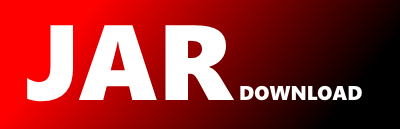
build.bazel.remote.execution.v2.ExecuteResponseKt.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remote-cache Show documentation
Show all versions of remote-cache Show documentation
Bitrise remote cache implementation for Gradle
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: build/bazel/remote/execution/v2/remote_execution.proto
// Generated files should ignore deprecation warnings
@file:Suppress("DEPRECATION")
package build.bazel.remote.execution.v2;
@kotlin.jvm.JvmName("-initializeexecuteResponse")
public inline fun executeResponse(block: build.bazel.remote.execution.v2.ExecuteResponseKt.Dsl.() -> kotlin.Unit): build.bazel.remote.execution.v2.ExecuteResponse =
build.bazel.remote.execution.v2.ExecuteResponseKt.Dsl._create(build.bazel.remote.execution.v2.ExecuteResponse.newBuilder()).apply { block() }._build()
/**
* ```
* The response message for
* [Execution.Execute][build.bazel.remote.execution.v2.Execution.Execute],
* which will be contained in the [response
* field][google.longrunning.Operation.response] of the
* [Operation][google.longrunning.Operation].
* ```
*
* Protobuf type `build.bazel.remote.execution.v2.ExecuteResponse`
*/
public object ExecuteResponseKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: build.bazel.remote.execution.v2.ExecuteResponse.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: build.bazel.remote.execution.v2.ExecuteResponse.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): build.bazel.remote.execution.v2.ExecuteResponse = _builder.build()
/**
* ```
* The result of the action.
* ```
*
* `.build.bazel.remote.execution.v2.ActionResult result = 1;`
*/
public var result: build.bazel.remote.execution.v2.ActionResult
@JvmName("getResult")
get() = _builder.getResult()
@JvmName("setResult")
set(value) {
_builder.setResult(value)
}
/**
* ```
* The result of the action.
* ```
*
* `.build.bazel.remote.execution.v2.ActionResult result = 1;`
*/
public fun clearResult() {
_builder.clearResult()
}
/**
* ```
* The result of the action.
* ```
*
* `.build.bazel.remote.execution.v2.ActionResult result = 1;`
* @return Whether the result field is set.
*/
public fun hasResult(): kotlin.Boolean {
return _builder.hasResult()
}
/**
* ```
* True if the result was served from cache, false if it was executed.
* ```
*
* `bool cached_result = 2;`
*/
public var cachedResult: kotlin.Boolean
@JvmName("getCachedResult")
get() = _builder.getCachedResult()
@JvmName("setCachedResult")
set(value) {
_builder.setCachedResult(value)
}
/**
* ```
* True if the result was served from cache, false if it was executed.
* ```
*
* `bool cached_result = 2;`
*/
public fun clearCachedResult() {
_builder.clearCachedResult()
}
/**
* ```
* If the status has a code other than `OK`, it indicates that the action did
* not finish execution. For example, if the operation times out during
* execution, the status will have a `DEADLINE_EXCEEDED` code. Servers MUST
* use this field for errors in execution, rather than the error field on the
* `Operation` object.
*
* If the status code is other than `OK`, then the result MUST NOT be cached.
* For an error status, the `result` field is optional; the server may
* populate the output-, stdout-, and stderr-related fields if it has any
* information available, such as the stdout and stderr of a timed-out action.
* ```
*
* `.google.rpc.Status status = 3;`
*/
public var status: com.google.rpc.Status
@JvmName("getStatus")
get() = _builder.getStatus()
@JvmName("setStatus")
set(value) {
_builder.setStatus(value)
}
/**
* ```
* If the status has a code other than `OK`, it indicates that the action did
* not finish execution. For example, if the operation times out during
* execution, the status will have a `DEADLINE_EXCEEDED` code. Servers MUST
* use this field for errors in execution, rather than the error field on the
* `Operation` object.
*
* If the status code is other than `OK`, then the result MUST NOT be cached.
* For an error status, the `result` field is optional; the server may
* populate the output-, stdout-, and stderr-related fields if it has any
* information available, such as the stdout and stderr of a timed-out action.
* ```
*
* `.google.rpc.Status status = 3;`
*/
public fun clearStatus() {
_builder.clearStatus()
}
/**
* ```
* If the status has a code other than `OK`, it indicates that the action did
* not finish execution. For example, if the operation times out during
* execution, the status will have a `DEADLINE_EXCEEDED` code. Servers MUST
* use this field for errors in execution, rather than the error field on the
* `Operation` object.
*
* If the status code is other than `OK`, then the result MUST NOT be cached.
* For an error status, the `result` field is optional; the server may
* populate the output-, stdout-, and stderr-related fields if it has any
* information available, such as the stdout and stderr of a timed-out action.
* ```
*
* `.google.rpc.Status status = 3;`
* @return Whether the status field is set.
*/
public fun hasStatus(): kotlin.Boolean {
return _builder.hasStatus()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ServerLogsProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
public val serverLogs: com.google.protobuf.kotlin.DslMap
@kotlin.jvm.JvmSynthetic
@JvmName("getServerLogsMap")
get() = com.google.protobuf.kotlin.DslMap(
_builder.getServerLogsMap()
)
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
@JvmName("putServerLogs")
public fun com.google.protobuf.kotlin.DslMap
.put(key: kotlin.String, value: build.bazel.remote.execution.v2.LogFile) {
_builder.putServerLogs(key, value)
}
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
@kotlin.jvm.JvmSynthetic
@JvmName("setServerLogs")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslMap
.set(key: kotlin.String, value: build.bazel.remote.execution.v2.LogFile) {
put(key, value)
}
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
@kotlin.jvm.JvmSynthetic
@JvmName("removeServerLogs")
public fun com.google.protobuf.kotlin.DslMap
.remove(key: kotlin.String) {
_builder.removeServerLogs(key)
}
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
@kotlin.jvm.JvmSynthetic
@JvmName("putAllServerLogs")
public fun com.google.protobuf.kotlin.DslMap
.putAll(map: kotlin.collections.Map) {
_builder.putAllServerLogs(map)
}
/**
* ```
* An optional list of additional log outputs the server wishes to provide. A
* server can use this to return execution-specific logs however it wishes.
* This is intended primarily to make it easier for users to debug issues that
* may be outside of the actual job execution, such as by identifying the
* worker executing the action or by providing logs from the worker's setup
* phase. The keys SHOULD be human readable so that a client can display them
* to a user.
* ```
*
* `map server_logs = 4;`
*/
@kotlin.jvm.JvmSynthetic
@JvmName("clearServerLogs")
public fun com.google.protobuf.kotlin.DslMap
.clear() {
_builder.clearServerLogs()
}
/**
* ```
* Freeform informational message with details on the execution of the action
* that may be displayed to the user upon failure or when requested explicitly.
* ```
*
* `string message = 5;`
*/
public var message: kotlin.String
@JvmName("getMessage")
get() = _builder.getMessage()
@JvmName("setMessage")
set(value) {
_builder.setMessage(value)
}
/**
* ```
* Freeform informational message with details on the execution of the action
* that may be displayed to the user upon failure or when requested explicitly.
* ```
*
* `string message = 5;`
*/
public fun clearMessage() {
_builder.clearMessage()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun build.bazel.remote.execution.v2.ExecuteResponse.copy(block: `build.bazel.remote.execution.v2`.ExecuteResponseKt.Dsl.() -> kotlin.Unit): build.bazel.remote.execution.v2.ExecuteResponse =
`build.bazel.remote.execution.v2`.ExecuteResponseKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val build.bazel.remote.execution.v2.ExecuteResponseOrBuilder.resultOrNull: build.bazel.remote.execution.v2.ActionResult?
get() = if (hasResult()) getResult() else null
public val build.bazel.remote.execution.v2.ExecuteResponseOrBuilder.statusOrNull: com.google.rpc.Status?
get() = if (hasStatus()) getStatus() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy