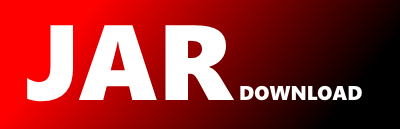
com.fivefaces.cloud.workflow.awsonprem.impl.StateMachineServiceImpl Maven / Gradle / Ivy
package com.fivefaces.cloud.workflow.awsonprem.impl;
import com.amazonaws.services.stepfunctions.builder.StateMachine;
import com.fivefaces.cloud.workflow.awsonprem.StateMachineConstants;
import com.fivefaces.cloud.workflow.awsonprem.StateMachineExecutor;
import com.fivefaces.cloud.workflow.awsonprem.StateMachineRepo;
import com.fivefaces.cloud.workflow.awsonprem.StateMachineService;
import com.fivefaces.cloud.workflow.awsonprem.model.Execution;
import com.fivefaces.cloud.workflow.awsonprem.model.ExecutionResult;
import com.fivefaces.cloud.workflow.awsonprem.utils.JsonUtil;
import com.jayway.jsonpath.Configuration;
import com.jayway.jsonpath.JsonPath;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import java.util.HashMap;
import java.util.Map;
import static com.jayway.jsonpath.Option.SUPPRESS_EXCEPTIONS;
@Service
@RequiredArgsConstructor
@Slf4j
public class StateMachineServiceImpl implements StateMachineService {
private final StateMachineRepo stateMachineRepo;
private final StateMachineExecutor stateMachineExecutor;
private final JsonUtil jsonUtil;
@Value("#{${cloud.aws-on-prem.api-endpoint-replacements:{}}}")
private Map apiEndpointReplacements;
@Value("#{${cloud.aws-on-prem.api-path-replacements:{}}}")
private Map apiPathReplacements;
@Value("#{${cloud.aws-on-prem.api-headers-additions:{}}}")
private Map apiHeadersAdditions;
@Value("${cloud.aws-on-prem.debug:true}")
private Boolean debug;
@Override
public ExecutionResult instantiateSyncWorkflow(String name, String input) {
StateMachine stateMachine = stateMachineRepo.findByName(name);
return stateMachineExecutor.execute(stateMachine, createExecution(input));
}
@Override
public ExecutionResult instantiateWorkflow(String name, String input) {
return instantiateSyncWorkflow(name, input);
}
private Execution createExecution(String input) {
Execution execution = new Execution(jsonUtil);
execution.getContext().put("ApiEndpointReplacements", getAMap(apiEndpointReplacements));
execution.getContext().put("ApiPathReplacements", getAMap(apiPathReplacements));
execution.getContext().put("ApiHeadersAddition", getAMap(apiHeadersAdditions));
execution.getContext().put(StateMachineConstants.DEBUG, debug);
execution.setInput(JsonPath.parse(input, new Configuration.ConfigurationBuilder().options(SUPPRESS_EXCEPTIONS).build()));
return execution;
}
private Map getAMap(Map map) {
if (map == null) {
return new HashMap<>();
}
return map;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy