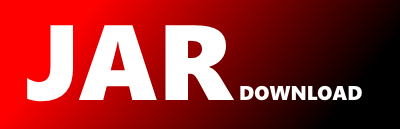
io.blockfrost.sdk.api.BlockService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blockfrost-java Show documentation
Show all versions of blockfrost-java Show documentation
Java SDK for the Blockfrost.io API
package io.blockfrost.sdk.api;
import io.blockfrost.sdk.api.exception.APIException;
import io.blockfrost.sdk.api.model.Block;
import io.blockfrost.sdk.api.util.OrderEnum;
import java.util.List;
public interface BlockService {
/**
* Latest block
* Return the latest block available to the backends, also known as the tip of the blockchain.
*
* @return Block
*/
Block getLatestBlock() throws APIException;
/**
* Specific block
* Return the content of a requested block.
*
* @param hashOrNumber Hash of the requested block. (required)
* @return Block
*/
Block getBlock(String hashOrNumber) throws APIException;
/**
* Specific block in a slot
* Return the content of a requested block for a specific slot.
*
* @param slotNumber Slot position for requested block. (required)
* @return Block
*/
Block getBlockInSlot(int slotNumber) throws APIException;
/**
* Specific block in a slot in an epoch
* Return the content of a requested block for a specific slot in an epoch
*
* @param epochNumber Epoch for specific epoch slot. (required)
* @param slotNumber Slot position for requested block. (required)
* @return Block
*/
Block getBlockInEpochInSlot(int epochNumber, int slotNumber) throws APIException;
/**
* Latest block transactions
* Return the transactions within the latest block.
*
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order Ordered by tx index in the block. The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<String>
*/
List getTransactionsInLatestBlock(int count, int page, OrderEnum order) throws APIException;
/**
* Latest block transactions
* Return the transactions within the latest block ordered from the point of view of the blockchain, not the page listing itself.
*
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<String>
*/
List getTransactionsInLatestBlock(int count, int page) throws APIException;
/**
* Latest block transactions
* Return all the transactions within the latest block.
*
* @param order Ordered by tx index in the block. The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List<String>
*/
List getAllTransactionsInLatestBlock(OrderEnum order) throws APIException;
/**
* Latest block transactions
* Return all the transactions within the latest block ordered from the point of view of the blockchain, not the page listing itself.
*
* @return List<String>
*/
List getAllTransactionsInLatestBlock() throws APIException;
/**
* Listing of next blocks
* Return the list of blocks following a specific block.
*
* @param hashOrNumber Hash of the requested block.
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<Block>
*/
List getNextBlocks(String hashOrNumber, int count, int page) throws APIException;
/**
* Listing of next blocks
* Return the list of all blocks following a specific block.
*
* @param hashOrNumber Hash of the requested block.
* @return List<Block>
*/
List getAllNextBlocks(String hashOrNumber) throws APIException;
/**
* Listing of previous blocks
* Return the list of blocks following a specific block.
*
* @param hashOrNumber Hash of the requested block.
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<Block>
*/
List getPreviousBlocks(String hashOrNumber, int count, int page) throws APIException;
/**
* Listing of previous blocks
* Return the list of all blocks following a specific block.
*
* @param hashOrNumber Hash of the requested block.
* @return List<Block>
*/
List getAllPreviousBlocks(String hashOrNumber) throws APIException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy