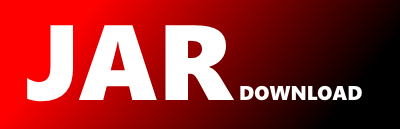
com.google.api.EndpointKt.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remote-cache Show documentation
Show all versions of remote-cache Show documentation
Bitrise remote cache implementation for Gradle
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/api/endpoint.proto
// Generated files should ignore deprecation warnings
@file:Suppress("DEPRECATION")
package com.google.api;
@kotlin.jvm.JvmName("-initializeendpoint")
public inline fun endpoint(block: com.google.api.EndpointKt.Dsl.() -> kotlin.Unit): com.google.api.Endpoint =
com.google.api.EndpointKt.Dsl._create(com.google.api.Endpoint.newBuilder()).apply { block() }._build()
/**
* ```
* `Endpoint` describes a network endpoint that serves a set of APIs.
* A service may expose any number of endpoints, and all endpoints share the
* same service configuration, such as quota configuration and monitoring
* configuration.
*
* Example service configuration:
*
* name: library-example.googleapis.com
* endpoints:
* # Below entry makes 'google.example.library.v1.Library'
* # API be served from endpoint address library-example.googleapis.com.
* # It also allows HTTP OPTIONS calls to be passed to the backend, for
* # it to decide whether the subsequent cross-origin request is
* # allowed to proceed.
* - name: library-example.googleapis.com
* allow_cors: true
* ```
*
* Protobuf type `google.api.Endpoint`
*/
public object EndpointKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: com.google.api.Endpoint.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: com.google.api.Endpoint.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): com.google.api.Endpoint = _builder.build()
/**
* ```
* The canonical name of this endpoint.
* ```
*
* `string name = 1;`
*/
public var name: kotlin.String
@JvmName("getName")
get() = _builder.getName()
@JvmName("setName")
set(value) {
_builder.setName(value)
}
/**
* ```
* The canonical name of this endpoint.
* ```
*
* `string name = 1;`
*/
public fun clearName() {
_builder.clearName()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class AliasesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @return A list containing the aliases.
*/
public val aliases: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getAliasesList()
)
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @param value The aliases to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAliases")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addAliases(value)
}
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @param value The aliases to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAliases")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @param values The aliases to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllAliases")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllAliases(values)
}
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @param values The aliases to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllAliases")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
* @param index The index to set the value at.
* @param value The aliases to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setAliases")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setAliases(index, value)
}/**
* ```
* DEPRECATED: This field is no longer supported. Instead of using aliases,
* please specify multiple [google.api.Endpoint][google.api.Endpoint] for each of the intended
* aliases.
*
* Additional names that this endpoint will be hosted on.
* ```
*
* `repeated string aliases = 2;`
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearAliases")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearAliases()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class FeaturesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @return A list containing the features.
*/
public val features: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getFeaturesList()
)
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @param value The features to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addFeatures")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addFeatures(value)
}
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @param value The features to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignFeatures")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @param values The features to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllFeatures")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllFeatures(values)
}
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @param values The features to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllFeatures")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
* @param index The index to set the value at.
* @param value The features to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setFeatures")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setFeatures(index, value)
}/**
* ```
* The list of features enabled on this endpoint.
* ```
*
* `repeated string features = 4;`
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearFeatures")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearFeatures()
}
/**
* ```
* The specification of an Internet routable address of API frontend that will
* handle requests to this [API Endpoint](https://cloud.google.com/apis/design/glossary).
* It should be either a valid IPv4 address or a fully-qualified domain name.
* For example, "8.8.8.8" or "myservice.appspot.com".
* ```
*
* `string target = 101;`
*/
public var target: kotlin.String
@JvmName("getTarget")
get() = _builder.getTarget()
@JvmName("setTarget")
set(value) {
_builder.setTarget(value)
}
/**
* ```
* The specification of an Internet routable address of API frontend that will
* handle requests to this [API Endpoint](https://cloud.google.com/apis/design/glossary).
* It should be either a valid IPv4 address or a fully-qualified domain name.
* For example, "8.8.8.8" or "myservice.appspot.com".
* ```
*
* `string target = 101;`
*/
public fun clearTarget() {
_builder.clearTarget()
}
/**
* ```
* Allowing
* [CORS](https://en.wikipedia.org/wiki/Cross-origin_resource_sharing), aka
* cross-domain traffic, would allow the backends served from this endpoint to
* receive and respond to HTTP OPTIONS requests. The response will be used by
* the browser to determine whether the subsequent cross-origin request is
* allowed to proceed.
* ```
*
* `bool allow_cors = 5;`
*/
public var allowCors: kotlin.Boolean
@JvmName("getAllowCors")
get() = _builder.getAllowCors()
@JvmName("setAllowCors")
set(value) {
_builder.setAllowCors(value)
}
/**
* ```
* Allowing
* [CORS](https://en.wikipedia.org/wiki/Cross-origin_resource_sharing), aka
* cross-domain traffic, would allow the backends served from this endpoint to
* receive and respond to HTTP OPTIONS requests. The response will be used by
* the browser to determine whether the subsequent cross-origin request is
* allowed to proceed.
* ```
*
* `bool allow_cors = 5;`
*/
public fun clearAllowCors() {
_builder.clearAllowCors()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun com.google.api.Endpoint.copy(block: `com.google.api`.EndpointKt.Dsl.() -> kotlin.Unit): com.google.api.Endpoint =
`com.google.api`.EndpointKt.Dsl._create(this.toBuilder()).apply { block() }._build()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy