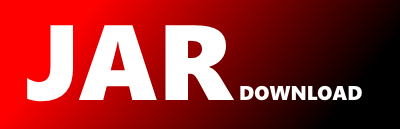
io.blockfrost.sdk.impl.retrofit.IPFSApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blockfrost-java Show documentation
Show all versions of blockfrost-java Show documentation
Java SDK for the Blockfrost.io API
package io.blockfrost.sdk.impl.retrofit;
import io.blockfrost.sdk.api.model.ipfs.PinItem;
import io.blockfrost.sdk.api.model.ipfs.PinResponse;
import io.blockfrost.sdk.api.model.ipfs.IPFSObject;
import okhttp3.MultipartBody;
import okhttp3.ResponseBody;
import retrofit2.Call;
import retrofit2.http.*;
import java.util.List;
public interface IPFSApi {
/**
* Add a file or directory to IPFS
*
* @return
*/
@Multipart
@POST("ipfs/add")
Call add(
@Header("project_id") String projectId,
@Part MultipartBody.Part file
);
/**
* Relay to an IPFS gateway
* Retrieve an object from the IFPS gateway (useful if you do not want to rely on a public gateway, such as `ipfs.blockfrost.dev`).
* @param ipFSPath (required)
* @return Call<ResponseBody>
*/
@GET("ipfs/gateway/{IPFS_path}")
Call get(
@Header("project_id") String projectId,
@Path("IPFS_path") String ipFSPath
);
/**
* Pin an object
* Pinned objects are counted in your user storage quota.
* @param ipFSPath (required)
* @return Call<IPFSAddPinResponse>
*/
@POST("ipfs/pin/add/{IPFS_path}")
Call pinAdd(
@Header("project_id") String projectId,
@Path("IPFS_path") String ipFSPath
);
/**
*
* List objects pinned to local storage
* @param count The number of results displayed on one page. (optional, default to 100)
* @param page The page number for listing the results. (optional, default to 1)
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last. (optional, default to asc)
* @return Call<List<PinItem>>
*/
@GET("ipfs/pin/list/")
Call> pinList(
@Header("project_id") String projectId,
@Query("count") Integer count, @Query("page") Integer page, @Query("order") String order
);
/**
*
* List objects pinned to local storage
* @param ipFSPath (required)
* @return Call<PinItem>
*/
@GET("ipfs/pin/list/{IPFS_path}")
Call pinListByIpfsPath(
@Header("project_id") String projectId,
@Path("IPFS_path") String ipFSPath
);
/**
*
* Remove pinned objects from local storage
* @param ipFSPath (required)
* @return Call<PinItem>
*/
@POST("ipfs/pin/remove/{IPFS_path}")
Call pinRemove(
@Header("project_id") String projectId,
@Path("IPFS_path") String ipFSPath
);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy