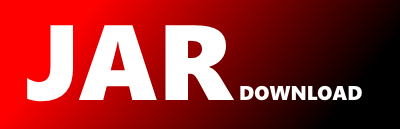
com.fivefaces.cloud.sms.BurstSMSService Maven / Gradle / Ivy
package com.fivefaces.cloud.sms;
import com.fivefaces.cloud.Utils;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.boot.web.client.RestTemplateBuilder;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.util.UriComponentsBuilder;
import java.util.Map;
@Profile("SMS_BURST")
@Slf4j
@Service
public class BurstSMSService implements SMSService{
private final RestTemplateBuilder restTemplateBuilder;
private final Utils utils;
private RestTemplate restTemplate;
private String lastUsedKey;
private String lastUsedSecret;
public BurstSMSService(RestTemplateBuilder restTemplateBuilder, Utils utils) {
this.restTemplateBuilder = restTemplateBuilder;
this.utils = utils;
}
@Override
public void sendSms(String phoneNumber, String message) {
UriComponentsBuilder builder = UriComponentsBuilder.fromHttpUrl(utils.getBurstSenderUrl() + "/send-sms.json")
.queryParam("message", message)
.queryParam("to", phoneNumber)
.queryParam("from", utils.getSmsSenderId())
.queryParam("countrycode", "+61");
getOrCreateRestTemplate().getForEntity(builder.build(false).toUri(), Map.class);
log.info("Sent Sms to " + phoneNumber);
}
private RestTemplate getOrCreateRestTemplate() {
String currentKey = utils.getBurstCurrentKey();
String currentSecret = utils.getBurstCurrentSecret();
if (!StringUtils.equals(lastUsedKey, currentKey) || !StringUtils.equals(lastUsedSecret, currentSecret)) {
lastUsedKey = currentKey;
lastUsedSecret = currentSecret;
restTemplate = restTemplateBuilder.basicAuthentication(currentKey, currentSecret).build();
}
return restTemplate;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy