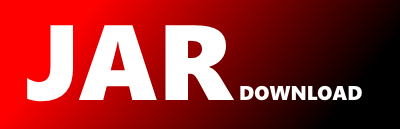
com.fivefaces.cloud.warehouse.WarehouseServiceImpl Maven / Gradle / Ivy
package com.fivefaces.cloud.warehouse;
import com.fivefaces.cloud.warehouse.repository.WarehouseRepository;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.Set;
@Slf4j
@Service
@Profile("WAREHOUSE_AWS_MYSQL")
@Transactional(transactionManager = "warehouseTransactionManager")
public class WarehouseServiceImpl implements WarehouseService {
private final WarehouseUtils warehouseUtils;
private final WarehouseRepository warehouseRepository;
public WarehouseServiceImpl(WarehouseRepository warehouseRepository, WarehouseUtils warehouseUtils) {
this.warehouseRepository = warehouseRepository;
this.warehouseUtils = warehouseUtils;
}
@Override
public void recordWarehouseEntry(final String previousRecord,
final String updatedRecord,
final String id,
final String destinationTable,
Set primaryUpdates) throws Exception {
try {
if (previousRecord == null) {
log.info("No previous record so doing an insert");
warehouseRepository.execute(warehouseRepository.generateInsertStatement(updatedRecord, destinationTable), updatedRecord);
} else {
boolean isPrimaryUpdate = warehouseUtils.isPrimaryUpdate(previousRecord, updatedRecord, primaryUpdates);
if (isPrimaryUpdate) {
log.info("Is a primary update");
warehouseRepository.execute(warehouseRepository.generateTieOffUpdateStatement(id, destinationTable));
warehouseRepository.execute(warehouseRepository.generateInsertStatement(updatedRecord, destinationTable), updatedRecord);
} else {
log.info("Is a secondary update");
final String secondarySQL = warehouseRepository.generateSecondaryUpdateStatement(previousRecord, updatedRecord, id, destinationTable);
if (secondarySQL != null) {
warehouseRepository.execute(warehouseRepository.generateSecondaryUpdateStatement(previousRecord, updatedRecord, id, destinationTable), updatedRecord);
}
}
}
} catch (Exception sqlException) {
log.info(sqlException.getMessage());
throw new Exception(sqlException);
}
}
@Override
public void recordWarehouseDeletionEntry(final String id, final String destinationTable) throws Exception {
try {
warehouseRepository.execute(warehouseRepository.generateTieOffUpdateStatement(id, destinationTable));
} catch (Exception sqlException) {
log.info(sqlException.getMessage());
throw new Exception(sqlException);
}
}
@Override
public void createTable(final String json, final String destinationTable) {
warehouseRepository.createTable(json, destinationTable);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy