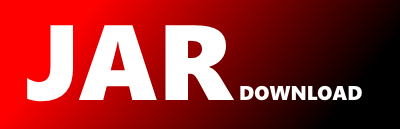
com.fivefaces.cloud.warehouse.repository.MySQLWarehouseRepository Maven / Gradle / Ivy
package com.fivefaces.cloud.warehouse.repository;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fivefaces.cloud.warehouse.WarehouseUtils;
import com.fivefaces.common.util.DateUtils;
import com.google.common.collect.MapDifference;
import lombok.extern.slf4j.Slf4j;
import org.hibernate.Session;
import org.json.JSONArray;
import org.json.JSONObject;
import org.springframework.context.annotation.Profile;
import org.springframework.stereotype.Repository;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import java.util.Set;
@Repository
@Profile("WAREHOUSE_AWS_MYSQL")
@Slf4j
public class MySQLWarehouseRepository implements WarehouseRepository {
private final WarehouseUtils warehouseUtils;
private final DateUtils dateUtils = new DateUtils();
@PersistenceContext(unitName = "warehousePersistenceUnit")
private EntityManager entityManager;
public MySQLWarehouseRepository(WarehouseUtils warehouseUtils) {
this.warehouseUtils = warehouseUtils;
}
@Override
public String generateInsertStatement(final String updatedRecord, final String destinationTable) {
JSONObject jsonObject = new JSONObject(updatedRecord);
Set keys = jsonObject.keySet();
StringBuilder fields = new StringBuilder();
StringBuilder values = new StringBuilder();
keys.forEach(fieldName -> {
if (fieldName.equals("last_modified_by")
|| fieldName.equals("type")
|| fieldName.equals("created_by")
|| fieldName.equals("last_modified_at_utc")
|| fieldName.equals("created_at_utc")) {
// Skip
} else {
Object obj = jsonObject.get(fieldName);
if (obj instanceof JSONArray) {
log.info("Skipping " + fieldName + " as is a jsonArray");
} else {
fields.append(fieldName).append(",");
if (!(obj instanceof JSONObject)) {
values.append("\"").append(jsonObject.get(fieldName).toString()).append("\",");
} else {
JSONObject embedded = (JSONObject) obj;
values.append("\"").append(embedded.get("id")).append("\",");
}
}
}
});
fields.deleteCharAt(fields.length() - 1);
final String currentUTC = dateUtils.getCurrentUTC();
values.append("\"").append(currentUTC).append("\",");
values.append("\"").append(currentUTC).append("\",");
values.append("\"").append(dateUtils.getEndOfTimeUTC()).append("\",");
values.append("?)");
return String.format("INSERT INTO `warehouse`.`%s` (%s, last_modified_at_utc, effective_date, expired_date, original_json) VALUES (%s", destinationTable, fields, values);
}
@Override
public String generateTieOffUpdateStatement(final String id, final String destinationTable) {
final String currentUTC = dateUtils.getCurrentUTC(-1);
return String.format("UPDATE `warehouse`.`%s` SET last_modified_at_utc = \"" + currentUTC + "\", expired_date = \"" + currentUTC + "\" where id = '%s' and expired_date = \"" + dateUtils.getEndOfTimeUTC() + "\" ", destinationTable, id);
}
@Override
public String generateSecondaryUpdateStatement(final String previousRecord, final String updatedRecord,
final String id, final String destinationTable) throws JsonProcessingException {
final String currentUTC = dateUtils.getCurrentUTC();
MapDifference differences = warehouseUtils.getDifferences(previousRecord, updatedRecord);
if (differences.areEqual()) {
return null;
}
JSONObject updatedJson = new JSONObject(updatedRecord);
StringBuilder fields = new StringBuilder("SET `last_modified_at_utc` = \"" + currentUTC + "\", `original_json` = ?, ");
differences.entriesDiffering().forEach((changedField, objectValueDifference) -> {
if (updatedJson.get(changedField) instanceof JSONArray) {
// skipping
log.info("Skipping " + changedField + " as is a jsonArray");
} else {
fields.append(changedField).append(" = '").append( objectValueDifference.rightValue() ).append("',");
}
});
fields.deleteCharAt(fields.length() - 1);
return String.format("UPDATE `warehouse`.`%s` %s where id = '%s' and expired_date = \"" + dateUtils.getEndOfTimeUTC() + "\"", destinationTable, fields, id);
}
@Override
public void execute(final String queryString) {
log.info("Executing for warehouse > " + queryString);
try (Session session = entityManager.unwrap(Session.class)) {
Query query = session.createNativeQuery(queryString);
query.executeUpdate();
}
}
@Override
public void execute(final String queryString, final String json ) {
log.info("Executing for warehouse > " + queryString);
try (Session session = entityManager.unwrap(Session.class)) {
Query query = session.createNativeQuery(queryString);
query.setParameter(1, json);
query.executeUpdate();
}
}
@Override
public void createTable(final String fieldsAsJson, final String destinationTable) {
JSONObject jsonObject = new JSONObject(fieldsAsJson);
Set keys = jsonObject.keySet();
StringBuilder fields = new StringBuilder();
keys.forEach(fieldName -> {
if (fieldName.equals("id")) {
fields.append("`").append(fieldName).append("` varchar(100) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci NOT NULL,\n");
} else {
fields.append("`").append(fieldName).append("` varchar(300) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci DEFAULT NULL,\n");
}
});
final String createTable = String.format("CREATE TABLE `warehouse`.`%s` (%s \n`last_modified_at_utc` datetime NOT NULL, \n`effective_date` datetime NOT NULL, \n`expired_date` datetime NOT NULL, `original_json` JSON NOT NULL, `id` varchar(50) NOT NULL, `version` varchar(12) NOT NULL, PRIMARY KEY (`id`,`effective_date`)) \nENGINE=MyISAM AUTO_INCREMENT=2 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci", destinationTable, fields);
execute(createTable);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy