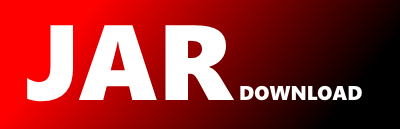
io.bloombox.schema.identity.bioprint.Aspects Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: identity/bioprint/Aspects.proto
package io.bloombox.schema.identity.bioprint;
public final class Aspects {
private Aspects() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
registry.add(io.bloombox.schema.identity.bioprint.Aspects.aspectGroup);
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Specifies groups of aspects, which are gathered into aspect categories for the purpose of data aggregation during
* Bioprint structure updates.
*
*
* Protobuf enum {@code bloombox.identity.bioprint.AspectCategory}
*/
public enum AspectCategory
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unknown, or unspecified, aspect-of-use category. Records associated with this value are rejected during processing.
*
*
* UNKNOWN_ASPECT_CATEGORY = 0;
*/
UNKNOWN_ASPECT_CATEGORY(0),
/**
*
* Includes product-categorical metrics, such as the product's main and secondary category, and materials data without
* including test results.
*
*
* CATEGORICAL = 1 [(.core.label) = "Categorical"];
*/
CATEGORICAL(1),
/**
*
* Includes data about the product's composition, to include, compound measurements (rounded and/or bounded), non-lab
* materials data (also included in the product-categorical aspect group), and ratios between compounds.
*
*
* COMPOSITIONAL = 2 [(.core.label) = "Compositional"];
*/
COMPOSITIONAL(2),
/**
*
* Includes situational information regarding the setting for purchase - i.e. the environment when the user buys the
* product. When, how, and where they buy would be considered purchase setting components.
*
*
* PURCHASE = 3 [(.core.label) = "Purchase"];
*/
PURCHASE(3),
/**
*
* Includes information regarding the setting for consumption - i.e. the environment around the consumer. When, how,
* and where they consume would be considered consumption setting components.
*
*
* CONSUMPTION = 4 [(.core.label) = "Consumption"];
*/
CONSUMPTION(4),
/**
*
* Includes information about the cost, financially and temporally, of consumption. Purchase tickets (i.e. the amount
* paid by the user in a transaction, with accompanying data), and consumption times (if available) are included here.
*
*
* COST = 5 [(.core.label) = "Cost"];
*/
COST(5),
UNRECOGNIZED(-1),
;
/**
*
* Unknown, or unspecified, aspect-of-use category. Records associated with this value are rejected during processing.
*
*
* UNKNOWN_ASPECT_CATEGORY = 0;
*/
public static final int UNKNOWN_ASPECT_CATEGORY_VALUE = 0;
/**
*
* Includes product-categorical metrics, such as the product's main and secondary category, and materials data without
* including test results.
*
*
* CATEGORICAL = 1 [(.core.label) = "Categorical"];
*/
public static final int CATEGORICAL_VALUE = 1;
/**
*
* Includes data about the product's composition, to include, compound measurements (rounded and/or bounded), non-lab
* materials data (also included in the product-categorical aspect group), and ratios between compounds.
*
*
* COMPOSITIONAL = 2 [(.core.label) = "Compositional"];
*/
public static final int COMPOSITIONAL_VALUE = 2;
/**
*
* Includes situational information regarding the setting for purchase - i.e. the environment when the user buys the
* product. When, how, and where they buy would be considered purchase setting components.
*
*
* PURCHASE = 3 [(.core.label) = "Purchase"];
*/
public static final int PURCHASE_VALUE = 3;
/**
*
* Includes information regarding the setting for consumption - i.e. the environment around the consumer. When, how,
* and where they consume would be considered consumption setting components.
*
*
* CONSUMPTION = 4 [(.core.label) = "Consumption"];
*/
public static final int CONSUMPTION_VALUE = 4;
/**
*
* Includes information about the cost, financially and temporally, of consumption. Purchase tickets (i.e. the amount
* paid by the user in a transaction, with accompanying data), and consumption times (if available) are included here.
*
*
* COST = 5 [(.core.label) = "Cost"];
*/
public static final int COST_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AspectCategory valueOf(int value) {
return forNumber(value);
}
public static AspectCategory forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_ASPECT_CATEGORY;
case 1: return CATEGORICAL;
case 2: return COMPOSITIONAL;
case 3: return PURCHASE;
case 4: return CONSUMPTION;
case 5: return COST;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AspectCategory> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AspectCategory findValueByNumber(int number) {
return AspectCategory.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.getDescriptor().getEnumTypes().get(0);
}
private static final AspectCategory[] VALUES = values();
public static AspectCategory valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AspectCategory(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.bioprint.AspectCategory)
}
/**
*
* Enumerates aspects of cannabis use. Each of these aspects is recorded against, as applicable, for each purchase or
* consumption feedback session logged by the consumer. Aspects of use are tied to "affinities," which describe the user
* response history for a given aspect-of-use over time, be it positive ("promotion") or negative ("rejection").
*
*
* Protobuf enum {@code bloombox.identity.bioprint.AspectOfUse}
*/
public enum AspectOfUse
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unknown, or unspecified, aspect-of-use. Records associated with this value are rejected during processing.
*
*
* UNKNOWN_ASPECT = 0;
*/
UNKNOWN_ASPECT(0),
/**
*
* Product major or minor category value (i.e. "FLOWERS", or "CHOCOLATE").
*
*
* PRODUCT_CATEGORY = 1 [(.core.label) = "Product Category", (.bloombox.identity.bioprint.aspect_group) = CATEGORICAL];
*/
PRODUCT_CATEGORY(1),
/**
*
* Product method-of-ingestion value (i.e. "SMOKED").
*
*
* METHOD_OF_CONSUMPTION = 2 [(.core.label) = "Method of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
METHOD_OF_CONSUMPTION(2),
/**
*
* Cannabinoids rounded to the nearest point value (i.e. "26.251%" becomes "26%").
*
*
* CANNABINOID_AMOUNT = 3 [(.core.label) = "Cannabinoid Amount", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
CANNABINOID_AMOUNT(3),
/**
*
* Cannabinoids bounded to the nearest 5% boundary (i.e. "0-5%, 5-10%" and so on).
*
*
* CANNABINOID_AMOUNT_BOUNDED = 4 [(.core.label) = "Cannabinoid Amount: Bounded", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
CANNABINOID_AMOUNT_BOUNDED(4),
/**
*
* Ratios between cannabinoids, in rounded form before comparison.
*
*
* CANNABINOID_RATIO = 5 [(.core.label) = "Cannabinoid Ratio", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
CANNABINOID_RATIO(5),
/**
*
* Ratios between cannabinoids, in bounded form (at a 5% boundary, see above).
*
*
* CANNABINOID_RATIO_BOUNDED = 6 [(.core.label) = "Cannabinoid Ratio: Bounded", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
CANNABINOID_RATIO_BOUNDED(6),
/**
*
* Bounded terpene ratio amounts, to the nearest 0.5% boundary (i.e. "0-0.5%, 0.5-1%" and so on).
*
*
* TERPENE_AMOUNT = 7 [(.core.label) = "Terpene Amount", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
TERPENE_AMOUNT(7),
/**
*
* Ratios between terpenes, in bounded form (see above for further info).
*
*
* TERPENE_RATIO = 8 [(.core.label) = "Terpene Ratio", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
TERPENE_RATIO(8),
/**
*
* Raw materials data for the subject product.
*
*
* MATERIAL_COMPOSITION = 9 [(.core.label) = "Material Composition", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
MATERIAL_COMPOSITION(9),
/**
*
* Product purchase times, bucketed into 30-minute increments.
*
*
* TIME_OF_PURCHASE = 10 [(.core.label) = "Time of Purchase", (.bloombox.identity.bioprint.aspect_group) = PURCHASE];
*/
TIME_OF_PURCHASE(10),
/**
*
* Product consumption times, bucketed into 30-minute increments.
*
*
* TIME_OF_CONSUMPTION = 11 [(.core.label) = "Time of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
TIME_OF_CONSUMPTION(11),
/**
*
* Physical location of purchase, by stacked jurisdiction.
*
*
* LOCATION_OF_PURCHASE = 12 [(.core.label) = "Location of Purchase", (.bloombox.identity.bioprint.aspect_group) = PURCHASE];
*/
LOCATION_OF_PURCHASE(12),
/**
*
* Physical location of consumption, by stacked jurisdiction.
*
*
* LOCATION_OF_CONSUMPTION = 13 [(.core.label) = "Location of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
LOCATION_OF_CONSUMPTION(13),
/**
*
* Duration of consumption, bucketed into 30-minute increments.
*
*
* DURATION_OF_CONSUMPTION = 14 [(.core.label) = "Duration of Consumption", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
DURATION_OF_CONSUMPTION(14),
/**
*
* Raw cost of the consumption session in question, broken down into $1-level buckets.
*
*
* COST_OF_CONSUMPTION = 15 [(.core.label) = "Cost of Consumption", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
COST_OF_CONSUMPTION(15),
/**
*
* Raw cost of the entire purchase that spawned consumption, broken down into $20-level buckets.
*
*
* COST_OF_PURCHASE = 16 [(.core.label) = "Cost of Purchase", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
COST_OF_PURCHASE(16),
UNRECOGNIZED(-1),
;
/**
*
* Unknown, or unspecified, aspect-of-use. Records associated with this value are rejected during processing.
*
*
* UNKNOWN_ASPECT = 0;
*/
public static final int UNKNOWN_ASPECT_VALUE = 0;
/**
*
* Product major or minor category value (i.e. "FLOWERS", or "CHOCOLATE").
*
*
* PRODUCT_CATEGORY = 1 [(.core.label) = "Product Category", (.bloombox.identity.bioprint.aspect_group) = CATEGORICAL];
*/
public static final int PRODUCT_CATEGORY_VALUE = 1;
/**
*
* Product method-of-ingestion value (i.e. "SMOKED").
*
*
* METHOD_OF_CONSUMPTION = 2 [(.core.label) = "Method of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
public static final int METHOD_OF_CONSUMPTION_VALUE = 2;
/**
*
* Cannabinoids rounded to the nearest point value (i.e. "26.251%" becomes "26%").
*
*
* CANNABINOID_AMOUNT = 3 [(.core.label) = "Cannabinoid Amount", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int CANNABINOID_AMOUNT_VALUE = 3;
/**
*
* Cannabinoids bounded to the nearest 5% boundary (i.e. "0-5%, 5-10%" and so on).
*
*
* CANNABINOID_AMOUNT_BOUNDED = 4 [(.core.label) = "Cannabinoid Amount: Bounded", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int CANNABINOID_AMOUNT_BOUNDED_VALUE = 4;
/**
*
* Ratios between cannabinoids, in rounded form before comparison.
*
*
* CANNABINOID_RATIO = 5 [(.core.label) = "Cannabinoid Ratio", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int CANNABINOID_RATIO_VALUE = 5;
/**
*
* Ratios between cannabinoids, in bounded form (at a 5% boundary, see above).
*
*
* CANNABINOID_RATIO_BOUNDED = 6 [(.core.label) = "Cannabinoid Ratio: Bounded", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int CANNABINOID_RATIO_BOUNDED_VALUE = 6;
/**
*
* Bounded terpene ratio amounts, to the nearest 0.5% boundary (i.e. "0-0.5%, 0.5-1%" and so on).
*
*
* TERPENE_AMOUNT = 7 [(.core.label) = "Terpene Amount", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int TERPENE_AMOUNT_VALUE = 7;
/**
*
* Ratios between terpenes, in bounded form (see above for further info).
*
*
* TERPENE_RATIO = 8 [(.core.label) = "Terpene Ratio", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int TERPENE_RATIO_VALUE = 8;
/**
*
* Raw materials data for the subject product.
*
*
* MATERIAL_COMPOSITION = 9 [(.core.label) = "Material Composition", (.bloombox.identity.bioprint.aspect_group) = COMPOSITIONAL];
*/
public static final int MATERIAL_COMPOSITION_VALUE = 9;
/**
*
* Product purchase times, bucketed into 30-minute increments.
*
*
* TIME_OF_PURCHASE = 10 [(.core.label) = "Time of Purchase", (.bloombox.identity.bioprint.aspect_group) = PURCHASE];
*/
public static final int TIME_OF_PURCHASE_VALUE = 10;
/**
*
* Product consumption times, bucketed into 30-minute increments.
*
*
* TIME_OF_CONSUMPTION = 11 [(.core.label) = "Time of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
public static final int TIME_OF_CONSUMPTION_VALUE = 11;
/**
*
* Physical location of purchase, by stacked jurisdiction.
*
*
* LOCATION_OF_PURCHASE = 12 [(.core.label) = "Location of Purchase", (.bloombox.identity.bioprint.aspect_group) = PURCHASE];
*/
public static final int LOCATION_OF_PURCHASE_VALUE = 12;
/**
*
* Physical location of consumption, by stacked jurisdiction.
*
*
* LOCATION_OF_CONSUMPTION = 13 [(.core.label) = "Location of Consumption", (.bloombox.identity.bioprint.aspect_group) = CONSUMPTION];
*/
public static final int LOCATION_OF_CONSUMPTION_VALUE = 13;
/**
*
* Duration of consumption, bucketed into 30-minute increments.
*
*
* DURATION_OF_CONSUMPTION = 14 [(.core.label) = "Duration of Consumption", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
public static final int DURATION_OF_CONSUMPTION_VALUE = 14;
/**
*
* Raw cost of the consumption session in question, broken down into $1-level buckets.
*
*
* COST_OF_CONSUMPTION = 15 [(.core.label) = "Cost of Consumption", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
public static final int COST_OF_CONSUMPTION_VALUE = 15;
/**
*
* Raw cost of the entire purchase that spawned consumption, broken down into $20-level buckets.
*
*
* COST_OF_PURCHASE = 16 [(.core.label) = "Cost of Purchase", (.bloombox.identity.bioprint.aspect_group) = COST];
*/
public static final int COST_OF_PURCHASE_VALUE = 16;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AspectOfUse valueOf(int value) {
return forNumber(value);
}
public static AspectOfUse forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_ASPECT;
case 1: return PRODUCT_CATEGORY;
case 2: return METHOD_OF_CONSUMPTION;
case 3: return CANNABINOID_AMOUNT;
case 4: return CANNABINOID_AMOUNT_BOUNDED;
case 5: return CANNABINOID_RATIO;
case 6: return CANNABINOID_RATIO_BOUNDED;
case 7: return TERPENE_AMOUNT;
case 8: return TERPENE_RATIO;
case 9: return MATERIAL_COMPOSITION;
case 10: return TIME_OF_PURCHASE;
case 11: return TIME_OF_CONSUMPTION;
case 12: return LOCATION_OF_PURCHASE;
case 13: return LOCATION_OF_CONSUMPTION;
case 14: return DURATION_OF_CONSUMPTION;
case 15: return COST_OF_CONSUMPTION;
case 16: return COST_OF_PURCHASE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AspectOfUse> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AspectOfUse findValueByNumber(int number) {
return AspectOfUse.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.getDescriptor().getEnumTypes().get(1);
}
private static final AspectOfUse[] VALUES = values();
public static AspectOfUse valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AspectOfUse(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.bioprint.AspectOfUse)
}
public interface AspectOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
int getAspectValue();
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse getAspect();
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
boolean hasProductCategory();
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getProductCategory();
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder getProductCategoryOrBuilder();
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
boolean hasConsumptionMethod();
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getConsumptionMethod();
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder getConsumptionMethodOrBuilder();
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
boolean hasCompound();
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getCompound();
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder getCompoundOrBuilder();
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
boolean hasCompoundRatio();
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getCompoundRatio();
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder getCompoundRatioOrBuilder();
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
boolean hasTiming();
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getTiming();
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder getTimingOrBuilder();
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
boolean hasLocation();
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getLocation();
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder getLocationOrBuilder();
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
boolean hasDuration();
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDuration();
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder getDurationOrBuilder();
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
boolean hasCost();
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getCost();
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder getCostOrBuilder();
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DetailCase getDetailCase();
}
/**
*
* Describes an aspect of cannabis use, by mapping an enumerated aspect to descriptive information concerning that use
* aspect. Aspect detail information can be concretely defined in a message for each aspect, each defined as a sub-
* message here and then mapped in via a protobuf "one-of" field.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect}
*/
public static final class Aspect extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect)
AspectOrBuilder {
private static final long serialVersionUID = 0L;
// Use Aspect.newBuilder() to construct.
private Aspect(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Aspect() {
aspect_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Aspect(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
aspect_ = rawValue;
break;
}
case 82: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder subBuilder = null;
if (detailCase_ == 10) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 10;
break;
}
case 90: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder subBuilder = null;
if (detailCase_ == 11) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 11;
break;
}
case 98: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder subBuilder = null;
if (detailCase_ == 12) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 12;
break;
}
case 106: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder subBuilder = null;
if (detailCase_ == 13) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 13;
break;
}
case 114: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder subBuilder = null;
if (detailCase_ == 14) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 14;
break;
}
case 122: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder subBuilder = null;
if (detailCase_ == 15) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 15;
break;
}
case 130: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder subBuilder = null;
if (detailCase_ == 16) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 16;
break;
}
case 138: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder subBuilder = null;
if (detailCase_ == 17) {
subBuilder = ((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_).toBuilder();
}
detail_ =
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_);
detail_ = subBuilder.buildPartial();
}
detailCase_ = 17;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder.class);
}
public interface ProductCategoryOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.ProductCategory)
com.google.protobuf.MessageOrBuilder {
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
int getKindValue();
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
io.opencannabis.schema.base.BaseProductKind.ProductKind getKind();
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
int getApothecaryValue();
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary();
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
int getCartridgeValue();
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge();
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
int getEdibleValue();
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible();
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
int getExtractValue();
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract();
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
int getPlantValue();
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
io.opencannabis.schema.product.PlantProduct.PlantType getPlant();
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.SubcategoryCase getSubcategoryCase();
}
/**
*
* Aspect description payload that carries detail information regarding a product's categorical identity. This would
* include the main product kind and any type-specific subcategory, as applicable.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.ProductCategory}
*/
public static final class ProductCategory extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.ProductCategory)
ProductCategoryOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProductCategory.newBuilder() to construct.
private ProductCategory(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProductCategory() {
kind_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProductCategory(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
kind_ = rawValue;
break;
}
case 80: {
int rawValue = input.readEnum();
subcategoryCase_ = 10;
subcategory_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
subcategoryCase_ = 11;
subcategory_ = rawValue;
break;
}
case 96: {
int rawValue = input.readEnum();
subcategoryCase_ = 12;
subcategory_ = rawValue;
break;
}
case 104: {
int rawValue = input.readEnum();
subcategoryCase_ = 13;
subcategory_ = rawValue;
break;
}
case 112: {
int rawValue = input.readEnum();
subcategoryCase_ = 14;
subcategory_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder.class);
}
private int subcategoryCase_ = 0;
private java.lang.Object subcategory_;
public enum SubcategoryCase
implements com.google.protobuf.Internal.EnumLite {
APOTHECARY(10),
CARTRIDGE(11),
EDIBLE(12),
EXTRACT(13),
PLANT(14),
SUBCATEGORY_NOT_SET(0);
private final int value;
private SubcategoryCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SubcategoryCase valueOf(int value) {
return forNumber(value);
}
public static SubcategoryCase forNumber(int value) {
switch (value) {
case 10: return APOTHECARY;
case 11: return CARTRIDGE;
case 12: return EDIBLE;
case 13: return EXTRACT;
case 14: return PLANT;
case 0: return SUBCATEGORY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public SubcategoryCase
getSubcategoryCase() {
return SubcategoryCase.forNumber(
subcategoryCase_);
}
public static final int KIND_FIELD_NUMBER = 1;
private int kind_;
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public int getKindValue() {
return kind_;
}
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public io.opencannabis.schema.base.BaseProductKind.ProductKind getKind() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.base.BaseProductKind.ProductKind result = io.opencannabis.schema.base.BaseProductKind.ProductKind.valueOf(kind_);
return result == null ? io.opencannabis.schema.base.BaseProductKind.ProductKind.UNRECOGNIZED : result;
}
public static final int APOTHECARY_FIELD_NUMBER = 10;
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public int getApothecaryValue() {
if (subcategoryCase_ == 10) {
return (java.lang.Integer) subcategory_;
}
return 0;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary() {
if (subcategoryCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType result = io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNSPECIFIED_APOTHECARY;
}
public static final int CARTRIDGE_FIELD_NUMBER = 11;
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public int getCartridgeValue() {
if (subcategoryCase_ == 11) {
return (java.lang.Integer) subcategory_;
}
return 0;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge() {
if (subcategoryCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.CartridgeProduct.CartridgeType result = io.opencannabis.schema.product.CartridgeProduct.CartridgeType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNSPECIFIED_CARTRIDGE;
}
public static final int EDIBLE_FIELD_NUMBER = 12;
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public int getEdibleValue() {
if (subcategoryCase_ == 12) {
return (java.lang.Integer) subcategory_;
}
return 0;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible() {
if (subcategoryCase_ == 12) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.EdibleProduct.EdibleType result = io.opencannabis.schema.product.EdibleProduct.EdibleType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.EdibleProduct.EdibleType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.EdibleProduct.EdibleType.UNSPECIFIED_EDIBLE;
}
public static final int EXTRACT_FIELD_NUMBER = 13;
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public int getExtractValue() {
if (subcategoryCase_ == 13) {
return (java.lang.Integer) subcategory_;
}
return 0;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract() {
if (subcategoryCase_ == 13) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ExtractProduct.ExtractType result = io.opencannabis.schema.product.ExtractProduct.ExtractType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.ExtractProduct.ExtractType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ExtractProduct.ExtractType.UNSPECIFIED_EXTRACT;
}
public static final int PLANT_FIELD_NUMBER = 14;
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public int getPlantValue() {
if (subcategoryCase_ == 14) {
return (java.lang.Integer) subcategory_;
}
return 0;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public io.opencannabis.schema.product.PlantProduct.PlantType getPlant() {
if (subcategoryCase_ == 14) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.PlantProduct.PlantType result = io.opencannabis.schema.product.PlantProduct.PlantType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.PlantProduct.PlantType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.PlantProduct.PlantType.UNSPECIFIED_PLANT;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (kind_ != io.opencannabis.schema.base.BaseProductKind.ProductKind.FLOWERS.getNumber()) {
output.writeEnum(1, kind_);
}
if (subcategoryCase_ == 10) {
output.writeEnum(10, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 12) {
output.writeEnum(12, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 13) {
output.writeEnum(13, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 14) {
output.writeEnum(14, ((java.lang.Integer) subcategory_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (kind_ != io.opencannabis.schema.base.BaseProductKind.ProductKind.FLOWERS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, kind_);
}
if (subcategoryCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, ((java.lang.Integer) subcategory_));
}
if (subcategoryCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, ((java.lang.Integer) subcategory_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) obj;
if (kind_ != other.kind_) return false;
if (!getSubcategoryCase().equals(other.getSubcategoryCase())) return false;
switch (subcategoryCase_) {
case 10:
if (getApothecaryValue()
!= other.getApothecaryValue()) return false;
break;
case 11:
if (getCartridgeValue()
!= other.getCartridgeValue()) return false;
break;
case 12:
if (getEdibleValue()
!= other.getEdibleValue()) return false;
break;
case 13:
if (getExtractValue()
!= other.getExtractValue()) return false;
break;
case 14:
if (getPlantValue()
!= other.getPlantValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + kind_;
switch (subcategoryCase_) {
case 10:
hash = (37 * hash) + APOTHECARY_FIELD_NUMBER;
hash = (53 * hash) + getApothecaryValue();
break;
case 11:
hash = (37 * hash) + CARTRIDGE_FIELD_NUMBER;
hash = (53 * hash) + getCartridgeValue();
break;
case 12:
hash = (37 * hash) + EDIBLE_FIELD_NUMBER;
hash = (53 * hash) + getEdibleValue();
break;
case 13:
hash = (37 * hash) + EXTRACT_FIELD_NUMBER;
hash = (53 * hash) + getExtractValue();
break;
case 14:
hash = (37 * hash) + PLANT_FIELD_NUMBER;
hash = (53 * hash) + getPlantValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Aspect description payload that carries detail information regarding a product's categorical identity. This would
* include the main product kind and any type-specific subcategory, as applicable.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.ProductCategory}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.ProductCategory)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
kind_ = 0;
subcategoryCase_ = 0;
subcategory_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory(this);
result.kind_ = kind_;
if (subcategoryCase_ == 10) {
result.subcategory_ = subcategory_;
}
if (subcategoryCase_ == 11) {
result.subcategory_ = subcategory_;
}
if (subcategoryCase_ == 12) {
result.subcategory_ = subcategory_;
}
if (subcategoryCase_ == 13) {
result.subcategory_ = subcategory_;
}
if (subcategoryCase_ == 14) {
result.subcategory_ = subcategory_;
}
result.subcategoryCase_ = subcategoryCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance()) return this;
if (other.kind_ != 0) {
setKindValue(other.getKindValue());
}
switch (other.getSubcategoryCase()) {
case APOTHECARY: {
setApothecaryValue(other.getApothecaryValue());
break;
}
case CARTRIDGE: {
setCartridgeValue(other.getCartridgeValue());
break;
}
case EDIBLE: {
setEdibleValue(other.getEdibleValue());
break;
}
case EXTRACT: {
setExtractValue(other.getExtractValue());
break;
}
case PLANT: {
setPlantValue(other.getPlantValue());
break;
}
case SUBCATEGORY_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int subcategoryCase_ = 0;
private java.lang.Object subcategory_;
public SubcategoryCase
getSubcategoryCase() {
return SubcategoryCase.forNumber(
subcategoryCase_);
}
public Builder clearSubcategory() {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
return this;
}
private int kind_ = 0;
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public int getKindValue() {
return kind_;
}
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public Builder setKindValue(int value) {
kind_ = value;
onChanged();
return this;
}
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public io.opencannabis.schema.base.BaseProductKind.ProductKind getKind() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.base.BaseProductKind.ProductKind result = io.opencannabis.schema.base.BaseProductKind.ProductKind.valueOf(kind_);
return result == null ? io.opencannabis.schema.base.BaseProductKind.ProductKind.UNRECOGNIZED : result;
}
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public Builder setKind(io.opencannabis.schema.base.BaseProductKind.ProductKind value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Main product category value.
*
*
* .opencannabis.base.ProductKind kind = 1;
*/
public Builder clearKind() {
kind_ = 0;
onChanged();
return this;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public int getApothecaryValue() {
if (subcategoryCase_ == 10) {
return ((java.lang.Integer) subcategory_).intValue();
}
return 0;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder setApothecaryValue(int value) {
subcategoryCase_ = 10;
subcategory_ = value;
onChanged();
return this;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType getApothecary() {
if (subcategoryCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType result = io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType.UNSPECIFIED_APOTHECARY;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder setApothecary(io.opencannabis.schema.product.ApothecaryProduct.ApothecaryType value) {
if (value == null) {
throw new NullPointerException();
}
subcategoryCase_ = 10;
subcategory_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Subcategory value for an apothecary product.
*
*
* .opencannabis.products.ApothecaryType apothecary = 10;
*/
public Builder clearApothecary() {
if (subcategoryCase_ == 10) {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
}
return this;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public int getCartridgeValue() {
if (subcategoryCase_ == 11) {
return ((java.lang.Integer) subcategory_).intValue();
}
return 0;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder setCartridgeValue(int value) {
subcategoryCase_ = 11;
subcategory_ = value;
onChanged();
return this;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public io.opencannabis.schema.product.CartridgeProduct.CartridgeType getCartridge() {
if (subcategoryCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.CartridgeProduct.CartridgeType result = io.opencannabis.schema.product.CartridgeProduct.CartridgeType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.CartridgeProduct.CartridgeType.UNSPECIFIED_CARTRIDGE;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder setCartridge(io.opencannabis.schema.product.CartridgeProduct.CartridgeType value) {
if (value == null) {
throw new NullPointerException();
}
subcategoryCase_ = 11;
subcategory_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Subcategory value for a cartridge product.
*
*
* .opencannabis.products.CartridgeType cartridge = 11;
*/
public Builder clearCartridge() {
if (subcategoryCase_ == 11) {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
}
return this;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public int getEdibleValue() {
if (subcategoryCase_ == 12) {
return ((java.lang.Integer) subcategory_).intValue();
}
return 0;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder setEdibleValue(int value) {
subcategoryCase_ = 12;
subcategory_ = value;
onChanged();
return this;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public io.opencannabis.schema.product.EdibleProduct.EdibleType getEdible() {
if (subcategoryCase_ == 12) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.EdibleProduct.EdibleType result = io.opencannabis.schema.product.EdibleProduct.EdibleType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.EdibleProduct.EdibleType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.EdibleProduct.EdibleType.UNSPECIFIED_EDIBLE;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder setEdible(io.opencannabis.schema.product.EdibleProduct.EdibleType value) {
if (value == null) {
throw new NullPointerException();
}
subcategoryCase_ = 12;
subcategory_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Subcategory value for an edible product.
*
*
* .opencannabis.products.EdibleType edible = 12;
*/
public Builder clearEdible() {
if (subcategoryCase_ == 12) {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
}
return this;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public int getExtractValue() {
if (subcategoryCase_ == 13) {
return ((java.lang.Integer) subcategory_).intValue();
}
return 0;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder setExtractValue(int value) {
subcategoryCase_ = 13;
subcategory_ = value;
onChanged();
return this;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public io.opencannabis.schema.product.ExtractProduct.ExtractType getExtract() {
if (subcategoryCase_ == 13) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.ExtractProduct.ExtractType result = io.opencannabis.schema.product.ExtractProduct.ExtractType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.ExtractProduct.ExtractType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.ExtractProduct.ExtractType.UNSPECIFIED_EXTRACT;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder setExtract(io.opencannabis.schema.product.ExtractProduct.ExtractType value) {
if (value == null) {
throw new NullPointerException();
}
subcategoryCase_ = 13;
subcategory_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Subcategory value for an extracted cannabis product.
*
*
* .opencannabis.products.ExtractType extract = 13;
*/
public Builder clearExtract() {
if (subcategoryCase_ == 13) {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
}
return this;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public int getPlantValue() {
if (subcategoryCase_ == 14) {
return ((java.lang.Integer) subcategory_).intValue();
}
return 0;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder setPlantValue(int value) {
subcategoryCase_ = 14;
subcategory_ = value;
onChanged();
return this;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public io.opencannabis.schema.product.PlantProduct.PlantType getPlant() {
if (subcategoryCase_ == 14) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.PlantProduct.PlantType result = io.opencannabis.schema.product.PlantProduct.PlantType.valueOf(
(java.lang.Integer) subcategory_);
return result == null ? io.opencannabis.schema.product.PlantProduct.PlantType.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.PlantProduct.PlantType.UNSPECIFIED_PLANT;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder setPlant(io.opencannabis.schema.product.PlantProduct.PlantType value) {
if (value == null) {
throw new NullPointerException();
}
subcategoryCase_ = 14;
subcategory_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Subcategory value for a cannabis plant or seed.
*
*
* .opencannabis.products.PlantType plant = 14;
*/
public Builder clearPlant() {
if (subcategoryCase_ == 14) {
subcategoryCase_ = 0;
subcategory_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.ProductCategory)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.ProductCategory)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProductCategory parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProductCategory(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MethodOfConsumptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.MethodOfConsumption)
com.google.protobuf.MessageOrBuilder {
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
boolean hasBioDelivery();
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo getBioDelivery();
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder getBioDeliveryOrBuilder();
}
/**
*
* Indicates *how* cannabis is consumed, i.e., the method of cannabis consumption employed by the consumer to make use
* of cannabis. This indicates whether it was smoked, eaten, somehow taken otherwise.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.MethodOfConsumption}
*/
public static final class MethodOfConsumption extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.MethodOfConsumption)
MethodOfConsumptionOrBuilder {
private static final long serialVersionUID = 0L;
// Use MethodOfConsumption.newBuilder() to construct.
private MethodOfConsumption(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MethodOfConsumption() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MethodOfConsumption(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder subBuilder = null;
if (bioDelivery_ != null) {
subBuilder = bioDelivery_.toBuilder();
}
bioDelivery_ = input.readMessage(io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(bioDelivery_);
bioDelivery_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder.class);
}
public static final int BIO_DELIVERY_FIELD_NUMBER = 1;
private io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo bioDelivery_;
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public boolean hasBioDelivery() {
return bioDelivery_ != null;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo getBioDelivery() {
return bioDelivery_ == null ? io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.getDefaultInstance() : bioDelivery_;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder getBioDeliveryOrBuilder() {
return getBioDelivery();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (bioDelivery_ != null) {
output.writeMessage(1, getBioDelivery());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (bioDelivery_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getBioDelivery());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) obj;
if (hasBioDelivery() != other.hasBioDelivery()) return false;
if (hasBioDelivery()) {
if (!getBioDelivery()
.equals(other.getBioDelivery())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasBioDelivery()) {
hash = (37 * hash) + BIO_DELIVERY_FIELD_NUMBER;
hash = (53 * hash) + getBioDelivery().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates *how* cannabis is consumed, i.e., the method of cannabis consumption employed by the consumer to make use
* of cannabis. This indicates whether it was smoked, eaten, somehow taken otherwise.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.MethodOfConsumption}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.MethodOfConsumption)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (bioDeliveryBuilder_ == null) {
bioDelivery_ = null;
} else {
bioDelivery_ = null;
bioDeliveryBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption(this);
if (bioDeliveryBuilder_ == null) {
result.bioDelivery_ = bioDelivery_;
} else {
result.bioDelivery_ = bioDeliveryBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance()) return this;
if (other.hasBioDelivery()) {
mergeBioDelivery(other.getBioDelivery());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo bioDelivery_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder> bioDeliveryBuilder_;
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public boolean hasBioDelivery() {
return bioDeliveryBuilder_ != null || bioDelivery_ != null;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo getBioDelivery() {
if (bioDeliveryBuilder_ == null) {
return bioDelivery_ == null ? io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.getDefaultInstance() : bioDelivery_;
} else {
return bioDeliveryBuilder_.getMessage();
}
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public Builder setBioDelivery(io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo value) {
if (bioDeliveryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
bioDelivery_ = value;
onChanged();
} else {
bioDeliveryBuilder_.setMessage(value);
}
return this;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public Builder setBioDelivery(
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder builderForValue) {
if (bioDeliveryBuilder_ == null) {
bioDelivery_ = builderForValue.build();
onChanged();
} else {
bioDeliveryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public Builder mergeBioDelivery(io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo value) {
if (bioDeliveryBuilder_ == null) {
if (bioDelivery_ != null) {
bioDelivery_ =
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.newBuilder(bioDelivery_).mergeFrom(value).buildPartial();
} else {
bioDelivery_ = value;
}
onChanged();
} else {
bioDeliveryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public Builder clearBioDelivery() {
if (bioDeliveryBuilder_ == null) {
bioDelivery_ = null;
onChanged();
} else {
bioDelivery_ = null;
bioDeliveryBuilder_ = null;
}
return this;
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder getBioDeliveryBuilder() {
onChanged();
return getBioDeliveryFieldBuilder().getBuilder();
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
public io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder getBioDeliveryOrBuilder() {
if (bioDeliveryBuilder_ != null) {
return bioDeliveryBuilder_.getMessageOrBuilder();
} else {
return bioDelivery_ == null ?
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.getDefaultInstance() : bioDelivery_;
}
}
/**
*
* Indicates the method of biodelivery, and details associated with that type of biodelivery method.
*
*
* .bloombox.consumption.BiodeliveryInfo bio_delivery = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder>
getBioDeliveryFieldBuilder() {
if (bioDeliveryBuilder_ == null) {
bioDeliveryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfo.Builder, io.bloombox.schema.consumption.Biodelivery.BiodeliveryInfoOrBuilder>(
getBioDelivery(),
getParentForChildren(),
isClean());
bioDelivery_ = null;
}
return bioDeliveryBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.MethodOfConsumption)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.MethodOfConsumption)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MethodOfConsumption parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MethodOfConsumption(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompoundMeasurementOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.CompoundMeasurement)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
boolean hasMinimum();
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
io.opencannabis.schema.product.struct.testing.TestValue getMinimum();
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMinimumOrBuilder();
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
boolean hasAverage();
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
io.opencannabis.schema.product.struct.testing.TestValue getAverage();
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getAverageOrBuilder();
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
boolean hasMaximum();
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
io.opencannabis.schema.product.struct.testing.TestValue getMaximum();
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMaximumOrBuilder();
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
boolean hasRounded();
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
io.opencannabis.schema.product.struct.testing.TestValue getRounded();
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getRoundedOrBuilder();
/**
*
* Specifies the absolute up/down presence of a given compound.
*
*
* bool presence = 5;
*/
boolean getPresence();
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
int getCannabinoidValue();
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid();
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
int getTerpeneValue();
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
io.opencannabis.schema.product.struct.testing.Terpene getTerpene();
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.CompoundCase getCompoundCase();
}
/**
*
* Indicates the average, or rounded, measurement for a given compound found to be present in some cannabis product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundMeasurement}
*/
public static final class CompoundMeasurement extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.CompoundMeasurement)
CompoundMeasurementOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompoundMeasurement.newBuilder() to construct.
private CompoundMeasurement(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompoundMeasurement() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompoundMeasurement(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.product.struct.testing.TestValue.Builder subBuilder = null;
if (minimum_ != null) {
subBuilder = minimum_.toBuilder();
}
minimum_ = input.readMessage(io.opencannabis.schema.product.struct.testing.TestValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(minimum_);
minimum_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.product.struct.testing.TestValue.Builder subBuilder = null;
if (average_ != null) {
subBuilder = average_.toBuilder();
}
average_ = input.readMessage(io.opencannabis.schema.product.struct.testing.TestValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(average_);
average_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.product.struct.testing.TestValue.Builder subBuilder = null;
if (maximum_ != null) {
subBuilder = maximum_.toBuilder();
}
maximum_ = input.readMessage(io.opencannabis.schema.product.struct.testing.TestValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(maximum_);
maximum_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.product.struct.testing.TestValue.Builder subBuilder = null;
if (rounded_ != null) {
subBuilder = rounded_.toBuilder();
}
rounded_ = input.readMessage(io.opencannabis.schema.product.struct.testing.TestValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rounded_);
rounded_ = subBuilder.buildPartial();
}
break;
}
case 40: {
presence_ = input.readBool();
break;
}
case 80: {
int rawValue = input.readEnum();
compoundCase_ = 10;
compound_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
compoundCase_ = 11;
compound_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder.class);
}
/**
*
* Specifies the type of compound for which measurements are being declared.
*
*
* Protobuf enum {@code bloombox.identity.bioprint.Aspect.CompoundMeasurement.CompoundType}
*/
public enum CompoundType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Specifies measurements for a cannabinoid.
*
*
* CANNABINOID = 0;
*/
CANNABINOID(0),
/**
*
* Specifies measurements for a terpene.
*
*
* TERPENE = 1;
*/
TERPENE(1),
/**
*
* Specifies measurements for a pesticide.
*
*
* PESTICIDE = 2;
*/
PESTICIDE(2),
/**
*
* Specifies measurements for a heavy metal.
*
*
* METAL = 3;
*/
METAL(3),
/**
*
* Specifies measurements for mold and mildew.
*
*
* MOLD_MILDEW = 4;
*/
MOLD_MILDEW(4),
/**
*
* Specifies measurements for moisture.
*
*
* MOISTURE = 5;
*/
MOISTURE(5),
UNRECOGNIZED(-1),
;
/**
*
* Specifies measurements for a cannabinoid.
*
*
* CANNABINOID = 0;
*/
public static final int CANNABINOID_VALUE = 0;
/**
*
* Specifies measurements for a terpene.
*
*
* TERPENE = 1;
*/
public static final int TERPENE_VALUE = 1;
/**
*
* Specifies measurements for a pesticide.
*
*
* PESTICIDE = 2;
*/
public static final int PESTICIDE_VALUE = 2;
/**
*
* Specifies measurements for a heavy metal.
*
*
* METAL = 3;
*/
public static final int METAL_VALUE = 3;
/**
*
* Specifies measurements for mold and mildew.
*
*
* MOLD_MILDEW = 4;
*/
public static final int MOLD_MILDEW_VALUE = 4;
/**
*
* Specifies measurements for moisture.
*
*
* MOISTURE = 5;
*/
public static final int MOISTURE_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CompoundType valueOf(int value) {
return forNumber(value);
}
public static CompoundType forNumber(int value) {
switch (value) {
case 0: return CANNABINOID;
case 1: return TERPENE;
case 2: return PESTICIDE;
case 3: return METAL;
case 4: return MOLD_MILDEW;
case 5: return MOISTURE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CompoundType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CompoundType findValueByNumber(int number) {
return CompoundType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDescriptor().getEnumTypes().get(0);
}
private static final CompoundType[] VALUES = values();
public static CompoundType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CompoundType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.bioprint.Aspect.CompoundMeasurement.CompoundType)
}
private int compoundCase_ = 0;
private java.lang.Object compound_;
public enum CompoundCase
implements com.google.protobuf.Internal.EnumLite {
CANNABINOID(10),
TERPENE(11),
COMPOUND_NOT_SET(0);
private final int value;
private CompoundCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CompoundCase valueOf(int value) {
return forNumber(value);
}
public static CompoundCase forNumber(int value) {
switch (value) {
case 10: return CANNABINOID;
case 11: return TERPENE;
case 0: return COMPOUND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CompoundCase
getCompoundCase() {
return CompoundCase.forNumber(
compoundCase_);
}
public static final int MINIMUM_FIELD_NUMBER = 1;
private io.opencannabis.schema.product.struct.testing.TestValue minimum_;
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public boolean hasMinimum() {
return minimum_ != null;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getMinimum() {
return minimum_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : minimum_;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMinimumOrBuilder() {
return getMinimum();
}
public static final int AVERAGE_FIELD_NUMBER = 2;
private io.opencannabis.schema.product.struct.testing.TestValue average_;
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public boolean hasAverage() {
return average_ != null;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getAverage() {
return average_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : average_;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getAverageOrBuilder() {
return getAverage();
}
public static final int MAXIMUM_FIELD_NUMBER = 3;
private io.opencannabis.schema.product.struct.testing.TestValue maximum_;
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public boolean hasMaximum() {
return maximum_ != null;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getMaximum() {
return maximum_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : maximum_;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMaximumOrBuilder() {
return getMaximum();
}
public static final int ROUNDED_FIELD_NUMBER = 4;
private io.opencannabis.schema.product.struct.testing.TestValue rounded_;
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public boolean hasRounded() {
return rounded_ != null;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getRounded() {
return rounded_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : rounded_;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getRoundedOrBuilder() {
return getRounded();
}
public static final int PRESENCE_FIELD_NUMBER = 5;
private boolean presence_;
/**
*
* Specifies the absolute up/down presence of a given compound.
*
*
* bool presence = 5;
*/
public boolean getPresence() {
return presence_;
}
public static final int CANNABINOID_FIELD_NUMBER = 10;
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public int getCannabinoidValue() {
if (compoundCase_ == 10) {
return (java.lang.Integer) compound_;
}
return 0;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid() {
if (compoundCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Cannabinoid result = io.opencannabis.schema.product.struct.testing.Cannabinoid.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Cannabinoid.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Cannabinoid.THC;
}
public static final int TERPENE_FIELD_NUMBER = 11;
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public int getTerpeneValue() {
if (compoundCase_ == 11) {
return (java.lang.Integer) compound_;
}
return 0;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public io.opencannabis.schema.product.struct.testing.Terpene getTerpene() {
if (compoundCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Terpene result = io.opencannabis.schema.product.struct.testing.Terpene.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Terpene.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Terpene.CAMPHENE;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (minimum_ != null) {
output.writeMessage(1, getMinimum());
}
if (average_ != null) {
output.writeMessage(2, getAverage());
}
if (maximum_ != null) {
output.writeMessage(3, getMaximum());
}
if (rounded_ != null) {
output.writeMessage(4, getRounded());
}
if (presence_ != false) {
output.writeBool(5, presence_);
}
if (compoundCase_ == 10) {
output.writeEnum(10, ((java.lang.Integer) compound_));
}
if (compoundCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) compound_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (minimum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMinimum());
}
if (average_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAverage());
}
if (maximum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMaximum());
}
if (rounded_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getRounded());
}
if (presence_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, presence_);
}
if (compoundCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, ((java.lang.Integer) compound_));
}
if (compoundCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) compound_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) obj;
if (hasMinimum() != other.hasMinimum()) return false;
if (hasMinimum()) {
if (!getMinimum()
.equals(other.getMinimum())) return false;
}
if (hasAverage() != other.hasAverage()) return false;
if (hasAverage()) {
if (!getAverage()
.equals(other.getAverage())) return false;
}
if (hasMaximum() != other.hasMaximum()) return false;
if (hasMaximum()) {
if (!getMaximum()
.equals(other.getMaximum())) return false;
}
if (hasRounded() != other.hasRounded()) return false;
if (hasRounded()) {
if (!getRounded()
.equals(other.getRounded())) return false;
}
if (getPresence()
!= other.getPresence()) return false;
if (!getCompoundCase().equals(other.getCompoundCase())) return false;
switch (compoundCase_) {
case 10:
if (getCannabinoidValue()
!= other.getCannabinoidValue()) return false;
break;
case 11:
if (getTerpeneValue()
!= other.getTerpeneValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMinimum()) {
hash = (37 * hash) + MINIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMinimum().hashCode();
}
if (hasAverage()) {
hash = (37 * hash) + AVERAGE_FIELD_NUMBER;
hash = (53 * hash) + getAverage().hashCode();
}
if (hasMaximum()) {
hash = (37 * hash) + MAXIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMaximum().hashCode();
}
if (hasRounded()) {
hash = (37 * hash) + ROUNDED_FIELD_NUMBER;
hash = (53 * hash) + getRounded().hashCode();
}
hash = (37 * hash) + PRESENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPresence());
switch (compoundCase_) {
case 10:
hash = (37 * hash) + CANNABINOID_FIELD_NUMBER;
hash = (53 * hash) + getCannabinoidValue();
break;
case 11:
hash = (37 * hash) + TERPENE_FIELD_NUMBER;
hash = (53 * hash) + getTerpeneValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates the average, or rounded, measurement for a given compound found to be present in some cannabis product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundMeasurement}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.CompoundMeasurement)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (minimumBuilder_ == null) {
minimum_ = null;
} else {
minimum_ = null;
minimumBuilder_ = null;
}
if (averageBuilder_ == null) {
average_ = null;
} else {
average_ = null;
averageBuilder_ = null;
}
if (maximumBuilder_ == null) {
maximum_ = null;
} else {
maximum_ = null;
maximumBuilder_ = null;
}
if (roundedBuilder_ == null) {
rounded_ = null;
} else {
rounded_ = null;
roundedBuilder_ = null;
}
presence_ = false;
compoundCase_ = 0;
compound_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement(this);
if (minimumBuilder_ == null) {
result.minimum_ = minimum_;
} else {
result.minimum_ = minimumBuilder_.build();
}
if (averageBuilder_ == null) {
result.average_ = average_;
} else {
result.average_ = averageBuilder_.build();
}
if (maximumBuilder_ == null) {
result.maximum_ = maximum_;
} else {
result.maximum_ = maximumBuilder_.build();
}
if (roundedBuilder_ == null) {
result.rounded_ = rounded_;
} else {
result.rounded_ = roundedBuilder_.build();
}
result.presence_ = presence_;
if (compoundCase_ == 10) {
result.compound_ = compound_;
}
if (compoundCase_ == 11) {
result.compound_ = compound_;
}
result.compoundCase_ = compoundCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance()) return this;
if (other.hasMinimum()) {
mergeMinimum(other.getMinimum());
}
if (other.hasAverage()) {
mergeAverage(other.getAverage());
}
if (other.hasMaximum()) {
mergeMaximum(other.getMaximum());
}
if (other.hasRounded()) {
mergeRounded(other.getRounded());
}
if (other.getPresence() != false) {
setPresence(other.getPresence());
}
switch (other.getCompoundCase()) {
case CANNABINOID: {
setCannabinoidValue(other.getCannabinoidValue());
break;
}
case TERPENE: {
setTerpeneValue(other.getTerpeneValue());
break;
}
case COMPOUND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int compoundCase_ = 0;
private java.lang.Object compound_;
public CompoundCase
getCompoundCase() {
return CompoundCase.forNumber(
compoundCase_);
}
public Builder clearCompound() {
compoundCase_ = 0;
compound_ = null;
onChanged();
return this;
}
private io.opencannabis.schema.product.struct.testing.TestValue minimum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder> minimumBuilder_;
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public boolean hasMinimum() {
return minimumBuilder_ != null || minimum_ != null;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getMinimum() {
if (minimumBuilder_ == null) {
return minimum_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : minimum_;
} else {
return minimumBuilder_.getMessage();
}
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public Builder setMinimum(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (minimumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
minimum_ = value;
onChanged();
} else {
minimumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public Builder setMinimum(
io.opencannabis.schema.product.struct.testing.TestValue.Builder builderForValue) {
if (minimumBuilder_ == null) {
minimum_ = builderForValue.build();
onChanged();
} else {
minimumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public Builder mergeMinimum(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (minimumBuilder_ == null) {
if (minimum_ != null) {
minimum_ =
io.opencannabis.schema.product.struct.testing.TestValue.newBuilder(minimum_).mergeFrom(value).buildPartial();
} else {
minimum_ = value;
}
onChanged();
} else {
minimumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public Builder clearMinimum() {
if (minimumBuilder_ == null) {
minimum_ = null;
onChanged();
} else {
minimum_ = null;
minimumBuilder_ = null;
}
return this;
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public io.opencannabis.schema.product.struct.testing.TestValue.Builder getMinimumBuilder() {
onChanged();
return getMinimumFieldBuilder().getBuilder();
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMinimumOrBuilder() {
if (minimumBuilder_ != null) {
return minimumBuilder_.getMessageOrBuilder();
} else {
return minimum_ == null ?
io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : minimum_;
}
}
/**
*
* Specifies the minimum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue minimum = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>
getMinimumFieldBuilder() {
if (minimumBuilder_ == null) {
minimumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>(
getMinimum(),
getParentForChildren(),
isClean());
minimum_ = null;
}
return minimumBuilder_;
}
private io.opencannabis.schema.product.struct.testing.TestValue average_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder> averageBuilder_;
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public boolean hasAverage() {
return averageBuilder_ != null || average_ != null;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getAverage() {
if (averageBuilder_ == null) {
return average_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : average_;
} else {
return averageBuilder_.getMessage();
}
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public Builder setAverage(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (averageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
average_ = value;
onChanged();
} else {
averageBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public Builder setAverage(
io.opencannabis.schema.product.struct.testing.TestValue.Builder builderForValue) {
if (averageBuilder_ == null) {
average_ = builderForValue.build();
onChanged();
} else {
averageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public Builder mergeAverage(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (averageBuilder_ == null) {
if (average_ != null) {
average_ =
io.opencannabis.schema.product.struct.testing.TestValue.newBuilder(average_).mergeFrom(value).buildPartial();
} else {
average_ = value;
}
onChanged();
} else {
averageBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public Builder clearAverage() {
if (averageBuilder_ == null) {
average_ = null;
onChanged();
} else {
average_ = null;
averageBuilder_ = null;
}
return this;
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public io.opencannabis.schema.product.struct.testing.TestValue.Builder getAverageBuilder() {
onChanged();
return getAverageFieldBuilder().getBuilder();
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getAverageOrBuilder() {
if (averageBuilder_ != null) {
return averageBuilder_.getMessageOrBuilder();
} else {
return average_ == null ?
io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : average_;
}
}
/**
*
* Specifies the average witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue average = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>
getAverageFieldBuilder() {
if (averageBuilder_ == null) {
averageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>(
getAverage(),
getParentForChildren(),
isClean());
average_ = null;
}
return averageBuilder_;
}
private io.opencannabis.schema.product.struct.testing.TestValue maximum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder> maximumBuilder_;
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public boolean hasMaximum() {
return maximumBuilder_ != null || maximum_ != null;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getMaximum() {
if (maximumBuilder_ == null) {
return maximum_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : maximum_;
} else {
return maximumBuilder_.getMessage();
}
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public Builder setMaximum(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (maximumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maximum_ = value;
onChanged();
} else {
maximumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public Builder setMaximum(
io.opencannabis.schema.product.struct.testing.TestValue.Builder builderForValue) {
if (maximumBuilder_ == null) {
maximum_ = builderForValue.build();
onChanged();
} else {
maximumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public Builder mergeMaximum(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (maximumBuilder_ == null) {
if (maximum_ != null) {
maximum_ =
io.opencannabis.schema.product.struct.testing.TestValue.newBuilder(maximum_).mergeFrom(value).buildPartial();
} else {
maximum_ = value;
}
onChanged();
} else {
maximumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public Builder clearMaximum() {
if (maximumBuilder_ == null) {
maximum_ = null;
onChanged();
} else {
maximum_ = null;
maximumBuilder_ = null;
}
return this;
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public io.opencannabis.schema.product.struct.testing.TestValue.Builder getMaximumBuilder() {
onChanged();
return getMaximumFieldBuilder().getBuilder();
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getMaximumOrBuilder() {
if (maximumBuilder_ != null) {
return maximumBuilder_.getMessageOrBuilder();
} else {
return maximum_ == null ?
io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : maximum_;
}
}
/**
*
* Specifies the maximum witnessed amount for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue maximum = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>
getMaximumFieldBuilder() {
if (maximumBuilder_ == null) {
maximumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>(
getMaximum(),
getParentForChildren(),
isClean());
maximum_ = null;
}
return maximumBuilder_;
}
private io.opencannabis.schema.product.struct.testing.TestValue rounded_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder> roundedBuilder_;
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public boolean hasRounded() {
return roundedBuilder_ != null || rounded_ != null;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public io.opencannabis.schema.product.struct.testing.TestValue getRounded() {
if (roundedBuilder_ == null) {
return rounded_ == null ? io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : rounded_;
} else {
return roundedBuilder_.getMessage();
}
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public Builder setRounded(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (roundedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rounded_ = value;
onChanged();
} else {
roundedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public Builder setRounded(
io.opencannabis.schema.product.struct.testing.TestValue.Builder builderForValue) {
if (roundedBuilder_ == null) {
rounded_ = builderForValue.build();
onChanged();
} else {
roundedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public Builder mergeRounded(io.opencannabis.schema.product.struct.testing.TestValue value) {
if (roundedBuilder_ == null) {
if (rounded_ != null) {
rounded_ =
io.opencannabis.schema.product.struct.testing.TestValue.newBuilder(rounded_).mergeFrom(value).buildPartial();
} else {
rounded_ = value;
}
onChanged();
} else {
roundedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public Builder clearRounded() {
if (roundedBuilder_ == null) {
rounded_ = null;
onChanged();
} else {
rounded_ = null;
roundedBuilder_ = null;
}
return this;
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public io.opencannabis.schema.product.struct.testing.TestValue.Builder getRoundedBuilder() {
onChanged();
return getRoundedFieldBuilder().getBuilder();
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
public io.opencannabis.schema.product.struct.testing.TestValueOrBuilder getRoundedOrBuilder() {
if (roundedBuilder_ != null) {
return roundedBuilder_.getMessageOrBuilder();
} else {
return rounded_ == null ?
io.opencannabis.schema.product.struct.testing.TestValue.getDefaultInstance() : rounded_;
}
}
/**
*
* Specifies the rounded-average value witnessed for a particular compound.
*
*
* .opencannabis.structs.labtesting.TestValue rounded = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>
getRoundedFieldBuilder() {
if (roundedBuilder_ == null) {
roundedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.product.struct.testing.TestValue, io.opencannabis.schema.product.struct.testing.TestValue.Builder, io.opencannabis.schema.product.struct.testing.TestValueOrBuilder>(
getRounded(),
getParentForChildren(),
isClean());
rounded_ = null;
}
return roundedBuilder_;
}
private boolean presence_ ;
/**
*
* Specifies the absolute up/down presence of a given compound.
*
*
* bool presence = 5;
*/
public boolean getPresence() {
return presence_;
}
/**
*
* Specifies the absolute up/down presence of a given compound.
*
*
* bool presence = 5;
*/
public Builder setPresence(boolean value) {
presence_ = value;
onChanged();
return this;
}
/**
*
* Specifies the absolute up/down presence of a given compound.
*
*
* bool presence = 5;
*/
public Builder clearPresence() {
presence_ = false;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public int getCannabinoidValue() {
if (compoundCase_ == 10) {
return ((java.lang.Integer) compound_).intValue();
}
return 0;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder setCannabinoidValue(int value) {
compoundCase_ = 10;
compound_ = value;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid() {
if (compoundCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Cannabinoid result = io.opencannabis.schema.product.struct.testing.Cannabinoid.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Cannabinoid.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Cannabinoid.THC;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder setCannabinoid(io.opencannabis.schema.product.struct.testing.Cannabinoid value) {
if (value == null) {
throw new NullPointerException();
}
compoundCase_ = 10;
compound_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder clearCannabinoid() {
if (compoundCase_ == 10) {
compoundCase_ = 0;
compound_ = null;
onChanged();
}
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public int getTerpeneValue() {
if (compoundCase_ == 11) {
return ((java.lang.Integer) compound_).intValue();
}
return 0;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder setTerpeneValue(int value) {
compoundCase_ = 11;
compound_ = value;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public io.opencannabis.schema.product.struct.testing.Terpene getTerpene() {
if (compoundCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Terpene result = io.opencannabis.schema.product.struct.testing.Terpene.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Terpene.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Terpene.CAMPHENE;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder setTerpene(io.opencannabis.schema.product.struct.testing.Terpene value) {
if (value == null) {
throw new NullPointerException();
}
compoundCase_ = 11;
compound_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder clearTerpene() {
if (compoundCase_ == 11) {
compoundCase_ = 0;
compound_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.CompoundMeasurement)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.CompoundMeasurement)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompoundMeasurement parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompoundMeasurement(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BoundedCompoundMeasurementOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement)
com.google.protobuf.MessageOrBuilder {
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
int getBucketValue();
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket getBucket();
}
/**
*
* Indicates the boundary/bucket identity of a given compound found to be present, or not present, in some cannabis
* product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement}
*/
public static final class BoundedCompoundMeasurement extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement)
BoundedCompoundMeasurementOrBuilder {
private static final long serialVersionUID = 0L;
// Use BoundedCompoundMeasurement.newBuilder() to construct.
private BoundedCompoundMeasurement(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BoundedCompoundMeasurement() {
bucket_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BoundedCompoundMeasurement(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
bucket_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.Builder.class);
}
/**
*
* Specifies the general bucket within which a compound's magnitude for a given test result can reside.
*
*
* Protobuf enum {@code bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket}
*/
public enum CompoundBucket
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The compound could not be detected with any significant presence.
*
*
* NOT_PRESENT = 0;
*/
NOT_PRESENT(0),
/**
*
* Trace amounts of the compound were detected.
*
*
* TRACE = 1;
*/
TRACE(1),
/**
*
* Minimal amounts of the compound were detected, relatively speaking.
*
*
* MINIMAL = 2;
*/
MINIMAL(2),
/**
*
* There are standard amounts of the compound detected, relatively speaking.
*
*
* STANDARD = 3;
*/
STANDARD(3),
/**
*
* There were significant amounts of the compound detected, relatively speaking.
*
*
* SIGNIFICANT = 4;
*/
SIGNIFICANT(4),
/**
*
* The compound was present in dominant amounts, relatively speaking.
*
*
* DOMINANT = 5;
*/
DOMINANT(5),
UNRECOGNIZED(-1),
;
/**
*
* The compound could not be detected with any significant presence.
*
*
* NOT_PRESENT = 0;
*/
public static final int NOT_PRESENT_VALUE = 0;
/**
*
* Trace amounts of the compound were detected.
*
*
* TRACE = 1;
*/
public static final int TRACE_VALUE = 1;
/**
*
* Minimal amounts of the compound were detected, relatively speaking.
*
*
* MINIMAL = 2;
*/
public static final int MINIMAL_VALUE = 2;
/**
*
* There are standard amounts of the compound detected, relatively speaking.
*
*
* STANDARD = 3;
*/
public static final int STANDARD_VALUE = 3;
/**
*
* There were significant amounts of the compound detected, relatively speaking.
*
*
* SIGNIFICANT = 4;
*/
public static final int SIGNIFICANT_VALUE = 4;
/**
*
* The compound was present in dominant amounts, relatively speaking.
*
*
* DOMINANT = 5;
*/
public static final int DOMINANT_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CompoundBucket valueOf(int value) {
return forNumber(value);
}
public static CompoundBucket forNumber(int value) {
switch (value) {
case 0: return NOT_PRESENT;
case 1: return TRACE;
case 2: return MINIMAL;
case 3: return STANDARD;
case 4: return SIGNIFICANT;
case 5: return DOMINANT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CompoundBucket> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CompoundBucket findValueByNumber(int number) {
return CompoundBucket.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.getDescriptor().getEnumTypes().get(0);
}
private static final CompoundBucket[] VALUES = values();
public static CompoundBucket valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CompoundBucket(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket)
}
public static final int BUCKET_FIELD_NUMBER = 1;
private int bucket_;
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public int getBucketValue() {
return bucket_;
}
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket getBucket() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket result = io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.valueOf(bucket_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (bucket_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.NOT_PRESENT.getNumber()) {
output.writeEnum(1, bucket_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (bucket_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.NOT_PRESENT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, bucket_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement) obj;
if (bucket_ != other.bucket_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BUCKET_FIELD_NUMBER;
hash = (53 * hash) + bucket_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates the boundary/bucket identity of a given compound found to be present, or not present, in some cannabis
* product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurementOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bucket_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement(this);
result.bucket_ = bucket_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.getDefaultInstance()) return this;
if (other.bucket_ != 0) {
setBucketValue(other.getBucketValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bucket_ = 0;
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public int getBucketValue() {
return bucket_;
}
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public Builder setBucketValue(int value) {
bucket_ = value;
onChanged();
return this;
}
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket getBucket() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket result = io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.valueOf(bucket_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket.UNRECOGNIZED : result;
}
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public Builder setBucket(io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement.CompoundBucket value) {
if (value == null) {
throw new NullPointerException();
}
bucket_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Bucket under which this compound should be filed.
*
*
* .bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement.CompoundBucket bucket = 1;
*/
public Builder clearBucket() {
bucket_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.BoundedCompoundMeasurement)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BoundedCompoundMeasurement parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BoundedCompoundMeasurement(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.BoundedCompoundMeasurement getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompoundRatioOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.CompoundRatio)
com.google.protobuf.MessageOrBuilder {
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
boolean hasLeft();
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getLeft();
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getLeftOrBuilder();
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
boolean hasRight();
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getRight();
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getRightOrBuilder();
}
/**
*
* Indicates the ratio between two compounds found to be present, or not present, in some cannabis product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundRatio}
*/
public static final class CompoundRatio extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.CompoundRatio)
CompoundRatioOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompoundRatio.newBuilder() to construct.
private CompoundRatio(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompoundRatio() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CompoundRatio(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder subBuilder = null;
if (left_ != null) {
subBuilder = left_.toBuilder();
}
left_ = input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(left_);
left_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder subBuilder = null;
if (right_ != null) {
subBuilder = right_.toBuilder();
}
right_ = input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(right_);
right_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder.class);
}
public interface RatioPortionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion)
com.google.protobuf.MessageOrBuilder {
/**
*
* Value of the ratio portion.
*
*
* uint32 value = 1;
*/
int getValue();
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
int getCannabinoidValue();
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid();
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
int getTerpeneValue();
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
io.opencannabis.schema.product.struct.testing.Terpene getTerpene();
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.CompoundCase getCompoundCase();
}
/**
*
* Specifies a left, or right, portion of a given compound ratio, along with the compound involved.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion}
*/
public static final class RatioPortion extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion)
RatioPortionOrBuilder {
private static final long serialVersionUID = 0L;
// Use RatioPortion.newBuilder() to construct.
private RatioPortion(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RatioPortion() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RatioPortion(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
value_ = input.readUInt32();
break;
}
case 80: {
int rawValue = input.readEnum();
compoundCase_ = 10;
compound_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
compoundCase_ = 11;
compound_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder.class);
}
private int compoundCase_ = 0;
private java.lang.Object compound_;
public enum CompoundCase
implements com.google.protobuf.Internal.EnumLite {
CANNABINOID(10),
TERPENE(11),
COMPOUND_NOT_SET(0);
private final int value;
private CompoundCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CompoundCase valueOf(int value) {
return forNumber(value);
}
public static CompoundCase forNumber(int value) {
switch (value) {
case 10: return CANNABINOID;
case 11: return TERPENE;
case 0: return COMPOUND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CompoundCase
getCompoundCase() {
return CompoundCase.forNumber(
compoundCase_);
}
public static final int VALUE_FIELD_NUMBER = 1;
private int value_;
/**
*
* Value of the ratio portion.
*
*
* uint32 value = 1;
*/
public int getValue() {
return value_;
}
public static final int CANNABINOID_FIELD_NUMBER = 10;
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public int getCannabinoidValue() {
if (compoundCase_ == 10) {
return (java.lang.Integer) compound_;
}
return 0;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid() {
if (compoundCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Cannabinoid result = io.opencannabis.schema.product.struct.testing.Cannabinoid.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Cannabinoid.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Cannabinoid.THC;
}
public static final int TERPENE_FIELD_NUMBER = 11;
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public int getTerpeneValue() {
if (compoundCase_ == 11) {
return (java.lang.Integer) compound_;
}
return 0;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public io.opencannabis.schema.product.struct.testing.Terpene getTerpene() {
if (compoundCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Terpene result = io.opencannabis.schema.product.struct.testing.Terpene.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Terpene.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Terpene.CAMPHENE;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (value_ != 0) {
output.writeUInt32(1, value_);
}
if (compoundCase_ == 10) {
output.writeEnum(10, ((java.lang.Integer) compound_));
}
if (compoundCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) compound_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (value_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, value_);
}
if (compoundCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, ((java.lang.Integer) compound_));
}
if (compoundCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) compound_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion) obj;
if (getValue()
!= other.getValue()) return false;
if (!getCompoundCase().equals(other.getCompoundCase())) return false;
switch (compoundCase_) {
case 10:
if (getCannabinoidValue()
!= other.getCannabinoidValue()) return false;
break;
case 11:
if (getTerpeneValue()
!= other.getTerpeneValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue();
switch (compoundCase_) {
case 10:
hash = (37 * hash) + CANNABINOID_FIELD_NUMBER;
hash = (53 * hash) + getCannabinoidValue();
break;
case 11:
hash = (37 * hash) + TERPENE_FIELD_NUMBER;
hash = (53 * hash) + getTerpeneValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a left, or right, portion of a given compound ratio, along with the compound involved.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
value_ = 0;
compoundCase_ = 0;
compound_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion(this);
result.value_ = value_;
if (compoundCase_ == 10) {
result.compound_ = compound_;
}
if (compoundCase_ == 11) {
result.compound_ = compound_;
}
result.compoundCase_ = compoundCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance()) return this;
if (other.getValue() != 0) {
setValue(other.getValue());
}
switch (other.getCompoundCase()) {
case CANNABINOID: {
setCannabinoidValue(other.getCannabinoidValue());
break;
}
case TERPENE: {
setTerpeneValue(other.getTerpeneValue());
break;
}
case COMPOUND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int compoundCase_ = 0;
private java.lang.Object compound_;
public CompoundCase
getCompoundCase() {
return CompoundCase.forNumber(
compoundCase_);
}
public Builder clearCompound() {
compoundCase_ = 0;
compound_ = null;
onChanged();
return this;
}
private int value_ ;
/**
*
* Value of the ratio portion.
*
*
* uint32 value = 1;
*/
public int getValue() {
return value_;
}
/**
*
* Value of the ratio portion.
*
*
* uint32 value = 1;
*/
public Builder setValue(int value) {
value_ = value;
onChanged();
return this;
}
/**
*
* Value of the ratio portion.
*
*
* uint32 value = 1;
*/
public Builder clearValue() {
value_ = 0;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public int getCannabinoidValue() {
if (compoundCase_ == 10) {
return ((java.lang.Integer) compound_).intValue();
}
return 0;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder setCannabinoidValue(int value) {
compoundCase_ = 10;
compound_ = value;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public io.opencannabis.schema.product.struct.testing.Cannabinoid getCannabinoid() {
if (compoundCase_ == 10) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Cannabinoid result = io.opencannabis.schema.product.struct.testing.Cannabinoid.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Cannabinoid.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Cannabinoid.THC;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder setCannabinoid(io.opencannabis.schema.product.struct.testing.Cannabinoid value) {
if (value == null) {
throw new NullPointerException();
}
compoundCase_ = 10;
compound_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given cannabinoid.
*
*
* .opencannabis.structs.labtesting.Cannabinoid cannabinoid = 10;
*/
public Builder clearCannabinoid() {
if (compoundCase_ == 10) {
compoundCase_ = 0;
compound_ = null;
onChanged();
}
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public int getTerpeneValue() {
if (compoundCase_ == 11) {
return ((java.lang.Integer) compound_).intValue();
}
return 0;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder setTerpeneValue(int value) {
compoundCase_ = 11;
compound_ = value;
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public io.opencannabis.schema.product.struct.testing.Terpene getTerpene() {
if (compoundCase_ == 11) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.product.struct.testing.Terpene result = io.opencannabis.schema.product.struct.testing.Terpene.valueOf(
(java.lang.Integer) compound_);
return result == null ? io.opencannabis.schema.product.struct.testing.Terpene.UNRECOGNIZED : result;
}
return io.opencannabis.schema.product.struct.testing.Terpene.CAMPHENE;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder setTerpene(io.opencannabis.schema.product.struct.testing.Terpene value) {
if (value == null) {
throw new NullPointerException();
}
compoundCase_ = 11;
compound_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies compound measurements for a given terpene or terpenoid.
*
*
* .opencannabis.structs.labtesting.Terpene terpene = 11;
*/
public Builder clearTerpene() {
if (compoundCase_ == 11) {
compoundCase_ = 0;
compound_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RatioPortion parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RatioPortion(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int LEFT_FIELD_NUMBER = 1;
private io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion left_;
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public boolean hasLeft() {
return left_ != null;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getLeft() {
return left_ == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : left_;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getLeftOrBuilder() {
return getLeft();
}
public static final int RIGHT_FIELD_NUMBER = 2;
private io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion right_;
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public boolean hasRight() {
return right_ != null;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getRight() {
return right_ == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : right_;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getRightOrBuilder() {
return getRight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (left_ != null) {
output.writeMessage(1, getLeft());
}
if (right_ != null) {
output.writeMessage(2, getRight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (left_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLeft());
}
if (right_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) obj;
if (hasLeft() != other.hasLeft()) return false;
if (hasLeft()) {
if (!getLeft()
.equals(other.getLeft())) return false;
}
if (hasRight() != other.hasRight()) return false;
if (hasRight()) {
if (!getRight()
.equals(other.getRight())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLeft()) {
hash = (37 * hash) + LEFT_FIELD_NUMBER;
hash = (53 * hash) + getLeft().hashCode();
}
if (hasRight()) {
hash = (37 * hash) + RIGHT_FIELD_NUMBER;
hash = (53 * hash) + getRight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates the ratio between two compounds found to be present, or not present, in some cannabis product.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CompoundRatio}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.CompoundRatio)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (leftBuilder_ == null) {
left_ = null;
} else {
left_ = null;
leftBuilder_ = null;
}
if (rightBuilder_ == null) {
right_ = null;
} else {
right_ = null;
rightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio(this);
if (leftBuilder_ == null) {
result.left_ = left_;
} else {
result.left_ = leftBuilder_.build();
}
if (rightBuilder_ == null) {
result.right_ = right_;
} else {
result.right_ = rightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance()) return this;
if (other.hasLeft()) {
mergeLeft(other.getLeft());
}
if (other.hasRight()) {
mergeRight(other.getRight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion left_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder> leftBuilder_;
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public boolean hasLeft() {
return leftBuilder_ != null || left_ != null;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getLeft() {
if (leftBuilder_ == null) {
return left_ == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : left_;
} else {
return leftBuilder_.getMessage();
}
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public Builder setLeft(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion value) {
if (leftBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
left_ = value;
onChanged();
} else {
leftBuilder_.setMessage(value);
}
return this;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public Builder setLeft(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder builderForValue) {
if (leftBuilder_ == null) {
left_ = builderForValue.build();
onChanged();
} else {
leftBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public Builder mergeLeft(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion value) {
if (leftBuilder_ == null) {
if (left_ != null) {
left_ =
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.newBuilder(left_).mergeFrom(value).buildPartial();
} else {
left_ = value;
}
onChanged();
} else {
leftBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public Builder clearLeft() {
if (leftBuilder_ == null) {
left_ = null;
onChanged();
} else {
left_ = null;
leftBuilder_ = null;
}
return this;
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder getLeftBuilder() {
onChanged();
return getLeftFieldBuilder().getBuilder();
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getLeftOrBuilder() {
if (leftBuilder_ != null) {
return leftBuilder_.getMessageOrBuilder();
} else {
return left_ == null ?
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : left_;
}
}
/**
*
* Left-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion left = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder>
getLeftFieldBuilder() {
if (leftBuilder_ == null) {
leftBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder>(
getLeft(),
getParentForChildren(),
isClean());
left_ = null;
}
return leftBuilder_;
}
private io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion right_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder> rightBuilder_;
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public boolean hasRight() {
return rightBuilder_ != null || right_ != null;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion getRight() {
if (rightBuilder_ == null) {
return right_ == null ? io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : right_;
} else {
return rightBuilder_.getMessage();
}
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public Builder setRight(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion value) {
if (rightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
right_ = value;
onChanged();
} else {
rightBuilder_.setMessage(value);
}
return this;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public Builder setRight(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder builderForValue) {
if (rightBuilder_ == null) {
right_ = builderForValue.build();
onChanged();
} else {
rightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public Builder mergeRight(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion value) {
if (rightBuilder_ == null) {
if (right_ != null) {
right_ =
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.newBuilder(right_).mergeFrom(value).buildPartial();
} else {
right_ = value;
}
onChanged();
} else {
rightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public Builder clearRight() {
if (rightBuilder_ == null) {
right_ = null;
onChanged();
} else {
right_ = null;
rightBuilder_ = null;
}
return this;
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder getRightBuilder() {
onChanged();
return getRightFieldBuilder().getBuilder();
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder getRightOrBuilder() {
if (rightBuilder_ != null) {
return rightBuilder_.getMessageOrBuilder();
} else {
return right_ == null ?
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.getDefaultInstance() : right_;
}
}
/**
*
* Right-side of the compound ratio.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio.RatioPortion right = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder>
getRightFieldBuilder() {
if (rightBuilder_ == null) {
rightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortion.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.RatioPortionOrBuilder>(
getRight(),
getParentForChildren(),
isClean());
right_ = null;
}
return rightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.CompoundRatio)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.CompoundRatio)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompoundRatio parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CompoundRatio(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TimingBoundaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.TimingBoundary)
com.google.protobuf.MessageOrBuilder {
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
boolean hasFirst();
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
io.opencannabis.schema.temporal.Timehash getFirst();
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
io.opencannabis.schema.temporal.TimehashOrBuilder getFirstOrBuilder();
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
boolean hasLatest();
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
io.opencannabis.schema.temporal.Timehash getLatest();
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
io.opencannabis.schema.temporal.TimehashOrBuilder getLatestOrBuilder();
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
boolean hasArea();
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
io.opencannabis.schema.temporal.Timehash getArea();
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
io.opencannabis.schema.temporal.TimehashOrBuilder getAreaOrBuilder();
}
/**
*
* Indicates a timing-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption timing and purchase timing boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.TimingBoundary}
*/
public static final class TimingBoundary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.TimingBoundary)
TimingBoundaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use TimingBoundary.newBuilder() to construct.
private TimingBoundary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TimingBoundary() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TimingBoundary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.temporal.Timehash.Builder subBuilder = null;
if (first_ != null) {
subBuilder = first_.toBuilder();
}
first_ = input.readMessage(io.opencannabis.schema.temporal.Timehash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(first_);
first_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.Timehash.Builder subBuilder = null;
if (latest_ != null) {
subBuilder = latest_.toBuilder();
}
latest_ = input.readMessage(io.opencannabis.schema.temporal.Timehash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(latest_);
latest_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.temporal.Timehash.Builder subBuilder = null;
if (area_ != null) {
subBuilder = area_.toBuilder();
}
area_ = input.readMessage(io.opencannabis.schema.temporal.Timehash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(area_);
area_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder.class);
}
public static final int FIRST_FIELD_NUMBER = 1;
private io.opencannabis.schema.temporal.Timehash first_;
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public boolean hasFirst() {
return first_ != null;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public io.opencannabis.schema.temporal.Timehash getFirst() {
return first_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : first_;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getFirstOrBuilder() {
return getFirst();
}
public static final int LATEST_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.Timehash latest_;
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public boolean hasLatest() {
return latest_ != null;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public io.opencannabis.schema.temporal.Timehash getLatest() {
return latest_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : latest_;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getLatestOrBuilder() {
return getLatest();
}
public static final int AREA_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.Timehash area_;
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public boolean hasArea() {
return area_ != null;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public io.opencannabis.schema.temporal.Timehash getArea() {
return area_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : area_;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getAreaOrBuilder() {
return getArea();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (first_ != null) {
output.writeMessage(1, getFirst());
}
if (latest_ != null) {
output.writeMessage(2, getLatest());
}
if (area_ != null) {
output.writeMessage(3, getArea());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (first_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFirst());
}
if (latest_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getLatest());
}
if (area_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getArea());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) obj;
if (hasFirst() != other.hasFirst()) return false;
if (hasFirst()) {
if (!getFirst()
.equals(other.getFirst())) return false;
}
if (hasLatest() != other.hasLatest()) return false;
if (hasLatest()) {
if (!getLatest()
.equals(other.getLatest())) return false;
}
if (hasArea() != other.hasArea()) return false;
if (hasArea()) {
if (!getArea()
.equals(other.getArea())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFirst()) {
hash = (37 * hash) + FIRST_FIELD_NUMBER;
hash = (53 * hash) + getFirst().hashCode();
}
if (hasLatest()) {
hash = (37 * hash) + LATEST_FIELD_NUMBER;
hash = (53 * hash) + getLatest().hashCode();
}
if (hasArea()) {
hash = (37 * hash) + AREA_FIELD_NUMBER;
hash = (53 * hash) + getArea().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates a timing-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption timing and purchase timing boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.TimingBoundary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.TimingBoundary)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (firstBuilder_ == null) {
first_ = null;
} else {
first_ = null;
firstBuilder_ = null;
}
if (latestBuilder_ == null) {
latest_ = null;
} else {
latest_ = null;
latestBuilder_ = null;
}
if (areaBuilder_ == null) {
area_ = null;
} else {
area_ = null;
areaBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary(this);
if (firstBuilder_ == null) {
result.first_ = first_;
} else {
result.first_ = firstBuilder_.build();
}
if (latestBuilder_ == null) {
result.latest_ = latest_;
} else {
result.latest_ = latestBuilder_.build();
}
if (areaBuilder_ == null) {
result.area_ = area_;
} else {
result.area_ = areaBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance()) return this;
if (other.hasFirst()) {
mergeFirst(other.getFirst());
}
if (other.hasLatest()) {
mergeLatest(other.getLatest());
}
if (other.hasArea()) {
mergeArea(other.getArea());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.temporal.Timehash first_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder> firstBuilder_;
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public boolean hasFirst() {
return firstBuilder_ != null || first_ != null;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public io.opencannabis.schema.temporal.Timehash getFirst() {
if (firstBuilder_ == null) {
return first_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : first_;
} else {
return firstBuilder_.getMessage();
}
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public Builder setFirst(io.opencannabis.schema.temporal.Timehash value) {
if (firstBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
first_ = value;
onChanged();
} else {
firstBuilder_.setMessage(value);
}
return this;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public Builder setFirst(
io.opencannabis.schema.temporal.Timehash.Builder builderForValue) {
if (firstBuilder_ == null) {
first_ = builderForValue.build();
onChanged();
} else {
firstBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public Builder mergeFirst(io.opencannabis.schema.temporal.Timehash value) {
if (firstBuilder_ == null) {
if (first_ != null) {
first_ =
io.opencannabis.schema.temporal.Timehash.newBuilder(first_).mergeFrom(value).buildPartial();
} else {
first_ = value;
}
onChanged();
} else {
firstBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public Builder clearFirst() {
if (firstBuilder_ == null) {
first_ = null;
onChanged();
} else {
first_ = null;
firstBuilder_ = null;
}
return this;
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public io.opencannabis.schema.temporal.Timehash.Builder getFirstBuilder() {
onChanged();
return getFirstFieldBuilder().getBuilder();
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getFirstOrBuilder() {
if (firstBuilder_ != null) {
return firstBuilder_.getMessageOrBuilder();
} else {
return first_ == null ?
io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : first_;
}
}
/**
*
* The first point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash first = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>
getFirstFieldBuilder() {
if (firstBuilder_ == null) {
firstBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>(
getFirst(),
getParentForChildren(),
isClean());
first_ = null;
}
return firstBuilder_;
}
private io.opencannabis.schema.temporal.Timehash latest_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder> latestBuilder_;
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public boolean hasLatest() {
return latestBuilder_ != null || latest_ != null;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public io.opencannabis.schema.temporal.Timehash getLatest() {
if (latestBuilder_ == null) {
return latest_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : latest_;
} else {
return latestBuilder_.getMessage();
}
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public Builder setLatest(io.opencannabis.schema.temporal.Timehash value) {
if (latestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
latest_ = value;
onChanged();
} else {
latestBuilder_.setMessage(value);
}
return this;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public Builder setLatest(
io.opencannabis.schema.temporal.Timehash.Builder builderForValue) {
if (latestBuilder_ == null) {
latest_ = builderForValue.build();
onChanged();
} else {
latestBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public Builder mergeLatest(io.opencannabis.schema.temporal.Timehash value) {
if (latestBuilder_ == null) {
if (latest_ != null) {
latest_ =
io.opencannabis.schema.temporal.Timehash.newBuilder(latest_).mergeFrom(value).buildPartial();
} else {
latest_ = value;
}
onChanged();
} else {
latestBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public Builder clearLatest() {
if (latestBuilder_ == null) {
latest_ = null;
onChanged();
} else {
latest_ = null;
latestBuilder_ = null;
}
return this;
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public io.opencannabis.schema.temporal.Timehash.Builder getLatestBuilder() {
onChanged();
return getLatestFieldBuilder().getBuilder();
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getLatestOrBuilder() {
if (latestBuilder_ != null) {
return latestBuilder_.getMessageOrBuilder();
} else {
return latest_ == null ?
io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : latest_;
}
}
/**
*
* The latest point in a timing boundary.
*
*
* .opencannabis.temporal.Timehash latest = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>
getLatestFieldBuilder() {
if (latestBuilder_ == null) {
latestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>(
getLatest(),
getParentForChildren(),
isClean());
latest_ = null;
}
return latestBuilder_;
}
private io.opencannabis.schema.temporal.Timehash area_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder> areaBuilder_;
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public boolean hasArea() {
return areaBuilder_ != null || area_ != null;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public io.opencannabis.schema.temporal.Timehash getArea() {
if (areaBuilder_ == null) {
return area_ == null ? io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : area_;
} else {
return areaBuilder_.getMessage();
}
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public Builder setArea(io.opencannabis.schema.temporal.Timehash value) {
if (areaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
area_ = value;
onChanged();
} else {
areaBuilder_.setMessage(value);
}
return this;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public Builder setArea(
io.opencannabis.schema.temporal.Timehash.Builder builderForValue) {
if (areaBuilder_ == null) {
area_ = builderForValue.build();
onChanged();
} else {
areaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public Builder mergeArea(io.opencannabis.schema.temporal.Timehash value) {
if (areaBuilder_ == null) {
if (area_ != null) {
area_ =
io.opencannabis.schema.temporal.Timehash.newBuilder(area_).mergeFrom(value).buildPartial();
} else {
area_ = value;
}
onChanged();
} else {
areaBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public Builder clearArea() {
if (areaBuilder_ == null) {
area_ = null;
onChanged();
} else {
area_ = null;
areaBuilder_ = null;
}
return this;
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public io.opencannabis.schema.temporal.Timehash.Builder getAreaBuilder() {
onChanged();
return getAreaFieldBuilder().getBuilder();
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
public io.opencannabis.schema.temporal.TimehashOrBuilder getAreaOrBuilder() {
if (areaBuilder_ != null) {
return areaBuilder_.getMessageOrBuilder();
} else {
return area_ == null ?
io.opencannabis.schema.temporal.Timehash.getDefaultInstance() : area_;
}
}
/**
*
* The narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.temporal.Timehash area = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>
getAreaFieldBuilder() {
if (areaBuilder_ == null) {
areaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Timehash, io.opencannabis.schema.temporal.Timehash.Builder, io.opencannabis.schema.temporal.TimehashOrBuilder>(
getArea(),
getParentForChildren(),
isClean());
area_ = null;
}
return areaBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.TimingBoundary)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.TimingBoundary)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TimingBoundary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TimingBoundary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LocationBoundaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.LocationBoundary)
com.google.protobuf.MessageOrBuilder {
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
boolean hasArea();
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
io.opencannabis.schema.geo.Geohash getArea();
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
io.opencannabis.schema.geo.GeohashOrBuilder getAreaOrBuilder();
}
/**
*
* Indicates a location-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption and purchase location boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.LocationBoundary}
*/
public static final class LocationBoundary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.LocationBoundary)
LocationBoundaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocationBoundary.newBuilder() to construct.
private LocationBoundary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocationBoundary() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocationBoundary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.geo.Geohash.Builder subBuilder = null;
if (area_ != null) {
subBuilder = area_.toBuilder();
}
area_ = input.readMessage(io.opencannabis.schema.geo.Geohash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(area_);
area_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder.class);
}
public static final int AREA_FIELD_NUMBER = 1;
private io.opencannabis.schema.geo.Geohash area_;
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public boolean hasArea() {
return area_ != null;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public io.opencannabis.schema.geo.Geohash getArea() {
return area_ == null ? io.opencannabis.schema.geo.Geohash.getDefaultInstance() : area_;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public io.opencannabis.schema.geo.GeohashOrBuilder getAreaOrBuilder() {
return getArea();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (area_ != null) {
output.writeMessage(1, getArea());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (area_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getArea());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) obj;
if (hasArea() != other.hasArea()) return false;
if (hasArea()) {
if (!getArea()
.equals(other.getArea())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasArea()) {
hash = (37 * hash) + AREA_FIELD_NUMBER;
hash = (53 * hash) + getArea().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates a location-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption and purchase location boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.LocationBoundary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.LocationBoundary)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (areaBuilder_ == null) {
area_ = null;
} else {
area_ = null;
areaBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary(this);
if (areaBuilder_ == null) {
result.area_ = area_;
} else {
result.area_ = areaBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance()) return this;
if (other.hasArea()) {
mergeArea(other.getArea());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.geo.Geohash area_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Geohash, io.opencannabis.schema.geo.Geohash.Builder, io.opencannabis.schema.geo.GeohashOrBuilder> areaBuilder_;
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public boolean hasArea() {
return areaBuilder_ != null || area_ != null;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public io.opencannabis.schema.geo.Geohash getArea() {
if (areaBuilder_ == null) {
return area_ == null ? io.opencannabis.schema.geo.Geohash.getDefaultInstance() : area_;
} else {
return areaBuilder_.getMessage();
}
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public Builder setArea(io.opencannabis.schema.geo.Geohash value) {
if (areaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
area_ = value;
onChanged();
} else {
areaBuilder_.setMessage(value);
}
return this;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public Builder setArea(
io.opencannabis.schema.geo.Geohash.Builder builderForValue) {
if (areaBuilder_ == null) {
area_ = builderForValue.build();
onChanged();
} else {
areaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public Builder mergeArea(io.opencannabis.schema.geo.Geohash value) {
if (areaBuilder_ == null) {
if (area_ != null) {
area_ =
io.opencannabis.schema.geo.Geohash.newBuilder(area_).mergeFrom(value).buildPartial();
} else {
area_ = value;
}
onChanged();
} else {
areaBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public Builder clearArea() {
if (areaBuilder_ == null) {
area_ = null;
onChanged();
} else {
area_ = null;
areaBuilder_ = null;
}
return this;
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public io.opencannabis.schema.geo.Geohash.Builder getAreaBuilder() {
onChanged();
return getAreaFieldBuilder().getBuilder();
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
public io.opencannabis.schema.geo.GeohashOrBuilder getAreaOrBuilder() {
if (areaBuilder_ != null) {
return areaBuilder_.getMessageOrBuilder();
} else {
return area_ == null ?
io.opencannabis.schema.geo.Geohash.getDefaultInstance() : area_;
}
}
/**
*
* Indicates the narrowest-common-area within which behavior of some kind was observed.
*
*
* .opencannabis.geo.Geohash area = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Geohash, io.opencannabis.schema.geo.Geohash.Builder, io.opencannabis.schema.geo.GeohashOrBuilder>
getAreaFieldBuilder() {
if (areaBuilder_ == null) {
areaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.geo.Geohash, io.opencannabis.schema.geo.Geohash.Builder, io.opencannabis.schema.geo.GeohashOrBuilder>(
getArea(),
getParentForChildren(),
isClean());
area_ = null;
}
return areaBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.LocationBoundary)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.LocationBoundary)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocationBoundary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocationBoundary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DurationBoundaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.DurationBoundary)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
boolean hasMinimum();
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
io.opencannabis.schema.temporal.Duration getMinimum();
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
io.opencannabis.schema.temporal.DurationOrBuilder getMinimumOrBuilder();
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
boolean hasAverage();
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
io.opencannabis.schema.temporal.Duration getAverage();
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
io.opencannabis.schema.temporal.DurationOrBuilder getAverageOrBuilder();
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
boolean hasMaximum();
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
io.opencannabis.schema.temporal.Duration getMaximum();
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
io.opencannabis.schema.temporal.DurationOrBuilder getMaximumOrBuilder();
}
/**
*
* Indicates a duration-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption and purchase duration boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.DurationBoundary}
*/
public static final class DurationBoundary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.DurationBoundary)
DurationBoundaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use DurationBoundary.newBuilder() to construct.
private DurationBoundary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DurationBoundary() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DurationBoundary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.temporal.Duration.Builder subBuilder = null;
if (minimum_ != null) {
subBuilder = minimum_.toBuilder();
}
minimum_ = input.readMessage(io.opencannabis.schema.temporal.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(minimum_);
minimum_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.Duration.Builder subBuilder = null;
if (average_ != null) {
subBuilder = average_.toBuilder();
}
average_ = input.readMessage(io.opencannabis.schema.temporal.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(average_);
average_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.temporal.Duration.Builder subBuilder = null;
if (maximum_ != null) {
subBuilder = maximum_.toBuilder();
}
maximum_ = input.readMessage(io.opencannabis.schema.temporal.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(maximum_);
maximum_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder.class);
}
public static final int MINIMUM_FIELD_NUMBER = 1;
private io.opencannabis.schema.temporal.Duration minimum_;
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public boolean hasMinimum() {
return minimum_ != null;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public io.opencannabis.schema.temporal.Duration getMinimum() {
return minimum_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : minimum_;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getMinimumOrBuilder() {
return getMinimum();
}
public static final int AVERAGE_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.Duration average_;
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public boolean hasAverage() {
return average_ != null;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public io.opencannabis.schema.temporal.Duration getAverage() {
return average_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : average_;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getAverageOrBuilder() {
return getAverage();
}
public static final int MAXIMUM_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.Duration maximum_;
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public boolean hasMaximum() {
return maximum_ != null;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public io.opencannabis.schema.temporal.Duration getMaximum() {
return maximum_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : maximum_;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getMaximumOrBuilder() {
return getMaximum();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (minimum_ != null) {
output.writeMessage(1, getMinimum());
}
if (average_ != null) {
output.writeMessage(2, getAverage());
}
if (maximum_ != null) {
output.writeMessage(3, getMaximum());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (minimum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMinimum());
}
if (average_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAverage());
}
if (maximum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMaximum());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) obj;
if (hasMinimum() != other.hasMinimum()) return false;
if (hasMinimum()) {
if (!getMinimum()
.equals(other.getMinimum())) return false;
}
if (hasAverage() != other.hasAverage()) return false;
if (hasAverage()) {
if (!getAverage()
.equals(other.getAverage())) return false;
}
if (hasMaximum() != other.hasMaximum()) return false;
if (hasMaximum()) {
if (!getMaximum()
.equals(other.getMaximum())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMinimum()) {
hash = (37 * hash) + MINIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMinimum().hashCode();
}
if (hasAverage()) {
hash = (37 * hash) + AVERAGE_FIELD_NUMBER;
hash = (53 * hash) + getAverage().hashCode();
}
if (hasMaximum()) {
hash = (37 * hash) + MAXIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMaximum().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates a duration-based boundary or bucket within which some cannabis use or product resides. This structure may
* be used both with consumption and purchase duration boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.DurationBoundary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.DurationBoundary)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (minimumBuilder_ == null) {
minimum_ = null;
} else {
minimum_ = null;
minimumBuilder_ = null;
}
if (averageBuilder_ == null) {
average_ = null;
} else {
average_ = null;
averageBuilder_ = null;
}
if (maximumBuilder_ == null) {
maximum_ = null;
} else {
maximum_ = null;
maximumBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary(this);
if (minimumBuilder_ == null) {
result.minimum_ = minimum_;
} else {
result.minimum_ = minimumBuilder_.build();
}
if (averageBuilder_ == null) {
result.average_ = average_;
} else {
result.average_ = averageBuilder_.build();
}
if (maximumBuilder_ == null) {
result.maximum_ = maximum_;
} else {
result.maximum_ = maximumBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance()) return this;
if (other.hasMinimum()) {
mergeMinimum(other.getMinimum());
}
if (other.hasAverage()) {
mergeAverage(other.getAverage());
}
if (other.hasMaximum()) {
mergeMaximum(other.getMaximum());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.temporal.Duration minimum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder> minimumBuilder_;
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public boolean hasMinimum() {
return minimumBuilder_ != null || minimum_ != null;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public io.opencannabis.schema.temporal.Duration getMinimum() {
if (minimumBuilder_ == null) {
return minimum_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : minimum_;
} else {
return minimumBuilder_.getMessage();
}
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public Builder setMinimum(io.opencannabis.schema.temporal.Duration value) {
if (minimumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
minimum_ = value;
onChanged();
} else {
minimumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public Builder setMinimum(
io.opencannabis.schema.temporal.Duration.Builder builderForValue) {
if (minimumBuilder_ == null) {
minimum_ = builderForValue.build();
onChanged();
} else {
minimumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public Builder mergeMinimum(io.opencannabis.schema.temporal.Duration value) {
if (minimumBuilder_ == null) {
if (minimum_ != null) {
minimum_ =
io.opencannabis.schema.temporal.Duration.newBuilder(minimum_).mergeFrom(value).buildPartial();
} else {
minimum_ = value;
}
onChanged();
} else {
minimumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public Builder clearMinimum() {
if (minimumBuilder_ == null) {
minimum_ = null;
onChanged();
} else {
minimum_ = null;
minimumBuilder_ = null;
}
return this;
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public io.opencannabis.schema.temporal.Duration.Builder getMinimumBuilder() {
onChanged();
return getMinimumFieldBuilder().getBuilder();
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getMinimumOrBuilder() {
if (minimumBuilder_ != null) {
return minimumBuilder_.getMessageOrBuilder();
} else {
return minimum_ == null ?
io.opencannabis.schema.temporal.Duration.getDefaultInstance() : minimum_;
}
}
/**
*
* Specifies a minimum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration minimum = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>
getMinimumFieldBuilder() {
if (minimumBuilder_ == null) {
minimumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>(
getMinimum(),
getParentForChildren(),
isClean());
minimum_ = null;
}
return minimumBuilder_;
}
private io.opencannabis.schema.temporal.Duration average_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder> averageBuilder_;
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public boolean hasAverage() {
return averageBuilder_ != null || average_ != null;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public io.opencannabis.schema.temporal.Duration getAverage() {
if (averageBuilder_ == null) {
return average_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : average_;
} else {
return averageBuilder_.getMessage();
}
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public Builder setAverage(io.opencannabis.schema.temporal.Duration value) {
if (averageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
average_ = value;
onChanged();
} else {
averageBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public Builder setAverage(
io.opencannabis.schema.temporal.Duration.Builder builderForValue) {
if (averageBuilder_ == null) {
average_ = builderForValue.build();
onChanged();
} else {
averageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public Builder mergeAverage(io.opencannabis.schema.temporal.Duration value) {
if (averageBuilder_ == null) {
if (average_ != null) {
average_ =
io.opencannabis.schema.temporal.Duration.newBuilder(average_).mergeFrom(value).buildPartial();
} else {
average_ = value;
}
onChanged();
} else {
averageBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public Builder clearAverage() {
if (averageBuilder_ == null) {
average_ = null;
onChanged();
} else {
average_ = null;
averageBuilder_ = null;
}
return this;
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public io.opencannabis.schema.temporal.Duration.Builder getAverageBuilder() {
onChanged();
return getAverageFieldBuilder().getBuilder();
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getAverageOrBuilder() {
if (averageBuilder_ != null) {
return averageBuilder_.getMessageOrBuilder();
} else {
return average_ == null ?
io.opencannabis.schema.temporal.Duration.getDefaultInstance() : average_;
}
}
/**
*
* Specifies an average duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration average = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>
getAverageFieldBuilder() {
if (averageBuilder_ == null) {
averageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>(
getAverage(),
getParentForChildren(),
isClean());
average_ = null;
}
return averageBuilder_;
}
private io.opencannabis.schema.temporal.Duration maximum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder> maximumBuilder_;
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public boolean hasMaximum() {
return maximumBuilder_ != null || maximum_ != null;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public io.opencannabis.schema.temporal.Duration getMaximum() {
if (maximumBuilder_ == null) {
return maximum_ == null ? io.opencannabis.schema.temporal.Duration.getDefaultInstance() : maximum_;
} else {
return maximumBuilder_.getMessage();
}
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public Builder setMaximum(io.opencannabis.schema.temporal.Duration value) {
if (maximumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maximum_ = value;
onChanged();
} else {
maximumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public Builder setMaximum(
io.opencannabis.schema.temporal.Duration.Builder builderForValue) {
if (maximumBuilder_ == null) {
maximum_ = builderForValue.build();
onChanged();
} else {
maximumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public Builder mergeMaximum(io.opencannabis.schema.temporal.Duration value) {
if (maximumBuilder_ == null) {
if (maximum_ != null) {
maximum_ =
io.opencannabis.schema.temporal.Duration.newBuilder(maximum_).mergeFrom(value).buildPartial();
} else {
maximum_ = value;
}
onChanged();
} else {
maximumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public Builder clearMaximum() {
if (maximumBuilder_ == null) {
maximum_ = null;
onChanged();
} else {
maximum_ = null;
maximumBuilder_ = null;
}
return this;
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public io.opencannabis.schema.temporal.Duration.Builder getMaximumBuilder() {
onChanged();
return getMaximumFieldBuilder().getBuilder();
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
public io.opencannabis.schema.temporal.DurationOrBuilder getMaximumOrBuilder() {
if (maximumBuilder_ != null) {
return maximumBuilder_.getMessageOrBuilder();
} else {
return maximum_ == null ?
io.opencannabis.schema.temporal.Duration.getDefaultInstance() : maximum_;
}
}
/**
*
* Specifies a maximum duration for a given time-based metric.
*
*
* .opencannabis.temporal.Duration maximum = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>
getMaximumFieldBuilder() {
if (maximumBuilder_ == null) {
maximumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.Duration, io.opencannabis.schema.temporal.Duration.Builder, io.opencannabis.schema.temporal.DurationOrBuilder>(
getMaximum(),
getParentForChildren(),
isClean());
maximum_ = null;
}
return maximumBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.DurationBoundary)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.DurationBoundary)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DurationBoundary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DurationBoundary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CostBoundaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.Aspect.CostBoundary)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
boolean hasMinimum();
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMinimum();
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMinimumOrBuilder();
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
boolean hasAverage();
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getAverage();
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getAverageOrBuilder();
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
boolean hasMaximum();
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMaximum();
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMaximumOrBuilder();
}
/**
*
* Indicates a cost-based boundary or bucket within which some cannabis use or product resides. This structure may be
* used both with consumption and purchase cost boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CostBoundary}
*/
public static final class CostBoundary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.Aspect.CostBoundary)
CostBoundaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use CostBoundary.newBuilder() to construct.
private CostBoundary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CostBoundary() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CostBoundary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (minimum_ != null) {
subBuilder = minimum_.toBuilder();
}
minimum_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(minimum_);
minimum_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (average_ != null) {
subBuilder = average_.toBuilder();
}
average_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(average_);
average_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (maximum_ != null) {
subBuilder = maximum_.toBuilder();
}
maximum_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(maximum_);
maximum_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder.class);
}
public static final int MINIMUM_FIELD_NUMBER = 1;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue minimum_;
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public boolean hasMinimum() {
return minimum_ != null;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMinimum() {
return minimum_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : minimum_;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMinimumOrBuilder() {
return getMinimum();
}
public static final int AVERAGE_FIELD_NUMBER = 2;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue average_;
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public boolean hasAverage() {
return average_ != null;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getAverage() {
return average_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : average_;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getAverageOrBuilder() {
return getAverage();
}
public static final int MAXIMUM_FIELD_NUMBER = 3;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue maximum_;
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public boolean hasMaximum() {
return maximum_ != null;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMaximum() {
return maximum_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : maximum_;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMaximumOrBuilder() {
return getMaximum();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (minimum_ != null) {
output.writeMessage(1, getMinimum());
}
if (average_ != null) {
output.writeMessage(2, getAverage());
}
if (maximum_ != null) {
output.writeMessage(3, getMaximum());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (minimum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMinimum());
}
if (average_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAverage());
}
if (maximum_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMaximum());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) obj;
if (hasMinimum() != other.hasMinimum()) return false;
if (hasMinimum()) {
if (!getMinimum()
.equals(other.getMinimum())) return false;
}
if (hasAverage() != other.hasAverage()) return false;
if (hasAverage()) {
if (!getAverage()
.equals(other.getAverage())) return false;
}
if (hasMaximum() != other.hasMaximum()) return false;
if (hasMaximum()) {
if (!getMaximum()
.equals(other.getMaximum())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMinimum()) {
hash = (37 * hash) + MINIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMinimum().hashCode();
}
if (hasAverage()) {
hash = (37 * hash) + AVERAGE_FIELD_NUMBER;
hash = (53 * hash) + getAverage().hashCode();
}
if (hasMaximum()) {
hash = (37 * hash) + MAXIMUM_FIELD_NUMBER;
hash = (53 * hash) + getMaximum().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Indicates a cost-based boundary or bucket within which some cannabis use or product resides. This structure may be
* used both with consumption and purchase cost boundaries.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect.CostBoundary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect.CostBoundary)
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (minimumBuilder_ == null) {
minimum_ = null;
} else {
minimum_ = null;
minimumBuilder_ = null;
}
if (averageBuilder_ == null) {
average_ = null;
} else {
average_ = null;
averageBuilder_ = null;
}
if (maximumBuilder_ == null) {
maximum_ = null;
} else {
maximum_ = null;
maximumBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary(this);
if (minimumBuilder_ == null) {
result.minimum_ = minimum_;
} else {
result.minimum_ = minimumBuilder_.build();
}
if (averageBuilder_ == null) {
result.average_ = average_;
} else {
result.average_ = averageBuilder_.build();
}
if (maximumBuilder_ == null) {
result.maximum_ = maximum_;
} else {
result.maximum_ = maximumBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance()) return this;
if (other.hasMinimum()) {
mergeMinimum(other.getMinimum());
}
if (other.hasAverage()) {
mergeAverage(other.getAverage());
}
if (other.hasMaximum()) {
mergeMaximum(other.getMaximum());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue minimum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> minimumBuilder_;
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public boolean hasMinimum() {
return minimumBuilder_ != null || minimum_ != null;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMinimum() {
if (minimumBuilder_ == null) {
return minimum_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : minimum_;
} else {
return minimumBuilder_.getMessage();
}
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public Builder setMinimum(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (minimumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
minimum_ = value;
onChanged();
} else {
minimumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public Builder setMinimum(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (minimumBuilder_ == null) {
minimum_ = builderForValue.build();
onChanged();
} else {
minimumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public Builder mergeMinimum(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (minimumBuilder_ == null) {
if (minimum_ != null) {
minimum_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(minimum_).mergeFrom(value).buildPartial();
} else {
minimum_ = value;
}
onChanged();
} else {
minimumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public Builder clearMinimum() {
if (minimumBuilder_ == null) {
minimum_ = null;
onChanged();
} else {
minimum_ = null;
minimumBuilder_ = null;
}
return this;
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getMinimumBuilder() {
onChanged();
return getMinimumFieldBuilder().getBuilder();
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMinimumOrBuilder() {
if (minimumBuilder_ != null) {
return minimumBuilder_.getMessageOrBuilder();
} else {
return minimum_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : minimum_;
}
}
/**
*
* Specifies a minimum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue minimum = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getMinimumFieldBuilder() {
if (minimumBuilder_ == null) {
minimumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getMinimum(),
getParentForChildren(),
isClean());
minimum_ = null;
}
return minimumBuilder_;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue average_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> averageBuilder_;
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public boolean hasAverage() {
return averageBuilder_ != null || average_ != null;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getAverage() {
if (averageBuilder_ == null) {
return average_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : average_;
} else {
return averageBuilder_.getMessage();
}
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public Builder setAverage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (averageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
average_ = value;
onChanged();
} else {
averageBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public Builder setAverage(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (averageBuilder_ == null) {
average_ = builderForValue.build();
onChanged();
} else {
averageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public Builder mergeAverage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (averageBuilder_ == null) {
if (average_ != null) {
average_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(average_).mergeFrom(value).buildPartial();
} else {
average_ = value;
}
onChanged();
} else {
averageBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public Builder clearAverage() {
if (averageBuilder_ == null) {
average_ = null;
onChanged();
} else {
average_ = null;
averageBuilder_ = null;
}
return this;
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getAverageBuilder() {
onChanged();
return getAverageFieldBuilder().getBuilder();
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getAverageOrBuilder() {
if (averageBuilder_ != null) {
return averageBuilder_.getMessageOrBuilder();
} else {
return average_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : average_;
}
}
/**
*
* Specifies an average cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue average = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getAverageFieldBuilder() {
if (averageBuilder_ == null) {
averageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getAverage(),
getParentForChildren(),
isClean());
average_ = null;
}
return averageBuilder_;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue maximum_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> maximumBuilder_;
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public boolean hasMaximum() {
return maximumBuilder_ != null || maximum_ != null;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getMaximum() {
if (maximumBuilder_ == null) {
return maximum_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : maximum_;
} else {
return maximumBuilder_.getMessage();
}
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public Builder setMaximum(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (maximumBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maximum_ = value;
onChanged();
} else {
maximumBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public Builder setMaximum(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (maximumBuilder_ == null) {
maximum_ = builderForValue.build();
onChanged();
} else {
maximumBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public Builder mergeMaximum(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (maximumBuilder_ == null) {
if (maximum_ != null) {
maximum_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(maximum_).mergeFrom(value).buildPartial();
} else {
maximum_ = value;
}
onChanged();
} else {
maximumBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public Builder clearMaximum() {
if (maximumBuilder_ == null) {
maximum_ = null;
onChanged();
} else {
maximum_ = null;
maximumBuilder_ = null;
}
return this;
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getMaximumBuilder() {
onChanged();
return getMaximumFieldBuilder().getBuilder();
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getMaximumOrBuilder() {
if (maximumBuilder_ != null) {
return maximumBuilder_.getMessageOrBuilder();
} else {
return maximum_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : maximum_;
}
}
/**
*
* Specifies a maximum cost boundary.
*
*
* .opencannabis.commerce.CurrencyValue maximum = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getMaximumFieldBuilder() {
if (maximumBuilder_ == null) {
maximumBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getMaximum(),
getParentForChildren(),
isClean());
maximum_ = null;
}
return maximumBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect.CostBoundary)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect.CostBoundary)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CostBoundary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CostBoundary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int detailCase_ = 0;
private java.lang.Object detail_;
public enum DetailCase
implements com.google.protobuf.Internal.EnumLite {
PRODUCT_CATEGORY(10),
CONSUMPTION_METHOD(11),
COMPOUND(12),
COMPOUND_RATIO(13),
TIMING(14),
LOCATION(15),
DURATION(16),
COST(17),
DETAIL_NOT_SET(0);
private final int value;
private DetailCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DetailCase valueOf(int value) {
return forNumber(value);
}
public static DetailCase forNumber(int value) {
switch (value) {
case 10: return PRODUCT_CATEGORY;
case 11: return CONSUMPTION_METHOD;
case 12: return COMPOUND;
case 13: return COMPOUND_RATIO;
case 14: return TIMING;
case 15: return LOCATION;
case 16: return DURATION;
case 17: return COST;
case 0: return DETAIL_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DetailCase
getDetailCase() {
return DetailCase.forNumber(
detailCase_);
}
public static final int ASPECT_FIELD_NUMBER = 1;
private int aspect_;
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public int getAspectValue() {
return aspect_;
}
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse getAspect() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse result = io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.valueOf(aspect_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.UNRECOGNIZED : result;
}
public static final int PRODUCT_CATEGORY_FIELD_NUMBER = 10;
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public boolean hasProductCategory() {
return detailCase_ == 10;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getProductCategory() {
if (detailCase_ == 10) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder getProductCategoryOrBuilder() {
if (detailCase_ == 10) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
public static final int CONSUMPTION_METHOD_FIELD_NUMBER = 11;
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public boolean hasConsumptionMethod() {
return detailCase_ == 11;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getConsumptionMethod() {
if (detailCase_ == 11) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder getConsumptionMethodOrBuilder() {
if (detailCase_ == 11) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
public static final int COMPOUND_FIELD_NUMBER = 12;
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public boolean hasCompound() {
return detailCase_ == 12;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getCompound() {
if (detailCase_ == 12) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder getCompoundOrBuilder() {
if (detailCase_ == 12) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
public static final int COMPOUND_RATIO_FIELD_NUMBER = 13;
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public boolean hasCompoundRatio() {
return detailCase_ == 13;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getCompoundRatio() {
if (detailCase_ == 13) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder getCompoundRatioOrBuilder() {
if (detailCase_ == 13) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
public static final int TIMING_FIELD_NUMBER = 14;
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public boolean hasTiming() {
return detailCase_ == 14;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getTiming() {
if (detailCase_ == 14) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder getTimingOrBuilder() {
if (detailCase_ == 14) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
public static final int LOCATION_FIELD_NUMBER = 15;
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public boolean hasLocation() {
return detailCase_ == 15;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getLocation() {
if (detailCase_ == 15) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder getLocationOrBuilder() {
if (detailCase_ == 15) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
public static final int DURATION_FIELD_NUMBER = 16;
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public boolean hasDuration() {
return detailCase_ == 16;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDuration() {
if (detailCase_ == 16) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder getDurationOrBuilder() {
if (detailCase_ == 16) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
public static final int COST_FIELD_NUMBER = 17;
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public boolean hasCost() {
return detailCase_ == 17;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getCost() {
if (detailCase_ == 17) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder getCostOrBuilder() {
if (detailCase_ == 17) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (aspect_ != io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.UNKNOWN_ASPECT.getNumber()) {
output.writeEnum(1, aspect_);
}
if (detailCase_ == 10) {
output.writeMessage(10, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_);
}
if (detailCase_ == 11) {
output.writeMessage(11, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_);
}
if (detailCase_ == 12) {
output.writeMessage(12, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_);
}
if (detailCase_ == 13) {
output.writeMessage(13, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_);
}
if (detailCase_ == 14) {
output.writeMessage(14, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_);
}
if (detailCase_ == 15) {
output.writeMessage(15, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_);
}
if (detailCase_ == 16) {
output.writeMessage(16, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_);
}
if (detailCase_ == 17) {
output.writeMessage(17, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (aspect_ != io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.UNKNOWN_ASPECT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, aspect_);
}
if (detailCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_);
}
if (detailCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_);
}
if (detailCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_);
}
if (detailCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_);
}
if (detailCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_);
}
if (detailCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_);
}
if (detailCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_);
}
if (detailCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.Aspect other = (io.bloombox.schema.identity.bioprint.Aspects.Aspect) obj;
if (aspect_ != other.aspect_) return false;
if (!getDetailCase().equals(other.getDetailCase())) return false;
switch (detailCase_) {
case 10:
if (!getProductCategory()
.equals(other.getProductCategory())) return false;
break;
case 11:
if (!getConsumptionMethod()
.equals(other.getConsumptionMethod())) return false;
break;
case 12:
if (!getCompound()
.equals(other.getCompound())) return false;
break;
case 13:
if (!getCompoundRatio()
.equals(other.getCompoundRatio())) return false;
break;
case 14:
if (!getTiming()
.equals(other.getTiming())) return false;
break;
case 15:
if (!getLocation()
.equals(other.getLocation())) return false;
break;
case 16:
if (!getDuration()
.equals(other.getDuration())) return false;
break;
case 17:
if (!getCost()
.equals(other.getCost())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASPECT_FIELD_NUMBER;
hash = (53 * hash) + aspect_;
switch (detailCase_) {
case 10:
hash = (37 * hash) + PRODUCT_CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getProductCategory().hashCode();
break;
case 11:
hash = (37 * hash) + CONSUMPTION_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getConsumptionMethod().hashCode();
break;
case 12:
hash = (37 * hash) + COMPOUND_FIELD_NUMBER;
hash = (53 * hash) + getCompound().hashCode();
break;
case 13:
hash = (37 * hash) + COMPOUND_RATIO_FIELD_NUMBER;
hash = (53 * hash) + getCompoundRatio().hashCode();
break;
case 14:
hash = (37 * hash) + TIMING_FIELD_NUMBER;
hash = (53 * hash) + getTiming().hashCode();
break;
case 15:
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
break;
case 16:
hash = (37 * hash) + DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDuration().hashCode();
break;
case 17:
hash = (37 * hash) + COST_FIELD_NUMBER;
hash = (53 * hash) + getCost().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.Aspect prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes an aspect of cannabis use, by mapping an enumerated aspect to descriptive information concerning that use
* aspect. Aspect detail information can be concretely defined in a message for each aspect, each defined as a sub-
* message here and then mapped in via a protobuf "one-of" field.
*
*
* Protobuf type {@code bloombox.identity.bioprint.Aspect}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.Aspect)
io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.class, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.Aspect.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
aspect_ = 0;
detailCase_ = 0;
detail_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_Aspect_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect build() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.Aspect result = new io.bloombox.schema.identity.bioprint.Aspects.Aspect(this);
result.aspect_ = aspect_;
if (detailCase_ == 10) {
if (productCategoryBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = productCategoryBuilder_.build();
}
}
if (detailCase_ == 11) {
if (consumptionMethodBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = consumptionMethodBuilder_.build();
}
}
if (detailCase_ == 12) {
if (compoundBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = compoundBuilder_.build();
}
}
if (detailCase_ == 13) {
if (compoundRatioBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = compoundRatioBuilder_.build();
}
}
if (detailCase_ == 14) {
if (timingBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = timingBuilder_.build();
}
}
if (detailCase_ == 15) {
if (locationBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = locationBuilder_.build();
}
}
if (detailCase_ == 16) {
if (durationBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = durationBuilder_.build();
}
}
if (detailCase_ == 17) {
if (costBuilder_ == null) {
result.detail_ = detail_;
} else {
result.detail_ = costBuilder_.build();
}
}
result.detailCase_ = detailCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.Aspect) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.Aspect)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.Aspect other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.Aspect.getDefaultInstance()) return this;
if (other.aspect_ != 0) {
setAspectValue(other.getAspectValue());
}
switch (other.getDetailCase()) {
case PRODUCT_CATEGORY: {
mergeProductCategory(other.getProductCategory());
break;
}
case CONSUMPTION_METHOD: {
mergeConsumptionMethod(other.getConsumptionMethod());
break;
}
case COMPOUND: {
mergeCompound(other.getCompound());
break;
}
case COMPOUND_RATIO: {
mergeCompoundRatio(other.getCompoundRatio());
break;
}
case TIMING: {
mergeTiming(other.getTiming());
break;
}
case LOCATION: {
mergeLocation(other.getLocation());
break;
}
case DURATION: {
mergeDuration(other.getDuration());
break;
}
case COST: {
mergeCost(other.getCost());
break;
}
case DETAIL_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.Aspect parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.Aspect) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int detailCase_ = 0;
private java.lang.Object detail_;
public DetailCase
getDetailCase() {
return DetailCase.forNumber(
detailCase_);
}
public Builder clearDetail() {
detailCase_ = 0;
detail_ = null;
onChanged();
return this;
}
private int aspect_ = 0;
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public int getAspectValue() {
return aspect_;
}
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public Builder setAspectValue(int value) {
aspect_ = value;
onChanged();
return this;
}
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse getAspect() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse result = io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.valueOf(aspect_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse.UNRECOGNIZED : result;
}
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public Builder setAspect(io.bloombox.schema.identity.bioprint.Aspects.AspectOfUse value) {
if (value == null) {
throw new NullPointerException();
}
aspect_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the known aspect-of-use described by this aspect record.
*
*
* .bloombox.identity.bioprint.AspectOfUse aspect = 1;
*/
public Builder clearAspect() {
aspect_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder> productCategoryBuilder_;
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public boolean hasProductCategory() {
return detailCase_ == 10;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory getProductCategory() {
if (productCategoryBuilder_ == null) {
if (detailCase_ == 10) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
} else {
if (detailCase_ == 10) {
return productCategoryBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public Builder setProductCategory(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory value) {
if (productCategoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
productCategoryBuilder_.setMessage(value);
}
detailCase_ = 10;
return this;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public Builder setProductCategory(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder builderForValue) {
if (productCategoryBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
productCategoryBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 10;
return this;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public Builder mergeProductCategory(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory value) {
if (productCategoryBuilder_ == null) {
if (detailCase_ == 10 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 10) {
productCategoryBuilder_.mergeFrom(value);
}
productCategoryBuilder_.setMessage(value);
}
detailCase_ = 10;
return this;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public Builder clearProductCategory() {
if (productCategoryBuilder_ == null) {
if (detailCase_ == 10) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 10) {
detailCase_ = 0;
detail_ = null;
}
productCategoryBuilder_.clear();
}
return this;
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder getProductCategoryBuilder() {
return getProductCategoryFieldBuilder().getBuilder();
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder getProductCategoryOrBuilder() {
if ((detailCase_ == 10) && (productCategoryBuilder_ != null)) {
return productCategoryBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 10) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
}
/**
*
* Specifies categorical details about the product, including its main kind and any subcategory, as applicable.
*
*
* .bloombox.identity.bioprint.Aspect.ProductCategory product_category = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder>
getProductCategoryFieldBuilder() {
if (productCategoryBuilder_ == null) {
if (!(detailCase_ == 10)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.getDefaultInstance();
}
productCategoryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategoryOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.ProductCategory) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 10;
onChanged();;
return productCategoryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder> consumptionMethodBuilder_;
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public boolean hasConsumptionMethod() {
return detailCase_ == 11;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption getConsumptionMethod() {
if (consumptionMethodBuilder_ == null) {
if (detailCase_ == 11) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
} else {
if (detailCase_ == 11) {
return consumptionMethodBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public Builder setConsumptionMethod(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption value) {
if (consumptionMethodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
consumptionMethodBuilder_.setMessage(value);
}
detailCase_ = 11;
return this;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public Builder setConsumptionMethod(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder builderForValue) {
if (consumptionMethodBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
consumptionMethodBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 11;
return this;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public Builder mergeConsumptionMethod(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption value) {
if (consumptionMethodBuilder_ == null) {
if (detailCase_ == 11 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 11) {
consumptionMethodBuilder_.mergeFrom(value);
}
consumptionMethodBuilder_.setMessage(value);
}
detailCase_ = 11;
return this;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public Builder clearConsumptionMethod() {
if (consumptionMethodBuilder_ == null) {
if (detailCase_ == 11) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 11) {
detailCase_ = 0;
detail_ = null;
}
consumptionMethodBuilder_.clear();
}
return this;
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder getConsumptionMethodBuilder() {
return getConsumptionMethodFieldBuilder().getBuilder();
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder getConsumptionMethodOrBuilder() {
if ((detailCase_ == 11) && (consumptionMethodBuilder_ != null)) {
return consumptionMethodBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 11) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
}
/**
*
* Indicates information about the method the consumer used to make use of cannabis.
*
*
* .bloombox.identity.bioprint.Aspect.MethodOfConsumption consumption_method = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder>
getConsumptionMethodFieldBuilder() {
if (consumptionMethodBuilder_ == null) {
if (!(detailCase_ == 11)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.getDefaultInstance();
}
consumptionMethodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumptionOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.MethodOfConsumption) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 11;
onChanged();;
return consumptionMethodBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder> compoundBuilder_;
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public boolean hasCompound() {
return detailCase_ == 12;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement getCompound() {
if (compoundBuilder_ == null) {
if (detailCase_ == 12) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
} else {
if (detailCase_ == 12) {
return compoundBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public Builder setCompound(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement value) {
if (compoundBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
compoundBuilder_.setMessage(value);
}
detailCase_ = 12;
return this;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public Builder setCompound(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder builderForValue) {
if (compoundBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
compoundBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 12;
return this;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public Builder mergeCompound(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement value) {
if (compoundBuilder_ == null) {
if (detailCase_ == 12 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 12) {
compoundBuilder_.mergeFrom(value);
}
compoundBuilder_.setMessage(value);
}
detailCase_ = 12;
return this;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public Builder clearCompound() {
if (compoundBuilder_ == null) {
if (detailCase_ == 12) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 12) {
detailCase_ = 0;
detail_ = null;
}
compoundBuilder_.clear();
}
return this;
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder getCompoundBuilder() {
return getCompoundFieldBuilder().getBuilder();
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder getCompoundOrBuilder() {
if ((detailCase_ == 12) && (compoundBuilder_ != null)) {
return compoundBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 12) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
}
/**
*
* Measurement, in rounded form, of some compound found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundMeasurement compound = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder>
getCompoundFieldBuilder() {
if (compoundBuilder_ == null) {
if (!(detailCase_ == 12)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.getDefaultInstance();
}
compoundBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurementOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundMeasurement) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 12;
onChanged();;
return compoundBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder> compoundRatioBuilder_;
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public boolean hasCompoundRatio() {
return detailCase_ == 13;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio getCompoundRatio() {
if (compoundRatioBuilder_ == null) {
if (detailCase_ == 13) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
} else {
if (detailCase_ == 13) {
return compoundRatioBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public Builder setCompoundRatio(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio value) {
if (compoundRatioBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
compoundRatioBuilder_.setMessage(value);
}
detailCase_ = 13;
return this;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public Builder setCompoundRatio(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder builderForValue) {
if (compoundRatioBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
compoundRatioBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 13;
return this;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public Builder mergeCompoundRatio(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio value) {
if (compoundRatioBuilder_ == null) {
if (detailCase_ == 13 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 13) {
compoundRatioBuilder_.mergeFrom(value);
}
compoundRatioBuilder_.setMessage(value);
}
detailCase_ = 13;
return this;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public Builder clearCompoundRatio() {
if (compoundRatioBuilder_ == null) {
if (detailCase_ == 13) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 13) {
detailCase_ = 0;
detail_ = null;
}
compoundRatioBuilder_.clear();
}
return this;
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder getCompoundRatioBuilder() {
return getCompoundRatioFieldBuilder().getBuilder();
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder getCompoundRatioOrBuilder() {
if ((detailCase_ == 13) && (compoundRatioBuilder_ != null)) {
return compoundRatioBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 13) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
}
/**
*
* Measurement, in ratio form, between two compounds found to be part of what constitutes some cannabis product.
*
*
* .bloombox.identity.bioprint.Aspect.CompoundRatio compound_ratio = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder>
getCompoundRatioFieldBuilder() {
if (compoundRatioBuilder_ == null) {
if (!(detailCase_ == 13)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.getDefaultInstance();
}
compoundRatioBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatioOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CompoundRatio) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 13;
onChanged();;
return compoundRatioBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder> timingBuilder_;
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public boolean hasTiming() {
return detailCase_ == 14;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary getTiming() {
if (timingBuilder_ == null) {
if (detailCase_ == 14) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
} else {
if (detailCase_ == 14) {
return timingBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public Builder setTiming(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary value) {
if (timingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
timingBuilder_.setMessage(value);
}
detailCase_ = 14;
return this;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public Builder setTiming(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder builderForValue) {
if (timingBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
timingBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 14;
return this;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public Builder mergeTiming(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary value) {
if (timingBuilder_ == null) {
if (detailCase_ == 14 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 14) {
timingBuilder_.mergeFrom(value);
}
timingBuilder_.setMessage(value);
}
detailCase_ = 14;
return this;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public Builder clearTiming() {
if (timingBuilder_ == null) {
if (detailCase_ == 14) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 14) {
detailCase_ = 0;
detail_ = null;
}
timingBuilder_.clear();
}
return this;
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder getTimingBuilder() {
return getTimingFieldBuilder().getBuilder();
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder getTimingOrBuilder() {
if ((detailCase_ == 14) && (timingBuilder_ != null)) {
return timingBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 14) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information relating to the time of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.TimingBoundary timing = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder>
getTimingFieldBuilder() {
if (timingBuilder_ == null) {
if (!(detailCase_ == 14)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.getDefaultInstance();
}
timingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundaryOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.TimingBoundary) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 14;
onChanged();;
return timingBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder> locationBuilder_;
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public boolean hasLocation() {
return detailCase_ == 15;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary getLocation() {
if (locationBuilder_ == null) {
if (detailCase_ == 15) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
} else {
if (detailCase_ == 15) {
return locationBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public Builder setLocation(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
locationBuilder_.setMessage(value);
}
detailCase_ = 15;
return this;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public Builder setLocation(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder builderForValue) {
if (locationBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
locationBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 15;
return this;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public Builder mergeLocation(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary value) {
if (locationBuilder_ == null) {
if (detailCase_ == 15 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 15) {
locationBuilder_.mergeFrom(value);
}
locationBuilder_.setMessage(value);
}
detailCase_ = 15;
return this;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
if (detailCase_ == 15) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 15) {
detailCase_ = 0;
detail_ = null;
}
locationBuilder_.clear();
}
return this;
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder getLocationBuilder() {
return getLocationFieldBuilder().getBuilder();
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder getLocationOrBuilder() {
if ((detailCase_ == 15) && (locationBuilder_ != null)) {
return locationBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 15) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information related to the location of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.LocationBoundary location = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
if (!(detailCase_ == 15)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.getDefaultInstance();
}
locationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundaryOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.LocationBoundary) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 15;
onChanged();;
return locationBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder> durationBuilder_;
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public boolean hasDuration() {
return detailCase_ == 16;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary getDuration() {
if (durationBuilder_ == null) {
if (detailCase_ == 16) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
} else {
if (detailCase_ == 16) {
return durationBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public Builder setDuration(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary value) {
if (durationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
durationBuilder_.setMessage(value);
}
detailCase_ = 16;
return this;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public Builder setDuration(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder builderForValue) {
if (durationBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
durationBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 16;
return this;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public Builder mergeDuration(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary value) {
if (durationBuilder_ == null) {
if (detailCase_ == 16 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 16) {
durationBuilder_.mergeFrom(value);
}
durationBuilder_.setMessage(value);
}
detailCase_ = 16;
return this;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public Builder clearDuration() {
if (durationBuilder_ == null) {
if (detailCase_ == 16) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 16) {
detailCase_ = 0;
detail_ = null;
}
durationBuilder_.clear();
}
return this;
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder getDurationBuilder() {
return getDurationFieldBuilder().getBuilder();
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder getDurationOrBuilder() {
if ((detailCase_ == 16) && (durationBuilder_ != null)) {
return durationBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 16) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
}
/**
*
* Contextual information related to the duration of consumption or purchase, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.DurationBoundary duration = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder>
getDurationFieldBuilder() {
if (durationBuilder_ == null) {
if (!(detailCase_ == 16)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.getDefaultInstance();
}
durationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundaryOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.DurationBoundary) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 16;
onChanged();;
return durationBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder> costBuilder_;
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public boolean hasCost() {
return detailCase_ == 17;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary getCost() {
if (costBuilder_ == null) {
if (detailCase_ == 17) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
} else {
if (detailCase_ == 17) {
return costBuilder_.getMessage();
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public Builder setCost(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary value) {
if (costBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
detail_ = value;
onChanged();
} else {
costBuilder_.setMessage(value);
}
detailCase_ = 17;
return this;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public Builder setCost(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder builderForValue) {
if (costBuilder_ == null) {
detail_ = builderForValue.build();
onChanged();
} else {
costBuilder_.setMessage(builderForValue.build());
}
detailCase_ = 17;
return this;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public Builder mergeCost(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary value) {
if (costBuilder_ == null) {
if (detailCase_ == 17 &&
detail_ != io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance()) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.newBuilder((io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_)
.mergeFrom(value).buildPartial();
} else {
detail_ = value;
}
onChanged();
} else {
if (detailCase_ == 17) {
costBuilder_.mergeFrom(value);
}
costBuilder_.setMessage(value);
}
detailCase_ = 17;
return this;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public Builder clearCost() {
if (costBuilder_ == null) {
if (detailCase_ == 17) {
detailCase_ = 0;
detail_ = null;
onChanged();
}
} else {
if (detailCase_ == 17) {
detailCase_ = 0;
detail_ = null;
}
costBuilder_.clear();
}
return this;
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder getCostBuilder() {
return getCostFieldBuilder().getBuilder();
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder getCostOrBuilder() {
if ((detailCase_ == 17) && (costBuilder_ != null)) {
return costBuilder_.getMessageOrBuilder();
} else {
if (detailCase_ == 17) {
return (io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_;
}
return io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
}
/**
*
* Indicates cost information, related to the consumption or purchase of cannabis, in exact and bucketed form.
*
*
* .bloombox.identity.bioprint.Aspect.CostBoundary cost = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder>
getCostFieldBuilder() {
if (costBuilder_ == null) {
if (!(detailCase_ == 17)) {
detail_ = io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.getDefaultInstance();
}
costBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary.Builder, io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundaryOrBuilder>(
(io.bloombox.schema.identity.bioprint.Aspects.Aspect.CostBoundary) detail_,
getParentForChildren(),
isClean());
detail_ = null;
}
detailCase_ = 17;
onChanged();;
return costBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.Aspect)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.Aspect)
private static final io.bloombox.schema.identity.bioprint.Aspects.Aspect DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.Aspect();
}
public static io.bloombox.schema.identity.bioprint.Aspects.Aspect getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Aspect parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Aspect(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.Aspect getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AspectGroupOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.bioprint.AspectGroup)
com.google.protobuf.MessageOrBuilder {
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
int getCategoryValue();
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
io.bloombox.schema.identity.bioprint.Aspects.AspectCategory getCategory();
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
java.util.List
getAspectList();
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
io.bloombox.schema.identity.bioprint.Aspects.Aspect getAspect(int index);
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
int getAspectCount();
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
java.util.List extends io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder>
getAspectOrBuilderList();
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder getAspectOrBuilder(
int index);
}
/**
*
* Describes a set of aspects that relate to one another in some manner. For instance, general composition or compound
* specific composition aspects, versus aspects that deal with how or when the user consumed. Aspect groups are another
* opportunity to map user affinities, in this case, in aggregate across any sub-aspects that apply.
*
*
* Protobuf type {@code bloombox.identity.bioprint.AspectGroup}
*/
public static final class AspectGroup extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.bioprint.AspectGroup)
AspectGroupOrBuilder {
private static final long serialVersionUID = 0L;
// Use AspectGroup.newBuilder() to construct.
private AspectGroup(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AspectGroup() {
category_ = 0;
aspect_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AspectGroup(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
category_ = rawValue;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
aspect_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
aspect_.add(
input.readMessage(io.bloombox.schema.identity.bioprint.Aspects.Aspect.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
aspect_ = java.util.Collections.unmodifiableList(aspect_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_AspectGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_AspectGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.class, io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.Builder.class);
}
private int bitField0_;
public static final int CATEGORY_FIELD_NUMBER = 1;
private int category_;
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectCategory getCategory() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.AspectCategory result = io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.valueOf(category_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.UNRECOGNIZED : result;
}
public static final int ASPECT_FIELD_NUMBER = 2;
private java.util.List aspect_;
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public java.util.List getAspectList() {
return aspect_;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public java.util.List extends io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder>
getAspectOrBuilderList() {
return aspect_;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public int getAspectCount() {
return aspect_.size();
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect getAspect(int index) {
return aspect_.get(index);
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder getAspectOrBuilder(
int index) {
return aspect_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (category_ != io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.UNKNOWN_ASPECT_CATEGORY.getNumber()) {
output.writeEnum(1, category_);
}
for (int i = 0; i < aspect_.size(); i++) {
output.writeMessage(2, aspect_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (category_ != io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.UNKNOWN_ASPECT_CATEGORY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, category_);
}
for (int i = 0; i < aspect_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, aspect_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.identity.bioprint.Aspects.AspectGroup)) {
return super.equals(obj);
}
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup other = (io.bloombox.schema.identity.bioprint.Aspects.AspectGroup) obj;
if (category_ != other.category_) return false;
if (!getAspectList()
.equals(other.getAspectList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + category_;
if (getAspectCount() > 0) {
hash = (37 * hash) + ASPECT_FIELD_NUMBER;
hash = (53 * hash) + getAspectList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.identity.bioprint.Aspects.AspectGroup prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a set of aspects that relate to one another in some manner. For instance, general composition or compound
* specific composition aspects, versus aspects that deal with how or when the user consumed. Aspect groups are another
* opportunity to map user affinities, in this case, in aggregate across any sub-aspects that apply.
*
*
* Protobuf type {@code bloombox.identity.bioprint.AspectGroup}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.bioprint.AspectGroup)
io.bloombox.schema.identity.bioprint.Aspects.AspectGroupOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_AspectGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_AspectGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.class, io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.Builder.class);
}
// Construct using io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAspectFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
category_ = 0;
if (aspectBuilder_ == null) {
aspect_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
aspectBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.identity.bioprint.Aspects.internal_static_bloombox_identity_bioprint_AspectGroup_descriptor;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.AspectGroup getDefaultInstanceForType() {
return io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.AspectGroup build() {
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.AspectGroup buildPartial() {
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup result = new io.bloombox.schema.identity.bioprint.Aspects.AspectGroup(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.category_ = category_;
if (aspectBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
aspect_ = java.util.Collections.unmodifiableList(aspect_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.aspect_ = aspect_;
} else {
result.aspect_ = aspectBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.identity.bioprint.Aspects.AspectGroup) {
return mergeFrom((io.bloombox.schema.identity.bioprint.Aspects.AspectGroup)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.identity.bioprint.Aspects.AspectGroup other) {
if (other == io.bloombox.schema.identity.bioprint.Aspects.AspectGroup.getDefaultInstance()) return this;
if (other.category_ != 0) {
setCategoryValue(other.getCategoryValue());
}
if (aspectBuilder_ == null) {
if (!other.aspect_.isEmpty()) {
if (aspect_.isEmpty()) {
aspect_ = other.aspect_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAspectIsMutable();
aspect_.addAll(other.aspect_);
}
onChanged();
}
} else {
if (!other.aspect_.isEmpty()) {
if (aspectBuilder_.isEmpty()) {
aspectBuilder_.dispose();
aspectBuilder_ = null;
aspect_ = other.aspect_;
bitField0_ = (bitField0_ & ~0x00000002);
aspectBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAspectFieldBuilder() : null;
} else {
aspectBuilder_.addAllMessages(other.aspect_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.identity.bioprint.Aspects.AspectGroup parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.identity.bioprint.Aspects.AspectGroup) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int category_ = 0;
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public int getCategoryValue() {
return category_;
}
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public Builder setCategoryValue(int value) {
category_ = value;
onChanged();
return this;
}
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectCategory getCategory() {
@SuppressWarnings("deprecation")
io.bloombox.schema.identity.bioprint.Aspects.AspectCategory result = io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.valueOf(category_);
return result == null ? io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.UNRECOGNIZED : result;
}
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public Builder setCategory(io.bloombox.schema.identity.bioprint.Aspects.AspectCategory value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Category defining this group of aspects. Only one instance of any given category group may be present in a Bioprint
* content payload - i.e., duplicate payloads (by category) are not allowed.
*
*
* .bloombox.identity.bioprint.AspectCategory category = 1;
*/
public Builder clearCategory() {
category_ = 0;
onChanged();
return this;
}
private java.util.List aspect_ =
java.util.Collections.emptyList();
private void ensureAspectIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
aspect_ = new java.util.ArrayList(aspect_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder, io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder> aspectBuilder_;
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public java.util.List getAspectList() {
if (aspectBuilder_ == null) {
return java.util.Collections.unmodifiableList(aspect_);
} else {
return aspectBuilder_.getMessageList();
}
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public int getAspectCount() {
if (aspectBuilder_ == null) {
return aspect_.size();
} else {
return aspectBuilder_.getCount();
}
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect getAspect(int index) {
if (aspectBuilder_ == null) {
return aspect_.get(index);
} else {
return aspectBuilder_.getMessage(index);
}
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder setAspect(
int index, io.bloombox.schema.identity.bioprint.Aspects.Aspect value) {
if (aspectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAspectIsMutable();
aspect_.set(index, value);
onChanged();
} else {
aspectBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder setAspect(
int index, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder builderForValue) {
if (aspectBuilder_ == null) {
ensureAspectIsMutable();
aspect_.set(index, builderForValue.build());
onChanged();
} else {
aspectBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder addAspect(io.bloombox.schema.identity.bioprint.Aspects.Aspect value) {
if (aspectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAspectIsMutable();
aspect_.add(value);
onChanged();
} else {
aspectBuilder_.addMessage(value);
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder addAspect(
int index, io.bloombox.schema.identity.bioprint.Aspects.Aspect value) {
if (aspectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAspectIsMutable();
aspect_.add(index, value);
onChanged();
} else {
aspectBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder addAspect(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder builderForValue) {
if (aspectBuilder_ == null) {
ensureAspectIsMutable();
aspect_.add(builderForValue.build());
onChanged();
} else {
aspectBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder addAspect(
int index, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder builderForValue) {
if (aspectBuilder_ == null) {
ensureAspectIsMutable();
aspect_.add(index, builderForValue.build());
onChanged();
} else {
aspectBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder addAllAspect(
java.lang.Iterable extends io.bloombox.schema.identity.bioprint.Aspects.Aspect> values) {
if (aspectBuilder_ == null) {
ensureAspectIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, aspect_);
onChanged();
} else {
aspectBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder clearAspect() {
if (aspectBuilder_ == null) {
aspect_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
aspectBuilder_.clear();
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public Builder removeAspect(int index) {
if (aspectBuilder_ == null) {
ensureAspectIsMutable();
aspect_.remove(index);
onChanged();
} else {
aspectBuilder_.remove(index);
}
return this;
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder getAspectBuilder(
int index) {
return getAspectFieldBuilder().getBuilder(index);
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder getAspectOrBuilder(
int index) {
if (aspectBuilder_ == null) {
return aspect_.get(index); } else {
return aspectBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public java.util.List extends io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder>
getAspectOrBuilderList() {
if (aspectBuilder_ != null) {
return aspectBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(aspect_);
}
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder addAspectBuilder() {
return getAspectFieldBuilder().addBuilder(
io.bloombox.schema.identity.bioprint.Aspects.Aspect.getDefaultInstance());
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder addAspectBuilder(
int index) {
return getAspectFieldBuilder().addBuilder(
index, io.bloombox.schema.identity.bioprint.Aspects.Aspect.getDefaultInstance());
}
/**
*
* Aspects constituting this aspect group. Structurally, aspect groups contain aspects, with each aspect payload
* constrained to a unique presence in each category (which themselves are unique per-Bioprint). Aspects are grouped
* in such a manner to create meaningful aggregations between individual aspects. Semantically, this relationship
* declares a super-category for a given aspect.
*
*
* repeated .bloombox.identity.bioprint.Aspect aspect = 2;
*/
public java.util.List
getAspectBuilderList() {
return getAspectFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder, io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder>
getAspectFieldBuilder() {
if (aspectBuilder_ == null) {
aspectBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.identity.bioprint.Aspects.Aspect, io.bloombox.schema.identity.bioprint.Aspects.Aspect.Builder, io.bloombox.schema.identity.bioprint.Aspects.AspectOrBuilder>(
aspect_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
aspect_ = null;
}
return aspectBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.bioprint.AspectGroup)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.bioprint.AspectGroup)
private static final io.bloombox.schema.identity.bioprint.Aspects.AspectGroup DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.identity.bioprint.Aspects.AspectGroup();
}
public static io.bloombox.schema.identity.bioprint.Aspects.AspectGroup getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AspectGroup parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AspectGroup(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.identity.bioprint.Aspects.AspectGroup getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ASPECT_GROUP_FIELD_NUMBER = 8005;
/**
*
* Describes the aspect group for a given aspect-of-use.
*
*
* extend .google.protobuf.EnumValueOptions { ... }
*/
public static final
com.google.protobuf.GeneratedMessage.GeneratedExtension<
com.google.protobuf.DescriptorProtos.EnumValueOptions,
io.bloombox.schema.identity.bioprint.Aspects.AspectCategory> aspectGroup = com.google.protobuf.GeneratedMessage
.newFileScopedGeneratedExtension(
io.bloombox.schema.identity.bioprint.Aspects.AspectCategory.class,
null);
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_bioprint_AspectGroup_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_bioprint_AspectGroup_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\037identity/bioprint/Aspects.proto\022\032bloom" +
"box.identity.bioprint\032 google/protobuf/d" +
"escriptor.proto\032\024core/Datamodel.proto\032\026b" +
"ase/ProductKind.proto\032\021geo/Geohash.proto" +
"\032\026temporal/Instant.proto\032\027temporal/Durat" +
"ion.proto\032\027temporal/Timehash.proto\032\027temp" +
"oral/Interval.proto\032\027commerce/Currency.p" +
"roto\032\031products/Apothecary.proto\032\030product" +
"s/Cartridge.proto\032\025products/Edible.proto" +
"\032\026products/Extract.proto\032\024products/Plant" +
".proto\032\"structs/labtesting/TestValue.pro" +
"to\032$structs/labtesting/TestResults.proto" +
"\032\'analytics/consumption/Biodelivery.prot" +
"o\"\361\025\n\006Aspect\0227\n\006aspect\030\001 \001(\0162\'.bloombox." +
"identity.bioprint.AspectOfUse\022N\n\020product" +
"_category\030\n \001(\01322.bloombox.identity.biop" +
"rint.Aspect.ProductCategoryH\000\022T\n\022consump" +
"tion_method\030\013 \001(\01326.bloombox.identity.bi" +
"oprint.Aspect.MethodOfConsumptionH\000\022J\n\010c" +
"ompound\030\014 \001(\01326.bloombox.identity.biopri" +
"nt.Aspect.CompoundMeasurementH\000\022J\n\016compo" +
"und_ratio\030\r \001(\01320.bloombox.identity.biop" +
"rint.Aspect.CompoundRatioH\000\022C\n\006timing\030\016 " +
"\001(\01321.bloombox.identity.bioprint.Aspect." +
"TimingBoundaryH\000\022G\n\010location\030\017 \001(\01323.blo" +
"ombox.identity.bioprint.Aspect.LocationB" +
"oundaryH\000\022G\n\010duration\030\020 \001(\01323.bloombox.i" +
"dentity.bioprint.Aspect.DurationBoundary" +
"H\000\022?\n\004cost\030\021 \001(\0132/.bloombox.identity.bio" +
"print.Aspect.CostBoundaryH\000\032\345\002\n\017ProductC" +
"ategory\022,\n\004kind\030\001 \001(\0162\036.opencannabis.bas" +
"e.ProductKind\022;\n\napothecary\030\n \001(\0162%.open" +
"cannabis.products.ApothecaryTypeH\000\0229\n\tca" +
"rtridge\030\013 \001(\0162$.opencannabis.products.Ca" +
"rtridgeTypeH\000\0223\n\006edible\030\014 \001(\0162!.opencann" +
"abis.products.EdibleTypeH\000\0225\n\007extract\030\r " +
"\001(\0162\".opencannabis.products.ExtractTypeH" +
"\000\0221\n\005plant\030\016 \001(\0162 .opencannabis.products" +
".PlantTypeH\000B\r\n\013subcategory\032R\n\023MethodOfC" +
"onsumption\022;\n\014bio_delivery\030\001 \001(\0132%.bloom" +
"box.consumption.BiodeliveryInfo\032\220\004\n\023Comp" +
"oundMeasurement\022;\n\007minimum\030\001 \001(\0132*.openc" +
"annabis.structs.labtesting.TestValue\022;\n\007" +
"average\030\002 \001(\0132*.opencannabis.structs.lab" +
"testing.TestValue\022;\n\007maximum\030\003 \001(\0132*.ope" +
"ncannabis.structs.labtesting.TestValue\022;" +
"\n\007rounded\030\004 \001(\0132*.opencannabis.structs.l" +
"abtesting.TestValue\022\020\n\010presence\030\005 \001(\010\022C\n" +
"\013cannabinoid\030\n \001(\0162,.opencannabis.struct" +
"s.labtesting.CannabinoidH\000\022;\n\007terpene\030\013 " +
"\001(\0162(.opencannabis.structs.labtesting.Te" +
"rpeneH\000\"e\n\014CompoundType\022\017\n\013CANNABINOID\020\000" +
"\022\013\n\007TERPENE\020\001\022\r\n\tPESTICIDE\020\002\022\t\n\005METAL\020\003\022" +
"\017\n\013MOLD_MILDEW\020\004\022\014\n\010MOISTURE\020\005B\n\n\010compou" +
"nd\032\342\001\n\032BoundedCompoundMeasurement\022\\\n\006buc" +
"ket\030\001 \001(\0162L.bloombox.identity.bioprint.A" +
"spect.BoundedCompoundMeasurement.Compoun" +
"dBucket\"f\n\016CompoundBucket\022\017\n\013NOT_PRESENT" +
"\020\000\022\t\n\005TRACE\020\001\022\013\n\007MINIMAL\020\002\022\014\n\010STANDARD\020\003" +
"\022\017\n\013SIGNIFICANT\020\004\022\014\n\010DOMINANT\020\005\032\330\002\n\rComp" +
"oundRatio\022K\n\004left\030\001 \001(\0132=.bloombox.ident" +
"ity.bioprint.Aspect.CompoundRatio.RatioP" +
"ortion\022L\n\005right\030\002 \001(\0132=.bloombox.identit" +
"y.bioprint.Aspect.CompoundRatio.RatioPor" +
"tion\032\253\001\n\014RatioPortion\022\r\n\005value\030\001 \001(\r\022C\n\013" +
"cannabinoid\030\n \001(\0162,.opencannabis.structs" +
".labtesting.CannabinoidH\000\022;\n\007terpene\030\013 \001" +
"(\0162(.opencannabis.structs.labtesting.Ter" +
"peneH\000B\n\n\010compound\032\240\001\n\016TimingBoundary\022.\n" +
"\005first\030\001 \001(\0132\037.opencannabis.temporal.Tim" +
"ehash\022/\n\006latest\030\002 \001(\0132\037.opencannabis.tem" +
"poral.Timehash\022-\n\004area\030\003 \001(\0132\037.opencanna" +
"bis.temporal.Timehash\032;\n\020LocationBoundar" +
"y\022\'\n\004area\030\001 \001(\0132\031.opencannabis.geo.Geoha" +
"sh\032\250\001\n\020DurationBoundary\0220\n\007minimum\030\001 \001(\013" +
"2\037.opencannabis.temporal.Duration\0220\n\007ave" +
"rage\030\002 \001(\0132\037.opencannabis.temporal.Durat" +
"ion\0220\n\007maximum\030\003 \001(\0132\037.opencannabis.temp" +
"oral.Duration\032\263\001\n\014CostBoundary\0225\n\007minimu" +
"m\030\001 \001(\0132$.opencannabis.commerce.Currency" +
"Value\0225\n\007average\030\002 \001(\0132$.opencannabis.co" +
"mmerce.CurrencyValue\0225\n\007maximum\030\003 \001(\0132$." +
"opencannabis.commerce.CurrencyValueB\010\n\006d" +
"etail\"\177\n\013AspectGroup\022<\n\010category\030\001 \001(\0162*" +
".bloombox.identity.bioprint.AspectCatego" +
"ry\0222\n\006aspect\030\002 \003(\0132\".bloombox.identity.b" +
"ioprint.Aspect*\307\001\n\016AspectCategory\022\033\n\027UNK" +
"NOWN_ASPECT_CATEGORY\020\000\022 \n\013CATEGORICAL\020\001\032" +
"\017\232\364\003\013Categorical\022$\n\rCOMPOSITIONAL\020\002\032\021\232\364\003" +
"\rCompositional\022\032\n\010PURCHASE\020\003\032\014\232\364\003\010Purcha" +
"se\022 \n\013CONSUMPTION\020\004\032\017\232\364\003\013Consumption\022\022\n\004" +
"COST\020\005\032\010\232\364\003\004Cost*\207\007\n\013AspectOfUse\022\022\n\016UNKN" +
"OWN_ASPECT\020\000\022.\n\020PRODUCT_CATEGORY\020\001\032\030\250\364\003\001" +
"\232\364\003\020Product Category\0228\n\025METHOD_OF_CONSUM" +
"PTION\020\002\032\035\250\364\003\004\232\364\003\025Method of Consumption\0222" +
"\n\022CANNABINOID_AMOUNT\020\003\032\032\250\364\003\002\232\364\003\022Cannabin" +
"oid Amount\022C\n\032CANNABINOID_AMOUNT_BOUNDED" +
"\020\004\032#\250\364\003\002\232\364\003\033Cannabinoid Amount: Bounded\022" +
"0\n\021CANNABINOID_RATIO\020\005\032\031\250\364\003\002\232\364\003\021Cannabin" +
"oid Ratio\022A\n\031CANNABINOID_RATIO_BOUNDED\020\006" +
"\032\"\250\364\003\002\232\364\003\032Cannabinoid Ratio: Bounded\022*\n\016" +
"TERPENE_AMOUNT\020\007\032\026\250\364\003\002\232\364\003\016Terpene Amount" +
"\022(\n\rTERPENE_RATIO\020\010\032\025\250\364\003\002\232\364\003\rTerpene Rat" +
"io\0226\n\024MATERIAL_COMPOSITION\020\t\032\034\250\364\003\002\232\364\003\024Ma" +
"terial Composition\022.\n\020TIME_OF_PURCHASE\020\n" +
"\032\030\250\364\003\003\232\364\003\020Time of Purchase\0224\n\023TIME_OF_CO" +
"NSUMPTION\020\013\032\033\250\364\003\004\232\364\003\023Time of Consumption" +
"\0226\n\024LOCATION_OF_PURCHASE\020\014\032\034\250\364\003\003\232\364\003\024Loca" +
"tion of Purchase\022<\n\027LOCATION_OF_CONSUMPT" +
"ION\020\r\032\037\250\364\003\004\232\364\003\027Location of Consumption\022<" +
"\n\027DURATION_OF_CONSUMPTION\020\016\032\037\250\364\003\005\232\364\003\027Dur" +
"ation of Consumption\0224\n\023COST_OF_CONSUMPT" +
"ION\020\017\032\033\250\364\003\005\232\364\003\023Cost of Consumption\022.\n\020CO" +
"ST_OF_PURCHASE\020\020\032\030\250\364\003\005\232\364\003\020Cost of Purcha" +
"se:d\n\014aspect_group\022!.google.protobuf.Enu" +
"mValueOptions\030\305> \001(\0162*.bloombox.identity" +
".bioprint.AspectCategoryB9\n$io.bloombox." +
"schema.identity.bioprintB\007AspectsH\001P\000\242\002\003" +
"BBSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.DescriptorProtos.getDescriptor(),
core.Datamodel.getDescriptor(),
io.opencannabis.schema.base.BaseProductKind.getDescriptor(),
io.opencannabis.schema.geo.GeohashOuterClass.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
io.opencannabis.schema.temporal.DurationOuterClass.getDescriptor(),
io.opencannabis.schema.temporal.TimehashOuterClass.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInterval.getDescriptor(),
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor(),
io.opencannabis.schema.product.ApothecaryProduct.getDescriptor(),
io.opencannabis.schema.product.CartridgeProduct.getDescriptor(),
io.opencannabis.schema.product.EdibleProduct.getDescriptor(),
io.opencannabis.schema.product.ExtractProduct.getDescriptor(),
io.opencannabis.schema.product.PlantProduct.getDescriptor(),
io.opencannabis.schema.product.struct.testing.BaseTestingSpec.getDescriptor(),
io.opencannabis.schema.product.struct.testing.LabTesting.getDescriptor(),
io.bloombox.schema.consumption.Biodelivery.getDescriptor(),
}, assigner);
internal_static_bloombox_identity_bioprint_Aspect_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_identity_bioprint_Aspect_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_descriptor,
new java.lang.String[] { "Aspect", "ProductCategory", "ConsumptionMethod", "Compound", "CompoundRatio", "Timing", "Location", "Duration", "Cost", "Detail", });
internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(0);
internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_ProductCategory_descriptor,
new java.lang.String[] { "Kind", "Apothecary", "Cartridge", "Edible", "Extract", "Plant", "Subcategory", });
internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(1);
internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_MethodOfConsumption_descriptor,
new java.lang.String[] { "BioDelivery", });
internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(2);
internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_CompoundMeasurement_descriptor,
new java.lang.String[] { "Minimum", "Average", "Maximum", "Rounded", "Presence", "Cannabinoid", "Terpene", "Compound", });
internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(3);
internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_BoundedCompoundMeasurement_descriptor,
new java.lang.String[] { "Bucket", });
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(4);
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor,
new java.lang.String[] { "Left", "Right", });
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_descriptor.getNestedTypes().get(0);
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_CompoundRatio_RatioPortion_descriptor,
new java.lang.String[] { "Value", "Cannabinoid", "Terpene", "Compound", });
internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(5);
internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_TimingBoundary_descriptor,
new java.lang.String[] { "First", "Latest", "Area", });
internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(6);
internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_LocationBoundary_descriptor,
new java.lang.String[] { "Area", });
internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(7);
internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_DurationBoundary_descriptor,
new java.lang.String[] { "Minimum", "Average", "Maximum", });
internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor =
internal_static_bloombox_identity_bioprint_Aspect_descriptor.getNestedTypes().get(8);
internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_Aspect_CostBoundary_descriptor,
new java.lang.String[] { "Minimum", "Average", "Maximum", });
internal_static_bloombox_identity_bioprint_AspectGroup_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_identity_bioprint_AspectGroup_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_bioprint_AspectGroup_descriptor,
new java.lang.String[] { "Category", "Aspect", });
aspectGroup.internalInit(descriptor.getExtensions().get(0));
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(io.bloombox.schema.identity.bioprint.Aspects.aspectGroup);
registry.add(core.Datamodel.label);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.DescriptorProtos.getDescriptor();
core.Datamodel.getDescriptor();
io.opencannabis.schema.base.BaseProductKind.getDescriptor();
io.opencannabis.schema.geo.GeohashOuterClass.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
io.opencannabis.schema.temporal.DurationOuterClass.getDescriptor();
io.opencannabis.schema.temporal.TimehashOuterClass.getDescriptor();
io.opencannabis.schema.temporal.TemporalInterval.getDescriptor();
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor();
io.opencannabis.schema.product.ApothecaryProduct.getDescriptor();
io.opencannabis.schema.product.CartridgeProduct.getDescriptor();
io.opencannabis.schema.product.EdibleProduct.getDescriptor();
io.opencannabis.schema.product.ExtractProduct.getDescriptor();
io.opencannabis.schema.product.PlantProduct.getDescriptor();
io.opencannabis.schema.product.struct.testing.BaseTestingSpec.getDescriptor();
io.opencannabis.schema.product.struct.testing.LabTesting.getDescriptor();
io.bloombox.schema.consumption.Biodelivery.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy