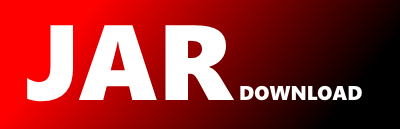
io.bloombox.schema.comms.CommsTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: comms/CommsTask.proto
package io.bloombox.schema.comms;
public final class CommsTask {
private CommsTask() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface SendOperationOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.SendOperation)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
java.lang.String getUuid();
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
com.google.protobuf.ByteString
getUuidBytes();
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
int getChannelValue();
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
io.bloombox.schema.comms.GenericComms.Channel getChannel();
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
int getStatusValue();
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
io.bloombox.schema.comms.CommsTask.SendOperation.Status getStatus();
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 4;
*/
boolean getDryRun();
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
boolean hasSms();
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
io.bloombox.schema.comms.SMSComms.SMSTransmission getSms();
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder getSmsOrBuilder();
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
boolean hasEmail();
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
io.bloombox.schema.comms.EmailComms.EmailTransmission getEmail();
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getEmailOrBuilder();
public io.bloombox.schema.comms.CommsTask.SendOperation.OperationCase getOperationCase();
}
/**
*
* Send a batch of either SMS or email messages.
*
*
* Protobuf type {@code bloombox.comms.SendOperation}
*/
public static final class SendOperation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.SendOperation)
SendOperationOrBuilder {
private static final long serialVersionUID = 0L;
// Use SendOperation.newBuilder() to construct.
private SendOperation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SendOperation() {
uuid_ = "";
channel_ = 0;
status_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SendOperation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
channel_ = rawValue;
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 32: {
dryRun_ = input.readBool();
break;
}
case 82: {
io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder subBuilder = null;
if (operationCase_ == 10) {
subBuilder = ((io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_).toBuilder();
}
operation_ =
input.readMessage(io.bloombox.schema.comms.SMSComms.SMSTransmission.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_);
operation_ = subBuilder.buildPartial();
}
operationCase_ = 10;
break;
}
case 90: {
io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder subBuilder = null;
if (operationCase_ == 11) {
subBuilder = ((io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_).toBuilder();
}
operation_ =
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailTransmission.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_);
operation_ = subBuilder.buildPartial();
}
operationCase_ = 11;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_SendOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_SendOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.SendOperation.class, io.bloombox.schema.comms.CommsTask.SendOperation.Builder.class);
}
/**
*
* Statuses that a send operation may assume.
*
*
* Protobuf enum {@code bloombox.comms.SendOperation.Status}
*/
public enum Status
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* This operation is unfiled, or waiting.
*
*
* PENDING = 0;
*/
PENDING(0),
/**
*
* The operation is executing now.
*
*
* SENDING = 1;
*/
SENDING(1),
/**
*
* An error occurred that prevented this operation from starting/continuing.
*
*
* ERROR = 2;
*/
ERROR(2),
/**
*
* The operation is complete.
*
*
* DONE = 3;
*/
DONE(3),
UNRECOGNIZED(-1),
;
/**
*
* This operation is unfiled, or waiting.
*
*
* PENDING = 0;
*/
public static final int PENDING_VALUE = 0;
/**
*
* The operation is executing now.
*
*
* SENDING = 1;
*/
public static final int SENDING_VALUE = 1;
/**
*
* An error occurred that prevented this operation from starting/continuing.
*
*
* ERROR = 2;
*/
public static final int ERROR_VALUE = 2;
/**
*
* The operation is complete.
*
*
* DONE = 3;
*/
public static final int DONE_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
public static Status forNumber(int value) {
switch (value) {
case 0: return PENDING;
case 1: return SENDING;
case 2: return ERROR;
case 3: return DONE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Status> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.SendOperation.getDescriptor().getEnumTypes().get(0);
}
private static final Status[] VALUES = values();
public static Status valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.comms.SendOperation.Status)
}
private int operationCase_ = 0;
private java.lang.Object operation_;
public enum OperationCase
implements com.google.protobuf.Internal.EnumLite {
SMS(10),
EMAIL(11),
OPERATION_NOT_SET(0);
private final int value;
private OperationCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OperationCase valueOf(int value) {
return forNumber(value);
}
public static OperationCase forNumber(int value) {
switch (value) {
case 10: return SMS;
case 11: return EMAIL;
case 0: return OPERATION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public OperationCase
getOperationCase() {
return OperationCase.forNumber(
operationCase_);
}
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_FIELD_NUMBER = 2;
private int channel_;
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public int getChannelValue() {
return channel_;
}
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.GenericComms.Channel result = io.bloombox.schema.comms.GenericComms.Channel.valueOf(channel_);
return result == null ? io.bloombox.schema.comms.GenericComms.Channel.UNRECOGNIZED : result;
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation.Status getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.CommsTask.SendOperation.Status result = io.bloombox.schema.comms.CommsTask.SendOperation.Status.valueOf(status_);
return result == null ? io.bloombox.schema.comms.CommsTask.SendOperation.Status.UNRECOGNIZED : result;
}
public static final int DRY_RUN_FIELD_NUMBER = 4;
private boolean dryRun_;
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 4;
*/
public boolean getDryRun() {
return dryRun_;
}
public static final int SMS_FIELD_NUMBER = 10;
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public boolean hasSms() {
return operationCase_ == 10;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public io.bloombox.schema.comms.SMSComms.SMSTransmission getSms() {
if (operationCase_ == 10) {
return (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_;
}
return io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder getSmsOrBuilder() {
if (operationCase_ == 10) {
return (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_;
}
return io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
}
public static final int EMAIL_FIELD_NUMBER = 11;
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public boolean hasEmail() {
return operationCase_ == 11;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission getEmail() {
if (operationCase_ == 11) {
return (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_;
}
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getEmailOrBuilder() {
if (operationCase_ == 11) {
return (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_;
}
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
if (channel_ != io.bloombox.schema.comms.GenericComms.Channel.UNSPECIFIED.getNumber()) {
output.writeEnum(2, channel_);
}
if (status_ != io.bloombox.schema.comms.CommsTask.SendOperation.Status.PENDING.getNumber()) {
output.writeEnum(3, status_);
}
if (dryRun_ != false) {
output.writeBool(4, dryRun_);
}
if (operationCase_ == 10) {
output.writeMessage(10, (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_);
}
if (operationCase_ == 11) {
output.writeMessage(11, (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
if (channel_ != io.bloombox.schema.comms.GenericComms.Channel.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, channel_);
}
if (status_ != io.bloombox.schema.comms.CommsTask.SendOperation.Status.PENDING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (dryRun_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, dryRun_);
}
if (operationCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_);
}
if (operationCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.CommsTask.SendOperation)) {
return super.equals(obj);
}
io.bloombox.schema.comms.CommsTask.SendOperation other = (io.bloombox.schema.comms.CommsTask.SendOperation) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (channel_ != other.channel_) return false;
if (status_ != other.status_) return false;
if (getDryRun()
!= other.getDryRun()) return false;
if (!getOperationCase().equals(other.getOperationCase())) return false;
switch (operationCase_) {
case 10:
if (!getSms()
.equals(other.getSms())) return false;
break;
case 11:
if (!getEmail()
.equals(other.getEmail())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + channel_;
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + DRY_RUN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDryRun());
switch (operationCase_) {
case 10:
hash = (37 * hash) + SMS_FIELD_NUMBER;
hash = (53 * hash) + getSms().hashCode();
break;
case 11:
hash = (37 * hash) + EMAIL_FIELD_NUMBER;
hash = (53 * hash) + getEmail().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.SendOperation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.CommsTask.SendOperation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Send a batch of either SMS or email messages.
*
*
* Protobuf type {@code bloombox.comms.SendOperation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.SendOperation)
io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_SendOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_SendOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.SendOperation.class, io.bloombox.schema.comms.CommsTask.SendOperation.Builder.class);
}
// Construct using io.bloombox.schema.comms.CommsTask.SendOperation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
channel_ = 0;
status_ = 0;
dryRun_ = false;
operationCase_ = 0;
operation_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_SendOperation_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.SendOperation getDefaultInstanceForType() {
return io.bloombox.schema.comms.CommsTask.SendOperation.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.SendOperation build() {
io.bloombox.schema.comms.CommsTask.SendOperation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.SendOperation buildPartial() {
io.bloombox.schema.comms.CommsTask.SendOperation result = new io.bloombox.schema.comms.CommsTask.SendOperation(this);
result.uuid_ = uuid_;
result.channel_ = channel_;
result.status_ = status_;
result.dryRun_ = dryRun_;
if (operationCase_ == 10) {
if (smsBuilder_ == null) {
result.operation_ = operation_;
} else {
result.operation_ = smsBuilder_.build();
}
}
if (operationCase_ == 11) {
if (emailBuilder_ == null) {
result.operation_ = operation_;
} else {
result.operation_ = emailBuilder_.build();
}
}
result.operationCase_ = operationCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.CommsTask.SendOperation) {
return mergeFrom((io.bloombox.schema.comms.CommsTask.SendOperation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.CommsTask.SendOperation other) {
if (other == io.bloombox.schema.comms.CommsTask.SendOperation.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
if (other.channel_ != 0) {
setChannelValue(other.getChannelValue());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getDryRun() != false) {
setDryRun(other.getDryRun());
}
switch (other.getOperationCase()) {
case SMS: {
mergeSms(other.getSms());
break;
}
case EMAIL: {
mergeEmail(other.getEmail());
break;
}
case OPERATION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.CommsTask.SendOperation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.CommsTask.SendOperation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int operationCase_ = 0;
private java.lang.Object operation_;
public OperationCase
getOperationCase() {
return OperationCase.forNumber(
operationCase_);
}
public Builder clearOperation() {
operationCase_ = 0;
operation_ = null;
onChanged();
return this;
}
private java.lang.Object uuid_ = "";
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* Unique ID for this operation.
*
*
* string uuid = 1;
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
private int channel_ = 0;
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public int getChannelValue() {
return channel_;
}
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public Builder setChannelValue(int value) {
channel_ = value;
onChanged();
return this;
}
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.GenericComms.Channel result = io.bloombox.schema.comms.GenericComms.Channel.valueOf(channel_);
return result == null ? io.bloombox.schema.comms.GenericComms.Channel.UNRECOGNIZED : result;
}
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public Builder setChannel(io.bloombox.schema.comms.GenericComms.Channel value) {
if (value == null) {
throw new NullPointerException();
}
channel_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Channel for which this send operation is operating.
*
*
* .bloombox.comms.Channel channel = 2;
*/
public Builder clearChannel() {
channel_ = 0;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation.Status getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.CommsTask.SendOperation.Status result = io.bloombox.schema.comms.CommsTask.SendOperation.Status.valueOf(status_);
return result == null ? io.bloombox.schema.comms.CommsTask.SendOperation.Status.UNRECOGNIZED : result;
}
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public Builder setStatus(io.bloombox.schema.comms.CommsTask.SendOperation.Status value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status for this operation.
*
*
* .bloombox.comms.SendOperation.Status status = 3;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private boolean dryRun_ ;
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 4;
*/
public boolean getDryRun() {
return dryRun_;
}
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 4;
*/
public Builder setDryRun(boolean value) {
dryRun_ = value;
onChanged();
return this;
}
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 4;
*/
public Builder clearDryRun() {
dryRun_ = false;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.SMSComms.SMSTransmission, io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder, io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder> smsBuilder_;
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public boolean hasSms() {
return operationCase_ == 10;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public io.bloombox.schema.comms.SMSComms.SMSTransmission getSms() {
if (smsBuilder_ == null) {
if (operationCase_ == 10) {
return (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_;
}
return io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
} else {
if (operationCase_ == 10) {
return smsBuilder_.getMessage();
}
return io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
}
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public Builder setSms(io.bloombox.schema.comms.SMSComms.SMSTransmission value) {
if (smsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
operation_ = value;
onChanged();
} else {
smsBuilder_.setMessage(value);
}
operationCase_ = 10;
return this;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public Builder setSms(
io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder builderForValue) {
if (smsBuilder_ == null) {
operation_ = builderForValue.build();
onChanged();
} else {
smsBuilder_.setMessage(builderForValue.build());
}
operationCase_ = 10;
return this;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public Builder mergeSms(io.bloombox.schema.comms.SMSComms.SMSTransmission value) {
if (smsBuilder_ == null) {
if (operationCase_ == 10 &&
operation_ != io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance()) {
operation_ = io.bloombox.schema.comms.SMSComms.SMSTransmission.newBuilder((io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_)
.mergeFrom(value).buildPartial();
} else {
operation_ = value;
}
onChanged();
} else {
if (operationCase_ == 10) {
smsBuilder_.mergeFrom(value);
}
smsBuilder_.setMessage(value);
}
operationCase_ = 10;
return this;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public Builder clearSms() {
if (smsBuilder_ == null) {
if (operationCase_ == 10) {
operationCase_ = 0;
operation_ = null;
onChanged();
}
} else {
if (operationCase_ == 10) {
operationCase_ = 0;
operation_ = null;
}
smsBuilder_.clear();
}
return this;
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder getSmsBuilder() {
return getSmsFieldBuilder().getBuilder();
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
public io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder getSmsOrBuilder() {
if ((operationCase_ == 10) && (smsBuilder_ != null)) {
return smsBuilder_.getMessageOrBuilder();
} else {
if (operationCase_ == 10) {
return (io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_;
}
return io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
}
}
/**
*
* Batch of SMS send operations.
*
*
* .bloombox.comms.SMSTransmission sms = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.SMSComms.SMSTransmission, io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder, io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder>
getSmsFieldBuilder() {
if (smsBuilder_ == null) {
if (!(operationCase_ == 10)) {
operation_ = io.bloombox.schema.comms.SMSComms.SMSTransmission.getDefaultInstance();
}
smsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.SMSComms.SMSTransmission, io.bloombox.schema.comms.SMSComms.SMSTransmission.Builder, io.bloombox.schema.comms.SMSComms.SMSTransmissionOrBuilder>(
(io.bloombox.schema.comms.SMSComms.SMSTransmission) operation_,
getParentForChildren(),
isClean());
operation_ = null;
}
operationCase_ = 10;
onChanged();;
return smsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder> emailBuilder_;
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public boolean hasEmail() {
return operationCase_ == 11;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission getEmail() {
if (emailBuilder_ == null) {
if (operationCase_ == 11) {
return (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_;
}
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
} else {
if (operationCase_ == 11) {
return emailBuilder_.getMessage();
}
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public Builder setEmail(io.bloombox.schema.comms.EmailComms.EmailTransmission value) {
if (emailBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
operation_ = value;
onChanged();
} else {
emailBuilder_.setMessage(value);
}
operationCase_ = 11;
return this;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public Builder setEmail(
io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder builderForValue) {
if (emailBuilder_ == null) {
operation_ = builderForValue.build();
onChanged();
} else {
emailBuilder_.setMessage(builderForValue.build());
}
operationCase_ = 11;
return this;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public Builder mergeEmail(io.bloombox.schema.comms.EmailComms.EmailTransmission value) {
if (emailBuilder_ == null) {
if (operationCase_ == 11 &&
operation_ != io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance()) {
operation_ = io.bloombox.schema.comms.EmailComms.EmailTransmission.newBuilder((io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_)
.mergeFrom(value).buildPartial();
} else {
operation_ = value;
}
onChanged();
} else {
if (operationCase_ == 11) {
emailBuilder_.mergeFrom(value);
}
emailBuilder_.setMessage(value);
}
operationCase_ = 11;
return this;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public Builder clearEmail() {
if (emailBuilder_ == null) {
if (operationCase_ == 11) {
operationCase_ = 0;
operation_ = null;
onChanged();
}
} else {
if (operationCase_ == 11) {
operationCase_ = 0;
operation_ = null;
}
emailBuilder_.clear();
}
return this;
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder getEmailBuilder() {
return getEmailFieldBuilder().getBuilder();
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getEmailOrBuilder() {
if ((operationCase_ == 11) && (emailBuilder_ != null)) {
return emailBuilder_.getMessageOrBuilder();
} else {
if (operationCase_ == 11) {
return (io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_;
}
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
}
/**
*
* Batch of email send operations.
*
*
* .bloombox.comms.EmailTransmission email = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>
getEmailFieldBuilder() {
if (emailBuilder_ == null) {
if (!(operationCase_ == 11)) {
operation_ = io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
emailBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>(
(io.bloombox.schema.comms.EmailComms.EmailTransmission) operation_,
getParentForChildren(),
isClean());
operation_ = null;
}
operationCase_ = 11;
onChanged();;
return emailBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.SendOperation)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.SendOperation)
private static final io.bloombox.schema.comms.CommsTask.SendOperation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.CommsTask.SendOperation();
}
public static io.bloombox.schema.comms.CommsTask.SendOperation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SendOperation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SendOperation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.SendOperation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TriggerOperationOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.TriggerOperation)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
java.lang.String getUuid();
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUuidBytes();
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
java.lang.String getPartner();
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
com.google.protobuf.ByteString
getPartnerBytes();
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
java.lang.String getLocation();
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
com.google.protobuf.ByteString
getLocationBytes();
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
java.lang.String getCampaign();
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
com.google.protobuf.ByteString
getCampaignBytes();
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
boolean hasSubmitted();
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSubmitted();
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSubmittedOrBuilder();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
boolean hasNotBefore();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
java.util.List getChannelList();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
int getChannelCount();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
io.bloombox.schema.comms.GenericComms.Channel getChannel(int index);
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
java.util.List
getChannelValueList();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
int getChannelValue(int index);
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
java.util.List
getOpList();
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
io.bloombox.schema.comms.CommsTask.SendOperation getOp(int index);
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
int getOpCount();
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
java.util.List extends io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder>
getOpOrBuilderList();
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder getOpOrBuilder(
int index);
/**
*
* Set to `true` to perform all processing, but send nothing.
*
*
* bool dry_run = 9;
*/
boolean getDryRun();
}
/**
*
* Specifies an operation to merge campaign targeting parameters and trigger execution.
*
*
* Protobuf type {@code bloombox.comms.TriggerOperation}
*/
public static final class TriggerOperation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.TriggerOperation)
TriggerOperationOrBuilder {
private static final long serialVersionUID = 0L;
// Use TriggerOperation.newBuilder() to construct.
private TriggerOperation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TriggerOperation() {
uuid_ = "";
partner_ = "";
location_ = "";
campaign_ = "";
channel_ = java.util.Collections.emptyList();
op_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TriggerOperation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
partner_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
location_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
campaign_ = s;
break;
}
case 42: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (submitted_ != null) {
subBuilder = submitted_.toBuilder();
}
submitted_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(submitted_);
submitted_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (notBefore_ != null) {
subBuilder = notBefore_.toBuilder();
}
notBefore_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(notBefore_);
notBefore_ = subBuilder.buildPartial();
}
break;
}
case 56: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
channel_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
channel_.add(rawValue);
break;
}
case 58: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
channel_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
channel_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) != 0)) {
op_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
op_.add(
input.readMessage(io.bloombox.schema.comms.CommsTask.SendOperation.parser(), extensionRegistry));
break;
}
case 72: {
dryRun_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) != 0)) {
channel_ = java.util.Collections.unmodifiableList(channel_);
}
if (((mutable_bitField0_ & 0x00000080) != 0)) {
op_ = java.util.Collections.unmodifiableList(op_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_TriggerOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_TriggerOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.TriggerOperation.class, io.bloombox.schema.comms.CommsTask.TriggerOperation.Builder.class);
}
private int bitField0_;
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARTNER_FIELD_NUMBER = 2;
private volatile java.lang.Object partner_;
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public java.lang.String getPartner() {
java.lang.Object ref = partner_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partner_ = s;
return s;
}
}
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public com.google.protobuf.ByteString
getPartnerBytes() {
java.lang.Object ref = partner_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partner_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCATION_FIELD_NUMBER = 3;
private volatile java.lang.Object location_;
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
location_ = s;
return s;
}
}
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_FIELD_NUMBER = 4;
private volatile java.lang.Object campaign_;
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public java.lang.String getCampaign() {
java.lang.Object ref = campaign_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaign_ = s;
return s;
}
}
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public com.google.protobuf.ByteString
getCampaignBytes() {
java.lang.Object ref = campaign_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBMITTED_FIELD_NUMBER = 5;
private io.opencannabis.schema.temporal.TemporalInstant.Instant submitted_;
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public boolean hasSubmitted() {
return submitted_ != null;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSubmitted() {
return submitted_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : submitted_;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSubmittedOrBuilder() {
return getSubmitted();
}
public static final int NOT_BEFORE_FIELD_NUMBER = 6;
private io.opencannabis.schema.temporal.TemporalInstant.Instant notBefore_;
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public boolean hasNotBefore() {
return notBefore_ != null;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore() {
return notBefore_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder() {
return getNotBefore();
}
public static final int CHANNEL_FIELD_NUMBER = 7;
private java.util.List channel_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel> channel_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>() {
public io.bloombox.schema.comms.GenericComms.Channel convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.GenericComms.Channel result = io.bloombox.schema.comms.GenericComms.Channel.valueOf(from);
return result == null ? io.bloombox.schema.comms.GenericComms.Channel.UNRECOGNIZED : result;
}
};
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List getChannelList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>(channel_, channel_converter_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelCount() {
return channel_.size();
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel(int index) {
return channel_converter_.convert(channel_.get(index));
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List
getChannelValueList() {
return channel_;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelValue(int index) {
return channel_.get(index);
}
private int channelMemoizedSerializedSize;
public static final int OP_FIELD_NUMBER = 8;
private java.util.List op_;
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public java.util.List getOpList() {
return op_;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public java.util.List extends io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder>
getOpOrBuilderList() {
return op_;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public int getOpCount() {
return op_.size();
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation getOp(int index) {
return op_.get(index);
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder getOpOrBuilder(
int index) {
return op_.get(index);
}
public static final int DRY_RUN_FIELD_NUMBER = 9;
private boolean dryRun_;
/**
*
* Set to `true` to perform all processing, but send nothing.
*
*
* bool dry_run = 9;
*/
public boolean getDryRun() {
return dryRun_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
if (!getPartnerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, partner_);
}
if (!getLocationBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, location_);
}
if (!getCampaignBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, campaign_);
}
if (submitted_ != null) {
output.writeMessage(5, getSubmitted());
}
if (notBefore_ != null) {
output.writeMessage(6, getNotBefore());
}
if (getChannelList().size() > 0) {
output.writeUInt32NoTag(58);
output.writeUInt32NoTag(channelMemoizedSerializedSize);
}
for (int i = 0; i < channel_.size(); i++) {
output.writeEnumNoTag(channel_.get(i));
}
for (int i = 0; i < op_.size(); i++) {
output.writeMessage(8, op_.get(i));
}
if (dryRun_ != false) {
output.writeBool(9, dryRun_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
if (!getPartnerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, partner_);
}
if (!getLocationBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, location_);
}
if (!getCampaignBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, campaign_);
}
if (submitted_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getSubmitted());
}
if (notBefore_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getNotBefore());
}
{
int dataSize = 0;
for (int i = 0; i < channel_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(channel_.get(i));
}
size += dataSize;
if (!getChannelList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}channelMemoizedSerializedSize = dataSize;
}
for (int i = 0; i < op_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, op_.get(i));
}
if (dryRun_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, dryRun_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.CommsTask.TriggerOperation)) {
return super.equals(obj);
}
io.bloombox.schema.comms.CommsTask.TriggerOperation other = (io.bloombox.schema.comms.CommsTask.TriggerOperation) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (!getPartner()
.equals(other.getPartner())) return false;
if (!getLocation()
.equals(other.getLocation())) return false;
if (!getCampaign()
.equals(other.getCampaign())) return false;
if (hasSubmitted() != other.hasSubmitted()) return false;
if (hasSubmitted()) {
if (!getSubmitted()
.equals(other.getSubmitted())) return false;
}
if (hasNotBefore() != other.hasNotBefore()) return false;
if (hasNotBefore()) {
if (!getNotBefore()
.equals(other.getNotBefore())) return false;
}
if (!channel_.equals(other.channel_)) return false;
if (!getOpList()
.equals(other.getOpList())) return false;
if (getDryRun()
!= other.getDryRun()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (37 * hash) + PARTNER_FIELD_NUMBER;
hash = (53 * hash) + getPartner().hashCode();
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
hash = (37 * hash) + CAMPAIGN_FIELD_NUMBER;
hash = (53 * hash) + getCampaign().hashCode();
if (hasSubmitted()) {
hash = (37 * hash) + SUBMITTED_FIELD_NUMBER;
hash = (53 * hash) + getSubmitted().hashCode();
}
if (hasNotBefore()) {
hash = (37 * hash) + NOT_BEFORE_FIELD_NUMBER;
hash = (53 * hash) + getNotBefore().hashCode();
}
if (getChannelCount() > 0) {
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + channel_.hashCode();
}
if (getOpCount() > 0) {
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + getOpList().hashCode();
}
hash = (37 * hash) + DRY_RUN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDryRun());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.CommsTask.TriggerOperation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies an operation to merge campaign targeting parameters and trigger execution.
*
*
* Protobuf type {@code bloombox.comms.TriggerOperation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.TriggerOperation)
io.bloombox.schema.comms.CommsTask.TriggerOperationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_TriggerOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_TriggerOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.TriggerOperation.class, io.bloombox.schema.comms.CommsTask.TriggerOperation.Builder.class);
}
// Construct using io.bloombox.schema.comms.CommsTask.TriggerOperation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOpFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
partner_ = "";
location_ = "";
campaign_ = "";
if (submittedBuilder_ == null) {
submitted_ = null;
} else {
submitted_ = null;
submittedBuilder_ = null;
}
if (notBeforeBuilder_ == null) {
notBefore_ = null;
} else {
notBefore_ = null;
notBeforeBuilder_ = null;
}
channel_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
if (opBuilder_ == null) {
op_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
opBuilder_.clear();
}
dryRun_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_TriggerOperation_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.TriggerOperation getDefaultInstanceForType() {
return io.bloombox.schema.comms.CommsTask.TriggerOperation.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.TriggerOperation build() {
io.bloombox.schema.comms.CommsTask.TriggerOperation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.TriggerOperation buildPartial() {
io.bloombox.schema.comms.CommsTask.TriggerOperation result = new io.bloombox.schema.comms.CommsTask.TriggerOperation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.uuid_ = uuid_;
result.partner_ = partner_;
result.location_ = location_;
result.campaign_ = campaign_;
if (submittedBuilder_ == null) {
result.submitted_ = submitted_;
} else {
result.submitted_ = submittedBuilder_.build();
}
if (notBeforeBuilder_ == null) {
result.notBefore_ = notBefore_;
} else {
result.notBefore_ = notBeforeBuilder_.build();
}
if (((bitField0_ & 0x00000040) != 0)) {
channel_ = java.util.Collections.unmodifiableList(channel_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.channel_ = channel_;
if (opBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
op_ = java.util.Collections.unmodifiableList(op_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.op_ = op_;
} else {
result.op_ = opBuilder_.build();
}
result.dryRun_ = dryRun_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.CommsTask.TriggerOperation) {
return mergeFrom((io.bloombox.schema.comms.CommsTask.TriggerOperation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.CommsTask.TriggerOperation other) {
if (other == io.bloombox.schema.comms.CommsTask.TriggerOperation.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
if (!other.getPartner().isEmpty()) {
partner_ = other.partner_;
onChanged();
}
if (!other.getLocation().isEmpty()) {
location_ = other.location_;
onChanged();
}
if (!other.getCampaign().isEmpty()) {
campaign_ = other.campaign_;
onChanged();
}
if (other.hasSubmitted()) {
mergeSubmitted(other.getSubmitted());
}
if (other.hasNotBefore()) {
mergeNotBefore(other.getNotBefore());
}
if (!other.channel_.isEmpty()) {
if (channel_.isEmpty()) {
channel_ = other.channel_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureChannelIsMutable();
channel_.addAll(other.channel_);
}
onChanged();
}
if (opBuilder_ == null) {
if (!other.op_.isEmpty()) {
if (op_.isEmpty()) {
op_ = other.op_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureOpIsMutable();
op_.addAll(other.op_);
}
onChanged();
}
} else {
if (!other.op_.isEmpty()) {
if (opBuilder_.isEmpty()) {
opBuilder_.dispose();
opBuilder_ = null;
op_ = other.op_;
bitField0_ = (bitField0_ & ~0x00000080);
opBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOpFieldBuilder() : null;
} else {
opBuilder_.addAllMessages(other.op_);
}
}
}
if (other.getDryRun() != false) {
setDryRun(other.getDryRun());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.CommsTask.TriggerOperation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.CommsTask.TriggerOperation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uuid_ = "";
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* Unique ID for this campaign execution.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
private java.lang.Object partner_ = "";
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public java.lang.String getPartner() {
java.lang.Object ref = partner_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partner_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public com.google.protobuf.ByteString
getPartnerBytes() {
java.lang.Object ref = partner_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partner_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public Builder setPartner(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partner_ = value;
onChanged();
return this;
}
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public Builder clearPartner() {
partner_ = getDefaultInstance().getPartner();
onChanged();
return this;
}
/**
*
* Specifies the partner under which we are performing this operation.
*
*
* string partner = 2;
*/
public Builder setPartnerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partner_ = value;
onChanged();
return this;
}
private java.lang.Object location_ = "";
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
location_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public Builder setLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
location_ = value;
onChanged();
return this;
}
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public Builder clearLocation() {
location_ = getDefaultInstance().getLocation();
onChanged();
return this;
}
/**
*
* Specifies the location under which we are performing this operation.
*
*
* string location = 3;
*/
public Builder setLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
location_ = value;
onChanged();
return this;
}
private java.lang.Object campaign_ = "";
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public java.lang.String getCampaign() {
java.lang.Object ref = campaign_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaign_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public com.google.protobuf.ByteString
getCampaignBytes() {
java.lang.Object ref = campaign_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public Builder setCampaign(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
campaign_ = value;
onChanged();
return this;
}
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public Builder clearCampaign() {
campaign_ = getDefaultInstance().getCampaign();
onChanged();
return this;
}
/**
*
* Specifies the ID for the campaign that we wish to send.
*
*
* string campaign = 4;
*/
public Builder setCampaignBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
campaign_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant submitted_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> submittedBuilder_;
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public boolean hasSubmitted() {
return submittedBuilder_ != null || submitted_ != null;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSubmitted() {
if (submittedBuilder_ == null) {
return submitted_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : submitted_;
} else {
return submittedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public Builder setSubmitted(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (submittedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
submitted_ = value;
onChanged();
} else {
submittedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public Builder setSubmitted(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (submittedBuilder_ == null) {
submitted_ = builderForValue.build();
onChanged();
} else {
submittedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public Builder mergeSubmitted(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (submittedBuilder_ == null) {
if (submitted_ != null) {
submitted_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(submitted_).mergeFrom(value).buildPartial();
} else {
submitted_ = value;
}
onChanged();
} else {
submittedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public Builder clearSubmitted() {
if (submittedBuilder_ == null) {
submitted_ = null;
onChanged();
} else {
submitted_ = null;
submittedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSubmittedBuilder() {
onChanged();
return getSubmittedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSubmittedOrBuilder() {
if (submittedBuilder_ != null) {
return submittedBuilder_.getMessageOrBuilder();
} else {
return submitted_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : submitted_;
}
}
/**
*
* Timestamp for when this trigger was submitted.
*
*
* .opencannabis.temporal.Instant submitted = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSubmittedFieldBuilder() {
if (submittedBuilder_ == null) {
submittedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSubmitted(),
getParentForChildren(),
isClean());
submitted_ = null;
}
return submittedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant notBefore_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> notBeforeBuilder_;
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public boolean hasNotBefore() {
return notBeforeBuilder_ != null || notBefore_ != null;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore() {
if (notBeforeBuilder_ == null) {
return notBefore_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
} else {
return notBeforeBuilder_.getMessage();
}
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public Builder setNotBefore(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (notBeforeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
notBefore_ = value;
onChanged();
} else {
notBeforeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public Builder setNotBefore(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (notBeforeBuilder_ == null) {
notBefore_ = builderForValue.build();
onChanged();
} else {
notBeforeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public Builder mergeNotBefore(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (notBeforeBuilder_ == null) {
if (notBefore_ != null) {
notBefore_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(notBefore_).mergeFrom(value).buildPartial();
} else {
notBefore_ = value;
}
onChanged();
} else {
notBeforeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public Builder clearNotBefore() {
if (notBeforeBuilder_ == null) {
notBefore_ = null;
onChanged();
} else {
notBefore_ = null;
notBeforeBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getNotBeforeBuilder() {
onChanged();
return getNotBeforeFieldBuilder().getBuilder();
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder() {
if (notBeforeBuilder_ != null) {
return notBeforeBuilder_.getMessageOrBuilder();
} else {
return notBefore_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
}
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getNotBeforeFieldBuilder() {
if (notBeforeBuilder_ == null) {
notBeforeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getNotBefore(),
getParentForChildren(),
isClean());
notBefore_ = null;
}
return notBeforeBuilder_;
}
private java.util.List channel_ =
java.util.Collections.emptyList();
private void ensureChannelIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
channel_ = new java.util.ArrayList(channel_);
bitField0_ |= 0x00000040;
}
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List getChannelList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>(channel_, channel_converter_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelCount() {
return channel_.size();
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel(int index) {
return channel_converter_.convert(channel_.get(index));
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder setChannel(
int index, io.bloombox.schema.comms.GenericComms.Channel value) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelIsMutable();
channel_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addChannel(io.bloombox.schema.comms.GenericComms.Channel value) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelIsMutable();
channel_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addAllChannel(
java.lang.Iterable extends io.bloombox.schema.comms.GenericComms.Channel> values) {
ensureChannelIsMutable();
for (io.bloombox.schema.comms.GenericComms.Channel value : values) {
channel_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder clearChannel() {
channel_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List
getChannelValueList() {
return java.util.Collections.unmodifiableList(channel_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelValue(int index) {
return channel_.get(index);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder setChannelValue(
int index, int value) {
ensureChannelIsMutable();
channel_.set(index, value);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addChannelValue(int value) {
ensureChannelIsMutable();
channel_.add(value);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addAllChannelValue(
java.lang.Iterable values) {
ensureChannelIsMutable();
for (int value : values) {
channel_.add(value);
}
onChanged();
return this;
}
private java.util.List op_ =
java.util.Collections.emptyList();
private void ensureOpIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
op_ = new java.util.ArrayList(op_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.CommsTask.SendOperation, io.bloombox.schema.comms.CommsTask.SendOperation.Builder, io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder> opBuilder_;
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public java.util.List getOpList() {
if (opBuilder_ == null) {
return java.util.Collections.unmodifiableList(op_);
} else {
return opBuilder_.getMessageList();
}
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public int getOpCount() {
if (opBuilder_ == null) {
return op_.size();
} else {
return opBuilder_.getCount();
}
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation getOp(int index) {
if (opBuilder_ == null) {
return op_.get(index);
} else {
return opBuilder_.getMessage(index);
}
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder setOp(
int index, io.bloombox.schema.comms.CommsTask.SendOperation value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.set(index, value);
onChanged();
} else {
opBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder setOp(
int index, io.bloombox.schema.comms.CommsTask.SendOperation.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.set(index, builderForValue.build());
onChanged();
} else {
opBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder addOp(io.bloombox.schema.comms.CommsTask.SendOperation value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.add(value);
onChanged();
} else {
opBuilder_.addMessage(value);
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder addOp(
int index, io.bloombox.schema.comms.CommsTask.SendOperation value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.add(index, value);
onChanged();
} else {
opBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder addOp(
io.bloombox.schema.comms.CommsTask.SendOperation.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.add(builderForValue.build());
onChanged();
} else {
opBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder addOp(
int index, io.bloombox.schema.comms.CommsTask.SendOperation.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.add(index, builderForValue.build());
onChanged();
} else {
opBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder addAllOp(
java.lang.Iterable extends io.bloombox.schema.comms.CommsTask.SendOperation> values) {
if (opBuilder_ == null) {
ensureOpIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, op_);
onChanged();
} else {
opBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder clearOp() {
if (opBuilder_ == null) {
op_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
opBuilder_.clear();
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public Builder removeOp(int index) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.remove(index);
onChanged();
} else {
opBuilder_.remove(index);
}
return this;
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation.Builder getOpBuilder(
int index) {
return getOpFieldBuilder().getBuilder(index);
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder getOpOrBuilder(
int index) {
if (opBuilder_ == null) {
return op_.get(index); } else {
return opBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public java.util.List extends io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder>
getOpOrBuilderList() {
if (opBuilder_ != null) {
return opBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(op_);
}
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation.Builder addOpBuilder() {
return getOpFieldBuilder().addBuilder(
io.bloombox.schema.comms.CommsTask.SendOperation.getDefaultInstance());
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public io.bloombox.schema.comms.CommsTask.SendOperation.Builder addOpBuilder(
int index) {
return getOpFieldBuilder().addBuilder(
index, io.bloombox.schema.comms.CommsTask.SendOperation.getDefaultInstance());
}
/**
*
* Attached/constituent send operations.
*
*
* repeated .bloombox.comms.SendOperation op = 8;
*/
public java.util.List
getOpBuilderList() {
return getOpFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.CommsTask.SendOperation, io.bloombox.schema.comms.CommsTask.SendOperation.Builder, io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder>
getOpFieldBuilder() {
if (opBuilder_ == null) {
opBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.CommsTask.SendOperation, io.bloombox.schema.comms.CommsTask.SendOperation.Builder, io.bloombox.schema.comms.CommsTask.SendOperationOrBuilder>(
op_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
op_ = null;
}
return opBuilder_;
}
private boolean dryRun_ ;
/**
*
* Set to `true` to perform all processing, but send nothing.
*
*
* bool dry_run = 9;
*/
public boolean getDryRun() {
return dryRun_;
}
/**
*
* Set to `true` to perform all processing, but send nothing.
*
*
* bool dry_run = 9;
*/
public Builder setDryRun(boolean value) {
dryRun_ = value;
onChanged();
return this;
}
/**
*
* Set to `true` to perform all processing, but send nothing.
*
*
* bool dry_run = 9;
*/
public Builder clearDryRun() {
dryRun_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.TriggerOperation)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.TriggerOperation)
private static final io.bloombox.schema.comms.CommsTask.TriggerOperation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.CommsTask.TriggerOperation();
}
public static io.bloombox.schema.comms.CommsTask.TriggerOperation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TriggerOperation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TriggerOperation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.TriggerOperation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AdEngineOperationOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.AdEngineOperation)
com.google.protobuf.MessageOrBuilder {
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
java.lang.String getExecution();
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
com.google.protobuf.ByteString
getExecutionBytes();
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
boolean hasCampaign();
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
io.bloombox.schema.marketing.MarketingCampaign.Campaign getCampaign();
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder getCampaignOrBuilder();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
java.util.List getChannelList();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
int getChannelCount();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
io.bloombox.schema.comms.GenericComms.Channel getChannel(int index);
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
java.util.List
getChannelValueList();
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
int getChannelValue(int index);
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
boolean hasMembership();
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
io.bloombox.schema.identity.AppUser.ConsumerMembership getMembership();
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder getMembershipOrBuilder();
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
boolean hasUser();
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
io.bloombox.schema.identity.AppUserKey.UserKey getUser();
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
boolean hasNotBefore();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore();
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder();
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 11;
*/
boolean getDryRun();
}
/**
*
* Specifies a marketing engine operation, in which a campaign and a set of ad groups are matched to a user's profile,
* and enqueued for transmission if targeting parameters don't exclude their profile.
*
*
* Protobuf type {@code bloombox.comms.AdEngineOperation}
*/
public static final class AdEngineOperation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.AdEngineOperation)
AdEngineOperationOrBuilder {
private static final long serialVersionUID = 0L;
// Use AdEngineOperation.newBuilder() to construct.
private AdEngineOperation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AdEngineOperation() {
execution_ = "";
channel_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AdEngineOperation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
execution_ = s;
break;
}
case 18: {
io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder subBuilder = null;
if (campaign_ != null) {
subBuilder = campaign_.toBuilder();
}
campaign_ = input.readMessage(io.bloombox.schema.marketing.MarketingCampaign.Campaign.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(campaign_);
campaign_ = subBuilder.buildPartial();
}
break;
}
case 56: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
channel_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
channel_.add(rawValue);
break;
}
case 58: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
channel_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
channel_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 66: {
io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder subBuilder = null;
if (membership_ != null) {
subBuilder = membership_.toBuilder();
}
membership_ = input.readMessage(io.bloombox.schema.identity.AppUser.ConsumerMembership.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(membership_);
membership_ = subBuilder.buildPartial();
}
break;
}
case 74: {
io.bloombox.schema.identity.AppUserKey.UserKey.Builder subBuilder = null;
if (user_ != null) {
subBuilder = user_.toBuilder();
}
user_ = input.readMessage(io.bloombox.schema.identity.AppUserKey.UserKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(user_);
user_ = subBuilder.buildPartial();
}
break;
}
case 82: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (notBefore_ != null) {
subBuilder = notBefore_.toBuilder();
}
notBefore_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(notBefore_);
notBefore_ = subBuilder.buildPartial();
}
break;
}
case 88: {
dryRun_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
channel_ = java.util.Collections.unmodifiableList(channel_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_AdEngineOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_AdEngineOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.AdEngineOperation.class, io.bloombox.schema.comms.CommsTask.AdEngineOperation.Builder.class);
}
private int bitField0_;
public static final int EXECUTION_FIELD_NUMBER = 1;
private volatile java.lang.Object execution_;
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public java.lang.String getExecution() {
java.lang.Object ref = execution_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
execution_ = s;
return s;
}
}
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public com.google.protobuf.ByteString
getExecutionBytes() {
java.lang.Object ref = execution_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
execution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_FIELD_NUMBER = 2;
private io.bloombox.schema.marketing.MarketingCampaign.Campaign campaign_;
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public boolean hasCampaign() {
return campaign_ != null;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public io.bloombox.schema.marketing.MarketingCampaign.Campaign getCampaign() {
return campaign_ == null ? io.bloombox.schema.marketing.MarketingCampaign.Campaign.getDefaultInstance() : campaign_;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder getCampaignOrBuilder() {
return getCampaign();
}
public static final int CHANNEL_FIELD_NUMBER = 7;
private java.util.List channel_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel> channel_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>() {
public io.bloombox.schema.comms.GenericComms.Channel convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.GenericComms.Channel result = io.bloombox.schema.comms.GenericComms.Channel.valueOf(from);
return result == null ? io.bloombox.schema.comms.GenericComms.Channel.UNRECOGNIZED : result;
}
};
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List getChannelList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>(channel_, channel_converter_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelCount() {
return channel_.size();
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel(int index) {
return channel_converter_.convert(channel_.get(index));
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List
getChannelValueList() {
return channel_;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelValue(int index) {
return channel_.get(index);
}
private int channelMemoizedSerializedSize;
public static final int MEMBERSHIP_FIELD_NUMBER = 8;
private io.bloombox.schema.identity.AppUser.ConsumerMembership membership_;
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public boolean hasMembership() {
return membership_ != null;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public io.bloombox.schema.identity.AppUser.ConsumerMembership getMembership() {
return membership_ == null ? io.bloombox.schema.identity.AppUser.ConsumerMembership.getDefaultInstance() : membership_;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder getMembershipOrBuilder() {
return getMembership();
}
public static final int USER_FIELD_NUMBER = 9;
private io.bloombox.schema.identity.AppUserKey.UserKey user_;
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public boolean hasUser() {
return user_ != null;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
return user_ == null ? io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
return getUser();
}
public static final int NOT_BEFORE_FIELD_NUMBER = 10;
private io.opencannabis.schema.temporal.TemporalInstant.Instant notBefore_;
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public boolean hasNotBefore() {
return notBefore_ != null;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore() {
return notBefore_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder() {
return getNotBefore();
}
public static final int DRY_RUN_FIELD_NUMBER = 11;
private boolean dryRun_;
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 11;
*/
public boolean getDryRun() {
return dryRun_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getExecutionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, execution_);
}
if (campaign_ != null) {
output.writeMessage(2, getCampaign());
}
if (getChannelList().size() > 0) {
output.writeUInt32NoTag(58);
output.writeUInt32NoTag(channelMemoizedSerializedSize);
}
for (int i = 0; i < channel_.size(); i++) {
output.writeEnumNoTag(channel_.get(i));
}
if (membership_ != null) {
output.writeMessage(8, getMembership());
}
if (user_ != null) {
output.writeMessage(9, getUser());
}
if (notBefore_ != null) {
output.writeMessage(10, getNotBefore());
}
if (dryRun_ != false) {
output.writeBool(11, dryRun_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getExecutionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, execution_);
}
if (campaign_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCampaign());
}
{
int dataSize = 0;
for (int i = 0; i < channel_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(channel_.get(i));
}
size += dataSize;
if (!getChannelList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}channelMemoizedSerializedSize = dataSize;
}
if (membership_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getMembership());
}
if (user_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getUser());
}
if (notBefore_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getNotBefore());
}
if (dryRun_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, dryRun_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.CommsTask.AdEngineOperation)) {
return super.equals(obj);
}
io.bloombox.schema.comms.CommsTask.AdEngineOperation other = (io.bloombox.schema.comms.CommsTask.AdEngineOperation) obj;
if (!getExecution()
.equals(other.getExecution())) return false;
if (hasCampaign() != other.hasCampaign()) return false;
if (hasCampaign()) {
if (!getCampaign()
.equals(other.getCampaign())) return false;
}
if (!channel_.equals(other.channel_)) return false;
if (hasMembership() != other.hasMembership()) return false;
if (hasMembership()) {
if (!getMembership()
.equals(other.getMembership())) return false;
}
if (hasUser() != other.hasUser()) return false;
if (hasUser()) {
if (!getUser()
.equals(other.getUser())) return false;
}
if (hasNotBefore() != other.hasNotBefore()) return false;
if (hasNotBefore()) {
if (!getNotBefore()
.equals(other.getNotBefore())) return false;
}
if (getDryRun()
!= other.getDryRun()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + EXECUTION_FIELD_NUMBER;
hash = (53 * hash) + getExecution().hashCode();
if (hasCampaign()) {
hash = (37 * hash) + CAMPAIGN_FIELD_NUMBER;
hash = (53 * hash) + getCampaign().hashCode();
}
if (getChannelCount() > 0) {
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + channel_.hashCode();
}
if (hasMembership()) {
hash = (37 * hash) + MEMBERSHIP_FIELD_NUMBER;
hash = (53 * hash) + getMembership().hashCode();
}
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
if (hasNotBefore()) {
hash = (37 * hash) + NOT_BEFORE_FIELD_NUMBER;
hash = (53 * hash) + getNotBefore().hashCode();
}
hash = (37 * hash) + DRY_RUN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDryRun());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.CommsTask.AdEngineOperation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a marketing engine operation, in which a campaign and a set of ad groups are matched to a user's profile,
* and enqueued for transmission if targeting parameters don't exclude their profile.
*
*
* Protobuf type {@code bloombox.comms.AdEngineOperation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.AdEngineOperation)
io.bloombox.schema.comms.CommsTask.AdEngineOperationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_AdEngineOperation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_AdEngineOperation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.CommsTask.AdEngineOperation.class, io.bloombox.schema.comms.CommsTask.AdEngineOperation.Builder.class);
}
// Construct using io.bloombox.schema.comms.CommsTask.AdEngineOperation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
execution_ = "";
if (campaignBuilder_ == null) {
campaign_ = null;
} else {
campaign_ = null;
campaignBuilder_ = null;
}
channel_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
if (membershipBuilder_ == null) {
membership_ = null;
} else {
membership_ = null;
membershipBuilder_ = null;
}
if (userBuilder_ == null) {
user_ = null;
} else {
user_ = null;
userBuilder_ = null;
}
if (notBeforeBuilder_ == null) {
notBefore_ = null;
} else {
notBefore_ = null;
notBeforeBuilder_ = null;
}
dryRun_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.CommsTask.internal_static_bloombox_comms_AdEngineOperation_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.AdEngineOperation getDefaultInstanceForType() {
return io.bloombox.schema.comms.CommsTask.AdEngineOperation.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.AdEngineOperation build() {
io.bloombox.schema.comms.CommsTask.AdEngineOperation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.AdEngineOperation buildPartial() {
io.bloombox.schema.comms.CommsTask.AdEngineOperation result = new io.bloombox.schema.comms.CommsTask.AdEngineOperation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.execution_ = execution_;
if (campaignBuilder_ == null) {
result.campaign_ = campaign_;
} else {
result.campaign_ = campaignBuilder_.build();
}
if (((bitField0_ & 0x00000004) != 0)) {
channel_ = java.util.Collections.unmodifiableList(channel_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.channel_ = channel_;
if (membershipBuilder_ == null) {
result.membership_ = membership_;
} else {
result.membership_ = membershipBuilder_.build();
}
if (userBuilder_ == null) {
result.user_ = user_;
} else {
result.user_ = userBuilder_.build();
}
if (notBeforeBuilder_ == null) {
result.notBefore_ = notBefore_;
} else {
result.notBefore_ = notBeforeBuilder_.build();
}
result.dryRun_ = dryRun_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.CommsTask.AdEngineOperation) {
return mergeFrom((io.bloombox.schema.comms.CommsTask.AdEngineOperation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.CommsTask.AdEngineOperation other) {
if (other == io.bloombox.schema.comms.CommsTask.AdEngineOperation.getDefaultInstance()) return this;
if (!other.getExecution().isEmpty()) {
execution_ = other.execution_;
onChanged();
}
if (other.hasCampaign()) {
mergeCampaign(other.getCampaign());
}
if (!other.channel_.isEmpty()) {
if (channel_.isEmpty()) {
channel_ = other.channel_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureChannelIsMutable();
channel_.addAll(other.channel_);
}
onChanged();
}
if (other.hasMembership()) {
mergeMembership(other.getMembership());
}
if (other.hasUser()) {
mergeUser(other.getUser());
}
if (other.hasNotBefore()) {
mergeNotBefore(other.getNotBefore());
}
if (other.getDryRun() != false) {
setDryRun(other.getDryRun());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.CommsTask.AdEngineOperation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.CommsTask.AdEngineOperation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object execution_ = "";
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public java.lang.String getExecution() {
java.lang.Object ref = execution_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
execution_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public com.google.protobuf.ByteString
getExecutionBytes() {
java.lang.Object ref = execution_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
execution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public Builder setExecution(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
execution_ = value;
onChanged();
return this;
}
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public Builder clearExecution() {
execution_ = getDefaultInstance().getExecution();
onChanged();
return this;
}
/**
*
* ID for the execution run that created this operation.
*
*
* string execution = 1;
*/
public Builder setExecutionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
execution_ = value;
onChanged();
return this;
}
private io.bloombox.schema.marketing.MarketingCampaign.Campaign campaign_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.marketing.MarketingCampaign.Campaign, io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder, io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder> campaignBuilder_;
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public boolean hasCampaign() {
return campaignBuilder_ != null || campaign_ != null;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public io.bloombox.schema.marketing.MarketingCampaign.Campaign getCampaign() {
if (campaignBuilder_ == null) {
return campaign_ == null ? io.bloombox.schema.marketing.MarketingCampaign.Campaign.getDefaultInstance() : campaign_;
} else {
return campaignBuilder_.getMessage();
}
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public Builder setCampaign(io.bloombox.schema.marketing.MarketingCampaign.Campaign value) {
if (campaignBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
campaign_ = value;
onChanged();
} else {
campaignBuilder_.setMessage(value);
}
return this;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public Builder setCampaign(
io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder builderForValue) {
if (campaignBuilder_ == null) {
campaign_ = builderForValue.build();
onChanged();
} else {
campaignBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public Builder mergeCampaign(io.bloombox.schema.marketing.MarketingCampaign.Campaign value) {
if (campaignBuilder_ == null) {
if (campaign_ != null) {
campaign_ =
io.bloombox.schema.marketing.MarketingCampaign.Campaign.newBuilder(campaign_).mergeFrom(value).buildPartial();
} else {
campaign_ = value;
}
onChanged();
} else {
campaignBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public Builder clearCampaign() {
if (campaignBuilder_ == null) {
campaign_ = null;
onChanged();
} else {
campaign_ = null;
campaignBuilder_ = null;
}
return this;
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder getCampaignBuilder() {
onChanged();
return getCampaignFieldBuilder().getBuilder();
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
public io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder getCampaignOrBuilder() {
if (campaignBuilder_ != null) {
return campaignBuilder_.getMessageOrBuilder();
} else {
return campaign_ == null ?
io.bloombox.schema.marketing.MarketingCampaign.Campaign.getDefaultInstance() : campaign_;
}
}
/**
*
* Campaign that we are executing this operation against, with adgroups included.
*
*
* .bloombox.marketing.Campaign campaign = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.marketing.MarketingCampaign.Campaign, io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder, io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder>
getCampaignFieldBuilder() {
if (campaignBuilder_ == null) {
campaignBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.marketing.MarketingCampaign.Campaign, io.bloombox.schema.marketing.MarketingCampaign.Campaign.Builder, io.bloombox.schema.marketing.MarketingCampaign.CampaignOrBuilder>(
getCampaign(),
getParentForChildren(),
isClean());
campaign_ = null;
}
return campaignBuilder_;
}
private java.util.List channel_ =
java.util.Collections.emptyList();
private void ensureChannelIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
channel_ = new java.util.ArrayList(channel_);
bitField0_ |= 0x00000004;
}
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List getChannelList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.GenericComms.Channel>(channel_, channel_converter_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelCount() {
return channel_.size();
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public io.bloombox.schema.comms.GenericComms.Channel getChannel(int index) {
return channel_converter_.convert(channel_.get(index));
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder setChannel(
int index, io.bloombox.schema.comms.GenericComms.Channel value) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelIsMutable();
channel_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addChannel(io.bloombox.schema.comms.GenericComms.Channel value) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelIsMutable();
channel_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addAllChannel(
java.lang.Iterable extends io.bloombox.schema.comms.GenericComms.Channel> values) {
ensureChannelIsMutable();
for (io.bloombox.schema.comms.GenericComms.Channel value : values) {
channel_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder clearChannel() {
channel_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public java.util.List
getChannelValueList() {
return java.util.Collections.unmodifiableList(channel_);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public int getChannelValue(int index) {
return channel_.get(index);
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder setChannelValue(
int index, int value) {
ensureChannelIsMutable();
channel_.set(index, value);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addChannelValue(int value) {
ensureChannelIsMutable();
channel_.add(value);
onChanged();
return this;
}
/**
*
* Communication channels to execute for the subject campaign.
*
*
* repeated .bloombox.comms.Channel channel = 7;
*/
public Builder addAllChannelValue(
java.lang.Iterable values) {
ensureChannelIsMutable();
for (int value : values) {
channel_.add(value);
}
onChanged();
return this;
}
private io.bloombox.schema.identity.AppUser.ConsumerMembership membership_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerMembership, io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder, io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder> membershipBuilder_;
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public boolean hasMembership() {
return membershipBuilder_ != null || membership_ != null;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public io.bloombox.schema.identity.AppUser.ConsumerMembership getMembership() {
if (membershipBuilder_ == null) {
return membership_ == null ? io.bloombox.schema.identity.AppUser.ConsumerMembership.getDefaultInstance() : membership_;
} else {
return membershipBuilder_.getMessage();
}
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public Builder setMembership(io.bloombox.schema.identity.AppUser.ConsumerMembership value) {
if (membershipBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
membership_ = value;
onChanged();
} else {
membershipBuilder_.setMessage(value);
}
return this;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public Builder setMembership(
io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder builderForValue) {
if (membershipBuilder_ == null) {
membership_ = builderForValue.build();
onChanged();
} else {
membershipBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public Builder mergeMembership(io.bloombox.schema.identity.AppUser.ConsumerMembership value) {
if (membershipBuilder_ == null) {
if (membership_ != null) {
membership_ =
io.bloombox.schema.identity.AppUser.ConsumerMembership.newBuilder(membership_).mergeFrom(value).buildPartial();
} else {
membership_ = value;
}
onChanged();
} else {
membershipBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public Builder clearMembership() {
if (membershipBuilder_ == null) {
membership_ = null;
onChanged();
} else {
membership_ = null;
membershipBuilder_ = null;
}
return this;
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder getMembershipBuilder() {
onChanged();
return getMembershipFieldBuilder().getBuilder();
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
public io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder getMembershipOrBuilder() {
if (membershipBuilder_ != null) {
return membershipBuilder_.getMessageOrBuilder();
} else {
return membership_ == null ?
io.bloombox.schema.identity.AppUser.ConsumerMembership.getDefaultInstance() : membership_;
}
}
/**
*
* Membership record for this user at the partner location in question.
*
*
* .bloombox.identity.ConsumerMembership membership = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerMembership, io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder, io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder>
getMembershipFieldBuilder() {
if (membershipBuilder_ == null) {
membershipBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerMembership, io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder, io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder>(
getMembership(),
getParentForChildren(),
isClean());
membership_ = null;
}
return membershipBuilder_;
}
private io.bloombox.schema.identity.AppUserKey.UserKey user_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder> userBuilder_;
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public boolean hasUser() {
return userBuilder_ != null || user_ != null;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
if (userBuilder_ == null) {
return user_ == null ? io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
} else {
return userBuilder_.getMessage();
}
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public Builder setUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
user_ = value;
onChanged();
} else {
userBuilder_.setMessage(value);
}
return this;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public Builder setUser(
io.bloombox.schema.identity.AppUserKey.UserKey.Builder builderForValue) {
if (userBuilder_ == null) {
user_ = builderForValue.build();
onChanged();
} else {
userBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public Builder mergeUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (user_ != null) {
user_ =
io.bloombox.schema.identity.AppUserKey.UserKey.newBuilder(user_).mergeFrom(value).buildPartial();
} else {
user_ = value;
}
onChanged();
} else {
userBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public Builder clearUser() {
if (userBuilder_ == null) {
user_ = null;
onChanged();
} else {
user_ = null;
userBuilder_ = null;
}
return this;
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey.Builder getUserBuilder() {
onChanged();
return getUserFieldBuilder().getBuilder();
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
if (userBuilder_ != null) {
return userBuilder_.getMessageOrBuilder();
} else {
return user_ == null ?
io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
}
}
/**
*
* Key for the user's account.
*
*
* .bloombox.identity.UserKey user = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>
getUserFieldBuilder() {
if (userBuilder_ == null) {
userBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>(
getUser(),
getParentForChildren(),
isClean());
user_ = null;
}
return userBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant notBefore_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> notBeforeBuilder_;
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public boolean hasNotBefore() {
return notBeforeBuilder_ != null || notBefore_ != null;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getNotBefore() {
if (notBeforeBuilder_ == null) {
return notBefore_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
} else {
return notBeforeBuilder_.getMessage();
}
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public Builder setNotBefore(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (notBeforeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
notBefore_ = value;
onChanged();
} else {
notBeforeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public Builder setNotBefore(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (notBeforeBuilder_ == null) {
notBefore_ = builderForValue.build();
onChanged();
} else {
notBeforeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public Builder mergeNotBefore(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (notBeforeBuilder_ == null) {
if (notBefore_ != null) {
notBefore_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(notBefore_).mergeFrom(value).buildPartial();
} else {
notBefore_ = value;
}
onChanged();
} else {
notBeforeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public Builder clearNotBefore() {
if (notBeforeBuilder_ == null) {
notBefore_ = null;
onChanged();
} else {
notBefore_ = null;
notBeforeBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getNotBeforeBuilder() {
onChanged();
return getNotBeforeFieldBuilder().getBuilder();
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getNotBeforeOrBuilder() {
if (notBeforeBuilder_ != null) {
return notBeforeBuilder_.getMessageOrBuilder();
} else {
return notBefore_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : notBefore_;
}
}
/**
*
* Timestamp for the minimum limit for beginning campaign execution.
*
*
* .opencannabis.temporal.Instant not_before = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getNotBeforeFieldBuilder() {
if (notBeforeBuilder_ == null) {
notBeforeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getNotBefore(),
getParentForChildren(),
isClean());
notBefore_ = null;
}
return notBeforeBuilder_;
}
private boolean dryRun_ ;
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 11;
*/
public boolean getDryRun() {
return dryRun_;
}
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 11;
*/
public Builder setDryRun(boolean value) {
dryRun_ = value;
onChanged();
return this;
}
/**
*
* Dry-run debug flag.
*
*
* bool dry_run = 11;
*/
public Builder clearDryRun() {
dryRun_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.AdEngineOperation)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.AdEngineOperation)
private static final io.bloombox.schema.comms.CommsTask.AdEngineOperation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.CommsTask.AdEngineOperation();
}
public static io.bloombox.schema.comms.CommsTask.AdEngineOperation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AdEngineOperation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AdEngineOperation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.CommsTask.AdEngineOperation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_SendOperation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_SendOperation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_TriggerOperation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_TriggerOperation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_AdEngineOperation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_AdEngineOperation_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\025comms/CommsTask.proto\022\016bloombox.comms\032" +
"\024core/Datamodel.proto\032\017comms/SMS.proto\032\021" +
"comms/Email.proto\032\021comms/Comms.proto\032\023id" +
"entity/User.proto\032\026identity/UserKey.prot" +
"o\032\030marketing/Campaign.proto\032\026temporal/In" +
"stant.proto\"\311\002\n\rSendOperation\022\014\n\004uuid\030\001 " +
"\001(\t\022(\n\007channel\030\002 \001(\0162\027.bloombox.comms.Ch" +
"annel\0224\n\006status\030\003 \001(\0162$.bloombox.comms.S" +
"endOperation.Status\022\017\n\007dry_run\030\004 \001(\010\022.\n\003" +
"sms\030\n \001(\0132\037.bloombox.comms.SMSTransmissi" +
"onH\000\0222\n\005email\030\013 \001(\0132!.bloombox.comms.Ema" +
"ilTransmissionH\000\"7\n\006Status\022\013\n\007PENDING\020\000\022" +
"\013\n\007SENDING\020\001\022\t\n\005ERROR\020\002\022\010\n\004DONE\020\003:\017\202\367\002\013\010" +
"\001\022\007batchesB\013\n\toperation\"\276\002\n\020TriggerOpera" +
"tion\022\024\n\004uuid\030\001 \001(\tB\006\302\265\003\002\010\002\022\017\n\007partner\030\002 " +
"\001(\t\022\020\n\010location\030\003 \001(\t\022\020\n\010campaign\030\004 \001(\t\022" +
"1\n\tsubmitted\030\005 \001(\0132\036.opencannabis.tempor" +
"al.Instant\0222\n\nnot_before\030\006 \001(\0132\036.opencan" +
"nabis.temporal.Instant\022(\n\007channel\030\007 \003(\0162" +
"\027.bloombox.comms.Channel\022)\n\002op\030\010 \003(\0132\035.b" +
"loombox.comms.SendOperation\022\017\n\007dry_run\030\t" +
" \001(\010:\022\202\367\002\016\010\001\022\nexecutions\"\252\002\n\021AdEngineOpe" +
"ration\022\021\n\texecution\030\001 \001(\t\022.\n\010campaign\030\002 " +
"\001(\0132\034.bloombox.marketing.Campaign\022(\n\007cha" +
"nnel\030\007 \003(\0162\027.bloombox.comms.Channel\0229\n\nm" +
"embership\030\010 \001(\0132%.bloombox.identity.Cons" +
"umerMembership\022(\n\004user\030\t \001(\0132\032.bloombox." +
"identity.UserKey\0222\n\nnot_before\030\n \001(\0132\036.o" +
"pencannabis.temporal.Instant\022\017\n\007dry_run\030" +
"\013 \001(\010B/\n\030io.bloombox.schema.commsB\tComms" +
"TaskH\001P\000\242\002\003BBSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.bloombox.schema.comms.SMSComms.getDescriptor(),
io.bloombox.schema.comms.EmailComms.getDescriptor(),
io.bloombox.schema.comms.GenericComms.getDescriptor(),
io.bloombox.schema.identity.AppUser.getDescriptor(),
io.bloombox.schema.identity.AppUserKey.getDescriptor(),
io.bloombox.schema.marketing.MarketingCampaign.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
}, assigner);
internal_static_bloombox_comms_SendOperation_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_comms_SendOperation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_SendOperation_descriptor,
new java.lang.String[] { "Uuid", "Channel", "Status", "DryRun", "Sms", "Email", "Operation", });
internal_static_bloombox_comms_TriggerOperation_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_comms_TriggerOperation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_TriggerOperation_descriptor,
new java.lang.String[] { "Uuid", "Partner", "Location", "Campaign", "Submitted", "NotBefore", "Channel", "Op", "DryRun", });
internal_static_bloombox_comms_AdEngineOperation_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_bloombox_comms_AdEngineOperation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_AdEngineOperation_descriptor,
new java.lang.String[] { "Execution", "Campaign", "Channel", "Membership", "User", "NotBefore", "DryRun", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.db);
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.bloombox.schema.comms.SMSComms.getDescriptor();
io.bloombox.schema.comms.EmailComms.getDescriptor();
io.bloombox.schema.comms.GenericComms.getDescriptor();
io.bloombox.schema.identity.AppUser.getDescriptor();
io.bloombox.schema.identity.AppUserKey.getDescriptor();
io.bloombox.schema.marketing.MarketingCampaign.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy