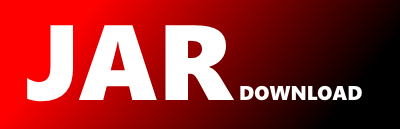
io.bloombox.schema.ledger.Accounts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ledger/Account.proto
package io.bloombox.schema.ledger;
public final class Accounts {
private Accounts() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Enumerates special actors on the ledger. These entries are considered system-level accounts, for various purposes,
* with access in limited capacities.
*
*
* Protobuf enum {@code bloombox.ledger.SpecialActor}
*/
public enum SpecialActor
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Built-in system account.
*
*
* SYSTEM = 0;
*/
SYSTEM(0),
/**
*
* Sandbox system account.
*
*
* SANDBOX = 1;
*/
SANDBOX(1),
UNRECOGNIZED(-1),
;
/**
*
* Built-in system account.
*
*
* SYSTEM = 0;
*/
public static final int SYSTEM_VALUE = 0;
/**
*
* Sandbox system account.
*
*
* SANDBOX = 1;
*/
public static final int SANDBOX_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SpecialActor valueOf(int value) {
return forNumber(value);
}
public static SpecialActor forNumber(int value) {
switch (value) {
case 0: return SYSTEM;
case 1: return SANDBOX;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SpecialActor> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SpecialActor findValueByNumber(int number) {
return SpecialActor.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.getDescriptor().getEnumTypes().get(0);
}
private static final SpecialActor[] VALUES = values();
public static SpecialActor valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SpecialActor(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.ledger.SpecialActor)
}
public interface ActorCertificateOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.ActorCertificate)
com.google.protobuf.MessageOrBuilder {
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
java.lang.String getId();
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
java.lang.String getSerial();
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
com.google.protobuf.ByteString
getSerialBytes();
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
boolean hasFingerprint();
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint();
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder();
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
java.lang.String getCommonName();
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
com.google.protobuf.ByteString
getCommonNameBytes();
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
java.lang.String getIssuerName();
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
com.google.protobuf.ByteString
getIssuerNameBytes();
/**
*
* Raw certificate information, in X509 format.
*
*
* bytes data = 10;
*/
com.google.protobuf.ByteString getData();
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
java.lang.String getEncoded();
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
com.google.protobuf.ByteString
getEncodedBytes();
public io.bloombox.schema.ledger.Accounts.ActorCertificate.PayloadCase getPayloadCase();
}
/**
*
* Certificate data accompanying an actor key. This is a certificate bound to the ECDSA keypair issued in parallel to
* the actor's ledger key, and certified by the ledger actor CA.
*
*
* Protobuf type {@code bloombox.ledger.ActorCertificate}
*/
public static final class ActorCertificate extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.ActorCertificate)
ActorCertificateOrBuilder {
private static final long serialVersionUID = 0L;
// Use ActorCertificate.newBuilder() to construct.
private ActorCertificate(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ActorCertificate() {
id_ = "";
serial_ = "";
commonName_ = "";
issuerName_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ActorCertificate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
serial_ = s;
break;
}
case 26: {
io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder subBuilder = null;
if (fingerprint_ != null) {
subBuilder = fingerprint_.toBuilder();
}
fingerprint_ = input.readMessage(io.opencannabis.schema.crypto.primitives.integrity.Hash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fingerprint_);
fingerprint_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
commonName_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
issuerName_ = s;
break;
}
case 82: {
payloadCase_ = 10;
payload_ = input.readBytes();
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
payloadCase_ = 11;
payload_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorCertificate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorCertificate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.ActorCertificate.class, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder.class);
}
private int payloadCase_ = 0;
private java.lang.Object payload_;
public enum PayloadCase
implements com.google.protobuf.Internal.EnumLite {
DATA(10),
ENCODED(11),
PAYLOAD_NOT_SET(0);
private final int value;
private PayloadCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PayloadCase valueOf(int value) {
return forNumber(value);
}
public static PayloadCase forNumber(int value) {
switch (value) {
case 10: return DATA;
case 11: return ENCODED;
case 0: return PAYLOAD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PayloadCase
getPayloadCase() {
return PayloadCase.forNumber(
payloadCase_);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERIAL_FIELD_NUMBER = 2;
private volatile java.lang.Object serial_;
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public java.lang.String getSerial() {
java.lang.Object ref = serial_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serial_ = s;
return s;
}
}
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public com.google.protobuf.ByteString
getSerialBytes() {
java.lang.Object ref = serial_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serial_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FINGERPRINT_FIELD_NUMBER = 3;
private io.opencannabis.schema.crypto.primitives.integrity.Hash fingerprint_;
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public boolean hasFingerprint() {
return fingerprint_ != null;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint() {
return fingerprint_ == null ? io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder() {
return getFingerprint();
}
public static final int COMMON_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object commonName_;
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public java.lang.String getCommonName() {
java.lang.Object ref = commonName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commonName_ = s;
return s;
}
}
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public com.google.protobuf.ByteString
getCommonNameBytes() {
java.lang.Object ref = commonName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commonName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISSUER_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object issuerName_;
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public java.lang.String getIssuerName() {
java.lang.Object ref = issuerName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
issuerName_ = s;
return s;
}
}
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public com.google.protobuf.ByteString
getIssuerNameBytes() {
java.lang.Object ref = issuerName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
issuerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 10;
/**
*
* Raw certificate information, in X509 format.
*
*
* bytes data = 10;
*/
public com.google.protobuf.ByteString getData() {
if (payloadCase_ == 10) {
return (com.google.protobuf.ByteString) payload_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int ENCODED_FIELD_NUMBER = 11;
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public java.lang.String getEncoded() {
java.lang.Object ref = "";
if (payloadCase_ == 11) {
ref = payload_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (payloadCase_ == 11) {
payload_ = s;
}
return s;
}
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public com.google.protobuf.ByteString
getEncodedBytes() {
java.lang.Object ref = "";
if (payloadCase_ == 11) {
ref = payload_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (payloadCase_ == 11) {
payload_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getSerialBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, serial_);
}
if (fingerprint_ != null) {
output.writeMessage(3, getFingerprint());
}
if (!getCommonNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, commonName_);
}
if (!getIssuerNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, issuerName_);
}
if (payloadCase_ == 10) {
output.writeBytes(
10, (com.google.protobuf.ByteString) payload_);
}
if (payloadCase_ == 11) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, payload_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getSerialBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, serial_);
}
if (fingerprint_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getFingerprint());
}
if (!getCommonNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, commonName_);
}
if (!getIssuerNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, issuerName_);
}
if (payloadCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
10, (com.google.protobuf.ByteString) payload_);
}
if (payloadCase_ == 11) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, payload_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.ActorCertificate)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.ActorCertificate other = (io.bloombox.schema.ledger.Accounts.ActorCertificate) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getSerial()
.equals(other.getSerial())) return false;
if (hasFingerprint() != other.hasFingerprint()) return false;
if (hasFingerprint()) {
if (!getFingerprint()
.equals(other.getFingerprint())) return false;
}
if (!getCommonName()
.equals(other.getCommonName())) return false;
if (!getIssuerName()
.equals(other.getIssuerName())) return false;
if (!getPayloadCase().equals(other.getPayloadCase())) return false;
switch (payloadCase_) {
case 10:
if (!getData()
.equals(other.getData())) return false;
break;
case 11:
if (!getEncoded()
.equals(other.getEncoded())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + SERIAL_FIELD_NUMBER;
hash = (53 * hash) + getSerial().hashCode();
if (hasFingerprint()) {
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
}
hash = (37 * hash) + COMMON_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCommonName().hashCode();
hash = (37 * hash) + ISSUER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getIssuerName().hashCode();
switch (payloadCase_) {
case 10:
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
break;
case 11:
hash = (37 * hash) + ENCODED_FIELD_NUMBER;
hash = (53 * hash) + getEncoded().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.ActorCertificate prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Certificate data accompanying an actor key. This is a certificate bound to the ECDSA keypair issued in parallel to
* the actor's ledger key, and certified by the ledger actor CA.
*
*
* Protobuf type {@code bloombox.ledger.ActorCertificate}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.ActorCertificate)
io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorCertificate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorCertificate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.ActorCertificate.class, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.ActorCertificate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
serial_ = "";
if (fingerprintBuilder_ == null) {
fingerprint_ = null;
} else {
fingerprint_ = null;
fingerprintBuilder_ = null;
}
commonName_ = "";
issuerName_ = "";
payloadCase_ = 0;
payload_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorCertificate_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorCertificate getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorCertificate build() {
io.bloombox.schema.ledger.Accounts.ActorCertificate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorCertificate buildPartial() {
io.bloombox.schema.ledger.Accounts.ActorCertificate result = new io.bloombox.schema.ledger.Accounts.ActorCertificate(this);
result.id_ = id_;
result.serial_ = serial_;
if (fingerprintBuilder_ == null) {
result.fingerprint_ = fingerprint_;
} else {
result.fingerprint_ = fingerprintBuilder_.build();
}
result.commonName_ = commonName_;
result.issuerName_ = issuerName_;
if (payloadCase_ == 10) {
result.payload_ = payload_;
}
if (payloadCase_ == 11) {
result.payload_ = payload_;
}
result.payloadCase_ = payloadCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.ActorCertificate) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.ActorCertificate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.ActorCertificate other) {
if (other == io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getSerial().isEmpty()) {
serial_ = other.serial_;
onChanged();
}
if (other.hasFingerprint()) {
mergeFingerprint(other.getFingerprint());
}
if (!other.getCommonName().isEmpty()) {
commonName_ = other.commonName_;
onChanged();
}
if (!other.getIssuerName().isEmpty()) {
issuerName_ = other.issuerName_;
onChanged();
}
switch (other.getPayloadCase()) {
case DATA: {
setData(other.getData());
break;
}
case ENCODED: {
payloadCase_ = 11;
payload_ = other.payload_;
onChanged();
break;
}
case PAYLOAD_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.ActorCertificate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.ActorCertificate) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int payloadCase_ = 0;
private java.lang.Object payload_;
public PayloadCase
getPayloadCase() {
return PayloadCase.forNumber(
payloadCase_);
}
public Builder clearPayload() {
payloadCase_ = 0;
payload_ = null;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* ID of the certificate.
*
*
* string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object serial_ = "";
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public java.lang.String getSerial() {
java.lang.Object ref = serial_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serial_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public com.google.protobuf.ByteString
getSerialBytes() {
java.lang.Object ref = serial_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serial_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public Builder setSerial(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serial_ = value;
onChanged();
return this;
}
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public Builder clearSerial() {
serial_ = getDefaultInstance().getSerial();
onChanged();
return this;
}
/**
*
* Serial number of the certificate.
*
*
* string serial = 2;
*/
public Builder setSerialBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serial_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.crypto.primitives.integrity.Hash fingerprint_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder> fingerprintBuilder_;
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public boolean hasFingerprint() {
return fingerprintBuilder_ != null || fingerprint_ != null;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint() {
if (fingerprintBuilder_ == null) {
return fingerprint_ == null ? io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
} else {
return fingerprintBuilder_.getMessage();
}
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public Builder setFingerprint(io.opencannabis.schema.crypto.primitives.integrity.Hash value) {
if (fingerprintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
onChanged();
} else {
fingerprintBuilder_.setMessage(value);
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public Builder setFingerprint(
io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder builderForValue) {
if (fingerprintBuilder_ == null) {
fingerprint_ = builderForValue.build();
onChanged();
} else {
fingerprintBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public Builder mergeFingerprint(io.opencannabis.schema.crypto.primitives.integrity.Hash value) {
if (fingerprintBuilder_ == null) {
if (fingerprint_ != null) {
fingerprint_ =
io.opencannabis.schema.crypto.primitives.integrity.Hash.newBuilder(fingerprint_).mergeFrom(value).buildPartial();
} else {
fingerprint_ = value;
}
onChanged();
} else {
fingerprintBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public Builder clearFingerprint() {
if (fingerprintBuilder_ == null) {
fingerprint_ = null;
onChanged();
} else {
fingerprint_ = null;
fingerprintBuilder_ = null;
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder getFingerprintBuilder() {
onChanged();
return getFingerprintFieldBuilder().getBuilder();
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
public io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder() {
if (fingerprintBuilder_ != null) {
return fingerprintBuilder_.getMessageOrBuilder();
} else {
return fingerprint_ == null ?
io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
}
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder>
getFingerprintFieldBuilder() {
if (fingerprintBuilder_ == null) {
fingerprintBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder>(
getFingerprint(),
getParentForChildren(),
isClean());
fingerprint_ = null;
}
return fingerprintBuilder_;
}
private java.lang.Object commonName_ = "";
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public java.lang.String getCommonName() {
java.lang.Object ref = commonName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commonName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public com.google.protobuf.ByteString
getCommonNameBytes() {
java.lang.Object ref = commonName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commonName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public Builder setCommonName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
commonName_ = value;
onChanged();
return this;
}
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public Builder clearCommonName() {
commonName_ = getDefaultInstance().getCommonName();
onChanged();
return this;
}
/**
*
* Certificate common name.
*
*
* string common_name = 4;
*/
public Builder setCommonNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
commonName_ = value;
onChanged();
return this;
}
private java.lang.Object issuerName_ = "";
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public java.lang.String getIssuerName() {
java.lang.Object ref = issuerName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
issuerName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public com.google.protobuf.ByteString
getIssuerNameBytes() {
java.lang.Object ref = issuerName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
issuerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public Builder setIssuerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
issuerName_ = value;
onChanged();
return this;
}
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public Builder clearIssuerName() {
issuerName_ = getDefaultInstance().getIssuerName();
onChanged();
return this;
}
/**
*
* Certificate issuer name.
*
*
* string issuer_name = 5;
*/
public Builder setIssuerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
issuerName_ = value;
onChanged();
return this;
}
/**
*
* Raw certificate information, in X509 format.
*
*
* bytes data = 10;
*/
public com.google.protobuf.ByteString getData() {
if (payloadCase_ == 10) {
return (com.google.protobuf.ByteString) payload_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
*
* Raw certificate information, in X509 format.
*
*
* bytes data = 10;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
payloadCase_ = 10;
payload_ = value;
onChanged();
return this;
}
/**
*
* Raw certificate information, in X509 format.
*
*
* bytes data = 10;
*/
public Builder clearData() {
if (payloadCase_ == 10) {
payloadCase_ = 0;
payload_ = null;
onChanged();
}
return this;
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public java.lang.String getEncoded() {
java.lang.Object ref = "";
if (payloadCase_ == 11) {
ref = payload_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (payloadCase_ == 11) {
payload_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public com.google.protobuf.ByteString
getEncodedBytes() {
java.lang.Object ref = "";
if (payloadCase_ == 11) {
ref = payload_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (payloadCase_ == 11) {
payload_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public Builder setEncoded(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
payloadCase_ = 11;
payload_ = value;
onChanged();
return this;
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public Builder clearEncoded() {
if (payloadCase_ == 11) {
payloadCase_ = 0;
payload_ = null;
onChanged();
}
return this;
}
/**
*
* Base64-encoded certificate data.
*
*
* string encoded = 11;
*/
public Builder setEncodedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
payloadCase_ = 11;
payload_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.ActorCertificate)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.ActorCertificate)
private static final io.bloombox.schema.ledger.Accounts.ActorCertificate DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.ActorCertificate();
}
public static io.bloombox.schema.ledger.Accounts.ActorCertificate getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ActorCertificate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ActorCertificate(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorCertificate getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ActorKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.ActorKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
int getKnownValue();
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
io.bloombox.schema.ledger.Accounts.SpecialActor getKnown();
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
boolean hasUser();
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
io.bloombox.schema.identity.AppUserKey.UserKey getUser();
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder();
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
boolean hasPartner();
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
io.bloombox.schema.partner.PartnerMeta.PartnerKey getPartner();
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder getPartnerOrBuilder();
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
boolean hasLocation();
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
io.bloombox.schema.partner.LocationAccountKey.LocationKey getLocation();
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder getLocationOrBuilder();
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
boolean hasDevice();
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey getDevice();
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder getDeviceOrBuilder();
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
boolean hasNode();
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
io.bloombox.schema.ledger.LedgerNode.Node getNode();
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder getNodeOrBuilder();
public io.bloombox.schema.ledger.Accounts.ActorKey.ActorCase getActorCase();
}
/**
*
* Specifies the concept of a ledger "actor," which unifies individuals and organizations under one referential
* structure, such that either may easily be the basis for a ledger transaction.
*
*
* Protobuf type {@code bloombox.ledger.ActorKey}
*/
public static final class ActorKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.ActorKey)
ActorKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use ActorKey.newBuilder() to construct.
private ActorKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ActorKey() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ActorKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
actorCase_ = 1;
actor_ = rawValue;
break;
}
case 82: {
io.bloombox.schema.identity.AppUserKey.UserKey.Builder subBuilder = null;
if (actorCase_ == 10) {
subBuilder = ((io.bloombox.schema.identity.AppUserKey.UserKey) actor_).toBuilder();
}
actor_ =
input.readMessage(io.bloombox.schema.identity.AppUserKey.UserKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.identity.AppUserKey.UserKey) actor_);
actor_ = subBuilder.buildPartial();
}
actorCase_ = 10;
break;
}
case 162: {
io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder subBuilder = null;
if (actorCase_ == 20) {
subBuilder = ((io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_).toBuilder();
}
actor_ =
input.readMessage(io.bloombox.schema.partner.PartnerMeta.PartnerKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_);
actor_ = subBuilder.buildPartial();
}
actorCase_ = 20;
break;
}
case 242: {
io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder subBuilder = null;
if (actorCase_ == 30) {
subBuilder = ((io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_).toBuilder();
}
actor_ =
input.readMessage(io.bloombox.schema.partner.LocationAccountKey.LocationKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_);
actor_ = subBuilder.buildPartial();
}
actorCase_ = 30;
break;
}
case 322: {
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder subBuilder = null;
if (actorCase_ == 40) {
subBuilder = ((io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_).toBuilder();
}
actor_ =
input.readMessage(io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_);
actor_ = subBuilder.buildPartial();
}
actorCase_ = 40;
break;
}
case 402: {
io.bloombox.schema.ledger.LedgerNode.Node.Builder subBuilder = null;
if (actorCase_ == 50) {
subBuilder = ((io.bloombox.schema.ledger.LedgerNode.Node) actor_).toBuilder();
}
actor_ =
input.readMessage(io.bloombox.schema.ledger.LedgerNode.Node.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.ledger.LedgerNode.Node) actor_);
actor_ = subBuilder.buildPartial();
}
actorCase_ = 50;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.ActorKey.class, io.bloombox.schema.ledger.Accounts.ActorKey.Builder.class);
}
private int actorCase_ = 0;
private java.lang.Object actor_;
public enum ActorCase
implements com.google.protobuf.Internal.EnumLite {
KNOWN(1),
USER(10),
PARTNER(20),
LOCATION(30),
DEVICE(40),
NODE(50),
ACTOR_NOT_SET(0);
private final int value;
private ActorCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActorCase valueOf(int value) {
return forNumber(value);
}
public static ActorCase forNumber(int value) {
switch (value) {
case 1: return KNOWN;
case 10: return USER;
case 20: return PARTNER;
case 30: return LOCATION;
case 40: return DEVICE;
case 50: return NODE;
case 0: return ACTOR_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ActorCase
getActorCase() {
return ActorCase.forNumber(
actorCase_);
}
public static final int KNOWN_FIELD_NUMBER = 1;
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public int getKnownValue() {
if (actorCase_ == 1) {
return (java.lang.Integer) actor_;
}
return 0;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public io.bloombox.schema.ledger.Accounts.SpecialActor getKnown() {
if (actorCase_ == 1) {
@SuppressWarnings("deprecation")
io.bloombox.schema.ledger.Accounts.SpecialActor result = io.bloombox.schema.ledger.Accounts.SpecialActor.valueOf(
(java.lang.Integer) actor_);
return result == null ? io.bloombox.schema.ledger.Accounts.SpecialActor.UNRECOGNIZED : result;
}
return io.bloombox.schema.ledger.Accounts.SpecialActor.SYSTEM;
}
public static final int USER_FIELD_NUMBER = 10;
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public boolean hasUser() {
return actorCase_ == 10;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
if (actorCase_ == 10) {
return (io.bloombox.schema.identity.AppUserKey.UserKey) actor_;
}
return io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
if (actorCase_ == 10) {
return (io.bloombox.schema.identity.AppUserKey.UserKey) actor_;
}
return io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
}
public static final int PARTNER_FIELD_NUMBER = 20;
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public boolean hasPartner() {
return actorCase_ == 20;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public io.bloombox.schema.partner.PartnerMeta.PartnerKey getPartner() {
if (actorCase_ == 20) {
return (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_;
}
return io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder getPartnerOrBuilder() {
if (actorCase_ == 20) {
return (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_;
}
return io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
}
public static final int LOCATION_FIELD_NUMBER = 30;
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public boolean hasLocation() {
return actorCase_ == 30;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public io.bloombox.schema.partner.LocationAccountKey.LocationKey getLocation() {
if (actorCase_ == 30) {
return (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_;
}
return io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder getLocationOrBuilder() {
if (actorCase_ == 30) {
return (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_;
}
return io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
}
public static final int DEVICE_FIELD_NUMBER = 40;
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public boolean hasDevice() {
return actorCase_ == 40;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey getDevice() {
if (actorCase_ == 40) {
return (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_;
}
return io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder getDeviceOrBuilder() {
if (actorCase_ == 40) {
return (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_;
}
return io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
}
public static final int NODE_FIELD_NUMBER = 50;
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public boolean hasNode() {
return actorCase_ == 50;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public io.bloombox.schema.ledger.LedgerNode.Node getNode() {
if (actorCase_ == 50) {
return (io.bloombox.schema.ledger.LedgerNode.Node) actor_;
}
return io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder getNodeOrBuilder() {
if (actorCase_ == 50) {
return (io.bloombox.schema.ledger.LedgerNode.Node) actor_;
}
return io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (actorCase_ == 1) {
output.writeEnum(1, ((java.lang.Integer) actor_));
}
if (actorCase_ == 10) {
output.writeMessage(10, (io.bloombox.schema.identity.AppUserKey.UserKey) actor_);
}
if (actorCase_ == 20) {
output.writeMessage(20, (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_);
}
if (actorCase_ == 30) {
output.writeMessage(30, (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_);
}
if (actorCase_ == 40) {
output.writeMessage(40, (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_);
}
if (actorCase_ == 50) {
output.writeMessage(50, (io.bloombox.schema.ledger.LedgerNode.Node) actor_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (actorCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, ((java.lang.Integer) actor_));
}
if (actorCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.bloombox.schema.identity.AppUserKey.UserKey) actor_);
}
if (actorCase_ == 20) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_);
}
if (actorCase_ == 30) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_);
}
if (actorCase_ == 40) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(40, (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_);
}
if (actorCase_ == 50) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(50, (io.bloombox.schema.ledger.LedgerNode.Node) actor_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.ActorKey)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.ActorKey other = (io.bloombox.schema.ledger.Accounts.ActorKey) obj;
if (!getActorCase().equals(other.getActorCase())) return false;
switch (actorCase_) {
case 1:
if (getKnownValue()
!= other.getKnownValue()) return false;
break;
case 10:
if (!getUser()
.equals(other.getUser())) return false;
break;
case 20:
if (!getPartner()
.equals(other.getPartner())) return false;
break;
case 30:
if (!getLocation()
.equals(other.getLocation())) return false;
break;
case 40:
if (!getDevice()
.equals(other.getDevice())) return false;
break;
case 50:
if (!getNode()
.equals(other.getNode())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (actorCase_) {
case 1:
hash = (37 * hash) + KNOWN_FIELD_NUMBER;
hash = (53 * hash) + getKnownValue();
break;
case 10:
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
break;
case 20:
hash = (37 * hash) + PARTNER_FIELD_NUMBER;
hash = (53 * hash) + getPartner().hashCode();
break;
case 30:
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
break;
case 40:
hash = (37 * hash) + DEVICE_FIELD_NUMBER;
hash = (53 * hash) + getDevice().hashCode();
break;
case 50:
hash = (37 * hash) + NODE_FIELD_NUMBER;
hash = (53 * hash) + getNode().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.ActorKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.ActorKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the concept of a ledger "actor," which unifies individuals and organizations under one referential
* structure, such that either may easily be the basis for a ledger transaction.
*
*
* Protobuf type {@code bloombox.ledger.ActorKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.ActorKey)
io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.ActorKey.class, io.bloombox.schema.ledger.Accounts.ActorKey.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.ActorKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
actorCase_ = 0;
actor_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_ActorKey_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorKey getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorKey build() {
io.bloombox.schema.ledger.Accounts.ActorKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorKey buildPartial() {
io.bloombox.schema.ledger.Accounts.ActorKey result = new io.bloombox.schema.ledger.Accounts.ActorKey(this);
if (actorCase_ == 1) {
result.actor_ = actor_;
}
if (actorCase_ == 10) {
if (userBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = userBuilder_.build();
}
}
if (actorCase_ == 20) {
if (partnerBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = partnerBuilder_.build();
}
}
if (actorCase_ == 30) {
if (locationBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = locationBuilder_.build();
}
}
if (actorCase_ == 40) {
if (deviceBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = deviceBuilder_.build();
}
}
if (actorCase_ == 50) {
if (nodeBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = nodeBuilder_.build();
}
}
result.actorCase_ = actorCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.ActorKey) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.ActorKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.ActorKey other) {
if (other == io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance()) return this;
switch (other.getActorCase()) {
case KNOWN: {
setKnownValue(other.getKnownValue());
break;
}
case USER: {
mergeUser(other.getUser());
break;
}
case PARTNER: {
mergePartner(other.getPartner());
break;
}
case LOCATION: {
mergeLocation(other.getLocation());
break;
}
case DEVICE: {
mergeDevice(other.getDevice());
break;
}
case NODE: {
mergeNode(other.getNode());
break;
}
case ACTOR_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.ActorKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.ActorKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int actorCase_ = 0;
private java.lang.Object actor_;
public ActorCase
getActorCase() {
return ActorCase.forNumber(
actorCase_);
}
public Builder clearActor() {
actorCase_ = 0;
actor_ = null;
onChanged();
return this;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public int getKnownValue() {
if (actorCase_ == 1) {
return ((java.lang.Integer) actor_).intValue();
}
return 0;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public Builder setKnownValue(int value) {
actorCase_ = 1;
actor_ = value;
onChanged();
return this;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public io.bloombox.schema.ledger.Accounts.SpecialActor getKnown() {
if (actorCase_ == 1) {
@SuppressWarnings("deprecation")
io.bloombox.schema.ledger.Accounts.SpecialActor result = io.bloombox.schema.ledger.Accounts.SpecialActor.valueOf(
(java.lang.Integer) actor_);
return result == null ? io.bloombox.schema.ledger.Accounts.SpecialActor.UNRECOGNIZED : result;
}
return io.bloombox.schema.ledger.Accounts.SpecialActor.SYSTEM;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public Builder setKnown(io.bloombox.schema.ledger.Accounts.SpecialActor value) {
if (value == null) {
throw new NullPointerException();
}
actorCase_ = 1;
actor_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a system account, such as reconciliation or sandbox access.
*
*
* .bloombox.ledger.SpecialActor known = 1;
*/
public Builder clearKnown() {
if (actorCase_ == 1) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder> userBuilder_;
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public boolean hasUser() {
return actorCase_ == 10;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
if (userBuilder_ == null) {
if (actorCase_ == 10) {
return (io.bloombox.schema.identity.AppUserKey.UserKey) actor_;
}
return io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
} else {
if (actorCase_ == 10) {
return userBuilder_.getMessage();
}
return io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
}
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public Builder setUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
userBuilder_.setMessage(value);
}
actorCase_ = 10;
return this;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public Builder setUser(
io.bloombox.schema.identity.AppUserKey.UserKey.Builder builderForValue) {
if (userBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
userBuilder_.setMessage(builderForValue.build());
}
actorCase_ = 10;
return this;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public Builder mergeUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (actorCase_ == 10 &&
actor_ != io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance()) {
actor_ = io.bloombox.schema.identity.AppUserKey.UserKey.newBuilder((io.bloombox.schema.identity.AppUserKey.UserKey) actor_)
.mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
if (actorCase_ == 10) {
userBuilder_.mergeFrom(value);
}
userBuilder_.setMessage(value);
}
actorCase_ = 10;
return this;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public Builder clearUser() {
if (userBuilder_ == null) {
if (actorCase_ == 10) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
} else {
if (actorCase_ == 10) {
actorCase_ = 0;
actor_ = null;
}
userBuilder_.clear();
}
return this;
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey.Builder getUserBuilder() {
return getUserFieldBuilder().getBuilder();
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
if ((actorCase_ == 10) && (userBuilder_ != null)) {
return userBuilder_.getMessageOrBuilder();
} else {
if (actorCase_ == 10) {
return (io.bloombox.schema.identity.AppUserKey.UserKey) actor_;
}
return io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
}
}
/**
*
* Specifies a user as the identity behind a particular ledger account.
*
*
* .bloombox.identity.UserKey user = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>
getUserFieldBuilder() {
if (userBuilder_ == null) {
if (!(actorCase_ == 10)) {
actor_ = io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance();
}
userBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>(
(io.bloombox.schema.identity.AppUserKey.UserKey) actor_,
getParentForChildren(),
isClean());
actor_ = null;
}
actorCase_ = 10;
onChanged();;
return userBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerMeta.PartnerKey, io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder, io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder> partnerBuilder_;
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public boolean hasPartner() {
return actorCase_ == 20;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public io.bloombox.schema.partner.PartnerMeta.PartnerKey getPartner() {
if (partnerBuilder_ == null) {
if (actorCase_ == 20) {
return (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_;
}
return io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
} else {
if (actorCase_ == 20) {
return partnerBuilder_.getMessage();
}
return io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public Builder setPartner(io.bloombox.schema.partner.PartnerMeta.PartnerKey value) {
if (partnerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
partnerBuilder_.setMessage(value);
}
actorCase_ = 20;
return this;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public Builder setPartner(
io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder builderForValue) {
if (partnerBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
partnerBuilder_.setMessage(builderForValue.build());
}
actorCase_ = 20;
return this;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public Builder mergePartner(io.bloombox.schema.partner.PartnerMeta.PartnerKey value) {
if (partnerBuilder_ == null) {
if (actorCase_ == 20 &&
actor_ != io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance()) {
actor_ = io.bloombox.schema.partner.PartnerMeta.PartnerKey.newBuilder((io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_)
.mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
if (actorCase_ == 20) {
partnerBuilder_.mergeFrom(value);
}
partnerBuilder_.setMessage(value);
}
actorCase_ = 20;
return this;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public Builder clearPartner() {
if (partnerBuilder_ == null) {
if (actorCase_ == 20) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
} else {
if (actorCase_ == 20) {
actorCase_ = 0;
actor_ = null;
}
partnerBuilder_.clear();
}
return this;
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder getPartnerBuilder() {
return getPartnerFieldBuilder().getBuilder();
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
public io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder getPartnerOrBuilder() {
if ((actorCase_ == 20) && (partnerBuilder_ != null)) {
return partnerBuilder_.getMessageOrBuilder();
} else {
if (actorCase_ == 20) {
return (io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_;
}
return io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner organization as the identity behind a particular ledger account.
*
*
* .bloombox.partner.PartnerKey partner = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerMeta.PartnerKey, io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder, io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder>
getPartnerFieldBuilder() {
if (partnerBuilder_ == null) {
if (!(actorCase_ == 20)) {
actor_ = io.bloombox.schema.partner.PartnerMeta.PartnerKey.getDefaultInstance();
}
partnerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerMeta.PartnerKey, io.bloombox.schema.partner.PartnerMeta.PartnerKey.Builder, io.bloombox.schema.partner.PartnerMeta.PartnerKeyOrBuilder>(
(io.bloombox.schema.partner.PartnerMeta.PartnerKey) actor_,
getParentForChildren(),
isClean());
actor_ = null;
}
actorCase_ = 20;
onChanged();;
return partnerBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.LocationAccountKey.LocationKey, io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder, io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder> locationBuilder_;
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public boolean hasLocation() {
return actorCase_ == 30;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public io.bloombox.schema.partner.LocationAccountKey.LocationKey getLocation() {
if (locationBuilder_ == null) {
if (actorCase_ == 30) {
return (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_;
}
return io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
} else {
if (actorCase_ == 30) {
return locationBuilder_.getMessage();
}
return io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public Builder setLocation(io.bloombox.schema.partner.LocationAccountKey.LocationKey value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
locationBuilder_.setMessage(value);
}
actorCase_ = 30;
return this;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public Builder setLocation(
io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder builderForValue) {
if (locationBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
locationBuilder_.setMessage(builderForValue.build());
}
actorCase_ = 30;
return this;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public Builder mergeLocation(io.bloombox.schema.partner.LocationAccountKey.LocationKey value) {
if (locationBuilder_ == null) {
if (actorCase_ == 30 &&
actor_ != io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance()) {
actor_ = io.bloombox.schema.partner.LocationAccountKey.LocationKey.newBuilder((io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_)
.mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
if (actorCase_ == 30) {
locationBuilder_.mergeFrom(value);
}
locationBuilder_.setMessage(value);
}
actorCase_ = 30;
return this;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
if (actorCase_ == 30) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
} else {
if (actorCase_ == 30) {
actorCase_ = 0;
actor_ = null;
}
locationBuilder_.clear();
}
return this;
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder getLocationBuilder() {
return getLocationFieldBuilder().getBuilder();
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
public io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder getLocationOrBuilder() {
if ((actorCase_ == 30) && (locationBuilder_ != null)) {
return locationBuilder_.getMessageOrBuilder();
} else {
if (actorCase_ == 30) {
return (io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_;
}
return io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner location as the identity behind a particular ledger account.
*
*
* .bloombox.partner.LocationKey location = 30;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.LocationAccountKey.LocationKey, io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder, io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
if (!(actorCase_ == 30)) {
actor_ = io.bloombox.schema.partner.LocationAccountKey.LocationKey.getDefaultInstance();
}
locationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.LocationAccountKey.LocationKey, io.bloombox.schema.partner.LocationAccountKey.LocationKey.Builder, io.bloombox.schema.partner.LocationAccountKey.LocationKeyOrBuilder>(
(io.bloombox.schema.partner.LocationAccountKey.LocationKey) actor_,
getParentForChildren(),
isClean());
actor_ = null;
}
actorCase_ = 30;
onChanged();;
return locationBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder> deviceBuilder_;
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public boolean hasDevice() {
return actorCase_ == 40;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey getDevice() {
if (deviceBuilder_ == null) {
if (actorCase_ == 40) {
return (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_;
}
return io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
} else {
if (actorCase_ == 40) {
return deviceBuilder_.getMessage();
}
return io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public Builder setDevice(io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey value) {
if (deviceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
deviceBuilder_.setMessage(value);
}
actorCase_ = 40;
return this;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public Builder setDevice(
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder builderForValue) {
if (deviceBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
deviceBuilder_.setMessage(builderForValue.build());
}
actorCase_ = 40;
return this;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public Builder mergeDevice(io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey value) {
if (deviceBuilder_ == null) {
if (actorCase_ == 40 &&
actor_ != io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance()) {
actor_ = io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.newBuilder((io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_)
.mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
if (actorCase_ == 40) {
deviceBuilder_.mergeFrom(value);
}
deviceBuilder_.setMessage(value);
}
actorCase_ = 40;
return this;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public Builder clearDevice() {
if (deviceBuilder_ == null) {
if (actorCase_ == 40) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
} else {
if (actorCase_ == 40) {
actorCase_ = 0;
actor_ = null;
}
deviceBuilder_.clear();
}
return this;
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder getDeviceBuilder() {
return getDeviceFieldBuilder().getBuilder();
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
public io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder getDeviceOrBuilder() {
if ((actorCase_ == 40) && (deviceBuilder_ != null)) {
return deviceBuilder_.getMessageOrBuilder();
} else {
if (actorCase_ == 40) {
return (io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_;
}
return io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
}
}
/**
*
* Specifies a partner co-located device as the identify behind a particular ledger account.
*
*
* .bloombox.partner.PartnerDeviceKey device = 40;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder>
getDeviceFieldBuilder() {
if (deviceBuilder_ == null) {
if (!(actorCase_ == 40)) {
actor_ = io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.getDefaultInstance();
}
deviceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey.Builder, io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKeyOrBuilder>(
(io.bloombox.schema.partner.PartnerDevices.PartnerDeviceKey) actor_,
getParentForChildren(),
isClean());
actor_ = null;
}
actorCase_ = 40;
onChanged();;
return deviceBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.LedgerNode.Node, io.bloombox.schema.ledger.LedgerNode.Node.Builder, io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder> nodeBuilder_;
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public boolean hasNode() {
return actorCase_ == 50;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public io.bloombox.schema.ledger.LedgerNode.Node getNode() {
if (nodeBuilder_ == null) {
if (actorCase_ == 50) {
return (io.bloombox.schema.ledger.LedgerNode.Node) actor_;
}
return io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
} else {
if (actorCase_ == 50) {
return nodeBuilder_.getMessage();
}
return io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
}
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public Builder setNode(io.bloombox.schema.ledger.LedgerNode.Node value) {
if (nodeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
nodeBuilder_.setMessage(value);
}
actorCase_ = 50;
return this;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public Builder setNode(
io.bloombox.schema.ledger.LedgerNode.Node.Builder builderForValue) {
if (nodeBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
nodeBuilder_.setMessage(builderForValue.build());
}
actorCase_ = 50;
return this;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public Builder mergeNode(io.bloombox.schema.ledger.LedgerNode.Node value) {
if (nodeBuilder_ == null) {
if (actorCase_ == 50 &&
actor_ != io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance()) {
actor_ = io.bloombox.schema.ledger.LedgerNode.Node.newBuilder((io.bloombox.schema.ledger.LedgerNode.Node) actor_)
.mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
if (actorCase_ == 50) {
nodeBuilder_.mergeFrom(value);
}
nodeBuilder_.setMessage(value);
}
actorCase_ = 50;
return this;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public Builder clearNode() {
if (nodeBuilder_ == null) {
if (actorCase_ == 50) {
actorCase_ = 0;
actor_ = null;
onChanged();
}
} else {
if (actorCase_ == 50) {
actorCase_ = 0;
actor_ = null;
}
nodeBuilder_.clear();
}
return this;
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public io.bloombox.schema.ledger.LedgerNode.Node.Builder getNodeBuilder() {
return getNodeFieldBuilder().getBuilder();
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
public io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder getNodeOrBuilder() {
if ((actorCase_ == 50) && (nodeBuilder_ != null)) {
return nodeBuilder_.getMessageOrBuilder();
} else {
if (actorCase_ == 50) {
return (io.bloombox.schema.ledger.LedgerNode.Node) actor_;
}
return io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
}
}
/**
*
* Specifies a data node as the identity behind a particular ledger account.
*
*
* .bloombox.ledger.Node node = 50;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.LedgerNode.Node, io.bloombox.schema.ledger.LedgerNode.Node.Builder, io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder>
getNodeFieldBuilder() {
if (nodeBuilder_ == null) {
if (!(actorCase_ == 50)) {
actor_ = io.bloombox.schema.ledger.LedgerNode.Node.getDefaultInstance();
}
nodeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.LedgerNode.Node, io.bloombox.schema.ledger.LedgerNode.Node.Builder, io.bloombox.schema.ledger.LedgerNode.NodeOrBuilder>(
(io.bloombox.schema.ledger.LedgerNode.Node) actor_,
getParentForChildren(),
isClean());
actor_ = null;
}
actorCase_ = 50;
onChanged();;
return nodeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.ActorKey)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.ActorKey)
private static final io.bloombox.schema.ledger.Accounts.ActorKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.ActorKey();
}
public static io.bloombox.schema.ledger.Accounts.ActorKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ActorKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ActorKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.ActorKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.AccountKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
java.lang.String getId();
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
boolean hasPair();
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
io.opencannabis.schema.crypto.primitives.Keypair getPair();
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getPairOrBuilder();
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
boolean hasIdentity();
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
io.opencannabis.schema.crypto.primitives.Keypair getIdentity();
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getIdentityOrBuilder();
}
/**
*
* Specifies the structure of a distributed ledger account, which is usually defined by its public/private keypair. In
* particular, the computation of UPPER(SHA3-B58(public_key)) is used to identify an account uniquely in public.
* Separately, a user account may be associated with a concrete identity via the `identity` property.
*
*
* Protobuf type {@code bloombox.ledger.AccountKey}
*/
public static final class AccountKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.AccountKey)
AccountKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountKey.newBuilder() to construct.
private AccountKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountKey() {
id_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AccountKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
io.opencannabis.schema.crypto.primitives.Keypair.Builder subBuilder = null;
if (pair_ != null) {
subBuilder = pair_.toBuilder();
}
pair_ = input.readMessage(io.opencannabis.schema.crypto.primitives.Keypair.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pair_);
pair_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.crypto.primitives.Keypair.Builder subBuilder = null;
if (identity_ != null) {
subBuilder = identity_.toBuilder();
}
identity_ = input.readMessage(io.opencannabis.schema.crypto.primitives.Keypair.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identity_);
identity_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AccountKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AccountKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.AccountKey.class, io.bloombox.schema.ledger.Accounts.AccountKey.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAIR_FIELD_NUMBER = 2;
private io.opencannabis.schema.crypto.primitives.Keypair pair_;
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public boolean hasPair() {
return pair_ != null;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public io.opencannabis.schema.crypto.primitives.Keypair getPair() {
return pair_ == null ? io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : pair_;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getPairOrBuilder() {
return getPair();
}
public static final int IDENTITY_FIELD_NUMBER = 3;
private io.opencannabis.schema.crypto.primitives.Keypair identity_;
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public boolean hasIdentity() {
return identity_ != null;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public io.opencannabis.schema.crypto.primitives.Keypair getIdentity() {
return identity_ == null ? io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : identity_;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getIdentityOrBuilder() {
return getIdentity();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (pair_ != null) {
output.writeMessage(2, getPair());
}
if (identity_ != null) {
output.writeMessage(3, getIdentity());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (pair_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPair());
}
if (identity_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getIdentity());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.AccountKey)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.AccountKey other = (io.bloombox.schema.ledger.Accounts.AccountKey) obj;
if (!getId()
.equals(other.getId())) return false;
if (hasPair() != other.hasPair()) return false;
if (hasPair()) {
if (!getPair()
.equals(other.getPair())) return false;
}
if (hasIdentity() != other.hasIdentity()) return false;
if (hasIdentity()) {
if (!getIdentity()
.equals(other.getIdentity())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
if (hasPair()) {
hash = (37 * hash) + PAIR_FIELD_NUMBER;
hash = (53 * hash) + getPair().hashCode();
}
if (hasIdentity()) {
hash = (37 * hash) + IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getIdentity().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AccountKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.AccountKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of a distributed ledger account, which is usually defined by its public/private keypair. In
* particular, the computation of UPPER(SHA3-B58(public_key)) is used to identify an account uniquely in public.
* Separately, a user account may be associated with a concrete identity via the `identity` property.
*
*
* Protobuf type {@code bloombox.ledger.AccountKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.AccountKey)
io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AccountKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AccountKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.AccountKey.class, io.bloombox.schema.ledger.Accounts.AccountKey.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.AccountKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
if (pairBuilder_ == null) {
pair_ = null;
} else {
pair_ = null;
pairBuilder_ = null;
}
if (identityBuilder_ == null) {
identity_ = null;
} else {
identity_ = null;
identityBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AccountKey_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AccountKey getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.AccountKey.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AccountKey build() {
io.bloombox.schema.ledger.Accounts.AccountKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AccountKey buildPartial() {
io.bloombox.schema.ledger.Accounts.AccountKey result = new io.bloombox.schema.ledger.Accounts.AccountKey(this);
result.id_ = id_;
if (pairBuilder_ == null) {
result.pair_ = pair_;
} else {
result.pair_ = pairBuilder_.build();
}
if (identityBuilder_ == null) {
result.identity_ = identity_;
} else {
result.identity_ = identityBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.AccountKey) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.AccountKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.AccountKey other) {
if (other == io.bloombox.schema.ledger.Accounts.AccountKey.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (other.hasPair()) {
mergePair(other.getPair());
}
if (other.hasIdentity()) {
mergeIdentity(other.getIdentity());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.AccountKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.AccountKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* The account key ID is the computed result of UPPER(B58(SHA3-512(B64(raw_public_key) + chain + salt)))[0:-32].
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.crypto.primitives.Keypair pair_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder> pairBuilder_;
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public boolean hasPair() {
return pairBuilder_ != null || pair_ != null;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public io.opencannabis.schema.crypto.primitives.Keypair getPair() {
if (pairBuilder_ == null) {
return pair_ == null ? io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : pair_;
} else {
return pairBuilder_.getMessage();
}
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public Builder setPair(io.opencannabis.schema.crypto.primitives.Keypair value) {
if (pairBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pair_ = value;
onChanged();
} else {
pairBuilder_.setMessage(value);
}
return this;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public Builder setPair(
io.opencannabis.schema.crypto.primitives.Keypair.Builder builderForValue) {
if (pairBuilder_ == null) {
pair_ = builderForValue.build();
onChanged();
} else {
pairBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public Builder mergePair(io.opencannabis.schema.crypto.primitives.Keypair value) {
if (pairBuilder_ == null) {
if (pair_ != null) {
pair_ =
io.opencannabis.schema.crypto.primitives.Keypair.newBuilder(pair_).mergeFrom(value).buildPartial();
} else {
pair_ = value;
}
onChanged();
} else {
pairBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public Builder clearPair() {
if (pairBuilder_ == null) {
pair_ = null;
onChanged();
} else {
pair_ = null;
pairBuilder_ = null;
}
return this;
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public io.opencannabis.schema.crypto.primitives.Keypair.Builder getPairBuilder() {
onChanged();
return getPairFieldBuilder().getBuilder();
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
public io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getPairOrBuilder() {
if (pairBuilder_ != null) {
return pairBuilder_.getMessageOrBuilder();
} else {
return pair_ == null ?
io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : pair_;
}
}
/**
*
* Reference to the public/private keypair for this ledger account. In rare cases, the private key may be included
* here (the structure supports it), but usually, it's used for the on-board cryptographic hash of the public key.
*
*
* .opencannabis.crypto.Keypair pair = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder>
getPairFieldBuilder() {
if (pairBuilder_ == null) {
pairBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder>(
getPair(),
getParentForChildren(),
isClean());
pair_ = null;
}
return pairBuilder_;
}
private io.opencannabis.schema.crypto.primitives.Keypair identity_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder> identityBuilder_;
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public boolean hasIdentity() {
return identityBuilder_ != null || identity_ != null;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public io.opencannabis.schema.crypto.primitives.Keypair getIdentity() {
if (identityBuilder_ == null) {
return identity_ == null ? io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : identity_;
} else {
return identityBuilder_.getMessage();
}
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public Builder setIdentity(io.opencannabis.schema.crypto.primitives.Keypair value) {
if (identityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identity_ = value;
onChanged();
} else {
identityBuilder_.setMessage(value);
}
return this;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public Builder setIdentity(
io.opencannabis.schema.crypto.primitives.Keypair.Builder builderForValue) {
if (identityBuilder_ == null) {
identity_ = builderForValue.build();
onChanged();
} else {
identityBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public Builder mergeIdentity(io.opencannabis.schema.crypto.primitives.Keypair value) {
if (identityBuilder_ == null) {
if (identity_ != null) {
identity_ =
io.opencannabis.schema.crypto.primitives.Keypair.newBuilder(identity_).mergeFrom(value).buildPartial();
} else {
identity_ = value;
}
onChanged();
} else {
identityBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public Builder clearIdentity() {
if (identityBuilder_ == null) {
identity_ = null;
onChanged();
} else {
identity_ = null;
identityBuilder_ = null;
}
return this;
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public io.opencannabis.schema.crypto.primitives.Keypair.Builder getIdentityBuilder() {
onChanged();
return getIdentityFieldBuilder().getBuilder();
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
public io.opencannabis.schema.crypto.primitives.KeypairOrBuilder getIdentityOrBuilder() {
if (identityBuilder_ != null) {
return identityBuilder_.getMessageOrBuilder();
} else {
return identity_ == null ?
io.opencannabis.schema.crypto.primitives.Keypair.getDefaultInstance() : identity_;
}
}
/**
*
* Reference to the identity public/private keypair for this actor. Ledger "actors" represent the real-world identity
* behind organizations or individuals who execute transactions on the ledger.
*
*
* .opencannabis.crypto.Keypair identity = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder>
getIdentityFieldBuilder() {
if (identityBuilder_ == null) {
identityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.Keypair, io.opencannabis.schema.crypto.primitives.Keypair.Builder, io.opencannabis.schema.crypto.primitives.KeypairOrBuilder>(
getIdentity(),
getParentForChildren(),
isClean());
identity_ = null;
}
return identityBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.AccountKey)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.AccountKey)
private static final io.bloombox.schema.ledger.Accounts.AccountKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.AccountKey();
}
public static io.bloombox.schema.ledger.Accounts.AccountKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AccountKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AccountKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LedgerIdentityOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.LedgerIdentity)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
boolean hasKey();
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.ledger.Accounts.AccountKey getKey();
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder getKeyOrBuilder();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
boolean hasActor();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
io.bloombox.schema.ledger.Accounts.ActorKey getActor();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
boolean hasCertificate();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder();
}
/**
*
* Specifies the notion of a concrete identity on the ledger. This is essentially the intersection of two items: 1) key
* material generated by the actor themselves, and 2) associated identity stored somewhere else, or stored with us.
*
*
* Protobuf type {@code bloombox.ledger.LedgerIdentity}
*/
public static final class LedgerIdentity extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.LedgerIdentity)
LedgerIdentityOrBuilder {
private static final long serialVersionUID = 0L;
// Use LedgerIdentity.newBuilder() to construct.
private LedgerIdentity(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LedgerIdentity() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LedgerIdentity(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.ledger.Accounts.AccountKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.bloombox.schema.ledger.Accounts.AccountKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.bloombox.schema.ledger.Accounts.ActorKey.Builder subBuilder = null;
if (actor_ != null) {
subBuilder = actor_.toBuilder();
}
actor_ = input.readMessage(io.bloombox.schema.ledger.Accounts.ActorKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(actor_);
actor_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder subBuilder = null;
if (certificate_ != null) {
subBuilder = certificate_.toBuilder();
}
certificate_ = input.readMessage(io.bloombox.schema.ledger.Accounts.ActorCertificate.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(certificate_);
certificate_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_LedgerIdentity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_LedgerIdentity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.LedgerIdentity.class, io.bloombox.schema.ledger.Accounts.LedgerIdentity.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private io.bloombox.schema.ledger.Accounts.AccountKey key_;
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.ledger.Accounts.AccountKey getKey() {
return key_ == null ? io.bloombox.schema.ledger.Accounts.AccountKey.getDefaultInstance() : key_;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int ACTOR_FIELD_NUMBER = 2;
private io.bloombox.schema.ledger.Accounts.ActorKey actor_;
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public boolean hasActor() {
return actor_ != null;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey getActor() {
return actor_ == null ? io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder() {
return getActor();
}
public static final int CERTIFICATE_FIELD_NUMBER = 3;
private io.bloombox.schema.ledger.Accounts.ActorCertificate certificate_;
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public boolean hasCertificate() {
return certificate_ != null;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate() {
return certificate_ == null ? io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder() {
return getCertificate();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (actor_ != null) {
output.writeMessage(2, getActor());
}
if (certificate_ != null) {
output.writeMessage(3, getCertificate());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (actor_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getActor());
}
if (certificate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCertificate());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.LedgerIdentity)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.LedgerIdentity other = (io.bloombox.schema.ledger.Accounts.LedgerIdentity) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasActor() != other.hasActor()) return false;
if (hasActor()) {
if (!getActor()
.equals(other.getActor())) return false;
}
if (hasCertificate() != other.hasCertificate()) return false;
if (hasCertificate()) {
if (!getCertificate()
.equals(other.getCertificate())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasActor()) {
hash = (37 * hash) + ACTOR_FIELD_NUMBER;
hash = (53 * hash) + getActor().hashCode();
}
if (hasCertificate()) {
hash = (37 * hash) + CERTIFICATE_FIELD_NUMBER;
hash = (53 * hash) + getCertificate().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.LedgerIdentity prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the notion of a concrete identity on the ledger. This is essentially the intersection of two items: 1) key
* material generated by the actor themselves, and 2) associated identity stored somewhere else, or stored with us.
*
*
* Protobuf type {@code bloombox.ledger.LedgerIdentity}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.LedgerIdentity)
io.bloombox.schema.ledger.Accounts.LedgerIdentityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_LedgerIdentity_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_LedgerIdentity_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.LedgerIdentity.class, io.bloombox.schema.ledger.Accounts.LedgerIdentity.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.LedgerIdentity.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
if (actorBuilder_ == null) {
actor_ = null;
} else {
actor_ = null;
actorBuilder_ = null;
}
if (certificateBuilder_ == null) {
certificate_ = null;
} else {
certificate_ = null;
certificateBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_LedgerIdentity_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.LedgerIdentity getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.LedgerIdentity.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.LedgerIdentity build() {
io.bloombox.schema.ledger.Accounts.LedgerIdentity result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.LedgerIdentity buildPartial() {
io.bloombox.schema.ledger.Accounts.LedgerIdentity result = new io.bloombox.schema.ledger.Accounts.LedgerIdentity(this);
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (actorBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = actorBuilder_.build();
}
if (certificateBuilder_ == null) {
result.certificate_ = certificate_;
} else {
result.certificate_ = certificateBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.LedgerIdentity) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.LedgerIdentity)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.LedgerIdentity other) {
if (other == io.bloombox.schema.ledger.Accounts.LedgerIdentity.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.hasActor()) {
mergeActor(other.getActor());
}
if (other.hasCertificate()) {
mergeCertificate(other.getCertificate());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.LedgerIdentity parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.LedgerIdentity) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.ledger.Accounts.AccountKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AccountKey, io.bloombox.schema.ledger.Accounts.AccountKey.Builder, io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder> keyBuilder_;
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.ledger.Accounts.AccountKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.bloombox.schema.ledger.Accounts.AccountKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(io.bloombox.schema.ledger.Accounts.AccountKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(
io.bloombox.schema.ledger.Accounts.AccountKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public Builder mergeKey(io.bloombox.schema.ledger.Accounts.AccountKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.bloombox.schema.ledger.Accounts.AccountKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.ledger.Accounts.AccountKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.bloombox.schema.ledger.Accounts.AccountKey.getDefaultInstance() : key_;
}
}
/**
*
* Specifies the key material behind this identity. In rare cases, this may include the private key (the structure
* supports such behavior), but in most cases only a cryptographic fingerprint of the public key is available.
*
*
* .bloombox.ledger.AccountKey key = 1 [(.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AccountKey, io.bloombox.schema.ledger.Accounts.AccountKey.Builder, io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AccountKey, io.bloombox.schema.ledger.Accounts.AccountKey.Builder, io.bloombox.schema.ledger.Accounts.AccountKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private io.bloombox.schema.ledger.Accounts.ActorKey actor_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder> actorBuilder_;
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public boolean hasActor() {
return actorBuilder_ != null || actor_ != null;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey getActor() {
if (actorBuilder_ == null) {
return actor_ == null ? io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
} else {
return actorBuilder_.getMessage();
}
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public Builder setActor(io.bloombox.schema.ledger.Accounts.ActorKey value) {
if (actorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
actorBuilder_.setMessage(value);
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public Builder setActor(
io.bloombox.schema.ledger.Accounts.ActorKey.Builder builderForValue) {
if (actorBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
actorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public Builder mergeActor(io.bloombox.schema.ledger.Accounts.ActorKey value) {
if (actorBuilder_ == null) {
if (actor_ != null) {
actor_ =
io.bloombox.schema.ledger.Accounts.ActorKey.newBuilder(actor_).mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
actorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public Builder clearActor() {
if (actorBuilder_ == null) {
actor_ = null;
onChanged();
} else {
actor_ = null;
actorBuilder_ = null;
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey.Builder getActorBuilder() {
onChanged();
return getActorFieldBuilder().getBuilder();
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
public io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder() {
if (actorBuilder_ != null) {
return actorBuilder_.getMessageOrBuilder();
} else {
return actor_ == null ?
io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
}
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder>
getActorFieldBuilder() {
if (actorBuilder_ == null) {
actorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder>(
getActor(),
getParentForChildren(),
isClean());
actor_ = null;
}
return actorBuilder_;
}
private io.bloombox.schema.ledger.Accounts.ActorCertificate certificate_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder> certificateBuilder_;
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public boolean hasCertificate() {
return certificateBuilder_ != null || certificate_ != null;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate() {
if (certificateBuilder_ == null) {
return certificate_ == null ? io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
} else {
return certificateBuilder_.getMessage();
}
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public Builder setCertificate(io.bloombox.schema.ledger.Accounts.ActorCertificate value) {
if (certificateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
certificate_ = value;
onChanged();
} else {
certificateBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public Builder setCertificate(
io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder builderForValue) {
if (certificateBuilder_ == null) {
certificate_ = builderForValue.build();
onChanged();
} else {
certificateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public Builder mergeCertificate(io.bloombox.schema.ledger.Accounts.ActorCertificate value) {
if (certificateBuilder_ == null) {
if (certificate_ != null) {
certificate_ =
io.bloombox.schema.ledger.Accounts.ActorCertificate.newBuilder(certificate_).mergeFrom(value).buildPartial();
} else {
certificate_ = value;
}
onChanged();
} else {
certificateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public Builder clearCertificate() {
if (certificateBuilder_ == null) {
certificate_ = null;
onChanged();
} else {
certificate_ = null;
certificateBuilder_ = null;
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder getCertificateBuilder() {
onChanged();
return getCertificateFieldBuilder().getBuilder();
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder() {
if (certificateBuilder_ != null) {
return certificateBuilder_.getMessageOrBuilder();
} else {
return certificate_ == null ?
io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
}
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder>
getCertificateFieldBuilder() {
if (certificateBuilder_ == null) {
certificateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder>(
getCertificate(),
getParentForChildren(),
isClean());
certificate_ = null;
}
return certificateBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.LedgerIdentity)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.LedgerIdentity)
private static final io.bloombox.schema.ledger.Accounts.LedgerIdentity DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.LedgerIdentity();
}
public static io.bloombox.schema.ledger.Accounts.LedgerIdentity getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LedgerIdentity parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LedgerIdentity(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.LedgerIdentity getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AssertionTicketOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.AssertionTicket)
com.google.protobuf.MessageOrBuilder {
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
boolean hasFingerprint();
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint();
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder();
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
boolean hasIssued();
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued();
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder();
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
java.lang.String getSignature();
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
com.google.protobuf.ByteString
getSignatureBytes();
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
java.lang.String getAssurer();
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
com.google.protobuf.ByteString
getAssurerBytes();
}
/**
*
* Specifies a structure that stamps a structure with server-side approval, indicating the subject payload was inspected
* and cryptographically verified.
*
*
* Protobuf type {@code bloombox.ledger.AssertionTicket}
*/
public static final class AssertionTicket extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.AssertionTicket)
AssertionTicketOrBuilder {
private static final long serialVersionUID = 0L;
// Use AssertionTicket.newBuilder() to construct.
private AssertionTicket(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AssertionTicket() {
signature_ = "";
assurer_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AssertionTicket(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder subBuilder = null;
if (fingerprint_ != null) {
subBuilder = fingerprint_.toBuilder();
}
fingerprint_ = input.readMessage(io.opencannabis.schema.crypto.primitives.integrity.Hash.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fingerprint_);
fingerprint_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (issued_ != null) {
subBuilder = issued_.toBuilder();
}
issued_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(issued_);
issued_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
signature_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
assurer_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AssertionTicket_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AssertionTicket_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.AssertionTicket.class, io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder.class);
}
public static final int FINGERPRINT_FIELD_NUMBER = 1;
private io.opencannabis.schema.crypto.primitives.integrity.Hash fingerprint_;
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public boolean hasFingerprint() {
return fingerprint_ != null;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint() {
return fingerprint_ == null ? io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder() {
return getFingerprint();
}
public static final int ISSUED_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant issued_;
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public boolean hasIssued() {
return issued_ != null;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued() {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder() {
return getIssued();
}
public static final int SIGNATURE_FIELD_NUMBER = 3;
private volatile java.lang.Object signature_;
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public java.lang.String getSignature() {
java.lang.Object ref = signature_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signature_ = s;
return s;
}
}
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public com.google.protobuf.ByteString
getSignatureBytes() {
java.lang.Object ref = signature_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signature_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSURER_FIELD_NUMBER = 4;
private volatile java.lang.Object assurer_;
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public java.lang.String getAssurer() {
java.lang.Object ref = assurer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
assurer_ = s;
return s;
}
}
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public com.google.protobuf.ByteString
getAssurerBytes() {
java.lang.Object ref = assurer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
assurer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (fingerprint_ != null) {
output.writeMessage(1, getFingerprint());
}
if (issued_ != null) {
output.writeMessage(2, getIssued());
}
if (!getSignatureBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, signature_);
}
if (!getAssurerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, assurer_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (fingerprint_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFingerprint());
}
if (issued_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIssued());
}
if (!getSignatureBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, signature_);
}
if (!getAssurerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, assurer_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.AssertionTicket)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.AssertionTicket other = (io.bloombox.schema.ledger.Accounts.AssertionTicket) obj;
if (hasFingerprint() != other.hasFingerprint()) return false;
if (hasFingerprint()) {
if (!getFingerprint()
.equals(other.getFingerprint())) return false;
}
if (hasIssued() != other.hasIssued()) return false;
if (hasIssued()) {
if (!getIssued()
.equals(other.getIssued())) return false;
}
if (!getSignature()
.equals(other.getSignature())) return false;
if (!getAssurer()
.equals(other.getAssurer())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFingerprint()) {
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
}
if (hasIssued()) {
hash = (37 * hash) + ISSUED_FIELD_NUMBER;
hash = (53 * hash) + getIssued().hashCode();
}
hash = (37 * hash) + SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getSignature().hashCode();
hash = (37 * hash) + ASSURER_FIELD_NUMBER;
hash = (53 * hash) + getAssurer().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.AssertionTicket prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a structure that stamps a structure with server-side approval, indicating the subject payload was inspected
* and cryptographically verified.
*
*
* Protobuf type {@code bloombox.ledger.AssertionTicket}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.AssertionTicket)
io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AssertionTicket_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AssertionTicket_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.AssertionTicket.class, io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.AssertionTicket.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (fingerprintBuilder_ == null) {
fingerprint_ = null;
} else {
fingerprint_ = null;
fingerprintBuilder_ = null;
}
if (issuedBuilder_ == null) {
issued_ = null;
} else {
issued_ = null;
issuedBuilder_ = null;
}
signature_ = "";
assurer_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_AssertionTicket_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AssertionTicket getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.AssertionTicket.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AssertionTicket build() {
io.bloombox.schema.ledger.Accounts.AssertionTicket result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AssertionTicket buildPartial() {
io.bloombox.schema.ledger.Accounts.AssertionTicket result = new io.bloombox.schema.ledger.Accounts.AssertionTicket(this);
if (fingerprintBuilder_ == null) {
result.fingerprint_ = fingerprint_;
} else {
result.fingerprint_ = fingerprintBuilder_.build();
}
if (issuedBuilder_ == null) {
result.issued_ = issued_;
} else {
result.issued_ = issuedBuilder_.build();
}
result.signature_ = signature_;
result.assurer_ = assurer_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.AssertionTicket) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.AssertionTicket)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.AssertionTicket other) {
if (other == io.bloombox.schema.ledger.Accounts.AssertionTicket.getDefaultInstance()) return this;
if (other.hasFingerprint()) {
mergeFingerprint(other.getFingerprint());
}
if (other.hasIssued()) {
mergeIssued(other.getIssued());
}
if (!other.getSignature().isEmpty()) {
signature_ = other.signature_;
onChanged();
}
if (!other.getAssurer().isEmpty()) {
assurer_ = other.assurer_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.AssertionTicket parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.AssertionTicket) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.crypto.primitives.integrity.Hash fingerprint_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder> fingerprintBuilder_;
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public boolean hasFingerprint() {
return fingerprintBuilder_ != null || fingerprint_ != null;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash getFingerprint() {
if (fingerprintBuilder_ == null) {
return fingerprint_ == null ? io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
} else {
return fingerprintBuilder_.getMessage();
}
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public Builder setFingerprint(io.opencannabis.schema.crypto.primitives.integrity.Hash value) {
if (fingerprintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
onChanged();
} else {
fingerprintBuilder_.setMessage(value);
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public Builder setFingerprint(
io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder builderForValue) {
if (fingerprintBuilder_ == null) {
fingerprint_ = builderForValue.build();
onChanged();
} else {
fingerprintBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public Builder mergeFingerprint(io.opencannabis.schema.crypto.primitives.integrity.Hash value) {
if (fingerprintBuilder_ == null) {
if (fingerprint_ != null) {
fingerprint_ =
io.opencannabis.schema.crypto.primitives.integrity.Hash.newBuilder(fingerprint_).mergeFrom(value).buildPartial();
} else {
fingerprint_ = value;
}
onChanged();
} else {
fingerprintBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public Builder clearFingerprint() {
if (fingerprintBuilder_ == null) {
fingerprint_ = null;
onChanged();
} else {
fingerprint_ = null;
fingerprintBuilder_ = null;
}
return this;
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder getFingerprintBuilder() {
onChanged();
return getFingerprintFieldBuilder().getBuilder();
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
public io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder getFingerprintOrBuilder() {
if (fingerprintBuilder_ != null) {
return fingerprintBuilder_.getMessageOrBuilder();
} else {
return fingerprint_ == null ?
io.opencannabis.schema.crypto.primitives.integrity.Hash.getDefaultInstance() : fingerprint_;
}
}
/**
*
* Cryptographic fingerprint of the transmitted certificate data.
*
*
* .opencannabis.crypto.Hash fingerprint = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder>
getFingerprintFieldBuilder() {
if (fingerprintBuilder_ == null) {
fingerprintBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.primitives.integrity.Hash, io.opencannabis.schema.crypto.primitives.integrity.Hash.Builder, io.opencannabis.schema.crypto.primitives.integrity.HashOrBuilder>(
getFingerprint(),
getParentForChildren(),
isClean());
fingerprint_ = null;
}
return fingerprintBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant issued_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> issuedBuilder_;
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public boolean hasIssued() {
return issuedBuilder_ != null || issued_ != null;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued() {
if (issuedBuilder_ == null) {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
} else {
return issuedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public Builder setIssued(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (issuedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
issued_ = value;
onChanged();
} else {
issuedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public Builder setIssued(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (issuedBuilder_ == null) {
issued_ = builderForValue.build();
onChanged();
} else {
issuedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public Builder mergeIssued(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (issuedBuilder_ == null) {
if (issued_ != null) {
issued_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(issued_).mergeFrom(value).buildPartial();
} else {
issued_ = value;
}
onChanged();
} else {
issuedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public Builder clearIssued() {
if (issuedBuilder_ == null) {
issued_ = null;
onChanged();
} else {
issued_ = null;
issuedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getIssuedBuilder() {
onChanged();
return getIssuedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder() {
if (issuedBuilder_ != null) {
return issuedBuilder_.getMessageOrBuilder();
} else {
return issued_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
}
}
/**
*
* Timestamp for when this assertion ticket was deemed valid.
*
*
* .opencannabis.temporal.Instant issued = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getIssuedFieldBuilder() {
if (issuedBuilder_ == null) {
issuedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getIssued(),
getParentForChildren(),
isClean());
issued_ = null;
}
return issuedBuilder_;
}
private java.lang.Object signature_ = "";
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public java.lang.String getSignature() {
java.lang.Object ref = signature_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signature_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public com.google.protobuf.ByteString
getSignatureBytes() {
java.lang.Object ref = signature_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signature_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public Builder setSignature(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signature_ = value;
onChanged();
return this;
}
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public Builder clearSignature() {
signature_ = getDefaultInstance().getSignature();
onChanged();
return this;
}
/**
*
* Signature from a node key assuring the ticket, encoded in hex.
*
*
* string signature = 3;
*/
public Builder setSignatureBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signature_ = value;
onChanged();
return this;
}
private java.lang.Object assurer_ = "";
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public java.lang.String getAssurer() {
java.lang.Object ref = assurer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
assurer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public com.google.protobuf.ByteString
getAssurerBytes() {
java.lang.Object ref = assurer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
assurer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public Builder setAssurer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
assurer_ = value;
onChanged();
return this;
}
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public Builder clearAssurer() {
assurer_ = getDefaultInstance().getAssurer();
onChanged();
return this;
}
/**
*
* Key ID of the assuring system node (regular identity reference, i.e. public key hash).
*
*
* string assurer = 4;
*/
public Builder setAssurerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
assurer_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.AssertionTicket)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.AssertionTicket)
private static final io.bloombox.schema.ledger.Accounts.AssertionTicket DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.AssertionTicket();
}
public static io.bloombox.schema.ledger.Accounts.AssertionTicket getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AssertionTicket parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AssertionTicket(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.AssertionTicket getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IdentityClaimOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.ledger.IdentityClaim)
com.google.protobuf.MessageOrBuilder {
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
java.lang.String getId();
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
java.lang.String getKey();
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
java.lang.String getSerial();
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
com.google.protobuf.ByteString
getSerialBytes();
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
java.lang.String getFingerprint();
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
com.google.protobuf.ByteString
getFingerprintBytes();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
boolean hasCertificate();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate();
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
boolean hasActor();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
io.bloombox.schema.ledger.Accounts.ActorKey getActor();
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder();
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
boolean hasAssertion();
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
io.bloombox.schema.ledger.Accounts.AssertionTicket getAssertion();
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder getAssertionOrBuilder();
}
/**
*
* Stored record specifying an asserted and certified identity claim on the ledger. Actors are required to register
* their identity before transacting, by generating a keypair, obtaining a certificate, and binding their key hashes
* and certificate hash to their object identifier, which is the digest of their ledger public key.
*
*
* Protobuf type {@code bloombox.ledger.IdentityClaim}
*/
public static final class IdentityClaim extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.ledger.IdentityClaim)
IdentityClaimOrBuilder {
private static final long serialVersionUID = 0L;
// Use IdentityClaim.newBuilder() to construct.
private IdentityClaim(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IdentityClaim() {
id_ = "";
key_ = "";
serial_ = "";
fingerprint_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IdentityClaim(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
serial_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
fingerprint_ = s;
break;
}
case 42: {
io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder subBuilder = null;
if (certificate_ != null) {
subBuilder = certificate_.toBuilder();
}
certificate_ = input.readMessage(io.bloombox.schema.ledger.Accounts.ActorCertificate.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(certificate_);
certificate_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.bloombox.schema.ledger.Accounts.ActorKey.Builder subBuilder = null;
if (actor_ != null) {
subBuilder = actor_.toBuilder();
}
actor_ = input.readMessage(io.bloombox.schema.ledger.Accounts.ActorKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(actor_);
actor_ = subBuilder.buildPartial();
}
break;
}
case 58: {
io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder subBuilder = null;
if (assertion_ != null) {
subBuilder = assertion_.toBuilder();
}
assertion_ = input.readMessage(io.bloombox.schema.ledger.Accounts.AssertionTicket.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(assertion_);
assertion_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_IdentityClaim_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_IdentityClaim_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.IdentityClaim.class, io.bloombox.schema.ledger.Accounts.IdentityClaim.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KEY_FIELD_NUMBER = 2;
private volatile java.lang.Object key_;
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERIAL_FIELD_NUMBER = 3;
private volatile java.lang.Object serial_;
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public java.lang.String getSerial() {
java.lang.Object ref = serial_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serial_ = s;
return s;
}
}
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public com.google.protobuf.ByteString
getSerialBytes() {
java.lang.Object ref = serial_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serial_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FINGERPRINT_FIELD_NUMBER = 4;
private volatile java.lang.Object fingerprint_;
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CERTIFICATE_FIELD_NUMBER = 5;
private io.bloombox.schema.ledger.Accounts.ActorCertificate certificate_;
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public boolean hasCertificate() {
return certificate_ != null;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate() {
return certificate_ == null ? io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder() {
return getCertificate();
}
public static final int ACTOR_FIELD_NUMBER = 6;
private io.bloombox.schema.ledger.Accounts.ActorKey actor_;
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public boolean hasActor() {
return actor_ != null;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey getActor() {
return actor_ == null ? io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder() {
return getActor();
}
public static final int ASSERTION_FIELD_NUMBER = 7;
private io.bloombox.schema.ledger.Accounts.AssertionTicket assertion_;
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public boolean hasAssertion() {
return assertion_ != null;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public io.bloombox.schema.ledger.Accounts.AssertionTicket getAssertion() {
return assertion_ == null ? io.bloombox.schema.ledger.Accounts.AssertionTicket.getDefaultInstance() : assertion_;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder getAssertionOrBuilder() {
return getAssertion();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, key_);
}
if (!getSerialBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, serial_);
}
if (!getFingerprintBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, fingerprint_);
}
if (certificate_ != null) {
output.writeMessage(5, getCertificate());
}
if (actor_ != null) {
output.writeMessage(6, getActor());
}
if (assertion_ != null) {
output.writeMessage(7, getAssertion());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, key_);
}
if (!getSerialBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, serial_);
}
if (!getFingerprintBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, fingerprint_);
}
if (certificate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getCertificate());
}
if (actor_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getActor());
}
if (assertion_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getAssertion());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.ledger.Accounts.IdentityClaim)) {
return super.equals(obj);
}
io.bloombox.schema.ledger.Accounts.IdentityClaim other = (io.bloombox.schema.ledger.Accounts.IdentityClaim) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getKey()
.equals(other.getKey())) return false;
if (!getSerial()
.equals(other.getSerial())) return false;
if (!getFingerprint()
.equals(other.getFingerprint())) return false;
if (hasCertificate() != other.hasCertificate()) return false;
if (hasCertificate()) {
if (!getCertificate()
.equals(other.getCertificate())) return false;
}
if (hasActor() != other.hasActor()) return false;
if (hasActor()) {
if (!getActor()
.equals(other.getActor())) return false;
}
if (hasAssertion() != other.hasAssertion()) return false;
if (hasAssertion()) {
if (!getAssertion()
.equals(other.getAssertion())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (37 * hash) + SERIAL_FIELD_NUMBER;
hash = (53 * hash) + getSerial().hashCode();
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
if (hasCertificate()) {
hash = (37 * hash) + CERTIFICATE_FIELD_NUMBER;
hash = (53 * hash) + getCertificate().hashCode();
}
if (hasActor()) {
hash = (37 * hash) + ACTOR_FIELD_NUMBER;
hash = (53 * hash) + getActor().hashCode();
}
if (hasAssertion()) {
hash = (37 * hash) + ASSERTION_FIELD_NUMBER;
hash = (53 * hash) + getAssertion().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.ledger.Accounts.IdentityClaim prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Stored record specifying an asserted and certified identity claim on the ledger. Actors are required to register
* their identity before transacting, by generating a keypair, obtaining a certificate, and binding their key hashes
* and certificate hash to their object identifier, which is the digest of their ledger public key.
*
*
* Protobuf type {@code bloombox.ledger.IdentityClaim}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.ledger.IdentityClaim)
io.bloombox.schema.ledger.Accounts.IdentityClaimOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_IdentityClaim_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_IdentityClaim_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.ledger.Accounts.IdentityClaim.class, io.bloombox.schema.ledger.Accounts.IdentityClaim.Builder.class);
}
// Construct using io.bloombox.schema.ledger.Accounts.IdentityClaim.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
key_ = "";
serial_ = "";
fingerprint_ = "";
if (certificateBuilder_ == null) {
certificate_ = null;
} else {
certificate_ = null;
certificateBuilder_ = null;
}
if (actorBuilder_ == null) {
actor_ = null;
} else {
actor_ = null;
actorBuilder_ = null;
}
if (assertionBuilder_ == null) {
assertion_ = null;
} else {
assertion_ = null;
assertionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.ledger.Accounts.internal_static_bloombox_ledger_IdentityClaim_descriptor;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.IdentityClaim getDefaultInstanceForType() {
return io.bloombox.schema.ledger.Accounts.IdentityClaim.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.IdentityClaim build() {
io.bloombox.schema.ledger.Accounts.IdentityClaim result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.IdentityClaim buildPartial() {
io.bloombox.schema.ledger.Accounts.IdentityClaim result = new io.bloombox.schema.ledger.Accounts.IdentityClaim(this);
result.id_ = id_;
result.key_ = key_;
result.serial_ = serial_;
result.fingerprint_ = fingerprint_;
if (certificateBuilder_ == null) {
result.certificate_ = certificate_;
} else {
result.certificate_ = certificateBuilder_.build();
}
if (actorBuilder_ == null) {
result.actor_ = actor_;
} else {
result.actor_ = actorBuilder_.build();
}
if (assertionBuilder_ == null) {
result.assertion_ = assertion_;
} else {
result.assertion_ = assertionBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.ledger.Accounts.IdentityClaim) {
return mergeFrom((io.bloombox.schema.ledger.Accounts.IdentityClaim)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.ledger.Accounts.IdentityClaim other) {
if (other == io.bloombox.schema.ledger.Accounts.IdentityClaim.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (!other.getSerial().isEmpty()) {
serial_ = other.serial_;
onChanged();
}
if (!other.getFingerprint().isEmpty()) {
fingerprint_ = other.fingerprint_;
onChanged();
}
if (other.hasCertificate()) {
mergeCertificate(other.getCertificate());
}
if (other.hasActor()) {
mergeActor(other.getActor());
}
if (other.hasAssertion()) {
mergeAssertion(other.getAssertion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.ledger.Accounts.IdentityClaim parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.ledger.Accounts.IdentityClaim) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* The account ID is the computed result of UPPER(SHA3-256-B58(public_ledger_key)).
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object key_ = "";
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(public_ec_key)).
*
*
* string key = 2;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private java.lang.Object serial_ = "";
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public java.lang.String getSerial() {
java.lang.Object ref = serial_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serial_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public com.google.protobuf.ByteString
getSerialBytes() {
java.lang.Object ref = serial_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serial_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public Builder setSerial(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serial_ = value;
onChanged();
return this;
}
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public Builder clearSerial() {
serial_ = getDefaultInstance().getSerial();
onChanged();
return this;
}
/**
*
* Serial number for the attached actor certificate.
*
*
* string serial = 3;
*/
public Builder setSerialBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serial_ = value;
onChanged();
return this;
}
private java.lang.Object fingerprint_ = "";
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public com.google.protobuf.ByteString
getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public Builder setFingerprint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
onChanged();
return this;
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public Builder clearFingerprint() {
fingerprint_ = getDefaultInstance().getFingerprint();
onChanged();
return this;
}
/**
*
* Specifies the computed result of UPPER(SHA3-256-B58(certificate)).
*
*
* string fingerprint = 4;
*/
public Builder setFingerprintBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fingerprint_ = value;
onChanged();
return this;
}
private io.bloombox.schema.ledger.Accounts.ActorCertificate certificate_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder> certificateBuilder_;
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public boolean hasCertificate() {
return certificateBuilder_ != null || certificate_ != null;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate getCertificate() {
if (certificateBuilder_ == null) {
return certificate_ == null ? io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
} else {
return certificateBuilder_.getMessage();
}
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public Builder setCertificate(io.bloombox.schema.ledger.Accounts.ActorCertificate value) {
if (certificateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
certificate_ = value;
onChanged();
} else {
certificateBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public Builder setCertificate(
io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder builderForValue) {
if (certificateBuilder_ == null) {
certificate_ = builderForValue.build();
onChanged();
} else {
certificateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public Builder mergeCertificate(io.bloombox.schema.ledger.Accounts.ActorCertificate value) {
if (certificateBuilder_ == null) {
if (certificate_ != null) {
certificate_ =
io.bloombox.schema.ledger.Accounts.ActorCertificate.newBuilder(certificate_).mergeFrom(value).buildPartial();
} else {
certificate_ = value;
}
onChanged();
} else {
certificateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public Builder clearCertificate() {
if (certificateBuilder_ == null) {
certificate_ = null;
onChanged();
} else {
certificate_ = null;
certificateBuilder_ = null;
}
return this;
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder getCertificateBuilder() {
onChanged();
return getCertificateFieldBuilder().getBuilder();
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
public io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder getCertificateOrBuilder() {
if (certificateBuilder_ != null) {
return certificateBuilder_.getMessageOrBuilder();
} else {
return certificate_ == null ?
io.bloombox.schema.ledger.Accounts.ActorCertificate.getDefaultInstance() : certificate_;
}
}
/**
*
* Specifies actual backing certificate information, issued by the central ledger PKI CA.
*
*
* .bloombox.ledger.ActorCertificate certificate = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder>
getCertificateFieldBuilder() {
if (certificateBuilder_ == null) {
certificateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorCertificate, io.bloombox.schema.ledger.Accounts.ActorCertificate.Builder, io.bloombox.schema.ledger.Accounts.ActorCertificateOrBuilder>(
getCertificate(),
getParentForChildren(),
isClean());
certificate_ = null;
}
return certificateBuilder_;
}
private io.bloombox.schema.ledger.Accounts.ActorKey actor_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder> actorBuilder_;
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public boolean hasActor() {
return actorBuilder_ != null || actor_ != null;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey getActor() {
if (actorBuilder_ == null) {
return actor_ == null ? io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
} else {
return actorBuilder_.getMessage();
}
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public Builder setActor(io.bloombox.schema.ledger.Accounts.ActorKey value) {
if (actorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
actor_ = value;
onChanged();
} else {
actorBuilder_.setMessage(value);
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public Builder setActor(
io.bloombox.schema.ledger.Accounts.ActorKey.Builder builderForValue) {
if (actorBuilder_ == null) {
actor_ = builderForValue.build();
onChanged();
} else {
actorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public Builder mergeActor(io.bloombox.schema.ledger.Accounts.ActorKey value) {
if (actorBuilder_ == null) {
if (actor_ != null) {
actor_ =
io.bloombox.schema.ledger.Accounts.ActorKey.newBuilder(actor_).mergeFrom(value).buildPartial();
} else {
actor_ = value;
}
onChanged();
} else {
actorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public Builder clearActor() {
if (actorBuilder_ == null) {
actor_ = null;
onChanged();
} else {
actor_ = null;
actorBuilder_ = null;
}
return this;
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public io.bloombox.schema.ledger.Accounts.ActorKey.Builder getActorBuilder() {
onChanged();
return getActorFieldBuilder().getBuilder();
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
public io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder getActorOrBuilder() {
if (actorBuilder_ != null) {
return actorBuilder_.getMessageOrBuilder();
} else {
return actor_ == null ?
io.bloombox.schema.ledger.Accounts.ActorKey.getDefaultInstance() : actor_;
}
}
/**
*
* The actor key links this particular account key to/from a known actor in the system, be it an organization or an
* individual. Actor keys keys may be encrypted.
*
*
* .bloombox.ledger.ActorKey actor = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder>
getActorFieldBuilder() {
if (actorBuilder_ == null) {
actorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.ActorKey, io.bloombox.schema.ledger.Accounts.ActorKey.Builder, io.bloombox.schema.ledger.Accounts.ActorKeyOrBuilder>(
getActor(),
getParentForChildren(),
isClean());
actor_ = null;
}
return actorBuilder_;
}
private io.bloombox.schema.ledger.Accounts.AssertionTicket assertion_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AssertionTicket, io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder, io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder> assertionBuilder_;
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public boolean hasAssertion() {
return assertionBuilder_ != null || assertion_ != null;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public io.bloombox.schema.ledger.Accounts.AssertionTicket getAssertion() {
if (assertionBuilder_ == null) {
return assertion_ == null ? io.bloombox.schema.ledger.Accounts.AssertionTicket.getDefaultInstance() : assertion_;
} else {
return assertionBuilder_.getMessage();
}
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public Builder setAssertion(io.bloombox.schema.ledger.Accounts.AssertionTicket value) {
if (assertionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
assertion_ = value;
onChanged();
} else {
assertionBuilder_.setMessage(value);
}
return this;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public Builder setAssertion(
io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder builderForValue) {
if (assertionBuilder_ == null) {
assertion_ = builderForValue.build();
onChanged();
} else {
assertionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public Builder mergeAssertion(io.bloombox.schema.ledger.Accounts.AssertionTicket value) {
if (assertionBuilder_ == null) {
if (assertion_ != null) {
assertion_ =
io.bloombox.schema.ledger.Accounts.AssertionTicket.newBuilder(assertion_).mergeFrom(value).buildPartial();
} else {
assertion_ = value;
}
onChanged();
} else {
assertionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public Builder clearAssertion() {
if (assertionBuilder_ == null) {
assertion_ = null;
onChanged();
} else {
assertion_ = null;
assertionBuilder_ = null;
}
return this;
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder getAssertionBuilder() {
onChanged();
return getAssertionFieldBuilder().getBuilder();
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
public io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder getAssertionOrBuilder() {
if (assertionBuilder_ != null) {
return assertionBuilder_.getMessageOrBuilder();
} else {
return assertion_ == null ?
io.bloombox.schema.ledger.Accounts.AssertionTicket.getDefaultInstance() : assertion_;
}
}
/**
*
* Assertion ticket, verifying that this was indeed verified by the server upon submission.
*
*
* .bloombox.ledger.AssertionTicket assertion = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AssertionTicket, io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder, io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder>
getAssertionFieldBuilder() {
if (assertionBuilder_ == null) {
assertionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.ledger.Accounts.AssertionTicket, io.bloombox.schema.ledger.Accounts.AssertionTicket.Builder, io.bloombox.schema.ledger.Accounts.AssertionTicketOrBuilder>(
getAssertion(),
getParentForChildren(),
isClean());
assertion_ = null;
}
return assertionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.ledger.IdentityClaim)
}
// @@protoc_insertion_point(class_scope:bloombox.ledger.IdentityClaim)
private static final io.bloombox.schema.ledger.Accounts.IdentityClaim DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.ledger.Accounts.IdentityClaim();
}
public static io.bloombox.schema.ledger.Accounts.IdentityClaim getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IdentityClaim parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IdentityClaim(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.ledger.Accounts.IdentityClaim getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_ActorCertificate_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_ActorCertificate_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_ActorKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_ActorKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_AccountKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_AccountKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_LedgerIdentity_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_LedgerIdentity_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_AssertionTicket_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_AssertionTicket_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_ledger_IdentityClaim_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_ledger_IdentityClaim_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024ledger/Account.proto\022\017bloombox.ledger\032" +
"\024core/Datamodel.proto\032\021ledger/Node.proto" +
"\032\026temporal/Instant.proto\032\026identity/UserK" +
"ey.proto\032\030partner/PartnerKey.proto\032\033part" +
"ner/PartnerDevice.proto\032\031partner/Locatio" +
"nKey.proto\032\034crypto/primitives/Keys.proto" +
"\032!crypto/primitives/Integrity.proto\"\266\001\n\020" +
"ActorCertificate\022\n\n\002id\030\001 \001(\t\022\016\n\006serial\030\002" +
" \001(\t\022.\n\013fingerprint\030\003 \001(\0132\031.opencannabis" +
".crypto.Hash\022\023\n\013common_name\030\004 \001(\t\022\023\n\013iss" +
"uer_name\030\005 \001(\t\022\016\n\004data\030\n \001(\014H\000\022\021\n\007encode" +
"d\030\013 \001(\tH\000B\t\n\007payload\"\260\002\n\010ActorKey\022.\n\005kno" +
"wn\030\001 \001(\0162\035.bloombox.ledger.SpecialActorH" +
"\000\022*\n\004user\030\n \001(\0132\032.bloombox.identity.User" +
"KeyH\000\022/\n\007partner\030\024 \001(\0132\034.bloombox.partne" +
"r.PartnerKeyH\000\0221\n\010location\030\036 \001(\0132\035.bloom" +
"box.partner.LocationKeyH\000\0224\n\006device\030( \001(" +
"\0132\".bloombox.partner.PartnerDeviceKeyH\000\022" +
"%\n\004node\0302 \001(\0132\025.bloombox.ledger.NodeH\000B\007" +
"\n\005actor\"|\n\nAccountKey\022\022\n\002id\030\001 \001(\tB\006\302\265\003\002\010" +
"\002\022*\n\004pair\030\002 \001(\0132\034.opencannabis.crypto.Ke" +
"ypair\022.\n\010identity\030\003 \001(\0132\034.opencannabis.c" +
"rypto.Keypair\"\266\001\n\016LedgerIdentity\0220\n\003key\030" +
"\001 \001(\0132\033.bloombox.ledger.AccountKeyB\006\302\265\003\002" +
"\010\001\022(\n\005actor\030\002 \001(\0132\031.bloombox.ledger.Acto" +
"rKey\0226\n\013certificate\030\003 \001(\0132!.bloombox.led" +
"ger.ActorCertificate:\020\202\367\002\014\010\002\022\010keypairs\"\225" +
"\001\n\017AssertionTicket\022.\n\013fingerprint\030\001 \001(\0132" +
"\031.opencannabis.crypto.Hash\022.\n\006issued\030\002 \001" +
"(\0132\036.opencannabis.temporal.Instant\022\021\n\tsi" +
"gnature\030\003 \001(\t\022\017\n\007assurer\030\004 \001(\t\"\374\001\n\rIdent" +
"ityClaim\022\022\n\002id\030\001 \001(\tB\006\302\265\003\002\010\002\022\013\n\003key\030\002 \001(" +
"\t\022\016\n\006serial\030\003 \001(\t\022\023\n\013fingerprint\030\004 \001(\t\0226" +
"\n\013certificate\030\005 \001(\0132!.bloombox.ledger.Ac" +
"torCertificate\022(\n\005actor\030\006 \001(\0132\031.bloombox" +
".ledger.ActorKey\0223\n\tassertion\030\007 \001(\0132 .bl" +
"oombox.ledger.AssertionTicket:\016\202\367\002\n\010\002\022\006c" +
"laims*\'\n\014SpecialActor\022\n\n\006SYSTEM\020\000\022\013\n\007SAN" +
"DBOX\020\001B/\n\031io.bloombox.schema.ledgerB\010Acc" +
"ountsH\001P\000\242\002\003BBSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.bloombox.schema.ledger.LedgerNode.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
io.bloombox.schema.identity.AppUserKey.getDescriptor(),
io.bloombox.schema.partner.PartnerMeta.getDescriptor(),
io.bloombox.schema.partner.PartnerDevices.getDescriptor(),
io.bloombox.schema.partner.LocationAccountKey.getDescriptor(),
io.opencannabis.schema.crypto.primitives.Keys.getDescriptor(),
io.opencannabis.schema.crypto.primitives.integrity.Integrity.getDescriptor(),
}, assigner);
internal_static_bloombox_ledger_ActorCertificate_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_ledger_ActorCertificate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_ActorCertificate_descriptor,
new java.lang.String[] { "Id", "Serial", "Fingerprint", "CommonName", "IssuerName", "Data", "Encoded", "Payload", });
internal_static_bloombox_ledger_ActorKey_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_ledger_ActorKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_ActorKey_descriptor,
new java.lang.String[] { "Known", "User", "Partner", "Location", "Device", "Node", "Actor", });
internal_static_bloombox_ledger_AccountKey_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_bloombox_ledger_AccountKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_AccountKey_descriptor,
new java.lang.String[] { "Id", "Pair", "Identity", });
internal_static_bloombox_ledger_LedgerIdentity_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_bloombox_ledger_LedgerIdentity_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_LedgerIdentity_descriptor,
new java.lang.String[] { "Key", "Actor", "Certificate", });
internal_static_bloombox_ledger_AssertionTicket_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_bloombox_ledger_AssertionTicket_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_AssertionTicket_descriptor,
new java.lang.String[] { "Fingerprint", "Issued", "Signature", "Assurer", });
internal_static_bloombox_ledger_IdentityClaim_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_bloombox_ledger_IdentityClaim_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_ledger_IdentityClaim_descriptor,
new java.lang.String[] { "Id", "Key", "Serial", "Fingerprint", "Certificate", "Actor", "Assertion", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.db);
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.bloombox.schema.ledger.LedgerNode.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
io.bloombox.schema.identity.AppUserKey.getDescriptor();
io.bloombox.schema.partner.PartnerMeta.getDescriptor();
io.bloombox.schema.partner.PartnerDevices.getDescriptor();
io.bloombox.schema.partner.LocationAccountKey.getDescriptor();
io.opencannabis.schema.crypto.primitives.Keys.getDescriptor();
io.opencannabis.schema.crypto.primitives.integrity.Integrity.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy