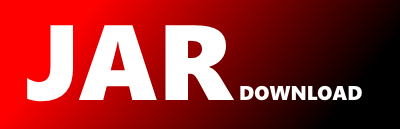
io.bloombox.schema.comms.EmailComms Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: comms/Email.proto
package io.bloombox.schema.comms;
public final class EmailComms {
private EmailComms() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface EmailContentOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailContent)
com.google.protobuf.MessageOrBuilder {
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
java.lang.String getSubject();
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
com.google.protobuf.ByteString
getSubjectBytes();
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
java.util.List
getContentList();
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
io.opencannabis.schema.content.GenericContent.Content getContent(int index);
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
int getContentCount();
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
java.util.List extends io.opencannabis.schema.content.GenericContent.ContentOrBuilder>
getContentOrBuilderList();
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
io.opencannabis.schema.content.GenericContent.ContentOrBuilder getContentOrBuilder(
int index);
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
java.util.List
getAttachmentList();
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
io.opencannabis.schema.media.MediaItemKey.MediaKey getAttachment(int index);
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
int getAttachmentCount();
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
java.util.List extends io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder>
getAttachmentOrBuilderList();
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder getAttachmentOrBuilder(
int index);
}
/**
*
* Specifies email content for a given ad group.
*
*
* Protobuf type {@code bloombox.comms.EmailContent}
*/
public static final class EmailContent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailContent)
EmailContentOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailContent.newBuilder() to construct.
private EmailContent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailContent() {
subject_ = "";
content_ = java.util.Collections.emptyList();
attachment_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailContent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
subject_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
content_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
content_.add(
input.readMessage(io.opencannabis.schema.content.GenericContent.Content.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
attachment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
attachment_.add(
input.readMessage(io.opencannabis.schema.media.MediaItemKey.MediaKey.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
content_ = java.util.Collections.unmodifiableList(content_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
attachment_ = java.util.Collections.unmodifiableList(attachment_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailContent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailContent.class, io.bloombox.schema.comms.EmailComms.EmailContent.Builder.class);
}
private int bitField0_;
public static final int SUBJECT_FIELD_NUMBER = 1;
private volatile java.lang.Object subject_;
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public java.lang.String getSubject() {
java.lang.Object ref = subject_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subject_ = s;
return s;
}
}
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public com.google.protobuf.ByteString
getSubjectBytes() {
java.lang.Object ref = subject_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subject_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTENT_FIELD_NUMBER = 2;
private java.util.List content_;
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public java.util.List getContentList() {
return content_;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public java.util.List extends io.opencannabis.schema.content.GenericContent.ContentOrBuilder>
getContentOrBuilderList() {
return content_;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public int getContentCount() {
return content_.size();
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.Content getContent(int index) {
return content_.get(index);
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.ContentOrBuilder getContentOrBuilder(
int index) {
return content_.get(index);
}
public static final int ATTACHMENT_FIELD_NUMBER = 3;
private java.util.List attachment_;
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public java.util.List getAttachmentList() {
return attachment_;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public java.util.List extends io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder>
getAttachmentOrBuilderList() {
return attachment_;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public int getAttachmentCount() {
return attachment_.size();
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKey getAttachment(int index) {
return attachment_.get(index);
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder getAttachmentOrBuilder(
int index) {
return attachment_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getSubjectBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, subject_);
}
for (int i = 0; i < content_.size(); i++) {
output.writeMessage(2, content_.get(i));
}
for (int i = 0; i < attachment_.size(); i++) {
output.writeMessage(3, attachment_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getSubjectBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, subject_);
}
for (int i = 0; i < content_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, content_.get(i));
}
for (int i = 0; i < attachment_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, attachment_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailContent)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailContent other = (io.bloombox.schema.comms.EmailComms.EmailContent) obj;
if (!getSubject()
.equals(other.getSubject())) return false;
if (!getContentList()
.equals(other.getContentList())) return false;
if (!getAttachmentList()
.equals(other.getAttachmentList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SUBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSubject().hashCode();
if (getContentCount() > 0) {
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContentList().hashCode();
}
if (getAttachmentCount() > 0) {
hash = (37 * hash) + ATTACHMENT_FIELD_NUMBER;
hash = (53 * hash) + getAttachmentList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailContent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailContent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies email content for a given ad group.
*
*
* Protobuf type {@code bloombox.comms.EmailContent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailContent)
io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailContent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailContent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailContent.class, io.bloombox.schema.comms.EmailComms.EmailContent.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailContent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getContentFieldBuilder();
getAttachmentFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
subject_ = "";
if (contentBuilder_ == null) {
content_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
contentBuilder_.clear();
}
if (attachmentBuilder_ == null) {
attachment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
attachmentBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailContent_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailContent getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailContent.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailContent build() {
io.bloombox.schema.comms.EmailComms.EmailContent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailContent buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailContent result = new io.bloombox.schema.comms.EmailComms.EmailContent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.subject_ = subject_;
if (contentBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
content_ = java.util.Collections.unmodifiableList(content_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.content_ = content_;
} else {
result.content_ = contentBuilder_.build();
}
if (attachmentBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
attachment_ = java.util.Collections.unmodifiableList(attachment_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.attachment_ = attachment_;
} else {
result.attachment_ = attachmentBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailContent) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailContent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailContent other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailContent.getDefaultInstance()) return this;
if (!other.getSubject().isEmpty()) {
subject_ = other.subject_;
onChanged();
}
if (contentBuilder_ == null) {
if (!other.content_.isEmpty()) {
if (content_.isEmpty()) {
content_ = other.content_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureContentIsMutable();
content_.addAll(other.content_);
}
onChanged();
}
} else {
if (!other.content_.isEmpty()) {
if (contentBuilder_.isEmpty()) {
contentBuilder_.dispose();
contentBuilder_ = null;
content_ = other.content_;
bitField0_ = (bitField0_ & ~0x00000002);
contentBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getContentFieldBuilder() : null;
} else {
contentBuilder_.addAllMessages(other.content_);
}
}
}
if (attachmentBuilder_ == null) {
if (!other.attachment_.isEmpty()) {
if (attachment_.isEmpty()) {
attachment_ = other.attachment_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAttachmentIsMutable();
attachment_.addAll(other.attachment_);
}
onChanged();
}
} else {
if (!other.attachment_.isEmpty()) {
if (attachmentBuilder_.isEmpty()) {
attachmentBuilder_.dispose();
attachmentBuilder_ = null;
attachment_ = other.attachment_;
bitField0_ = (bitField0_ & ~0x00000004);
attachmentBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAttachmentFieldBuilder() : null;
} else {
attachmentBuilder_.addAllMessages(other.attachment_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailContent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailContent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object subject_ = "";
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public java.lang.String getSubject() {
java.lang.Object ref = subject_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subject_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public com.google.protobuf.ByteString
getSubjectBytes() {
java.lang.Object ref = subject_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subject_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public Builder setSubject(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
subject_ = value;
onChanged();
return this;
}
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public Builder clearSubject() {
subject_ = getDefaultInstance().getSubject();
onChanged();
return this;
}
/**
*
* Email subject line.
*
*
* string subject = 1;
*/
public Builder setSubjectBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
subject_ = value;
onChanged();
return this;
}
private java.util.List content_ =
java.util.Collections.emptyList();
private void ensureContentIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
content_ = new java.util.ArrayList(content_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.content.GenericContent.Content, io.opencannabis.schema.content.GenericContent.Content.Builder, io.opencannabis.schema.content.GenericContent.ContentOrBuilder> contentBuilder_;
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public java.util.List getContentList() {
if (contentBuilder_ == null) {
return java.util.Collections.unmodifiableList(content_);
} else {
return contentBuilder_.getMessageList();
}
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public int getContentCount() {
if (contentBuilder_ == null) {
return content_.size();
} else {
return contentBuilder_.getCount();
}
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.Content getContent(int index) {
if (contentBuilder_ == null) {
return content_.get(index);
} else {
return contentBuilder_.getMessage(index);
}
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder setContent(
int index, io.opencannabis.schema.content.GenericContent.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.set(index, value);
onChanged();
} else {
contentBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder setContent(
int index, io.opencannabis.schema.content.GenericContent.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.set(index, builderForValue.build());
onChanged();
} else {
contentBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder addContent(io.opencannabis.schema.content.GenericContent.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.add(value);
onChanged();
} else {
contentBuilder_.addMessage(value);
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder addContent(
int index, io.opencannabis.schema.content.GenericContent.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.add(index, value);
onChanged();
} else {
contentBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder addContent(
io.opencannabis.schema.content.GenericContent.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.add(builderForValue.build());
onChanged();
} else {
contentBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder addContent(
int index, io.opencannabis.schema.content.GenericContent.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.add(index, builderForValue.build());
onChanged();
} else {
contentBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder addAllContent(
java.lang.Iterable extends io.opencannabis.schema.content.GenericContent.Content> values) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, content_);
onChanged();
} else {
contentBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder clearContent() {
if (contentBuilder_ == null) {
content_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
contentBuilder_.clear();
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public Builder removeContent(int index) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.remove(index);
onChanged();
} else {
contentBuilder_.remove(index);
}
return this;
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.Content.Builder getContentBuilder(
int index) {
return getContentFieldBuilder().getBuilder(index);
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.ContentOrBuilder getContentOrBuilder(
int index) {
if (contentBuilder_ == null) {
return content_.get(index); } else {
return contentBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public java.util.List extends io.opencannabis.schema.content.GenericContent.ContentOrBuilder>
getContentOrBuilderList() {
if (contentBuilder_ != null) {
return contentBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(content_);
}
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.Content.Builder addContentBuilder() {
return getContentFieldBuilder().addBuilder(
io.opencannabis.schema.content.GenericContent.Content.getDefaultInstance());
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public io.opencannabis.schema.content.GenericContent.Content.Builder addContentBuilder(
int index) {
return getContentFieldBuilder().addBuilder(
index, io.opencannabis.schema.content.GenericContent.Content.getDefaultInstance());
}
/**
*
* Email content.
*
*
* repeated .opencannabis.content.Content content = 2;
*/
public java.util.List
getContentBuilderList() {
return getContentFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.content.GenericContent.Content, io.opencannabis.schema.content.GenericContent.Content.Builder, io.opencannabis.schema.content.GenericContent.ContentOrBuilder>
getContentFieldBuilder() {
if (contentBuilder_ == null) {
contentBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.content.GenericContent.Content, io.opencannabis.schema.content.GenericContent.Content.Builder, io.opencannabis.schema.content.GenericContent.ContentOrBuilder>(
content_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
content_ = null;
}
return contentBuilder_;
}
private java.util.List attachment_ =
java.util.Collections.emptyList();
private void ensureAttachmentIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
attachment_ = new java.util.ArrayList(attachment_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.media.MediaItemKey.MediaKey, io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder, io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder> attachmentBuilder_;
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public java.util.List getAttachmentList() {
if (attachmentBuilder_ == null) {
return java.util.Collections.unmodifiableList(attachment_);
} else {
return attachmentBuilder_.getMessageList();
}
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public int getAttachmentCount() {
if (attachmentBuilder_ == null) {
return attachment_.size();
} else {
return attachmentBuilder_.getCount();
}
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKey getAttachment(int index) {
if (attachmentBuilder_ == null) {
return attachment_.get(index);
} else {
return attachmentBuilder_.getMessage(index);
}
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder setAttachment(
int index, io.opencannabis.schema.media.MediaItemKey.MediaKey value) {
if (attachmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttachmentIsMutable();
attachment_.set(index, value);
onChanged();
} else {
attachmentBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder setAttachment(
int index, io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder builderForValue) {
if (attachmentBuilder_ == null) {
ensureAttachmentIsMutable();
attachment_.set(index, builderForValue.build());
onChanged();
} else {
attachmentBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder addAttachment(io.opencannabis.schema.media.MediaItemKey.MediaKey value) {
if (attachmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttachmentIsMutable();
attachment_.add(value);
onChanged();
} else {
attachmentBuilder_.addMessage(value);
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder addAttachment(
int index, io.opencannabis.schema.media.MediaItemKey.MediaKey value) {
if (attachmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAttachmentIsMutable();
attachment_.add(index, value);
onChanged();
} else {
attachmentBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder addAttachment(
io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder builderForValue) {
if (attachmentBuilder_ == null) {
ensureAttachmentIsMutable();
attachment_.add(builderForValue.build());
onChanged();
} else {
attachmentBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder addAttachment(
int index, io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder builderForValue) {
if (attachmentBuilder_ == null) {
ensureAttachmentIsMutable();
attachment_.add(index, builderForValue.build());
onChanged();
} else {
attachmentBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder addAllAttachment(
java.lang.Iterable extends io.opencannabis.schema.media.MediaItemKey.MediaKey> values) {
if (attachmentBuilder_ == null) {
ensureAttachmentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, attachment_);
onChanged();
} else {
attachmentBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder clearAttachment() {
if (attachmentBuilder_ == null) {
attachment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
attachmentBuilder_.clear();
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public Builder removeAttachment(int index) {
if (attachmentBuilder_ == null) {
ensureAttachmentIsMutable();
attachment_.remove(index);
onChanged();
} else {
attachmentBuilder_.remove(index);
}
return this;
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder getAttachmentBuilder(
int index) {
return getAttachmentFieldBuilder().getBuilder(index);
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder getAttachmentOrBuilder(
int index) {
if (attachmentBuilder_ == null) {
return attachment_.get(index); } else {
return attachmentBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public java.util.List extends io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder>
getAttachmentOrBuilderList() {
if (attachmentBuilder_ != null) {
return attachmentBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(attachment_);
}
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder addAttachmentBuilder() {
return getAttachmentFieldBuilder().addBuilder(
io.opencannabis.schema.media.MediaItemKey.MediaKey.getDefaultInstance());
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder addAttachmentBuilder(
int index) {
return getAttachmentFieldBuilder().addBuilder(
index, io.opencannabis.schema.media.MediaItemKey.MediaKey.getDefaultInstance());
}
/**
*
* Email attachments.
*
*
* repeated .opencannabis.media.MediaKey attachment = 3;
*/
public java.util.List
getAttachmentBuilderList() {
return getAttachmentFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.media.MediaItemKey.MediaKey, io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder, io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder>
getAttachmentFieldBuilder() {
if (attachmentBuilder_ == null) {
attachmentBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.media.MediaItemKey.MediaKey, io.opencannabis.schema.media.MediaItemKey.MediaKey.Builder, io.opencannabis.schema.media.MediaItemKey.MediaKeyOrBuilder>(
attachment_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
attachment_ = null;
}
return attachmentBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailContent)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailContent)
private static final io.bloombox.schema.comms.EmailComms.EmailContent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailContent();
}
public static io.bloombox.schema.comms.EmailComms.EmailContent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailContent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailContent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailContent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMessage)
com.google.protobuf.MessageOrBuilder {
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
boolean hasSender();
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getSender();
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getSenderOrBuilder();
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
boolean hasRecipient();
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getRecipient();
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getRecipientOrBuilder();
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
boolean hasContent();
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
io.bloombox.schema.comms.EmailComms.EmailContent getContent();
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder getContentOrBuilder();
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
boolean hasReplyTo();
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getReplyTo();
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getReplyToOrBuilder();
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
java.util.List
getCcList();
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getCc(int index);
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
int getCcCount();
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getCcOrBuilderList();
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getCcOrBuilder(
int index);
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
java.util.List
getBccList();
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getBcc(int index);
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
int getBccCount();
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getBccOrBuilderList();
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getBccOrBuilder(
int index);
}
/**
*
* Specifies the structure of an individual email message.
*
*
* Protobuf type {@code bloombox.comms.EmailMessage}
*/
public static final class EmailMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMessage)
EmailMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailMessage.newBuilder() to construct.
private EmailMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailMessage() {
cc_ = java.util.Collections.emptyList();
bcc_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder subBuilder = null;
if (sender_ != null) {
subBuilder = sender_.toBuilder();
}
sender_ = input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(sender_);
sender_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder subBuilder = null;
if (recipient_ != null) {
subBuilder = recipient_.toBuilder();
}
recipient_ = input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(recipient_);
recipient_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.bloombox.schema.comms.EmailComms.EmailContent.Builder subBuilder = null;
if (content_ != null) {
subBuilder = content_.toBuilder();
}
content_ = input.readMessage(io.bloombox.schema.comms.EmailComms.EmailContent.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(content_);
content_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder subBuilder = null;
if (replyTo_ != null) {
subBuilder = replyTo_.toBuilder();
}
replyTo_ = input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(replyTo_);
replyTo_ = subBuilder.buildPartial();
}
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
cc_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
cc_.add(
input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
bcc_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
bcc_.add(
input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) != 0)) {
cc_ = java.util.Collections.unmodifiableList(cc_);
}
if (((mutable_bitField0_ & 0x00000020) != 0)) {
bcc_ = java.util.Collections.unmodifiableList(bcc_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMessage.class, io.bloombox.schema.comms.EmailComms.EmailMessage.Builder.class);
}
private int bitField0_;
public static final int SENDER_FIELD_NUMBER = 1;
private io.opencannabis.schema.contact.ContactEmail.EmailAddress sender_;
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public boolean hasSender() {
return sender_ != null;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getSender() {
return sender_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : sender_;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getSenderOrBuilder() {
return getSender();
}
public static final int RECIPIENT_FIELD_NUMBER = 2;
private io.opencannabis.schema.contact.ContactEmail.EmailAddress recipient_;
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public boolean hasRecipient() {
return recipient_ != null;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getRecipient() {
return recipient_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : recipient_;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getRecipientOrBuilder() {
return getRecipient();
}
public static final int CONTENT_FIELD_NUMBER = 3;
private io.bloombox.schema.comms.EmailComms.EmailContent content_;
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public boolean hasContent() {
return content_ != null;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public io.bloombox.schema.comms.EmailComms.EmailContent getContent() {
return content_ == null ? io.bloombox.schema.comms.EmailComms.EmailContent.getDefaultInstance() : content_;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder getContentOrBuilder() {
return getContent();
}
public static final int REPLY_TO_FIELD_NUMBER = 4;
private io.opencannabis.schema.contact.ContactEmail.EmailAddress replyTo_;
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public boolean hasReplyTo() {
return replyTo_ != null;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getReplyTo() {
return replyTo_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : replyTo_;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getReplyToOrBuilder() {
return getReplyTo();
}
public static final int CC_FIELD_NUMBER = 5;
private java.util.List cc_;
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public java.util.List getCcList() {
return cc_;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getCcOrBuilderList() {
return cc_;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public int getCcCount() {
return cc_.size();
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getCc(int index) {
return cc_.get(index);
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getCcOrBuilder(
int index) {
return cc_.get(index);
}
public static final int BCC_FIELD_NUMBER = 6;
private java.util.List bcc_;
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public java.util.List getBccList() {
return bcc_;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getBccOrBuilderList() {
return bcc_;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public int getBccCount() {
return bcc_.size();
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getBcc(int index) {
return bcc_.get(index);
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getBccOrBuilder(
int index) {
return bcc_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (sender_ != null) {
output.writeMessage(1, getSender());
}
if (recipient_ != null) {
output.writeMessage(2, getRecipient());
}
if (content_ != null) {
output.writeMessage(3, getContent());
}
if (replyTo_ != null) {
output.writeMessage(4, getReplyTo());
}
for (int i = 0; i < cc_.size(); i++) {
output.writeMessage(5, cc_.get(i));
}
for (int i = 0; i < bcc_.size(); i++) {
output.writeMessage(6, bcc_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (sender_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getSender());
}
if (recipient_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRecipient());
}
if (content_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getContent());
}
if (replyTo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getReplyTo());
}
for (int i = 0; i < cc_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, cc_.get(i));
}
for (int i = 0; i < bcc_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, bcc_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMessage)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMessage other = (io.bloombox.schema.comms.EmailComms.EmailMessage) obj;
if (hasSender() != other.hasSender()) return false;
if (hasSender()) {
if (!getSender()
.equals(other.getSender())) return false;
}
if (hasRecipient() != other.hasRecipient()) return false;
if (hasRecipient()) {
if (!getRecipient()
.equals(other.getRecipient())) return false;
}
if (hasContent() != other.hasContent()) return false;
if (hasContent()) {
if (!getContent()
.equals(other.getContent())) return false;
}
if (hasReplyTo() != other.hasReplyTo()) return false;
if (hasReplyTo()) {
if (!getReplyTo()
.equals(other.getReplyTo())) return false;
}
if (!getCcList()
.equals(other.getCcList())) return false;
if (!getBccList()
.equals(other.getBccList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSender()) {
hash = (37 * hash) + SENDER_FIELD_NUMBER;
hash = (53 * hash) + getSender().hashCode();
}
if (hasRecipient()) {
hash = (37 * hash) + RECIPIENT_FIELD_NUMBER;
hash = (53 * hash) + getRecipient().hashCode();
}
if (hasContent()) {
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContent().hashCode();
}
if (hasReplyTo()) {
hash = (37 * hash) + REPLY_TO_FIELD_NUMBER;
hash = (53 * hash) + getReplyTo().hashCode();
}
if (getCcCount() > 0) {
hash = (37 * hash) + CC_FIELD_NUMBER;
hash = (53 * hash) + getCcList().hashCode();
}
if (getBccCount() > 0) {
hash = (37 * hash) + BCC_FIELD_NUMBER;
hash = (53 * hash) + getBccList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of an individual email message.
*
*
* Protobuf type {@code bloombox.comms.EmailMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMessage)
io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMessage.class, io.bloombox.schema.comms.EmailComms.EmailMessage.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCcFieldBuilder();
getBccFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (senderBuilder_ == null) {
sender_ = null;
} else {
sender_ = null;
senderBuilder_ = null;
}
if (recipientBuilder_ == null) {
recipient_ = null;
} else {
recipient_ = null;
recipientBuilder_ = null;
}
if (contentBuilder_ == null) {
content_ = null;
} else {
content_ = null;
contentBuilder_ = null;
}
if (replyToBuilder_ == null) {
replyTo_ = null;
} else {
replyTo_ = null;
replyToBuilder_ = null;
}
if (ccBuilder_ == null) {
cc_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ccBuilder_.clear();
}
if (bccBuilder_ == null) {
bcc_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
bccBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMessage_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMessage getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMessage.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMessage build() {
io.bloombox.schema.comms.EmailComms.EmailMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMessage buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMessage result = new io.bloombox.schema.comms.EmailComms.EmailMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (senderBuilder_ == null) {
result.sender_ = sender_;
} else {
result.sender_ = senderBuilder_.build();
}
if (recipientBuilder_ == null) {
result.recipient_ = recipient_;
} else {
result.recipient_ = recipientBuilder_.build();
}
if (contentBuilder_ == null) {
result.content_ = content_;
} else {
result.content_ = contentBuilder_.build();
}
if (replyToBuilder_ == null) {
result.replyTo_ = replyTo_;
} else {
result.replyTo_ = replyToBuilder_.build();
}
if (ccBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
cc_ = java.util.Collections.unmodifiableList(cc_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.cc_ = cc_;
} else {
result.cc_ = ccBuilder_.build();
}
if (bccBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
bcc_ = java.util.Collections.unmodifiableList(bcc_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.bcc_ = bcc_;
} else {
result.bcc_ = bccBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMessage) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMessage other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMessage.getDefaultInstance()) return this;
if (other.hasSender()) {
mergeSender(other.getSender());
}
if (other.hasRecipient()) {
mergeRecipient(other.getRecipient());
}
if (other.hasContent()) {
mergeContent(other.getContent());
}
if (other.hasReplyTo()) {
mergeReplyTo(other.getReplyTo());
}
if (ccBuilder_ == null) {
if (!other.cc_.isEmpty()) {
if (cc_.isEmpty()) {
cc_ = other.cc_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureCcIsMutable();
cc_.addAll(other.cc_);
}
onChanged();
}
} else {
if (!other.cc_.isEmpty()) {
if (ccBuilder_.isEmpty()) {
ccBuilder_.dispose();
ccBuilder_ = null;
cc_ = other.cc_;
bitField0_ = (bitField0_ & ~0x00000010);
ccBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCcFieldBuilder() : null;
} else {
ccBuilder_.addAllMessages(other.cc_);
}
}
}
if (bccBuilder_ == null) {
if (!other.bcc_.isEmpty()) {
if (bcc_.isEmpty()) {
bcc_ = other.bcc_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureBccIsMutable();
bcc_.addAll(other.bcc_);
}
onChanged();
}
} else {
if (!other.bcc_.isEmpty()) {
if (bccBuilder_.isEmpty()) {
bccBuilder_.dispose();
bccBuilder_ = null;
bcc_ = other.bcc_;
bitField0_ = (bitField0_ & ~0x00000020);
bccBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getBccFieldBuilder() : null;
} else {
bccBuilder_.addAllMessages(other.bcc_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMessage) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private io.opencannabis.schema.contact.ContactEmail.EmailAddress sender_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> senderBuilder_;
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public boolean hasSender() {
return senderBuilder_ != null || sender_ != null;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getSender() {
if (senderBuilder_ == null) {
return sender_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : sender_;
} else {
return senderBuilder_.getMessage();
}
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public Builder setSender(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (senderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sender_ = value;
onChanged();
} else {
senderBuilder_.setMessage(value);
}
return this;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public Builder setSender(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (senderBuilder_ == null) {
sender_ = builderForValue.build();
onChanged();
} else {
senderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public Builder mergeSender(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (senderBuilder_ == null) {
if (sender_ != null) {
sender_ =
io.opencannabis.schema.contact.ContactEmail.EmailAddress.newBuilder(sender_).mergeFrom(value).buildPartial();
} else {
sender_ = value;
}
onChanged();
} else {
senderBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public Builder clearSender() {
if (senderBuilder_ == null) {
sender_ = null;
onChanged();
} else {
sender_ = null;
senderBuilder_ = null;
}
return this;
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getSenderBuilder() {
onChanged();
return getSenderFieldBuilder().getBuilder();
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getSenderOrBuilder() {
if (senderBuilder_ != null) {
return senderBuilder_.getMessageOrBuilder();
} else {
return sender_ == null ?
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : sender_;
}
}
/**
*
* Sender for the email message.
*
*
* .opencannabis.contact.EmailAddress sender = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getSenderFieldBuilder() {
if (senderBuilder_ == null) {
senderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
getSender(),
getParentForChildren(),
isClean());
sender_ = null;
}
return senderBuilder_;
}
private io.opencannabis.schema.contact.ContactEmail.EmailAddress recipient_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> recipientBuilder_;
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public boolean hasRecipient() {
return recipientBuilder_ != null || recipient_ != null;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getRecipient() {
if (recipientBuilder_ == null) {
return recipient_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : recipient_;
} else {
return recipientBuilder_.getMessage();
}
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public Builder setRecipient(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (recipientBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
recipient_ = value;
onChanged();
} else {
recipientBuilder_.setMessage(value);
}
return this;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public Builder setRecipient(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (recipientBuilder_ == null) {
recipient_ = builderForValue.build();
onChanged();
} else {
recipientBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public Builder mergeRecipient(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (recipientBuilder_ == null) {
if (recipient_ != null) {
recipient_ =
io.opencannabis.schema.contact.ContactEmail.EmailAddress.newBuilder(recipient_).mergeFrom(value).buildPartial();
} else {
recipient_ = value;
}
onChanged();
} else {
recipientBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public Builder clearRecipient() {
if (recipientBuilder_ == null) {
recipient_ = null;
onChanged();
} else {
recipient_ = null;
recipientBuilder_ = null;
}
return this;
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getRecipientBuilder() {
onChanged();
return getRecipientFieldBuilder().getBuilder();
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getRecipientOrBuilder() {
if (recipientBuilder_ != null) {
return recipientBuilder_.getMessageOrBuilder();
} else {
return recipient_ == null ?
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : recipient_;
}
}
/**
*
* Sender for the email recipient.
*
*
* .opencannabis.contact.EmailAddress recipient = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getRecipientFieldBuilder() {
if (recipientBuilder_ == null) {
recipientBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
getRecipient(),
getParentForChildren(),
isClean());
recipient_ = null;
}
return recipientBuilder_;
}
private io.bloombox.schema.comms.EmailComms.EmailContent content_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailContent, io.bloombox.schema.comms.EmailComms.EmailContent.Builder, io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder> contentBuilder_;
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public boolean hasContent() {
return contentBuilder_ != null || content_ != null;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public io.bloombox.schema.comms.EmailComms.EmailContent getContent() {
if (contentBuilder_ == null) {
return content_ == null ? io.bloombox.schema.comms.EmailComms.EmailContent.getDefaultInstance() : content_;
} else {
return contentBuilder_.getMessage();
}
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public Builder setContent(io.bloombox.schema.comms.EmailComms.EmailContent value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
content_ = value;
onChanged();
} else {
contentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public Builder setContent(
io.bloombox.schema.comms.EmailComms.EmailContent.Builder builderForValue) {
if (contentBuilder_ == null) {
content_ = builderForValue.build();
onChanged();
} else {
contentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public Builder mergeContent(io.bloombox.schema.comms.EmailComms.EmailContent value) {
if (contentBuilder_ == null) {
if (content_ != null) {
content_ =
io.bloombox.schema.comms.EmailComms.EmailContent.newBuilder(content_).mergeFrom(value).buildPartial();
} else {
content_ = value;
}
onChanged();
} else {
contentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public Builder clearContent() {
if (contentBuilder_ == null) {
content_ = null;
onChanged();
} else {
content_ = null;
contentBuilder_ = null;
}
return this;
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public io.bloombox.schema.comms.EmailComms.EmailContent.Builder getContentBuilder() {
onChanged();
return getContentFieldBuilder().getBuilder();
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
public io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder getContentOrBuilder() {
if (contentBuilder_ != null) {
return contentBuilder_.getMessageOrBuilder();
} else {
return content_ == null ?
io.bloombox.schema.comms.EmailComms.EmailContent.getDefaultInstance() : content_;
}
}
/**
*
* Content for the email message.
*
*
* .bloombox.comms.EmailContent content = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailContent, io.bloombox.schema.comms.EmailComms.EmailContent.Builder, io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder>
getContentFieldBuilder() {
if (contentBuilder_ == null) {
contentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailContent, io.bloombox.schema.comms.EmailComms.EmailContent.Builder, io.bloombox.schema.comms.EmailComms.EmailContentOrBuilder>(
getContent(),
getParentForChildren(),
isClean());
content_ = null;
}
return contentBuilder_;
}
private io.opencannabis.schema.contact.ContactEmail.EmailAddress replyTo_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> replyToBuilder_;
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public boolean hasReplyTo() {
return replyToBuilder_ != null || replyTo_ != null;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getReplyTo() {
if (replyToBuilder_ == null) {
return replyTo_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : replyTo_;
} else {
return replyToBuilder_.getMessage();
}
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public Builder setReplyTo(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (replyToBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
replyTo_ = value;
onChanged();
} else {
replyToBuilder_.setMessage(value);
}
return this;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public Builder setReplyTo(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (replyToBuilder_ == null) {
replyTo_ = builderForValue.build();
onChanged();
} else {
replyToBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public Builder mergeReplyTo(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (replyToBuilder_ == null) {
if (replyTo_ != null) {
replyTo_ =
io.opencannabis.schema.contact.ContactEmail.EmailAddress.newBuilder(replyTo_).mergeFrom(value).buildPartial();
} else {
replyTo_ = value;
}
onChanged();
} else {
replyToBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public Builder clearReplyTo() {
if (replyToBuilder_ == null) {
replyTo_ = null;
onChanged();
} else {
replyTo_ = null;
replyToBuilder_ = null;
}
return this;
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getReplyToBuilder() {
onChanged();
return getReplyToFieldBuilder().getBuilder();
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getReplyToOrBuilder() {
if (replyToBuilder_ != null) {
return replyToBuilder_.getMessageOrBuilder();
} else {
return replyTo_ == null ?
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : replyTo_;
}
}
/**
*
* Reply-to email address, if applicable.
*
*
* .opencannabis.contact.EmailAddress reply_to = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getReplyToFieldBuilder() {
if (replyToBuilder_ == null) {
replyToBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
getReplyTo(),
getParentForChildren(),
isClean());
replyTo_ = null;
}
return replyToBuilder_;
}
private java.util.List cc_ =
java.util.Collections.emptyList();
private void ensureCcIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
cc_ = new java.util.ArrayList(cc_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> ccBuilder_;
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public java.util.List getCcList() {
if (ccBuilder_ == null) {
return java.util.Collections.unmodifiableList(cc_);
} else {
return ccBuilder_.getMessageList();
}
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public int getCcCount() {
if (ccBuilder_ == null) {
return cc_.size();
} else {
return ccBuilder_.getCount();
}
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getCc(int index) {
if (ccBuilder_ == null) {
return cc_.get(index);
} else {
return ccBuilder_.getMessage(index);
}
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder setCc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (ccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCcIsMutable();
cc_.set(index, value);
onChanged();
} else {
ccBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder setCc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (ccBuilder_ == null) {
ensureCcIsMutable();
cc_.set(index, builderForValue.build());
onChanged();
} else {
ccBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder addCc(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (ccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCcIsMutable();
cc_.add(value);
onChanged();
} else {
ccBuilder_.addMessage(value);
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder addCc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (ccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCcIsMutable();
cc_.add(index, value);
onChanged();
} else {
ccBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder addCc(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (ccBuilder_ == null) {
ensureCcIsMutable();
cc_.add(builderForValue.build());
onChanged();
} else {
ccBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder addCc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (ccBuilder_ == null) {
ensureCcIsMutable();
cc_.add(index, builderForValue.build());
onChanged();
} else {
ccBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder addAllCc(
java.lang.Iterable extends io.opencannabis.schema.contact.ContactEmail.EmailAddress> values) {
if (ccBuilder_ == null) {
ensureCcIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cc_);
onChanged();
} else {
ccBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder clearCc() {
if (ccBuilder_ == null) {
cc_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
ccBuilder_.clear();
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public Builder removeCc(int index) {
if (ccBuilder_ == null) {
ensureCcIsMutable();
cc_.remove(index);
onChanged();
} else {
ccBuilder_.remove(index);
}
return this;
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getCcBuilder(
int index) {
return getCcFieldBuilder().getBuilder(index);
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getCcOrBuilder(
int index) {
if (ccBuilder_ == null) {
return cc_.get(index); } else {
return ccBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getCcOrBuilderList() {
if (ccBuilder_ != null) {
return ccBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(cc_);
}
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder addCcBuilder() {
return getCcFieldBuilder().addBuilder(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance());
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder addCcBuilder(
int index) {
return getCcFieldBuilder().addBuilder(
index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance());
}
/**
*
* Email addresses to add on CC.
*
*
* repeated .opencannabis.contact.EmailAddress cc = 5;
*/
public java.util.List
getCcBuilderList() {
return getCcFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getCcFieldBuilder() {
if (ccBuilder_ == null) {
ccBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
cc_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
cc_ = null;
}
return ccBuilder_;
}
private java.util.List bcc_ =
java.util.Collections.emptyList();
private void ensureBccIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
bcc_ = new java.util.ArrayList(bcc_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> bccBuilder_;
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public java.util.List getBccList() {
if (bccBuilder_ == null) {
return java.util.Collections.unmodifiableList(bcc_);
} else {
return bccBuilder_.getMessageList();
}
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public int getBccCount() {
if (bccBuilder_ == null) {
return bcc_.size();
} else {
return bccBuilder_.getCount();
}
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getBcc(int index) {
if (bccBuilder_ == null) {
return bcc_.get(index);
} else {
return bccBuilder_.getMessage(index);
}
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder setBcc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (bccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBccIsMutable();
bcc_.set(index, value);
onChanged();
} else {
bccBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder setBcc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (bccBuilder_ == null) {
ensureBccIsMutable();
bcc_.set(index, builderForValue.build());
onChanged();
} else {
bccBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder addBcc(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (bccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBccIsMutable();
bcc_.add(value);
onChanged();
} else {
bccBuilder_.addMessage(value);
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder addBcc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (bccBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBccIsMutable();
bcc_.add(index, value);
onChanged();
} else {
bccBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder addBcc(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (bccBuilder_ == null) {
ensureBccIsMutable();
bcc_.add(builderForValue.build());
onChanged();
} else {
bccBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder addBcc(
int index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (bccBuilder_ == null) {
ensureBccIsMutable();
bcc_.add(index, builderForValue.build());
onChanged();
} else {
bccBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder addAllBcc(
java.lang.Iterable extends io.opencannabis.schema.contact.ContactEmail.EmailAddress> values) {
if (bccBuilder_ == null) {
ensureBccIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bcc_);
onChanged();
} else {
bccBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder clearBcc() {
if (bccBuilder_ == null) {
bcc_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
bccBuilder_.clear();
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public Builder removeBcc(int index) {
if (bccBuilder_ == null) {
ensureBccIsMutable();
bcc_.remove(index);
onChanged();
} else {
bccBuilder_.remove(index);
}
return this;
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getBccBuilder(
int index) {
return getBccFieldBuilder().getBuilder(index);
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getBccOrBuilder(
int index) {
if (bccBuilder_ == null) {
return bcc_.get(index); } else {
return bccBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public java.util.List extends io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getBccOrBuilderList() {
if (bccBuilder_ != null) {
return bccBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(bcc_);
}
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder addBccBuilder() {
return getBccFieldBuilder().addBuilder(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance());
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder addBccBuilder(
int index) {
return getBccFieldBuilder().addBuilder(
index, io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance());
}
/**
*
* Email addresses to add on BCC.
*
*
* repeated .opencannabis.contact.EmailAddress bcc = 6;
*/
public java.util.List
getBccBuilderList() {
return getBccFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getBccFieldBuilder() {
if (bccBuilder_ == null) {
bccBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
bcc_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
bcc_ = null;
}
return bccBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMessage)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMessage)
private static final io.bloombox.schema.comms.EmailComms.EmailMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMessage();
}
public static io.bloombox.schema.comms.EmailComms.EmailMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailMessage(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PublisherMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.PublisherMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
java.lang.String getUrl();
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
com.google.protobuf.ByteString
getUrlBytes();
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
java.lang.String getGooglePlus();
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
com.google.protobuf.ByteString
getGooglePlusBytes();
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
java.lang.String getTwitter();
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
com.google.protobuf.ByteString
getTwitterBytes();
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
java.lang.String getFacebook();
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
com.google.protobuf.ByteString
getFacebookBytes();
}
/**
*
* Specifies information about the publisher of an action.
*
*
* Protobuf type {@code bloombox.comms.PublisherMetadata}
*/
public static final class PublisherMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.PublisherMetadata)
PublisherMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use PublisherMetadata.newBuilder() to construct.
private PublisherMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PublisherMetadata() {
name_ = "";
url_ = "";
googlePlus_ = "";
twitter_ = "";
facebook_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PublisherMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
url_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
googlePlus_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
twitter_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
facebook_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_PublisherMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_PublisherMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.PublisherMetadata.class, io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int URL_FIELD_NUMBER = 2;
private volatile java.lang.Object url_;
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
url_ = s;
return s;
}
}
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GOOGLE_PLUS_FIELD_NUMBER = 3;
private volatile java.lang.Object googlePlus_;
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public java.lang.String getGooglePlus() {
java.lang.Object ref = googlePlus_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
googlePlus_ = s;
return s;
}
}
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public com.google.protobuf.ByteString
getGooglePlusBytes() {
java.lang.Object ref = googlePlus_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
googlePlus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TWITTER_FIELD_NUMBER = 4;
private volatile java.lang.Object twitter_;
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public java.lang.String getTwitter() {
java.lang.Object ref = twitter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
twitter_ = s;
return s;
}
}
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public com.google.protobuf.ByteString
getTwitterBytes() {
java.lang.Object ref = twitter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
twitter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FACEBOOK_FIELD_NUMBER = 5;
private volatile java.lang.Object facebook_;
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public java.lang.String getFacebook() {
java.lang.Object ref = facebook_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
facebook_ = s;
return s;
}
}
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public com.google.protobuf.ByteString
getFacebookBytes() {
java.lang.Object ref = facebook_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
facebook_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, url_);
}
if (!getGooglePlusBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, googlePlus_);
}
if (!getTwitterBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, twitter_);
}
if (!getFacebookBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, facebook_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, url_);
}
if (!getGooglePlusBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, googlePlus_);
}
if (!getTwitterBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, twitter_);
}
if (!getFacebookBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, facebook_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.PublisherMetadata)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.PublisherMetadata other = (io.bloombox.schema.comms.EmailComms.PublisherMetadata) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getUrl()
.equals(other.getUrl())) return false;
if (!getGooglePlus()
.equals(other.getGooglePlus())) return false;
if (!getTwitter()
.equals(other.getTwitter())) return false;
if (!getFacebook()
.equals(other.getFacebook())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
hash = (37 * hash) + GOOGLE_PLUS_FIELD_NUMBER;
hash = (53 * hash) + getGooglePlus().hashCode();
hash = (37 * hash) + TWITTER_FIELD_NUMBER;
hash = (53 * hash) + getTwitter().hashCode();
hash = (37 * hash) + FACEBOOK_FIELD_NUMBER;
hash = (53 * hash) + getFacebook().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.PublisherMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies information about the publisher of an action.
*
*
* Protobuf type {@code bloombox.comms.PublisherMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.PublisherMetadata)
io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_PublisherMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_PublisherMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.PublisherMetadata.class, io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.PublisherMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
url_ = "";
googlePlus_ = "";
twitter_ = "";
facebook_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_PublisherMetadata_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.PublisherMetadata getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.PublisherMetadata.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.PublisherMetadata build() {
io.bloombox.schema.comms.EmailComms.PublisherMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.PublisherMetadata buildPartial() {
io.bloombox.schema.comms.EmailComms.PublisherMetadata result = new io.bloombox.schema.comms.EmailComms.PublisherMetadata(this);
result.name_ = name_;
result.url_ = url_;
result.googlePlus_ = googlePlus_;
result.twitter_ = twitter_;
result.facebook_ = facebook_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.PublisherMetadata) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.PublisherMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.PublisherMetadata other) {
if (other == io.bloombox.schema.comms.EmailComms.PublisherMetadata.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getUrl().isEmpty()) {
url_ = other.url_;
onChanged();
}
if (!other.getGooglePlus().isEmpty()) {
googlePlus_ = other.googlePlus_;
onChanged();
}
if (!other.getTwitter().isEmpty()) {
twitter_ = other.twitter_;
onChanged();
}
if (!other.getFacebook().isEmpty()) {
facebook_ = other.facebook_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.PublisherMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.PublisherMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name of the publishing organization.
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object url_ = "";
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
url_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public Builder setUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
url_ = value;
onChanged();
return this;
}
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public Builder clearUrl() {
url_ = getDefaultInstance().getUrl();
onChanged();
return this;
}
/**
*
* Main URL for the organization's website.
*
*
* string url = 2;
*/
public Builder setUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
url_ = value;
onChanged();
return this;
}
private java.lang.Object googlePlus_ = "";
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public java.lang.String getGooglePlus() {
java.lang.Object ref = googlePlus_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
googlePlus_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public com.google.protobuf.ByteString
getGooglePlusBytes() {
java.lang.Object ref = googlePlus_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
googlePlus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public Builder setGooglePlus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
googlePlus_ = value;
onChanged();
return this;
}
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public Builder clearGooglePlus() {
googlePlus_ = getDefaultInstance().getGooglePlus();
onChanged();
return this;
}
/**
*
* URL for the organization's Google+.
*
*
* string google_plus = 3;
*/
public Builder setGooglePlusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
googlePlus_ = value;
onChanged();
return this;
}
private java.lang.Object twitter_ = "";
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public java.lang.String getTwitter() {
java.lang.Object ref = twitter_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
twitter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public com.google.protobuf.ByteString
getTwitterBytes() {
java.lang.Object ref = twitter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
twitter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public Builder setTwitter(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
twitter_ = value;
onChanged();
return this;
}
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public Builder clearTwitter() {
twitter_ = getDefaultInstance().getTwitter();
onChanged();
return this;
}
/**
*
* URL for the organization's Twitter.
*
*
* string twitter = 4;
*/
public Builder setTwitterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
twitter_ = value;
onChanged();
return this;
}
private java.lang.Object facebook_ = "";
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public java.lang.String getFacebook() {
java.lang.Object ref = facebook_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
facebook_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public com.google.protobuf.ByteString
getFacebookBytes() {
java.lang.Object ref = facebook_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
facebook_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public Builder setFacebook(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
facebook_ = value;
onChanged();
return this;
}
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public Builder clearFacebook() {
facebook_ = getDefaultInstance().getFacebook();
onChanged();
return this;
}
/**
*
* URL for the organization's Facebook.
*
*
* string facebook = 5;
*/
public Builder setFacebookBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
facebook_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.PublisherMetadata)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.PublisherMetadata)
private static final io.bloombox.schema.comms.EmailComms.PublisherMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.PublisherMetadata();
}
public static io.bloombox.schema.comms.EmailComms.PublisherMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PublisherMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PublisherMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.PublisherMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* Specifies schema-based metadata appended to a message.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata}
*/
public static final class EmailMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata)
EmailMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailMetadata.newBuilder() to construct.
private EmailMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailMetadata() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.Builder.class);
}
/**
*
* Specifies the type of metadata block to append.
*
*
* Protobuf enum {@code bloombox.comms.EmailMetadata.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Go-to view action.
*
*
* VIEW = 0;
*/
VIEW(0),
/**
*
* Go-to track action.
*
*
* TRACK = 1;
*/
TRACK(1),
/**
*
* One-click action.
*
*
* ONE_CLICK = 2;
*/
ONE_CLICK(2),
/**
*
* Attached order.
*
*
* ORDER = 3;
*/
ORDER(3),
UNRECOGNIZED(-1),
;
/**
*
* Go-to view action.
*
*
* VIEW = 0;
*/
public static final int VIEW_VALUE = 0;
/**
*
* Go-to track action.
*
*
* TRACK = 1;
*/
public static final int TRACK_VALUE = 1;
/**
*
* One-click action.
*
*
* ONE_CLICK = 2;
*/
public static final int ONE_CLICK_VALUE = 2;
/**
*
* Attached order.
*
*
* ORDER = 3;
*/
public static final int ORDER_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return VIEW;
case 1: return TRACK;
case 2: return ONE_CLICK;
case 3: return ORDER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.comms.EmailMetadata.Type)
}
public interface GoToViewOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata.GoToView)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the action.
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Name of the action.
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
java.lang.String getTarget();
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
com.google.protobuf.ByteString
getTargetBytes();
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
boolean hasPublisher();
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
io.bloombox.schema.comms.EmailComms.PublisherMetadata getPublisher();
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder getPublisherOrBuilder();
}
/**
*
* Specifies the structure of an attached go-to action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.GoToView}
*/
public static final class GoToView extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata.GoToView)
GoToViewOrBuilder {
private static final long serialVersionUID = 0L;
// Use GoToView.newBuilder() to construct.
private GoToView(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GoToView() {
name_ = "";
target_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GoToView(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
target_ = s;
break;
}
case 34: {
io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder subBuilder = null;
if (publisher_ != null) {
subBuilder = publisher_.toBuilder();
}
publisher_ = input.readMessage(io.bloombox.schema.comms.EmailComms.PublisherMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(publisher_);
publisher_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToView_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TARGET_FIELD_NUMBER = 3;
private volatile java.lang.Object target_;
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public java.lang.String getTarget() {
java.lang.Object ref = target_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
target_ = s;
return s;
}
}
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public com.google.protobuf.ByteString
getTargetBytes() {
java.lang.Object ref = target_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
target_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PUBLISHER_FIELD_NUMBER = 4;
private io.bloombox.schema.comms.EmailComms.PublisherMetadata publisher_;
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public boolean hasPublisher() {
return publisher_ != null;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public io.bloombox.schema.comms.EmailComms.PublisherMetadata getPublisher() {
return publisher_ == null ? io.bloombox.schema.comms.EmailComms.PublisherMetadata.getDefaultInstance() : publisher_;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder getPublisherOrBuilder() {
return getPublisher();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getTargetBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, target_);
}
if (publisher_ != null) {
output.writeMessage(4, getPublisher());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getTargetBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, target_);
}
if (publisher_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPublisher());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView other = (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getTarget()
.equals(other.getTarget())) return false;
if (hasPublisher() != other.hasPublisher()) return false;
if (hasPublisher()) {
if (!getPublisher()
.equals(other.getPublisher())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + TARGET_FIELD_NUMBER;
hash = (53 * hash) + getTarget().hashCode();
if (hasPublisher()) {
hash = (37 * hash) + PUBLISHER_FIELD_NUMBER;
hash = (53 * hash) + getPublisher().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of an attached go-to action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.GoToView}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata.GoToView)
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToView_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
target_ = "";
if (publisherBuilder_ == null) {
publisher_ = null;
} else {
publisher_ = null;
publisherBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView result = new io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView(this);
result.name_ = name_;
result.target_ = target_;
if (publisherBuilder_ == null) {
result.publisher_ = publisher_;
} else {
result.publisher_ = publisherBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getTarget().isEmpty()) {
target_ = other.target_;
onChanged();
}
if (other.hasPublisher()) {
mergePublisher(other.getPublisher());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name of the action.
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object target_ = "";
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public java.lang.String getTarget() {
java.lang.Object ref = target_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
target_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public com.google.protobuf.ByteString
getTargetBytes() {
java.lang.Object ref = target_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
target_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public Builder setTarget(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
return this;
}
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public Builder clearTarget() {
target_ = getDefaultInstance().getTarget();
onChanged();
return this;
}
/**
*
* URL target for the action.
*
*
* string target = 3;
*/
public Builder setTargetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
target_ = value;
onChanged();
return this;
}
private io.bloombox.schema.comms.EmailComms.PublisherMetadata publisher_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.PublisherMetadata, io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder, io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder> publisherBuilder_;
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public boolean hasPublisher() {
return publisherBuilder_ != null || publisher_ != null;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public io.bloombox.schema.comms.EmailComms.PublisherMetadata getPublisher() {
if (publisherBuilder_ == null) {
return publisher_ == null ? io.bloombox.schema.comms.EmailComms.PublisherMetadata.getDefaultInstance() : publisher_;
} else {
return publisherBuilder_.getMessage();
}
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public Builder setPublisher(io.bloombox.schema.comms.EmailComms.PublisherMetadata value) {
if (publisherBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
publisher_ = value;
onChanged();
} else {
publisherBuilder_.setMessage(value);
}
return this;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public Builder setPublisher(
io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder builderForValue) {
if (publisherBuilder_ == null) {
publisher_ = builderForValue.build();
onChanged();
} else {
publisherBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public Builder mergePublisher(io.bloombox.schema.comms.EmailComms.PublisherMetadata value) {
if (publisherBuilder_ == null) {
if (publisher_ != null) {
publisher_ =
io.bloombox.schema.comms.EmailComms.PublisherMetadata.newBuilder(publisher_).mergeFrom(value).buildPartial();
} else {
publisher_ = value;
}
onChanged();
} else {
publisherBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public Builder clearPublisher() {
if (publisherBuilder_ == null) {
publisher_ = null;
onChanged();
} else {
publisher_ = null;
publisherBuilder_ = null;
}
return this;
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder getPublisherBuilder() {
onChanged();
return getPublisherFieldBuilder().getBuilder();
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
public io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder getPublisherOrBuilder() {
if (publisherBuilder_ != null) {
return publisherBuilder_.getMessageOrBuilder();
} else {
return publisher_ == null ?
io.bloombox.schema.comms.EmailComms.PublisherMetadata.getDefaultInstance() : publisher_;
}
}
/**
*
* Information about the action's publisher.
*
*
* .bloombox.comms.PublisherMetadata publisher = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.PublisherMetadata, io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder, io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder>
getPublisherFieldBuilder() {
if (publisherBuilder_ == null) {
publisherBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.PublisherMetadata, io.bloombox.schema.comms.EmailComms.PublisherMetadata.Builder, io.bloombox.schema.comms.EmailComms.PublisherMetadataOrBuilder>(
getPublisher(),
getParentForChildren(),
isClean());
publisher_ = null;
}
return publisherBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata.GoToView)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata.GoToView)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GoToView parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GoToView(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GoToTrackOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata.GoToTrack)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
boolean hasDeliveryAddress();
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
io.opencannabis.schema.geo.Address getDeliveryAddress();
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
io.opencannabis.schema.geo.AddressOrBuilder getDeliveryAddressOrBuilder();
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
boolean hasEstimatedReady();
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedReady();
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedReadyOrBuilder();
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
boolean hasEstimatedArrival();
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedArrival();
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedArrivalOrBuilder();
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
boolean hasPartner();
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
io.bloombox.schema.partner.PartnerAccount.Partner getPartner();
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
boolean hasOrder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
io.opencannabis.schema.commerce.CommercialOrder.Order getOrder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder();
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
java.lang.String getTrackingUrl();
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
com.google.protobuf.ByteString
getTrackingUrlBytes();
}
/**
*
* Specifies the structure of an attached One-Click action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.GoToTrack}
*/
public static final class GoToTrack extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata.GoToTrack)
GoToTrackOrBuilder {
private static final long serialVersionUID = 0L;
// Use GoToTrack.newBuilder() to construct.
private GoToTrack(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GoToTrack() {
trackingUrl_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GoToTrack(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.geo.Address.Builder subBuilder = null;
if (deliveryAddress_ != null) {
subBuilder = deliveryAddress_.toBuilder();
}
deliveryAddress_ = input.readMessage(io.opencannabis.schema.geo.Address.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(deliveryAddress_);
deliveryAddress_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (estimatedReady_ != null) {
subBuilder = estimatedReady_.toBuilder();
}
estimatedReady_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(estimatedReady_);
estimatedReady_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (estimatedArrival_ != null) {
subBuilder = estimatedArrival_.toBuilder();
}
estimatedArrival_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(estimatedArrival_);
estimatedArrival_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.bloombox.schema.partner.PartnerAccount.Partner.Builder subBuilder = null;
if (partner_ != null) {
subBuilder = partner_.toBuilder();
}
partner_ = input.readMessage(io.bloombox.schema.partner.PartnerAccount.Partner.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(partner_);
partner_ = subBuilder.buildPartial();
}
break;
}
case 42: {
io.opencannabis.schema.commerce.CommercialOrder.Order.Builder subBuilder = null;
if (order_ != null) {
subBuilder = order_.toBuilder();
}
order_ = input.readMessage(io.opencannabis.schema.commerce.CommercialOrder.Order.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(order_);
order_ = subBuilder.buildPartial();
}
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
trackingUrl_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToTrack_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder.class);
}
public static final int DELIVERY_ADDRESS_FIELD_NUMBER = 1;
private io.opencannabis.schema.geo.Address deliveryAddress_;
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public boolean hasDeliveryAddress() {
return deliveryAddress_ != null;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public io.opencannabis.schema.geo.Address getDeliveryAddress() {
return deliveryAddress_ == null ? io.opencannabis.schema.geo.Address.getDefaultInstance() : deliveryAddress_;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public io.opencannabis.schema.geo.AddressOrBuilder getDeliveryAddressOrBuilder() {
return getDeliveryAddress();
}
public static final int ESTIMATED_READY_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant estimatedReady_;
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public boolean hasEstimatedReady() {
return estimatedReady_ != null;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedReady() {
return estimatedReady_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedReady_;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedReadyOrBuilder() {
return getEstimatedReady();
}
public static final int ESTIMATED_ARRIVAL_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalInstant.Instant estimatedArrival_;
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public boolean hasEstimatedArrival() {
return estimatedArrival_ != null;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedArrival() {
return estimatedArrival_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedArrival_;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedArrivalOrBuilder() {
return getEstimatedArrival();
}
public static final int PARTNER_FIELD_NUMBER = 4;
private io.bloombox.schema.partner.PartnerAccount.Partner partner_;
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public boolean hasPartner() {
return partner_ != null;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner getPartner() {
return partner_ == null ? io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder() {
return getPartner();
}
public static final int ORDER_FIELD_NUMBER = 5;
private io.opencannabis.schema.commerce.CommercialOrder.Order order_;
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public boolean hasOrder() {
return order_ != null;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order getOrder() {
return order_ == null ? io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder() {
return getOrder();
}
public static final int TRACKING_URL_FIELD_NUMBER = 6;
private volatile java.lang.Object trackingUrl_;
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public java.lang.String getTrackingUrl() {
java.lang.Object ref = trackingUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
trackingUrl_ = s;
return s;
}
}
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public com.google.protobuf.ByteString
getTrackingUrlBytes() {
java.lang.Object ref = trackingUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
trackingUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (deliveryAddress_ != null) {
output.writeMessage(1, getDeliveryAddress());
}
if (estimatedReady_ != null) {
output.writeMessage(2, getEstimatedReady());
}
if (estimatedArrival_ != null) {
output.writeMessage(3, getEstimatedArrival());
}
if (partner_ != null) {
output.writeMessage(4, getPartner());
}
if (order_ != null) {
output.writeMessage(5, getOrder());
}
if (!getTrackingUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, trackingUrl_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (deliveryAddress_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getDeliveryAddress());
}
if (estimatedReady_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getEstimatedReady());
}
if (estimatedArrival_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getEstimatedArrival());
}
if (partner_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPartner());
}
if (order_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getOrder());
}
if (!getTrackingUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, trackingUrl_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack other = (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) obj;
if (hasDeliveryAddress() != other.hasDeliveryAddress()) return false;
if (hasDeliveryAddress()) {
if (!getDeliveryAddress()
.equals(other.getDeliveryAddress())) return false;
}
if (hasEstimatedReady() != other.hasEstimatedReady()) return false;
if (hasEstimatedReady()) {
if (!getEstimatedReady()
.equals(other.getEstimatedReady())) return false;
}
if (hasEstimatedArrival() != other.hasEstimatedArrival()) return false;
if (hasEstimatedArrival()) {
if (!getEstimatedArrival()
.equals(other.getEstimatedArrival())) return false;
}
if (hasPartner() != other.hasPartner()) return false;
if (hasPartner()) {
if (!getPartner()
.equals(other.getPartner())) return false;
}
if (hasOrder() != other.hasOrder()) return false;
if (hasOrder()) {
if (!getOrder()
.equals(other.getOrder())) return false;
}
if (!getTrackingUrl()
.equals(other.getTrackingUrl())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDeliveryAddress()) {
hash = (37 * hash) + DELIVERY_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDeliveryAddress().hashCode();
}
if (hasEstimatedReady()) {
hash = (37 * hash) + ESTIMATED_READY_FIELD_NUMBER;
hash = (53 * hash) + getEstimatedReady().hashCode();
}
if (hasEstimatedArrival()) {
hash = (37 * hash) + ESTIMATED_ARRIVAL_FIELD_NUMBER;
hash = (53 * hash) + getEstimatedArrival().hashCode();
}
if (hasPartner()) {
hash = (37 * hash) + PARTNER_FIELD_NUMBER;
hash = (53 * hash) + getPartner().hashCode();
}
if (hasOrder()) {
hash = (37 * hash) + ORDER_FIELD_NUMBER;
hash = (53 * hash) + getOrder().hashCode();
}
hash = (37 * hash) + TRACKING_URL_FIELD_NUMBER;
hash = (53 * hash) + getTrackingUrl().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of an attached One-Click action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.GoToTrack}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata.GoToTrack)
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToTrack_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (deliveryAddressBuilder_ == null) {
deliveryAddress_ = null;
} else {
deliveryAddress_ = null;
deliveryAddressBuilder_ = null;
}
if (estimatedReadyBuilder_ == null) {
estimatedReady_ = null;
} else {
estimatedReady_ = null;
estimatedReadyBuilder_ = null;
}
if (estimatedArrivalBuilder_ == null) {
estimatedArrival_ = null;
} else {
estimatedArrival_ = null;
estimatedArrivalBuilder_ = null;
}
if (partnerBuilder_ == null) {
partner_ = null;
} else {
partner_ = null;
partnerBuilder_ = null;
}
if (orderBuilder_ == null) {
order_ = null;
} else {
order_ = null;
orderBuilder_ = null;
}
trackingUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack result = new io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack(this);
if (deliveryAddressBuilder_ == null) {
result.deliveryAddress_ = deliveryAddress_;
} else {
result.deliveryAddress_ = deliveryAddressBuilder_.build();
}
if (estimatedReadyBuilder_ == null) {
result.estimatedReady_ = estimatedReady_;
} else {
result.estimatedReady_ = estimatedReadyBuilder_.build();
}
if (estimatedArrivalBuilder_ == null) {
result.estimatedArrival_ = estimatedArrival_;
} else {
result.estimatedArrival_ = estimatedArrivalBuilder_.build();
}
if (partnerBuilder_ == null) {
result.partner_ = partner_;
} else {
result.partner_ = partnerBuilder_.build();
}
if (orderBuilder_ == null) {
result.order_ = order_;
} else {
result.order_ = orderBuilder_.build();
}
result.trackingUrl_ = trackingUrl_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance()) return this;
if (other.hasDeliveryAddress()) {
mergeDeliveryAddress(other.getDeliveryAddress());
}
if (other.hasEstimatedReady()) {
mergeEstimatedReady(other.getEstimatedReady());
}
if (other.hasEstimatedArrival()) {
mergeEstimatedArrival(other.getEstimatedArrival());
}
if (other.hasPartner()) {
mergePartner(other.getPartner());
}
if (other.hasOrder()) {
mergeOrder(other.getOrder());
}
if (!other.getTrackingUrl().isEmpty()) {
trackingUrl_ = other.trackingUrl_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.geo.Address deliveryAddress_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Address, io.opencannabis.schema.geo.Address.Builder, io.opencannabis.schema.geo.AddressOrBuilder> deliveryAddressBuilder_;
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public boolean hasDeliveryAddress() {
return deliveryAddressBuilder_ != null || deliveryAddress_ != null;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public io.opencannabis.schema.geo.Address getDeliveryAddress() {
if (deliveryAddressBuilder_ == null) {
return deliveryAddress_ == null ? io.opencannabis.schema.geo.Address.getDefaultInstance() : deliveryAddress_;
} else {
return deliveryAddressBuilder_.getMessage();
}
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public Builder setDeliveryAddress(io.opencannabis.schema.geo.Address value) {
if (deliveryAddressBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deliveryAddress_ = value;
onChanged();
} else {
deliveryAddressBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public Builder setDeliveryAddress(
io.opencannabis.schema.geo.Address.Builder builderForValue) {
if (deliveryAddressBuilder_ == null) {
deliveryAddress_ = builderForValue.build();
onChanged();
} else {
deliveryAddressBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public Builder mergeDeliveryAddress(io.opencannabis.schema.geo.Address value) {
if (deliveryAddressBuilder_ == null) {
if (deliveryAddress_ != null) {
deliveryAddress_ =
io.opencannabis.schema.geo.Address.newBuilder(deliveryAddress_).mergeFrom(value).buildPartial();
} else {
deliveryAddress_ = value;
}
onChanged();
} else {
deliveryAddressBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public Builder clearDeliveryAddress() {
if (deliveryAddressBuilder_ == null) {
deliveryAddress_ = null;
onChanged();
} else {
deliveryAddress_ = null;
deliveryAddressBuilder_ = null;
}
return this;
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public io.opencannabis.schema.geo.Address.Builder getDeliveryAddressBuilder() {
onChanged();
return getDeliveryAddressFieldBuilder().getBuilder();
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
public io.opencannabis.schema.geo.AddressOrBuilder getDeliveryAddressOrBuilder() {
if (deliveryAddressBuilder_ != null) {
return deliveryAddressBuilder_.getMessageOrBuilder();
} else {
return deliveryAddress_ == null ?
io.opencannabis.schema.geo.Address.getDefaultInstance() : deliveryAddress_;
}
}
/**
*
* Specifies the delivery address for an order.
*
*
* .opencannabis.geo.Address delivery_address = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Address, io.opencannabis.schema.geo.Address.Builder, io.opencannabis.schema.geo.AddressOrBuilder>
getDeliveryAddressFieldBuilder() {
if (deliveryAddressBuilder_ == null) {
deliveryAddressBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Address, io.opencannabis.schema.geo.Address.Builder, io.opencannabis.schema.geo.AddressOrBuilder>(
getDeliveryAddress(),
getParentForChildren(),
isClean());
deliveryAddress_ = null;
}
return deliveryAddressBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant estimatedReady_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> estimatedReadyBuilder_;
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public boolean hasEstimatedReady() {
return estimatedReadyBuilder_ != null || estimatedReady_ != null;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedReady() {
if (estimatedReadyBuilder_ == null) {
return estimatedReady_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedReady_;
} else {
return estimatedReadyBuilder_.getMessage();
}
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public Builder setEstimatedReady(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (estimatedReadyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
estimatedReady_ = value;
onChanged();
} else {
estimatedReadyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public Builder setEstimatedReady(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (estimatedReadyBuilder_ == null) {
estimatedReady_ = builderForValue.build();
onChanged();
} else {
estimatedReadyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public Builder mergeEstimatedReady(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (estimatedReadyBuilder_ == null) {
if (estimatedReady_ != null) {
estimatedReady_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(estimatedReady_).mergeFrom(value).buildPartial();
} else {
estimatedReady_ = value;
}
onChanged();
} else {
estimatedReadyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public Builder clearEstimatedReady() {
if (estimatedReadyBuilder_ == null) {
estimatedReady_ = null;
onChanged();
} else {
estimatedReady_ = null;
estimatedReadyBuilder_ = null;
}
return this;
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getEstimatedReadyBuilder() {
onChanged();
return getEstimatedReadyFieldBuilder().getBuilder();
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedReadyOrBuilder() {
if (estimatedReadyBuilder_ != null) {
return estimatedReadyBuilder_.getMessageOrBuilder();
} else {
return estimatedReady_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedReady_;
}
}
/**
*
* Estimated departure of a delivery order, or availability of a pickup order.
*
*
* .opencannabis.temporal.Instant estimated_ready = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getEstimatedReadyFieldBuilder() {
if (estimatedReadyBuilder_ == null) {
estimatedReadyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getEstimatedReady(),
getParentForChildren(),
isClean());
estimatedReady_ = null;
}
return estimatedReadyBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant estimatedArrival_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> estimatedArrivalBuilder_;
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public boolean hasEstimatedArrival() {
return estimatedArrivalBuilder_ != null || estimatedArrival_ != null;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstimatedArrival() {
if (estimatedArrivalBuilder_ == null) {
return estimatedArrival_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedArrival_;
} else {
return estimatedArrivalBuilder_.getMessage();
}
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public Builder setEstimatedArrival(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (estimatedArrivalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
estimatedArrival_ = value;
onChanged();
} else {
estimatedArrivalBuilder_.setMessage(value);
}
return this;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public Builder setEstimatedArrival(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (estimatedArrivalBuilder_ == null) {
estimatedArrival_ = builderForValue.build();
onChanged();
} else {
estimatedArrivalBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public Builder mergeEstimatedArrival(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (estimatedArrivalBuilder_ == null) {
if (estimatedArrival_ != null) {
estimatedArrival_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(estimatedArrival_).mergeFrom(value).buildPartial();
} else {
estimatedArrival_ = value;
}
onChanged();
} else {
estimatedArrivalBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public Builder clearEstimatedArrival() {
if (estimatedArrivalBuilder_ == null) {
estimatedArrival_ = null;
onChanged();
} else {
estimatedArrival_ = null;
estimatedArrivalBuilder_ = null;
}
return this;
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getEstimatedArrivalBuilder() {
onChanged();
return getEstimatedArrivalFieldBuilder().getBuilder();
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstimatedArrivalOrBuilder() {
if (estimatedArrivalBuilder_ != null) {
return estimatedArrivalBuilder_.getMessageOrBuilder();
} else {
return estimatedArrival_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : estimatedArrival_;
}
}
/**
*
* Estimated arrival of a delivery order.
*
*
* .opencannabis.temporal.Instant estimated_arrival = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getEstimatedArrivalFieldBuilder() {
if (estimatedArrivalBuilder_ == null) {
estimatedArrivalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getEstimatedArrival(),
getParentForChildren(),
isClean());
estimatedArrival_ = null;
}
return estimatedArrivalBuilder_;
}
private io.bloombox.schema.partner.PartnerAccount.Partner partner_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder> partnerBuilder_;
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public boolean hasPartner() {
return partnerBuilder_ != null || partner_ != null;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner getPartner() {
if (partnerBuilder_ == null) {
return partner_ == null ? io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
} else {
return partnerBuilder_.getMessage();
}
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public Builder setPartner(io.bloombox.schema.partner.PartnerAccount.Partner value) {
if (partnerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partner_ = value;
onChanged();
} else {
partnerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public Builder setPartner(
io.bloombox.schema.partner.PartnerAccount.Partner.Builder builderForValue) {
if (partnerBuilder_ == null) {
partner_ = builderForValue.build();
onChanged();
} else {
partnerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public Builder mergePartner(io.bloombox.schema.partner.PartnerAccount.Partner value) {
if (partnerBuilder_ == null) {
if (partner_ != null) {
partner_ =
io.bloombox.schema.partner.PartnerAccount.Partner.newBuilder(partner_).mergeFrom(value).buildPartial();
} else {
partner_ = value;
}
onChanged();
} else {
partnerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public Builder clearPartner() {
if (partnerBuilder_ == null) {
partner_ = null;
onChanged();
} else {
partner_ = null;
partnerBuilder_ = null;
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner.Builder getPartnerBuilder() {
onChanged();
return getPartnerFieldBuilder().getBuilder();
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
public io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder() {
if (partnerBuilder_ != null) {
return partnerBuilder_.getMessageOrBuilder();
} else {
return partner_ == null ?
io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
}
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder>
getPartnerFieldBuilder() {
if (partnerBuilder_ == null) {
partnerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder>(
getPartner(),
getParentForChildren(),
isClean());
partner_ = null;
}
return partnerBuilder_;
}
private io.opencannabis.schema.commerce.CommercialOrder.Order order_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder> orderBuilder_;
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public boolean hasOrder() {
return orderBuilder_ != null || order_ != null;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order getOrder() {
if (orderBuilder_ == null) {
return order_ == null ? io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
} else {
return orderBuilder_.getMessage();
}
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public Builder setOrder(io.opencannabis.schema.commerce.CommercialOrder.Order value) {
if (orderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
order_ = value;
onChanged();
} else {
orderBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public Builder setOrder(
io.opencannabis.schema.commerce.CommercialOrder.Order.Builder builderForValue) {
if (orderBuilder_ == null) {
order_ = builderForValue.build();
onChanged();
} else {
orderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public Builder mergeOrder(io.opencannabis.schema.commerce.CommercialOrder.Order value) {
if (orderBuilder_ == null) {
if (order_ != null) {
order_ =
io.opencannabis.schema.commerce.CommercialOrder.Order.newBuilder(order_).mergeFrom(value).buildPartial();
} else {
order_ = value;
}
onChanged();
} else {
orderBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public Builder clearOrder() {
if (orderBuilder_ == null) {
order_ = null;
onChanged();
} else {
order_ = null;
orderBuilder_ = null;
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order.Builder getOrderBuilder() {
onChanged();
return getOrderFieldBuilder().getBuilder();
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder() {
if (orderBuilder_ != null) {
return orderBuilder_.getMessageOrBuilder();
} else {
return order_ == null ?
io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
}
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder>
getOrderFieldBuilder() {
if (orderBuilder_ == null) {
orderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder>(
getOrder(),
getParentForChildren(),
isClean());
order_ = null;
}
return orderBuilder_;
}
private java.lang.Object trackingUrl_ = "";
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public java.lang.String getTrackingUrl() {
java.lang.Object ref = trackingUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
trackingUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public com.google.protobuf.ByteString
getTrackingUrlBytes() {
java.lang.Object ref = trackingUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
trackingUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public Builder setTrackingUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
trackingUrl_ = value;
onChanged();
return this;
}
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public Builder clearTrackingUrl() {
trackingUrl_ = getDefaultInstance().getTrackingUrl();
onChanged();
return this;
}
/**
*
* Order status/tracking URL.
*
*
* string tracking_url = 6;
*/
public Builder setTrackingUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
trackingUrl_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata.GoToTrack)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata.GoToTrack)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GoToTrack parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GoToTrack(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OneClickActionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata.OneClickAction)
com.google.protobuf.MessageOrBuilder {
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
int getTypeValue();
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType getType();
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
java.lang.String getName();
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
java.lang.String getTarget();
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
com.google.protobuf.ByteString
getTargetBytes();
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
java.lang.String getDescription();
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
*
* Specifies the structure of an attached One-Click action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.OneClickAction}
*/
public static final class OneClickAction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata.OneClickAction)
OneClickActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use OneClickAction.newBuilder() to construct.
private OneClickAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OneClickAction() {
type_ = 0;
name_ = "";
target_ = "";
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OneClickAction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
target_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OneClickAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder.class);
}
/**
*
* Specifies known types of one-click actions.
*
*
* Protobuf enum {@code bloombox.comms.EmailMetadata.OneClickAction.ActionType}
*/
public enum ActionType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Confirmation-style action.
*
*
* CONFIRM = 0;
*/
CONFIRM(0),
/**
*
* Save-style action.
*
*
* SAVE = 1;
*/
SAVE(1),
/**
*
* RSVP for an event.
*
*
* RSVP = 2;
*/
RSVP(2),
UNRECOGNIZED(-1),
;
/**
*
* Confirmation-style action.
*
*
* CONFIRM = 0;
*/
public static final int CONFIRM_VALUE = 0;
/**
*
* Save-style action.
*
*
* SAVE = 1;
*/
public static final int SAVE_VALUE = 1;
/**
*
* RSVP for an event.
*
*
* RSVP = 2;
*/
public static final int RSVP_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActionType valueOf(int value) {
return forNumber(value);
}
public static ActionType forNumber(int value) {
switch (value) {
case 0: return CONFIRM;
case 1: return SAVE;
case 2: return RSVP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ActionType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ActionType findValueByNumber(int number) {
return ActionType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDescriptor().getEnumTypes().get(0);
}
private static final ActionType[] VALUES = values();
public static ActionType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ActionType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.comms.EmailMetadata.OneClickAction.ActionType)
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType result = io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.valueOf(type_);
return result == null ? io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.UNRECOGNIZED : result;
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TARGET_FIELD_NUMBER = 3;
private volatile java.lang.Object target_;
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public java.lang.String getTarget() {
java.lang.Object ref = target_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
target_ = s;
return s;
}
}
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public com.google.protobuf.ByteString
getTargetBytes() {
java.lang.Object ref = target_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
target_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 4;
private volatile java.lang.Object description_;
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.CONFIRM.getNumber()) {
output.writeEnum(1, type_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (!getTargetBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, target_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, description_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.CONFIRM.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (!getTargetBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, target_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, description_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction other = (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) obj;
if (type_ != other.type_) return false;
if (!getName()
.equals(other.getName())) return false;
if (!getTarget()
.equals(other.getTarget())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + TARGET_FIELD_NUMBER;
hash = (53 * hash) + getTarget().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of an attached One-Click action.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.OneClickAction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata.OneClickAction)
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OneClickAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
name_ = "";
target_ = "";
description_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction result = new io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction(this);
result.type_ = type_;
result.name_ = name_;
result.target_ = target_;
result.description_ = description_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getTarget().isEmpty()) {
target_ = other.target_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int type_ = 0;
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType result = io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.valueOf(type_);
return result == null ? io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType.UNRECOGNIZED : result;
}
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public Builder setType(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.ActionType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Type of one-click-action to attach.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction.ActionType type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name for the action payload.
*
*
* string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object target_ = "";
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public java.lang.String getTarget() {
java.lang.Object ref = target_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
target_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public com.google.protobuf.ByteString
getTargetBytes() {
java.lang.Object ref = target_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
target_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public Builder setTarget(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
return this;
}
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public Builder clearTarget() {
target_ = getDefaultInstance().getTarget();
onChanged();
return this;
}
/**
*
* Action URL/target.
*
*
* string target = 3;
*/
public Builder setTargetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
target_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Narrative description for the action.
*
*
* string description = 4;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata.OneClickAction)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata.OneClickAction)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OneClickAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OneClickAction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata.OrderMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
boolean hasPartner();
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
io.bloombox.schema.partner.PartnerAccount.Partner getPartner();
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
boolean hasOrder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
io.opencannabis.schema.commerce.CommercialOrder.Order getOrder();
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder();
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
java.lang.String getCurrency();
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
com.google.protobuf.ByteString
getCurrencyBytes();
/**
*
* Subtotal value for the entire order.
*
*
* double subtotal = 4;
*/
double getSubtotal();
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
java.lang.String getStatusUrl();
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
com.google.protobuf.ByteString
getStatusUrlBytes();
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
java.lang.String getMobileUrl();
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
com.google.protobuf.ByteString
getMobileUrlBytes();
}
/**
*
* Specifies the structure of attached order metadata.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.OrderMetadata}
*/
public static final class OrderMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata.OrderMetadata)
OrderMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use OrderMetadata.newBuilder() to construct.
private OrderMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OrderMetadata() {
currency_ = "";
statusUrl_ = "";
mobileUrl_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OrderMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.partner.PartnerAccount.Partner.Builder subBuilder = null;
if (partner_ != null) {
subBuilder = partner_.toBuilder();
}
partner_ = input.readMessage(io.bloombox.schema.partner.PartnerAccount.Partner.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(partner_);
partner_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.commerce.CommercialOrder.Order.Builder subBuilder = null;
if (order_ != null) {
subBuilder = order_.toBuilder();
}
order_ = input.readMessage(io.opencannabis.schema.commerce.CommercialOrder.Order.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(order_);
order_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
currency_ = s;
break;
}
case 33: {
subtotal_ = input.readDouble();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
statusUrl_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
mobileUrl_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OrderMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder.class);
}
public static final int PARTNER_FIELD_NUMBER = 1;
private io.bloombox.schema.partner.PartnerAccount.Partner partner_;
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public boolean hasPartner() {
return partner_ != null;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner getPartner() {
return partner_ == null ? io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder() {
return getPartner();
}
public static final int ORDER_FIELD_NUMBER = 2;
private io.opencannabis.schema.commerce.CommercialOrder.Order order_;
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public boolean hasOrder() {
return order_ != null;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order getOrder() {
return order_ == null ? io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder() {
return getOrder();
}
public static final int CURRENCY_FIELD_NUMBER = 3;
private volatile java.lang.Object currency_;
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public java.lang.String getCurrency() {
java.lang.Object ref = currency_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency_ = s;
return s;
}
}
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public com.google.protobuf.ByteString
getCurrencyBytes() {
java.lang.Object ref = currency_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBTOTAL_FIELD_NUMBER = 4;
private double subtotal_;
/**
*
* Subtotal value for the entire order.
*
*
* double subtotal = 4;
*/
public double getSubtotal() {
return subtotal_;
}
public static final int STATUS_URL_FIELD_NUMBER = 5;
private volatile java.lang.Object statusUrl_;
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public java.lang.String getStatusUrl() {
java.lang.Object ref = statusUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
statusUrl_ = s;
return s;
}
}
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public com.google.protobuf.ByteString
getStatusUrlBytes() {
java.lang.Object ref = statusUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statusUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MOBILE_URL_FIELD_NUMBER = 6;
private volatile java.lang.Object mobileUrl_;
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public java.lang.String getMobileUrl() {
java.lang.Object ref = mobileUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mobileUrl_ = s;
return s;
}
}
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public com.google.protobuf.ByteString
getMobileUrlBytes() {
java.lang.Object ref = mobileUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mobileUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (partner_ != null) {
output.writeMessage(1, getPartner());
}
if (order_ != null) {
output.writeMessage(2, getOrder());
}
if (!getCurrencyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, currency_);
}
if (subtotal_ != 0D) {
output.writeDouble(4, subtotal_);
}
if (!getStatusUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, statusUrl_);
}
if (!getMobileUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, mobileUrl_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (partner_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPartner());
}
if (order_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getOrder());
}
if (!getCurrencyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, currency_);
}
if (subtotal_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, subtotal_);
}
if (!getStatusUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, statusUrl_);
}
if (!getMobileUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, mobileUrl_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata other = (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) obj;
if (hasPartner() != other.hasPartner()) return false;
if (hasPartner()) {
if (!getPartner()
.equals(other.getPartner())) return false;
}
if (hasOrder() != other.hasOrder()) return false;
if (hasOrder()) {
if (!getOrder()
.equals(other.getOrder())) return false;
}
if (!getCurrency()
.equals(other.getCurrency())) return false;
if (java.lang.Double.doubleToLongBits(getSubtotal())
!= java.lang.Double.doubleToLongBits(
other.getSubtotal())) return false;
if (!getStatusUrl()
.equals(other.getStatusUrl())) return false;
if (!getMobileUrl()
.equals(other.getMobileUrl())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPartner()) {
hash = (37 * hash) + PARTNER_FIELD_NUMBER;
hash = (53 * hash) + getPartner().hashCode();
}
if (hasOrder()) {
hash = (37 * hash) + ORDER_FIELD_NUMBER;
hash = (53 * hash) + getOrder().hashCode();
}
hash = (37 * hash) + CURRENCY_FIELD_NUMBER;
hash = (53 * hash) + getCurrency().hashCode();
hash = (37 * hash) + SUBTOTAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSubtotal()));
hash = (37 * hash) + STATUS_URL_FIELD_NUMBER;
hash = (53 * hash) + getStatusUrl().hashCode();
hash = (37 * hash) + MOBILE_URL_FIELD_NUMBER;
hash = (53 * hash) + getMobileUrl().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of attached order metadata.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.OrderMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata.OrderMetadata)
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OrderMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (partnerBuilder_ == null) {
partner_ = null;
} else {
partner_ = null;
partnerBuilder_ = null;
}
if (orderBuilder_ == null) {
order_ = null;
} else {
order_ = null;
orderBuilder_ = null;
}
currency_ = "";
subtotal_ = 0D;
statusUrl_ = "";
mobileUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata result = new io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata(this);
if (partnerBuilder_ == null) {
result.partner_ = partner_;
} else {
result.partner_ = partnerBuilder_.build();
}
if (orderBuilder_ == null) {
result.order_ = order_;
} else {
result.order_ = orderBuilder_.build();
}
result.currency_ = currency_;
result.subtotal_ = subtotal_;
result.statusUrl_ = statusUrl_;
result.mobileUrl_ = mobileUrl_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance()) return this;
if (other.hasPartner()) {
mergePartner(other.getPartner());
}
if (other.hasOrder()) {
mergeOrder(other.getOrder());
}
if (!other.getCurrency().isEmpty()) {
currency_ = other.currency_;
onChanged();
}
if (other.getSubtotal() != 0D) {
setSubtotal(other.getSubtotal());
}
if (!other.getStatusUrl().isEmpty()) {
statusUrl_ = other.statusUrl_;
onChanged();
}
if (!other.getMobileUrl().isEmpty()) {
mobileUrl_ = other.mobileUrl_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.partner.PartnerAccount.Partner partner_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder> partnerBuilder_;
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public boolean hasPartner() {
return partnerBuilder_ != null || partner_ != null;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner getPartner() {
if (partnerBuilder_ == null) {
return partner_ == null ? io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
} else {
return partnerBuilder_.getMessage();
}
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public Builder setPartner(io.bloombox.schema.partner.PartnerAccount.Partner value) {
if (partnerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partner_ = value;
onChanged();
} else {
partnerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public Builder setPartner(
io.bloombox.schema.partner.PartnerAccount.Partner.Builder builderForValue) {
if (partnerBuilder_ == null) {
partner_ = builderForValue.build();
onChanged();
} else {
partnerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public Builder mergePartner(io.bloombox.schema.partner.PartnerAccount.Partner value) {
if (partnerBuilder_ == null) {
if (partner_ != null) {
partner_ =
io.bloombox.schema.partner.PartnerAccount.Partner.newBuilder(partner_).mergeFrom(value).buildPartial();
} else {
partner_ = value;
}
onChanged();
} else {
partnerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public Builder clearPartner() {
if (partnerBuilder_ == null) {
partner_ = null;
onChanged();
} else {
partner_ = null;
partnerBuilder_ = null;
}
return this;
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public io.bloombox.schema.partner.PartnerAccount.Partner.Builder getPartnerBuilder() {
onChanged();
return getPartnerFieldBuilder().getBuilder();
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
public io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder getPartnerOrBuilder() {
if (partnerBuilder_ != null) {
return partnerBuilder_.getMessageOrBuilder();
} else {
return partner_ == null ?
io.bloombox.schema.partner.PartnerAccount.Partner.getDefaultInstance() : partner_;
}
}
/**
*
* Specifies info about the partner fulfilling the order.
*
*
* .bloombox.partner.Partner partner = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder>
getPartnerFieldBuilder() {
if (partnerBuilder_ == null) {
partnerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerAccount.Partner, io.bloombox.schema.partner.PartnerAccount.Partner.Builder, io.bloombox.schema.partner.PartnerAccount.PartnerOrBuilder>(
getPartner(),
getParentForChildren(),
isClean());
partner_ = null;
}
return partnerBuilder_;
}
private io.opencannabis.schema.commerce.CommercialOrder.Order order_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder> orderBuilder_;
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public boolean hasOrder() {
return orderBuilder_ != null || order_ != null;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order getOrder() {
if (orderBuilder_ == null) {
return order_ == null ? io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
} else {
return orderBuilder_.getMessage();
}
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public Builder setOrder(io.opencannabis.schema.commerce.CommercialOrder.Order value) {
if (orderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
order_ = value;
onChanged();
} else {
orderBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public Builder setOrder(
io.opencannabis.schema.commerce.CommercialOrder.Order.Builder builderForValue) {
if (orderBuilder_ == null) {
order_ = builderForValue.build();
onChanged();
} else {
orderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public Builder mergeOrder(io.opencannabis.schema.commerce.CommercialOrder.Order value) {
if (orderBuilder_ == null) {
if (order_ != null) {
order_ =
io.opencannabis.schema.commerce.CommercialOrder.Order.newBuilder(order_).mergeFrom(value).buildPartial();
} else {
order_ = value;
}
onChanged();
} else {
orderBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public Builder clearOrder() {
if (orderBuilder_ == null) {
order_ = null;
onChanged();
} else {
order_ = null;
orderBuilder_ = null;
}
return this;
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public io.opencannabis.schema.commerce.CommercialOrder.Order.Builder getOrderBuilder() {
onChanged();
return getOrderFieldBuilder().getBuilder();
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder getOrderOrBuilder() {
if (orderBuilder_ != null) {
return orderBuilder_.getMessageOrBuilder();
} else {
return order_ == null ?
io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance() : order_;
}
}
/**
*
* Specifies the order attached to this notification.
*
*
* .opencannabis.commerce.Order order = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder>
getOrderFieldBuilder() {
if (orderBuilder_ == null) {
orderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.Order, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder>(
getOrder(),
getParentForChildren(),
isClean());
order_ = null;
}
return orderBuilder_;
}
private java.lang.Object currency_ = "";
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public java.lang.String getCurrency() {
java.lang.Object ref = currency_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public com.google.protobuf.ByteString
getCurrencyBytes() {
java.lang.Object ref = currency_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public Builder setCurrency(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
currency_ = value;
onChanged();
return this;
}
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public Builder clearCurrency() {
currency_ = getDefaultInstance().getCurrency();
onChanged();
return this;
}
/**
*
* Type of currency for this metadata.
*
*
* string currency = 3;
*/
public Builder setCurrencyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
currency_ = value;
onChanged();
return this;
}
private double subtotal_ ;
/**
*
* Subtotal value for the entire order.
*
*
* double subtotal = 4;
*/
public double getSubtotal() {
return subtotal_;
}
/**
*
* Subtotal value for the entire order.
*
*
* double subtotal = 4;
*/
public Builder setSubtotal(double value) {
subtotal_ = value;
onChanged();
return this;
}
/**
*
* Subtotal value for the entire order.
*
*
* double subtotal = 4;
*/
public Builder clearSubtotal() {
subtotal_ = 0D;
onChanged();
return this;
}
private java.lang.Object statusUrl_ = "";
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public java.lang.String getStatusUrl() {
java.lang.Object ref = statusUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
statusUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public com.google.protobuf.ByteString
getStatusUrlBytes() {
java.lang.Object ref = statusUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statusUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public Builder setStatusUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
statusUrl_ = value;
onChanged();
return this;
}
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public Builder clearStatusUrl() {
statusUrl_ = getDefaultInstance().getStatusUrl();
onChanged();
return this;
}
/**
*
* URL to view status for an order.
*
*
* string status_url = 5;
*/
public Builder setStatusUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
statusUrl_ = value;
onChanged();
return this;
}
private java.lang.Object mobileUrl_ = "";
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public java.lang.String getMobileUrl() {
java.lang.Object ref = mobileUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mobileUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public com.google.protobuf.ByteString
getMobileUrlBytes() {
java.lang.Object ref = mobileUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mobileUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public Builder setMobileUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mobileUrl_ = value;
onChanged();
return this;
}
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public Builder clearMobileUrl() {
mobileUrl_ = getDefaultInstance().getMobileUrl();
onChanged();
return this;
}
/**
*
* URL to open status on mobile.
*
*
* string mobile_url = 6;
*/
public Builder setMobileUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mobileUrl_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata.OrderMetadata)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata.OrderMetadata)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OrderMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OrderMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SchemaBlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailMetadata.SchemaBlock)
com.google.protobuf.MessageOrBuilder {
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
int getTypeValue();
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.Type getType();
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
boolean hasView();
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getView();
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder getViewOrBuilder();
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
boolean hasTrack();
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getTrack();
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder getTrackOrBuilder();
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
boolean hasOneClick();
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getOneClick();
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder getOneClickOrBuilder();
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
boolean hasOrder();
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getOrder();
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder getOrderOrBuilder();
public io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.BlockCase getBlockCase();
}
/**
*
* Individual block of schema-based metadata.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.SchemaBlock}
*/
public static final class SchemaBlock extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailMetadata.SchemaBlock)
SchemaBlockOrBuilder {
private static final long serialVersionUID = 0L;
// Use SchemaBlock.newBuilder() to construct.
private SchemaBlock(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SchemaBlock() {
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SchemaBlock(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 82: {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder subBuilder = null;
if (blockCase_ == 10) {
subBuilder = ((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_).toBuilder();
}
block_ =
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_);
block_ = subBuilder.buildPartial();
}
blockCase_ = 10;
break;
}
case 122: {
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder subBuilder = null;
if (blockCase_ == 15) {
subBuilder = ((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_).toBuilder();
}
block_ =
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_);
block_ = subBuilder.buildPartial();
}
blockCase_ = 15;
break;
}
case 162: {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder subBuilder = null;
if (blockCase_ == 20) {
subBuilder = ((io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_).toBuilder();
}
block_ =
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_);
block_ = subBuilder.buildPartial();
}
blockCase_ = 20;
break;
}
case 242: {
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder subBuilder = null;
if (blockCase_ == 30) {
subBuilder = ((io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_).toBuilder();
}
block_ =
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_);
block_ = subBuilder.buildPartial();
}
blockCase_ = 30;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_SchemaBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.Builder.class);
}
private int blockCase_ = 0;
private java.lang.Object block_;
public enum BlockCase
implements com.google.protobuf.Internal.EnumLite {
VIEW(10),
TRACK(15),
ONE_CLICK(20),
ORDER(30),
BLOCK_NOT_SET(0);
private final int value;
private BlockCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BlockCase valueOf(int value) {
return forNumber(value);
}
public static BlockCase forNumber(int value) {
switch (value) {
case 10: return VIEW;
case 15: return TRACK;
case 20: return ONE_CLICK;
case 30: return ORDER;
case 0: return BLOCK_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public BlockCase
getBlockCase() {
return BlockCase.forNumber(
blockCase_);
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.Type getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.EmailComms.EmailMetadata.Type result = io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.valueOf(type_);
return result == null ? io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.UNRECOGNIZED : result;
}
public static final int VIEW_FIELD_NUMBER = 10;
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public boolean hasView() {
return blockCase_ == 10;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getView() {
if (blockCase_ == 10) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder getViewOrBuilder() {
if (blockCase_ == 10) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
public static final int TRACK_FIELD_NUMBER = 15;
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public boolean hasTrack() {
return blockCase_ == 15;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getTrack() {
if (blockCase_ == 15) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder getTrackOrBuilder() {
if (blockCase_ == 15) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
public static final int ONE_CLICK_FIELD_NUMBER = 20;
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public boolean hasOneClick() {
return blockCase_ == 20;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getOneClick() {
if (blockCase_ == 20) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder getOneClickOrBuilder() {
if (blockCase_ == 20) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
public static final int ORDER_FIELD_NUMBER = 30;
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public boolean hasOrder() {
return blockCase_ == 30;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getOrder() {
if (blockCase_ == 30) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder getOrderOrBuilder() {
if (blockCase_ == 30) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.VIEW.getNumber()) {
output.writeEnum(1, type_);
}
if (blockCase_ == 10) {
output.writeMessage(10, (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_);
}
if (blockCase_ == 15) {
output.writeMessage(15, (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_);
}
if (blockCase_ == 20) {
output.writeMessage(20, (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_);
}
if (blockCase_ == 30) {
output.writeMessage(30, (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.VIEW.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (blockCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_);
}
if (blockCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_);
}
if (blockCase_ == 20) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_);
}
if (blockCase_ == 30) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock other = (io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock) obj;
if (type_ != other.type_) return false;
if (!getBlockCase().equals(other.getBlockCase())) return false;
switch (blockCase_) {
case 10:
if (!getView()
.equals(other.getView())) return false;
break;
case 15:
if (!getTrack()
.equals(other.getTrack())) return false;
break;
case 20:
if (!getOneClick()
.equals(other.getOneClick())) return false;
break;
case 30:
if (!getOrder()
.equals(other.getOrder())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
switch (blockCase_) {
case 10:
hash = (37 * hash) + VIEW_FIELD_NUMBER;
hash = (53 * hash) + getView().hashCode();
break;
case 15:
hash = (37 * hash) + TRACK_FIELD_NUMBER;
hash = (53 * hash) + getTrack().hashCode();
break;
case 20:
hash = (37 * hash) + ONE_CLICK_FIELD_NUMBER;
hash = (53 * hash) + getOneClick().hashCode();
break;
case 30:
hash = (37 * hash) + ORDER_FIELD_NUMBER;
hash = (53 * hash) + getOrder().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Individual block of schema-based metadata.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata.SchemaBlock}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata.SchemaBlock)
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlockOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_SchemaBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
blockCase_ = 0;
block_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock result = new io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock(this);
result.type_ = type_;
if (blockCase_ == 10) {
if (viewBuilder_ == null) {
result.block_ = block_;
} else {
result.block_ = viewBuilder_.build();
}
}
if (blockCase_ == 15) {
if (trackBuilder_ == null) {
result.block_ = block_;
} else {
result.block_ = trackBuilder_.build();
}
}
if (blockCase_ == 20) {
if (oneClickBuilder_ == null) {
result.block_ = block_;
} else {
result.block_ = oneClickBuilder_.build();
}
}
if (blockCase_ == 30) {
if (orderBuilder_ == null) {
result.block_ = block_;
} else {
result.block_ = orderBuilder_.build();
}
}
result.blockCase_ = blockCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
switch (other.getBlockCase()) {
case VIEW: {
mergeView(other.getView());
break;
}
case TRACK: {
mergeTrack(other.getTrack());
break;
}
case ONE_CLICK: {
mergeOneClick(other.getOneClick());
break;
}
case ORDER: {
mergeOrder(other.getOrder());
break;
}
case BLOCK_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int blockCase_ = 0;
private java.lang.Object block_;
public BlockCase
getBlockCase() {
return BlockCase.forNumber(
blockCase_);
}
public Builder clearBlock() {
blockCase_ = 0;
block_ = null;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.Type getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.EmailComms.EmailMetadata.Type result = io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.valueOf(type_);
return result == null ? io.bloombox.schema.comms.EmailComms.EmailMetadata.Type.UNRECOGNIZED : result;
}
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public Builder setType(io.bloombox.schema.comms.EmailComms.EmailMetadata.Type value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Type of schema block we're specifying.
*
*
* .bloombox.comms.EmailMetadata.Type type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder> viewBuilder_;
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public boolean hasView() {
return blockCase_ == 10;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView getView() {
if (viewBuilder_ == null) {
if (blockCase_ == 10) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
} else {
if (blockCase_ == 10) {
return viewBuilder_.getMessage();
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public Builder setView(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView value) {
if (viewBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
block_ = value;
onChanged();
} else {
viewBuilder_.setMessage(value);
}
blockCase_ = 10;
return this;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public Builder setView(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder builderForValue) {
if (viewBuilder_ == null) {
block_ = builderForValue.build();
onChanged();
} else {
viewBuilder_.setMessage(builderForValue.build());
}
blockCase_ = 10;
return this;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public Builder mergeView(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView value) {
if (viewBuilder_ == null) {
if (blockCase_ == 10 &&
block_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance()) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.newBuilder((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_)
.mergeFrom(value).buildPartial();
} else {
block_ = value;
}
onChanged();
} else {
if (blockCase_ == 10) {
viewBuilder_.mergeFrom(value);
}
viewBuilder_.setMessage(value);
}
blockCase_ = 10;
return this;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public Builder clearView() {
if (viewBuilder_ == null) {
if (blockCase_ == 10) {
blockCase_ = 0;
block_ = null;
onChanged();
}
} else {
if (blockCase_ == 10) {
blockCase_ = 0;
block_ = null;
}
viewBuilder_.clear();
}
return this;
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder getViewBuilder() {
return getViewFieldBuilder().getBuilder();
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder getViewOrBuilder() {
if ((blockCase_ == 10) && (viewBuilder_ != null)) {
return viewBuilder_.getMessageOrBuilder();
} else {
if (blockCase_ == 10) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
}
/**
*
* Specifies a block for a go-to view action.
*
*
* .bloombox.comms.EmailMetadata.GoToView view = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder>
getViewFieldBuilder() {
if (viewBuilder_ == null) {
if (!(blockCase_ == 10)) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.getDefaultInstance();
}
viewBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToViewOrBuilder>(
(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToView) block_,
getParentForChildren(),
isClean());
block_ = null;
}
blockCase_ = 10;
onChanged();;
return viewBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder> trackBuilder_;
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public boolean hasTrack() {
return blockCase_ == 15;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack getTrack() {
if (trackBuilder_ == null) {
if (blockCase_ == 15) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
} else {
if (blockCase_ == 15) {
return trackBuilder_.getMessage();
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public Builder setTrack(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack value) {
if (trackBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
block_ = value;
onChanged();
} else {
trackBuilder_.setMessage(value);
}
blockCase_ = 15;
return this;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public Builder setTrack(
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder builderForValue) {
if (trackBuilder_ == null) {
block_ = builderForValue.build();
onChanged();
} else {
trackBuilder_.setMessage(builderForValue.build());
}
blockCase_ = 15;
return this;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public Builder mergeTrack(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack value) {
if (trackBuilder_ == null) {
if (blockCase_ == 15 &&
block_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance()) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.newBuilder((io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_)
.mergeFrom(value).buildPartial();
} else {
block_ = value;
}
onChanged();
} else {
if (blockCase_ == 15) {
trackBuilder_.mergeFrom(value);
}
trackBuilder_.setMessage(value);
}
blockCase_ = 15;
return this;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public Builder clearTrack() {
if (trackBuilder_ == null) {
if (blockCase_ == 15) {
blockCase_ = 0;
block_ = null;
onChanged();
}
} else {
if (blockCase_ == 15) {
blockCase_ = 0;
block_ = null;
}
trackBuilder_.clear();
}
return this;
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder getTrackBuilder() {
return getTrackFieldBuilder().getBuilder();
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder getTrackOrBuilder() {
if ((blockCase_ == 15) && (trackBuilder_ != null)) {
return trackBuilder_.getMessageOrBuilder();
} else {
if (blockCase_ == 15) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
}
/**
*
* Specifies a block for a go-to track action.
*
*
* .bloombox.comms.EmailMetadata.GoToTrack track = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder>
getTrackFieldBuilder() {
if (trackBuilder_ == null) {
if (!(blockCase_ == 15)) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.getDefaultInstance();
}
trackBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrackOrBuilder>(
(io.bloombox.schema.comms.EmailComms.EmailMetadata.GoToTrack) block_,
getParentForChildren(),
isClean());
block_ = null;
}
blockCase_ = 15;
onChanged();;
return trackBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder> oneClickBuilder_;
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public boolean hasOneClick() {
return blockCase_ == 20;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction getOneClick() {
if (oneClickBuilder_ == null) {
if (blockCase_ == 20) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
} else {
if (blockCase_ == 20) {
return oneClickBuilder_.getMessage();
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public Builder setOneClick(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction value) {
if (oneClickBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
block_ = value;
onChanged();
} else {
oneClickBuilder_.setMessage(value);
}
blockCase_ = 20;
return this;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public Builder setOneClick(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder builderForValue) {
if (oneClickBuilder_ == null) {
block_ = builderForValue.build();
onChanged();
} else {
oneClickBuilder_.setMessage(builderForValue.build());
}
blockCase_ = 20;
return this;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public Builder mergeOneClick(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction value) {
if (oneClickBuilder_ == null) {
if (blockCase_ == 20 &&
block_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance()) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.newBuilder((io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_)
.mergeFrom(value).buildPartial();
} else {
block_ = value;
}
onChanged();
} else {
if (blockCase_ == 20) {
oneClickBuilder_.mergeFrom(value);
}
oneClickBuilder_.setMessage(value);
}
blockCase_ = 20;
return this;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public Builder clearOneClick() {
if (oneClickBuilder_ == null) {
if (blockCase_ == 20) {
blockCase_ = 0;
block_ = null;
onChanged();
}
} else {
if (blockCase_ == 20) {
blockCase_ = 0;
block_ = null;
}
oneClickBuilder_.clear();
}
return this;
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder getOneClickBuilder() {
return getOneClickFieldBuilder().getBuilder();
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder getOneClickOrBuilder() {
if ((blockCase_ == 20) && (oneClickBuilder_ != null)) {
return oneClickBuilder_.getMessageOrBuilder();
} else {
if (blockCase_ == 20) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
}
/**
*
* Specifies a block for a one-click action.
*
*
* .bloombox.comms.EmailMetadata.OneClickAction one_click = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder>
getOneClickFieldBuilder() {
if (oneClickBuilder_ == null) {
if (!(blockCase_ == 20)) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.getDefaultInstance();
}
oneClickBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickActionOrBuilder>(
(io.bloombox.schema.comms.EmailComms.EmailMetadata.OneClickAction) block_,
getParentForChildren(),
isClean());
block_ = null;
}
blockCase_ = 20;
onChanged();;
return oneClickBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder> orderBuilder_;
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public boolean hasOrder() {
return blockCase_ == 30;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata getOrder() {
if (orderBuilder_ == null) {
if (blockCase_ == 30) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
} else {
if (blockCase_ == 30) {
return orderBuilder_.getMessage();
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public Builder setOrder(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata value) {
if (orderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
block_ = value;
onChanged();
} else {
orderBuilder_.setMessage(value);
}
blockCase_ = 30;
return this;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public Builder setOrder(
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder builderForValue) {
if (orderBuilder_ == null) {
block_ = builderForValue.build();
onChanged();
} else {
orderBuilder_.setMessage(builderForValue.build());
}
blockCase_ = 30;
return this;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public Builder mergeOrder(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata value) {
if (orderBuilder_ == null) {
if (blockCase_ == 30 &&
block_ != io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance()) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.newBuilder((io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_)
.mergeFrom(value).buildPartial();
} else {
block_ = value;
}
onChanged();
} else {
if (blockCase_ == 30) {
orderBuilder_.mergeFrom(value);
}
orderBuilder_.setMessage(value);
}
blockCase_ = 30;
return this;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public Builder clearOrder() {
if (orderBuilder_ == null) {
if (blockCase_ == 30) {
blockCase_ = 0;
block_ = null;
onChanged();
}
} else {
if (blockCase_ == 30) {
blockCase_ = 0;
block_ = null;
}
orderBuilder_.clear();
}
return this;
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder getOrderBuilder() {
return getOrderFieldBuilder().getBuilder();
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
public io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder getOrderOrBuilder() {
if ((blockCase_ == 30) && (orderBuilder_ != null)) {
return orderBuilder_.getMessageOrBuilder();
} else {
if (blockCase_ == 30) {
return (io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_;
}
return io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
}
/**
*
* Specifies a block for order metadata.
*
*
* .bloombox.comms.EmailMetadata.OrderMetadata order = 30;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder>
getOrderFieldBuilder() {
if (orderBuilder_ == null) {
if (!(blockCase_ == 30)) {
block_ = io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.getDefaultInstance();
}
orderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata.Builder, io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadataOrBuilder>(
(io.bloombox.schema.comms.EmailComms.EmailMetadata.OrderMetadata) block_,
getParentForChildren(),
isClean());
block_ = null;
}
blockCase_ = 30;
onChanged();;
return orderBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata.SchemaBlock)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata.SchemaBlock)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SchemaBlock parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SchemaBlock(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata.SchemaBlock getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailMetadata other = (io.bloombox.schema.comms.EmailComms.EmailMetadata) obj;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies schema-based metadata appended to a message.
*
*
* Protobuf type {@code bloombox.comms.EmailMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailMetadata)
io.bloombox.schema.comms.EmailComms.EmailMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailMetadata.class, io.bloombox.schema.comms.EmailComms.EmailMetadata.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailMetadata_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailMetadata.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata build() {
io.bloombox.schema.comms.EmailComms.EmailMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailMetadata result = new io.bloombox.schema.comms.EmailComms.EmailMetadata(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailMetadata) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailMetadata other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailMetadata.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailMetadata)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailMetadata)
private static final io.bloombox.schema.comms.EmailComms.EmailMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailMetadata();
}
public static io.bloombox.schema.comms.EmailComms.EmailMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailTransmissionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailTransmission)
com.google.protobuf.MessageOrBuilder {
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
boolean hasMessage();
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailMessage getMessage();
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder getMessageOrBuilder();
}
/**
*
* Specifies the structure of a transmission operation for an individual email message.
*
*
* Protobuf type {@code bloombox.comms.EmailTransmission}
*/
public static final class EmailTransmission extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailTransmission)
EmailTransmissionOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailTransmission.newBuilder() to construct.
private EmailTransmission(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailTransmission() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailTransmission(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.comms.EmailComms.EmailMessage.Builder subBuilder = null;
if (message_ != null) {
subBuilder = message_.toBuilder();
}
message_ = input.readMessage(io.bloombox.schema.comms.EmailComms.EmailMessage.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(message_);
message_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailTransmission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailTransmission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailTransmission.class, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder.class);
}
public static final int MESSAGE_FIELD_NUMBER = 1;
private io.bloombox.schema.comms.EmailComms.EmailMessage message_;
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public boolean hasMessage() {
return message_ != null;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMessage getMessage() {
return message_ == null ? io.bloombox.schema.comms.EmailComms.EmailMessage.getDefaultInstance() : message_;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder getMessageOrBuilder() {
return getMessage();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (message_ != null) {
output.writeMessage(1, getMessage());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (message_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMessage());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailTransmission)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailTransmission other = (io.bloombox.schema.comms.EmailComms.EmailTransmission) obj;
if (hasMessage() != other.hasMessage()) return false;
if (hasMessage()) {
if (!getMessage()
.equals(other.getMessage())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMessage()) {
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailTransmission prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of a transmission operation for an individual email message.
*
*
* Protobuf type {@code bloombox.comms.EmailTransmission}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailTransmission)
io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailTransmission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailTransmission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailTransmission.class, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailTransmission.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (messageBuilder_ == null) {
message_ = null;
} else {
message_ = null;
messageBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailTransmission_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailTransmission getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailTransmission build() {
io.bloombox.schema.comms.EmailComms.EmailTransmission result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailTransmission buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailTransmission result = new io.bloombox.schema.comms.EmailComms.EmailTransmission(this);
if (messageBuilder_ == null) {
result.message_ = message_;
} else {
result.message_ = messageBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailTransmission) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailTransmission)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailTransmission other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance()) return this;
if (other.hasMessage()) {
mergeMessage(other.getMessage());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailTransmission parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailTransmission) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.comms.EmailComms.EmailMessage message_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMessage, io.bloombox.schema.comms.EmailComms.EmailMessage.Builder, io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder> messageBuilder_;
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public boolean hasMessage() {
return messageBuilder_ != null || message_ != null;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMessage getMessage() {
if (messageBuilder_ == null) {
return message_ == null ? io.bloombox.schema.comms.EmailComms.EmailMessage.getDefaultInstance() : message_;
} else {
return messageBuilder_.getMessage();
}
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public Builder setMessage(io.bloombox.schema.comms.EmailComms.EmailMessage value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
messageBuilder_.setMessage(value);
}
return this;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public Builder setMessage(
io.bloombox.schema.comms.EmailComms.EmailMessage.Builder builderForValue) {
if (messageBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
messageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public Builder mergeMessage(io.bloombox.schema.comms.EmailComms.EmailMessage value) {
if (messageBuilder_ == null) {
if (message_ != null) {
message_ =
io.bloombox.schema.comms.EmailComms.EmailMessage.newBuilder(message_).mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
messageBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public Builder clearMessage() {
if (messageBuilder_ == null) {
message_ = null;
onChanged();
} else {
message_ = null;
messageBuilder_ = null;
}
return this;
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMessage.Builder getMessageBuilder() {
onChanged();
return getMessageFieldBuilder().getBuilder();
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder getMessageOrBuilder() {
if (messageBuilder_ != null) {
return messageBuilder_.getMessageOrBuilder();
} else {
return message_ == null ?
io.bloombox.schema.comms.EmailComms.EmailMessage.getDefaultInstance() : message_;
}
}
/**
*
* Message payload to transmit in this operation.
*
*
* .bloombox.comms.EmailMessage message = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMessage, io.bloombox.schema.comms.EmailComms.EmailMessage.Builder, io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder>
getMessageFieldBuilder() {
if (messageBuilder_ == null) {
messageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailMessage, io.bloombox.schema.comms.EmailComms.EmailMessage.Builder, io.bloombox.schema.comms.EmailComms.EmailMessageOrBuilder>(
getMessage(),
getParentForChildren(),
isClean());
message_ = null;
}
return messageBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailTransmission)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailTransmission)
private static final io.bloombox.schema.comms.EmailComms.EmailTransmission DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailTransmission();
}
public static io.bloombox.schema.comms.EmailComms.EmailTransmission getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailTransmission parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailTransmission(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailTransmission getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailBatchOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailBatch)
com.google.protobuf.MessageOrBuilder {
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
java.util.List
getOpList();
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailTransmission getOp(int index);
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
int getOpCount();
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
java.util.List extends io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>
getOpOrBuilderList();
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getOpOrBuilder(
int index);
}
/**
*
* Specifies the structure of a batch of email transmission operations.
*
*
* Protobuf type {@code bloombox.comms.EmailBatch}
*/
public static final class EmailBatch extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailBatch)
EmailBatchOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailBatch.newBuilder() to construct.
private EmailBatch(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailBatch() {
op_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailBatch(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
op_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
op_.add(
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailTransmission.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
op_ = java.util.Collections.unmodifiableList(op_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailBatch_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailBatch_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailBatch.class, io.bloombox.schema.comms.EmailComms.EmailBatch.Builder.class);
}
public static final int OP_FIELD_NUMBER = 1;
private java.util.List op_;
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public java.util.List getOpList() {
return op_;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public java.util.List extends io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>
getOpOrBuilderList() {
return op_;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public int getOpCount() {
return op_.size();
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission getOp(int index) {
return op_.get(index);
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getOpOrBuilder(
int index) {
return op_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < op_.size(); i++) {
output.writeMessage(1, op_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < op_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, op_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailBatch)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailBatch other = (io.bloombox.schema.comms.EmailComms.EmailBatch) obj;
if (!getOpList()
.equals(other.getOpList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getOpCount() > 0) {
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + getOpList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailBatch prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of a batch of email transmission operations.
*
*
* Protobuf type {@code bloombox.comms.EmailBatch}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailBatch)
io.bloombox.schema.comms.EmailComms.EmailBatchOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailBatch_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailBatch_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailBatch.class, io.bloombox.schema.comms.EmailComms.EmailBatch.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailBatch.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOpFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (opBuilder_ == null) {
op_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
opBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailBatch_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailBatch getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailBatch.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailBatch build() {
io.bloombox.schema.comms.EmailComms.EmailBatch result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailBatch buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailBatch result = new io.bloombox.schema.comms.EmailComms.EmailBatch(this);
int from_bitField0_ = bitField0_;
if (opBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
op_ = java.util.Collections.unmodifiableList(op_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.op_ = op_;
} else {
result.op_ = opBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailBatch) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailBatch)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailBatch other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailBatch.getDefaultInstance()) return this;
if (opBuilder_ == null) {
if (!other.op_.isEmpty()) {
if (op_.isEmpty()) {
op_ = other.op_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureOpIsMutable();
op_.addAll(other.op_);
}
onChanged();
}
} else {
if (!other.op_.isEmpty()) {
if (opBuilder_.isEmpty()) {
opBuilder_.dispose();
opBuilder_ = null;
op_ = other.op_;
bitField0_ = (bitField0_ & ~0x00000001);
opBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOpFieldBuilder() : null;
} else {
opBuilder_.addAllMessages(other.op_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailBatch parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailBatch) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List op_ =
java.util.Collections.emptyList();
private void ensureOpIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
op_ = new java.util.ArrayList(op_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder> opBuilder_;
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public java.util.List getOpList() {
if (opBuilder_ == null) {
return java.util.Collections.unmodifiableList(op_);
} else {
return opBuilder_.getMessageList();
}
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public int getOpCount() {
if (opBuilder_ == null) {
return op_.size();
} else {
return opBuilder_.getCount();
}
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission getOp(int index) {
if (opBuilder_ == null) {
return op_.get(index);
} else {
return opBuilder_.getMessage(index);
}
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder setOp(
int index, io.bloombox.schema.comms.EmailComms.EmailTransmission value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.set(index, value);
onChanged();
} else {
opBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder setOp(
int index, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.set(index, builderForValue.build());
onChanged();
} else {
opBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder addOp(io.bloombox.schema.comms.EmailComms.EmailTransmission value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.add(value);
onChanged();
} else {
opBuilder_.addMessage(value);
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder addOp(
int index, io.bloombox.schema.comms.EmailComms.EmailTransmission value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOpIsMutable();
op_.add(index, value);
onChanged();
} else {
opBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder addOp(
io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.add(builderForValue.build());
onChanged();
} else {
opBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder addOp(
int index, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder builderForValue) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.add(index, builderForValue.build());
onChanged();
} else {
opBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder addAllOp(
java.lang.Iterable extends io.bloombox.schema.comms.EmailComms.EmailTransmission> values) {
if (opBuilder_ == null) {
ensureOpIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, op_);
onChanged();
} else {
opBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder clearOp() {
if (opBuilder_ == null) {
op_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
opBuilder_.clear();
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public Builder removeOp(int index) {
if (opBuilder_ == null) {
ensureOpIsMutable();
op_.remove(index);
onChanged();
} else {
opBuilder_.remove(index);
}
return this;
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder getOpBuilder(
int index) {
return getOpFieldBuilder().getBuilder(index);
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder getOpOrBuilder(
int index) {
if (opBuilder_ == null) {
return op_.get(index); } else {
return opBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public java.util.List extends io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>
getOpOrBuilderList() {
if (opBuilder_ != null) {
return opBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(op_);
}
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder addOpBuilder() {
return getOpFieldBuilder().addBuilder(
io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance());
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder addOpBuilder(
int index) {
return getOpFieldBuilder().addBuilder(
index, io.bloombox.schema.comms.EmailComms.EmailTransmission.getDefaultInstance());
}
/**
*
* Transmission operations for this batch.
*
*
* repeated .bloombox.comms.EmailTransmission op = 1;
*/
public java.util.List
getOpBuilderList() {
return getOpFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>
getOpFieldBuilder() {
if (opBuilder_ == null) {
opBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailTransmission, io.bloombox.schema.comms.EmailComms.EmailTransmission.Builder, io.bloombox.schema.comms.EmailComms.EmailTransmissionOrBuilder>(
op_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
op_ = null;
}
return opBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailBatch)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailBatch)
private static final io.bloombox.schema.comms.EmailComms.EmailBatch DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailBatch();
}
public static io.bloombox.schema.comms.EmailComms.EmailBatch getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailBatch parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailBatch(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailBatch getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailSenderOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailSender)
com.google.protobuf.MessageOrBuilder {
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
java.util.List getRoleList();
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
int getRoleCount();
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailSender.Role getRole(int index);
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
java.util.List
getRoleValueList();
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
int getRoleValue(int index);
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
boolean hasContact();
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddress getContact();
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getContactOrBuilder();
}
/**
*
* Specifies an email sender record.
*
*
* Protobuf type {@code bloombox.comms.EmailSender}
*/
public static final class EmailSender extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailSender)
EmailSenderOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailSender.newBuilder() to construct.
private EmailSender(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailSender() {
role_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailSender(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
role_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
role_.add(rawValue);
break;
}
case 10: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
role_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
role_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 18: {
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder subBuilder = null;
if (contact_ != null) {
subBuilder = contact_.toBuilder();
}
contact_ = input.readMessage(io.opencannabis.schema.contact.ContactEmail.EmailAddress.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(contact_);
contact_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
role_ = java.util.Collections.unmodifiableList(role_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSender_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSender_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailSender.class, io.bloombox.schema.comms.EmailComms.EmailSender.Builder.class);
}
/**
*
* Enumerates available roles for an email contact.
*
*
* Protobuf enum {@code bloombox.comms.EmailSender.Role}
*/
public enum Role
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Main sender identity.
*
*
* SENDER = 0;
*/
SENDER(0),
/**
*
* Always include as CC.
*
*
* CC = 1;
*/
CC(1),
/**
*
* Always include as BCC.
*
*
* BCC = 2;
*/
BCC(2),
/**
*
* Use as the reply-to.
*
*
* REPLY_TO = 3;
*/
REPLY_TO(3),
UNRECOGNIZED(-1),
;
/**
*
* Main sender identity.
*
*
* SENDER = 0;
*/
public static final int SENDER_VALUE = 0;
/**
*
* Always include as CC.
*
*
* CC = 1;
*/
public static final int CC_VALUE = 1;
/**
*
* Always include as BCC.
*
*
* BCC = 2;
*/
public static final int BCC_VALUE = 2;
/**
*
* Use as the reply-to.
*
*
* REPLY_TO = 3;
*/
public static final int REPLY_TO_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Role valueOf(int value) {
return forNumber(value);
}
public static Role forNumber(int value) {
switch (value) {
case 0: return SENDER;
case 1: return CC;
case 2: return BCC;
case 3: return REPLY_TO;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Role> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Role findValueByNumber(int number) {
return Role.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.EmailSender.getDescriptor().getEnumTypes().get(0);
}
private static final Role[] VALUES = values();
public static Role valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Role(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.comms.EmailSender.Role)
}
private int bitField0_;
public static final int ROLE_FIELD_NUMBER = 1;
private java.util.List role_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.EmailComms.EmailSender.Role> role_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.comms.EmailComms.EmailSender.Role>() {
public io.bloombox.schema.comms.EmailComms.EmailSender.Role convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.comms.EmailComms.EmailSender.Role result = io.bloombox.schema.comms.EmailComms.EmailSender.Role.valueOf(from);
return result == null ? io.bloombox.schema.comms.EmailComms.EmailSender.Role.UNRECOGNIZED : result;
}
};
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public java.util.List getRoleList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.EmailComms.EmailSender.Role>(role_, role_converter_);
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public int getRoleCount() {
return role_.size();
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender.Role getRole(int index) {
return role_converter_.convert(role_.get(index));
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public java.util.List
getRoleValueList() {
return role_;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public int getRoleValue(int index) {
return role_.get(index);
}
private int roleMemoizedSerializedSize;
public static final int CONTACT_FIELD_NUMBER = 2;
private io.opencannabis.schema.contact.ContactEmail.EmailAddress contact_;
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public boolean hasContact() {
return contact_ != null;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getContact() {
return contact_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : contact_;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getContactOrBuilder() {
return getContact();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getRoleList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(roleMemoizedSerializedSize);
}
for (int i = 0; i < role_.size(); i++) {
output.writeEnumNoTag(role_.get(i));
}
if (contact_ != null) {
output.writeMessage(2, getContact());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < role_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(role_.get(i));
}
size += dataSize;
if (!getRoleList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}roleMemoizedSerializedSize = dataSize;
}
if (contact_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getContact());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailSender)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailSender other = (io.bloombox.schema.comms.EmailComms.EmailSender) obj;
if (!role_.equals(other.role_)) return false;
if (hasContact() != other.hasContact()) return false;
if (hasContact()) {
if (!getContact()
.equals(other.getContact())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getRoleCount() > 0) {
hash = (37 * hash) + ROLE_FIELD_NUMBER;
hash = (53 * hash) + role_.hashCode();
}
if (hasContact()) {
hash = (37 * hash) + CONTACT_FIELD_NUMBER;
hash = (53 * hash) + getContact().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSender parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailSender prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies an email sender record.
*
*
* Protobuf type {@code bloombox.comms.EmailSender}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailSender)
io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSender_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSender_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailSender.class, io.bloombox.schema.comms.EmailComms.EmailSender.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailSender.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
role_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
if (contactBuilder_ == null) {
contact_ = null;
} else {
contact_ = null;
contactBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSender_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSender getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailSender.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSender build() {
io.bloombox.schema.comms.EmailComms.EmailSender result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSender buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailSender result = new io.bloombox.schema.comms.EmailComms.EmailSender(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) != 0)) {
role_ = java.util.Collections.unmodifiableList(role_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.role_ = role_;
if (contactBuilder_ == null) {
result.contact_ = contact_;
} else {
result.contact_ = contactBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailSender) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailSender)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailSender other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailSender.getDefaultInstance()) return this;
if (!other.role_.isEmpty()) {
if (role_.isEmpty()) {
role_ = other.role_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRoleIsMutable();
role_.addAll(other.role_);
}
onChanged();
}
if (other.hasContact()) {
mergeContact(other.getContact());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailSender parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailSender) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List role_ =
java.util.Collections.emptyList();
private void ensureRoleIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
role_ = new java.util.ArrayList(role_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public java.util.List getRoleList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.comms.EmailComms.EmailSender.Role>(role_, role_converter_);
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public int getRoleCount() {
return role_.size();
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender.Role getRole(int index) {
return role_converter_.convert(role_.get(index));
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder setRole(
int index, io.bloombox.schema.comms.EmailComms.EmailSender.Role value) {
if (value == null) {
throw new NullPointerException();
}
ensureRoleIsMutable();
role_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder addRole(io.bloombox.schema.comms.EmailComms.EmailSender.Role value) {
if (value == null) {
throw new NullPointerException();
}
ensureRoleIsMutable();
role_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder addAllRole(
java.lang.Iterable extends io.bloombox.schema.comms.EmailComms.EmailSender.Role> values) {
ensureRoleIsMutable();
for (io.bloombox.schema.comms.EmailComms.EmailSender.Role value : values) {
role_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder clearRole() {
role_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public java.util.List
getRoleValueList() {
return java.util.Collections.unmodifiableList(role_);
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public int getRoleValue(int index) {
return role_.get(index);
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder setRoleValue(
int index, int value) {
ensureRoleIsMutable();
role_.set(index, value);
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder addRoleValue(int value) {
ensureRoleIsMutable();
role_.add(value);
onChanged();
return this;
}
/**
*
* Roles for this contact.
*
*
* repeated .bloombox.comms.EmailSender.Role role = 1;
*/
public Builder addAllRoleValue(
java.lang.Iterable values) {
ensureRoleIsMutable();
for (int value : values) {
role_.add(value);
}
onChanged();
return this;
}
private io.opencannabis.schema.contact.ContactEmail.EmailAddress contact_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder> contactBuilder_;
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public boolean hasContact() {
return contactBuilder_ != null || contact_ != null;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress getContact() {
if (contactBuilder_ == null) {
return contact_ == null ? io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : contact_;
} else {
return contactBuilder_.getMessage();
}
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public Builder setContact(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (contactBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
contact_ = value;
onChanged();
} else {
contactBuilder_.setMessage(value);
}
return this;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public Builder setContact(
io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder builderForValue) {
if (contactBuilder_ == null) {
contact_ = builderForValue.build();
onChanged();
} else {
contactBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public Builder mergeContact(io.opencannabis.schema.contact.ContactEmail.EmailAddress value) {
if (contactBuilder_ == null) {
if (contact_ != null) {
contact_ =
io.opencannabis.schema.contact.ContactEmail.EmailAddress.newBuilder(contact_).mergeFrom(value).buildPartial();
} else {
contact_ = value;
}
onChanged();
} else {
contactBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public Builder clearContact() {
if (contactBuilder_ == null) {
contact_ = null;
onChanged();
} else {
contact_ = null;
contactBuilder_ = null;
}
return this;
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder getContactBuilder() {
onChanged();
return getContactFieldBuilder().getBuilder();
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
public io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder getContactOrBuilder() {
if (contactBuilder_ != null) {
return contactBuilder_.getMessageOrBuilder();
} else {
return contact_ == null ?
io.opencannabis.schema.contact.ContactEmail.EmailAddress.getDefaultInstance() : contact_;
}
}
/**
*
* Email address details/contact.
*
*
* .opencannabis.contact.EmailAddress contact = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>
getContactFieldBuilder() {
if (contactBuilder_ == null) {
contactBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.contact.ContactEmail.EmailAddress, io.opencannabis.schema.contact.ContactEmail.EmailAddress.Builder, io.opencannabis.schema.contact.ContactEmail.EmailAddressOrBuilder>(
getContact(),
getParentForChildren(),
isClean());
contact_ = null;
}
return contactBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailSender)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailSender)
private static final io.bloombox.schema.comms.EmailComms.EmailSender DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailSender();
}
public static io.bloombox.schema.comms.EmailComms.EmailSender getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailSender parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailSender(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSender getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmailSettingsOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.comms.EmailSettings)
com.google.protobuf.MessageOrBuilder {
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
java.util.List
getSenderList();
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailSender getSender(int index);
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
int getSenderCount();
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
java.util.List extends io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder>
getSenderOrBuilderList();
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder getSenderOrBuilder(
int index);
/**
*
* Enable/disable plaintext emails.
*
*
* bool enable_text = 2;
*/
boolean getEnableText();
/**
*
* Enable/disable HTML emails.
*
*
* bool enable_html = 3;
*/
boolean getEnableHtml();
/**
*
* Subscription manager group number. Optional.
*
*
* uint32 asm_group = 4;
*/
int getAsmGroup();
}
/**
*
* Email-specific settings.
*
*
* Protobuf type {@code bloombox.comms.EmailSettings}
*/
public static final class EmailSettings extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.comms.EmailSettings)
EmailSettingsOrBuilder {
private static final long serialVersionUID = 0L;
// Use EmailSettings.newBuilder() to construct.
private EmailSettings(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EmailSettings() {
sender_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EmailSettings(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
sender_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
sender_.add(
input.readMessage(io.bloombox.schema.comms.EmailComms.EmailSender.parser(), extensionRegistry));
break;
}
case 16: {
enableText_ = input.readBool();
break;
}
case 24: {
enableHtml_ = input.readBool();
break;
}
case 32: {
asmGroup_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
sender_ = java.util.Collections.unmodifiableList(sender_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailSettings.class, io.bloombox.schema.comms.EmailComms.EmailSettings.Builder.class);
}
private int bitField0_;
public static final int SENDER_FIELD_NUMBER = 1;
private java.util.List sender_;
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public java.util.List getSenderList() {
return sender_;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public java.util.List extends io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder>
getSenderOrBuilderList() {
return sender_;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public int getSenderCount() {
return sender_.size();
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender getSender(int index) {
return sender_.get(index);
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder getSenderOrBuilder(
int index) {
return sender_.get(index);
}
public static final int ENABLE_TEXT_FIELD_NUMBER = 2;
private boolean enableText_;
/**
*
* Enable/disable plaintext emails.
*
*
* bool enable_text = 2;
*/
public boolean getEnableText() {
return enableText_;
}
public static final int ENABLE_HTML_FIELD_NUMBER = 3;
private boolean enableHtml_;
/**
*
* Enable/disable HTML emails.
*
*
* bool enable_html = 3;
*/
public boolean getEnableHtml() {
return enableHtml_;
}
public static final int ASM_GROUP_FIELD_NUMBER = 4;
private int asmGroup_;
/**
*
* Subscription manager group number. Optional.
*
*
* uint32 asm_group = 4;
*/
public int getAsmGroup() {
return asmGroup_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < sender_.size(); i++) {
output.writeMessage(1, sender_.get(i));
}
if (enableText_ != false) {
output.writeBool(2, enableText_);
}
if (enableHtml_ != false) {
output.writeBool(3, enableHtml_);
}
if (asmGroup_ != 0) {
output.writeUInt32(4, asmGroup_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < sender_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, sender_.get(i));
}
if (enableText_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, enableText_);
}
if (enableHtml_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, enableHtml_);
}
if (asmGroup_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, asmGroup_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.comms.EmailComms.EmailSettings)) {
return super.equals(obj);
}
io.bloombox.schema.comms.EmailComms.EmailSettings other = (io.bloombox.schema.comms.EmailComms.EmailSettings) obj;
if (!getSenderList()
.equals(other.getSenderList())) return false;
if (getEnableText()
!= other.getEnableText()) return false;
if (getEnableHtml()
!= other.getEnableHtml()) return false;
if (getAsmGroup()
!= other.getAsmGroup()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSenderCount() > 0) {
hash = (37 * hash) + SENDER_FIELD_NUMBER;
hash = (53 * hash) + getSenderList().hashCode();
}
hash = (37 * hash) + ENABLE_TEXT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEnableText());
hash = (37 * hash) + ENABLE_HTML_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEnableHtml());
hash = (37 * hash) + ASM_GROUP_FIELD_NUMBER;
hash = (53 * hash) + getAsmGroup();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.comms.EmailComms.EmailSettings prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Email-specific settings.
*
*
* Protobuf type {@code bloombox.comms.EmailSettings}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.comms.EmailSettings)
io.bloombox.schema.comms.EmailComms.EmailSettingsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.comms.EmailComms.EmailSettings.class, io.bloombox.schema.comms.EmailComms.EmailSettings.Builder.class);
}
// Construct using io.bloombox.schema.comms.EmailComms.EmailSettings.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getSenderFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (senderBuilder_ == null) {
sender_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
senderBuilder_.clear();
}
enableText_ = false;
enableHtml_ = false;
asmGroup_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.comms.EmailComms.internal_static_bloombox_comms_EmailSettings_descriptor;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSettings getDefaultInstanceForType() {
return io.bloombox.schema.comms.EmailComms.EmailSettings.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSettings build() {
io.bloombox.schema.comms.EmailComms.EmailSettings result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSettings buildPartial() {
io.bloombox.schema.comms.EmailComms.EmailSettings result = new io.bloombox.schema.comms.EmailComms.EmailSettings(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (senderBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
sender_ = java.util.Collections.unmodifiableList(sender_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.sender_ = sender_;
} else {
result.sender_ = senderBuilder_.build();
}
result.enableText_ = enableText_;
result.enableHtml_ = enableHtml_;
result.asmGroup_ = asmGroup_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.comms.EmailComms.EmailSettings) {
return mergeFrom((io.bloombox.schema.comms.EmailComms.EmailSettings)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.comms.EmailComms.EmailSettings other) {
if (other == io.bloombox.schema.comms.EmailComms.EmailSettings.getDefaultInstance()) return this;
if (senderBuilder_ == null) {
if (!other.sender_.isEmpty()) {
if (sender_.isEmpty()) {
sender_ = other.sender_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSenderIsMutable();
sender_.addAll(other.sender_);
}
onChanged();
}
} else {
if (!other.sender_.isEmpty()) {
if (senderBuilder_.isEmpty()) {
senderBuilder_.dispose();
senderBuilder_ = null;
sender_ = other.sender_;
bitField0_ = (bitField0_ & ~0x00000001);
senderBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSenderFieldBuilder() : null;
} else {
senderBuilder_.addAllMessages(other.sender_);
}
}
}
if (other.getEnableText() != false) {
setEnableText(other.getEnableText());
}
if (other.getEnableHtml() != false) {
setEnableHtml(other.getEnableHtml());
}
if (other.getAsmGroup() != 0) {
setAsmGroup(other.getAsmGroup());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.comms.EmailComms.EmailSettings parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.comms.EmailComms.EmailSettings) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List sender_ =
java.util.Collections.emptyList();
private void ensureSenderIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
sender_ = new java.util.ArrayList(sender_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailSender, io.bloombox.schema.comms.EmailComms.EmailSender.Builder, io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder> senderBuilder_;
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public java.util.List getSenderList() {
if (senderBuilder_ == null) {
return java.util.Collections.unmodifiableList(sender_);
} else {
return senderBuilder_.getMessageList();
}
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public int getSenderCount() {
if (senderBuilder_ == null) {
return sender_.size();
} else {
return senderBuilder_.getCount();
}
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender getSender(int index) {
if (senderBuilder_ == null) {
return sender_.get(index);
} else {
return senderBuilder_.getMessage(index);
}
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder setSender(
int index, io.bloombox.schema.comms.EmailComms.EmailSender value) {
if (senderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSenderIsMutable();
sender_.set(index, value);
onChanged();
} else {
senderBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder setSender(
int index, io.bloombox.schema.comms.EmailComms.EmailSender.Builder builderForValue) {
if (senderBuilder_ == null) {
ensureSenderIsMutable();
sender_.set(index, builderForValue.build());
onChanged();
} else {
senderBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder addSender(io.bloombox.schema.comms.EmailComms.EmailSender value) {
if (senderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSenderIsMutable();
sender_.add(value);
onChanged();
} else {
senderBuilder_.addMessage(value);
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder addSender(
int index, io.bloombox.schema.comms.EmailComms.EmailSender value) {
if (senderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSenderIsMutable();
sender_.add(index, value);
onChanged();
} else {
senderBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder addSender(
io.bloombox.schema.comms.EmailComms.EmailSender.Builder builderForValue) {
if (senderBuilder_ == null) {
ensureSenderIsMutable();
sender_.add(builderForValue.build());
onChanged();
} else {
senderBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder addSender(
int index, io.bloombox.schema.comms.EmailComms.EmailSender.Builder builderForValue) {
if (senderBuilder_ == null) {
ensureSenderIsMutable();
sender_.add(index, builderForValue.build());
onChanged();
} else {
senderBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder addAllSender(
java.lang.Iterable extends io.bloombox.schema.comms.EmailComms.EmailSender> values) {
if (senderBuilder_ == null) {
ensureSenderIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sender_);
onChanged();
} else {
senderBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder clearSender() {
if (senderBuilder_ == null) {
sender_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
senderBuilder_.clear();
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public Builder removeSender(int index) {
if (senderBuilder_ == null) {
ensureSenderIsMutable();
sender_.remove(index);
onChanged();
} else {
senderBuilder_.remove(index);
}
return this;
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender.Builder getSenderBuilder(
int index) {
return getSenderFieldBuilder().getBuilder(index);
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder getSenderOrBuilder(
int index) {
if (senderBuilder_ == null) {
return sender_.get(index); } else {
return senderBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public java.util.List extends io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder>
getSenderOrBuilderList() {
if (senderBuilder_ != null) {
return senderBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sender_);
}
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender.Builder addSenderBuilder() {
return getSenderFieldBuilder().addBuilder(
io.bloombox.schema.comms.EmailComms.EmailSender.getDefaultInstance());
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public io.bloombox.schema.comms.EmailComms.EmailSender.Builder addSenderBuilder(
int index) {
return getSenderFieldBuilder().addBuilder(
index, io.bloombox.schema.comms.EmailComms.EmailSender.getDefaultInstance());
}
/**
*
* Origin email address to send the email from.
*
*
* repeated .bloombox.comms.EmailSender sender = 1;
*/
public java.util.List
getSenderBuilderList() {
return getSenderFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailSender, io.bloombox.schema.comms.EmailComms.EmailSender.Builder, io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder>
getSenderFieldBuilder() {
if (senderBuilder_ == null) {
senderBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.comms.EmailComms.EmailSender, io.bloombox.schema.comms.EmailComms.EmailSender.Builder, io.bloombox.schema.comms.EmailComms.EmailSenderOrBuilder>(
sender_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
sender_ = null;
}
return senderBuilder_;
}
private boolean enableText_ ;
/**
*
* Enable/disable plaintext emails.
*
*
* bool enable_text = 2;
*/
public boolean getEnableText() {
return enableText_;
}
/**
*
* Enable/disable plaintext emails.
*
*
* bool enable_text = 2;
*/
public Builder setEnableText(boolean value) {
enableText_ = value;
onChanged();
return this;
}
/**
*
* Enable/disable plaintext emails.
*
*
* bool enable_text = 2;
*/
public Builder clearEnableText() {
enableText_ = false;
onChanged();
return this;
}
private boolean enableHtml_ ;
/**
*
* Enable/disable HTML emails.
*
*
* bool enable_html = 3;
*/
public boolean getEnableHtml() {
return enableHtml_;
}
/**
*
* Enable/disable HTML emails.
*
*
* bool enable_html = 3;
*/
public Builder setEnableHtml(boolean value) {
enableHtml_ = value;
onChanged();
return this;
}
/**
*
* Enable/disable HTML emails.
*
*
* bool enable_html = 3;
*/
public Builder clearEnableHtml() {
enableHtml_ = false;
onChanged();
return this;
}
private int asmGroup_ ;
/**
*
* Subscription manager group number. Optional.
*
*
* uint32 asm_group = 4;
*/
public int getAsmGroup() {
return asmGroup_;
}
/**
*
* Subscription manager group number. Optional.
*
*
* uint32 asm_group = 4;
*/
public Builder setAsmGroup(int value) {
asmGroup_ = value;
onChanged();
return this;
}
/**
*
* Subscription manager group number. Optional.
*
*
* uint32 asm_group = 4;
*/
public Builder clearAsmGroup() {
asmGroup_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.comms.EmailSettings)
}
// @@protoc_insertion_point(class_scope:bloombox.comms.EmailSettings)
private static final io.bloombox.schema.comms.EmailComms.EmailSettings DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.comms.EmailComms.EmailSettings();
}
public static io.bloombox.schema.comms.EmailComms.EmailSettings getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EmailSettings parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EmailSettings(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.comms.EmailComms.EmailSettings getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailContent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailContent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_PublisherMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_PublisherMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_GoToView_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_GoToTrack_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_OneClickAction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_OrderMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailMetadata_SchemaBlock_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailTransmission_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailTransmission_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailBatch_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailBatch_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailSender_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailSender_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_comms_EmailSettings_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_comms_EmailSettings_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\021comms/Email.proto\022\016bloombox.comms\032\021geo" +
"/Address.proto\032\024commerce/Order.proto\032\024co" +
"re/Datamodel.proto\032\024media/MediaKey.proto" +
"\032\025partner/Partner.proto\032\025content/Content" +
".proto\032\026temporal/Instant.proto\032\032contact/" +
"EmailAddress.proto\"\201\001\n\014EmailContent\022\017\n\007s" +
"ubject\030\001 \001(\t\022.\n\007content\030\002 \003(\0132\035.opencann" +
"abis.content.Content\0220\n\nattachment\030\003 \003(\013" +
"2\034.opencannabis.media.MediaKey\"\277\002\n\014Email" +
"Message\0222\n\006sender\030\001 \001(\0132\".opencannabis.c" +
"ontact.EmailAddress\0225\n\trecipient\030\002 \001(\0132\"" +
".opencannabis.contact.EmailAddress\022-\n\007co" +
"ntent\030\003 \001(\0132\034.bloombox.comms.EmailConten" +
"t\0224\n\010reply_to\030\004 \001(\0132\".opencannabis.conta" +
"ct.EmailAddress\022.\n\002cc\030\005 \003(\0132\".opencannab" +
"is.contact.EmailAddress\022/\n\003bcc\030\006 \003(\0132\".o" +
"pencannabis.contact.EmailAddress\"f\n\021Publ" +
"isherMetadata\022\014\n\004name\030\001 \001(\t\022\013\n\003url\030\002 \001(\t" +
"\022\023\n\013google_plus\030\003 \001(\t\022\017\n\007twitter\030\004 \001(\t\022\020" +
"\n\010facebook\030\005 \001(\t\"\375\010\n\rEmailMetadata\032^\n\010Go" +
"ToView\022\014\n\004name\030\001 \001(\t\022\016\n\006target\030\003 \001(\t\0224\n\t" +
"publisher\030\004 \001(\0132!.bloombox.comms.Publish" +
"erMetadata\032\243\002\n\tGoToTrack\0223\n\020delivery_add" +
"ress\030\001 \001(\0132\031.opencannabis.geo.Address\0227\n" +
"\017estimated_ready\030\002 \001(\0132\036.opencannabis.te" +
"mporal.Instant\0229\n\021estimated_arrival\030\003 \001(" +
"\0132\036.opencannabis.temporal.Instant\022*\n\007par" +
"tner\030\004 \001(\0132\031.bloombox.partner.Partner\022+\n" +
"\005order\030\005 \001(\0132\034.opencannabis.commerce.Ord" +
"er\022\024\n\014tracking_url\030\006 \001(\t\032\271\001\n\016OneClickAct" +
"ion\022E\n\004type\030\001 \001(\01627.bloombox.comms.Email" +
"Metadata.OneClickAction.ActionType\022\014\n\004na" +
"me\030\002 \001(\t\022\016\n\006target\030\003 \001(\t\022\023\n\013description\030" +
"\004 \001(\t\"-\n\nActionType\022\013\n\007CONFIRM\020\000\022\010\n\004SAVE" +
"\020\001\022\010\n\004RSVP\020\002\032\264\001\n\rOrderMetadata\022*\n\007partne" +
"r\030\001 \001(\0132\031.bloombox.partner.Partner\022+\n\005or" +
"der\030\002 \001(\0132\034.opencannabis.commerce.Order\022" +
"\020\n\010currency\030\003 \001(\t\022\020\n\010subtotal\030\004 \001(\001\022\022\n\ns" +
"tatus_url\030\005 \001(\t\022\022\n\nmobile_url\030\006 \001(\t\032\273\002\n\013" +
"SchemaBlock\0220\n\004type\030\001 \001(\0162\".bloombox.com" +
"ms.EmailMetadata.Type\0226\n\004view\030\n \001(\0132&.bl" +
"oombox.comms.EmailMetadata.GoToViewH\000\0228\n" +
"\005track\030\017 \001(\0132\'.bloombox.comms.EmailMetad" +
"ata.GoToTrackH\000\022A\n\tone_click\030\024 \001(\0132,.blo" +
"ombox.comms.EmailMetadata.OneClickAction" +
"H\000\022<\n\005order\030\036 \001(\0132+.bloombox.comms.Email" +
"Metadata.OrderMetadataH\000B\007\n\005block\"5\n\004Typ" +
"e\022\010\n\004VIEW\020\000\022\t\n\005TRACK\020\001\022\r\n\tONE_CLICK\020\002\022\t\n" +
"\005ORDER\020\003\"B\n\021EmailTransmission\022-\n\007message" +
"\030\001 \001(\0132\034.bloombox.comms.EmailMessage\";\n\n" +
"EmailBatch\022-\n\002op\030\001 \003(\0132!.bloombox.comms." +
"EmailTransmission\"\245\001\n\013EmailSender\022.\n\004rol" +
"e\030\001 \003(\0162 .bloombox.comms.EmailSender.Rol" +
"e\0223\n\007contact\030\002 \001(\0132\".opencannabis.contac" +
"t.EmailAddress\"1\n\004Role\022\n\n\006SENDER\020\000\022\006\n\002CC" +
"\020\001\022\007\n\003BCC\020\002\022\014\n\010REPLY_TO\020\003\"y\n\rEmailSettin" +
"gs\022+\n\006sender\030\001 \003(\0132\033.bloombox.comms.Emai" +
"lSender\022\023\n\013enable_text\030\002 \001(\010\022\023\n\013enable_h" +
"tml\030\003 \001(\010\022\021\n\tasm_group\030\004 \001(\rB0\n\030io.bloom" +
"box.schema.commsB\nEmailCommsH\001P\000\242\002\003BBSb\006" +
"proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
io.opencannabis.schema.geo.AddressOuterClass.getDescriptor(),
io.opencannabis.schema.commerce.CommercialOrder.getDescriptor(),
core.Datamodel.getDescriptor(),
io.opencannabis.schema.media.MediaItemKey.getDescriptor(),
io.bloombox.schema.partner.PartnerAccount.getDescriptor(),
io.opencannabis.schema.content.GenericContent.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
io.opencannabis.schema.contact.ContactEmail.getDescriptor(),
}, assigner);
internal_static_bloombox_comms_EmailContent_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_comms_EmailContent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailContent_descriptor,
new java.lang.String[] { "Subject", "Content", "Attachment", });
internal_static_bloombox_comms_EmailMessage_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_comms_EmailMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMessage_descriptor,
new java.lang.String[] { "Sender", "Recipient", "Content", "ReplyTo", "Cc", "Bcc", });
internal_static_bloombox_comms_PublisherMetadata_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_bloombox_comms_PublisherMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_PublisherMetadata_descriptor,
new java.lang.String[] { "Name", "Url", "GooglePlus", "Twitter", "Facebook", });
internal_static_bloombox_comms_EmailMetadata_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_bloombox_comms_EmailMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_descriptor,
new java.lang.String[] { });
internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor =
internal_static_bloombox_comms_EmailMetadata_descriptor.getNestedTypes().get(0);
internal_static_bloombox_comms_EmailMetadata_GoToView_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_GoToView_descriptor,
new java.lang.String[] { "Name", "Target", "Publisher", });
internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor =
internal_static_bloombox_comms_EmailMetadata_descriptor.getNestedTypes().get(1);
internal_static_bloombox_comms_EmailMetadata_GoToTrack_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_GoToTrack_descriptor,
new java.lang.String[] { "DeliveryAddress", "EstimatedReady", "EstimatedArrival", "Partner", "Order", "TrackingUrl", });
internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor =
internal_static_bloombox_comms_EmailMetadata_descriptor.getNestedTypes().get(2);
internal_static_bloombox_comms_EmailMetadata_OneClickAction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_OneClickAction_descriptor,
new java.lang.String[] { "Type", "Name", "Target", "Description", });
internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor =
internal_static_bloombox_comms_EmailMetadata_descriptor.getNestedTypes().get(3);
internal_static_bloombox_comms_EmailMetadata_OrderMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_OrderMetadata_descriptor,
new java.lang.String[] { "Partner", "Order", "Currency", "Subtotal", "StatusUrl", "MobileUrl", });
internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor =
internal_static_bloombox_comms_EmailMetadata_descriptor.getNestedTypes().get(4);
internal_static_bloombox_comms_EmailMetadata_SchemaBlock_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailMetadata_SchemaBlock_descriptor,
new java.lang.String[] { "Type", "View", "Track", "OneClick", "Order", "Block", });
internal_static_bloombox_comms_EmailTransmission_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_bloombox_comms_EmailTransmission_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailTransmission_descriptor,
new java.lang.String[] { "Message", });
internal_static_bloombox_comms_EmailBatch_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_bloombox_comms_EmailBatch_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailBatch_descriptor,
new java.lang.String[] { "Op", });
internal_static_bloombox_comms_EmailSender_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_bloombox_comms_EmailSender_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailSender_descriptor,
new java.lang.String[] { "Role", "Contact", });
internal_static_bloombox_comms_EmailSettings_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_bloombox_comms_EmailSettings_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_comms_EmailSettings_descriptor,
new java.lang.String[] { "Sender", "EnableText", "EnableHtml", "AsmGroup", });
io.opencannabis.schema.geo.AddressOuterClass.getDescriptor();
io.opencannabis.schema.commerce.CommercialOrder.getDescriptor();
core.Datamodel.getDescriptor();
io.opencannabis.schema.media.MediaItemKey.getDescriptor();
io.bloombox.schema.partner.PartnerAccount.getDescriptor();
io.opencannabis.schema.content.GenericContent.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
io.opencannabis.schema.contact.ContactEmail.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy