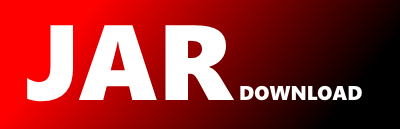
com.fivefaces.cloud.workflow.awsonprem.impl.ApiGatewayStateInvokerImpl Maven / Gradle / Ivy
package com.fivefaces.cloud.workflow.awsonprem.impl;
import com.amazonaws.services.stepfunctions.builder.states.TaskState;
import com.fivefaces.cloud.workflow.awsonprem.ApiGatewayStateInvoker;
import com.fivefaces.cloud.workflow.awsonprem.ObjectValueEnricher;
import com.fivefaces.cloud.workflow.awsonprem.exception.StateMachineFailedException;
import com.fivefaces.cloud.workflow.awsonprem.model.Execution;
import com.fivefaces.cloud.workflow.awsonprem.utils.JsonUtil;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Service;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Service
@Slf4j
public class ApiGatewayStateInvokerImpl implements ApiGatewayStateInvoker {
private final JsonUtil jsonUtil;
private final ObjectValueEnricher objectValueEnricher;
private final HttpClient httpClient;
public ApiGatewayStateInvokerImpl(JsonUtil jsonUtil, ObjectValueEnricher objectValueEnricher) {
this.jsonUtil = jsonUtil;
this.objectValueEnricher = objectValueEnricher;
this.httpClient = HttpClient.newBuilder().version(HttpClient.Version.HTTP_2).build();
}
@Override
public Object execute(TaskState state, Execution execution) {
String responseBody;
try {
Map params = jsonUtil.readMap(state.getParameters());
String address = getAddress(params, execution);
Object requestBody = params.get("RequestBody");
objectValueEnricher.enrich(requestBody, execution);
HttpResponse response = send(getMethod(params), address, getHeaders(params, execution),
jsonUtil.write(requestBody));
if (HttpStatus.valueOf(response.statusCode()).is2xxSuccessful()) {
return new HashMap<>() {{
put("ResponseBody", jsonUtil.read(response.body()));
put("StatusCode", response.statusCode());
put("Headers", response.headers().map());
}};
}
responseBody = response.body();
} catch (Exception e) {
throw new IllegalStateException("Could not execute state " + state.getType() + " " + state.getResource() + " " + state.getComment(), e);
}
throw new StateMachineFailedException(responseBody);
}
private HttpResponse send(String method, String address, Map> headers, String jsonInput) throws Exception {
HttpRequest.Builder builder = HttpRequest.newBuilder()
.method(method, HttpRequest.BodyPublishers.ofString(jsonInput != null ? jsonInput : ""))
.uri(URI.create(address));
headers.forEach((key, values) -> values.forEach(value -> builder.header(key, value)));
HttpRequest request = builder.build();
return this.httpClient.send(request, HttpResponse.BodyHandlers.ofString());
}
private String getMethod(Map params) {
String requestedMethod = (String) params.get("Method");
return StringUtils.isNotBlank(requestedMethod) ? requestedMethod : "GET";
}
private String getAddress(Map params, Execution execution) {
String apiEndpoint = (String) params.get("ApiEndpoint");
if (execution.getContext().containsKey("ApiEndpointReplacements")) {
Map replacements = (Map) execution.getContext().get("ApiEndpointReplacements");
for (String key : replacements.keySet()) {
String value = replacements.get(key);
apiEndpoint = apiEndpoint.replaceAll(key, value);
}
}
String path = (String) params.get("Path");
if (execution.getContext().containsKey("ApiPathReplacements")) {
Map replacements = (Map) execution.getContext().get("ApiPathReplacements");
for (String key : replacements.keySet()) {
String value = replacements.get(key);
path = path.replaceAll(key, value);
}
}
return ((apiEndpoint.startsWith("http://") || apiEndpoint.startsWith("https://")) ? "" : "https://") + apiEndpoint + (apiEndpoint.endsWith("/") || path.startsWith("/") ? "" : "/") + path;
}
private Map> getHeaders(Map params, Execution execution) {
Map> headers = params.containsKey("Headers") ? (Map>) params.get("Headers") : new HashMap<>();
if (!(headers.containsKey("Content-Type") || headers.containsKey("content-type"))) {
headers.put("Content-Type", List.of("application/json"));
}
if (execution.getContext().containsKey("ApiHeadersAddition")) {
Map additions = (Map) execution.getContext().get("ApiHeadersAddition");
for (String key : additions.keySet()) {
String value = additions.get(key);
headers.put(key, List.of(value));
}
}
return headers;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy