessage());
return String.format("Could not get file %s", e.getMessage());
}
}
@Override
public void delete(String destination, String fileKey) {
try {
File file = new File(destination + fileKey);
if (file.delete()) {
log.info("File is deleted successfully.");
} else {
log.error("File is not deleted successfully.");
}
} catch (Exception e) {
log.error(e.getMessage());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy