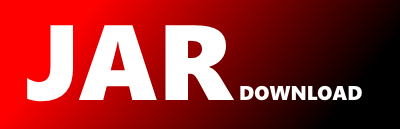
io.blockfrost.sdk.impl.retrofit.TransactionsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blockfrost-java Show documentation
Show all versions of blockfrost-java Show documentation
Java SDK for the Blockfrost.io API
package io.blockfrost.sdk.impl.retrofit;
import io.blockfrost.sdk.api.model.*;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.http.*;
import java.util.List;
public interface TransactionsApi {
/**
* Submit a transaction
* Submit an already serialized transaction to the network.
*
* @return Call<String>
*/
@POST("tx/submit")
Call txSubmitPost(
@Header("project_id") String projectId,
@Body RequestBody signedTxn
);
/**
* Transaction delegation certificates
* Obtain information about delegation certificates of a specific transaction.
*
* @param hash Hash of the requested transaction. (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/delegations")
Call> txsHashDelegationsGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Specific transaction
* Return content of the requested transaction.
*
* @param hash Hash of the requested transaction (required)
* @return Call<TxContent>
*/
@GET("txs/{hash}")
Call txsHashGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction metadata in CBOR
* Obtain the transaction metadata in CBOR.
*
* @param hash Hash of the requested transaction (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/metadata/cbor")
Call> txsHashMetadataCborGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction metadata
* Obtain the transaction metadata.
*
* @param hash Hash of the requested transaction (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/metadata")
Call> txsHashMetadataGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction MIRs
* Obtain information about Move Instantaneous Rewards (MIRs) of a specific transaction.
*
* @param hash Hash of the requested transaction. (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/mirs")
Call> txsHashMirsGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction networkStake pool retirement certificates
* Obtain information about networkStake pool retirements within a specific transaction.
*
* @param hash Hash of the requested transaction (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/pool_retires")
Call> txsHashPoolRetiresGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction networkStake pool registration and update certificates
* Obtain information about networkStake pool registration and update certificates of a specific transaction.
*
* @param hash Hash of the requested transaction (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/pool_updates")
Call> txsHashPoolUpdatesGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction networkStake addresses certificates
* Obtain information about (de)registration of networkStake addresses within a transaction.
*
* @param hash Hash of the requested transaction. (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/stakes")
Call> txsHashStakesGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction UTXOs
* Return the inputs and UTXOs of the specific transaction.
*
* @param hash Hash of the requested transaction (required)
* @return Call<TxContentUtxo>
*/
@GET("txs/{hash}/utxos")
Call txsHashUtxosGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
/**
* Transaction withdrawal
* Obtain information about withdrawals of a specific transaction.
*
* @param hash Hash of the requested transaction. (required)
* @return Call<List<Object>>
*/
@GET("txs/{hash}/withdrawals")
Call> txsHashWithdrawalsGet(
@Header("project_id") String projectId,
@Path("hash") String hash
);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy