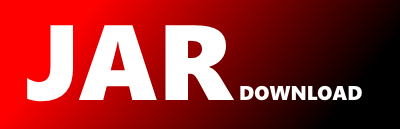
com.fivefaces.cloud.warehouse.WarehouseUtils Maven / Gradle / Ivy
package com.fivefaces.cloud.warehouse;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.collect.MapDifference;
import com.google.common.collect.Maps;
import lombok.AllArgsConstructor;
import org.springframework.stereotype.Component;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
@AllArgsConstructor
@Component
public class WarehouseUtils {
public boolean isPrimaryUpdate(final String previousRecord, final String updatedRecord, Set primaryUpdates) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
TypeReference> type =
new TypeReference<>() {
};
Map leftMap = mapper.readValue(previousRecord, type);
Map rightMap = mapper.readValue(updatedRecord, type);
MapDifference difference = Maps.difference(leftMap, rightMap);
final Boolean[] isPrimaryUpdate = new Boolean[] {false};
difference.entriesDiffering().forEach( (changedField, objectValueDifference) -> {
if (primaryUpdates.contains(changedField)) {
isPrimaryUpdate[0] = true;
}
});
return isPrimaryUpdate[0];
}
public MapDifference getDifferences(final String previousRecord, final String updatedRecord) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
TypeReference> type =
new TypeReference<>() {
};
Map leftMap = mapper.readValue(previousRecord, type);
Map rightMap = mapper.readValue(updatedRecord, type);
return Maps.difference(leftMap, rightMap);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy