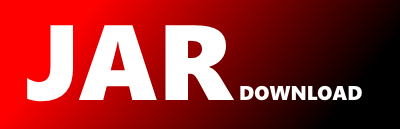
com.fivefaces.cloud.workflow.AWSSQSService Maven / Gradle / Ivy
package com.fivefaces.cloud.workflow;
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.model.Message;
import com.amazonaws.services.sqs.model.ReceiveMessageRequest;
import com.amazonaws.services.stepfunctions.AWSStepFunctions;
import com.amazonaws.services.stepfunctions.model.SendTaskFailureRequest;
import com.amazonaws.services.stepfunctions.model.SendTaskSuccessRequest;
import com.fivefaces.cloud.Utils;
import com.fivefaces.cloud.workflow.function.Function;
import lombok.extern.slf4j.Slf4j;
import org.json.JSONObject;
import org.springframework.context.annotation.Profile;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
import java.util.List;
@Profile("WORKFLOW_LISTENER_AWS")
@Slf4j
@Service
public class AWSSQSService {
private final Utils utils;
private final ProcessFactory processFactory;
private AmazonSQS sqsClient;
private AWSStepFunctions stepFunctionsClient;
public AWSSQSService(Utils utils, ProcessFactory processFactory) {
this.utils = utils;
this.processFactory = processFactory;
}
@Scheduled(fixedRate=2000)
public void receiveMessagesIndefinitely() {
try {
String queueUrl = getSQSClient().getQueueUrl(utils.getSQSQueue()).getQueueUrl();
final ReceiveMessageRequest request = new ReceiveMessageRequest();
request.setMaxNumberOfMessages(1);
request.setWaitTimeSeconds(0);
request.setQueueUrl(queueUrl);
List messages = getSQSClient().receiveMessage(request).getMessages();
for (Message message : messages) {
JSONObject jsonParameters = new JSONObject(message.getBody());
try {
JSONObject taskResult = process_message(jsonParameters);
jsonParameters.put("taskresult", taskResult);
sendTaskSuccess(jsonParameters, taskResult);
} catch (Exception e) {
sendTaskFailure(jsonParameters, e.getMessage());
} finally {
getSQSClient().deleteMessage(queueUrl, message.getReceiptHandle());
}
}
} catch (Exception ignore) {
}
}
private JSONObject process_message(JSONObject jsomParameters) throws Exception {
Function function = processFactory.getFunction(jsomParameters.getString("function"));
function.setup(jsomParameters);
JSONObject result = function.process(jsomParameters);
function.cleanup(jsomParameters);
return result;
}
private void sendTaskSuccess(JSONObject jsomParameters, JSONObject body) {
final SendTaskSuccessRequest sendTaskSuccessRequest = new SendTaskSuccessRequest();
sendTaskSuccessRequest.setTaskToken(jsomParameters.getString("TaskToken"));
sendTaskSuccessRequest.setOutput(body.toString());
getStepFunctionsClient().sendTaskSuccess(sendTaskSuccessRequest);
}
private void sendTaskFailure(JSONObject jsomParameters, String error) {
final SendTaskFailureRequest sendTaskFailureRequest = new SendTaskFailureRequest();
sendTaskFailureRequest.setTaskToken(jsomParameters.getString("TaskToken"));
sendTaskFailureRequest.setCause(error);
sendTaskFailureRequest.setError(error);
getStepFunctionsClient().sendTaskFailure(sendTaskFailureRequest);
}
private AmazonSQS getSQSClient() {
if (sqsClient == null) {
sqsClient = utils.generateSQSClient();
}
return sqsClient;
}
private AWSStepFunctions getStepFunctionsClient() {
if (stepFunctionsClient == null) {
stepFunctionsClient = utils.generateStepFunctionClient();
}
return stepFunctionsClient;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy