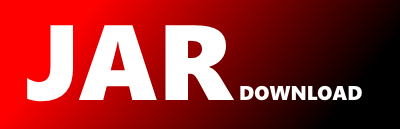
io.blockfrost.sdk.impl.TransactionServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blockfrost-java Show documentation
Show all versions of blockfrost-java Show documentation
Java SDK for the Blockfrost.io API
package io.blockfrost.sdk.impl;
import io.blockfrost.sdk.api.TransactionService;
import io.blockfrost.sdk.api.exception.APIException;
import io.blockfrost.sdk.api.model.*;
import io.blockfrost.sdk.impl.retrofit.TransactionsApi;
import okhttp3.MediaType;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.Response;
import java.io.IOException;
import java.util.List;
public class TransactionServiceImpl extends BaseService implements TransactionService {
TransactionsApi transactionsApi;
public TransactionServiceImpl(String baseUrl, String projectId) {
super(baseUrl, projectId);
transactionsApi = getRetrofit().create(TransactionsApi.class);
}
@Override
public Transaction getTransaction(String hash) throws APIException {
Call transactionCall = transactionsApi.txsHashGet(getProjectId(), hash);
try {
Response transactionResponse = transactionCall.execute();
return processResponse(transactionResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction for hash " + hash, exp);
}
}
@Override
public TransactionUtxo getTransactionUtxo(String hash) throws APIException {
Call transactionUtxoCall = transactionsApi.txsHashUtxosGet(getProjectId(), hash);
try {
Response transactionUtxoResponse = transactionUtxoCall.execute();
return processResponse(transactionUtxoResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction utxos for hash " + hash, exp);
}
}
@Override
public List getTransactionStakes(String hash) throws APIException {
Call> transactionStakesCall = transactionsApi.txsHashStakesGet(getProjectId(), hash);
try {
Response> transactionStakesResponse = transactionStakesCall.execute();
return processResponse(transactionStakesResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction stakes for hash " + hash, exp);
}
}
@Override
public List getTransactionDelegations(String hash) throws APIException {
Call> transactionDelegationCall = transactionsApi.txsHashDelegationsGet(getProjectId(), hash);
try {
Response> transactionDelegationsResponse = transactionDelegationCall.execute();
return processResponse(transactionDelegationsResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction delegations for hash " + hash, exp);
}
}
@Override
public List getTransactionWithdrawals(String hash) throws APIException {
Call> transactionWithdrawalCall = transactionsApi.txsHashWithdrawalsGet(getProjectId(), hash);
try {
Response> transactionWithdrawalsResponse = transactionWithdrawalCall.execute();
return processResponse(transactionWithdrawalsResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction withdrawals for hash " + hash, exp);
}
}
@Override
public List getTransactionMirs(String hash) throws APIException {
Call> transactionMirCall = transactionsApi.txsHashMirsGet(getProjectId(), hash);
try {
Response> transactionMirsResponse = transactionMirCall.execute();
return processResponse(transactionMirsResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction mirs for hash " + hash, exp);
}
}
@Override
public List getTransactionPoolUpdates(String hash) throws APIException {
Call> transactionPoolUpdateCall = transactionsApi.txsHashPoolUpdatesGet(getProjectId(), hash);
try {
Response> transactionPoolUpdatesResponse = transactionPoolUpdateCall.execute();
return processResponse(transactionPoolUpdatesResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction pool updates for hash " + hash, exp);
}
}
@Override
public List getTransactionPoolRetires(String hash) throws APIException {
Call> transactionPoolRetireCall = transactionsApi.txsHashPoolRetiresGet(getProjectId(), hash);
try {
Response> transactionPoolRetiresResponse = transactionPoolRetireCall.execute();
return processResponse(transactionPoolRetiresResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction pool retires for hash " + hash, exp);
}
}
@Override
public List getTransactionMetadata(String hash) throws APIException {
Call> transactionMetadataCall = transactionsApi.txsHashMetadataGet(getProjectId(), hash);
try {
Response> transactionMetadataResponse = transactionMetadataCall.execute();
return processResponse(transactionMetadataResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction metadata for hash " + hash, exp);
}
}
@Override
public List getTransactionMetadataCbor(String hash) throws APIException {
Call> transactionCborMetadataCall = transactionsApi.txsHashMetadataCborGet(getProjectId(), hash);
try {
Response> transactionCborMetadataResponse = transactionCborMetadataCall.execute();
return processResponse(transactionCborMetadataResponse);
} catch (IOException exp) {
throw new APIException("Exception while fetching transaction cbor metadata for hash " + hash, exp);
}
}
@Override
public String submitTransaction(byte[] serializedTransaction) throws APIException {
RequestBody requestBody = RequestBody.create(MediaType.parse("application/cbor"), serializedTransaction);
Call transactionSubmitCall = transactionsApi.txSubmitPost(getProjectId(), requestBody);
try {
Response transactionSubmitResponse = transactionSubmitCall.execute();
return processResponse(transactionSubmitResponse);
} catch (IOException exp) {
throw new APIException("Exception while submitting transaction " + new String(serializedTransaction), exp);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy