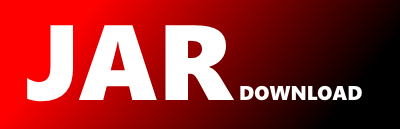
io.bloombox.schema.identity.AppUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: identity/User.proto
package io.bloombox.schema.identity;
public final class AppUser {
private AppUser() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Types of media that may be attached to a user.
*
*
* Protobuf enum {@code bloombox.identity.UserMediaType}
*/
public enum UserMediaType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* A portrait image of the person that this user represents.
*
*
* PICTURE = 0;
*/
PICTURE(0),
/**
*
* Picture of the user's driver's license.
*
*
* DRIVERS_LICENSE = 1;
*/
DRIVERS_LICENSE(1),
/**
*
* Picture of the user's doctor's rec.
*
*
* DOCTOR_REC = 2;
*/
DOCTOR_REC(2),
UNRECOGNIZED(-1),
;
/**
*
* A portrait image of the person that this user represents.
*
*
* PICTURE = 0;
*/
public static final int PICTURE_VALUE = 0;
/**
*
* Picture of the user's driver's license.
*
*
* DRIVERS_LICENSE = 1;
*/
public static final int DRIVERS_LICENSE_VALUE = 1;
/**
*
* Picture of the user's doctor's rec.
*
*
* DOCTOR_REC = 2;
*/
public static final int DOCTOR_REC_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static UserMediaType valueOf(int value) {
return forNumber(value);
}
public static UserMediaType forNumber(int value) {
switch (value) {
case 0: return PICTURE;
case 1: return DRIVERS_LICENSE;
case 2: return DOCTOR_REC;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
UserMediaType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public UserMediaType findValueByNumber(int number) {
return UserMediaType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.getDescriptor().getEnumTypes().get(0);
}
private static final UserMediaType[] VALUES = values();
public static UserMediaType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private UserMediaType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.UserMediaType)
}
/**
*
* Enumerates providers through which users may authenticate.
*
*
* Protobuf enum {@code bloombox.identity.IdentityProvider}
*/
public enum IdentityProvider
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Email/password-based authentication.
*
*
* EMAIL = 0;
*/
EMAIL(0),
/**
*
* Authentication via Google OpenID/OAuth2.
*
*
* GOOGLE = 1;
*/
GOOGLE(1),
/**
*
* OAuth2 Graph authentication via Facebook.
*
*
* FACEBOOK = 2;
*/
FACEBOOK(2),
/**
*
* OAuth2 account authentication via Twitter.
*
*
* TWITTER = 3;
*/
TWITTER(3),
/**
*
* Phone-based authentication.
*
*
* PHONE = 4;
*/
PHONE(4),
UNRECOGNIZED(-1),
;
/**
*
* Email/password-based authentication.
*
*
* EMAIL = 0;
*/
public static final int EMAIL_VALUE = 0;
/**
*
* Authentication via Google OpenID/OAuth2.
*
*
* GOOGLE = 1;
*/
public static final int GOOGLE_VALUE = 1;
/**
*
* OAuth2 Graph authentication via Facebook.
*
*
* FACEBOOK = 2;
*/
public static final int FACEBOOK_VALUE = 2;
/**
*
* OAuth2 account authentication via Twitter.
*
*
* TWITTER = 3;
*/
public static final int TWITTER_VALUE = 3;
/**
*
* Phone-based authentication.
*
*
* PHONE = 4;
*/
public static final int PHONE_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IdentityProvider valueOf(int value) {
return forNumber(value);
}
public static IdentityProvider forNumber(int value) {
switch (value) {
case 0: return EMAIL;
case 1: return GOOGLE;
case 2: return FACEBOOK;
case 3: return TWITTER;
case 4: return PHONE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
IdentityProvider> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public IdentityProvider findValueByNumber(int number) {
return IdentityProvider.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.getDescriptor().getEnumTypes().get(1);
}
private static final IdentityProvider[] VALUES = values();
public static IdentityProvider valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private IdentityProvider(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.IdentityProvider)
}
/**
*
* Enumerates sources for user enrollments.
*
*
* Protobuf enum {@code bloombox.identity.EnrollmentSource}
*/
public enum EnrollmentSource
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unknown or unspecified enrollment source.
*
*
* UNSPECIFIED = 0;
*/
UNSPECIFIED(0),
/**
*
* Online enrollment.
*
*
* ONLINE = 1;
*/
ONLINE(1),
/**
*
* Internal application to Bloombox.
*
*
* INTERNAL_APP = 2;
*/
INTERNAL_APP(2),
/**
*
* Partner application integration.
*
*
* PARTNER_APP = 3;
*/
PARTNER_APP(3),
/**
*
* In-store physical sign up.
*
*
* IN_STORE = 4;
*/
IN_STORE(4),
/**
*
* Internally imported user.
*
*
* IMPORT = 5;
*/
IMPORT(5),
UNRECOGNIZED(-1),
;
/**
*
* Unknown or unspecified enrollment source.
*
*
* UNSPECIFIED = 0;
*/
public static final int UNSPECIFIED_VALUE = 0;
/**
*
* Online enrollment.
*
*
* ONLINE = 1;
*/
public static final int ONLINE_VALUE = 1;
/**
*
* Internal application to Bloombox.
*
*
* INTERNAL_APP = 2;
*/
public static final int INTERNAL_APP_VALUE = 2;
/**
*
* Partner application integration.
*
*
* PARTNER_APP = 3;
*/
public static final int PARTNER_APP_VALUE = 3;
/**
*
* In-store physical sign up.
*
*
* IN_STORE = 4;
*/
public static final int IN_STORE_VALUE = 4;
/**
*
* Internally imported user.
*
*
* IMPORT = 5;
*/
public static final int IMPORT_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EnrollmentSource valueOf(int value) {
return forNumber(value);
}
public static EnrollmentSource forNumber(int value) {
switch (value) {
case 0: return UNSPECIFIED;
case 1: return ONLINE;
case 2: return INTERNAL_APP;
case 3: return PARTNER_APP;
case 4: return IN_STORE;
case 5: return IMPORT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EnrollmentSource> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EnrollmentSource findValueByNumber(int number) {
return EnrollmentSource.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.getDescriptor().getEnumTypes().get(2);
}
private static final EnrollmentSource[] VALUES = values();
public static EnrollmentSource valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private EnrollmentSource(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.EnrollmentSource)
}
/**
*
* Enumerates sources for user referrals
*
*
* Protobuf enum {@code bloombox.identity.ReferralSource}
*/
public enum ReferralSource
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unknown or unspecified enrollment source.
*
*
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* Outdoor, real-world advertising.
*
*
* OUTDOOR = 1;
*/
OUTDOOR(1),
/**
*
* Digital advertising.
*
*
* DIGITAL = 2;
*/
DIGITAL(2),
/**
*
* Social Media referral.
*
*
* SOCIAL_MEDIA = 3;
*/
SOCIAL_MEDIA(3),
/**
*
* Friend or Colleague.
*
*
* FRIEND = 4;
*/
FRIEND(4),
UNRECOGNIZED(-1),
;
/**
*
* Unknown or unspecified enrollment source.
*
*
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* Outdoor, real-world advertising.
*
*
* OUTDOOR = 1;
*/
public static final int OUTDOOR_VALUE = 1;
/**
*
* Digital advertising.
*
*
* DIGITAL = 2;
*/
public static final int DIGITAL_VALUE = 2;
/**
*
* Social Media referral.
*
*
* SOCIAL_MEDIA = 3;
*/
public static final int SOCIAL_MEDIA_VALUE = 3;
/**
*
* Friend or Colleague.
*
*
* FRIEND = 4;
*/
public static final int FRIEND_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReferralSource valueOf(int value) {
return forNumber(value);
}
public static ReferralSource forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return OUTDOOR;
case 2: return DIGITAL;
case 3: return SOCIAL_MEDIA;
case 4: return FRIEND;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReferralSource> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReferralSource findValueByNumber(int number) {
return ReferralSource.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.getDescriptor().getEnumTypes().get(3);
}
private static final ReferralSource[] VALUES = values();
public static ReferralSource valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReferralSource(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.ReferralSource)
}
/**
*
* Specifies the type of consumer profile in use for an account.
*
*
* Protobuf enum {@code bloombox.identity.ConsumerType}
*/
public enum ConsumerType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The consumer type is not yet determined.
*
*
* UNVALIDATED = 0;
*/
UNVALIDATED(0, 0),
/**
*
* The consumer is a recreational user.
*
*
* RECREATIONAL = 1;
*/
RECREATIONAL(1, 1),
/**
*
* The consumer is a validated medical user. Considered a superset of 'RECREATIONAL'.
*
*
* MEDICAL = 2;
*/
MEDICAL(3, 2),
UNRECOGNIZED(-1, -1),
;
/**
*
* Specifies the alternate name for recreational-use.
*
*
* ADULT_USE = 1;
*/
public static final ConsumerType ADULT_USE = RECREATIONAL;
/**
*
* The consumer type is not yet determined.
*
*
* UNVALIDATED = 0;
*/
public static final int UNVALIDATED_VALUE = 0;
/**
*
* The consumer is a recreational user.
*
*
* RECREATIONAL = 1;
*/
public static final int RECREATIONAL_VALUE = 1;
/**
*
* Specifies the alternate name for recreational-use.
*
*
* ADULT_USE = 1;
*/
public static final int ADULT_USE_VALUE = 1;
/**
*
* The consumer is a validated medical user. Considered a superset of 'RECREATIONAL'.
*
*
* MEDICAL = 2;
*/
public static final int MEDICAL_VALUE = 2;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConsumerType valueOf(int value) {
return forNumber(value);
}
public static ConsumerType forNumber(int value) {
switch (value) {
case 0: return UNVALIDATED;
case 1: return RECREATIONAL;
case 2: return MEDICAL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ConsumerType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConsumerType findValueByNumber(int number) {
return ConsumerType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.getDescriptor().getEnumTypes().get(4);
}
private static final ConsumerType[] VALUES = {
UNVALIDATED, RECREATIONAL, ADULT_USE, MEDICAL,
};
public static ConsumerType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ConsumerType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.ConsumerType)
}
public interface UserOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.User)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
java.lang.String getUid();
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUidBytes();
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
boolean hasFlags();
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
io.bloombox.schema.identity.AppUser.UserFlags getFlags();
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder getFlagsOrBuilder();
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
boolean hasPerson();
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
io.opencannabis.schema.person.Person getPerson();
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
io.opencannabis.schema.person.PersonOrBuilder getPersonOrBuilder();
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
java.util.List
getIdentificationList();
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
io.bloombox.schema.identity.IdentityID.ID getIdentification(int index);
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
int getIdentificationCount();
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
java.util.List extends io.bloombox.schema.identity.IdentityID.IDOrBuilder>
getIdentificationOrBuilderList();
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
io.bloombox.schema.identity.IdentityID.IDOrBuilder getIdentificationOrBuilder(
int index);
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
java.util.List
getDoctorRecList();
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec getDoctorRec(int index);
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
int getDoctorRecCount();
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
java.util.List extends io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder>
getDoctorRecOrBuilderList();
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder getDoctorRecOrBuilder(
int index);
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
boolean hasSeen();
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
boolean hasSignup();
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSignup();
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignupOrBuilder();
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
int getIdentitiesCount();
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
boolean containsIdentities(
java.lang.String key);
/**
* Use {@link #getIdentitiesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getIdentities();
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
java.util.Map
getIdentitiesMap();
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrDefault(
java.lang.String key,
io.bloombox.schema.identity.AppUser.UserIdentity defaultValue);
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrThrow(
java.lang.String key);
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
int getMediaCount();
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
boolean containsMedia(
java.lang.String key);
/**
* Use {@link #getMediaMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMedia();
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
java.util.Map
getMediaMap();
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrDefault(
java.lang.String key,
io.opencannabis.schema.media.AttachedMedia.MediaItem defaultValue);
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrThrow(
java.lang.String key);
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
boolean hasConsumer();
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
io.bloombox.schema.identity.AppUser.ConsumerProfile getConsumer();
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder getConsumerOrBuilder();
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
boolean hasIndustry();
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
io.bloombox.schema.identity.AppUser.IndustryProfile getIndustry();
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder getIndustryOrBuilder();
}
/**
*
* Represents an individual who uses software.
*
*
* Protobuf type {@code bloombox.identity.User}
*/
public static final class User extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.User)
UserOrBuilder {
private static final long serialVersionUID = 0L;
// Use User.newBuilder() to construct.
private User(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private User() {
uid_ = "";
identification_ = java.util.Collections.emptyList();
doctorRec_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private User(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uid_ = s;
break;
}
case 18: {
io.bloombox.schema.identity.AppUser.UserFlags.Builder subBuilder = null;
if (flags_ != null) {
subBuilder = flags_.toBuilder();
}
flags_ = input.readMessage(io.bloombox.schema.identity.AppUser.UserFlags.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(flags_);
flags_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.person.Person.Builder subBuilder = null;
if (person_ != null) {
subBuilder = person_.toBuilder();
}
person_ = input.readMessage(io.opencannabis.schema.person.Person.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(person_);
person_ = subBuilder.buildPartial();
}
break;
}
case 162: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
identification_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
identification_.add(
input.readMessage(io.bloombox.schema.identity.IdentityID.ID.parser(), extensionRegistry));
break;
}
case 170: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
doctorRec_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
doctorRec_.add(
input.readMessage(io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.parser(), extensionRegistry));
break;
}
case 242: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
case 250: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (signup_ != null) {
subBuilder = signup_.toBuilder();
}
signup_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signup_);
signup_ = subBuilder.buildPartial();
}
break;
}
case 322: {
if (!((mutable_bitField0_ & 0x00000080) != 0)) {
identities_ = com.google.protobuf.MapField.newMapField(
IdentitiesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000080;
}
com.google.protobuf.MapEntry
identities__ = input.readMessage(
IdentitiesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
identities_.getMutableMap().put(
identities__.getKey(), identities__.getValue());
break;
}
case 330: {
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
media_ = com.google.protobuf.MapField.newMapField(
MediaDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000100;
}
com.google.protobuf.MapEntry
media__ = input.readMessage(
MediaDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
media_.getMutableMap().put(
media__.getKey(), media__.getValue());
break;
}
case 802: {
io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder subBuilder = null;
if (consumer_ != null) {
subBuilder = consumer_.toBuilder();
}
consumer_ = input.readMessage(io.bloombox.schema.identity.AppUser.ConsumerProfile.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consumer_);
consumer_ = subBuilder.buildPartial();
}
break;
}
case 810: {
io.bloombox.schema.identity.AppUser.IndustryProfile.Builder subBuilder = null;
if (industry_ != null) {
subBuilder = industry_.toBuilder();
}
industry_ = input.readMessage(io.bloombox.schema.identity.AppUser.IndustryProfile.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(industry_);
industry_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) != 0)) {
identification_ = java.util.Collections.unmodifiableList(identification_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
doctorRec_ = java.util.Collections.unmodifiableList(doctorRec_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 40:
return internalGetIdentities();
case 41:
return internalGetMedia();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.User.class, io.bloombox.schema.identity.AppUser.User.Builder.class);
}
private int bitField0_;
public static final int UID_FIELD_NUMBER = 1;
private volatile java.lang.Object uid_;
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uid_ = s;
return s;
}
}
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FLAGS_FIELD_NUMBER = 2;
private io.bloombox.schema.identity.AppUser.UserFlags flags_;
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public boolean hasFlags() {
return flags_ != null;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public io.bloombox.schema.identity.AppUser.UserFlags getFlags() {
return flags_ == null ? io.bloombox.schema.identity.AppUser.UserFlags.getDefaultInstance() : flags_;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder getFlagsOrBuilder() {
return getFlags();
}
public static final int PERSON_FIELD_NUMBER = 3;
private io.opencannabis.schema.person.Person person_;
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public boolean hasPerson() {
return person_ != null;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public io.opencannabis.schema.person.Person getPerson() {
return person_ == null ? io.opencannabis.schema.person.Person.getDefaultInstance() : person_;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public io.opencannabis.schema.person.PersonOrBuilder getPersonOrBuilder() {
return getPerson();
}
public static final int IDENTIFICATION_FIELD_NUMBER = 20;
private java.util.List identification_;
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public java.util.List getIdentificationList() {
return identification_;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public java.util.List extends io.bloombox.schema.identity.IdentityID.IDOrBuilder>
getIdentificationOrBuilderList() {
return identification_;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public int getIdentificationCount() {
return identification_.size();
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.ID getIdentification(int index) {
return identification_.get(index);
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.IDOrBuilder getIdentificationOrBuilder(
int index) {
return identification_.get(index);
}
public static final int DOCTOR_REC_FIELD_NUMBER = 21;
private java.util.List doctorRec_;
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public java.util.List getDoctorRecList() {
return doctorRec_;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public java.util.List extends io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder>
getDoctorRecOrBuilderList() {
return doctorRec_;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public int getDoctorRecCount() {
return doctorRec_.size();
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec getDoctorRec(int index) {
return doctorRec_.get(index);
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder getDoctorRecOrBuilder(
int index) {
return doctorRec_.get(index);
}
public static final int SEEN_FIELD_NUMBER = 30;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
public static final int SIGNUP_FIELD_NUMBER = 31;
private io.opencannabis.schema.temporal.TemporalInstant.Instant signup_;
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public boolean hasSignup() {
return signup_ != null;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSignup() {
return signup_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signup_;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignupOrBuilder() {
return getSignup();
}
public static final int IDENTITIES_FIELD_NUMBER = 40;
private static final class IdentitiesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, io.bloombox.schema.identity.AppUser.UserIdentity> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_IdentitiesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
io.bloombox.schema.identity.AppUser.UserIdentity.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, io.bloombox.schema.identity.AppUser.UserIdentity> identities_;
private com.google.protobuf.MapField
internalGetIdentities() {
if (identities_ == null) {
return com.google.protobuf.MapField.emptyMapField(
IdentitiesDefaultEntryHolder.defaultEntry);
}
return identities_;
}
public int getIdentitiesCount() {
return internalGetIdentities().getMap().size();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public boolean containsIdentities(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetIdentities().getMap().containsKey(key);
}
/**
* Use {@link #getIdentitiesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getIdentities() {
return getIdentitiesMap();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public java.util.Map getIdentitiesMap() {
return internalGetIdentities().getMap();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrDefault(
java.lang.String key,
io.bloombox.schema.identity.AppUser.UserIdentity defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetIdentities().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetIdentities().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MEDIA_FIELD_NUMBER = 41;
private static final class MediaDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, io.opencannabis.schema.media.AttachedMedia.MediaItem> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_MediaEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
io.opencannabis.schema.media.AttachedMedia.MediaItem.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, io.opencannabis.schema.media.AttachedMedia.MediaItem> media_;
private com.google.protobuf.MapField
internalGetMedia() {
if (media_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MediaDefaultEntryHolder.defaultEntry);
}
return media_;
}
public int getMediaCount() {
return internalGetMedia().getMap().size();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public boolean containsMedia(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMedia().getMap().containsKey(key);
}
/**
* Use {@link #getMediaMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMedia() {
return getMediaMap();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public java.util.Map getMediaMap() {
return internalGetMedia().getMap();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrDefault(
java.lang.String key,
io.opencannabis.schema.media.AttachedMedia.MediaItem defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMedia().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMedia().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CONSUMER_FIELD_NUMBER = 100;
private io.bloombox.schema.identity.AppUser.ConsumerProfile consumer_;
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public boolean hasConsumer() {
return consumer_ != null;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerProfile getConsumer() {
return consumer_ == null ? io.bloombox.schema.identity.AppUser.ConsumerProfile.getDefaultInstance() : consumer_;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder getConsumerOrBuilder() {
return getConsumer();
}
public static final int INDUSTRY_FIELD_NUMBER = 101;
private io.bloombox.schema.identity.AppUser.IndustryProfile industry_;
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public boolean hasIndustry() {
return industry_ != null;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.IndustryProfile getIndustry() {
return industry_ == null ? io.bloombox.schema.identity.AppUser.IndustryProfile.getDefaultInstance() : industry_;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder getIndustryOrBuilder() {
return getIndustry();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uid_);
}
if (flags_ != null) {
output.writeMessage(2, getFlags());
}
if (person_ != null) {
output.writeMessage(3, getPerson());
}
for (int i = 0; i < identification_.size(); i++) {
output.writeMessage(20, identification_.get(i));
}
for (int i = 0; i < doctorRec_.size(); i++) {
output.writeMessage(21, doctorRec_.get(i));
}
if (seen_ != null) {
output.writeMessage(30, getSeen());
}
if (signup_ != null) {
output.writeMessage(31, getSignup());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetIdentities(),
IdentitiesDefaultEntryHolder.defaultEntry,
40);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMedia(),
MediaDefaultEntryHolder.defaultEntry,
41);
if (consumer_ != null) {
output.writeMessage(100, getConsumer());
}
if (industry_ != null) {
output.writeMessage(101, getIndustry());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uid_);
}
if (flags_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getFlags());
}
if (person_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPerson());
}
for (int i = 0; i < identification_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, identification_.get(i));
}
for (int i = 0; i < doctorRec_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, doctorRec_.get(i));
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, getSeen());
}
if (signup_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(31, getSignup());
}
for (java.util.Map.Entry entry
: internalGetIdentities().getMap().entrySet()) {
com.google.protobuf.MapEntry
identities__ = IdentitiesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(40, identities__);
}
for (java.util.Map.Entry entry
: internalGetMedia().getMap().entrySet()) {
com.google.protobuf.MapEntry
media__ = MediaDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(41, media__);
}
if (consumer_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, getConsumer());
}
if (industry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(101, getIndustry());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.User)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.User other = (io.bloombox.schema.identity.AppUser.User) obj;
if (!getUid()
.equals(other.getUid())) return false;
if (hasFlags() != other.hasFlags()) return false;
if (hasFlags()) {
if (!getFlags()
.equals(other.getFlags())) return false;
}
if (hasPerson() != other.hasPerson()) return false;
if (hasPerson()) {
if (!getPerson()
.equals(other.getPerson())) return false;
}
if (!getIdentificationList()
.equals(other.getIdentificationList())) return false;
if (!getDoctorRecList()
.equals(other.getDoctorRecList())) return false;
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (hasSignup() != other.hasSignup()) return false;
if (hasSignup()) {
if (!getSignup()
.equals(other.getSignup())) return false;
}
if (!internalGetIdentities().equals(
other.internalGetIdentities())) return false;
if (!internalGetMedia().equals(
other.internalGetMedia())) return false;
if (hasConsumer() != other.hasConsumer()) return false;
if (hasConsumer()) {
if (!getConsumer()
.equals(other.getConsumer())) return false;
}
if (hasIndustry() != other.hasIndustry()) return false;
if (hasIndustry()) {
if (!getIndustry()
.equals(other.getIndustry())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UID_FIELD_NUMBER;
hash = (53 * hash) + getUid().hashCode();
if (hasFlags()) {
hash = (37 * hash) + FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getFlags().hashCode();
}
if (hasPerson()) {
hash = (37 * hash) + PERSON_FIELD_NUMBER;
hash = (53 * hash) + getPerson().hashCode();
}
if (getIdentificationCount() > 0) {
hash = (37 * hash) + IDENTIFICATION_FIELD_NUMBER;
hash = (53 * hash) + getIdentificationList().hashCode();
}
if (getDoctorRecCount() > 0) {
hash = (37 * hash) + DOCTOR_REC_FIELD_NUMBER;
hash = (53 * hash) + getDoctorRecList().hashCode();
}
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
if (hasSignup()) {
hash = (37 * hash) + SIGNUP_FIELD_NUMBER;
hash = (53 * hash) + getSignup().hashCode();
}
if (!internalGetIdentities().getMap().isEmpty()) {
hash = (37 * hash) + IDENTITIES_FIELD_NUMBER;
hash = (53 * hash) + internalGetIdentities().hashCode();
}
if (!internalGetMedia().getMap().isEmpty()) {
hash = (37 * hash) + MEDIA_FIELD_NUMBER;
hash = (53 * hash) + internalGetMedia().hashCode();
}
if (hasConsumer()) {
hash = (37 * hash) + CONSUMER_FIELD_NUMBER;
hash = (53 * hash) + getConsumer().hashCode();
}
if (hasIndustry()) {
hash = (37 * hash) + INDUSTRY_FIELD_NUMBER;
hash = (53 * hash) + getIndustry().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.User parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.User parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.User parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.User prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents an individual who uses software.
*
*
* Protobuf type {@code bloombox.identity.User}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.User)
io.bloombox.schema.identity.AppUser.UserOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 40:
return internalGetIdentities();
case 41:
return internalGetMedia();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 40:
return internalGetMutableIdentities();
case 41:
return internalGetMutableMedia();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.User.class, io.bloombox.schema.identity.AppUser.User.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.User.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIdentificationFieldBuilder();
getDoctorRecFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uid_ = "";
if (flagsBuilder_ == null) {
flags_ = null;
} else {
flags_ = null;
flagsBuilder_ = null;
}
if (personBuilder_ == null) {
person_ = null;
} else {
person_ = null;
personBuilder_ = null;
}
if (identificationBuilder_ == null) {
identification_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
identificationBuilder_.clear();
}
if (doctorRecBuilder_ == null) {
doctorRec_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
doctorRecBuilder_.clear();
}
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
if (signupBuilder_ == null) {
signup_ = null;
} else {
signup_ = null;
signupBuilder_ = null;
}
internalGetMutableIdentities().clear();
internalGetMutableMedia().clear();
if (consumerBuilder_ == null) {
consumer_ = null;
} else {
consumer_ = null;
consumerBuilder_ = null;
}
if (industryBuilder_ == null) {
industry_ = null;
} else {
industry_ = null;
industryBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_User_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.User getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.User.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.User build() {
io.bloombox.schema.identity.AppUser.User result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.User buildPartial() {
io.bloombox.schema.identity.AppUser.User result = new io.bloombox.schema.identity.AppUser.User(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.uid_ = uid_;
if (flagsBuilder_ == null) {
result.flags_ = flags_;
} else {
result.flags_ = flagsBuilder_.build();
}
if (personBuilder_ == null) {
result.person_ = person_;
} else {
result.person_ = personBuilder_.build();
}
if (identificationBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
identification_ = java.util.Collections.unmodifiableList(identification_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.identification_ = identification_;
} else {
result.identification_ = identificationBuilder_.build();
}
if (doctorRecBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
doctorRec_ = java.util.Collections.unmodifiableList(doctorRec_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.doctorRec_ = doctorRec_;
} else {
result.doctorRec_ = doctorRecBuilder_.build();
}
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
if (signupBuilder_ == null) {
result.signup_ = signup_;
} else {
result.signup_ = signupBuilder_.build();
}
result.identities_ = internalGetIdentities();
result.identities_.makeImmutable();
result.media_ = internalGetMedia();
result.media_.makeImmutable();
if (consumerBuilder_ == null) {
result.consumer_ = consumer_;
} else {
result.consumer_ = consumerBuilder_.build();
}
if (industryBuilder_ == null) {
result.industry_ = industry_;
} else {
result.industry_ = industryBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.User) {
return mergeFrom((io.bloombox.schema.identity.AppUser.User)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.User other) {
if (other == io.bloombox.schema.identity.AppUser.User.getDefaultInstance()) return this;
if (!other.getUid().isEmpty()) {
uid_ = other.uid_;
onChanged();
}
if (other.hasFlags()) {
mergeFlags(other.getFlags());
}
if (other.hasPerson()) {
mergePerson(other.getPerson());
}
if (identificationBuilder_ == null) {
if (!other.identification_.isEmpty()) {
if (identification_.isEmpty()) {
identification_ = other.identification_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureIdentificationIsMutable();
identification_.addAll(other.identification_);
}
onChanged();
}
} else {
if (!other.identification_.isEmpty()) {
if (identificationBuilder_.isEmpty()) {
identificationBuilder_.dispose();
identificationBuilder_ = null;
identification_ = other.identification_;
bitField0_ = (bitField0_ & ~0x00000008);
identificationBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getIdentificationFieldBuilder() : null;
} else {
identificationBuilder_.addAllMessages(other.identification_);
}
}
}
if (doctorRecBuilder_ == null) {
if (!other.doctorRec_.isEmpty()) {
if (doctorRec_.isEmpty()) {
doctorRec_ = other.doctorRec_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureDoctorRecIsMutable();
doctorRec_.addAll(other.doctorRec_);
}
onChanged();
}
} else {
if (!other.doctorRec_.isEmpty()) {
if (doctorRecBuilder_.isEmpty()) {
doctorRecBuilder_.dispose();
doctorRecBuilder_ = null;
doctorRec_ = other.doctorRec_;
bitField0_ = (bitField0_ & ~0x00000010);
doctorRecBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDoctorRecFieldBuilder() : null;
} else {
doctorRecBuilder_.addAllMessages(other.doctorRec_);
}
}
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
if (other.hasSignup()) {
mergeSignup(other.getSignup());
}
internalGetMutableIdentities().mergeFrom(
other.internalGetIdentities());
internalGetMutableMedia().mergeFrom(
other.internalGetMedia());
if (other.hasConsumer()) {
mergeConsumer(other.getConsumer());
}
if (other.hasIndustry()) {
mergeIndustry(other.getIndustry());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.User parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.User) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uid_ = "";
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public Builder setUid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uid_ = value;
onChanged();
return this;
}
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public Builder clearUid() {
uid_ = getDefaultInstance().getUid();
onChanged();
return this;
}
/**
*
* Unique ID for the user.
*
*
* string uid = 1 [(.gen_bq_schema.description) = "Unique ID for the user.", (.core.field) = { ... }
*/
public Builder setUidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uid_ = value;
onChanged();
return this;
}
private io.bloombox.schema.identity.AppUser.UserFlags flags_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.UserFlags, io.bloombox.schema.identity.AppUser.UserFlags.Builder, io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder> flagsBuilder_;
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public boolean hasFlags() {
return flagsBuilder_ != null || flags_ != null;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public io.bloombox.schema.identity.AppUser.UserFlags getFlags() {
if (flagsBuilder_ == null) {
return flags_ == null ? io.bloombox.schema.identity.AppUser.UserFlags.getDefaultInstance() : flags_;
} else {
return flagsBuilder_.getMessage();
}
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public Builder setFlags(io.bloombox.schema.identity.AppUser.UserFlags value) {
if (flagsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
flags_ = value;
onChanged();
} else {
flagsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public Builder setFlags(
io.bloombox.schema.identity.AppUser.UserFlags.Builder builderForValue) {
if (flagsBuilder_ == null) {
flags_ = builderForValue.build();
onChanged();
} else {
flagsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public Builder mergeFlags(io.bloombox.schema.identity.AppUser.UserFlags value) {
if (flagsBuilder_ == null) {
if (flags_ != null) {
flags_ =
io.bloombox.schema.identity.AppUser.UserFlags.newBuilder(flags_).mergeFrom(value).buildPartial();
} else {
flags_ = value;
}
onChanged();
} else {
flagsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public Builder clearFlags() {
if (flagsBuilder_ == null) {
flags_ = null;
onChanged();
} else {
flags_ = null;
flagsBuilder_ = null;
}
return this;
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public io.bloombox.schema.identity.AppUser.UserFlags.Builder getFlagsBuilder() {
onChanged();
return getFlagsFieldBuilder().getBuilder();
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
public io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder getFlagsOrBuilder() {
if (flagsBuilder_ != null) {
return flagsBuilder_.getMessageOrBuilder();
} else {
return flags_ == null ?
io.bloombox.schema.identity.AppUser.UserFlags.getDefaultInstance() : flags_;
}
}
/**
*
* Boolean flags for this user.
*
*
* .bloombox.identity.UserFlags flags = 2 [(.gen_bq_schema.description) = "Boolean flags for this user.", (.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.UserFlags, io.bloombox.schema.identity.AppUser.UserFlags.Builder, io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder>
getFlagsFieldBuilder() {
if (flagsBuilder_ == null) {
flagsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.UserFlags, io.bloombox.schema.identity.AppUser.UserFlags.Builder, io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder>(
getFlags(),
getParentForChildren(),
isClean());
flags_ = null;
}
return flagsBuilder_;
}
private io.opencannabis.schema.person.Person person_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.person.Person, io.opencannabis.schema.person.Person.Builder, io.opencannabis.schema.person.PersonOrBuilder> personBuilder_;
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public boolean hasPerson() {
return personBuilder_ != null || person_ != null;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public io.opencannabis.schema.person.Person getPerson() {
if (personBuilder_ == null) {
return person_ == null ? io.opencannabis.schema.person.Person.getDefaultInstance() : person_;
} else {
return personBuilder_.getMessage();
}
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public Builder setPerson(io.opencannabis.schema.person.Person value) {
if (personBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
person_ = value;
onChanged();
} else {
personBuilder_.setMessage(value);
}
return this;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public Builder setPerson(
io.opencannabis.schema.person.Person.Builder builderForValue) {
if (personBuilder_ == null) {
person_ = builderForValue.build();
onChanged();
} else {
personBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public Builder mergePerson(io.opencannabis.schema.person.Person value) {
if (personBuilder_ == null) {
if (person_ != null) {
person_ =
io.opencannabis.schema.person.Person.newBuilder(person_).mergeFrom(value).buildPartial();
} else {
person_ = value;
}
onChanged();
} else {
personBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public Builder clearPerson() {
if (personBuilder_ == null) {
person_ = null;
onChanged();
} else {
person_ = null;
personBuilder_ = null;
}
return this;
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public io.opencannabis.schema.person.Person.Builder getPersonBuilder() {
onChanged();
return getPersonFieldBuilder().getBuilder();
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
public io.opencannabis.schema.person.PersonOrBuilder getPersonOrBuilder() {
if (personBuilder_ != null) {
return personBuilder_.getMessageOrBuilder();
} else {
return person_ == null ?
io.opencannabis.schema.person.Person.getDefaultInstance() : person_;
}
}
/**
*
* Person's information that backs this user.
*
*
* .opencannabis.person.Person person = 3 [(.gen_bq_schema.description) = "Person\'s information that backs this user."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.person.Person, io.opencannabis.schema.person.Person.Builder, io.opencannabis.schema.person.PersonOrBuilder>
getPersonFieldBuilder() {
if (personBuilder_ == null) {
personBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.person.Person, io.opencannabis.schema.person.Person.Builder, io.opencannabis.schema.person.PersonOrBuilder>(
getPerson(),
getParentForChildren(),
isClean());
person_ = null;
}
return personBuilder_;
}
private java.util.List identification_ =
java.util.Collections.emptyList();
private void ensureIdentificationIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
identification_ = new java.util.ArrayList(identification_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.IdentityID.ID, io.bloombox.schema.identity.IdentityID.ID.Builder, io.bloombox.schema.identity.IdentityID.IDOrBuilder> identificationBuilder_;
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public java.util.List getIdentificationList() {
if (identificationBuilder_ == null) {
return java.util.Collections.unmodifiableList(identification_);
} else {
return identificationBuilder_.getMessageList();
}
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public int getIdentificationCount() {
if (identificationBuilder_ == null) {
return identification_.size();
} else {
return identificationBuilder_.getCount();
}
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.ID getIdentification(int index) {
if (identificationBuilder_ == null) {
return identification_.get(index);
} else {
return identificationBuilder_.getMessage(index);
}
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder setIdentification(
int index, io.bloombox.schema.identity.IdentityID.ID value) {
if (identificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIdentificationIsMutable();
identification_.set(index, value);
onChanged();
} else {
identificationBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder setIdentification(
int index, io.bloombox.schema.identity.IdentityID.ID.Builder builderForValue) {
if (identificationBuilder_ == null) {
ensureIdentificationIsMutable();
identification_.set(index, builderForValue.build());
onChanged();
} else {
identificationBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder addIdentification(io.bloombox.schema.identity.IdentityID.ID value) {
if (identificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIdentificationIsMutable();
identification_.add(value);
onChanged();
} else {
identificationBuilder_.addMessage(value);
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder addIdentification(
int index, io.bloombox.schema.identity.IdentityID.ID value) {
if (identificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIdentificationIsMutable();
identification_.add(index, value);
onChanged();
} else {
identificationBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder addIdentification(
io.bloombox.schema.identity.IdentityID.ID.Builder builderForValue) {
if (identificationBuilder_ == null) {
ensureIdentificationIsMutable();
identification_.add(builderForValue.build());
onChanged();
} else {
identificationBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder addIdentification(
int index, io.bloombox.schema.identity.IdentityID.ID.Builder builderForValue) {
if (identificationBuilder_ == null) {
ensureIdentificationIsMutable();
identification_.add(index, builderForValue.build());
onChanged();
} else {
identificationBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder addAllIdentification(
java.lang.Iterable extends io.bloombox.schema.identity.IdentityID.ID> values) {
if (identificationBuilder_ == null) {
ensureIdentificationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, identification_);
onChanged();
} else {
identificationBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder clearIdentification() {
if (identificationBuilder_ == null) {
identification_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
identificationBuilder_.clear();
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public Builder removeIdentification(int index) {
if (identificationBuilder_ == null) {
ensureIdentificationIsMutable();
identification_.remove(index);
onChanged();
} else {
identificationBuilder_.remove(index);
}
return this;
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.ID.Builder getIdentificationBuilder(
int index) {
return getIdentificationFieldBuilder().getBuilder(index);
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.IDOrBuilder getIdentificationOrBuilder(
int index) {
if (identificationBuilder_ == null) {
return identification_.get(index); } else {
return identificationBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public java.util.List extends io.bloombox.schema.identity.IdentityID.IDOrBuilder>
getIdentificationOrBuilderList() {
if (identificationBuilder_ != null) {
return identificationBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(identification_);
}
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.ID.Builder addIdentificationBuilder() {
return getIdentificationFieldBuilder().addBuilder(
io.bloombox.schema.identity.IdentityID.ID.getDefaultInstance());
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.IdentityID.ID.Builder addIdentificationBuilder(
int index) {
return getIdentificationFieldBuilder().addBuilder(
index, io.bloombox.schema.identity.IdentityID.ID.getDefaultInstance());
}
/**
*
* Government ID associated with this user.
*
*
* repeated .bloombox.identity.ID identification = 20 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Government ID associated with this user.", (.core.collection) = { ... }
*/
public java.util.List
getIdentificationBuilderList() {
return getIdentificationFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.IdentityID.ID, io.bloombox.schema.identity.IdentityID.ID.Builder, io.bloombox.schema.identity.IdentityID.IDOrBuilder>
getIdentificationFieldBuilder() {
if (identificationBuilder_ == null) {
identificationBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.IdentityID.ID, io.bloombox.schema.identity.IdentityID.ID.Builder, io.bloombox.schema.identity.IdentityID.IDOrBuilder>(
identification_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
identification_ = null;
}
return identificationBuilder_;
}
private java.util.List doctorRec_ =
java.util.Collections.emptyList();
private void ensureDoctorRecIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
doctorRec_ = new java.util.ArrayList(doctorRec_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder> doctorRecBuilder_;
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public java.util.List getDoctorRecList() {
if (doctorRecBuilder_ == null) {
return java.util.Collections.unmodifiableList(doctorRec_);
} else {
return doctorRecBuilder_.getMessageList();
}
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public int getDoctorRecCount() {
if (doctorRecBuilder_ == null) {
return doctorRec_.size();
} else {
return doctorRecBuilder_.getCount();
}
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec getDoctorRec(int index) {
if (doctorRecBuilder_ == null) {
return doctorRec_.get(index);
} else {
return doctorRecBuilder_.getMessage(index);
}
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder setDoctorRec(
int index, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec value) {
if (doctorRecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDoctorRecIsMutable();
doctorRec_.set(index, value);
onChanged();
} else {
doctorRecBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder setDoctorRec(
int index, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder builderForValue) {
if (doctorRecBuilder_ == null) {
ensureDoctorRecIsMutable();
doctorRec_.set(index, builderForValue.build());
onChanged();
} else {
doctorRecBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder addDoctorRec(io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec value) {
if (doctorRecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDoctorRecIsMutable();
doctorRec_.add(value);
onChanged();
} else {
doctorRecBuilder_.addMessage(value);
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder addDoctorRec(
int index, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec value) {
if (doctorRecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDoctorRecIsMutable();
doctorRec_.add(index, value);
onChanged();
} else {
doctorRecBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder addDoctorRec(
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder builderForValue) {
if (doctorRecBuilder_ == null) {
ensureDoctorRecIsMutable();
doctorRec_.add(builderForValue.build());
onChanged();
} else {
doctorRecBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder addDoctorRec(
int index, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder builderForValue) {
if (doctorRecBuilder_ == null) {
ensureDoctorRecIsMutable();
doctorRec_.add(index, builderForValue.build());
onChanged();
} else {
doctorRecBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder addAllDoctorRec(
java.lang.Iterable extends io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec> values) {
if (doctorRecBuilder_ == null) {
ensureDoctorRecIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, doctorRec_);
onChanged();
} else {
doctorRecBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder clearDoctorRec() {
if (doctorRecBuilder_ == null) {
doctorRec_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
doctorRecBuilder_.clear();
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public Builder removeDoctorRec(int index) {
if (doctorRecBuilder_ == null) {
ensureDoctorRecIsMutable();
doctorRec_.remove(index);
onChanged();
} else {
doctorRecBuilder_.remove(index);
}
return this;
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder getDoctorRecBuilder(
int index) {
return getDoctorRecFieldBuilder().getBuilder(index);
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder getDoctorRecOrBuilder(
int index) {
if (doctorRecBuilder_ == null) {
return doctorRec_.get(index); } else {
return doctorRecBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public java.util.List extends io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder>
getDoctorRecOrBuilderList() {
if (doctorRecBuilder_ != null) {
return doctorRecBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(doctorRec_);
}
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder addDoctorRecBuilder() {
return getDoctorRecFieldBuilder().addBuilder(
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.getDefaultInstance());
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder addDoctorRecBuilder(
int index) {
return getDoctorRecFieldBuilder().addBuilder(
index, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.getDefaultInstance());
}
/**
*
* Doctor's recommendations associated with this user.
*
*
* repeated .bloombox.identity.ids.UserDoctorRec doctor_rec = 21 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Doctor\'s recommendations associated with this user.", (.core.collection) = { ... }
*/
public java.util.List
getDoctorRecBuilderList() {
return getDoctorRecFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder>
getDoctorRecFieldBuilder() {
if (doctorRecBuilder_ == null) {
doctorRecBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRec.Builder, io.bloombox.schema.identity.ids.PrescriptionID.UserDoctorRecOrBuilder>(
doctorRec_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
doctorRec_ = null;
}
return doctorRecBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Last time this user was seen, via auth/login/enrollment etc.
*
*
* .opencannabis.temporal.Instant seen = 30 [(.gen_bq_schema.description) = "Last time this user was seen, via auth/login/enrollment etc."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant signup_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> signupBuilder_;
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public boolean hasSignup() {
return signupBuilder_ != null || signup_ != null;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSignup() {
if (signupBuilder_ == null) {
return signup_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signup_;
} else {
return signupBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public Builder setSignup(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (signupBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signup_ = value;
onChanged();
} else {
signupBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public Builder setSignup(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (signupBuilder_ == null) {
signup_ = builderForValue.build();
onChanged();
} else {
signupBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public Builder mergeSignup(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (signupBuilder_ == null) {
if (signup_ != null) {
signup_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(signup_).mergeFrom(value).buildPartial();
} else {
signup_ = value;
}
onChanged();
} else {
signupBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public Builder clearSignup() {
if (signupBuilder_ == null) {
signup_ = null;
onChanged();
} else {
signup_ = null;
signupBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSignupBuilder() {
onChanged();
return getSignupFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignupOrBuilder() {
if (signupBuilder_ != null) {
return signupBuilder_.getMessageOrBuilder();
} else {
return signup_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signup_;
}
}
/**
*
* Timestamp for when this user was created.
*
*
* .opencannabis.temporal.Instant signup = 31 [(.gen_bq_schema.description) = "Timestamp for when this user was created."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSignupFieldBuilder() {
if (signupBuilder_ == null) {
signupBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSignup(),
getParentForChildren(),
isClean());
signup_ = null;
}
return signupBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, io.bloombox.schema.identity.AppUser.UserIdentity> identities_;
private com.google.protobuf.MapField
internalGetIdentities() {
if (identities_ == null) {
return com.google.protobuf.MapField.emptyMapField(
IdentitiesDefaultEntryHolder.defaultEntry);
}
return identities_;
}
private com.google.protobuf.MapField
internalGetMutableIdentities() {
onChanged();;
if (identities_ == null) {
identities_ = com.google.protobuf.MapField.newMapField(
IdentitiesDefaultEntryHolder.defaultEntry);
}
if (!identities_.isMutable()) {
identities_ = identities_.copy();
}
return identities_;
}
public int getIdentitiesCount() {
return internalGetIdentities().getMap().size();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public boolean containsIdentities(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetIdentities().getMap().containsKey(key);
}
/**
* Use {@link #getIdentitiesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getIdentities() {
return getIdentitiesMap();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public java.util.Map getIdentitiesMap() {
return internalGetIdentities().getMap();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrDefault(
java.lang.String key,
io.bloombox.schema.identity.AppUser.UserIdentity defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetIdentities().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public io.bloombox.schema.identity.AppUser.UserIdentity getIdentitiesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetIdentities().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearIdentities() {
internalGetMutableIdentities().getMutableMap()
.clear();
return this;
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public Builder removeIdentities(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableIdentities().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableIdentities() {
return internalGetMutableIdentities().getMutableMap();
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public Builder putIdentities(
java.lang.String key,
io.bloombox.schema.identity.AppUser.UserIdentity value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableIdentities().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Identities associated with this user.
*
*
* map<string, .bloombox.identity.UserIdentity> identities = 40 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Identities associated with this user."];
*/
public Builder putAllIdentities(
java.util.Map values) {
internalGetMutableIdentities().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, io.opencannabis.schema.media.AttachedMedia.MediaItem> media_;
private com.google.protobuf.MapField
internalGetMedia() {
if (media_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MediaDefaultEntryHolder.defaultEntry);
}
return media_;
}
private com.google.protobuf.MapField
internalGetMutableMedia() {
onChanged();;
if (media_ == null) {
media_ = com.google.protobuf.MapField.newMapField(
MediaDefaultEntryHolder.defaultEntry);
}
if (!media_.isMutable()) {
media_ = media_.copy();
}
return media_;
}
public int getMediaCount() {
return internalGetMedia().getMap().size();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public boolean containsMedia(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetMedia().getMap().containsKey(key);
}
/**
* Use {@link #getMediaMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getMedia() {
return getMediaMap();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public java.util.Map getMediaMap() {
return internalGetMedia().getMap();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrDefault(
java.lang.String key,
io.opencannabis.schema.media.AttachedMedia.MediaItem defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMedia().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public io.opencannabis.schema.media.AttachedMedia.MediaItem getMediaOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetMedia().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMedia() {
internalGetMutableMedia().getMutableMap()
.clear();
return this;
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public Builder removeMedia(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMedia().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMedia() {
return internalGetMutableMedia().getMutableMap();
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public Builder putMedia(
java.lang.String key,
io.opencannabis.schema.media.AttachedMedia.MediaItem value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableMedia().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Media associated with this user.
*
*
* map<string, .opencannabis.media.MediaItem> media = 41 [(.gen_bq_schema.ignore) = true, (.gen_bq_schema.description) = "Media associated with this user."];
*/
public Builder putAllMedia(
java.util.Map values) {
internalGetMutableMedia().getMutableMap()
.putAll(values);
return this;
}
private io.bloombox.schema.identity.AppUser.ConsumerProfile consumer_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerProfile, io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder, io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder> consumerBuilder_;
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public boolean hasConsumer() {
return consumerBuilder_ != null || consumer_ != null;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerProfile getConsumer() {
if (consumerBuilder_ == null) {
return consumer_ == null ? io.bloombox.schema.identity.AppUser.ConsumerProfile.getDefaultInstance() : consumer_;
} else {
return consumerBuilder_.getMessage();
}
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public Builder setConsumer(io.bloombox.schema.identity.AppUser.ConsumerProfile value) {
if (consumerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consumer_ = value;
onChanged();
} else {
consumerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public Builder setConsumer(
io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder builderForValue) {
if (consumerBuilder_ == null) {
consumer_ = builderForValue.build();
onChanged();
} else {
consumerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public Builder mergeConsumer(io.bloombox.schema.identity.AppUser.ConsumerProfile value) {
if (consumerBuilder_ == null) {
if (consumer_ != null) {
consumer_ =
io.bloombox.schema.identity.AppUser.ConsumerProfile.newBuilder(consumer_).mergeFrom(value).buildPartial();
} else {
consumer_ = value;
}
onChanged();
} else {
consumerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public Builder clearConsumer() {
if (consumerBuilder_ == null) {
consumer_ = null;
onChanged();
} else {
consumer_ = null;
consumerBuilder_ = null;
}
return this;
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder getConsumerBuilder() {
onChanged();
return getConsumerFieldBuilder().getBuilder();
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder getConsumerOrBuilder() {
if (consumerBuilder_ != null) {
return consumerBuilder_.getMessageOrBuilder();
} else {
return consumer_ == null ?
io.bloombox.schema.identity.AppUser.ConsumerProfile.getDefaultInstance() : consumer_;
}
}
/**
*
* Consumer profile for this user.
*
*
* .bloombox.identity.ConsumerProfile consumer = 100 [(.gen_bq_schema.description) = "Consumer profile for this user."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerProfile, io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder, io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder>
getConsumerFieldBuilder() {
if (consumerBuilder_ == null) {
consumerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerProfile, io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder, io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder>(
getConsumer(),
getParentForChildren(),
isClean());
consumer_ = null;
}
return consumerBuilder_;
}
private io.bloombox.schema.identity.AppUser.IndustryProfile industry_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.IndustryProfile, io.bloombox.schema.identity.AppUser.IndustryProfile.Builder, io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder> industryBuilder_;
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public boolean hasIndustry() {
return industryBuilder_ != null || industry_ != null;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.IndustryProfile getIndustry() {
if (industryBuilder_ == null) {
return industry_ == null ? io.bloombox.schema.identity.AppUser.IndustryProfile.getDefaultInstance() : industry_;
} else {
return industryBuilder_.getMessage();
}
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public Builder setIndustry(io.bloombox.schema.identity.AppUser.IndustryProfile value) {
if (industryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
industry_ = value;
onChanged();
} else {
industryBuilder_.setMessage(value);
}
return this;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public Builder setIndustry(
io.bloombox.schema.identity.AppUser.IndustryProfile.Builder builderForValue) {
if (industryBuilder_ == null) {
industry_ = builderForValue.build();
onChanged();
} else {
industryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public Builder mergeIndustry(io.bloombox.schema.identity.AppUser.IndustryProfile value) {
if (industryBuilder_ == null) {
if (industry_ != null) {
industry_ =
io.bloombox.schema.identity.AppUser.IndustryProfile.newBuilder(industry_).mergeFrom(value).buildPartial();
} else {
industry_ = value;
}
onChanged();
} else {
industryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public Builder clearIndustry() {
if (industryBuilder_ == null) {
industry_ = null;
onChanged();
} else {
industry_ = null;
industryBuilder_ = null;
}
return this;
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.IndustryProfile.Builder getIndustryBuilder() {
onChanged();
return getIndustryFieldBuilder().getBuilder();
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
public io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder getIndustryOrBuilder() {
if (industryBuilder_ != null) {
return industryBuilder_.getMessageOrBuilder();
} else {
return industry_ == null ?
io.bloombox.schema.identity.AppUser.IndustryProfile.getDefaultInstance() : industry_;
}
}
/**
*
* Industry profile for this user.
*
*
* .bloombox.identity.IndustryProfile industry = 101 [(.gen_bq_schema.description) = "Industry profile for this user."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.IndustryProfile, io.bloombox.schema.identity.AppUser.IndustryProfile.Builder, io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder>
getIndustryFieldBuilder() {
if (industryBuilder_ == null) {
industryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.IndustryProfile, io.bloombox.schema.identity.AppUser.IndustryProfile.Builder, io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder>(
getIndustry(),
getParentForChildren(),
isClean());
industry_ = null;
}
return industryBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.User)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.User)
private static final io.bloombox.schema.identity.AppUser.User DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.User();
}
public static io.bloombox.schema.identity.AppUser.User getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public User parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new User(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.User getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserFlagsOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.UserFlags)
com.google.protobuf.MessageOrBuilder {
/**
*
* Indicates that the account has been validated.
*
*
* bool validated = 1 [(.gen_bq_schema.description) = "Indicates that the account has been validated."];
*/
boolean getValidated();
/**
*
* Indicates that the account is currently suspended.
*
*
* bool suspended = 2 [(.gen_bq_schema.description) = "Indicates that the account is currently suspended."];
*/
boolean getSuspended();
/**
*
* Indicates that the account has admin privileges.
*
*
* bool admin = 3 [(.gen_bq_schema.description) = "Indicates that the account has admin privileges."];
*/
boolean getAdmin();
/**
*
* Indicates that the account has access to beta systems.
*
*
* bool beta = 4 [(.gen_bq_schema.description) = "Indicates that the account has access to beta systems."];
*/
boolean getBeta();
/**
*
* Indicates that the account has access to sandbox systems.
*
*
* bool sandbox = 5 [(.gen_bq_schema.description) = "Indicates that the account has access to sandbox systems."];
*/
boolean getSandbox();
}
/**
*
* Boolean flags that may be set on a user account.
*
*
* Protobuf type {@code bloombox.identity.UserFlags}
*/
public static final class UserFlags extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.UserFlags)
UserFlagsOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserFlags.newBuilder() to construct.
private UserFlags(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserFlags() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UserFlags(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
validated_ = input.readBool();
break;
}
case 16: {
suspended_ = input.readBool();
break;
}
case 24: {
admin_ = input.readBool();
break;
}
case 32: {
beta_ = input.readBool();
break;
}
case 40: {
sandbox_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserFlags_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserFlags_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.UserFlags.class, io.bloombox.schema.identity.AppUser.UserFlags.Builder.class);
}
public static final int VALIDATED_FIELD_NUMBER = 1;
private boolean validated_;
/**
*
* Indicates that the account has been validated.
*
*
* bool validated = 1 [(.gen_bq_schema.description) = "Indicates that the account has been validated."];
*/
public boolean getValidated() {
return validated_;
}
public static final int SUSPENDED_FIELD_NUMBER = 2;
private boolean suspended_;
/**
*
* Indicates that the account is currently suspended.
*
*
* bool suspended = 2 [(.gen_bq_schema.description) = "Indicates that the account is currently suspended."];
*/
public boolean getSuspended() {
return suspended_;
}
public static final int ADMIN_FIELD_NUMBER = 3;
private boolean admin_;
/**
*
* Indicates that the account has admin privileges.
*
*
* bool admin = 3 [(.gen_bq_schema.description) = "Indicates that the account has admin privileges."];
*/
public boolean getAdmin() {
return admin_;
}
public static final int BETA_FIELD_NUMBER = 4;
private boolean beta_;
/**
*
* Indicates that the account has access to beta systems.
*
*
* bool beta = 4 [(.gen_bq_schema.description) = "Indicates that the account has access to beta systems."];
*/
public boolean getBeta() {
return beta_;
}
public static final int SANDBOX_FIELD_NUMBER = 5;
private boolean sandbox_;
/**
*
* Indicates that the account has access to sandbox systems.
*
*
* bool sandbox = 5 [(.gen_bq_schema.description) = "Indicates that the account has access to sandbox systems."];
*/
public boolean getSandbox() {
return sandbox_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (validated_ != false) {
output.writeBool(1, validated_);
}
if (suspended_ != false) {
output.writeBool(2, suspended_);
}
if (admin_ != false) {
output.writeBool(3, admin_);
}
if (beta_ != false) {
output.writeBool(4, beta_);
}
if (sandbox_ != false) {
output.writeBool(5, sandbox_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (validated_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, validated_);
}
if (suspended_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, suspended_);
}
if (admin_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, admin_);
}
if (beta_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, beta_);
}
if (sandbox_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, sandbox_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.UserFlags)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.UserFlags other = (io.bloombox.schema.identity.AppUser.UserFlags) obj;
if (getValidated()
!= other.getValidated()) return false;
if (getSuspended()
!= other.getSuspended()) return false;
if (getAdmin()
!= other.getAdmin()) return false;
if (getBeta()
!= other.getBeta()) return false;
if (getSandbox()
!= other.getSandbox()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALIDATED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getValidated());
hash = (37 * hash) + SUSPENDED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSuspended());
hash = (37 * hash) + ADMIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAdmin());
hash = (37 * hash) + BETA_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBeta());
hash = (37 * hash) + SANDBOX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSandbox());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserFlags parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.UserFlags prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Boolean flags that may be set on a user account.
*
*
* Protobuf type {@code bloombox.identity.UserFlags}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.UserFlags)
io.bloombox.schema.identity.AppUser.UserFlagsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserFlags_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserFlags_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.UserFlags.class, io.bloombox.schema.identity.AppUser.UserFlags.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.UserFlags.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
validated_ = false;
suspended_ = false;
admin_ = false;
beta_ = false;
sandbox_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserFlags_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserFlags getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.UserFlags.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserFlags build() {
io.bloombox.schema.identity.AppUser.UserFlags result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserFlags buildPartial() {
io.bloombox.schema.identity.AppUser.UserFlags result = new io.bloombox.schema.identity.AppUser.UserFlags(this);
result.validated_ = validated_;
result.suspended_ = suspended_;
result.admin_ = admin_;
result.beta_ = beta_;
result.sandbox_ = sandbox_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.UserFlags) {
return mergeFrom((io.bloombox.schema.identity.AppUser.UserFlags)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.UserFlags other) {
if (other == io.bloombox.schema.identity.AppUser.UserFlags.getDefaultInstance()) return this;
if (other.getValidated() != false) {
setValidated(other.getValidated());
}
if (other.getSuspended() != false) {
setSuspended(other.getSuspended());
}
if (other.getAdmin() != false) {
setAdmin(other.getAdmin());
}
if (other.getBeta() != false) {
setBeta(other.getBeta());
}
if (other.getSandbox() != false) {
setSandbox(other.getSandbox());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.UserFlags parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.UserFlags) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean validated_ ;
/**
*
* Indicates that the account has been validated.
*
*
* bool validated = 1 [(.gen_bq_schema.description) = "Indicates that the account has been validated."];
*/
public boolean getValidated() {
return validated_;
}
/**
*
* Indicates that the account has been validated.
*
*
* bool validated = 1 [(.gen_bq_schema.description) = "Indicates that the account has been validated."];
*/
public Builder setValidated(boolean value) {
validated_ = value;
onChanged();
return this;
}
/**
*
* Indicates that the account has been validated.
*
*
* bool validated = 1 [(.gen_bq_schema.description) = "Indicates that the account has been validated."];
*/
public Builder clearValidated() {
validated_ = false;
onChanged();
return this;
}
private boolean suspended_ ;
/**
*
* Indicates that the account is currently suspended.
*
*
* bool suspended = 2 [(.gen_bq_schema.description) = "Indicates that the account is currently suspended."];
*/
public boolean getSuspended() {
return suspended_;
}
/**
*
* Indicates that the account is currently suspended.
*
*
* bool suspended = 2 [(.gen_bq_schema.description) = "Indicates that the account is currently suspended."];
*/
public Builder setSuspended(boolean value) {
suspended_ = value;
onChanged();
return this;
}
/**
*
* Indicates that the account is currently suspended.
*
*
* bool suspended = 2 [(.gen_bq_schema.description) = "Indicates that the account is currently suspended."];
*/
public Builder clearSuspended() {
suspended_ = false;
onChanged();
return this;
}
private boolean admin_ ;
/**
*
* Indicates that the account has admin privileges.
*
*
* bool admin = 3 [(.gen_bq_schema.description) = "Indicates that the account has admin privileges."];
*/
public boolean getAdmin() {
return admin_;
}
/**
*
* Indicates that the account has admin privileges.
*
*
* bool admin = 3 [(.gen_bq_schema.description) = "Indicates that the account has admin privileges."];
*/
public Builder setAdmin(boolean value) {
admin_ = value;
onChanged();
return this;
}
/**
*
* Indicates that the account has admin privileges.
*
*
* bool admin = 3 [(.gen_bq_schema.description) = "Indicates that the account has admin privileges."];
*/
public Builder clearAdmin() {
admin_ = false;
onChanged();
return this;
}
private boolean beta_ ;
/**
*
* Indicates that the account has access to beta systems.
*
*
* bool beta = 4 [(.gen_bq_schema.description) = "Indicates that the account has access to beta systems."];
*/
public boolean getBeta() {
return beta_;
}
/**
*
* Indicates that the account has access to beta systems.
*
*
* bool beta = 4 [(.gen_bq_schema.description) = "Indicates that the account has access to beta systems."];
*/
public Builder setBeta(boolean value) {
beta_ = value;
onChanged();
return this;
}
/**
*
* Indicates that the account has access to beta systems.
*
*
* bool beta = 4 [(.gen_bq_schema.description) = "Indicates that the account has access to beta systems."];
*/
public Builder clearBeta() {
beta_ = false;
onChanged();
return this;
}
private boolean sandbox_ ;
/**
*
* Indicates that the account has access to sandbox systems.
*
*
* bool sandbox = 5 [(.gen_bq_schema.description) = "Indicates that the account has access to sandbox systems."];
*/
public boolean getSandbox() {
return sandbox_;
}
/**
*
* Indicates that the account has access to sandbox systems.
*
*
* bool sandbox = 5 [(.gen_bq_schema.description) = "Indicates that the account has access to sandbox systems."];
*/
public Builder setSandbox(boolean value) {
sandbox_ = value;
onChanged();
return this;
}
/**
*
* Indicates that the account has access to sandbox systems.
*
*
* bool sandbox = 5 [(.gen_bq_schema.description) = "Indicates that the account has access to sandbox systems."];
*/
public Builder clearSandbox() {
sandbox_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.UserFlags)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.UserFlags)
private static final io.bloombox.schema.identity.AppUser.UserFlags DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.UserFlags();
}
public static io.bloombox.schema.identity.AppUser.UserFlags getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserFlags parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserFlags(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserFlags getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UserIdentityOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.UserIdentity)
com.google.protobuf.MessageOrBuilder {
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
int getProviderValue();
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
io.bloombox.schema.identity.AppUser.IdentityProvider getProvider();
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
java.lang.String getId();
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
boolean hasSeen();
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
}
/**
*
* Represents an identity that may be associated with a user account.
*
*
* Protobuf type {@code bloombox.identity.UserIdentity}
*/
public static final class UserIdentity extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.UserIdentity)
UserIdentityOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserIdentity.newBuilder() to construct.
private UserIdentity(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserIdentity() {
provider_ = 0;
id_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UserIdentity(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
provider_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserIdentity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserIdentity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.UserIdentity.class, io.bloombox.schema.identity.AppUser.UserIdentity.Builder.class);
}
public static final int PROVIDER_FIELD_NUMBER = 1;
private int provider_;
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public int getProviderValue() {
return provider_;
}
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public io.bloombox.schema.identity.AppUser.IdentityProvider getProvider() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.IdentityProvider result = io.bloombox.schema.identity.AppUser.IdentityProvider.valueOf(provider_);
return result == null ? io.bloombox.schema.identity.AppUser.IdentityProvider.UNRECOGNIZED : result;
}
public static final int ID_FIELD_NUMBER = 2;
private volatile java.lang.Object id_;
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEEN_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (provider_ != io.bloombox.schema.identity.AppUser.IdentityProvider.EMAIL.getNumber()) {
output.writeEnum(1, provider_);
}
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, id_);
}
if (seen_ != null) {
output.writeMessage(3, getSeen());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (provider_ != io.bloombox.schema.identity.AppUser.IdentityProvider.EMAIL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, provider_);
}
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, id_);
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSeen());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.UserIdentity)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.UserIdentity other = (io.bloombox.schema.identity.AppUser.UserIdentity) obj;
if (provider_ != other.provider_) return false;
if (!getId()
.equals(other.getId())) return false;
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROVIDER_FIELD_NUMBER;
hash = (53 * hash) + provider_;
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.UserIdentity parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.UserIdentity prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents an identity that may be associated with a user account.
*
*
* Protobuf type {@code bloombox.identity.UserIdentity}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.UserIdentity)
io.bloombox.schema.identity.AppUser.UserIdentityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserIdentity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserIdentity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.UserIdentity.class, io.bloombox.schema.identity.AppUser.UserIdentity.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.UserIdentity.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
provider_ = 0;
id_ = "";
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_UserIdentity_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserIdentity getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.UserIdentity.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserIdentity build() {
io.bloombox.schema.identity.AppUser.UserIdentity result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserIdentity buildPartial() {
io.bloombox.schema.identity.AppUser.UserIdentity result = new io.bloombox.schema.identity.AppUser.UserIdentity(this);
result.provider_ = provider_;
result.id_ = id_;
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.UserIdentity) {
return mergeFrom((io.bloombox.schema.identity.AppUser.UserIdentity)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.UserIdentity other) {
if (other == io.bloombox.schema.identity.AppUser.UserIdentity.getDefaultInstance()) return this;
if (other.provider_ != 0) {
setProviderValue(other.getProviderValue());
}
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.UserIdentity parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.UserIdentity) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int provider_ = 0;
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public int getProviderValue() {
return provider_;
}
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public Builder setProviderValue(int value) {
provider_ = value;
onChanged();
return this;
}
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public io.bloombox.schema.identity.AppUser.IdentityProvider getProvider() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.IdentityProvider result = io.bloombox.schema.identity.AppUser.IdentityProvider.valueOf(provider_);
return result == null ? io.bloombox.schema.identity.AppUser.IdentityProvider.UNRECOGNIZED : result;
}
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public Builder setProvider(io.bloombox.schema.identity.AppUser.IdentityProvider value) {
if (value == null) {
throw new NullPointerException();
}
provider_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Provider for this external/foreign account.
*
*
* .bloombox.identity.IdentityProvider provider = 1 [(.gen_bq_schema.description) = "Provider for this external/foreign account."];
*/
public Builder clearProvider() {
provider_ = 0;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Unique ID for this account with the specified provider.
*
*
* string id = 2 [(.gen_bq_schema.description) = "Unique ID for this account with the specified provider.", (.core.field) = { ... }
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Timestamp for when this identity was last user to authenticate the underlying user.
*
*
* .opencannabis.temporal.Instant seen = 3 [(.gen_bq_schema.description) = "Timestamp for when this identity was last user to authenticate the underlying user."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.UserIdentity)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.UserIdentity)
private static final io.bloombox.schema.identity.AppUser.UserIdentity DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.UserIdentity();
}
public static io.bloombox.schema.identity.AppUser.UserIdentity getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserIdentity parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserIdentity(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.UserIdentity getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConsumerProfileOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.ConsumerProfile)
com.google.protobuf.MessageOrBuilder {
/**
*
* Profile active/inactive flag.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile active/inactive flag."];
*/
boolean getActive();
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
java.util.List
getFavoriteDispensariesList();
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
int getFavoriteDispensariesCount();
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
java.lang.String getFavoriteDispensaries(int index);
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
com.google.protobuf.ByteString
getFavoriteDispensariesBytes(int index);
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
int getEnrollmentSourceValue();
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
io.bloombox.schema.identity.AppUser.EnrollmentSource getEnrollmentSource();
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
java.lang.String getEnrollmentChannel();
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
com.google.protobuf.ByteString
getEnrollmentChannelBytes();
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
boolean hasPreferences();
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
io.bloombox.schema.identity.AppUser.ConsumerPreferences getPreferences();
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder getPreferencesOrBuilder();
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
int getTypeValue();
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
io.bloombox.schema.identity.AppUser.ConsumerType getType();
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
int getReferralSourceValue();
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
io.bloombox.schema.identity.AppUser.ReferralSource getReferralSource();
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
java.lang.String getReferralDetail();
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
com.google.protobuf.ByteString
getReferralDetailBytes();
}
/**
*
* User profile information for a cannabis consumer.
*
*
* Protobuf type {@code bloombox.identity.ConsumerProfile}
*/
public static final class ConsumerProfile extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.ConsumerProfile)
ConsumerProfileOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConsumerProfile.newBuilder() to construct.
private ConsumerProfile(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConsumerProfile() {
favoriteDispensaries_ = com.google.protobuf.LazyStringArrayList.EMPTY;
enrollmentSource_ = 0;
enrollmentChannel_ = "";
type_ = 0;
referralSource_ = 0;
referralDetail_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConsumerProfile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
active_ = input.readBool();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
favoriteDispensaries_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
favoriteDispensaries_.add(s);
break;
}
case 24: {
int rawValue = input.readEnum();
enrollmentSource_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
enrollmentChannel_ = s;
break;
}
case 42: {
io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder subBuilder = null;
if (preferences_ != null) {
subBuilder = preferences_.toBuilder();
}
preferences_ = input.readMessage(io.bloombox.schema.identity.AppUser.ConsumerPreferences.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(preferences_);
preferences_ = subBuilder.buildPartial();
}
break;
}
case 48: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 56: {
int rawValue = input.readEnum();
referralSource_ = rawValue;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
referralDetail_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
favoriteDispensaries_ = favoriteDispensaries_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerProfile_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerProfile.class, io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder.class);
}
private int bitField0_;
public static final int ACTIVE_FIELD_NUMBER = 1;
private boolean active_;
/**
*
* Profile active/inactive flag.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile active/inactive flag."];
*/
public boolean getActive() {
return active_;
}
public static final int FAVORITE_DISPENSARIES_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList favoriteDispensaries_;
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public com.google.protobuf.ProtocolStringList
getFavoriteDispensariesList() {
return favoriteDispensaries_;
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public int getFavoriteDispensariesCount() {
return favoriteDispensaries_.size();
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public java.lang.String getFavoriteDispensaries(int index) {
return favoriteDispensaries_.get(index);
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public com.google.protobuf.ByteString
getFavoriteDispensariesBytes(int index) {
return favoriteDispensaries_.getByteString(index);
}
public static final int ENROLLMENT_SOURCE_FIELD_NUMBER = 3;
private int enrollmentSource_;
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public int getEnrollmentSourceValue() {
return enrollmentSource_;
}
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public io.bloombox.schema.identity.AppUser.EnrollmentSource getEnrollmentSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.EnrollmentSource result = io.bloombox.schema.identity.AppUser.EnrollmentSource.valueOf(enrollmentSource_);
return result == null ? io.bloombox.schema.identity.AppUser.EnrollmentSource.UNRECOGNIZED : result;
}
public static final int ENROLLMENT_CHANNEL_FIELD_NUMBER = 4;
private volatile java.lang.Object enrollmentChannel_;
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public java.lang.String getEnrollmentChannel() {
java.lang.Object ref = enrollmentChannel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
enrollmentChannel_ = s;
return s;
}
}
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public com.google.protobuf.ByteString
getEnrollmentChannelBytes() {
java.lang.Object ref = enrollmentChannel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enrollmentChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PREFERENCES_FIELD_NUMBER = 5;
private io.bloombox.schema.identity.AppUser.ConsumerPreferences preferences_;
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public boolean hasPreferences() {
return preferences_ != null;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerPreferences getPreferences() {
return preferences_ == null ? io.bloombox.schema.identity.AppUser.ConsumerPreferences.getDefaultInstance() : preferences_;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder getPreferencesOrBuilder() {
return getPreferences();
}
public static final int TYPE_FIELD_NUMBER = 6;
private int type_;
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.ConsumerType result = io.bloombox.schema.identity.AppUser.ConsumerType.valueOf(type_);
return result == null ? io.bloombox.schema.identity.AppUser.ConsumerType.UNRECOGNIZED : result;
}
public static final int REFERRAL_SOURCE_FIELD_NUMBER = 7;
private int referralSource_;
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public int getReferralSourceValue() {
return referralSource_;
}
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public io.bloombox.schema.identity.AppUser.ReferralSource getReferralSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.ReferralSource result = io.bloombox.schema.identity.AppUser.ReferralSource.valueOf(referralSource_);
return result == null ? io.bloombox.schema.identity.AppUser.ReferralSource.UNRECOGNIZED : result;
}
public static final int REFERRAL_DETAIL_FIELD_NUMBER = 8;
private volatile java.lang.Object referralDetail_;
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public java.lang.String getReferralDetail() {
java.lang.Object ref = referralDetail_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralDetail_ = s;
return s;
}
}
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public com.google.protobuf.ByteString
getReferralDetailBytes() {
java.lang.Object ref = referralDetail_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralDetail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (active_ != false) {
output.writeBool(1, active_);
}
for (int i = 0; i < favoriteDispensaries_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, favoriteDispensaries_.getRaw(i));
}
if (enrollmentSource_ != io.bloombox.schema.identity.AppUser.EnrollmentSource.UNSPECIFIED.getNumber()) {
output.writeEnum(3, enrollmentSource_);
}
if (!getEnrollmentChannelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, enrollmentChannel_);
}
if (preferences_ != null) {
output.writeMessage(5, getPreferences());
}
if (type_ != io.bloombox.schema.identity.AppUser.ConsumerType.UNVALIDATED.getNumber()) {
output.writeEnum(6, type_);
}
if (referralSource_ != io.bloombox.schema.identity.AppUser.ReferralSource.UNKNOWN.getNumber()) {
output.writeEnum(7, referralSource_);
}
if (!getReferralDetailBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, referralDetail_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (active_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, active_);
}
{
int dataSize = 0;
for (int i = 0; i < favoriteDispensaries_.size(); i++) {
dataSize += computeStringSizeNoTag(favoriteDispensaries_.getRaw(i));
}
size += dataSize;
size += 1 * getFavoriteDispensariesList().size();
}
if (enrollmentSource_ != io.bloombox.schema.identity.AppUser.EnrollmentSource.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, enrollmentSource_);
}
if (!getEnrollmentChannelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, enrollmentChannel_);
}
if (preferences_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getPreferences());
}
if (type_ != io.bloombox.schema.identity.AppUser.ConsumerType.UNVALIDATED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, type_);
}
if (referralSource_ != io.bloombox.schema.identity.AppUser.ReferralSource.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, referralSource_);
}
if (!getReferralDetailBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, referralDetail_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.ConsumerProfile)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.ConsumerProfile other = (io.bloombox.schema.identity.AppUser.ConsumerProfile) obj;
if (getActive()
!= other.getActive()) return false;
if (!getFavoriteDispensariesList()
.equals(other.getFavoriteDispensariesList())) return false;
if (enrollmentSource_ != other.enrollmentSource_) return false;
if (!getEnrollmentChannel()
.equals(other.getEnrollmentChannel())) return false;
if (hasPreferences() != other.hasPreferences()) return false;
if (hasPreferences()) {
if (!getPreferences()
.equals(other.getPreferences())) return false;
}
if (type_ != other.type_) return false;
if (referralSource_ != other.referralSource_) return false;
if (!getReferralDetail()
.equals(other.getReferralDetail())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getActive());
if (getFavoriteDispensariesCount() > 0) {
hash = (37 * hash) + FAVORITE_DISPENSARIES_FIELD_NUMBER;
hash = (53 * hash) + getFavoriteDispensariesList().hashCode();
}
hash = (37 * hash) + ENROLLMENT_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + enrollmentSource_;
hash = (37 * hash) + ENROLLMENT_CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getEnrollmentChannel().hashCode();
if (hasPreferences()) {
hash = (37 * hash) + PREFERENCES_FIELD_NUMBER;
hash = (53 * hash) + getPreferences().hashCode();
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + REFERRAL_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + referralSource_;
hash = (37 * hash) + REFERRAL_DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getReferralDetail().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.ConsumerProfile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* User profile information for a cannabis consumer.
*
*
* Protobuf type {@code bloombox.identity.ConsumerProfile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.ConsumerProfile)
io.bloombox.schema.identity.AppUser.ConsumerProfileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerProfile_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerProfile.class, io.bloombox.schema.identity.AppUser.ConsumerProfile.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.ConsumerProfile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
active_ = false;
favoriteDispensaries_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
enrollmentSource_ = 0;
enrollmentChannel_ = "";
if (preferencesBuilder_ == null) {
preferences_ = null;
} else {
preferences_ = null;
preferencesBuilder_ = null;
}
type_ = 0;
referralSource_ = 0;
referralDetail_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerProfile_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerProfile getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.ConsumerProfile.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerProfile build() {
io.bloombox.schema.identity.AppUser.ConsumerProfile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerProfile buildPartial() {
io.bloombox.schema.identity.AppUser.ConsumerProfile result = new io.bloombox.schema.identity.AppUser.ConsumerProfile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.active_ = active_;
if (((bitField0_ & 0x00000002) != 0)) {
favoriteDispensaries_ = favoriteDispensaries_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.favoriteDispensaries_ = favoriteDispensaries_;
result.enrollmentSource_ = enrollmentSource_;
result.enrollmentChannel_ = enrollmentChannel_;
if (preferencesBuilder_ == null) {
result.preferences_ = preferences_;
} else {
result.preferences_ = preferencesBuilder_.build();
}
result.type_ = type_;
result.referralSource_ = referralSource_;
result.referralDetail_ = referralDetail_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.ConsumerProfile) {
return mergeFrom((io.bloombox.schema.identity.AppUser.ConsumerProfile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.ConsumerProfile other) {
if (other == io.bloombox.schema.identity.AppUser.ConsumerProfile.getDefaultInstance()) return this;
if (other.getActive() != false) {
setActive(other.getActive());
}
if (!other.favoriteDispensaries_.isEmpty()) {
if (favoriteDispensaries_.isEmpty()) {
favoriteDispensaries_ = other.favoriteDispensaries_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureFavoriteDispensariesIsMutable();
favoriteDispensaries_.addAll(other.favoriteDispensaries_);
}
onChanged();
}
if (other.enrollmentSource_ != 0) {
setEnrollmentSourceValue(other.getEnrollmentSourceValue());
}
if (!other.getEnrollmentChannel().isEmpty()) {
enrollmentChannel_ = other.enrollmentChannel_;
onChanged();
}
if (other.hasPreferences()) {
mergePreferences(other.getPreferences());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.referralSource_ != 0) {
setReferralSourceValue(other.getReferralSourceValue());
}
if (!other.getReferralDetail().isEmpty()) {
referralDetail_ = other.referralDetail_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.ConsumerProfile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.ConsumerProfile) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private boolean active_ ;
/**
*
* Profile active/inactive flag.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile active/inactive flag."];
*/
public boolean getActive() {
return active_;
}
/**
*
* Profile active/inactive flag.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile active/inactive flag."];
*/
public Builder setActive(boolean value) {
active_ = value;
onChanged();
return this;
}
/**
*
* Profile active/inactive flag.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile active/inactive flag."];
*/
public Builder clearActive() {
active_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList favoriteDispensaries_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureFavoriteDispensariesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
favoriteDispensaries_ = new com.google.protobuf.LazyStringArrayList(favoriteDispensaries_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public com.google.protobuf.ProtocolStringList
getFavoriteDispensariesList() {
return favoriteDispensaries_.getUnmodifiableView();
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public int getFavoriteDispensariesCount() {
return favoriteDispensaries_.size();
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public java.lang.String getFavoriteDispensaries(int index) {
return favoriteDispensaries_.get(index);
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public com.google.protobuf.ByteString
getFavoriteDispensariesBytes(int index) {
return favoriteDispensaries_.getByteString(index);
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public Builder setFavoriteDispensaries(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureFavoriteDispensariesIsMutable();
favoriteDispensaries_.set(index, value);
onChanged();
return this;
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public Builder addFavoriteDispensaries(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureFavoriteDispensariesIsMutable();
favoriteDispensaries_.add(value);
onChanged();
return this;
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public Builder addAllFavoriteDispensaries(
java.lang.Iterable values) {
ensureFavoriteDispensariesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, favoriteDispensaries_);
onChanged();
return this;
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public Builder clearFavoriteDispensaries() {
favoriteDispensaries_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Enumerates favorite dispensaries for a user.
*
*
* repeated string favorite_dispensaries = 2 [(.gen_bq_schema.description) = "Enumerates favorite dispensaries for a user."];
*/
public Builder addFavoriteDispensariesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureFavoriteDispensariesIsMutable();
favoriteDispensaries_.add(value);
onChanged();
return this;
}
private int enrollmentSource_ = 0;
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public int getEnrollmentSourceValue() {
return enrollmentSource_;
}
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public Builder setEnrollmentSourceValue(int value) {
enrollmentSource_ = value;
onChanged();
return this;
}
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public io.bloombox.schema.identity.AppUser.EnrollmentSource getEnrollmentSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.EnrollmentSource result = io.bloombox.schema.identity.AppUser.EnrollmentSource.valueOf(enrollmentSource_);
return result == null ? io.bloombox.schema.identity.AppUser.EnrollmentSource.UNRECOGNIZED : result;
}
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public Builder setEnrollmentSource(io.bloombox.schema.identity.AppUser.EnrollmentSource value) {
if (value == null) {
throw new NullPointerException();
}
enrollmentSource_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies enrollment source attribution information.
*
*
* .bloombox.identity.EnrollmentSource enrollment_source = 3 [(.gen_bq_schema.description) = "Specifies enrollment source attribution information."];
*/
public Builder clearEnrollmentSource() {
enrollmentSource_ = 0;
onChanged();
return this;
}
private java.lang.Object enrollmentChannel_ = "";
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public java.lang.String getEnrollmentChannel() {
java.lang.Object ref = enrollmentChannel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
enrollmentChannel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public com.google.protobuf.ByteString
getEnrollmentChannelBytes() {
java.lang.Object ref = enrollmentChannel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
enrollmentChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public Builder setEnrollmentChannel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
enrollmentChannel_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public Builder clearEnrollmentChannel() {
enrollmentChannel_ = getDefaultInstance().getEnrollmentChannel();
onChanged();
return this;
}
/**
*
* Arbitrary string for the channel through which this user enrolled.
*
*
* string enrollment_channel = 4 [(.gen_bq_schema.description) = "Arbitrary string for the channel through which this user enrolled."];
*/
public Builder setEnrollmentChannelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
enrollmentChannel_ = value;
onChanged();
return this;
}
private io.bloombox.schema.identity.AppUser.ConsumerPreferences preferences_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerPreferences, io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder, io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder> preferencesBuilder_;
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public boolean hasPreferences() {
return preferencesBuilder_ != null || preferences_ != null;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerPreferences getPreferences() {
if (preferencesBuilder_ == null) {
return preferences_ == null ? io.bloombox.schema.identity.AppUser.ConsumerPreferences.getDefaultInstance() : preferences_;
} else {
return preferencesBuilder_.getMessage();
}
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public Builder setPreferences(io.bloombox.schema.identity.AppUser.ConsumerPreferences value) {
if (preferencesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
preferences_ = value;
onChanged();
} else {
preferencesBuilder_.setMessage(value);
}
return this;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public Builder setPreferences(
io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder builderForValue) {
if (preferencesBuilder_ == null) {
preferences_ = builderForValue.build();
onChanged();
} else {
preferencesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public Builder mergePreferences(io.bloombox.schema.identity.AppUser.ConsumerPreferences value) {
if (preferencesBuilder_ == null) {
if (preferences_ != null) {
preferences_ =
io.bloombox.schema.identity.AppUser.ConsumerPreferences.newBuilder(preferences_).mergeFrom(value).buildPartial();
} else {
preferences_ = value;
}
onChanged();
} else {
preferencesBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public Builder clearPreferences() {
if (preferencesBuilder_ == null) {
preferences_ = null;
onChanged();
} else {
preferences_ = null;
preferencesBuilder_ = null;
}
return this;
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder getPreferencesBuilder() {
onChanged();
return getPreferencesFieldBuilder().getBuilder();
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder getPreferencesOrBuilder() {
if (preferencesBuilder_ != null) {
return preferencesBuilder_.getMessageOrBuilder();
} else {
return preferences_ == null ?
io.bloombox.schema.identity.AppUser.ConsumerPreferences.getDefaultInstance() : preferences_;
}
}
/**
*
* Preferences attached to a consumer account.
*
*
* .bloombox.identity.ConsumerPreferences preferences = 5 [(.gen_bq_schema.description) = "Preferences attached to a consumer account."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerPreferences, io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder, io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder>
getPreferencesFieldBuilder() {
if (preferencesBuilder_ == null) {
preferencesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ConsumerPreferences, io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder, io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder>(
getPreferences(),
getParentForChildren(),
isClean());
preferences_ = null;
}
return preferencesBuilder_;
}
private int type_ = 0;
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public io.bloombox.schema.identity.AppUser.ConsumerType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.ConsumerType result = io.bloombox.schema.identity.AppUser.ConsumerType.valueOf(type_);
return result == null ? io.bloombox.schema.identity.AppUser.ConsumerType.UNRECOGNIZED : result;
}
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public Builder setType(io.bloombox.schema.identity.AppUser.ConsumerType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the primary consumer type for this account.
*
*
* .bloombox.identity.ConsumerType type = 6 [(.gen_bq_schema.description) = "Specifies the primary consumer type for this account."];
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private int referralSource_ = 0;
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public int getReferralSourceValue() {
return referralSource_;
}
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public Builder setReferralSourceValue(int value) {
referralSource_ = value;
onChanged();
return this;
}
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public io.bloombox.schema.identity.AppUser.ReferralSource getReferralSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.ReferralSource result = io.bloombox.schema.identity.AppUser.ReferralSource.valueOf(referralSource_);
return result == null ? io.bloombox.schema.identity.AppUser.ReferralSource.UNRECOGNIZED : result;
}
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public Builder setReferralSource(io.bloombox.schema.identity.AppUser.ReferralSource value) {
if (value == null) {
throw new NullPointerException();
}
referralSource_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies referral source attribution information.
*
*
* .bloombox.identity.ReferralSource referral_source = 7 [(.gen_bq_schema.description) = "Specifies referral source attribution information."];
*/
public Builder clearReferralSource() {
referralSource_ = 0;
onChanged();
return this;
}
private java.lang.Object referralDetail_ = "";
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public java.lang.String getReferralDetail() {
java.lang.Object ref = referralDetail_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralDetail_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public com.google.protobuf.ByteString
getReferralDetailBytes() {
java.lang.Object ref = referralDetail_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralDetail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public Builder setReferralDetail(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
referralDetail_ = value;
onChanged();
return this;
}
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public Builder clearReferralDetail() {
referralDetail_ = getDefaultInstance().getReferralDetail();
onChanged();
return this;
}
/**
*
* Specifies the referrer's name.
*
*
* string referral_detail = 8 [(.gen_bq_schema.description) = "Specifies the referrer\'s name."];
*/
public Builder setReferralDetailBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
referralDetail_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.ConsumerProfile)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.ConsumerProfile)
private static final io.bloombox.schema.identity.AppUser.ConsumerProfile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.ConsumerProfile();
}
public static io.bloombox.schema.identity.AppUser.ConsumerProfile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ConsumerProfile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConsumerProfile(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerProfile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MenuPreferencesOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.MenuPreferences)
com.google.protobuf.MessageOrBuilder {
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
java.util.List getSectionList();
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
int getSectionCount();
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
io.opencannabis.schema.menu.section.Section getSection(int index);
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
java.util.List
getSectionValueList();
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
int getSectionValue(int index);
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
java.util.List getFeelingList();
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
int getFeelingCount();
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
io.opencannabis.schema.product.struct.testing.Feeling getFeeling(int index);
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
java.util.List
getFeelingValueList();
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
int getFeelingValue(int index);
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
java.util.List getTasteNoteList();
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
int getTasteNoteCount();
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
io.opencannabis.schema.product.struct.testing.TasteNote getTasteNote(int index);
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
java.util.List
getTasteNoteValueList();
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
int getTasteNoteValue(int index);
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
int getDesiredPotencyValue();
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
io.opencannabis.schema.product.struct.testing.PotencyEstimate getDesiredPotency();
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
int getCannabinoidRatioValue();
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
io.opencannabis.schema.product.struct.testing.CannabinoidRatio getCannabinoidRatio();
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
java.util.List getSpeciesList();
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
int getSpeciesCount();
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
io.opencannabis.schema.product.struct.Species getSpecies(int index);
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
java.util.List
getSpeciesValueList();
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
int getSpeciesValue(int index);
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
java.util.List getGrowList();
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
int getGrowCount();
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
io.opencannabis.schema.product.struct.Grow getGrow(int index);
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
java.util.List
getGrowValueList();
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
int getGrowValue(int index);
}
/**
*
* Consumer preferences related to menus and products.
*
*
* Protobuf type {@code bloombox.identity.MenuPreferences}
*/
public static final class MenuPreferences extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.MenuPreferences)
MenuPreferencesOrBuilder {
private static final long serialVersionUID = 0L;
// Use MenuPreferences.newBuilder() to construct.
private MenuPreferences(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MenuPreferences() {
section_ = java.util.Collections.emptyList();
feeling_ = java.util.Collections.emptyList();
tasteNote_ = java.util.Collections.emptyList();
desiredPotency_ = 0;
cannabinoidRatio_ = 0;
species_ = java.util.Collections.emptyList();
grow_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MenuPreferences(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
section_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
section_.add(rawValue);
break;
}
case 10: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
section_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
section_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 16: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
feeling_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
feeling_.add(rawValue);
break;
}
case 18: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
feeling_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
feeling_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 24: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
tasteNote_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
tasteNote_.add(rawValue);
break;
}
case 26: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
tasteNote_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
tasteNote_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 32: {
int rawValue = input.readEnum();
desiredPotency_ = rawValue;
break;
}
case 40: {
int rawValue = input.readEnum();
cannabinoidRatio_ = rawValue;
break;
}
case 48: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
species_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
species_.add(rawValue);
break;
}
case 50: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
species_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
species_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 56: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
grow_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
grow_.add(rawValue);
break;
}
case 58: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
grow_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
grow_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
section_ = java.util.Collections.unmodifiableList(section_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
feeling_ = java.util.Collections.unmodifiableList(feeling_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
tasteNote_ = java.util.Collections.unmodifiableList(tasteNote_);
}
if (((mutable_bitField0_ & 0x00000020) != 0)) {
species_ = java.util.Collections.unmodifiableList(species_);
}
if (((mutable_bitField0_ & 0x00000040) != 0)) {
grow_ = java.util.Collections.unmodifiableList(grow_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_MenuPreferences_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_MenuPreferences_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.MenuPreferences.class, io.bloombox.schema.identity.AppUser.MenuPreferences.Builder.class);
}
private int bitField0_;
public static final int SECTION_FIELD_NUMBER = 1;
private java.util.List section_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.menu.section.Section> section_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.menu.section.Section>() {
public io.opencannabis.schema.menu.section.Section convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.menu.section.Section result = io.opencannabis.schema.menu.section.Section.valueOf(from);
return result == null ? io.opencannabis.schema.menu.section.Section.UNRECOGNIZED : result;
}
};
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public java.util.List getSectionList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.menu.section.Section>(section_, section_converter_);
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public int getSectionCount() {
return section_.size();
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public io.opencannabis.schema.menu.section.Section getSection(int index) {
return section_converter_.convert(section_.get(index));
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public java.util.List
getSectionValueList() {
return section_;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public int getSectionValue(int index) {
return section_.get(index);
}
private int sectionMemoizedSerializedSize;
public static final int FEELING_FIELD_NUMBER = 2;
private java.util.List feeling_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.Feeling> feeling_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.Feeling>() {
public io.opencannabis.schema.product.struct.testing.Feeling convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Feeling result = io.opencannabis.schema.product.struct.testing.Feeling.valueOf(from);
return result == null ? io.opencannabis.schema.product.struct.testing.Feeling.UNRECOGNIZED : result;
}
};
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public java.util.List getFeelingList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.Feeling>(feeling_, feeling_converter_);
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public int getFeelingCount() {
return feeling_.size();
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public io.opencannabis.schema.product.struct.testing.Feeling getFeeling(int index) {
return feeling_converter_.convert(feeling_.get(index));
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public java.util.List
getFeelingValueList() {
return feeling_;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public int getFeelingValue(int index) {
return feeling_.get(index);
}
private int feelingMemoizedSerializedSize;
public static final int TASTE_NOTE_FIELD_NUMBER = 3;
private java.util.List tasteNote_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.TasteNote> tasteNote_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.TasteNote>() {
public io.opencannabis.schema.product.struct.testing.TasteNote convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.TasteNote result = io.opencannabis.schema.product.struct.testing.TasteNote.valueOf(from);
return result == null ? io.opencannabis.schema.product.struct.testing.TasteNote.UNRECOGNIZED : result;
}
};
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public java.util.List getTasteNoteList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.TasteNote>(tasteNote_, tasteNote_converter_);
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public int getTasteNoteCount() {
return tasteNote_.size();
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public io.opencannabis.schema.product.struct.testing.TasteNote getTasteNote(int index) {
return tasteNote_converter_.convert(tasteNote_.get(index));
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public java.util.List
getTasteNoteValueList() {
return tasteNote_;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public int getTasteNoteValue(int index) {
return tasteNote_.get(index);
}
private int tasteNoteMemoizedSerializedSize;
public static final int DESIRED_POTENCY_FIELD_NUMBER = 4;
private int desiredPotency_;
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public int getDesiredPotencyValue() {
return desiredPotency_;
}
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public io.opencannabis.schema.product.struct.testing.PotencyEstimate getDesiredPotency() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.PotencyEstimate result = io.opencannabis.schema.product.struct.testing.PotencyEstimate.valueOf(desiredPotency_);
return result == null ? io.opencannabis.schema.product.struct.testing.PotencyEstimate.UNRECOGNIZED : result;
}
public static final int CANNABINOID_RATIO_FIELD_NUMBER = 5;
private int cannabinoidRatio_;
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public int getCannabinoidRatioValue() {
return cannabinoidRatio_;
}
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public io.opencannabis.schema.product.struct.testing.CannabinoidRatio getCannabinoidRatio() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.CannabinoidRatio result = io.opencannabis.schema.product.struct.testing.CannabinoidRatio.valueOf(cannabinoidRatio_);
return result == null ? io.opencannabis.schema.product.struct.testing.CannabinoidRatio.UNRECOGNIZED : result;
}
public static final int SPECIES_FIELD_NUMBER = 6;
private java.util.List species_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.Species> species_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.Species>() {
public io.opencannabis.schema.product.struct.Species convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.Species result = io.opencannabis.schema.product.struct.Species.valueOf(from);
return result == null ? io.opencannabis.schema.product.struct.Species.UNRECOGNIZED : result;
}
};
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public java.util.List getSpeciesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.Species>(species_, species_converter_);
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public int getSpeciesCount() {
return species_.size();
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public io.opencannabis.schema.product.struct.Species getSpecies(int index) {
return species_converter_.convert(species_.get(index));
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public java.util.List
getSpeciesValueList() {
return species_;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public int getSpeciesValue(int index) {
return species_.get(index);
}
private int speciesMemoizedSerializedSize;
public static final int GROW_FIELD_NUMBER = 7;
private java.util.List grow_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.Grow> grow_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.product.struct.Grow>() {
public io.opencannabis.schema.product.struct.Grow convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.Grow result = io.opencannabis.schema.product.struct.Grow.valueOf(from);
return result == null ? io.opencannabis.schema.product.struct.Grow.UNRECOGNIZED : result;
}
};
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public java.util.List getGrowList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.Grow>(grow_, grow_converter_);
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public int getGrowCount() {
return grow_.size();
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public io.opencannabis.schema.product.struct.Grow getGrow(int index) {
return grow_converter_.convert(grow_.get(index));
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public java.util.List
getGrowValueList() {
return grow_;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public int getGrowValue(int index) {
return grow_.get(index);
}
private int growMemoizedSerializedSize;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getSectionList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(sectionMemoizedSerializedSize);
}
for (int i = 0; i < section_.size(); i++) {
output.writeEnumNoTag(section_.get(i));
}
if (getFeelingList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(feelingMemoizedSerializedSize);
}
for (int i = 0; i < feeling_.size(); i++) {
output.writeEnumNoTag(feeling_.get(i));
}
if (getTasteNoteList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(tasteNoteMemoizedSerializedSize);
}
for (int i = 0; i < tasteNote_.size(); i++) {
output.writeEnumNoTag(tasteNote_.get(i));
}
if (desiredPotency_ != io.opencannabis.schema.product.struct.testing.PotencyEstimate.LIGHT.getNumber()) {
output.writeEnum(4, desiredPotency_);
}
if (cannabinoidRatio_ != io.opencannabis.schema.product.struct.testing.CannabinoidRatio.NO_CANNABINOID_PREFERENCE.getNumber()) {
output.writeEnum(5, cannabinoidRatio_);
}
if (getSpeciesList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(speciesMemoizedSerializedSize);
}
for (int i = 0; i < species_.size(); i++) {
output.writeEnumNoTag(species_.get(i));
}
if (getGrowList().size() > 0) {
output.writeUInt32NoTag(58);
output.writeUInt32NoTag(growMemoizedSerializedSize);
}
for (int i = 0; i < grow_.size(); i++) {
output.writeEnumNoTag(grow_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < section_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(section_.get(i));
}
size += dataSize;
if (!getSectionList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}sectionMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < feeling_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(feeling_.get(i));
}
size += dataSize;
if (!getFeelingList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}feelingMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < tasteNote_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(tasteNote_.get(i));
}
size += dataSize;
if (!getTasteNoteList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}tasteNoteMemoizedSerializedSize = dataSize;
}
if (desiredPotency_ != io.opencannabis.schema.product.struct.testing.PotencyEstimate.LIGHT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, desiredPotency_);
}
if (cannabinoidRatio_ != io.opencannabis.schema.product.struct.testing.CannabinoidRatio.NO_CANNABINOID_PREFERENCE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, cannabinoidRatio_);
}
{
int dataSize = 0;
for (int i = 0; i < species_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(species_.get(i));
}
size += dataSize;
if (!getSpeciesList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}speciesMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < grow_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(grow_.get(i));
}
size += dataSize;
if (!getGrowList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}growMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.MenuPreferences)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.MenuPreferences other = (io.bloombox.schema.identity.AppUser.MenuPreferences) obj;
if (!section_.equals(other.section_)) return false;
if (!feeling_.equals(other.feeling_)) return false;
if (!tasteNote_.equals(other.tasteNote_)) return false;
if (desiredPotency_ != other.desiredPotency_) return false;
if (cannabinoidRatio_ != other.cannabinoidRatio_) return false;
if (!species_.equals(other.species_)) return false;
if (!grow_.equals(other.grow_)) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSectionCount() > 0) {
hash = (37 * hash) + SECTION_FIELD_NUMBER;
hash = (53 * hash) + section_.hashCode();
}
if (getFeelingCount() > 0) {
hash = (37 * hash) + FEELING_FIELD_NUMBER;
hash = (53 * hash) + feeling_.hashCode();
}
if (getTasteNoteCount() > 0) {
hash = (37 * hash) + TASTE_NOTE_FIELD_NUMBER;
hash = (53 * hash) + tasteNote_.hashCode();
}
hash = (37 * hash) + DESIRED_POTENCY_FIELD_NUMBER;
hash = (53 * hash) + desiredPotency_;
hash = (37 * hash) + CANNABINOID_RATIO_FIELD_NUMBER;
hash = (53 * hash) + cannabinoidRatio_;
if (getSpeciesCount() > 0) {
hash = (37 * hash) + SPECIES_FIELD_NUMBER;
hash = (53 * hash) + species_.hashCode();
}
if (getGrowCount() > 0) {
hash = (37 * hash) + GROW_FIELD_NUMBER;
hash = (53 * hash) + grow_.hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.MenuPreferences prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Consumer preferences related to menus and products.
*
*
* Protobuf type {@code bloombox.identity.MenuPreferences}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.MenuPreferences)
io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_MenuPreferences_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_MenuPreferences_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.MenuPreferences.class, io.bloombox.schema.identity.AppUser.MenuPreferences.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.MenuPreferences.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
section_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
feeling_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
tasteNote_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
desiredPotency_ = 0;
cannabinoidRatio_ = 0;
species_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
grow_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_MenuPreferences_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.MenuPreferences getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.MenuPreferences.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.MenuPreferences build() {
io.bloombox.schema.identity.AppUser.MenuPreferences result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.MenuPreferences buildPartial() {
io.bloombox.schema.identity.AppUser.MenuPreferences result = new io.bloombox.schema.identity.AppUser.MenuPreferences(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) != 0)) {
section_ = java.util.Collections.unmodifiableList(section_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.section_ = section_;
if (((bitField0_ & 0x00000002) != 0)) {
feeling_ = java.util.Collections.unmodifiableList(feeling_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.feeling_ = feeling_;
if (((bitField0_ & 0x00000004) != 0)) {
tasteNote_ = java.util.Collections.unmodifiableList(tasteNote_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.tasteNote_ = tasteNote_;
result.desiredPotency_ = desiredPotency_;
result.cannabinoidRatio_ = cannabinoidRatio_;
if (((bitField0_ & 0x00000020) != 0)) {
species_ = java.util.Collections.unmodifiableList(species_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.species_ = species_;
if (((bitField0_ & 0x00000040) != 0)) {
grow_ = java.util.Collections.unmodifiableList(grow_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.grow_ = grow_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.MenuPreferences) {
return mergeFrom((io.bloombox.schema.identity.AppUser.MenuPreferences)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.MenuPreferences other) {
if (other == io.bloombox.schema.identity.AppUser.MenuPreferences.getDefaultInstance()) return this;
if (!other.section_.isEmpty()) {
if (section_.isEmpty()) {
section_ = other.section_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSectionIsMutable();
section_.addAll(other.section_);
}
onChanged();
}
if (!other.feeling_.isEmpty()) {
if (feeling_.isEmpty()) {
feeling_ = other.feeling_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureFeelingIsMutable();
feeling_.addAll(other.feeling_);
}
onChanged();
}
if (!other.tasteNote_.isEmpty()) {
if (tasteNote_.isEmpty()) {
tasteNote_ = other.tasteNote_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureTasteNoteIsMutable();
tasteNote_.addAll(other.tasteNote_);
}
onChanged();
}
if (other.desiredPotency_ != 0) {
setDesiredPotencyValue(other.getDesiredPotencyValue());
}
if (other.cannabinoidRatio_ != 0) {
setCannabinoidRatioValue(other.getCannabinoidRatioValue());
}
if (!other.species_.isEmpty()) {
if (species_.isEmpty()) {
species_ = other.species_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureSpeciesIsMutable();
species_.addAll(other.species_);
}
onChanged();
}
if (!other.grow_.isEmpty()) {
if (grow_.isEmpty()) {
grow_ = other.grow_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureGrowIsMutable();
grow_.addAll(other.grow_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.MenuPreferences parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.MenuPreferences) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List section_ =
java.util.Collections.emptyList();
private void ensureSectionIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
section_ = new java.util.ArrayList(section_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public java.util.List getSectionList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.menu.section.Section>(section_, section_converter_);
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public int getSectionCount() {
return section_.size();
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public io.opencannabis.schema.menu.section.Section getSection(int index) {
return section_converter_.convert(section_.get(index));
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder setSection(
int index, io.opencannabis.schema.menu.section.Section value) {
if (value == null) {
throw new NullPointerException();
}
ensureSectionIsMutable();
section_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder addSection(io.opencannabis.schema.menu.section.Section value) {
if (value == null) {
throw new NullPointerException();
}
ensureSectionIsMutable();
section_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder addAllSection(
java.lang.Iterable extends io.opencannabis.schema.menu.section.Section> values) {
ensureSectionIsMutable();
for (io.opencannabis.schema.menu.section.Section value : values) {
section_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder clearSection() {
section_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public java.util.List
getSectionValueList() {
return java.util.Collections.unmodifiableList(section_);
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public int getSectionValue(int index) {
return section_.get(index);
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder setSectionValue(
int index, int value) {
ensureSectionIsMutable();
section_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder addSectionValue(int value) {
ensureSectionIsMutable();
section_.add(value);
onChanged();
return this;
}
/**
*
* Preferred menu sections or product types.
*
*
* repeated .opencannabis.products.menu.section.Section section = 1 [(.gen_bq_schema.description) = "Preferred menu sections or product types."];
*/
public Builder addAllSectionValue(
java.lang.Iterable values) {
ensureSectionIsMutable();
for (int value : values) {
section_.add(value);
}
onChanged();
return this;
}
private java.util.List feeling_ =
java.util.Collections.emptyList();
private void ensureFeelingIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
feeling_ = new java.util.ArrayList(feeling_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public java.util.List getFeelingList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.Feeling>(feeling_, feeling_converter_);
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public int getFeelingCount() {
return feeling_.size();
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public io.opencannabis.schema.product.struct.testing.Feeling getFeeling(int index) {
return feeling_converter_.convert(feeling_.get(index));
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder setFeeling(
int index, io.opencannabis.schema.product.struct.testing.Feeling value) {
if (value == null) {
throw new NullPointerException();
}
ensureFeelingIsMutable();
feeling_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder addFeeling(io.opencannabis.schema.product.struct.testing.Feeling value) {
if (value == null) {
throw new NullPointerException();
}
ensureFeelingIsMutable();
feeling_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder addAllFeeling(
java.lang.Iterable extends io.opencannabis.schema.product.struct.testing.Feeling> values) {
ensureFeelingIsMutable();
for (io.opencannabis.schema.product.struct.testing.Feeling value : values) {
feeling_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder clearFeeling() {
feeling_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public java.util.List
getFeelingValueList() {
return java.util.Collections.unmodifiableList(feeling_);
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public int getFeelingValue(int index) {
return feeling_.get(index);
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder setFeelingValue(
int index, int value) {
ensureFeelingIsMutable();
feeling_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder addFeelingValue(int value) {
ensureFeelingIsMutable();
feeling_.add(value);
onChanged();
return this;
}
/**
*
* Preferred feelings or experiential states.
*
*
* repeated .opencannabis.structs.labtesting.Feeling feeling = 2 [(.gen_bq_schema.description) = "Preferred feelings or experiential states."];
*/
public Builder addAllFeelingValue(
java.lang.Iterable values) {
ensureFeelingIsMutable();
for (int value : values) {
feeling_.add(value);
}
onChanged();
return this;
}
private java.util.List tasteNote_ =
java.util.Collections.emptyList();
private void ensureTasteNoteIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
tasteNote_ = new java.util.ArrayList(tasteNote_);
bitField0_ |= 0x00000004;
}
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public java.util.List getTasteNoteList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.testing.TasteNote>(tasteNote_, tasteNote_converter_);
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public int getTasteNoteCount() {
return tasteNote_.size();
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public io.opencannabis.schema.product.struct.testing.TasteNote getTasteNote(int index) {
return tasteNote_converter_.convert(tasteNote_.get(index));
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder setTasteNote(
int index, io.opencannabis.schema.product.struct.testing.TasteNote value) {
if (value == null) {
throw new NullPointerException();
}
ensureTasteNoteIsMutable();
tasteNote_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder addTasteNote(io.opencannabis.schema.product.struct.testing.TasteNote value) {
if (value == null) {
throw new NullPointerException();
}
ensureTasteNoteIsMutable();
tasteNote_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder addAllTasteNote(
java.lang.Iterable extends io.opencannabis.schema.product.struct.testing.TasteNote> values) {
ensureTasteNoteIsMutable();
for (io.opencannabis.schema.product.struct.testing.TasteNote value : values) {
tasteNote_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder clearTasteNote() {
tasteNote_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public java.util.List
getTasteNoteValueList() {
return java.util.Collections.unmodifiableList(tasteNote_);
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public int getTasteNoteValue(int index) {
return tasteNote_.get(index);
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder setTasteNoteValue(
int index, int value) {
ensureTasteNoteIsMutable();
tasteNote_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder addTasteNoteValue(int value) {
ensureTasteNoteIsMutable();
tasteNote_.add(value);
onChanged();
return this;
}
/**
*
* Preferred tasting notes.
*
*
* repeated .opencannabis.structs.labtesting.TasteNote taste_note = 3 [(.gen_bq_schema.description) = "Preferred tasting notes."];
*/
public Builder addAllTasteNoteValue(
java.lang.Iterable values) {
ensureTasteNoteIsMutable();
for (int value : values) {
tasteNote_.add(value);
}
onChanged();
return this;
}
private int desiredPotency_ = 0;
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public int getDesiredPotencyValue() {
return desiredPotency_;
}
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public Builder setDesiredPotencyValue(int value) {
desiredPotency_ = value;
onChanged();
return this;
}
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public io.opencannabis.schema.product.struct.testing.PotencyEstimate getDesiredPotency() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.PotencyEstimate result = io.opencannabis.schema.product.struct.testing.PotencyEstimate.valueOf(desiredPotency_);
return result == null ? io.opencannabis.schema.product.struct.testing.PotencyEstimate.UNRECOGNIZED : result;
}
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public Builder setDesiredPotency(io.opencannabis.schema.product.struct.testing.PotencyEstimate value) {
if (value == null) {
throw new NullPointerException();
}
desiredPotency_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Desired potency level.
*
*
* .opencannabis.structs.labtesting.PotencyEstimate desired_potency = 4 [(.gen_bq_schema.description) = "Desired potency level."];
*/
public Builder clearDesiredPotency() {
desiredPotency_ = 0;
onChanged();
return this;
}
private int cannabinoidRatio_ = 0;
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public int getCannabinoidRatioValue() {
return cannabinoidRatio_;
}
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public Builder setCannabinoidRatioValue(int value) {
cannabinoidRatio_ = value;
onChanged();
return this;
}
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public io.opencannabis.schema.product.struct.testing.CannabinoidRatio getCannabinoidRatio() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.CannabinoidRatio result = io.opencannabis.schema.product.struct.testing.CannabinoidRatio.valueOf(cannabinoidRatio_);
return result == null ? io.opencannabis.schema.product.struct.testing.CannabinoidRatio.UNRECOGNIZED : result;
}
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public Builder setCannabinoidRatio(io.opencannabis.schema.product.struct.testing.CannabinoidRatio value) {
if (value == null) {
throw new NullPointerException();
}
cannabinoidRatio_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Desired cannabinoid ratio.
*
*
* .opencannabis.structs.labtesting.CannabinoidRatio cannabinoid_ratio = 5 [(.gen_bq_schema.description) = "Desired cannabinoid ratio."];
*/
public Builder clearCannabinoidRatio() {
cannabinoidRatio_ = 0;
onChanged();
return this;
}
private java.util.List species_ =
java.util.Collections.emptyList();
private void ensureSpeciesIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
species_ = new java.util.ArrayList(species_);
bitField0_ |= 0x00000020;
}
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public java.util.List getSpeciesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.Species>(species_, species_converter_);
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public int getSpeciesCount() {
return species_.size();
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public io.opencannabis.schema.product.struct.Species getSpecies(int index) {
return species_converter_.convert(species_.get(index));
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder setSpecies(
int index, io.opencannabis.schema.product.struct.Species value) {
if (value == null) {
throw new NullPointerException();
}
ensureSpeciesIsMutable();
species_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder addSpecies(io.opencannabis.schema.product.struct.Species value) {
if (value == null) {
throw new NullPointerException();
}
ensureSpeciesIsMutable();
species_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder addAllSpecies(
java.lang.Iterable extends io.opencannabis.schema.product.struct.Species> values) {
ensureSpeciesIsMutable();
for (io.opencannabis.schema.product.struct.Species value : values) {
species_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder clearSpecies() {
species_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public java.util.List
getSpeciesValueList() {
return java.util.Collections.unmodifiableList(species_);
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public int getSpeciesValue(int index) {
return species_.get(index);
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder setSpeciesValue(
int index, int value) {
ensureSpeciesIsMutable();
species_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder addSpeciesValue(int value) {
ensureSpeciesIsMutable();
species_.add(value);
onChanged();
return this;
}
/**
*
* Preferred species types.
*
*
* repeated .opencannabis.structs.Species species = 6 [(.gen_bq_schema.description) = "Preferred species types."];
*/
public Builder addAllSpeciesValue(
java.lang.Iterable values) {
ensureSpeciesIsMutable();
for (int value : values) {
species_.add(value);
}
onChanged();
return this;
}
private java.util.List grow_ =
java.util.Collections.emptyList();
private void ensureGrowIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
grow_ = new java.util.ArrayList(grow_);
bitField0_ |= 0x00000040;
}
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public java.util.List getGrowList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.product.struct.Grow>(grow_, grow_converter_);
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public int getGrowCount() {
return grow_.size();
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public io.opencannabis.schema.product.struct.Grow getGrow(int index) {
return grow_converter_.convert(grow_.get(index));
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder setGrow(
int index, io.opencannabis.schema.product.struct.Grow value) {
if (value == null) {
throw new NullPointerException();
}
ensureGrowIsMutable();
grow_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder addGrow(io.opencannabis.schema.product.struct.Grow value) {
if (value == null) {
throw new NullPointerException();
}
ensureGrowIsMutable();
grow_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder addAllGrow(
java.lang.Iterable extends io.opencannabis.schema.product.struct.Grow> values) {
ensureGrowIsMutable();
for (io.opencannabis.schema.product.struct.Grow value : values) {
grow_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder clearGrow() {
grow_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public java.util.List
getGrowValueList() {
return java.util.Collections.unmodifiableList(grow_);
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public int getGrowValue(int index) {
return grow_.get(index);
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder setGrowValue(
int index, int value) {
ensureGrowIsMutable();
grow_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder addGrowValue(int value) {
ensureGrowIsMutable();
grow_.add(value);
onChanged();
return this;
}
/**
*
* Preferred grow types.
*
*
* repeated .opencannabis.structs.Grow grow = 7 [(.gen_bq_schema.description) = "Preferred grow types."];
*/
public Builder addAllGrowValue(
java.lang.Iterable values) {
ensureGrowIsMutable();
for (int value : values) {
grow_.add(value);
}
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.MenuPreferences)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.MenuPreferences)
private static final io.bloombox.schema.identity.AppUser.MenuPreferences DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.MenuPreferences();
}
public static io.bloombox.schema.identity.AppUser.MenuPreferences getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MenuPreferences parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MenuPreferences(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.MenuPreferences getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProductTypePreferenceOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.ProductTypePreference)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
int getMajorValue();
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
io.opencannabis.schema.base.BaseProductKind.ProductKind getMajor();
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
int getApothecaryValue();
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary();
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
int getCartridgeValue();
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge();
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
int getEdibleValue();
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible();
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
int getExtractValue();
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract();
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
int getPlantValue();
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
io.opencannabis.schema.product.PlantProduct.PlantType getPlant();
public io.bloombox.schema.identity.AppUser.ProductTypePreference.MinorCase getMinorCase();
}
/**
*
* Specifies preferences for the consumer, related to the types of products they enjoy.
*
*
* Protobuf type {@code bloombox.identity.ProductTypePreference}
*/
public static final class ProductTypePreference extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.ProductTypePreference)
ProductTypePreferenceOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProductTypePreference.newBuilder() to construct.
private ProductTypePreference(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProductTypePreference() {
major_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProductTypePreference(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
major_ = rawValue;
break;
}
case 80: {
int rawValue = input.readEnum();
minorCase_ = 10;
minor_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
minorCase_ = 11;
minor_ = rawValue;
break;
}
case 96: {
int rawValue = input.readEnum();
minorCase_ = 12;
minor_ = rawValue;
break;
}
case 104: {
int rawValue = input.readEnum();
minorCase_ = 13;
minor_ = rawValue;
break;
}
case 112: {
int rawValue = input.readEnum();
minorCase_ = 14;
minor_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ProductTypePreference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ProductTypePreference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ProductTypePreference.class, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder.class);
}
private int minorCase_ = 0;
private java.lang.Object minor_;
public enum MinorCase
implements com.google.protobuf.Internal.EnumLite {
APOTHECARY(10),
CARTRIDGE(11),
EDIBLE(12),
EXTRACT(13),
PLANT(14),
MINOR_NOT_SET(0);
private final int value;
private MinorCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MinorCase valueOf(int value) {
return forNumber(value);
}
public static MinorCase forNumber(int value) {
switch (value) {
case 10: return APOTHECARY;
case 11: return CARTRIDGE;
case 12: return EDIBLE;
case 13: return EXTRACT;
case 14: return PLANT;
case 0: return MINOR_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public MinorCase
getMinorCase() {
return MinorCase.forNumber(
minorCase_);
}
public static final int MAJOR_FIELD_NUMBER = 1;
private int major_;
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public int getMajorValue() {
return major_;
}
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public io.opencannabis.schema.base.BaseProductKind.ProductKind getMajor() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.base.BaseProductKind.ProductKind result = io.opencannabis.schema.base.BaseProductKind.ProductKind.valueOf(major_);
return result == null ? io.opencannabis.schema.base.BaseProductKind.ProductKind.UNRECOGNIZED : result;
}
public static final int APOTHECARY_FIELD_NUMBER = 10;
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public int getApothecaryValue() {
if (minorCase_ == 10) {
return (java.lang.Integer) minor_;
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary() {
if (minorCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType result = io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNSPECIFIED_APOTHECARY;
}
public static final int CARTRIDGE_FIELD_NUMBER = 11;
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public int getCartridgeValue() {
if (minorCase_ == 11) {
return (java.lang.Integer) minor_;
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge() {
if (minorCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.CartridgeProduct.CartridgeType result = io.opencannabis.schema.product.CartridgeProduct.CartridgeType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNSPECIFIED_CARTRIDGE;
}
public static final int EDIBLE_FIELD_NUMBER = 12;
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public int getEdibleValue() {
if (minorCase_ == 12) {
return (java.lang.Integer) minor_;
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible() {
if (minorCase_ == 12) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.EdibleProduct.EdibleType result = io.opencannabis.schema.product.EdibleProduct.EdibleType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.EdibleProduct.EdibleType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.EdibleProduct.EdibleType.UNSPECIFIED_EDIBLE;
}
public static final int EXTRACT_FIELD_NUMBER = 13;
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public int getExtractValue() {
if (minorCase_ == 13) {
return (java.lang.Integer) minor_;
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract() {
if (minorCase_ == 13) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ExtractProduct.ExtractType result = io.opencannabis.schema.product.ExtractProduct.ExtractType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.ExtractProduct.ExtractType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ExtractProduct.ExtractType.UNSPECIFIED_EXTRACT;
}
public static final int PLANT_FIELD_NUMBER = 14;
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public int getPlantValue() {
if (minorCase_ == 14) {
return (java.lang.Integer) minor_;
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public io.opencannabis.schema.product.PlantProduct.PlantType getPlant() {
if (minorCase_ == 14) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.PlantProduct.PlantType result = io.opencannabis.schema.product.PlantProduct.PlantType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.PlantProduct.PlantType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.PlantProduct.PlantType.UNSPECIFIED_PLANT;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (major_ != io.opencannabis.schema.base.BaseProductKind.ProductKind.FLOWERS.getNumber()) {
output.writeEnum(1, major_);
}
if (minorCase_ == 10) {
output.writeEnum(10, ((java.lang.Integer) minor_));
}
if (minorCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) minor_));
}
if (minorCase_ == 12) {
output.writeEnum(12, ((java.lang.Integer) minor_));
}
if (minorCase_ == 13) {
output.writeEnum(13, ((java.lang.Integer) minor_));
}
if (minorCase_ == 14) {
output.writeEnum(14, ((java.lang.Integer) minor_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (major_ != io.opencannabis.schema.base.BaseProductKind.ProductKind.FLOWERS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, major_);
}
if (minorCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, ((java.lang.Integer) minor_));
}
if (minorCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) minor_));
}
if (minorCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, ((java.lang.Integer) minor_));
}
if (minorCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, ((java.lang.Integer) minor_));
}
if (minorCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, ((java.lang.Integer) minor_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.ProductTypePreference)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.ProductTypePreference other = (io.bloombox.schema.identity.AppUser.ProductTypePreference) obj;
if (major_ != other.major_) return false;
if (!getMinorCase().equals(other.getMinorCase())) return false;
switch (minorCase_) {
case 10:
if (getApothecaryValue()
!= other.getApothecaryValue()) return false;
break;
case 11:
if (getCartridgeValue()
!= other.getCartridgeValue()) return false;
break;
case 12:
if (getEdibleValue()
!= other.getEdibleValue()) return false;
break;
case 13:
if (getExtractValue()
!= other.getExtractValue()) return false;
break;
case 14:
if (getPlantValue()
!= other.getPlantValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MAJOR_FIELD_NUMBER;
hash = (53 * hash) + major_;
switch (minorCase_) {
case 10:
hash = (37 * hash) + APOTHECARY_FIELD_NUMBER;
hash = (53 * hash) + getApothecaryValue();
break;
case 11:
hash = (37 * hash) + CARTRIDGE_FIELD_NUMBER;
hash = (53 * hash) + getCartridgeValue();
break;
case 12:
hash = (37 * hash) + EDIBLE_FIELD_NUMBER;
hash = (53 * hash) + getEdibleValue();
break;
case 13:
hash = (37 * hash) + EXTRACT_FIELD_NUMBER;
hash = (53 * hash) + getExtractValue();
break;
case 14:
hash = (37 * hash) + PLANT_FIELD_NUMBER;
hash = (53 * hash) + getPlantValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.ProductTypePreference prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies preferences for the consumer, related to the types of products they enjoy.
*
*
* Protobuf type {@code bloombox.identity.ProductTypePreference}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.ProductTypePreference)
io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ProductTypePreference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ProductTypePreference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ProductTypePreference.class, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.ProductTypePreference.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
major_ = 0;
minorCase_ = 0;
minor_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ProductTypePreference_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ProductTypePreference getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.ProductTypePreference.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ProductTypePreference build() {
io.bloombox.schema.identity.AppUser.ProductTypePreference result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ProductTypePreference buildPartial() {
io.bloombox.schema.identity.AppUser.ProductTypePreference result = new io.bloombox.schema.identity.AppUser.ProductTypePreference(this);
result.major_ = major_;
if (minorCase_ == 10) {
result.minor_ = minor_;
}
if (minorCase_ == 11) {
result.minor_ = minor_;
}
if (minorCase_ == 12) {
result.minor_ = minor_;
}
if (minorCase_ == 13) {
result.minor_ = minor_;
}
if (minorCase_ == 14) {
result.minor_ = minor_;
}
result.minorCase_ = minorCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.ProductTypePreference) {
return mergeFrom((io.bloombox.schema.identity.AppUser.ProductTypePreference)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.ProductTypePreference other) {
if (other == io.bloombox.schema.identity.AppUser.ProductTypePreference.getDefaultInstance()) return this;
if (other.major_ != 0) {
setMajorValue(other.getMajorValue());
}
switch (other.getMinorCase()) {
case APOTHECARY: {
setApothecaryValue(other.getApothecaryValue());
break;
}
case CARTRIDGE: {
setCartridgeValue(other.getCartridgeValue());
break;
}
case EDIBLE: {
setEdibleValue(other.getEdibleValue());
break;
}
case EXTRACT: {
setExtractValue(other.getExtractValue());
break;
}
case PLANT: {
setPlantValue(other.getPlantValue());
break;
}
case MINOR_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.ProductTypePreference parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.ProductTypePreference) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int minorCase_ = 0;
private java.lang.Object minor_;
public MinorCase
getMinorCase() {
return MinorCase.forNumber(
minorCase_);
}
public Builder clearMinor() {
minorCase_ = 0;
minor_ = null;
onChanged();
return this;
}
private int major_ = 0;
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public int getMajorValue() {
return major_;
}
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public Builder setMajorValue(int value) {
major_ = value;
onChanged();
return this;
}
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public io.opencannabis.schema.base.BaseProductKind.ProductKind getMajor() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.base.BaseProductKind.ProductKind result = io.opencannabis.schema.base.BaseProductKind.ProductKind.valueOf(major_);
return result == null ? io.opencannabis.schema.base.BaseProductKind.ProductKind.UNRECOGNIZED : result;
}
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public Builder setMajor(io.opencannabis.schema.base.BaseProductKind.ProductKind value) {
if (value == null) {
throw new NullPointerException();
}
major_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a major kind of cannabis product that a consumer might have a preference for.
*
*
* .opencannabis.base.ProductKind major = 1;
*/
public Builder clearMajor() {
major_ = 0;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public int getApothecaryValue() {
if (minorCase_ == 10) {
return ((java.lang.Integer) minor_).intValue();
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder setApothecaryValue(int value) {
minorCase_ = 10;
minor_ = value;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary() {
if (minorCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType result = io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNSPECIFIED_APOTHECARY;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder setApothecary(io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType value) {
if (value == null) {
throw new NullPointerException();
}
minorCase_ = 10;
minor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Apothecary'.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder clearApothecary() {
if (minorCase_ == 10) {
minorCase_ = 0;
minor_ = null;
onChanged();
}
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public int getCartridgeValue() {
if (minorCase_ == 11) {
return ((java.lang.Integer) minor_).intValue();
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder setCartridgeValue(int value) {
minorCase_ = 11;
minor_ = value;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge() {
if (minorCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.CartridgeProduct.CartridgeType result = io.opencannabis.schema.product.CartridgeProduct.CartridgeType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNSPECIFIED_CARTRIDGE;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder setCartridge(io.opencannabis.schema.product.CartridgeProduct.CartridgeType value) {
if (value == null) {
throw new NullPointerException();
}
minorCase_ = 11;
minor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Cartridge'.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder clearCartridge() {
if (minorCase_ == 11) {
minorCase_ = 0;
minor_ = null;
onChanged();
}
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public int getEdibleValue() {
if (minorCase_ == 12) {
return ((java.lang.Integer) minor_).intValue();
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder setEdibleValue(int value) {
minorCase_ = 12;
minor_ = value;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible() {
if (minorCase_ == 12) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.EdibleProduct.EdibleType result = io.opencannabis.schema.product.EdibleProduct.EdibleType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.EdibleProduct.EdibleType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.EdibleProduct.EdibleType.UNSPECIFIED_EDIBLE;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder setEdible(io.opencannabis.schema.product.EdibleProduct.EdibleType value) {
if (value == null) {
throw new NullPointerException();
}
minorCase_ = 12;
minor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Edible'.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder clearEdible() {
if (minorCase_ == 12) {
minorCase_ = 0;
minor_ = null;
onChanged();
}
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public int getExtractValue() {
if (minorCase_ == 13) {
return ((java.lang.Integer) minor_).intValue();
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder setExtractValue(int value) {
minorCase_ = 13;
minor_ = value;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract() {
if (minorCase_ == 13) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ExtractProduct.ExtractType result = io.opencannabis.schema.product.ExtractProduct.ExtractType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.ExtractProduct.ExtractType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ExtractProduct.ExtractType.UNSPECIFIED_EXTRACT;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder setExtract(io.opencannabis.schema.product.ExtractProduct.ExtractType value) {
if (value == null) {
throw new NullPointerException();
}
minorCase_ = 13;
minor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Extract'.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder clearExtract() {
if (minorCase_ == 13) {
minorCase_ = 0;
minor_ = null;
onChanged();
}
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public int getPlantValue() {
if (minorCase_ == 14) {
return ((java.lang.Integer) minor_).intValue();
}
return 0;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder setPlantValue(int value) {
minorCase_ = 14;
minor_ = value;
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public io.opencannabis.schema.product.PlantProduct.PlantType getPlant() {
if (minorCase_ == 14) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.PlantProduct.PlantType result = io.opencannabis.schema.product.PlantProduct.PlantType.valueOf(
(java.lang.Integer) minor_);
return result == null ? io.opencannabis.schema.product.PlantProduct.PlantType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.PlantProduct.PlantType.UNSPECIFIED_PLANT;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder setPlant(io.opencannabis.schema.product.PlantProduct.PlantType value) {
if (value == null) {
throw new NullPointerException();
}
minorCase_ = 14;
minor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Declares a specific sub-type for a major type of 'Plant'.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder clearPlant() {
if (minorCase_ == 14) {
minorCase_ = 0;
minor_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.ProductTypePreference)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.ProductTypePreference)
private static final io.bloombox.schema.identity.AppUser.ProductTypePreference DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.ProductTypePreference();
}
public static io.bloombox.schema.identity.AppUser.ProductTypePreference getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProductTypePreference parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProductTypePreference(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ProductTypePreference getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConsumerPreferencesOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.ConsumerPreferences)
com.google.protobuf.MessageOrBuilder {
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
boolean hasMenu();
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
io.bloombox.schema.identity.AppUser.MenuPreferences getMenu();
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder getMenuOrBuilder();
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
java.util.List getMethodList();
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
int getMethodCount();
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod getMethod(int index);
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
java.util.List
getMethodValueList();
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
int getMethodValue(int index);
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
java.util.List
getKindList();
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
io.bloombox.schema.identity.AppUser.ProductTypePreference getKind(int index);
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
int getKindCount();
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
java.util.List extends io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder>
getKindOrBuilderList();
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder getKindOrBuilder(
int index);
}
/**
*
* Specifies preferences related to a user's profile as a cannabis consumer.
*
*
* Protobuf type {@code bloombox.identity.ConsumerPreferences}
*/
public static final class ConsumerPreferences extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.ConsumerPreferences)
ConsumerPreferencesOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConsumerPreferences.newBuilder() to construct.
private ConsumerPreferences(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConsumerPreferences() {
method_ = java.util.Collections.emptyList();
kind_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConsumerPreferences(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 82: {
io.bloombox.schema.identity.AppUser.MenuPreferences.Builder subBuilder = null;
if (menu_ != null) {
subBuilder = menu_.toBuilder();
}
menu_ = input.readMessage(io.bloombox.schema.identity.AppUser.MenuPreferences.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(menu_);
menu_ = subBuilder.buildPartial();
}
break;
}
case 88: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
method_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
method_.add(rawValue);
break;
}
case 90: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
method_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
method_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 98: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
kind_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
kind_.add(
input.readMessage(io.bloombox.schema.identity.AppUser.ProductTypePreference.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
method_ = java.util.Collections.unmodifiableList(method_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
kind_ = java.util.Collections.unmodifiableList(kind_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerPreferences_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerPreferences_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerPreferences.class, io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder.class);
}
private int bitField0_;
public static final int MENU_FIELD_NUMBER = 10;
private io.bloombox.schema.identity.AppUser.MenuPreferences menu_;
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public boolean hasMenu() {
return menu_ != null;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public io.bloombox.schema.identity.AppUser.MenuPreferences getMenu() {
return menu_ == null ? io.bloombox.schema.identity.AppUser.MenuPreferences.getDefaultInstance() : menu_;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder getMenuOrBuilder() {
return getMenu();
}
public static final int METHOD_FIELD_NUMBER = 11;
private java.util.List method_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod> method_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod>() {
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod result = io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod.valueOf(from);
return result == null ? io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod.UNRECOGNIZED : result;
}
};
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public java.util.List getMethodList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod>(method_, method_converter_);
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public int getMethodCount() {
return method_.size();
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod getMethod(int index) {
return method_converter_.convert(method_.get(index));
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public java.util.List
getMethodValueList() {
return method_;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public int getMethodValue(int index) {
return method_.get(index);
}
private int methodMemoizedSerializedSize;
public static final int KIND_FIELD_NUMBER = 12;
private java.util.List kind_;
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public java.util.List getKindList() {
return kind_;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public java.util.List extends io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder>
getKindOrBuilderList() {
return kind_;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public int getKindCount() {
return kind_.size();
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreference getKind(int index) {
return kind_.get(index);
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder getKindOrBuilder(
int index) {
return kind_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (menu_ != null) {
output.writeMessage(10, getMenu());
}
if (getMethodList().size() > 0) {
output.writeUInt32NoTag(90);
output.writeUInt32NoTag(methodMemoizedSerializedSize);
}
for (int i = 0; i < method_.size(); i++) {
output.writeEnumNoTag(method_.get(i));
}
for (int i = 0; i < kind_.size(); i++) {
output.writeMessage(12, kind_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (menu_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getMenu());
}
{
int dataSize = 0;
for (int i = 0; i < method_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(method_.get(i));
}
size += dataSize;
if (!getMethodList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}methodMemoizedSerializedSize = dataSize;
}
for (int i = 0; i < kind_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, kind_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.ConsumerPreferences)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.ConsumerPreferences other = (io.bloombox.schema.identity.AppUser.ConsumerPreferences) obj;
if (hasMenu() != other.hasMenu()) return false;
if (hasMenu()) {
if (!getMenu()
.equals(other.getMenu())) return false;
}
if (!method_.equals(other.method_)) return false;
if (!getKindList()
.equals(other.getKindList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMenu()) {
hash = (37 * hash) + MENU_FIELD_NUMBER;
hash = (53 * hash) + getMenu().hashCode();
}
if (getMethodCount() > 0) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_.hashCode();
}
if (getKindCount() > 0) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKindList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.ConsumerPreferences prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies preferences related to a user's profile as a cannabis consumer.
*
*
* Protobuf type {@code bloombox.identity.ConsumerPreferences}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.ConsumerPreferences)
io.bloombox.schema.identity.AppUser.ConsumerPreferencesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerPreferences_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerPreferences_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerPreferences.class, io.bloombox.schema.identity.AppUser.ConsumerPreferences.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.ConsumerPreferences.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getKindFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (menuBuilder_ == null) {
menu_ = null;
} else {
menu_ = null;
menuBuilder_ = null;
}
method_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
if (kindBuilder_ == null) {
kind_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
kindBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerPreferences_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerPreferences getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.ConsumerPreferences.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerPreferences build() {
io.bloombox.schema.identity.AppUser.ConsumerPreferences result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerPreferences buildPartial() {
io.bloombox.schema.identity.AppUser.ConsumerPreferences result = new io.bloombox.schema.identity.AppUser.ConsumerPreferences(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (menuBuilder_ == null) {
result.menu_ = menu_;
} else {
result.menu_ = menuBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
method_ = java.util.Collections.unmodifiableList(method_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.method_ = method_;
if (kindBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
kind_ = java.util.Collections.unmodifiableList(kind_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.kind_ = kind_;
} else {
result.kind_ = kindBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.ConsumerPreferences) {
return mergeFrom((io.bloombox.schema.identity.AppUser.ConsumerPreferences)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.ConsumerPreferences other) {
if (other == io.bloombox.schema.identity.AppUser.ConsumerPreferences.getDefaultInstance()) return this;
if (other.hasMenu()) {
mergeMenu(other.getMenu());
}
if (!other.method_.isEmpty()) {
if (method_.isEmpty()) {
method_ = other.method_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureMethodIsMutable();
method_.addAll(other.method_);
}
onChanged();
}
if (kindBuilder_ == null) {
if (!other.kind_.isEmpty()) {
if (kind_.isEmpty()) {
kind_ = other.kind_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureKindIsMutable();
kind_.addAll(other.kind_);
}
onChanged();
}
} else {
if (!other.kind_.isEmpty()) {
if (kindBuilder_.isEmpty()) {
kindBuilder_.dispose();
kindBuilder_ = null;
kind_ = other.kind_;
bitField0_ = (bitField0_ & ~0x00000004);
kindBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getKindFieldBuilder() : null;
} else {
kindBuilder_.addAllMessages(other.kind_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.ConsumerPreferences parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.ConsumerPreferences) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private io.bloombox.schema.identity.AppUser.MenuPreferences menu_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.MenuPreferences, io.bloombox.schema.identity.AppUser.MenuPreferences.Builder, io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder> menuBuilder_;
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public boolean hasMenu() {
return menuBuilder_ != null || menu_ != null;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public io.bloombox.schema.identity.AppUser.MenuPreferences getMenu() {
if (menuBuilder_ == null) {
return menu_ == null ? io.bloombox.schema.identity.AppUser.MenuPreferences.getDefaultInstance() : menu_;
} else {
return menuBuilder_.getMessage();
}
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public Builder setMenu(io.bloombox.schema.identity.AppUser.MenuPreferences value) {
if (menuBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
menu_ = value;
onChanged();
} else {
menuBuilder_.setMessage(value);
}
return this;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public Builder setMenu(
io.bloombox.schema.identity.AppUser.MenuPreferences.Builder builderForValue) {
if (menuBuilder_ == null) {
menu_ = builderForValue.build();
onChanged();
} else {
menuBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public Builder mergeMenu(io.bloombox.schema.identity.AppUser.MenuPreferences value) {
if (menuBuilder_ == null) {
if (menu_ != null) {
menu_ =
io.bloombox.schema.identity.AppUser.MenuPreferences.newBuilder(menu_).mergeFrom(value).buildPartial();
} else {
menu_ = value;
}
onChanged();
} else {
menuBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public Builder clearMenu() {
if (menuBuilder_ == null) {
menu_ = null;
onChanged();
} else {
menu_ = null;
menuBuilder_ = null;
}
return this;
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public io.bloombox.schema.identity.AppUser.MenuPreferences.Builder getMenuBuilder() {
onChanged();
return getMenuFieldBuilder().getBuilder();
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
public io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder getMenuOrBuilder() {
if (menuBuilder_ != null) {
return menuBuilder_.getMessageOrBuilder();
} else {
return menu_ == null ?
io.bloombox.schema.identity.AppUser.MenuPreferences.getDefaultInstance() : menu_;
}
}
/**
*
* Preferred menu sections/product types, and so on.
*
*
* .bloombox.identity.MenuPreferences menu = 10 [(.gen_bq_schema.description) = "Preferred menu sections/product types, and so on."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.MenuPreferences, io.bloombox.schema.identity.AppUser.MenuPreferences.Builder, io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder>
getMenuFieldBuilder() {
if (menuBuilder_ == null) {
menuBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUser.MenuPreferences, io.bloombox.schema.identity.AppUser.MenuPreferences.Builder, io.bloombox.schema.identity.AppUser.MenuPreferencesOrBuilder>(
getMenu(),
getParentForChildren(),
isClean());
menu_ = null;
}
return menuBuilder_;
}
private java.util.List method_ =
java.util.Collections.emptyList();
private void ensureMethodIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
method_ = new java.util.ArrayList(method_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public java.util.List getMethodList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod>(method_, method_converter_);
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public int getMethodCount() {
return method_.size();
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod getMethod(int index) {
return method_converter_.convert(method_.get(index));
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder setMethod(
int index, io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod value) {
if (value == null) {
throw new NullPointerException();
}
ensureMethodIsMutable();
method_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder addMethod(io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod value) {
if (value == null) {
throw new NullPointerException();
}
ensureMethodIsMutable();
method_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder addAllMethod(
java.lang.Iterable extends io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod> values) {
ensureMethodIsMutable();
for (io.bloombox.schema.consumption.Biodelivery.BiodeliveryMethod value : values) {
method_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder clearMethod() {
method_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public java.util.List
getMethodValueList() {
return java.util.Collections.unmodifiableList(method_);
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public int getMethodValue(int index) {
return method_.get(index);
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder setMethodValue(
int index, int value) {
ensureMethodIsMutable();
method_.set(index, value);
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder addMethodValue(int value) {
ensureMethodIsMutable();
method_.add(value);
onChanged();
return this;
}
/**
*
* Preferred biodelivery method for this consumer.
*
*
* repeated .bloombox.consumption.BiodeliveryMethod method = 11;
*/
public Builder addAllMethodValue(
java.lang.Iterable values) {
ensureMethodIsMutable();
for (int value : values) {
method_.add(value);
}
onChanged();
return this;
}
private java.util.List kind_ =
java.util.Collections.emptyList();
private void ensureKindIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
kind_ = new java.util.ArrayList(kind_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ProductTypePreference, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder, io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder> kindBuilder_;
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public java.util.List getKindList() {
if (kindBuilder_ == null) {
return java.util.Collections.unmodifiableList(kind_);
} else {
return kindBuilder_.getMessageList();
}
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public int getKindCount() {
if (kindBuilder_ == null) {
return kind_.size();
} else {
return kindBuilder_.getCount();
}
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreference getKind(int index) {
if (kindBuilder_ == null) {
return kind_.get(index);
} else {
return kindBuilder_.getMessage(index);
}
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder setKind(
int index, io.bloombox.schema.identity.AppUser.ProductTypePreference value) {
if (kindBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKindIsMutable();
kind_.set(index, value);
onChanged();
} else {
kindBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder setKind(
int index, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder builderForValue) {
if (kindBuilder_ == null) {
ensureKindIsMutable();
kind_.set(index, builderForValue.build());
onChanged();
} else {
kindBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder addKind(io.bloombox.schema.identity.AppUser.ProductTypePreference value) {
if (kindBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKindIsMutable();
kind_.add(value);
onChanged();
} else {
kindBuilder_.addMessage(value);
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder addKind(
int index, io.bloombox.schema.identity.AppUser.ProductTypePreference value) {
if (kindBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKindIsMutable();
kind_.add(index, value);
onChanged();
} else {
kindBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder addKind(
io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder builderForValue) {
if (kindBuilder_ == null) {
ensureKindIsMutable();
kind_.add(builderForValue.build());
onChanged();
} else {
kindBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder addKind(
int index, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder builderForValue) {
if (kindBuilder_ == null) {
ensureKindIsMutable();
kind_.add(index, builderForValue.build());
onChanged();
} else {
kindBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder addAllKind(
java.lang.Iterable extends io.bloombox.schema.identity.AppUser.ProductTypePreference> values) {
if (kindBuilder_ == null) {
ensureKindIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, kind_);
onChanged();
} else {
kindBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder clearKind() {
if (kindBuilder_ == null) {
kind_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
kindBuilder_.clear();
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public Builder removeKind(int index) {
if (kindBuilder_ == null) {
ensureKindIsMutable();
kind_.remove(index);
onChanged();
} else {
kindBuilder_.remove(index);
}
return this;
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder getKindBuilder(
int index) {
return getKindFieldBuilder().getBuilder(index);
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder getKindOrBuilder(
int index) {
if (kindBuilder_ == null) {
return kind_.get(index); } else {
return kindBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public java.util.List extends io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder>
getKindOrBuilderList() {
if (kindBuilder_ != null) {
return kindBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(kind_);
}
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder addKindBuilder() {
return getKindFieldBuilder().addBuilder(
io.bloombox.schema.identity.AppUser.ProductTypePreference.getDefaultInstance());
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder addKindBuilder(
int index) {
return getKindFieldBuilder().addBuilder(
index, io.bloombox.schema.identity.AppUser.ProductTypePreference.getDefaultInstance());
}
/**
*
* Preferred cannabis product types for this consumer.
*
*
* repeated .bloombox.identity.ProductTypePreference kind = 12;
*/
public java.util.List
getKindBuilderList() {
return getKindFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ProductTypePreference, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder, io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder>
getKindFieldBuilder() {
if (kindBuilder_ == null) {
kindBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.AppUser.ProductTypePreference, io.bloombox.schema.identity.AppUser.ProductTypePreference.Builder, io.bloombox.schema.identity.AppUser.ProductTypePreferenceOrBuilder>(
kind_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
kind_ = null;
}
return kindBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.ConsumerPreferences)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.ConsumerPreferences)
private static final io.bloombox.schema.identity.AppUser.ConsumerPreferences DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.ConsumerPreferences();
}
public static io.bloombox.schema.identity.AppUser.ConsumerPreferences getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ConsumerPreferences parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConsumerPreferences(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerPreferences getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConsumerMembershipOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.ConsumerMembership)
com.google.protobuf.MessageOrBuilder {
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
int getReferralSourceValue();
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
io.bloombox.schema.identity.AppUser.EnrollmentSource getReferralSource();
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
java.lang.String getReferralChannel();
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
com.google.protobuf.ByteString
getReferralChannelBytes();
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
boolean hasSignedUpAt();
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSignedUpAt();
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignedUpAtOrBuilder();
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
boolean hasSeen();
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
java.lang.String getForeignId();
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
com.google.protobuf.ByteString
getForeignIdBytes();
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
boolean hasKey();
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
io.bloombox.schema.identity.AppMemberKey.MembershipKey getKey();
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder getKeyOrBuilder();
}
/**
*
* Represents a consumer's membership at a particular dispensary.
*
*
* Protobuf type {@code bloombox.identity.ConsumerMembership}
*/
public static final class ConsumerMembership extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.ConsumerMembership)
ConsumerMembershipOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConsumerMembership.newBuilder() to construct.
private ConsumerMembership(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConsumerMembership() {
referralSource_ = 0;
referralChannel_ = "";
foreignId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConsumerMembership(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
referralSource_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
referralChannel_ = s;
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (signedUpAt_ != null) {
subBuilder = signedUpAt_.toBuilder();
}
signedUpAt_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signedUpAt_);
signedUpAt_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
foreignId_ = s;
break;
}
case 50: {
io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.bloombox.schema.identity.AppMemberKey.MembershipKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerMembership_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerMembership_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerMembership.class, io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder.class);
}
public static final int REFERRAL_SOURCE_FIELD_NUMBER = 1;
private int referralSource_;
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public int getReferralSourceValue() {
return referralSource_;
}
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public io.bloombox.schema.identity.AppUser.EnrollmentSource getReferralSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.EnrollmentSource result = io.bloombox.schema.identity.AppUser.EnrollmentSource.valueOf(referralSource_);
return result == null ? io.bloombox.schema.identity.AppUser.EnrollmentSource.UNRECOGNIZED : result;
}
public static final int REFERRAL_CHANNEL_FIELD_NUMBER = 2;
private volatile java.lang.Object referralChannel_;
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public java.lang.String getReferralChannel() {
java.lang.Object ref = referralChannel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralChannel_ = s;
return s;
}
}
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public com.google.protobuf.ByteString
getReferralChannelBytes() {
java.lang.Object ref = referralChannel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIGNED_UP_AT_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalInstant.Instant signedUpAt_;
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public boolean hasSignedUpAt() {
return signedUpAt_ != null;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSignedUpAt() {
return signedUpAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signedUpAt_;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignedUpAtOrBuilder() {
return getSignedUpAt();
}
public static final int SEEN_FIELD_NUMBER = 4;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
public static final int FOREIGN_ID_FIELD_NUMBER = 5;
private volatile java.lang.Object foreignId_;
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public java.lang.String getForeignId() {
java.lang.Object ref = foreignId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
foreignId_ = s;
return s;
}
}
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public com.google.protobuf.ByteString
getForeignIdBytes() {
java.lang.Object ref = foreignId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
foreignId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_FIELD_NUMBER = 6;
private io.bloombox.schema.identity.AppMemberKey.MembershipKey key_;
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public io.bloombox.schema.identity.AppMemberKey.MembershipKey getKey() {
return key_ == null ? io.bloombox.schema.identity.AppMemberKey.MembershipKey.getDefaultInstance() : key_;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (referralSource_ != io.bloombox.schema.identity.AppUser.EnrollmentSource.UNSPECIFIED.getNumber()) {
output.writeEnum(1, referralSource_);
}
if (!getReferralChannelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, referralChannel_);
}
if (signedUpAt_ != null) {
output.writeMessage(3, getSignedUpAt());
}
if (seen_ != null) {
output.writeMessage(4, getSeen());
}
if (!getForeignIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, foreignId_);
}
if (key_ != null) {
output.writeMessage(6, getKey());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (referralSource_ != io.bloombox.schema.identity.AppUser.EnrollmentSource.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, referralSource_);
}
if (!getReferralChannelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, referralChannel_);
}
if (signedUpAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSignedUpAt());
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSeen());
}
if (!getForeignIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, foreignId_);
}
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getKey());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.ConsumerMembership)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.ConsumerMembership other = (io.bloombox.schema.identity.AppUser.ConsumerMembership) obj;
if (referralSource_ != other.referralSource_) return false;
if (!getReferralChannel()
.equals(other.getReferralChannel())) return false;
if (hasSignedUpAt() != other.hasSignedUpAt()) return false;
if (hasSignedUpAt()) {
if (!getSignedUpAt()
.equals(other.getSignedUpAt())) return false;
}
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (!getForeignId()
.equals(other.getForeignId())) return false;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REFERRAL_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + referralSource_;
hash = (37 * hash) + REFERRAL_CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getReferralChannel().hashCode();
if (hasSignedUpAt()) {
hash = (37 * hash) + SIGNED_UP_AT_FIELD_NUMBER;
hash = (53 * hash) + getSignedUpAt().hashCode();
}
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
hash = (37 * hash) + FOREIGN_ID_FIELD_NUMBER;
hash = (53 * hash) + getForeignId().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.ConsumerMembership prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents a consumer's membership at a particular dispensary.
*
*
* Protobuf type {@code bloombox.identity.ConsumerMembership}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.ConsumerMembership)
io.bloombox.schema.identity.AppUser.ConsumerMembershipOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerMembership_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerMembership_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.ConsumerMembership.class, io.bloombox.schema.identity.AppUser.ConsumerMembership.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.ConsumerMembership.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
referralSource_ = 0;
referralChannel_ = "";
if (signedUpAtBuilder_ == null) {
signedUpAt_ = null;
} else {
signedUpAt_ = null;
signedUpAtBuilder_ = null;
}
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
foreignId_ = "";
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_ConsumerMembership_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerMembership getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.ConsumerMembership.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerMembership build() {
io.bloombox.schema.identity.AppUser.ConsumerMembership result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerMembership buildPartial() {
io.bloombox.schema.identity.AppUser.ConsumerMembership result = new io.bloombox.schema.identity.AppUser.ConsumerMembership(this);
result.referralSource_ = referralSource_;
result.referralChannel_ = referralChannel_;
if (signedUpAtBuilder_ == null) {
result.signedUpAt_ = signedUpAt_;
} else {
result.signedUpAt_ = signedUpAtBuilder_.build();
}
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
result.foreignId_ = foreignId_;
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.ConsumerMembership) {
return mergeFrom((io.bloombox.schema.identity.AppUser.ConsumerMembership)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.ConsumerMembership other) {
if (other == io.bloombox.schema.identity.AppUser.ConsumerMembership.getDefaultInstance()) return this;
if (other.referralSource_ != 0) {
setReferralSourceValue(other.getReferralSourceValue());
}
if (!other.getReferralChannel().isEmpty()) {
referralChannel_ = other.referralChannel_;
onChanged();
}
if (other.hasSignedUpAt()) {
mergeSignedUpAt(other.getSignedUpAt());
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
if (!other.getForeignId().isEmpty()) {
foreignId_ = other.foreignId_;
onChanged();
}
if (other.hasKey()) {
mergeKey(other.getKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.ConsumerMembership parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.ConsumerMembership) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int referralSource_ = 0;
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public int getReferralSourceValue() {
return referralSource_;
}
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public Builder setReferralSourceValue(int value) {
referralSource_ = value;
onChanged();
return this;
}
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public io.bloombox.schema.identity.AppUser.EnrollmentSource getReferralSource() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.AppUser.EnrollmentSource result = io.bloombox.schema.identity.AppUser.EnrollmentSource.valueOf(referralSource_);
return result == null ? io.bloombox.schema.identity.AppUser.EnrollmentSource.UNRECOGNIZED : result;
}
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public Builder setReferralSource(io.bloombox.schema.identity.AppUser.EnrollmentSource value) {
if (value == null) {
throw new NullPointerException();
}
referralSource_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Referral source for this enrollment.
*
*
* .bloombox.identity.EnrollmentSource referral_source = 1 [(.gen_bq_schema.description) = "Referral source for this enrollment."];
*/
public Builder clearReferralSource() {
referralSource_ = 0;
onChanged();
return this;
}
private java.lang.Object referralChannel_ = "";
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public java.lang.String getReferralChannel() {
java.lang.Object ref = referralChannel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralChannel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public com.google.protobuf.ByteString
getReferralChannelBytes() {
java.lang.Object ref = referralChannel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public Builder setReferralChannel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
referralChannel_ = value;
onChanged();
return this;
}
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public Builder clearReferralChannel() {
referralChannel_ = getDefaultInstance().getReferralChannel();
onChanged();
return this;
}
/**
*
* Referral channel token - an artbirary, end-system provided value.
*
*
* string referral_channel = 2 [(.gen_bq_schema.description) = "Referral channel token - an artbirary, end-system provided value."];
*/
public Builder setReferralChannelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
referralChannel_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant signedUpAt_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> signedUpAtBuilder_;
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public boolean hasSignedUpAt() {
return signedUpAtBuilder_ != null || signedUpAt_ != null;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSignedUpAt() {
if (signedUpAtBuilder_ == null) {
return signedUpAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signedUpAt_;
} else {
return signedUpAtBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public Builder setSignedUpAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (signedUpAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signedUpAt_ = value;
onChanged();
} else {
signedUpAtBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public Builder setSignedUpAt(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (signedUpAtBuilder_ == null) {
signedUpAt_ = builderForValue.build();
onChanged();
} else {
signedUpAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public Builder mergeSignedUpAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (signedUpAtBuilder_ == null) {
if (signedUpAt_ != null) {
signedUpAt_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(signedUpAt_).mergeFrom(value).buildPartial();
} else {
signedUpAt_ = value;
}
onChanged();
} else {
signedUpAtBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public Builder clearSignedUpAt() {
if (signedUpAtBuilder_ == null) {
signedUpAt_ = null;
onChanged();
} else {
signedUpAt_ = null;
signedUpAtBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSignedUpAtBuilder() {
onChanged();
return getSignedUpAtFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSignedUpAtOrBuilder() {
if (signedUpAtBuilder_ != null) {
return signedUpAtBuilder_.getMessageOrBuilder();
} else {
return signedUpAt_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : signedUpAt_;
}
}
/**
*
* Timestamp for when this profile enrolled.
*
*
* .opencannabis.temporal.Instant signed_up_at = 3 [(.gen_bq_schema.description) = "Timestamp for when this profile enrolled."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSignedUpAtFieldBuilder() {
if (signedUpAtBuilder_ == null) {
signedUpAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSignedUpAt(),
getParentForChildren(),
isClean());
signedUpAt_ = null;
}
return signedUpAtBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Timestamp for when this profile was last seen.
*
*
* .opencannabis.temporal.Instant seen = 4 [(.gen_bq_schema.description) = "Timestamp for when this profile was last seen."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
private java.lang.Object foreignId_ = "";
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public java.lang.String getForeignId() {
java.lang.Object ref = foreignId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
foreignId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public com.google.protobuf.ByteString
getForeignIdBytes() {
java.lang.Object ref = foreignId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
foreignId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public Builder setForeignId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
foreignId_ = value;
onChanged();
return this;
}
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public Builder clearForeignId() {
foreignId_ = getDefaultInstance().getForeignId();
onChanged();
return this;
}
/**
*
* Foreign ID for this membership, in the partner-colocated membership system.
*
*
* string foreign_id = 5 [(.gen_bq_schema.description) = "Foreign ID for this membership, in the partner-colocated membership system."];
*/
public Builder setForeignIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
foreignId_ = value;
onChanged();
return this;
}
private io.bloombox.schema.identity.AppMemberKey.MembershipKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppMemberKey.MembershipKey, io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder, io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder> keyBuilder_;
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public io.bloombox.schema.identity.AppMemberKey.MembershipKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.bloombox.schema.identity.AppMemberKey.MembershipKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public Builder setKey(io.bloombox.schema.identity.AppMemberKey.MembershipKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public Builder setKey(
io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public Builder mergeKey(io.bloombox.schema.identity.AppMemberKey.MembershipKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.bloombox.schema.identity.AppMemberKey.MembershipKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
public io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.bloombox.schema.identity.AppMemberKey.MembershipKey.getDefaultInstance() : key_;
}
}
/**
*
* Key representing this user's membership at this location.
*
*
* .bloombox.identity.MembershipKey key = 6 [(.gen_bq_schema.description) = "Membership key representing this user\'s membership at this location."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppMemberKey.MembershipKey, io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder, io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppMemberKey.MembershipKey, io.bloombox.schema.identity.AppMemberKey.MembershipKey.Builder, io.bloombox.schema.identity.AppMemberKey.MembershipKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.ConsumerMembership)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.ConsumerMembership)
private static final io.bloombox.schema.identity.AppUser.ConsumerMembership DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.ConsumerMembership();
}
public static io.bloombox.schema.identity.AppUser.ConsumerMembership getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ConsumerMembership parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConsumerMembership(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.ConsumerMembership getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IndustryProfileOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.IndustryProfile)
com.google.protobuf.MessageOrBuilder {
/**
*
* Profile inactive/active status.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile inactive/active status."];
*/
boolean getActive();
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
int getPartnersCount();
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
boolean containsPartners(
java.lang.String key);
/**
* Use {@link #getPartnersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getPartners();
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
java.util.Map
getPartnersMap();
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrDefault(
java.lang.String key,
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy defaultValue);
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrThrow(
java.lang.String key);
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
boolean hasSettings();
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings getSettings();
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder getSettingsOrBuilder();
}
/**
*
* Profile for a cannabis industry professional of some sort.
*
*
* Protobuf type {@code bloombox.identity.IndustryProfile}
*/
public static final class IndustryProfile extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.IndustryProfile)
IndustryProfileOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndustryProfile.newBuilder() to construct.
private IndustryProfile(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndustryProfile() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IndustryProfile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
active_ = input.readBool();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
partners_ = com.google.protobuf.MapField.newMapField(
PartnersDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
partners__ = input.readMessage(
PartnersDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
partners_.getMutableMap().put(
partners__.getKey(), partners__.getValue());
break;
}
case 26: {
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder subBuilder = null;
if (settings_ != null) {
subBuilder = settings_.toBuilder();
}
settings_ = input.readMessage(io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(settings_);
settings_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetPartners();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.IndustryProfile.class, io.bloombox.schema.identity.AppUser.IndustryProfile.Builder.class);
}
private int bitField0_;
public static final int ACTIVE_FIELD_NUMBER = 1;
private boolean active_;
/**
*
* Profile inactive/active status.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile inactive/active status."];
*/
public boolean getActive() {
return active_;
}
public static final int PARTNERS_FIELD_NUMBER = 2;
private static final class PartnersDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_PartnersEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy> partners_;
private com.google.protobuf.MapField
internalGetPartners() {
if (partners_ == null) {
return com.google.protobuf.MapField.emptyMapField(
PartnersDefaultEntryHolder.defaultEntry);
}
return partners_;
}
public int getPartnersCount() {
return internalGetPartners().getMap().size();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public boolean containsPartners(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetPartners().getMap().containsKey(key);
}
/**
* Use {@link #getPartnersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getPartners() {
return getPartnersMap();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public java.util.Map getPartnersMap() {
return internalGetPartners().getMap();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrDefault(
java.lang.String key,
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPartners().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPartners().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SETTINGS_FIELD_NUMBER = 3;
private io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings settings_;
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public boolean hasSettings() {
return settings_ != null;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings getSettings() {
return settings_ == null ? io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.getDefaultInstance() : settings_;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder getSettingsOrBuilder() {
return getSettings();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (active_ != false) {
output.writeBool(1, active_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetPartners(),
PartnersDefaultEntryHolder.defaultEntry,
2);
if (settings_ != null) {
output.writeMessage(3, getSettings());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (active_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, active_);
}
for (java.util.Map.Entry entry
: internalGetPartners().getMap().entrySet()) {
com.google.protobuf.MapEntry
partners__ = PartnersDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, partners__);
}
if (settings_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSettings());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.AppUser.IndustryProfile)) {
return super.equals(obj);
}
io.bloombox.schema.identity.AppUser.IndustryProfile other = (io.bloombox.schema.identity.AppUser.IndustryProfile) obj;
if (getActive()
!= other.getActive()) return false;
if (!internalGetPartners().equals(
other.internalGetPartners())) return false;
if (hasSettings() != other.hasSettings()) return false;
if (hasSettings()) {
if (!getSettings()
.equals(other.getSettings())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getActive());
if (!internalGetPartners().getMap().isEmpty()) {
hash = (37 * hash) + PARTNERS_FIELD_NUMBER;
hash = (53 * hash) + internalGetPartners().hashCode();
}
if (hasSettings()) {
hash = (37 * hash) + SETTINGS_FIELD_NUMBER;
hash = (53 * hash) + getSettings().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.AppUser.IndustryProfile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Profile for a cannabis industry professional of some sort.
*
*
* Protobuf type {@code bloombox.identity.IndustryProfile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.IndustryProfile)
io.bloombox.schema.identity.AppUser.IndustryProfileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetPartners();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutablePartners();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.AppUser.IndustryProfile.class, io.bloombox.schema.identity.AppUser.IndustryProfile.Builder.class);
}
// Construct using io.bloombox.schema.identity.AppUser.IndustryProfile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
active_ = false;
internalGetMutablePartners().clear();
if (settingsBuilder_ == null) {
settings_ = null;
} else {
settings_ = null;
settingsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.AppUser.internal_static_bloombox_identity_IndustryProfile_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.IndustryProfile getDefaultInstanceForType() {
return io.bloombox.schema.identity.AppUser.IndustryProfile.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.IndustryProfile build() {
io.bloombox.schema.identity.AppUser.IndustryProfile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.IndustryProfile buildPartial() {
io.bloombox.schema.identity.AppUser.IndustryProfile result = new io.bloombox.schema.identity.AppUser.IndustryProfile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.active_ = active_;
result.partners_ = internalGetPartners();
result.partners_.makeImmutable();
if (settingsBuilder_ == null) {
result.settings_ = settings_;
} else {
result.settings_ = settingsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.AppUser.IndustryProfile) {
return mergeFrom((io.bloombox.schema.identity.AppUser.IndustryProfile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.AppUser.IndustryProfile other) {
if (other == io.bloombox.schema.identity.AppUser.IndustryProfile.getDefaultInstance()) return this;
if (other.getActive() != false) {
setActive(other.getActive());
}
internalGetMutablePartners().mergeFrom(
other.internalGetPartners());
if (other.hasSettings()) {
mergeSettings(other.getSettings());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.AppUser.IndustryProfile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.AppUser.IndustryProfile) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private boolean active_ ;
/**
*
* Profile inactive/active status.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile inactive/active status."];
*/
public boolean getActive() {
return active_;
}
/**
*
* Profile inactive/active status.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile inactive/active status."];
*/
public Builder setActive(boolean value) {
active_ = value;
onChanged();
return this;
}
/**
*
* Profile inactive/active status.
*
*
* bool active = 1 [(.gen_bq_schema.description) = "Profile inactive/active status."];
*/
public Builder clearActive() {
active_ = false;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy> partners_;
private com.google.protobuf.MapField
internalGetPartners() {
if (partners_ == null) {
return com.google.protobuf.MapField.emptyMapField(
PartnersDefaultEntryHolder.defaultEntry);
}
return partners_;
}
private com.google.protobuf.MapField
internalGetMutablePartners() {
onChanged();;
if (partners_ == null) {
partners_ = com.google.protobuf.MapField.newMapField(
PartnersDefaultEntryHolder.defaultEntry);
}
if (!partners_.isMutable()) {
partners_ = partners_.copy();
}
return partners_;
}
public int getPartnersCount() {
return internalGetPartners().getMap().size();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public boolean containsPartners(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetPartners().getMap().containsKey(key);
}
/**
* Use {@link #getPartnersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getPartners() {
return getPartnersMap();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public java.util.Map getPartnersMap() {
return internalGetPartners().getMap();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrDefault(
java.lang.String key,
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPartners().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy getPartnersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPartners().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearPartners() {
internalGetMutablePartners().getMutableMap()
.clear();
return this;
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public Builder removePartners(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutablePartners().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutablePartners() {
return internalGetMutablePartners().getMutableMap();
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public Builder putPartners(
java.lang.String key,
io.bloombox.schema.security.access.PartnerPermissions.AccessPolicy value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutablePartners().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Map of partner accesses levels to partner codes.
*
*
* map<string, .bloombox.security.access.AccessPolicy> partners = 2 [(.gen_bq_schema.description) = "Map of partner accesses levels to partner and location codes."];
*/
public Builder putAllPartners(
java.util.Map values) {
internalGetMutablePartners().getMutableMap()
.putAll(values);
return this;
}
private io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings settings_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder> settingsBuilder_;
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public boolean hasSettings() {
return settingsBuilder_ != null || settings_ != null;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings getSettings() {
if (settingsBuilder_ == null) {
return settings_ == null ? io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.getDefaultInstance() : settings_;
} else {
return settingsBuilder_.getMessage();
}
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public Builder setSettings(io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings value) {
if (settingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
settings_ = value;
onChanged();
} else {
settingsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public Builder setSettings(
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder builderForValue) {
if (settingsBuilder_ == null) {
settings_ = builderForValue.build();
onChanged();
} else {
settingsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public Builder mergeSettings(io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings value) {
if (settingsBuilder_ == null) {
if (settings_ != null) {
settings_ =
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.newBuilder(settings_).mergeFrom(value).buildPartial();
} else {
settings_ = value;
}
onChanged();
} else {
settingsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public Builder clearSettings() {
if (settingsBuilder_ == null) {
settings_ = null;
onChanged();
} else {
settings_ = null;
settingsBuilder_ = null;
}
return this;
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder getSettingsBuilder() {
onChanged();
return getSettingsFieldBuilder().getBuilder();
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
public io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder getSettingsOrBuilder() {
if (settingsBuilder_ != null) {
return settingsBuilder_.getMessageOrBuilder();
} else {
return settings_ == null ?
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.getDefaultInstance() : settings_;
}
}
/**
*
* Settings for the user's industry profile.
*
*
* .bloombox.identity.industry.StaffSettings settings = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder>
getSettingsFieldBuilder() {
if (settingsBuilder_ == null) {
settingsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettings.Builder, io.bloombox.schema.identity.industry.UserStaffSettings.StaffSettingsOrBuilder>(
getSettings(),
getParentForChildren(),
isClean());
settings_ = null;
}
return settingsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.IndustryProfile)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.IndustryProfile)
private static final io.bloombox.schema.identity.AppUser.IndustryProfile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.AppUser.IndustryProfile();
}
public static io.bloombox.schema.identity.AppUser.IndustryProfile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndustryProfile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IndustryProfile(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.AppUser.IndustryProfile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_User_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_User_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_User_IdentitiesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_User_IdentitiesEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_User_MediaEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_User_MediaEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_UserFlags_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_UserFlags_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_UserIdentity_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_UserIdentity_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_ConsumerProfile_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_ConsumerProfile_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_MenuPreferences_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_MenuPreferences_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_ProductTypePreference_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_ProductTypePreference_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_ConsumerPreferences_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_ConsumerPreferences_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_ConsumerMembership_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_ConsumerMembership_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_IndustryProfile_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_IndustryProfile_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_IndustryProfile_PartnersEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_IndustryProfile_PartnersEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\023identity/User.proto\022\021bloombox.identity" +
"\032\016bq_field.proto\032\024core/Datamodel.proto\032\026" +
"base/ProductKind.proto\032\025media/MediaItem." +
"proto\032\026temporal/Instant.proto\032\'analytics" +
"/consumption/Biodelivery.proto\032\023person/P" +
"erson.proto\032\021identity/ID.proto\032 identity" +
"/ids/UserDoctorRec.proto\032\034identity/Membe" +
"rshipKey.proto\032%identity/industry/StaffS" +
"ettings.proto\032\031products/Apothecary.proto" +
"\032\030products/Cartridge.proto\032\026products/Ext" +
"ract.proto\032\025products/Edible.proto\032\024produ" +
"cts/Plant.proto\032\033products/menu/Section.p" +
"roto\032\022structs/Grow.proto\032\025structs/Specie" +
"s.proto\032$structs/labtesting/TestResults." +
"proto\032(security/access/PartnerPermission" +
"s.proto\"\333\t\n\004User\022-\n\003uid\030\001 \001(\tB \302\265\003\002\010\002\212@\027" +
"Unique ID for the user.\022R\n\005flags\030\002 \001(\0132\034" +
".bloombox.identity.UserFlagsB%\302\265\003\002\010\004\212@\034B" +
"oolean flags for this user.\022Z\n\006person\030\003 " +
"\001(\0132\033.opencannabis.person.PersonB-\212@*Per" +
"son\'s information that backs this user.\022" +
"s\n\016identification\030\024 \003(\0132\025.bloombox.ident" +
"ity.IDBD\200@\001\212@(Government ID associated w" +
"ith this user.\322\265\003\022\010\001\032\016identification\022\210\001\n" +
"\ndoctor_rec\030\025 \003(\0132$.bloombox.identity.id" +
"s.UserDoctorRecBN\200@\001\212@3Doctor\'s recommen" +
"dations associated with this user.\322\265\003\021\010\001" +
"\032\rprescriptions\022m\n\004seen\030\036 \001(\0132\036.opencann" +
"abis.temporal.InstantB?\212@
© 2015 - 2025 Weber Informatics LLC | Privacy Policy