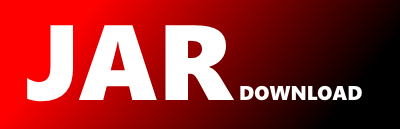
com.fivefaces.cloud.workflow.awsonprem.impl.AnotherMachineStateInvokerImpl Maven / Gradle / Ivy
package com.fivefaces.cloud.workflow.awsonprem.impl;
import com.amazonaws.services.stepfunctions.builder.states.TaskState;
import com.fivefaces.cloud.workflow.awsonprem.AnotherMachineStateInvoker;
import com.fivefaces.cloud.workflow.awsonprem.ObjectValueEnricher;
import com.fivefaces.cloud.workflow.awsonprem.StateMachineService;
import com.fivefaces.cloud.workflow.awsonprem.exception.StateMachineFailedException;
import com.fivefaces.cloud.workflow.awsonprem.model.Execution;
import com.fivefaces.cloud.workflow.awsonprem.model.ExecutionResult;
import com.fivefaces.cloud.workflow.awsonprem.model.ExecutionResultStatus;
import com.fivefaces.cloud.workflow.awsonprem.utils.JsonUtil;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import java.util.HashMap;
import java.util.Map;
@Service
@Slf4j
public class AnotherMachineStateInvokerImpl implements AnotherMachineStateInvoker {
private final JsonUtil jsonUtil;
private final ObjectValueEnricher objectValueEnricher;
private final StateMachineService stateMachineService;
public AnotherMachineStateInvokerImpl(JsonUtil jsonUtil, ObjectValueEnricher objectValueEnricher,
@Lazy StateMachineService stateMachineService) {
this.jsonUtil = jsonUtil;
this.objectValueEnricher = objectValueEnricher;
this.stateMachineService = stateMachineService;
}
@Override
public Object execute(TaskState state, Execution execution) {
try {
Map params = jsonUtil.readMap(state.getParameters());
String stateMachineName = getLocalStateMachineName(params);
Object requestBody = params.get("Input");
objectValueEnricher.enrich(requestBody, execution);
log.info(String.format("Executing state machine name %s with requestBody %s", stateMachineName, requestBody));
ExecutionResult result = stateMachineService.instantiateSyncWorkflow(stateMachineName, jsonUtil.write(requestBody));
log.info(String.format("Execution state machine name %s completed with status %s and output %s", stateMachineName, result.getStatus(), result.getOutput()));
if (result.getStatus() == ExecutionResultStatus.FAILED) {
throw new StateMachineFailedException("Failed to execute task " + stateMachineName + " with error: " + result.getErrorMessage());
}
return new HashMap<>() {{
put("ResponseBody", jsonUtil.read(result.getOutput()));
put("Status", "SUCCEEDED");
}};
} catch (Exception e) {
if (e instanceof StateMachineFailedException) {
throw e;
}
throw new IllegalStateException("Could not execute state " + state.getType() + " " + state.getResource() + " " + state.getComment(), e);
}
}
private String getLocalStateMachineName(Map params) {
String fullArn = (String) params.get("StateMachineArn");
String more = StringUtils.substringAfterLast(fullArn, "stateMachine:");
return more.replaceAll("\\$\\{var.environment}_", "");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy