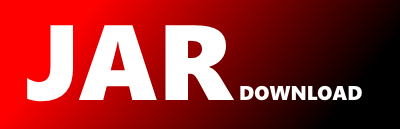
io.bitsensor.lib.entity.FalsePositive Maven / Gradle / Ivy
package io.bitsensor.lib.entity;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.google.re2j.Pattern;
import io.bitsensor.lib.entity.proto.Datapoint;
import io.bitsensor.lib.entity.proto.Detection;
import javax.validation.constraints.NotNull;
import java.util.AbstractMap.SimpleEntry;
import java.util.Date;
import java.util.List;
public class FalsePositive {
@NotNull
@JsonFormat(shape = JsonFormat.Shape.STRING)
private Long detectionHash;
/**
* List of key-value pairs in the DataPoint that must match before the @{@link FalsePositive} is considered to be a
* match
*/
private List> triggerSet;
/**
* List of jregex {@link Pattern} that must match, to declare a {@link Detection} as false positive
*/
private List keyPatterns;
private Date creationDate = new Date();
private String dataPointIdentifier;
private Detection basedOnDetection;
/**
* @param detectionHash Hash or ruleHash that the false positive matches on
* @param dataPointIdentifier {@link Datapoint} from which the false positive is added
* @param triggerNameSet List of keys and values that all have to match before the {@link Detection} is a false
* positive
*/
public FalsePositive(Long detectionHash,
String dataPointIdentifier,
List> triggerNameSet,
List keyPatterns
) {
this(detectionHash, dataPointIdentifier, triggerNameSet, keyPatterns, null);
}
/**
* @param detectionHash Hash or ruleHash that the false positive matches on
* @param triggerNameSet List of keys and values that all have to match before the {@link Detection} is a false
* positive
* @param dataPointIdentifier {@link Datapoint} from which the false positive is added
* @param basedOnDetection {@link Detection} that has been triggered but is treated as false positive
*/
public FalsePositive(Long detectionHash,
String dataPointIdentifier,
List> triggerNameSet,
List keyPatterns,
Detection basedOnDetection) {
this.setDetection(basedOnDetection);
this.setDetectionHash(detectionHash);
this.setDataPointIdentifier(dataPointIdentifier);
this.setKeyPatterns(keyPatterns);
this.setTriggerSet(triggerNameSet);
}
public FalsePositive() {
}
public Long getDetectionHash() {
return detectionHash;
}
public void setDetectionHash(Long detectionHash) {
this.detectionHash = detectionHash;
}
/**
* @return {@link List} of {@link java.util.Map.Entry} with as key the {@link Datapoint} key, and the value it
* should have. A {@link FalsePositive} should only match if all keys and values match.
*/
public List> getTriggerSet() {
return triggerSet;
}
public void setTriggerSet(List> triggerSet) {
this.triggerSet = triggerSet;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public String getDataPointIdentifier() {
return dataPointIdentifier;
}
public void setDataPointIdentifier(String dataPointIdentifier) {
this.dataPointIdentifier = dataPointIdentifier;
}
public Detection getDetection() {
return basedOnDetection;
}
public void setDetection(Detection basedOnDetection) {
this.basedOnDetection = basedOnDetection;
}
public List getKeyPatterns() {
return keyPatterns;
}
public void setKeyPatterns(List keyPatterns) {
this.keyPatterns = keyPatterns;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy