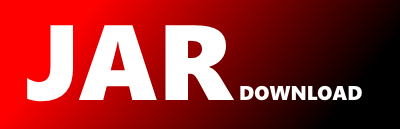
io.blockfrost.sdk.api.AssetService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blockfrost-java Show documentation
Show all versions of blockfrost-java Show documentation
Java SDK for the Blockfrost.io API
package io.blockfrost.sdk.api;
import io.blockfrost.sdk.api.exception.APIException;
import io.blockfrost.sdk.api.model.*;
import io.blockfrost.sdk.api.util.OrderEnum;
import java.util.List;
public interface AssetService {
/**
* Specific asset
* Information about a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @return Asset
*/
Asset getAsset(String asset) throws APIException;
/**
* Assets
* List of assets.
*
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<Asset>
*/
List getAssets(int count, int page, OrderEnum order) throws APIException;
/**
* Assets
* List of assets ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<Asset>
*/
List getAssets(int count, int page) throws APIException;
/**
* Asset history
* History of a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<AssetHistory>
*/
List getAssetHistory(String asset, int count, int page, OrderEnum order) throws APIException;
/**
* Asset history
* History of a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<AssetHistory>
*/
List getAssetHistory(String asset, int count, int page) throws APIException;
/**
* Asset history
* Entire History of a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<AssetHistory>
*/
List getEntireAssetHistory(String asset, OrderEnum order) throws APIException;
/**
* Asset history
* Entire History of a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @return List<AssetHistory>
*/
List getEntireAssetHistory(String asset) throws APIException;
/**
* Asset transactions
* List of a specific asset transactions
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<AssetTransaction>
*/
List getAssetTransactions(String asset, int count, int page, OrderEnum order) throws APIException;
/**
* Asset transactions
* List of a specific asset transactions ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<AssetTransaction>
*/
List getAssetTransactions(String asset, int count, int page) throws APIException;
/**
* Asset transactions
* A list of all transactions for a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<AssetTransaction>
*/
List getAllAssetTransactions(String asset, OrderEnum order) throws APIException;
/**
* Asset transactions
* A list of all transactions for a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @return List<AssetTransaction>
*/
List getAllAssetTransactions(String asset) throws APIException;
/**
* Asset addresses
* List of addresses containing a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<AssetAddress>
*/
List getAssetAddresses(String asset, int count, int page, OrderEnum order) throws APIException;
/**
* Asset addresses
* List of addresses containing a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<AssetAddress>
*/
List getAssetAddresses(String asset, int count, int page) throws APIException;
/**
* Asset addresses
* List of addresses containing a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<AssetAddress>
*/
List getAllAssetAddresses(String asset, OrderEnum order) throws APIException;
/**
* Asset addresses
* List of addresses containing a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @return List<AssetAddress>
*/
List getAllAssetAddresses(String asset) throws APIException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy
*
* @param policyId Specific policy_id (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<PolicyAsset>>
*/
List getPolicyAssets(String policyId, int count, int page, OrderEnum order) throws APIException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param policyId Specific policy_id (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<PolicyAsset>>
*/
List getPolicyAssets(String policyId, int count, int page) throws APIException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy
*
* @param policyId Specific policy_id (required)
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<PolicyAsset>>
*/
List getAllPolicyAssets(String policyId, OrderEnum order) throws APIException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param policyId Specific policy_id (required)
* @return List<PolicyAsset>>
*/
List getAllPolicyAssets(String policyId) throws APIException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy