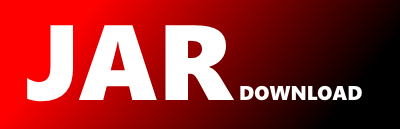
io.bloombox.schema.licensure.Licensure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: licensing/Licensure.proto
package io.bloombox.schema.licensure;
public final class Licensure {
private Licensure() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Specifies the supertypes of licenses that are supported by the system. By default, all licenses are considered
* "permanent," meaning they do not have expiration parameters built in besides regular compliance.
*
*
* Protobuf enum {@code bloombox.licensure.LicenseType}
*/
public enum LicenseType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Permanent licenses are the default supertype for a license, and indicate that a license does not, by default,
* expire based on time. Compliance with all other licensure stipulations is of course required. A license that is
* considered "permanent" may still be revoked for any reason, including time-based expiry, but such a mechanism is
* not implied to necessitate a separately license.
*
*
* PERMANENT = 0;
*/
PERMANENT(0),
/**
*
* Temporary licenses are, by nature, issued on a preliminary or temporary basis. They are frequently issued
* separately from permanent licenses and so are treated differently by OCS.
*
*
* TEMPORARY = 1;
*/
TEMPORARY(1),
/**
*
* Compound licenses allow multiple privileges in one license structure. In some jurisdictions, compound licenses are
* referred to as "microbusinesses." Many compound licensees perform numerous functions in the supply chain, and carry
* privileges for each role with their one compound license.
*
*
* COMPOUND = 2;
*/
COMPOUND(2),
UNRECOGNIZED(-1),
;
/**
*
* Permanent licenses are the default supertype for a license, and indicate that a license does not, by default,
* expire based on time. Compliance with all other licensure stipulations is of course required. A license that is
* considered "permanent" may still be revoked for any reason, including time-based expiry, but such a mechanism is
* not implied to necessitate a separately license.
*
*
* PERMANENT = 0;
*/
public static final int PERMANENT_VALUE = 0;
/**
*
* Temporary licenses are, by nature, issued on a preliminary or temporary basis. They are frequently issued
* separately from permanent licenses and so are treated differently by OCS.
*
*
* TEMPORARY = 1;
*/
public static final int TEMPORARY_VALUE = 1;
/**
*
* Compound licenses allow multiple privileges in one license structure. In some jurisdictions, compound licenses are
* referred to as "microbusinesses." Many compound licensees perform numerous functions in the supply chain, and carry
* privileges for each role with their one compound license.
*
*
* COMPOUND = 2;
*/
public static final int COMPOUND_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LicenseType valueOf(int value) {
return forNumber(value);
}
public static LicenseType forNumber(int value) {
switch (value) {
case 0: return PERMANENT;
case 1: return TEMPORARY;
case 2: return COMPOUND;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LicenseType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LicenseType findValueByNumber(int number) {
return LicenseType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.getDescriptor().getEnumTypes().get(0);
}
private static final LicenseType[] VALUES = values();
public static LicenseType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private LicenseType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.licensure.LicenseType)
}
/**
*
* Enumerates different types of cannabis licenses that relate to the larger data model. Licensing is based on the role
* of a given organization or individual in the supply chain.
*
*
* Protobuf enum {@code bloombox.licensure.LicensePrivilege}
*/
public enum LicensePrivilege
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Specifies that the license type of a given license payload is unknown. This value is not meant for explicit use and
* instead provides a backstop for parsing errors with license types.
*
*
* UNKNOWN_LICENSE = 0;
*/
UNKNOWN_LICENSE(0),
/**
*
* Specifies a cultivation license, which is defined as, a license to grow cannabis plants in some amount, to some age
* and the right to harvest those plants and sell them or pass them to a licensed distributor.
*
*
* CULTIVATION = 1;
*/
CULTIVATION(1),
/**
*
* Specifies a distribution license, which allows an organization to broker deals between producers and buyers, and is
* usually also licensed for transport. Distributors can vend to other distributors, retailers, or manufacturers, and
* are additionally responsible for testing.
*
*
* DISTRIBUTION = 2;
*/
DISTRIBUTION(2),
/**
*
* Specifies a license type that allows an organization to physically transport cannabis materials. This can include
* trucks/automobiles, trains, boats, etc, but only allows delivery to wholesale or retail actors. Transport in the
* last mile of the supply chain (from retail to consumer) is considered a 'delivery' license.
*
*
* TRANSPORT = 3;
*/
TRANSPORT(3),
/**
*
* Specifies a license to perform lab testing on cannabis products, and produce certified reports about the content
* and composition of cannabis products.
*
*
* LABORATORY = 4;
*/
LABORATORY(4),
/**
*
* Specifies a license to purchase legal cannabis precursor products, and synthesize them into secondary products, for
* instance, edibles, concentrates, cartridges, and so on.
*
*
* MANUFACTURING = 5;
*/
MANUFACTURING(5),
/**
*
* Specifies a license to vend or dispense cannabis products directly to consumers. Regular retail licensing covers
* brick and mortar locations, not delivery - at least where OCS is concerned, that is considered a different license
* type of 'delivery'. Retailers may only purchase from licensed distributors.
*
*
* RETAIL = 6;
*/
RETAIL(6),
/**
*
* Specifies a non-storefront retail license, which is to say, a license to deliver cannabis products directly to
* consumers. Often, delivery licenses are bundled with existing retail licenses. Like retail licensees, delivery
* licensees may only purchase from licensed distributors.
*
*
* DELIVERY = 7;
*/
DELIVERY(7),
/**
*
* Specifies a license to hold cannabis events. Covers only the sale of cannabis products, not necessarily the onsite
* consumption of cannabis products (must be bundled with a 'consumption') privilege.
*
*
* EVENTS = 8;
*/
EVENTS(8),
/**
*
* Specifies a license that allows consumption of cannabis at a given business premises or event. This license type
* covers cannabis lounges, cannabis bars, and cannabis festivals/events.
*
*
* CONSUMPTION = 9;
*/
CONSUMPTION(9),
UNRECOGNIZED(-1),
;
/**
*
* Specifies that the license type of a given license payload is unknown. This value is not meant for explicit use and
* instead provides a backstop for parsing errors with license types.
*
*
* UNKNOWN_LICENSE = 0;
*/
public static final int UNKNOWN_LICENSE_VALUE = 0;
/**
*
* Specifies a cultivation license, which is defined as, a license to grow cannabis plants in some amount, to some age
* and the right to harvest those plants and sell them or pass them to a licensed distributor.
*
*
* CULTIVATION = 1;
*/
public static final int CULTIVATION_VALUE = 1;
/**
*
* Specifies a distribution license, which allows an organization to broker deals between producers and buyers, and is
* usually also licensed for transport. Distributors can vend to other distributors, retailers, or manufacturers, and
* are additionally responsible for testing.
*
*
* DISTRIBUTION = 2;
*/
public static final int DISTRIBUTION_VALUE = 2;
/**
*
* Specifies a license type that allows an organization to physically transport cannabis materials. This can include
* trucks/automobiles, trains, boats, etc, but only allows delivery to wholesale or retail actors. Transport in the
* last mile of the supply chain (from retail to consumer) is considered a 'delivery' license.
*
*
* TRANSPORT = 3;
*/
public static final int TRANSPORT_VALUE = 3;
/**
*
* Specifies a license to perform lab testing on cannabis products, and produce certified reports about the content
* and composition of cannabis products.
*
*
* LABORATORY = 4;
*/
public static final int LABORATORY_VALUE = 4;
/**
*
* Specifies a license to purchase legal cannabis precursor products, and synthesize them into secondary products, for
* instance, edibles, concentrates, cartridges, and so on.
*
*
* MANUFACTURING = 5;
*/
public static final int MANUFACTURING_VALUE = 5;
/**
*
* Specifies a license to vend or dispense cannabis products directly to consumers. Regular retail licensing covers
* brick and mortar locations, not delivery - at least where OCS is concerned, that is considered a different license
* type of 'delivery'. Retailers may only purchase from licensed distributors.
*
*
* RETAIL = 6;
*/
public static final int RETAIL_VALUE = 6;
/**
*
* Specifies a non-storefront retail license, which is to say, a license to deliver cannabis products directly to
* consumers. Often, delivery licenses are bundled with existing retail licenses. Like retail licensees, delivery
* licensees may only purchase from licensed distributors.
*
*
* DELIVERY = 7;
*/
public static final int DELIVERY_VALUE = 7;
/**
*
* Specifies a license to hold cannabis events. Covers only the sale of cannabis products, not necessarily the onsite
* consumption of cannabis products (must be bundled with a 'consumption') privilege.
*
*
* EVENTS = 8;
*/
public static final int EVENTS_VALUE = 8;
/**
*
* Specifies a license that allows consumption of cannabis at a given business premises or event. This license type
* covers cannabis lounges, cannabis bars, and cannabis festivals/events.
*
*
* CONSUMPTION = 9;
*/
public static final int CONSUMPTION_VALUE = 9;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LicensePrivilege valueOf(int value) {
return forNumber(value);
}
public static LicensePrivilege forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_LICENSE;
case 1: return CULTIVATION;
case 2: return DISTRIBUTION;
case 3: return TRANSPORT;
case 4: return LABORATORY;
case 5: return MANUFACTURING;
case 6: return RETAIL;
case 7: return DELIVERY;
case 8: return EVENTS;
case 9: return CONSUMPTION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LicensePrivilege> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LicensePrivilege findValueByNumber(int number) {
return LicensePrivilege.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.getDescriptor().getEnumTypes().get(1);
}
private static final LicensePrivilege[] VALUES = values();
public static LicensePrivilege valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private LicensePrivilege(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.licensure.LicensePrivilege)
}
/**
*
* Specifies states that a given license may exist in. Expiration, revocation, and other actions that may deny access,
* are enumerated here with descriptions.
*
*
* Protobuf enum {@code bloombox.licensure.LicenseStatus}
*/
public enum LicenseStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Specifies a license status that is unknown, or pending review or action to verify.
*
*
* PENDING = 0;
*/
PENDING(0),
/**
*
* Specifies an active license that grants specified privileges, stipulating the privileges being active and valid for
* the time the subject license is in this status.
*
*
* ACTIVE = 1;
*/
ACTIVE(1),
/**
*
* Specifies a license state where, for reasons other than regular expiry, the subject license is to be considered
* no-longer-valid, with no ability to leverage or assert the privileges granted in the license. If the license is
* superseded by a new license (or a permanent license issued for a previously-temporary licensee), the status
* 'superseded' is used in place of this (implying that 'revoked') is only used for punitive license states. This
* license state is considered terminal.
*
*
* REVOKED = 2;
*/
REVOKED(2),
/**
*
* Specifies a license state where, due to time constraints placed on the license, it is no longer considered valid.
* Expired licenses do not imply any punitive action directed at the licensee. Expiration is a terminal status.
*
*
* EXPIRED = 3;
*/
EXPIRED(3),
/**
*
* Specifies a license that is temporarily not-to-be-considered-valid. In cases where remediation activities must be
* enforced by a regulatory agency, a license may be placed in this state for some period of time. Suspension is not
* considered a terminal state for the subject license or licensee and does not necessarily imply punitive action.
*
*
* SUSPENDED = 4;
*/
SUSPENDED(4),
/**
*
* Specifies that a license is no longer considered valid, but that the license has been superseded by another, valid
* license, that may grant a different set of privileges (but never a subset, lest this subject license be considered
* 'revoked' rather than 'superseded').
*
*
* SUPERSEDED = 5;
*/
SUPERSEDED(5),
UNRECOGNIZED(-1),
;
/**
*
* Specifies a license status that is unknown, or pending review or action to verify.
*
*
* PENDING = 0;
*/
public static final int PENDING_VALUE = 0;
/**
*
* Specifies an active license that grants specified privileges, stipulating the privileges being active and valid for
* the time the subject license is in this status.
*
*
* ACTIVE = 1;
*/
public static final int ACTIVE_VALUE = 1;
/**
*
* Specifies a license state where, for reasons other than regular expiry, the subject license is to be considered
* no-longer-valid, with no ability to leverage or assert the privileges granted in the license. If the license is
* superseded by a new license (or a permanent license issued for a previously-temporary licensee), the status
* 'superseded' is used in place of this (implying that 'revoked') is only used for punitive license states. This
* license state is considered terminal.
*
*
* REVOKED = 2;
*/
public static final int REVOKED_VALUE = 2;
/**
*
* Specifies a license state where, due to time constraints placed on the license, it is no longer considered valid.
* Expired licenses do not imply any punitive action directed at the licensee. Expiration is a terminal status.
*
*
* EXPIRED = 3;
*/
public static final int EXPIRED_VALUE = 3;
/**
*
* Specifies a license that is temporarily not-to-be-considered-valid. In cases where remediation activities must be
* enforced by a regulatory agency, a license may be placed in this state for some period of time. Suspension is not
* considered a terminal state for the subject license or licensee and does not necessarily imply punitive action.
*
*
* SUSPENDED = 4;
*/
public static final int SUSPENDED_VALUE = 4;
/**
*
* Specifies that a license is no longer considered valid, but that the license has been superseded by another, valid
* license, that may grant a different set of privileges (but never a subset, lest this subject license be considered
* 'revoked' rather than 'superseded').
*
*
* SUPERSEDED = 5;
*/
public static final int SUPERSEDED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LicenseStatus valueOf(int value) {
return forNumber(value);
}
public static LicenseStatus forNumber(int value) {
switch (value) {
case 0: return PENDING;
case 1: return ACTIVE;
case 2: return REVOKED;
case 3: return EXPIRED;
case 4: return SUSPENDED;
case 5: return SUPERSEDED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LicenseStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LicenseStatus findValueByNumber(int number) {
return LicenseStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.getDescriptor().getEnumTypes().get(2);
}
private static final LicenseStatus[] VALUES = values();
public static LicenseStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private LicenseStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.licensure.LicenseStatus)
}
public interface LicensingAuthorityOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicensingAuthority)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
boolean hasLocal();
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getLocal();
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder getLocalOrBuilder();
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
boolean hasCounty();
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getCounty();
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder getCountyOrBuilder();
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
boolean hasState();
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getState();
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder getStateOrBuilder();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.JurisdictionCase getJurisdictionCase();
}
/**
*
* Specifies the jurisdictional authority that issued or is in the process of issuing a license. Jurisdiction is a
* hierarchical concept, and so, this structure is filled out according to the "leaf" jurisdiction that actually issued
* the subject license.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority}
*/
public static final class LicensingAuthority extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicensingAuthority)
LicensingAuthorityOrBuilder {
private static final long serialVersionUID = 0L;
// Use LicensingAuthority.newBuilder() to construct.
private LicensingAuthority(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LicensingAuthority() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LicensingAuthority(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder subBuilder = null;
if (jurisdictionCase_ == 1) {
subBuilder = ((io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_).toBuilder();
}
jurisdiction_ =
input.readMessage(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_);
jurisdiction_ = subBuilder.buildPartial();
}
jurisdictionCase_ = 1;
break;
}
case 18: {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder subBuilder = null;
if (jurisdictionCase_ == 2) {
subBuilder = ((io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_).toBuilder();
}
jurisdiction_ =
input.readMessage(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_);
jurisdiction_ = subBuilder.buildPartial();
}
jurisdictionCase_ = 2;
break;
}
case 26: {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder subBuilder = null;
if (jurisdictionCase_ == 3) {
subBuilder = ((io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_).toBuilder();
}
jurisdiction_ =
input.readMessage(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_);
jurisdiction_ = subBuilder.buildPartial();
}
jurisdictionCase_ = 3;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder.class);
}
public interface LocalLicenseOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicensingAuthority.LocalLicense)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
java.lang.String getMunicipality();
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
com.google.protobuf.ByteString
getMunicipalityBytes();
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
java.lang.String getCountyName();
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
com.google.protobuf.ByteString
getCountyNameBytes();
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
int getCaliforniaCountyValue();
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty();
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
java.lang.String getProvinceName();
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
com.google.protobuf.ByteString
getProvinceNameBytes();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
int getUsStateValue();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
io.opencannabis.schema.geo.usa.USState getUsState();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.CountyCase getCountyCase();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.ProvinceCase getProvinceCase();
}
/**
*
* Specifies the concept of a local licensing authority, meaning a municipality with the statutory authority to
* authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.LocalLicense}
*/
public static final class LocalLicense extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicensingAuthority.LocalLicense)
LocalLicenseOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocalLicense.newBuilder() to construct.
private LocalLicense(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocalLicense() {
municipality_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocalLicense(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
municipality_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
countyCase_ = 2;
county_ = s;
break;
}
case 24: {
int rawValue = input.readEnum();
countyCase_ = 3;
county_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
provinceCase_ = 4;
province_ = s;
break;
}
case 40: {
int rawValue = input.readEnum();
provinceCase_ = 5;
province_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder.class);
}
private int countyCase_ = 0;
private java.lang.Object county_;
public enum CountyCase
implements com.google.protobuf.Internal.EnumLite {
COUNTY_NAME(2),
CALIFORNIA_COUNTY(3),
COUNTY_NOT_SET(0);
private final int value;
private CountyCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CountyCase valueOf(int value) {
return forNumber(value);
}
public static CountyCase forNumber(int value) {
switch (value) {
case 2: return COUNTY_NAME;
case 3: return CALIFORNIA_COUNTY;
case 0: return COUNTY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CountyCase
getCountyCase() {
return CountyCase.forNumber(
countyCase_);
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public enum ProvinceCase
implements com.google.protobuf.Internal.EnumLite {
PROVINCE_NAME(4),
US_STATE(5),
PROVINCE_NOT_SET(0);
private final int value;
private ProvinceCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProvinceCase valueOf(int value) {
return forNumber(value);
}
public static ProvinceCase forNumber(int value) {
switch (value) {
case 4: return PROVINCE_NAME;
case 5: return US_STATE;
case 0: return PROVINCE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
public static final int MUNICIPALITY_FIELD_NUMBER = 1;
private volatile java.lang.Object municipality_;
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public java.lang.String getMunicipality() {
java.lang.Object ref = municipality_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
municipality_ = s;
return s;
}
}
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public com.google.protobuf.ByteString
getMunicipalityBytes() {
java.lang.Object ref = municipality_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
municipality_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COUNTY_NAME_FIELD_NUMBER = 2;
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public java.lang.String getCountyName() {
java.lang.Object ref = "";
if (countyCase_ == 2) {
ref = county_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (countyCase_ == 2) {
county_ = s;
}
return s;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public com.google.protobuf.ByteString
getCountyNameBytes() {
java.lang.Object ref = "";
if (countyCase_ == 2) {
ref = county_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (countyCase_ == 2) {
county_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALIFORNIA_COUNTY_FIELD_NUMBER = 3;
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public int getCaliforniaCountyValue() {
if (countyCase_ == 3) {
return (java.lang.Integer) county_;
}
return 0;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty() {
if (countyCase_ == 3) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.California.CaliforniaCounty result = io.opencannabis.schema.geo.usa.California.CaliforniaCounty.valueOf(
(java.lang.Integer) county_);
return result == null ? io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNKNOWN_COUNTY;
}
public static final int PROVINCE_NAME_FIELD_NUMBER = 4;
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 4) {
province_ = s;
}
return s;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 4) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int US_STATE_FIELD_NUMBER = 5;
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public int getUsStateValue() {
if (provinceCase_ == 5) {
return (java.lang.Integer) province_;
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 5) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getMunicipalityBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, municipality_);
}
if (countyCase_ == 2) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, county_);
}
if (countyCase_ == 3) {
output.writeEnum(3, ((java.lang.Integer) county_));
}
if (provinceCase_ == 4) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, province_);
}
if (provinceCase_ == 5) {
output.writeEnum(5, ((java.lang.Integer) province_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getMunicipalityBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, municipality_);
}
if (countyCase_ == 2) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, county_);
}
if (countyCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, ((java.lang.Integer) county_));
}
if (provinceCase_ == 4) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, province_);
}
if (provinceCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, ((java.lang.Integer) province_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense other = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) obj;
if (!getMunicipality()
.equals(other.getMunicipality())) return false;
if (!getCountyCase().equals(other.getCountyCase())) return false;
switch (countyCase_) {
case 2:
if (!getCountyName()
.equals(other.getCountyName())) return false;
break;
case 3:
if (getCaliforniaCountyValue()
!= other.getCaliforniaCountyValue()) return false;
break;
case 0:
default:
}
if (!getProvinceCase().equals(other.getProvinceCase())) return false;
switch (provinceCase_) {
case 4:
if (!getProvinceName()
.equals(other.getProvinceName())) return false;
break;
case 5:
if (getUsStateValue()
!= other.getUsStateValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MUNICIPALITY_FIELD_NUMBER;
hash = (53 * hash) + getMunicipality().hashCode();
switch (countyCase_) {
case 2:
hash = (37 * hash) + COUNTY_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCountyName().hashCode();
break;
case 3:
hash = (37 * hash) + CALIFORNIA_COUNTY_FIELD_NUMBER;
hash = (53 * hash) + getCaliforniaCountyValue();
break;
case 0:
default:
}
switch (provinceCase_) {
case 4:
hash = (37 * hash) + PROVINCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getProvinceName().hashCode();
break;
case 5:
hash = (37 * hash) + US_STATE_FIELD_NUMBER;
hash = (53 * hash) + getUsStateValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the concept of a local licensing authority, meaning a municipality with the statutory authority to
* authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.LocalLicense}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicensingAuthority.LocalLicense)
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
municipality_ = "";
countyCase_ = 0;
county_ = null;
provinceCase_ = 0;
province_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense build() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense buildPartial() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense result = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense(this);
result.municipality_ = municipality_;
if (countyCase_ == 2) {
result.county_ = county_;
}
if (countyCase_ == 3) {
result.county_ = county_;
}
if (provinceCase_ == 4) {
result.province_ = province_;
}
if (provinceCase_ == 5) {
result.province_ = province_;
}
result.countyCase_ = countyCase_;
result.provinceCase_ = provinceCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense other) {
if (other == io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance()) return this;
if (!other.getMunicipality().isEmpty()) {
municipality_ = other.municipality_;
onChanged();
}
switch (other.getCountyCase()) {
case COUNTY_NAME: {
countyCase_ = 2;
county_ = other.county_;
onChanged();
break;
}
case CALIFORNIA_COUNTY: {
setCaliforniaCountyValue(other.getCaliforniaCountyValue());
break;
}
case COUNTY_NOT_SET: {
break;
}
}
switch (other.getProvinceCase()) {
case PROVINCE_NAME: {
provinceCase_ = 4;
province_ = other.province_;
onChanged();
break;
}
case US_STATE: {
setUsStateValue(other.getUsStateValue());
break;
}
case PROVINCE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int countyCase_ = 0;
private java.lang.Object county_;
public CountyCase
getCountyCase() {
return CountyCase.forNumber(
countyCase_);
}
public Builder clearCounty() {
countyCase_ = 0;
county_ = null;
onChanged();
return this;
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
public Builder clearProvince() {
provinceCase_ = 0;
province_ = null;
onChanged();
return this;
}
private java.lang.Object municipality_ = "";
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public java.lang.String getMunicipality() {
java.lang.Object ref = municipality_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
municipality_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public com.google.protobuf.ByteString
getMunicipalityBytes() {
java.lang.Object ref = municipality_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
municipality_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public Builder setMunicipality(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
municipality_ = value;
onChanged();
return this;
}
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public Builder clearMunicipality() {
municipality_ = getDefaultInstance().getMunicipality();
onChanged();
return this;
}
/**
*
* Name of the municipality for this license.
*
*
* string municipality = 1;
*/
public Builder setMunicipalityBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
municipality_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public java.lang.String getCountyName() {
java.lang.Object ref = "";
if (countyCase_ == 2) {
ref = county_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (countyCase_ == 2) {
county_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public com.google.protobuf.ByteString
getCountyNameBytes() {
java.lang.Object ref = "";
if (countyCase_ == 2) {
ref = county_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (countyCase_ == 2) {
county_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public Builder setCountyName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
countyCase_ = 2;
county_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public Builder clearCountyName() {
if (countyCase_ == 2) {
countyCase_ = 0;
county_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string county_name = 2;
*/
public Builder setCountyNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
countyCase_ = 2;
county_ = value;
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public int getCaliforniaCountyValue() {
if (countyCase_ == 3) {
return ((java.lang.Integer) county_).intValue();
}
return 0;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public Builder setCaliforniaCountyValue(int value) {
countyCase_ = 3;
county_ = value;
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty() {
if (countyCase_ == 3) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.California.CaliforniaCounty result = io.opencannabis.schema.geo.usa.California.CaliforniaCounty.valueOf(
(java.lang.Integer) county_);
return result == null ? io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNKNOWN_COUNTY;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public Builder setCaliforniaCounty(io.opencannabis.schema.geo.usa.California.CaliforniaCounty value) {
if (value == null) {
throw new NullPointerException();
}
countyCase_ = 3;
county_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 3;
*/
public Builder clearCaliforniaCounty() {
if (countyCase_ == 3) {
countyCase_ = 0;
county_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 4) {
province_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 4) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder setProvinceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 4;
province_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder clearProvinceName() {
if (provinceCase_ == 4) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder setProvinceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
provinceCase_ = 4;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public int getUsStateValue() {
if (provinceCase_ == 5) {
return ((java.lang.Integer) province_).intValue();
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder setUsStateValue(int value) {
provinceCase_ = 5;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 5) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder setUsState(io.opencannabis.schema.geo.usa.USState value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 5;
province_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder clearUsState() {
if (provinceCase_ == 5) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicensingAuthority.LocalLicense)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicensingAuthority.LocalLicense)
private static final io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense();
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocalLicense parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocalLicense(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CountyLicenseOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicensingAuthority.CountyLicense)
com.google.protobuf.MessageOrBuilder {
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
java.lang.String getName();
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
int getCaliforniaCountyValue();
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty();
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
java.lang.String getProvinceName();
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
com.google.protobuf.ByteString
getProvinceNameBytes();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
int getUsStateValue();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
io.opencannabis.schema.geo.usa.USState getUsState();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.CountyCase getCountyCase();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.ProvinceCase getProvinceCase();
}
/**
*
* Specifies the concept of a county-level licensing authority, meaning a provincial jurisdiction with the statutory
* authority to authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.CountyLicense}
*/
public static final class CountyLicense extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicensingAuthority.CountyLicense)
CountyLicenseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CountyLicense.newBuilder() to construct.
private CountyLicense(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CountyLicense() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CountyLicense(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
countyCase_ = 1;
county_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
countyCase_ = 2;
county_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
provinceCase_ = 4;
province_ = s;
break;
}
case 40: {
int rawValue = input.readEnum();
provinceCase_ = 5;
province_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder.class);
}
private int countyCase_ = 0;
private java.lang.Object county_;
public enum CountyCase
implements com.google.protobuf.Internal.EnumLite {
NAME(1),
CALIFORNIA_COUNTY(2),
COUNTY_NOT_SET(0);
private final int value;
private CountyCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CountyCase valueOf(int value) {
return forNumber(value);
}
public static CountyCase forNumber(int value) {
switch (value) {
case 1: return NAME;
case 2: return CALIFORNIA_COUNTY;
case 0: return COUNTY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CountyCase
getCountyCase() {
return CountyCase.forNumber(
countyCase_);
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public enum ProvinceCase
implements com.google.protobuf.Internal.EnumLite {
PROVINCE_NAME(4),
US_STATE(5),
PROVINCE_NOT_SET(0);
private final int value;
private ProvinceCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProvinceCase valueOf(int value) {
return forNumber(value);
}
public static ProvinceCase forNumber(int value) {
switch (value) {
case 4: return PROVINCE_NAME;
case 5: return US_STATE;
case 0: return PROVINCE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = "";
if (countyCase_ == 1) {
ref = county_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (countyCase_ == 1) {
county_ = s;
}
return s;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = "";
if (countyCase_ == 1) {
ref = county_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (countyCase_ == 1) {
county_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALIFORNIA_COUNTY_FIELD_NUMBER = 2;
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public int getCaliforniaCountyValue() {
if (countyCase_ == 2) {
return (java.lang.Integer) county_;
}
return 0;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty() {
if (countyCase_ == 2) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.California.CaliforniaCounty result = io.opencannabis.schema.geo.usa.California.CaliforniaCounty.valueOf(
(java.lang.Integer) county_);
return result == null ? io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNKNOWN_COUNTY;
}
public static final int PROVINCE_NAME_FIELD_NUMBER = 4;
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 4) {
province_ = s;
}
return s;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 4) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int US_STATE_FIELD_NUMBER = 5;
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public int getUsStateValue() {
if (provinceCase_ == 5) {
return (java.lang.Integer) province_;
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 5) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (countyCase_ == 1) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, county_);
}
if (countyCase_ == 2) {
output.writeEnum(2, ((java.lang.Integer) county_));
}
if (provinceCase_ == 4) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, province_);
}
if (provinceCase_ == 5) {
output.writeEnum(5, ((java.lang.Integer) province_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (countyCase_ == 1) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, county_);
}
if (countyCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, ((java.lang.Integer) county_));
}
if (provinceCase_ == 4) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, province_);
}
if (provinceCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, ((java.lang.Integer) province_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense other = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) obj;
if (!getCountyCase().equals(other.getCountyCase())) return false;
switch (countyCase_) {
case 1:
if (!getName()
.equals(other.getName())) return false;
break;
case 2:
if (getCaliforniaCountyValue()
!= other.getCaliforniaCountyValue()) return false;
break;
case 0:
default:
}
if (!getProvinceCase().equals(other.getProvinceCase())) return false;
switch (provinceCase_) {
case 4:
if (!getProvinceName()
.equals(other.getProvinceName())) return false;
break;
case 5:
if (getUsStateValue()
!= other.getUsStateValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (countyCase_) {
case 1:
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
break;
case 2:
hash = (37 * hash) + CALIFORNIA_COUNTY_FIELD_NUMBER;
hash = (53 * hash) + getCaliforniaCountyValue();
break;
case 0:
default:
}
switch (provinceCase_) {
case 4:
hash = (37 * hash) + PROVINCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getProvinceName().hashCode();
break;
case 5:
hash = (37 * hash) + US_STATE_FIELD_NUMBER;
hash = (53 * hash) + getUsStateValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the concept of a county-level licensing authority, meaning a provincial jurisdiction with the statutory
* authority to authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.CountyLicense}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicensingAuthority.CountyLicense)
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
countyCase_ = 0;
county_ = null;
provinceCase_ = 0;
province_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense build() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense buildPartial() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense result = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense(this);
if (countyCase_ == 1) {
result.county_ = county_;
}
if (countyCase_ == 2) {
result.county_ = county_;
}
if (provinceCase_ == 4) {
result.province_ = province_;
}
if (provinceCase_ == 5) {
result.province_ = province_;
}
result.countyCase_ = countyCase_;
result.provinceCase_ = provinceCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense other) {
if (other == io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance()) return this;
switch (other.getCountyCase()) {
case NAME: {
countyCase_ = 1;
county_ = other.county_;
onChanged();
break;
}
case CALIFORNIA_COUNTY: {
setCaliforniaCountyValue(other.getCaliforniaCountyValue());
break;
}
case COUNTY_NOT_SET: {
break;
}
}
switch (other.getProvinceCase()) {
case PROVINCE_NAME: {
provinceCase_ = 4;
province_ = other.province_;
onChanged();
break;
}
case US_STATE: {
setUsStateValue(other.getUsStateValue());
break;
}
case PROVINCE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int countyCase_ = 0;
private java.lang.Object county_;
public CountyCase
getCountyCase() {
return CountyCase.forNumber(
countyCase_);
}
public Builder clearCounty() {
countyCase_ = 0;
county_ = null;
onChanged();
return this;
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
public Builder clearProvince() {
provinceCase_ = 0;
province_ = null;
onChanged();
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = "";
if (countyCase_ == 1) {
ref = county_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (countyCase_ == 1) {
county_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = "";
if (countyCase_ == 1) {
ref = county_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (countyCase_ == 1) {
county_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
countyCase_ = 1;
county_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public Builder clearName() {
if (countyCase_ == 1) {
countyCase_ = 0;
county_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name of a given county.
*
*
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
countyCase_ = 1;
county_ = value;
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public int getCaliforniaCountyValue() {
if (countyCase_ == 2) {
return ((java.lang.Integer) county_).intValue();
}
return 0;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public Builder setCaliforniaCountyValue(int value) {
countyCase_ = 2;
county_ = value;
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public io.opencannabis.schema.geo.usa.California.CaliforniaCounty getCaliforniaCounty() {
if (countyCase_ == 2) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.California.CaliforniaCounty result = io.opencannabis.schema.geo.usa.California.CaliforniaCounty.valueOf(
(java.lang.Integer) county_);
return result == null ? io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.California.CaliforniaCounty.UNKNOWN_COUNTY;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public Builder setCaliforniaCounty(io.opencannabis.schema.geo.usa.California.CaliforniaCounty value) {
if (value == null) {
throw new NullPointerException();
}
countyCase_ = 2;
county_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a California county.
*
*
* .opencannabis.geo.usa.ca.CaliforniaCounty california_county = 2;
*/
public Builder clearCaliforniaCounty() {
if (countyCase_ == 2) {
countyCase_ = 0;
county_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 4) {
province_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 4) {
ref = province_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 4) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder setProvinceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 4;
province_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder clearProvinceName() {
if (provinceCase_ == 4) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 4;
*/
public Builder setProvinceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
provinceCase_ = 4;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public int getUsStateValue() {
if (provinceCase_ == 5) {
return ((java.lang.Integer) province_).intValue();
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder setUsStateValue(int value) {
provinceCase_ = 5;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 5) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder setUsState(io.opencannabis.schema.geo.usa.USState value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 5;
province_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 5;
*/
public Builder clearUsState() {
if (provinceCase_ == 5) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicensingAuthority.CountyLicense)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicensingAuthority.CountyLicense)
private static final io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense();
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CountyLicense parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CountyLicense(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StateLicenseOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicensingAuthority.StateLicense)
com.google.protobuf.MessageOrBuilder {
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
java.lang.String getProvinceName();
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
com.google.protobuf.ByteString
getProvinceNameBytes();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
int getUsStateValue();
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
io.opencannabis.schema.geo.usa.USState getUsState();
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
java.lang.String getAgencyName();
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
com.google.protobuf.ByteString
getAgencyNameBytes();
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
int getCaliforniaAgencyValue();
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency getCaliforniaAgency();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.ProvinceCase getProvinceCase();
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.AgencyCase getAgencyCase();
}
/**
*
* Specifies the concept of a state-level licensing authority, meaning a state or regional jurisdiction with the
* statutory authority to authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.StateLicense}
*/
public static final class StateLicense extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicensingAuthority.StateLicense)
StateLicenseOrBuilder {
private static final long serialVersionUID = 0L;
// Use StateLicense.newBuilder() to construct.
private StateLicense(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StateLicense() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StateLicense(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
provinceCase_ = 1;
province_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
provinceCase_ = 2;
province_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
agencyCase_ = 3;
agency_ = s;
break;
}
case 32: {
int rawValue = input.readEnum();
agencyCase_ = 4;
agency_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_StateLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder.class);
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public enum ProvinceCase
implements com.google.protobuf.Internal.EnumLite {
PROVINCE_NAME(1),
US_STATE(2),
PROVINCE_NOT_SET(0);
private final int value;
private ProvinceCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProvinceCase valueOf(int value) {
return forNumber(value);
}
public static ProvinceCase forNumber(int value) {
switch (value) {
case 1: return PROVINCE_NAME;
case 2: return US_STATE;
case 0: return PROVINCE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
private int agencyCase_ = 0;
private java.lang.Object agency_;
public enum AgencyCase
implements com.google.protobuf.Internal.EnumLite {
AGENCY_NAME(3),
CALIFORNIA_AGENCY(4),
AGENCY_NOT_SET(0);
private final int value;
private AgencyCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AgencyCase valueOf(int value) {
return forNumber(value);
}
public static AgencyCase forNumber(int value) {
switch (value) {
case 3: return AGENCY_NAME;
case 4: return CALIFORNIA_AGENCY;
case 0: return AGENCY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public AgencyCase
getAgencyCase() {
return AgencyCase.forNumber(
agencyCase_);
}
public static final int PROVINCE_NAME_FIELD_NUMBER = 1;
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 1) {
ref = province_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 1) {
province_ = s;
}
return s;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 1) {
ref = province_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 1) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int US_STATE_FIELD_NUMBER = 2;
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public int getUsStateValue() {
if (provinceCase_ == 2) {
return (java.lang.Integer) province_;
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 2) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
public static final int AGENCY_NAME_FIELD_NUMBER = 3;
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public java.lang.String getAgencyName() {
java.lang.Object ref = "";
if (agencyCase_ == 3) {
ref = agency_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (agencyCase_ == 3) {
agency_ = s;
}
return s;
}
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public com.google.protobuf.ByteString
getAgencyNameBytes() {
java.lang.Object ref = "";
if (agencyCase_ == 3) {
ref = agency_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (agencyCase_ == 3) {
agency_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALIFORNIA_AGENCY_FIELD_NUMBER = 4;
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public int getCaliforniaAgencyValue() {
if (agencyCase_ == 4) {
return (java.lang.Integer) agency_;
}
return 0;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency getCaliforniaAgency() {
if (agencyCase_ == 4) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency result = io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.valueOf(
(java.lang.Integer) agency_);
return result == null ? io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.UNRECOGNIZED : result;
}
return io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.UNKNOWN_AGENCY;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (provinceCase_ == 1) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, province_);
}
if (provinceCase_ == 2) {
output.writeEnum(2, ((java.lang.Integer) province_));
}
if (agencyCase_ == 3) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, agency_);
}
if (agencyCase_ == 4) {
output.writeEnum(4, ((java.lang.Integer) agency_));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (provinceCase_ == 1) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, province_);
}
if (provinceCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, ((java.lang.Integer) province_));
}
if (agencyCase_ == 3) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, agency_);
}
if (agencyCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, ((java.lang.Integer) agency_));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense other = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) obj;
if (!getProvinceCase().equals(other.getProvinceCase())) return false;
switch (provinceCase_) {
case 1:
if (!getProvinceName()
.equals(other.getProvinceName())) return false;
break;
case 2:
if (getUsStateValue()
!= other.getUsStateValue()) return false;
break;
case 0:
default:
}
if (!getAgencyCase().equals(other.getAgencyCase())) return false;
switch (agencyCase_) {
case 3:
if (!getAgencyName()
.equals(other.getAgencyName())) return false;
break;
case 4:
if (getCaliforniaAgencyValue()
!= other.getCaliforniaAgencyValue()) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (provinceCase_) {
case 1:
hash = (37 * hash) + PROVINCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getProvinceName().hashCode();
break;
case 2:
hash = (37 * hash) + US_STATE_FIELD_NUMBER;
hash = (53 * hash) + getUsStateValue();
break;
case 0:
default:
}
switch (agencyCase_) {
case 3:
hash = (37 * hash) + AGENCY_NAME_FIELD_NUMBER;
hash = (53 * hash) + getAgencyName().hashCode();
break;
case 4:
hash = (37 * hash) + CALIFORNIA_AGENCY_FIELD_NUMBER;
hash = (53 * hash) + getCaliforniaAgencyValue();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the concept of a state-level licensing authority, meaning a state or regional jurisdiction with the
* statutory authority to authorize industrial cannabis privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority.StateLicense}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicensingAuthority.StateLicense)
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_StateLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
provinceCase_ = 0;
province_ = null;
agencyCase_ = 0;
agency_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense build() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense buildPartial() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense result = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense(this);
if (provinceCase_ == 1) {
result.province_ = province_;
}
if (provinceCase_ == 2) {
result.province_ = province_;
}
if (agencyCase_ == 3) {
result.agency_ = agency_;
}
if (agencyCase_ == 4) {
result.agency_ = agency_;
}
result.provinceCase_ = provinceCase_;
result.agencyCase_ = agencyCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense other) {
if (other == io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance()) return this;
switch (other.getProvinceCase()) {
case PROVINCE_NAME: {
provinceCase_ = 1;
province_ = other.province_;
onChanged();
break;
}
case US_STATE: {
setUsStateValue(other.getUsStateValue());
break;
}
case PROVINCE_NOT_SET: {
break;
}
}
switch (other.getAgencyCase()) {
case AGENCY_NAME: {
agencyCase_ = 3;
agency_ = other.agency_;
onChanged();
break;
}
case CALIFORNIA_AGENCY: {
setCaliforniaAgencyValue(other.getCaliforniaAgencyValue());
break;
}
case AGENCY_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int provinceCase_ = 0;
private java.lang.Object province_;
public ProvinceCase
getProvinceCase() {
return ProvinceCase.forNumber(
provinceCase_);
}
public Builder clearProvince() {
provinceCase_ = 0;
province_ = null;
onChanged();
return this;
}
private int agencyCase_ = 0;
private java.lang.Object agency_;
public AgencyCase
getAgencyCase() {
return AgencyCase.forNumber(
agencyCase_);
}
public Builder clearAgency() {
agencyCase_ = 0;
agency_ = null;
onChanged();
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public java.lang.String getProvinceName() {
java.lang.Object ref = "";
if (provinceCase_ == 1) {
ref = province_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (provinceCase_ == 1) {
province_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public com.google.protobuf.ByteString
getProvinceNameBytes() {
java.lang.Object ref = "";
if (provinceCase_ == 1) {
ref = province_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (provinceCase_ == 1) {
province_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public Builder setProvinceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 1;
province_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public Builder clearProvinceName() {
if (provinceCase_ == 1) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given state or province.
*
*
* string province_name = 1;
*/
public Builder setProvinceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
provinceCase_ = 1;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public int getUsStateValue() {
if (provinceCase_ == 2) {
return ((java.lang.Integer) province_).intValue();
}
return 0;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public Builder setUsStateValue(int value) {
provinceCase_ = 2;
province_ = value;
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public io.opencannabis.schema.geo.usa.USState getUsState() {
if (provinceCase_ == 2) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.geo.usa.USState result = io.opencannabis.schema.geo.usa.USState.valueOf(
(java.lang.Integer) province_);
return result == null ? io.opencannabis.schema.geo.usa.USState.UNRECOGNIZED : result;
}
return io.opencannabis.schema.geo.usa.USState.UNSPECIFIED;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public Builder setUsState(io.opencannabis.schema.geo.usa.USState value) {
if (value == null) {
throw new NullPointerException();
}
provinceCase_ = 2;
province_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a U.S. state.
*
*
* .opencannabis.geo.usa.USState us_state = 2;
*/
public Builder clearUsState() {
if (provinceCase_ == 2) {
provinceCase_ = 0;
province_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public java.lang.String getAgencyName() {
java.lang.Object ref = "";
if (agencyCase_ == 3) {
ref = agency_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (agencyCase_ == 3) {
agency_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public com.google.protobuf.ByteString
getAgencyNameBytes() {
java.lang.Object ref = "";
if (agencyCase_ == 3) {
ref = agency_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (agencyCase_ == 3) {
agency_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public Builder setAgencyName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
agencyCase_ = 3;
agency_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public Builder clearAgencyName() {
if (agencyCase_ == 3) {
agencyCase_ = 0;
agency_ = null;
onChanged();
}
return this;
}
/**
*
* Arbitrary name for a given regulatory/licensing agency.
*
*
* string agency_name = 3;
*/
public Builder setAgencyNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
agencyCase_ = 3;
agency_ = value;
onChanged();
return this;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public int getCaliforniaAgencyValue() {
if (agencyCase_ == 4) {
return ((java.lang.Integer) agency_).intValue();
}
return 0;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public Builder setCaliforniaAgencyValue(int value) {
agencyCase_ = 4;
agency_ = value;
onChanged();
return this;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency getCaliforniaAgency() {
if (agencyCase_ == 4) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency result = io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.valueOf(
(java.lang.Integer) agency_);
return result == null ? io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.UNRECOGNIZED : result;
}
return io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency.UNKNOWN_AGENCY;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public Builder setCaliforniaAgency(io.opencannabis.schema.regulatory.usa.California.CaliforniaAgency value) {
if (value == null) {
throw new NullPointerException();
}
agencyCase_ = 4;
agency_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies a specific executive agency in the US State of California.
*
*
* .opencannabis.regulatory.usa.ca.CaliforniaAgency california_agency = 4;
*/
public Builder clearCaliforniaAgency() {
if (agencyCase_ == 4) {
agencyCase_ = 0;
agency_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicensingAuthority.StateLicense)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicensingAuthority.StateLicense)
private static final io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense();
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StateLicense parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StateLicense(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int jurisdictionCase_ = 0;
private java.lang.Object jurisdiction_;
public enum JurisdictionCase
implements com.google.protobuf.Internal.EnumLite {
LOCAL(1),
COUNTY(2),
STATE(3),
JURISDICTION_NOT_SET(0);
private final int value;
private JurisdictionCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static JurisdictionCase valueOf(int value) {
return forNumber(value);
}
public static JurisdictionCase forNumber(int value) {
switch (value) {
case 1: return LOCAL;
case 2: return COUNTY;
case 3: return STATE;
case 0: return JURISDICTION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public JurisdictionCase
getJurisdictionCase() {
return JurisdictionCase.forNumber(
jurisdictionCase_);
}
public static final int LOCAL_FIELD_NUMBER = 1;
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public boolean hasLocal() {
return jurisdictionCase_ == 1;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getLocal() {
if (jurisdictionCase_ == 1) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder getLocalOrBuilder() {
if (jurisdictionCase_ == 1) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
public static final int COUNTY_FIELD_NUMBER = 2;
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public boolean hasCounty() {
return jurisdictionCase_ == 2;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getCounty() {
if (jurisdictionCase_ == 2) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder getCountyOrBuilder() {
if (jurisdictionCase_ == 2) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
public static final int STATE_FIELD_NUMBER = 3;
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public boolean hasState() {
return jurisdictionCase_ == 3;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getState() {
if (jurisdictionCase_ == 3) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder getStateOrBuilder() {
if (jurisdictionCase_ == 3) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (jurisdictionCase_ == 1) {
output.writeMessage(1, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_);
}
if (jurisdictionCase_ == 2) {
output.writeMessage(2, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_);
}
if (jurisdictionCase_ == 3) {
output.writeMessage(3, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (jurisdictionCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_);
}
if (jurisdictionCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_);
}
if (jurisdictionCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicensingAuthority other = (io.bloombox.schema.licensure.Licensure.LicensingAuthority) obj;
if (!getJurisdictionCase().equals(other.getJurisdictionCase())) return false;
switch (jurisdictionCase_) {
case 1:
if (!getLocal()
.equals(other.getLocal())) return false;
break;
case 2:
if (!getCounty()
.equals(other.getCounty())) return false;
break;
case 3:
if (!getState()
.equals(other.getState())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (jurisdictionCase_) {
case 1:
hash = (37 * hash) + LOCAL_FIELD_NUMBER;
hash = (53 * hash) + getLocal().hashCode();
break;
case 2:
hash = (37 * hash) + COUNTY_FIELD_NUMBER;
hash = (53 * hash) + getCounty().hashCode();
break;
case 3:
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicensingAuthority prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the jurisdictional authority that issued or is in the process of issuing a license. Jurisdiction is a
* hierarchical concept, and so, this structure is filled out according to the "leaf" jurisdiction that actually issued
* the subject license.
*
*
* Protobuf type {@code bloombox.licensure.LicensingAuthority}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicensingAuthority)
io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.class, io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicensingAuthority.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
jurisdictionCase_ = 0;
jurisdiction_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicensingAuthority_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority build() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority buildPartial() {
io.bloombox.schema.licensure.Licensure.LicensingAuthority result = new io.bloombox.schema.licensure.Licensure.LicensingAuthority(this);
if (jurisdictionCase_ == 1) {
if (localBuilder_ == null) {
result.jurisdiction_ = jurisdiction_;
} else {
result.jurisdiction_ = localBuilder_.build();
}
}
if (jurisdictionCase_ == 2) {
if (countyBuilder_ == null) {
result.jurisdiction_ = jurisdiction_;
} else {
result.jurisdiction_ = countyBuilder_.build();
}
}
if (jurisdictionCase_ == 3) {
if (stateBuilder_ == null) {
result.jurisdiction_ = jurisdiction_;
} else {
result.jurisdiction_ = stateBuilder_.build();
}
}
result.jurisdictionCase_ = jurisdictionCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicensingAuthority) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicensingAuthority)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicensingAuthority other) {
if (other == io.bloombox.schema.licensure.Licensure.LicensingAuthority.getDefaultInstance()) return this;
switch (other.getJurisdictionCase()) {
case LOCAL: {
mergeLocal(other.getLocal());
break;
}
case COUNTY: {
mergeCounty(other.getCounty());
break;
}
case STATE: {
mergeState(other.getState());
break;
}
case JURISDICTION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicensingAuthority parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicensingAuthority) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int jurisdictionCase_ = 0;
private java.lang.Object jurisdiction_;
public JurisdictionCase
getJurisdictionCase() {
return JurisdictionCase.forNumber(
jurisdictionCase_);
}
public Builder clearJurisdiction() {
jurisdictionCase_ = 0;
jurisdiction_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder> localBuilder_;
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public boolean hasLocal() {
return jurisdictionCase_ == 1;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense getLocal() {
if (localBuilder_ == null) {
if (jurisdictionCase_ == 1) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
} else {
if (jurisdictionCase_ == 1) {
return localBuilder_.getMessage();
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public Builder setLocal(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense value) {
if (localBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jurisdiction_ = value;
onChanged();
} else {
localBuilder_.setMessage(value);
}
jurisdictionCase_ = 1;
return this;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public Builder setLocal(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder builderForValue) {
if (localBuilder_ == null) {
jurisdiction_ = builderForValue.build();
onChanged();
} else {
localBuilder_.setMessage(builderForValue.build());
}
jurisdictionCase_ = 1;
return this;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public Builder mergeLocal(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense value) {
if (localBuilder_ == null) {
if (jurisdictionCase_ == 1 &&
jurisdiction_ != io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance()) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.newBuilder((io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_)
.mergeFrom(value).buildPartial();
} else {
jurisdiction_ = value;
}
onChanged();
} else {
if (jurisdictionCase_ == 1) {
localBuilder_.mergeFrom(value);
}
localBuilder_.setMessage(value);
}
jurisdictionCase_ = 1;
return this;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public Builder clearLocal() {
if (localBuilder_ == null) {
if (jurisdictionCase_ == 1) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
onChanged();
}
} else {
if (jurisdictionCase_ == 1) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
}
localBuilder_.clear();
}
return this;
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder getLocalBuilder() {
return getLocalFieldBuilder().getBuilder();
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder getLocalOrBuilder() {
if ((jurisdictionCase_ == 1) && (localBuilder_ != null)) {
return localBuilder_.getMessageOrBuilder();
} else {
if (jurisdictionCase_ == 1) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a "local" (or municipal) jurisdiction, which is to say, a township or city.
*
*
* .bloombox.licensure.LicensingAuthority.LocalLicense local = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder>
getLocalFieldBuilder() {
if (localBuilder_ == null) {
if (!(jurisdictionCase_ == 1)) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.getDefaultInstance();
}
localBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicenseOrBuilder>(
(io.bloombox.schema.licensure.Licensure.LicensingAuthority.LocalLicense) jurisdiction_,
getParentForChildren(),
isClean());
jurisdiction_ = null;
}
jurisdictionCase_ = 1;
onChanged();;
return localBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder> countyBuilder_;
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public boolean hasCounty() {
return jurisdictionCase_ == 2;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense getCounty() {
if (countyBuilder_ == null) {
if (jurisdictionCase_ == 2) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
} else {
if (jurisdictionCase_ == 2) {
return countyBuilder_.getMessage();
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public Builder setCounty(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense value) {
if (countyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jurisdiction_ = value;
onChanged();
} else {
countyBuilder_.setMessage(value);
}
jurisdictionCase_ = 2;
return this;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public Builder setCounty(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder builderForValue) {
if (countyBuilder_ == null) {
jurisdiction_ = builderForValue.build();
onChanged();
} else {
countyBuilder_.setMessage(builderForValue.build());
}
jurisdictionCase_ = 2;
return this;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public Builder mergeCounty(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense value) {
if (countyBuilder_ == null) {
if (jurisdictionCase_ == 2 &&
jurisdiction_ != io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance()) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.newBuilder((io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_)
.mergeFrom(value).buildPartial();
} else {
jurisdiction_ = value;
}
onChanged();
} else {
if (jurisdictionCase_ == 2) {
countyBuilder_.mergeFrom(value);
}
countyBuilder_.setMessage(value);
}
jurisdictionCase_ = 2;
return this;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public Builder clearCounty() {
if (countyBuilder_ == null) {
if (jurisdictionCase_ == 2) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
onChanged();
}
} else {
if (jurisdictionCase_ == 2) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
}
countyBuilder_.clear();
}
return this;
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder getCountyBuilder() {
return getCountyFieldBuilder().getBuilder();
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder getCountyOrBuilder() {
if ((jurisdictionCase_ == 2) && (countyBuilder_ != null)) {
return countyBuilder_.getMessageOrBuilder();
} else {
if (jurisdictionCase_ == 2) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a semi-local (provincial) jurisdiction, which is to say, a county.
*
*
* .bloombox.licensure.LicensingAuthority.CountyLicense county = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder>
getCountyFieldBuilder() {
if (countyBuilder_ == null) {
if (!(jurisdictionCase_ == 2)) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.getDefaultInstance();
}
countyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicenseOrBuilder>(
(io.bloombox.schema.licensure.Licensure.LicensingAuthority.CountyLicense) jurisdiction_,
getParentForChildren(),
isClean());
jurisdiction_ = null;
}
jurisdictionCase_ = 2;
onChanged();;
return countyBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder> stateBuilder_;
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public boolean hasState() {
return jurisdictionCase_ == 3;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense getState() {
if (stateBuilder_ == null) {
if (jurisdictionCase_ == 3) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
} else {
if (jurisdictionCase_ == 3) {
return stateBuilder_.getMessage();
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public Builder setState(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense value) {
if (stateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jurisdiction_ = value;
onChanged();
} else {
stateBuilder_.setMessage(value);
}
jurisdictionCase_ = 3;
return this;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public Builder setState(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder builderForValue) {
if (stateBuilder_ == null) {
jurisdiction_ = builderForValue.build();
onChanged();
} else {
stateBuilder_.setMessage(builderForValue.build());
}
jurisdictionCase_ = 3;
return this;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public Builder mergeState(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense value) {
if (stateBuilder_ == null) {
if (jurisdictionCase_ == 3 &&
jurisdiction_ != io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance()) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.newBuilder((io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_)
.mergeFrom(value).buildPartial();
} else {
jurisdiction_ = value;
}
onChanged();
} else {
if (jurisdictionCase_ == 3) {
stateBuilder_.mergeFrom(value);
}
stateBuilder_.setMessage(value);
}
jurisdictionCase_ = 3;
return this;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public Builder clearState() {
if (stateBuilder_ == null) {
if (jurisdictionCase_ == 3) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
onChanged();
}
} else {
if (jurisdictionCase_ == 3) {
jurisdictionCase_ = 0;
jurisdiction_ = null;
}
stateBuilder_.clear();
}
return this;
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder getStateBuilder() {
return getStateFieldBuilder().getBuilder();
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder getStateOrBuilder() {
if ((jurisdictionCase_ == 3) && (stateBuilder_ != null)) {
return stateBuilder_.getMessageOrBuilder();
} else {
if (jurisdictionCase_ == 3) {
return (io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_;
}
return io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
}
/**
*
* Specifies a license issued by a regional jurisdiction, which is to say, a state.
*
*
* .bloombox.licensure.LicensingAuthority.StateLicense state = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder>
getStateFieldBuilder() {
if (stateBuilder_ == null) {
if (!(jurisdictionCase_ == 3)) {
jurisdiction_ = io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.getDefaultInstance();
}
stateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicenseOrBuilder>(
(io.bloombox.schema.licensure.Licensure.LicensingAuthority.StateLicense) jurisdiction_,
getParentForChildren(),
isClean());
jurisdiction_ = null;
}
jurisdictionCase_ = 3;
onChanged();;
return stateBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicensingAuthority)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicensingAuthority)
private static final io.bloombox.schema.licensure.Licensure.LicensingAuthority DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicensingAuthority();
}
public static io.bloombox.schema.licensure.Licensure.LicensingAuthority getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LicensingAuthority parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LicensingAuthority(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicensingAuthority getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LicenseKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicenseKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
java.lang.String getId();
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
java.lang.String getJid();
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
com.google.protobuf.ByteString
getJidBytes();
}
/**
*
* Specifies a unique key that identifies a regulatory license document. It is generally compose of an auto-generated
* UUID value, and a reference to any jurisdictionally-issued document or license codes.
*
*
* Protobuf type {@code bloombox.licensure.LicenseKey}
*/
public static final class LicenseKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicenseKey)
LicenseKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use LicenseKey.newBuilder() to construct.
private LicenseKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LicenseKey() {
id_ = "";
jid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LicenseKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
jid_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseKey.class, io.bloombox.schema.licensure.Licensure.LicenseKey.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JID_FIELD_NUMBER = 2;
private volatile java.lang.Object jid_;
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public java.lang.String getJid() {
java.lang.Object ref = jid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jid_ = s;
return s;
}
}
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public com.google.protobuf.ByteString
getJidBytes() {
java.lang.Object ref = jid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getJidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, jid_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getJidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, jid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicenseKey)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicenseKey other = (io.bloombox.schema.licensure.Licensure.LicenseKey) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getJid()
.equals(other.getJid())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + JID_FIELD_NUMBER;
hash = (53 * hash) + getJid().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicenseKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a unique key that identifies a regulatory license document. It is generally compose of an auto-generated
* UUID value, and a reference to any jurisdictionally-issued document or license codes.
*
*
* Protobuf type {@code bloombox.licensure.LicenseKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicenseKey)
io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseKey.class, io.bloombox.schema.licensure.Licensure.LicenseKey.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicenseKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
jid_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseKey_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseKey getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicenseKey.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseKey build() {
io.bloombox.schema.licensure.Licensure.LicenseKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseKey buildPartial() {
io.bloombox.schema.licensure.Licensure.LicenseKey result = new io.bloombox.schema.licensure.Licensure.LicenseKey(this);
result.id_ = id_;
result.jid_ = jid_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicenseKey) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicenseKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicenseKey other) {
if (other == io.bloombox.schema.licensure.Licensure.LicenseKey.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getJid().isEmpty()) {
jid_ = other.jid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicenseKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicenseKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Generated UUID for this particular license. Because a license ID may not yet have been issued by the licensing
* agency or jurisdiction, this ID serves as the primary key.
*
*
* string id = 1 [(.core.field) = { ... }
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object jid_ = "";
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public java.lang.String getJid() {
java.lang.Object ref = jid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public com.google.protobuf.ByteString
getJidBytes() {
java.lang.Object ref = jid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public Builder setJid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jid_ = value;
onChanged();
return this;
}
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public Builder clearJid() {
jid_ = getDefaultInstance().getJid();
onChanged();
return this;
}
/**
*
* Jurisdictionally-issued ID code. If the license carries an ID code issued by the state or jurisdiction, it is
* recorded here as part of the license's primary key.
*
*
* string jid = 2;
*/
public Builder setJidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jid_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicenseKey)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicenseKey)
private static final io.bloombox.schema.licensure.Licensure.LicenseKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicenseKey();
}
public static io.bloombox.schema.licensure.Licensure.LicenseKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LicenseKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LicenseKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LicenseEventOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicenseEvent)
com.google.protobuf.MessageOrBuilder {
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
int getStatusValue();
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus();
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
java.util.List getPrivilegeList();
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
int getPrivilegeCount();
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index);
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
java.util.List
getPrivilegeValueList();
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
int getPrivilegeValue(int index);
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
java.lang.String getMessage();
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
boolean hasOccurred();
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred();
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder();
}
/**
*
* Specifies an individual event in the lifecycle of a given industrial cannabis license. History for a given license
* can be reconstructed from a set of license events.
*
*
* Protobuf type {@code bloombox.licensure.LicenseEvent}
*/
public static final class LicenseEvent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicenseEvent)
LicenseEventOrBuilder {
private static final long serialVersionUID = 0L;
// Use LicenseEvent.newBuilder() to construct.
private LicenseEvent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LicenseEvent() {
status_ = 0;
privilege_ = java.util.Collections.emptyList();
message_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LicenseEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 16: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
privilege_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
privilege_.add(rawValue);
break;
}
case 18: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
privilege_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
privilege_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 34: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (occurred_ != null) {
subBuilder = occurred_.toBuilder();
}
occurred_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(occurred_);
occurred_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
privilege_ = java.util.Collections.unmodifiableList(privilege_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseEvent.class, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseStatus result = io.bloombox.schema.licensure.Licensure.LicenseStatus.valueOf(status_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseStatus.UNRECOGNIZED : result;
}
public static final int PRIVILEGE_FIELD_NUMBER = 2;
private java.util.List privilege_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege> privilege_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>() {
public io.bloombox.schema.licensure.Licensure.LicensePrivilege convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicensePrivilege result = io.bloombox.schema.licensure.Licensure.LicensePrivilege.valueOf(from);
return result == null ? io.bloombox.schema.licensure.Licensure.LicensePrivilege.UNRECOGNIZED : result;
}
};
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public java.util.List getPrivilegeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>(privilege_, privilege_converter_);
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public int getPrivilegeCount() {
return privilege_.size();
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index) {
return privilege_converter_.convert(privilege_.get(index));
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public java.util.List
getPrivilegeValueList() {
return privilege_;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public int getPrivilegeValue(int index) {
return privilege_.get(index);
}
private int privilegeMemoizedSerializedSize;
public static final int MESSAGE_FIELD_NUMBER = 3;
private volatile java.lang.Object message_;
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OCCURRED_FIELD_NUMBER = 4;
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public boolean hasOccurred() {
return occurred_ != null;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
return getOccurred();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (status_ != io.bloombox.schema.licensure.Licensure.LicenseStatus.PENDING.getNumber()) {
output.writeEnum(1, status_);
}
if (getPrivilegeList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(privilegeMemoizedSerializedSize);
}
for (int i = 0; i < privilege_.size(); i++) {
output.writeEnumNoTag(privilege_.get(i));
}
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, message_);
}
if (occurred_ != null) {
output.writeMessage(4, getOccurred());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != io.bloombox.schema.licensure.Licensure.LicenseStatus.PENDING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
{
int dataSize = 0;
for (int i = 0; i < privilege_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(privilege_.get(i));
}
size += dataSize;
if (!getPrivilegeList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}privilegeMemoizedSerializedSize = dataSize;
}
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, message_);
}
if (occurred_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOccurred());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicenseEvent)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicenseEvent other = (io.bloombox.schema.licensure.Licensure.LicenseEvent) obj;
if (status_ != other.status_) return false;
if (!privilege_.equals(other.privilege_)) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (hasOccurred() != other.hasOccurred()) return false;
if (hasOccurred()) {
if (!getOccurred()
.equals(other.getOccurred())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (getPrivilegeCount() > 0) {
hash = (37 * hash) + PRIVILEGE_FIELD_NUMBER;
hash = (53 * hash) + privilege_.hashCode();
}
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
if (hasOccurred()) {
hash = (37 * hash) + OCCURRED_FIELD_NUMBER;
hash = (53 * hash) + getOccurred().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicenseEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies an individual event in the lifecycle of a given industrial cannabis license. History for a given license
* can be reconstructed from a set of license events.
*
*
* Protobuf type {@code bloombox.licensure.LicenseEvent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicenseEvent)
io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseEvent.class, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicenseEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
privilege_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
message_ = "";
if (occurredBuilder_ == null) {
occurred_ = null;
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseEvent_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseEvent getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicenseEvent.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseEvent build() {
io.bloombox.schema.licensure.Licensure.LicenseEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseEvent buildPartial() {
io.bloombox.schema.licensure.Licensure.LicenseEvent result = new io.bloombox.schema.licensure.Licensure.LicenseEvent(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.status_ = status_;
if (((bitField0_ & 0x00000002) != 0)) {
privilege_ = java.util.Collections.unmodifiableList(privilege_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.privilege_ = privilege_;
result.message_ = message_;
if (occurredBuilder_ == null) {
result.occurred_ = occurred_;
} else {
result.occurred_ = occurredBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicenseEvent) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicenseEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicenseEvent other) {
if (other == io.bloombox.schema.licensure.Licensure.LicenseEvent.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.privilege_.isEmpty()) {
if (privilege_.isEmpty()) {
privilege_ = other.privilege_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensurePrivilegeIsMutable();
privilege_.addAll(other.privilege_);
}
onChanged();
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (other.hasOccurred()) {
mergeOccurred(other.getOccurred());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicenseEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicenseEvent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int status_ = 0;
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseStatus result = io.bloombox.schema.licensure.Licensure.LicenseStatus.valueOf(status_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseStatus.UNRECOGNIZED : result;
}
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public Builder setStatus(io.bloombox.schema.licensure.Licensure.LicenseStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status for the license after this event takes place.
*
*
* .bloombox.licensure.LicenseStatus status = 1;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.util.List privilege_ =
java.util.Collections.emptyList();
private void ensurePrivilegeIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
privilege_ = new java.util.ArrayList(privilege_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public java.util.List getPrivilegeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>(privilege_, privilege_converter_);
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public int getPrivilegeCount() {
return privilege_.size();
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index) {
return privilege_converter_.convert(privilege_.get(index));
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder setPrivilege(
int index, io.bloombox.schema.licensure.Licensure.LicensePrivilege value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrivilegeIsMutable();
privilege_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder addPrivilege(io.bloombox.schema.licensure.Licensure.LicensePrivilege value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrivilegeIsMutable();
privilege_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder addAllPrivilege(
java.lang.Iterable extends io.bloombox.schema.licensure.Licensure.LicensePrivilege> values) {
ensurePrivilegeIsMutable();
for (io.bloombox.schema.licensure.Licensure.LicensePrivilege value : values) {
privilege_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder clearPrivilege() {
privilege_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public java.util.List
getPrivilegeValueList() {
return java.util.Collections.unmodifiableList(privilege_);
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public int getPrivilegeValue(int index) {
return privilege_.get(index);
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder setPrivilegeValue(
int index, int value) {
ensurePrivilegeIsMutable();
privilege_.set(index, value);
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder addPrivilegeValue(int value) {
ensurePrivilegeIsMutable();
privilege_.add(value);
onChanged();
return this;
}
/**
*
* Privileges attached to this license after this event takes place. If this property is left empty, privileges are
* considered unchanged from the previous license event.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 2;
*/
public Builder addAllPrivilegeValue(
java.lang.Iterable values) {
ensurePrivilegeIsMutable();
for (int value : values) {
privilege_.add(value);
}
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* Specifies an arbitrary message associated with this license event. Optional.
*
*
* string message = 3;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> occurredBuilder_;
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public boolean hasOccurred() {
return occurredBuilder_ != null || occurred_ != null;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
if (occurredBuilder_ == null) {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
} else {
return occurredBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public Builder setOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
occurred_ = value;
onChanged();
} else {
occurredBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public Builder setOccurred(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (occurredBuilder_ == null) {
occurred_ = builderForValue.build();
onChanged();
} else {
occurredBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public Builder mergeOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (occurred_ != null) {
occurred_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(occurred_).mergeFrom(value).buildPartial();
} else {
occurred_ = value;
}
onChanged();
} else {
occurredBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public Builder clearOccurred() {
if (occurredBuilder_ == null) {
occurred_ = null;
onChanged();
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getOccurredBuilder() {
onChanged();
return getOccurredFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
if (occurredBuilder_ != null) {
return occurredBuilder_.getMessageOrBuilder();
} else {
return occurred_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
}
/**
*
* Timestamp for when this license event was committed.
*
*
* .opencannabis.temporal.Instant occurred = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getOccurredFieldBuilder() {
if (occurredBuilder_ == null) {
occurredBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getOccurred(),
getParentForChildren(),
isClean());
occurred_ = null;
}
return occurredBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicenseEvent)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicenseEvent)
private static final io.bloombox.schema.licensure.Licensure.LicenseEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicenseEvent();
}
public static io.bloombox.schema.licensure.Licensure.LicenseEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LicenseEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LicenseEvent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LicenseTimestampsOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.LicenseTimestamps)
com.google.protobuf.MessageOrBuilder {
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
boolean hasSubmitted();
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
io.opencannabis.schema.temporal.TemporalDate.Date getSubmitted();
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getSubmittedOrBuilder();
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
boolean hasIssued();
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
io.opencannabis.schema.temporal.TemporalDate.Date getIssued();
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getIssuedOrBuilder();
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
boolean hasReceived();
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
io.opencannabis.schema.temporal.TemporalDate.Date getReceived();
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getReceivedOrBuilder();
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
boolean hasVerified();
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getVerified();
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getVerifiedOrBuilder();
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
boolean hasApplied();
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getApplied();
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getAppliedOrBuilder();
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
boolean hasModified();
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getModified();
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder();
}
/**
*
* Specifies standard timestamps for a given regulatory license, that are generally considered immutable or at least
* only updated by changes in the license content or privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicenseTimestamps}
*/
public static final class LicenseTimestamps extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.LicenseTimestamps)
LicenseTimestampsOrBuilder {
private static final long serialVersionUID = 0L;
// Use LicenseTimestamps.newBuilder() to construct.
private LicenseTimestamps(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LicenseTimestamps() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LicenseTimestamps(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.temporal.TemporalDate.Date.Builder subBuilder = null;
if (submitted_ != null) {
subBuilder = submitted_.toBuilder();
}
submitted_ = input.readMessage(io.opencannabis.schema.temporal.TemporalDate.Date.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(submitted_);
submitted_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalDate.Date.Builder subBuilder = null;
if (issued_ != null) {
subBuilder = issued_.toBuilder();
}
issued_ = input.readMessage(io.opencannabis.schema.temporal.TemporalDate.Date.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(issued_);
issued_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalDate.Date.Builder subBuilder = null;
if (received_ != null) {
subBuilder = received_.toBuilder();
}
received_ = input.readMessage(io.opencannabis.schema.temporal.TemporalDate.Date.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(received_);
received_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (verified_ != null) {
subBuilder = verified_.toBuilder();
}
verified_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(verified_);
verified_ = subBuilder.buildPartial();
}
break;
}
case 42: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (applied_ != null) {
subBuilder = applied_.toBuilder();
}
applied_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(applied_);
applied_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (modified_ != null) {
subBuilder = modified_.toBuilder();
}
modified_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(modified_);
modified_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.class, io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder.class);
}
public static final int SUBMITTED_FIELD_NUMBER = 1;
private io.opencannabis.schema.temporal.TemporalDate.Date submitted_;
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public boolean hasSubmitted() {
return submitted_ != null;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getSubmitted() {
return submitted_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : submitted_;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getSubmittedOrBuilder() {
return getSubmitted();
}
public static final int ISSUED_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalDate.Date issued_;
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public boolean hasIssued() {
return issued_ != null;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getIssued() {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : issued_;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getIssuedOrBuilder() {
return getIssued();
}
public static final int RECEIVED_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalDate.Date received_;
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public boolean hasReceived() {
return received_ != null;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getReceived() {
return received_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : received_;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getReceivedOrBuilder() {
return getReceived();
}
public static final int VERIFIED_FIELD_NUMBER = 4;
private io.opencannabis.schema.temporal.TemporalInstant.Instant verified_;
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public boolean hasVerified() {
return verified_ != null;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getVerified() {
return verified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : verified_;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getVerifiedOrBuilder() {
return getVerified();
}
public static final int APPLIED_FIELD_NUMBER = 5;
private io.opencannabis.schema.temporal.TemporalInstant.Instant applied_;
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public boolean hasApplied() {
return applied_ != null;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getApplied() {
return applied_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : applied_;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getAppliedOrBuilder() {
return getApplied();
}
public static final int MODIFIED_FIELD_NUMBER = 6;
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public boolean hasModified() {
return modified_ != null;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
return getModified();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (submitted_ != null) {
output.writeMessage(1, getSubmitted());
}
if (issued_ != null) {
output.writeMessage(2, getIssued());
}
if (received_ != null) {
output.writeMessage(3, getReceived());
}
if (verified_ != null) {
output.writeMessage(4, getVerified());
}
if (applied_ != null) {
output.writeMessage(5, getApplied());
}
if (modified_ != null) {
output.writeMessage(6, getModified());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (submitted_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getSubmitted());
}
if (issued_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIssued());
}
if (received_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getReceived());
}
if (verified_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getVerified());
}
if (applied_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getApplied());
}
if (modified_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getModified());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.LicenseTimestamps)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.LicenseTimestamps other = (io.bloombox.schema.licensure.Licensure.LicenseTimestamps) obj;
if (hasSubmitted() != other.hasSubmitted()) return false;
if (hasSubmitted()) {
if (!getSubmitted()
.equals(other.getSubmitted())) return false;
}
if (hasIssued() != other.hasIssued()) return false;
if (hasIssued()) {
if (!getIssued()
.equals(other.getIssued())) return false;
}
if (hasReceived() != other.hasReceived()) return false;
if (hasReceived()) {
if (!getReceived()
.equals(other.getReceived())) return false;
}
if (hasVerified() != other.hasVerified()) return false;
if (hasVerified()) {
if (!getVerified()
.equals(other.getVerified())) return false;
}
if (hasApplied() != other.hasApplied()) return false;
if (hasApplied()) {
if (!getApplied()
.equals(other.getApplied())) return false;
}
if (hasModified() != other.hasModified()) return false;
if (hasModified()) {
if (!getModified()
.equals(other.getModified())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSubmitted()) {
hash = (37 * hash) + SUBMITTED_FIELD_NUMBER;
hash = (53 * hash) + getSubmitted().hashCode();
}
if (hasIssued()) {
hash = (37 * hash) + ISSUED_FIELD_NUMBER;
hash = (53 * hash) + getIssued().hashCode();
}
if (hasReceived()) {
hash = (37 * hash) + RECEIVED_FIELD_NUMBER;
hash = (53 * hash) + getReceived().hashCode();
}
if (hasVerified()) {
hash = (37 * hash) + VERIFIED_FIELD_NUMBER;
hash = (53 * hash) + getVerified().hashCode();
}
if (hasApplied()) {
hash = (37 * hash) + APPLIED_FIELD_NUMBER;
hash = (53 * hash) + getApplied().hashCode();
}
if (hasModified()) {
hash = (37 * hash) + MODIFIED_FIELD_NUMBER;
hash = (53 * hash) + getModified().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.LicenseTimestamps prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies standard timestamps for a given regulatory license, that are generally considered immutable or at least
* only updated by changes in the license content or privileges.
*
*
* Protobuf type {@code bloombox.licensure.LicenseTimestamps}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.LicenseTimestamps)
io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.class, io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.LicenseTimestamps.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (submittedBuilder_ == null) {
submitted_ = null;
} else {
submitted_ = null;
submittedBuilder_ = null;
}
if (issuedBuilder_ == null) {
issued_ = null;
} else {
issued_ = null;
issuedBuilder_ = null;
}
if (receivedBuilder_ == null) {
received_ = null;
} else {
received_ = null;
receivedBuilder_ = null;
}
if (verifiedBuilder_ == null) {
verified_ = null;
} else {
verified_ = null;
verifiedBuilder_ = null;
}
if (appliedBuilder_ == null) {
applied_ = null;
} else {
applied_ = null;
appliedBuilder_ = null;
}
if (modifiedBuilder_ == null) {
modified_ = null;
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_LicenseTimestamps_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.LicenseTimestamps.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps build() {
io.bloombox.schema.licensure.Licensure.LicenseTimestamps result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps buildPartial() {
io.bloombox.schema.licensure.Licensure.LicenseTimestamps result = new io.bloombox.schema.licensure.Licensure.LicenseTimestamps(this);
if (submittedBuilder_ == null) {
result.submitted_ = submitted_;
} else {
result.submitted_ = submittedBuilder_.build();
}
if (issuedBuilder_ == null) {
result.issued_ = issued_;
} else {
result.issued_ = issuedBuilder_.build();
}
if (receivedBuilder_ == null) {
result.received_ = received_;
} else {
result.received_ = receivedBuilder_.build();
}
if (verifiedBuilder_ == null) {
result.verified_ = verified_;
} else {
result.verified_ = verifiedBuilder_.build();
}
if (appliedBuilder_ == null) {
result.applied_ = applied_;
} else {
result.applied_ = appliedBuilder_.build();
}
if (modifiedBuilder_ == null) {
result.modified_ = modified_;
} else {
result.modified_ = modifiedBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.LicenseTimestamps) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.LicenseTimestamps)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.LicenseTimestamps other) {
if (other == io.bloombox.schema.licensure.Licensure.LicenseTimestamps.getDefaultInstance()) return this;
if (other.hasSubmitted()) {
mergeSubmitted(other.getSubmitted());
}
if (other.hasIssued()) {
mergeIssued(other.getIssued());
}
if (other.hasReceived()) {
mergeReceived(other.getReceived());
}
if (other.hasVerified()) {
mergeVerified(other.getVerified());
}
if (other.hasApplied()) {
mergeApplied(other.getApplied());
}
if (other.hasModified()) {
mergeModified(other.getModified());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.LicenseTimestamps parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.LicenseTimestamps) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.temporal.TemporalDate.Date submitted_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder> submittedBuilder_;
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public boolean hasSubmitted() {
return submittedBuilder_ != null || submitted_ != null;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getSubmitted() {
if (submittedBuilder_ == null) {
return submitted_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : submitted_;
} else {
return submittedBuilder_.getMessage();
}
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public Builder setSubmitted(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (submittedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
submitted_ = value;
onChanged();
} else {
submittedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public Builder setSubmitted(
io.opencannabis.schema.temporal.TemporalDate.Date.Builder builderForValue) {
if (submittedBuilder_ == null) {
submitted_ = builderForValue.build();
onChanged();
} else {
submittedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public Builder mergeSubmitted(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (submittedBuilder_ == null) {
if (submitted_ != null) {
submitted_ =
io.opencannabis.schema.temporal.TemporalDate.Date.newBuilder(submitted_).mergeFrom(value).buildPartial();
} else {
submitted_ = value;
}
onChanged();
} else {
submittedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public Builder clearSubmitted() {
if (submittedBuilder_ == null) {
submitted_ = null;
onChanged();
} else {
submitted_ = null;
submittedBuilder_ = null;
}
return this;
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date.Builder getSubmittedBuilder() {
onChanged();
return getSubmittedFieldBuilder().getBuilder();
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getSubmittedOrBuilder() {
if (submittedBuilder_ != null) {
return submittedBuilder_.getMessageOrBuilder();
} else {
return submitted_ == null ?
io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : submitted_;
}
}
/**
*
* Date the license application was submitted to the jurisdictional or licensing agency.
*
*
* .opencannabis.temporal.Date submitted = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>
getSubmittedFieldBuilder() {
if (submittedBuilder_ == null) {
submittedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>(
getSubmitted(),
getParentForChildren(),
isClean());
submitted_ = null;
}
return submittedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalDate.Date issued_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder> issuedBuilder_;
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public boolean hasIssued() {
return issuedBuilder_ != null || issued_ != null;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getIssued() {
if (issuedBuilder_ == null) {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : issued_;
} else {
return issuedBuilder_.getMessage();
}
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public Builder setIssued(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (issuedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
issued_ = value;
onChanged();
} else {
issuedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public Builder setIssued(
io.opencannabis.schema.temporal.TemporalDate.Date.Builder builderForValue) {
if (issuedBuilder_ == null) {
issued_ = builderForValue.build();
onChanged();
} else {
issuedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public Builder mergeIssued(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (issuedBuilder_ == null) {
if (issued_ != null) {
issued_ =
io.opencannabis.schema.temporal.TemporalDate.Date.newBuilder(issued_).mergeFrom(value).buildPartial();
} else {
issued_ = value;
}
onChanged();
} else {
issuedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public Builder clearIssued() {
if (issuedBuilder_ == null) {
issued_ = null;
onChanged();
} else {
issued_ = null;
issuedBuilder_ = null;
}
return this;
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date.Builder getIssuedBuilder() {
onChanged();
return getIssuedFieldBuilder().getBuilder();
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getIssuedOrBuilder() {
if (issuedBuilder_ != null) {
return issuedBuilder_.getMessageOrBuilder();
} else {
return issued_ == null ?
io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : issued_;
}
}
/**
*
* Date the license was issued.
*
*
* .opencannabis.temporal.Date issued = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>
getIssuedFieldBuilder() {
if (issuedBuilder_ == null) {
issuedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>(
getIssued(),
getParentForChildren(),
isClean());
issued_ = null;
}
return issuedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalDate.Date received_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder> receivedBuilder_;
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public boolean hasReceived() {
return receivedBuilder_ != null || received_ != null;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date getReceived() {
if (receivedBuilder_ == null) {
return received_ == null ? io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : received_;
} else {
return receivedBuilder_.getMessage();
}
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public Builder setReceived(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (receivedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
received_ = value;
onChanged();
} else {
receivedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public Builder setReceived(
io.opencannabis.schema.temporal.TemporalDate.Date.Builder builderForValue) {
if (receivedBuilder_ == null) {
received_ = builderForValue.build();
onChanged();
} else {
receivedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public Builder mergeReceived(io.opencannabis.schema.temporal.TemporalDate.Date value) {
if (receivedBuilder_ == null) {
if (received_ != null) {
received_ =
io.opencannabis.schema.temporal.TemporalDate.Date.newBuilder(received_).mergeFrom(value).buildPartial();
} else {
received_ = value;
}
onChanged();
} else {
receivedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public Builder clearReceived() {
if (receivedBuilder_ == null) {
received_ = null;
onChanged();
} else {
received_ = null;
receivedBuilder_ = null;
}
return this;
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public io.opencannabis.schema.temporal.TemporalDate.Date.Builder getReceivedBuilder() {
onChanged();
return getReceivedFieldBuilder().getBuilder();
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
public io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder getReceivedOrBuilder() {
if (receivedBuilder_ != null) {
return receivedBuilder_.getMessageOrBuilder();
} else {
return received_ == null ?
io.opencannabis.schema.temporal.TemporalDate.Date.getDefaultInstance() : received_;
}
}
/**
*
* Date the license was processed as an incoming payload by ledger systems.
*
*
* .opencannabis.temporal.Date received = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>
getReceivedFieldBuilder() {
if (receivedBuilder_ == null) {
receivedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalDate.Date, io.opencannabis.schema.temporal.TemporalDate.Date.Builder, io.opencannabis.schema.temporal.TemporalDate.DateOrBuilder>(
getReceived(),
getParentForChildren(),
isClean());
received_ = null;
}
return receivedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant verified_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> verifiedBuilder_;
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public boolean hasVerified() {
return verifiedBuilder_ != null || verified_ != null;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getVerified() {
if (verifiedBuilder_ == null) {
return verified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : verified_;
} else {
return verifiedBuilder_.getMessage();
}
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public Builder setVerified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (verifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
verified_ = value;
onChanged();
} else {
verifiedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public Builder setVerified(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (verifiedBuilder_ == null) {
verified_ = builderForValue.build();
onChanged();
} else {
verifiedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public Builder mergeVerified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (verifiedBuilder_ == null) {
if (verified_ != null) {
verified_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(verified_).mergeFrom(value).buildPartial();
} else {
verified_ = value;
}
onChanged();
} else {
verifiedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public Builder clearVerified() {
if (verifiedBuilder_ == null) {
verified_ = null;
onChanged();
} else {
verified_ = null;
verifiedBuilder_ = null;
}
return this;
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getVerifiedBuilder() {
onChanged();
return getVerifiedFieldBuilder().getBuilder();
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getVerifiedOrBuilder() {
if (verifiedBuilder_ != null) {
return verifiedBuilder_.getMessageOrBuilder();
} else {
return verified_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : verified_;
}
}
/**
*
* Date and time the license was last verified.
*
*
* .opencannabis.temporal.Instant verified = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getVerifiedFieldBuilder() {
if (verifiedBuilder_ == null) {
verifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getVerified(),
getParentForChildren(),
isClean());
verified_ = null;
}
return verifiedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant applied_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> appliedBuilder_;
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public boolean hasApplied() {
return appliedBuilder_ != null || applied_ != null;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getApplied() {
if (appliedBuilder_ == null) {
return applied_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : applied_;
} else {
return appliedBuilder_.getMessage();
}
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public Builder setApplied(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (appliedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
applied_ = value;
onChanged();
} else {
appliedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public Builder setApplied(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (appliedBuilder_ == null) {
applied_ = builderForValue.build();
onChanged();
} else {
appliedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public Builder mergeApplied(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (appliedBuilder_ == null) {
if (applied_ != null) {
applied_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(applied_).mergeFrom(value).buildPartial();
} else {
applied_ = value;
}
onChanged();
} else {
appliedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public Builder clearApplied() {
if (appliedBuilder_ == null) {
applied_ = null;
onChanged();
} else {
applied_ = null;
appliedBuilder_ = null;
}
return this;
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getAppliedBuilder() {
onChanged();
return getAppliedFieldBuilder().getBuilder();
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getAppliedOrBuilder() {
if (appliedBuilder_ != null) {
return appliedBuilder_.getMessageOrBuilder();
} else {
return applied_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : applied_;
}
}
/**
*
* Date and time the license was applied and committed.
*
*
* .opencannabis.temporal.Instant applied = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getAppliedFieldBuilder() {
if (appliedBuilder_ == null) {
appliedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getApplied(),
getParentForChildren(),
isClean());
applied_ = null;
}
return appliedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> modifiedBuilder_;
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public boolean hasModified() {
return modifiedBuilder_ != null || modified_ != null;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
if (modifiedBuilder_ == null) {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
} else {
return modifiedBuilder_.getMessage();
}
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public Builder setModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
modified_ = value;
onChanged();
} else {
modifiedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public Builder setModified(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (modifiedBuilder_ == null) {
modified_ = builderForValue.build();
onChanged();
} else {
modifiedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public Builder mergeModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (modified_ != null) {
modified_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(modified_).mergeFrom(value).buildPartial();
} else {
modified_ = value;
}
onChanged();
} else {
modifiedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public Builder clearModified() {
if (modifiedBuilder_ == null) {
modified_ = null;
onChanged();
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getModifiedBuilder() {
onChanged();
return getModifiedFieldBuilder().getBuilder();
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
if (modifiedBuilder_ != null) {
return modifiedBuilder_.getMessageOrBuilder();
} else {
return modified_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
}
/**
*
* Date and time the license was last modified.
*
*
* .opencannabis.temporal.Instant modified = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getModifiedFieldBuilder() {
if (modifiedBuilder_ == null) {
modifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getModified(),
getParentForChildren(),
isClean());
modified_ = null;
}
return modifiedBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.LicenseTimestamps)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.LicenseTimestamps)
private static final io.bloombox.schema.licensure.Licensure.LicenseTimestamps DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.LicenseTimestamps();
}
public static io.bloombox.schema.licensure.Licensure.LicenseTimestamps getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LicenseTimestamps parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LicenseTimestamps(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegulatoryLicenseOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.licensure.RegulatoryLicense)
com.google.protobuf.MessageOrBuilder {
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
boolean hasKey();
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.licensure.Licensure.LicenseKey getKey();
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder getKeyOrBuilder();
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
int getTypeValue();
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
io.bloombox.schema.licensure.Licensure.LicenseType getType();
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
boolean hasAuthority();
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthority getAuthority();
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder getAuthorityOrBuilder();
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
int getStatusValue();
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus();
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
java.util.List
getEventList();
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
io.bloombox.schema.licensure.Licensure.LicenseEvent getEvent(int index);
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
int getEventCount();
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
java.util.List extends io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder>
getEventOrBuilderList();
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder getEventOrBuilder(
int index);
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
java.util.List getPrivilegeList();
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
int getPrivilegeCount();
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index);
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
java.util.List
getPrivilegeValueList();
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
int getPrivilegeValue(int index);
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
boolean hasTimestamps();
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
io.bloombox.schema.licensure.Licensure.LicenseTimestamps getTimestamps();
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder getTimestampsOrBuilder();
}
/**
*
* Specifies a persistable claim with details about a regulatory license, presumably granting industrial cannabis
* participation privileges to a given licensee, under the statutory authority of a given licensing agency or
* jurisdiction. Licenses are generally considered sub-objects to existing licensee records.
*
*
* Protobuf type {@code bloombox.licensure.RegulatoryLicense}
*/
public static final class RegulatoryLicense extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.licensure.RegulatoryLicense)
RegulatoryLicenseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RegulatoryLicense.newBuilder() to construct.
private RegulatoryLicense(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RegulatoryLicense() {
type_ = 0;
status_ = 0;
event_ = java.util.Collections.emptyList();
privilege_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RegulatoryLicense(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.licensure.Licensure.LicenseKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.bloombox.schema.licensure.Licensure.LicenseKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 26: {
io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder subBuilder = null;
if (authority_ != null) {
subBuilder = authority_.toBuilder();
}
authority_ = input.readMessage(io.bloombox.schema.licensure.Licensure.LicensingAuthority.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(authority_);
authority_ = subBuilder.buildPartial();
}
break;
}
case 32: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
event_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
event_.add(
input.readMessage(io.bloombox.schema.licensure.Licensure.LicenseEvent.parser(), extensionRegistry));
break;
}
case 48: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
privilege_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
privilege_.add(rawValue);
break;
}
case 50: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
privilege_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
privilege_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 58: {
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder subBuilder = null;
if (timestamps_ != null) {
subBuilder = timestamps_.toBuilder();
}
timestamps_ = input.readMessage(io.bloombox.schema.licensure.Licensure.LicenseTimestamps.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timestamps_);
timestamps_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) != 0)) {
event_ = java.util.Collections.unmodifiableList(event_);
}
if (((mutable_bitField0_ & 0x00000020) != 0)) {
privilege_ = java.util.Collections.unmodifiableList(privilege_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_RegulatoryLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_RegulatoryLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.RegulatoryLicense.class, io.bloombox.schema.licensure.Licensure.RegulatoryLicense.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private io.bloombox.schema.licensure.Licensure.LicenseKey key_;
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.licensure.Licensure.LicenseKey getKey() {
return key_ == null ? io.bloombox.schema.licensure.Licensure.LicenseKey.getDefaultInstance() : key_;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicenseType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseType result = io.bloombox.schema.licensure.Licensure.LicenseType.valueOf(type_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseType.UNRECOGNIZED : result;
}
public static final int AUTHORITY_FIELD_NUMBER = 3;
private io.bloombox.schema.licensure.Licensure.LicensingAuthority authority_;
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public boolean hasAuthority() {
return authority_ != null;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority getAuthority() {
return authority_ == null ? io.bloombox.schema.licensure.Licensure.LicensingAuthority.getDefaultInstance() : authority_;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder getAuthorityOrBuilder() {
return getAuthority();
}
public static final int STATUS_FIELD_NUMBER = 4;
private int status_;
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseStatus result = io.bloombox.schema.licensure.Licensure.LicenseStatus.valueOf(status_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseStatus.UNRECOGNIZED : result;
}
public static final int EVENT_FIELD_NUMBER = 5;
private java.util.List event_;
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public java.util.List getEventList() {
return event_;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public java.util.List extends io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder>
getEventOrBuilderList() {
return event_;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public int getEventCount() {
return event_.size();
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEvent getEvent(int index) {
return event_.get(index);
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder getEventOrBuilder(
int index) {
return event_.get(index);
}
public static final int PRIVILEGE_FIELD_NUMBER = 6;
private java.util.List privilege_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege> privilege_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>() {
public io.bloombox.schema.licensure.Licensure.LicensePrivilege convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicensePrivilege result = io.bloombox.schema.licensure.Licensure.LicensePrivilege.valueOf(from);
return result == null ? io.bloombox.schema.licensure.Licensure.LicensePrivilege.UNRECOGNIZED : result;
}
};
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public java.util.List getPrivilegeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>(privilege_, privilege_converter_);
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public int getPrivilegeCount() {
return privilege_.size();
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index) {
return privilege_converter_.convert(privilege_.get(index));
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public java.util.List
getPrivilegeValueList() {
return privilege_;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public int getPrivilegeValue(int index) {
return privilege_.get(index);
}
private int privilegeMemoizedSerializedSize;
public static final int TIMESTAMPS_FIELD_NUMBER = 7;
private io.bloombox.schema.licensure.Licensure.LicenseTimestamps timestamps_;
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public boolean hasTimestamps() {
return timestamps_ != null;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps getTimestamps() {
return timestamps_ == null ? io.bloombox.schema.licensure.Licensure.LicenseTimestamps.getDefaultInstance() : timestamps_;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder getTimestampsOrBuilder() {
return getTimestamps();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (type_ != io.bloombox.schema.licensure.Licensure.LicenseType.PERMANENT.getNumber()) {
output.writeEnum(2, type_);
}
if (authority_ != null) {
output.writeMessage(3, getAuthority());
}
if (status_ != io.bloombox.schema.licensure.Licensure.LicenseStatus.PENDING.getNumber()) {
output.writeEnum(4, status_);
}
for (int i = 0; i < event_.size(); i++) {
output.writeMessage(5, event_.get(i));
}
if (getPrivilegeList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(privilegeMemoizedSerializedSize);
}
for (int i = 0; i < privilege_.size(); i++) {
output.writeEnumNoTag(privilege_.get(i));
}
if (timestamps_ != null) {
output.writeMessage(7, getTimestamps());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (type_ != io.bloombox.schema.licensure.Licensure.LicenseType.PERMANENT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
if (authority_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getAuthority());
}
if (status_ != io.bloombox.schema.licensure.Licensure.LicenseStatus.PENDING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, status_);
}
for (int i = 0; i < event_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, event_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < privilege_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(privilege_.get(i));
}
size += dataSize;
if (!getPrivilegeList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}privilegeMemoizedSerializedSize = dataSize;
}
if (timestamps_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getTimestamps());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.licensure.Licensure.RegulatoryLicense)) {
return super.equals(obj);
}
io.bloombox.schema.licensure.Licensure.RegulatoryLicense other = (io.bloombox.schema.licensure.Licensure.RegulatoryLicense) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (type_ != other.type_) return false;
if (hasAuthority() != other.hasAuthority()) return false;
if (hasAuthority()) {
if (!getAuthority()
.equals(other.getAuthority())) return false;
}
if (status_ != other.status_) return false;
if (!getEventList()
.equals(other.getEventList())) return false;
if (!privilege_.equals(other.privilege_)) return false;
if (hasTimestamps() != other.hasTimestamps()) return false;
if (hasTimestamps()) {
if (!getTimestamps()
.equals(other.getTimestamps())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasAuthority()) {
hash = (37 * hash) + AUTHORITY_FIELD_NUMBER;
hash = (53 * hash) + getAuthority().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (getEventCount() > 0) {
hash = (37 * hash) + EVENT_FIELD_NUMBER;
hash = (53 * hash) + getEventList().hashCode();
}
if (getPrivilegeCount() > 0) {
hash = (37 * hash) + PRIVILEGE_FIELD_NUMBER;
hash = (53 * hash) + privilege_.hashCode();
}
if (hasTimestamps()) {
hash = (37 * hash) + TIMESTAMPS_FIELD_NUMBER;
hash = (53 * hash) + getTimestamps().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.licensure.Licensure.RegulatoryLicense prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a persistable claim with details about a regulatory license, presumably granting industrial cannabis
* participation privileges to a given licensee, under the statutory authority of a given licensing agency or
* jurisdiction. Licenses are generally considered sub-objects to existing licensee records.
*
*
* Protobuf type {@code bloombox.licensure.RegulatoryLicense}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.licensure.RegulatoryLicense)
io.bloombox.schema.licensure.Licensure.RegulatoryLicenseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_RegulatoryLicense_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_RegulatoryLicense_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.licensure.Licensure.RegulatoryLicense.class, io.bloombox.schema.licensure.Licensure.RegulatoryLicense.Builder.class);
}
// Construct using io.bloombox.schema.licensure.Licensure.RegulatoryLicense.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEventFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
type_ = 0;
if (authorityBuilder_ == null) {
authority_ = null;
} else {
authority_ = null;
authorityBuilder_ = null;
}
status_ = 0;
if (eventBuilder_ == null) {
event_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
eventBuilder_.clear();
}
privilege_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
if (timestampsBuilder_ == null) {
timestamps_ = null;
} else {
timestamps_ = null;
timestampsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.licensure.Licensure.internal_static_bloombox_licensure_RegulatoryLicense_descriptor;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.RegulatoryLicense getDefaultInstanceForType() {
return io.bloombox.schema.licensure.Licensure.RegulatoryLicense.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.RegulatoryLicense build() {
io.bloombox.schema.licensure.Licensure.RegulatoryLicense result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.RegulatoryLicense buildPartial() {
io.bloombox.schema.licensure.Licensure.RegulatoryLicense result = new io.bloombox.schema.licensure.Licensure.RegulatoryLicense(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
result.type_ = type_;
if (authorityBuilder_ == null) {
result.authority_ = authority_;
} else {
result.authority_ = authorityBuilder_.build();
}
result.status_ = status_;
if (eventBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
event_ = java.util.Collections.unmodifiableList(event_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.event_ = event_;
} else {
result.event_ = eventBuilder_.build();
}
if (((bitField0_ & 0x00000020) != 0)) {
privilege_ = java.util.Collections.unmodifiableList(privilege_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.privilege_ = privilege_;
if (timestampsBuilder_ == null) {
result.timestamps_ = timestamps_;
} else {
result.timestamps_ = timestampsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.licensure.Licensure.RegulatoryLicense) {
return mergeFrom((io.bloombox.schema.licensure.Licensure.RegulatoryLicense)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.licensure.Licensure.RegulatoryLicense other) {
if (other == io.bloombox.schema.licensure.Licensure.RegulatoryLicense.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasAuthority()) {
mergeAuthority(other.getAuthority());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (eventBuilder_ == null) {
if (!other.event_.isEmpty()) {
if (event_.isEmpty()) {
event_ = other.event_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureEventIsMutable();
event_.addAll(other.event_);
}
onChanged();
}
} else {
if (!other.event_.isEmpty()) {
if (eventBuilder_.isEmpty()) {
eventBuilder_.dispose();
eventBuilder_ = null;
event_ = other.event_;
bitField0_ = (bitField0_ & ~0x00000010);
eventBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEventFieldBuilder() : null;
} else {
eventBuilder_.addAllMessages(other.event_);
}
}
}
if (!other.privilege_.isEmpty()) {
if (privilege_.isEmpty()) {
privilege_ = other.privilege_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensurePrivilegeIsMutable();
privilege_.addAll(other.privilege_);
}
onChanged();
}
if (other.hasTimestamps()) {
mergeTimestamps(other.getTimestamps());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.licensure.Licensure.RegulatoryLicense parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.licensure.Licensure.RegulatoryLicense) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private io.bloombox.schema.licensure.Licensure.LicenseKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseKey, io.bloombox.schema.licensure.Licensure.LicenseKey.Builder, io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder> keyBuilder_;
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.licensure.Licensure.LicenseKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.bloombox.schema.licensure.Licensure.LicenseKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(io.bloombox.schema.licensure.Licensure.LicenseKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(
io.bloombox.schema.licensure.Licensure.LicenseKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public Builder mergeKey(io.bloombox.schema.licensure.Licensure.LicenseKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.bloombox.schema.licensure.Licensure.LicenseKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.licensure.Licensure.LicenseKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.bloombox.schema.licensure.Licensure.LicenseKey.getDefaultInstance() : key_;
}
}
/**
*
* Primary key for a regulatory license record. This includes an auto-generated UUID, and any external license ID
* allocated and issued by the licensing agency.
*
*
* .bloombox.licensure.LicenseKey key = 1 [(.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseKey, io.bloombox.schema.licensure.Licensure.LicenseKey.Builder, io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseKey, io.bloombox.schema.licensure.Licensure.LicenseKey.Builder, io.bloombox.schema.licensure.Licensure.LicenseKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private int type_ = 0;
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public io.bloombox.schema.licensure.Licensure.LicenseType getType() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseType result = io.bloombox.schema.licensure.Licensure.LicenseType.valueOf(type_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseType.UNRECOGNIZED : result;
}
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public Builder setType(io.bloombox.schema.licensure.Licensure.LicenseType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the main type of a given regulatory license. These types are enumerated in the declared type, but
* generally, this value is either 'temporary' or 'permanent'.
*
*
* .bloombox.licensure.LicenseType type = 2;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private io.bloombox.schema.licensure.Licensure.LicensingAuthority authority_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority, io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder> authorityBuilder_;
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public boolean hasAuthority() {
return authorityBuilder_ != null || authority_ != null;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority getAuthority() {
if (authorityBuilder_ == null) {
return authority_ == null ? io.bloombox.schema.licensure.Licensure.LicensingAuthority.getDefaultInstance() : authority_;
} else {
return authorityBuilder_.getMessage();
}
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public Builder setAuthority(io.bloombox.schema.licensure.Licensure.LicensingAuthority value) {
if (authorityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
authority_ = value;
onChanged();
} else {
authorityBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public Builder setAuthority(
io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder builderForValue) {
if (authorityBuilder_ == null) {
authority_ = builderForValue.build();
onChanged();
} else {
authorityBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public Builder mergeAuthority(io.bloombox.schema.licensure.Licensure.LicensingAuthority value) {
if (authorityBuilder_ == null) {
if (authority_ != null) {
authority_ =
io.bloombox.schema.licensure.Licensure.LicensingAuthority.newBuilder(authority_).mergeFrom(value).buildPartial();
} else {
authority_ = value;
}
onChanged();
} else {
authorityBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public Builder clearAuthority() {
if (authorityBuilder_ == null) {
authority_ = null;
onChanged();
} else {
authority_ = null;
authorityBuilder_ = null;
}
return this;
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder getAuthorityBuilder() {
onChanged();
return getAuthorityFieldBuilder().getBuilder();
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
public io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder getAuthorityOrBuilder() {
if (authorityBuilder_ != null) {
return authorityBuilder_.getMessageOrBuilder();
} else {
return authority_ == null ?
io.bloombox.schema.licensure.Licensure.LicensingAuthority.getDefaultInstance() : authority_;
}
}
/**
*
* Specifies the agency or jurisdiction with which this license was or will be issued. It is under the authority of
* this governing body that the privileges embedded in this license apply to the licensee.
*
*
* .bloombox.licensure.LicensingAuthority authority = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority, io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder>
getAuthorityFieldBuilder() {
if (authorityBuilder_ == null) {
authorityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicensingAuthority, io.bloombox.schema.licensure.Licensure.LicensingAuthority.Builder, io.bloombox.schema.licensure.Licensure.LicensingAuthorityOrBuilder>(
getAuthority(),
getParentForChildren(),
isClean());
authority_ = null;
}
return authorityBuilder_;
}
private int status_ = 0;
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public io.bloombox.schema.licensure.Licensure.LicenseStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.licensure.Licensure.LicenseStatus result = io.bloombox.schema.licensure.Licensure.LicenseStatus.valueOf(status_);
return result == null ? io.bloombox.schema.licensure.Licensure.LicenseStatus.UNRECOGNIZED : result;
}
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public Builder setStatus(io.bloombox.schema.licensure.Licensure.LicenseStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Indicate the current, up-to-date status of this regulatory license record, considering all available informaiton.
* Docs about each state are available on the declared type.
*
*
* .bloombox.licensure.LicenseStatus status = 4;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.util.List event_ =
java.util.Collections.emptyList();
private void ensureEventIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
event_ = new java.util.ArrayList(event_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseEvent, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder, io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder> eventBuilder_;
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public java.util.List getEventList() {
if (eventBuilder_ == null) {
return java.util.Collections.unmodifiableList(event_);
} else {
return eventBuilder_.getMessageList();
}
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public int getEventCount() {
if (eventBuilder_ == null) {
return event_.size();
} else {
return eventBuilder_.getCount();
}
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEvent getEvent(int index) {
if (eventBuilder_ == null) {
return event_.get(index);
} else {
return eventBuilder_.getMessage(index);
}
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder setEvent(
int index, io.bloombox.schema.licensure.Licensure.LicenseEvent value) {
if (eventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventIsMutable();
event_.set(index, value);
onChanged();
} else {
eventBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder setEvent(
int index, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder builderForValue) {
if (eventBuilder_ == null) {
ensureEventIsMutable();
event_.set(index, builderForValue.build());
onChanged();
} else {
eventBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder addEvent(io.bloombox.schema.licensure.Licensure.LicenseEvent value) {
if (eventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventIsMutable();
event_.add(value);
onChanged();
} else {
eventBuilder_.addMessage(value);
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder addEvent(
int index, io.bloombox.schema.licensure.Licensure.LicenseEvent value) {
if (eventBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventIsMutable();
event_.add(index, value);
onChanged();
} else {
eventBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder addEvent(
io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder builderForValue) {
if (eventBuilder_ == null) {
ensureEventIsMutable();
event_.add(builderForValue.build());
onChanged();
} else {
eventBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder addEvent(
int index, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder builderForValue) {
if (eventBuilder_ == null) {
ensureEventIsMutable();
event_.add(index, builderForValue.build());
onChanged();
} else {
eventBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder addAllEvent(
java.lang.Iterable extends io.bloombox.schema.licensure.Licensure.LicenseEvent> values) {
if (eventBuilder_ == null) {
ensureEventIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, event_);
onChanged();
} else {
eventBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder clearEvent() {
if (eventBuilder_ == null) {
event_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
eventBuilder_.clear();
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public Builder removeEvent(int index) {
if (eventBuilder_ == null) {
ensureEventIsMutable();
event_.remove(index);
onChanged();
} else {
eventBuilder_.remove(index);
}
return this;
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder getEventBuilder(
int index) {
return getEventFieldBuilder().getBuilder(index);
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder getEventOrBuilder(
int index) {
if (eventBuilder_ == null) {
return event_.get(index); } else {
return eventBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public java.util.List extends io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder>
getEventOrBuilderList() {
if (eventBuilder_ != null) {
return eventBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(event_);
}
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder addEventBuilder() {
return getEventFieldBuilder().addBuilder(
io.bloombox.schema.licensure.Licensure.LicenseEvent.getDefaultInstance());
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder addEventBuilder(
int index) {
return getEventFieldBuilder().addBuilder(
index, io.bloombox.schema.licensure.Licensure.LicenseEvent.getDefaultInstance());
}
/**
*
* History of events as they occurred, to this license, or involving this license. When a license record is first
* allocated, this property is left empty, and begins with an entry after the first mutation of a license record.
*
*
* repeated .bloombox.licensure.LicenseEvent event = 5;
*/
public java.util.List
getEventBuilderList() {
return getEventFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseEvent, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder, io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder>
getEventFieldBuilder() {
if (eventBuilder_ == null) {
eventBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseEvent, io.bloombox.schema.licensure.Licensure.LicenseEvent.Builder, io.bloombox.schema.licensure.Licensure.LicenseEventOrBuilder>(
event_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
event_ = null;
}
return eventBuilder_;
}
private java.util.List privilege_ =
java.util.Collections.emptyList();
private void ensurePrivilegeIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
privilege_ = new java.util.ArrayList(privilege_);
bitField0_ |= 0x00000020;
}
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public java.util.List getPrivilegeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.bloombox.schema.licensure.Licensure.LicensePrivilege>(privilege_, privilege_converter_);
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public int getPrivilegeCount() {
return privilege_.size();
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public io.bloombox.schema.licensure.Licensure.LicensePrivilege getPrivilege(int index) {
return privilege_converter_.convert(privilege_.get(index));
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder setPrivilege(
int index, io.bloombox.schema.licensure.Licensure.LicensePrivilege value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrivilegeIsMutable();
privilege_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder addPrivilege(io.bloombox.schema.licensure.Licensure.LicensePrivilege value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrivilegeIsMutable();
privilege_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder addAllPrivilege(
java.lang.Iterable extends io.bloombox.schema.licensure.Licensure.LicensePrivilege> values) {
ensurePrivilegeIsMutable();
for (io.bloombox.schema.licensure.Licensure.LicensePrivilege value : values) {
privilege_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder clearPrivilege() {
privilege_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public java.util.List
getPrivilegeValueList() {
return java.util.Collections.unmodifiableList(privilege_);
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public int getPrivilegeValue(int index) {
return privilege_.get(index);
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder setPrivilegeValue(
int index, int value) {
ensurePrivilegeIsMutable();
privilege_.set(index, value);
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder addPrivilegeValue(int value) {
ensurePrivilegeIsMutable();
privilege_.add(value);
onChanged();
return this;
}
/**
*
* Specifies privileges granted pursuant to the issuance, and validity, of this license. Privileges specified are used
* to govern behavior and obtain authorization for various actions.
*
*
* repeated .bloombox.licensure.LicensePrivilege privilege = 6;
*/
public Builder addAllPrivilegeValue(
java.lang.Iterable values) {
ensurePrivilegeIsMutable();
for (int value : values) {
privilege_.add(value);
}
onChanged();
return this;
}
private io.bloombox.schema.licensure.Licensure.LicenseTimestamps timestamps_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseTimestamps, io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder, io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder> timestampsBuilder_;
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public boolean hasTimestamps() {
return timestampsBuilder_ != null || timestamps_ != null;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps getTimestamps() {
if (timestampsBuilder_ == null) {
return timestamps_ == null ? io.bloombox.schema.licensure.Licensure.LicenseTimestamps.getDefaultInstance() : timestamps_;
} else {
return timestampsBuilder_.getMessage();
}
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public Builder setTimestamps(io.bloombox.schema.licensure.Licensure.LicenseTimestamps value) {
if (timestampsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timestamps_ = value;
onChanged();
} else {
timestampsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public Builder setTimestamps(
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder builderForValue) {
if (timestampsBuilder_ == null) {
timestamps_ = builderForValue.build();
onChanged();
} else {
timestampsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public Builder mergeTimestamps(io.bloombox.schema.licensure.Licensure.LicenseTimestamps value) {
if (timestampsBuilder_ == null) {
if (timestamps_ != null) {
timestamps_ =
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.newBuilder(timestamps_).mergeFrom(value).buildPartial();
} else {
timestamps_ = value;
}
onChanged();
} else {
timestampsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public Builder clearTimestamps() {
if (timestampsBuilder_ == null) {
timestamps_ = null;
onChanged();
} else {
timestamps_ = null;
timestampsBuilder_ = null;
}
return this;
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder getTimestampsBuilder() {
onChanged();
return getTimestampsFieldBuilder().getBuilder();
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
public io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder getTimestampsOrBuilder() {
if (timestampsBuilder_ != null) {
return timestampsBuilder_.getMessageOrBuilder();
} else {
return timestamps_ == null ?
io.bloombox.schema.licensure.Licensure.LicenseTimestamps.getDefaultInstance() : timestamps_;
}
}
/**
*
* Timestamps that record when various actions or states became active.
*
*
* .bloombox.licensure.LicenseTimestamps timestamps = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseTimestamps, io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder, io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder>
getTimestampsFieldBuilder() {
if (timestampsBuilder_ == null) {
timestampsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.licensure.Licensure.LicenseTimestamps, io.bloombox.schema.licensure.Licensure.LicenseTimestamps.Builder, io.bloombox.schema.licensure.Licensure.LicenseTimestampsOrBuilder>(
getTimestamps(),
getParentForChildren(),
isClean());
timestamps_ = null;
}
return timestampsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.licensure.RegulatoryLicense)
}
// @@protoc_insertion_point(class_scope:bloombox.licensure.RegulatoryLicense)
private static final io.bloombox.schema.licensure.Licensure.RegulatoryLicense DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.licensure.Licensure.RegulatoryLicense();
}
public static io.bloombox.schema.licensure.Licensure.RegulatoryLicense getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RegulatoryLicense parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegulatoryLicense(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.licensure.Licensure.RegulatoryLicense getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicensingAuthority_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicensingAuthority_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicensingAuthority_StateLicense_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicenseKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicenseKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicenseEvent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicenseEvent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_LicenseTimestamps_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_LicenseTimestamps_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_licensure_RegulatoryLicense_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_licensure_RegulatoryLicense_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031licensing/Licensure.proto\022\022bloombox.li" +
"censure\032\024core/Datamodel.proto\032\021geo/USSta" +
"te.proto\032\031geo/usa/ca/CACounty.proto\032\023tem" +
"poral/Date.proto\032\026temporal/Instant.proto" +
"\032 regulatory/usa/ca/CAAgency.proto\"\205\007\n\022L" +
"icensingAuthority\022D\n\005local\030\001 \001(\01323.bloom" +
"box.licensure.LicensingAuthority.LocalLi" +
"censeH\000\022F\n\006county\030\002 \001(\01324.bloombox.licen" +
"sure.LicensingAuthority.CountyLicenseH\000\022" +
"D\n\005state\030\003 \001(\01323.bloombox.licensure.Lice" +
"nsingAuthority.StateLicenseH\000\032\345\001\n\014LocalL" +
"icense\022\024\n\014municipality\030\001 \001(\t\022\025\n\013county_n" +
"ame\030\002 \001(\tH\000\022F\n\021california_county\030\003 \001(\0162)" +
".opencannabis.geo.usa.ca.CaliforniaCount" +
"yH\000\022\027\n\rprovince_name\030\004 \001(\tH\001\0221\n\010us_state" +
"\030\005 \001(\0162\035.opencannabis.geo.usa.USStateH\001B" +
"\010\n\006countyB\n\n\010province\032\311\001\n\rCountyLicense\022" +
"\016\n\004name\030\001 \001(\tH\000\022F\n\021california_county\030\002 \001" +
"(\0162).opencannabis.geo.usa.ca.CaliforniaC" +
"ountyH\000\022\027\n\rprovince_name\030\004 \001(\tH\001\0221\n\010us_s" +
"tate\030\005 \001(\0162\035.opencannabis.geo.usa.USStat" +
"eH\001B\010\n\006countyB\n\n\010province\032\326\001\n\014StateLicen" +
"se\022\027\n\rprovince_name\030\001 \001(\tH\000\0221\n\010us_state\030" +
"\002 \001(\0162\035.opencannabis.geo.usa.USStateH\000\022\025" +
"\n\013agency_name\030\003 \001(\tH\001\022M\n\021california_agen" +
"cy\030\004 \001(\01620.opencannabis.regulatory.usa.c" +
"a.CaliforniaAgencyH\001B\n\n\010provinceB\010\n\006agen" +
"cyB\016\n\014jurisdiction\"-\n\nLicenseKey\022\022\n\002id\030\001" +
" \001(\tB\006\302\265\003\002\010\002\022\013\n\003jid\030\002 \001(\t\"\275\001\n\014LicenseEve" +
"nt\0221\n\006status\030\001 \001(\0162!.bloombox.licensure." +
"LicenseStatus\0227\n\tprivilege\030\002 \003(\0162$.bloom" +
"box.licensure.LicensePrivilege\022\017\n\007messag" +
"e\030\003 \001(\t\0220\n\010occurred\030\004 \001(\0132\036.opencannabis" +
".temporal.Instant\"\264\002\n\021LicenseTimestamps\022" +
".\n\tsubmitted\030\001 \001(\0132\033.opencannabis.tempor" +
"al.Date\022+\n\006issued\030\002 \001(\0132\033.opencannabis.t" +
"emporal.Date\022-\n\010received\030\003 \001(\0132\033.opencan" +
"nabis.temporal.Date\0220\n\010verified\030\004 \001(\0132\036." +
"opencannabis.temporal.Instant\022/\n\007applied" +
"\030\005 \001(\0132\036.opencannabis.temporal.Instant\0220" +
"\n\010modified\030\006 \001(\0132\036.opencannabis.temporal" +
".Instant\"\234\003\n\021RegulatoryLicense\0223\n\003key\030\001 " +
"\001(\0132\036.bloombox.licensure.LicenseKeyB\006\302\265\003" +
"\002\010\001\022-\n\004type\030\002 \001(\0162\037.bloombox.licensure.L" +
"icenseType\0229\n\tauthority\030\003 \001(\0132&.bloombox" +
".licensure.LicensingAuthority\0221\n\006status\030" +
"\004 \001(\0162!.bloombox.licensure.LicenseStatus" +
"\022/\n\005event\030\005 \003(\0132 .bloombox.licensure.Lic" +
"enseEvent\0227\n\tprivilege\030\006 \003(\0162$.bloombox." +
"licensure.LicensePrivilege\0229\n\ntimestamps" +
"\030\007 \001(\0132%.bloombox.licensure.LicenseTimes" +
"tamps:\020\202\367\002\014\010\002\022\010licenses*9\n\013LicenseType\022\r" +
"\n\tPERMANENT\020\000\022\r\n\tTEMPORARY\020\001\022\014\n\010COMPOUND" +
"\020\002*\263\001\n\020LicensePrivilege\022\023\n\017UNKNOWN_LICEN" +
"SE\020\000\022\017\n\013CULTIVATION\020\001\022\020\n\014DISTRIBUTION\020\002\022" +
"\r\n\tTRANSPORT\020\003\022\016\n\nLABORATORY\020\004\022\021\n\rMANUFA" +
"CTURING\020\005\022\n\n\006RETAIL\020\006\022\014\n\010DELIVERY\020\007\022\n\n\006E" +
"VENTS\020\010\022\017\n\013CONSUMPTION\020\t*a\n\rLicenseStatu" +
"s\022\013\n\007PENDING\020\000\022\n\n\006ACTIVE\020\001\022\013\n\007REVOKED\020\002\022" +
"\013\n\007EXPIRED\020\003\022\r\n\tSUSPENDED\020\004\022\016\n\nSUPERSEDE" +
"D\020\005B3\n\034io.bloombox.schema.licensureB\tLic" +
"ensureH\001P\000\242\002\003BBSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.opencannabis.schema.geo.usa.USStateOuterClass.getDescriptor(),
io.opencannabis.schema.geo.usa.California.getDescriptor(),
io.opencannabis.schema.temporal.TemporalDate.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
io.opencannabis.schema.regulatory.usa.California.getDescriptor(),
}, assigner);
internal_static_bloombox_licensure_LicensingAuthority_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_licensure_LicensingAuthority_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicensingAuthority_descriptor,
new java.lang.String[] { "Local", "County", "State", "Jurisdiction", });
internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor =
internal_static_bloombox_licensure_LicensingAuthority_descriptor.getNestedTypes().get(0);
internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicensingAuthority_LocalLicense_descriptor,
new java.lang.String[] { "Municipality", "CountyName", "CaliforniaCounty", "ProvinceName", "UsState", "County", "Province", });
internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor =
internal_static_bloombox_licensure_LicensingAuthority_descriptor.getNestedTypes().get(1);
internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicensingAuthority_CountyLicense_descriptor,
new java.lang.String[] { "Name", "CaliforniaCounty", "ProvinceName", "UsState", "County", "Province", });
internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor =
internal_static_bloombox_licensure_LicensingAuthority_descriptor.getNestedTypes().get(2);
internal_static_bloombox_licensure_LicensingAuthority_StateLicense_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicensingAuthority_StateLicense_descriptor,
new java.lang.String[] { "ProvinceName", "UsState", "AgencyName", "CaliforniaAgency", "Province", "Agency", });
internal_static_bloombox_licensure_LicenseKey_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_licensure_LicenseKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicenseKey_descriptor,
new java.lang.String[] { "Id", "Jid", });
internal_static_bloombox_licensure_LicenseEvent_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_bloombox_licensure_LicenseEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicenseEvent_descriptor,
new java.lang.String[] { "Status", "Privilege", "Message", "Occurred", });
internal_static_bloombox_licensure_LicenseTimestamps_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_bloombox_licensure_LicenseTimestamps_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_LicenseTimestamps_descriptor,
new java.lang.String[] { "Submitted", "Issued", "Received", "Verified", "Applied", "Modified", });
internal_static_bloombox_licensure_RegulatoryLicense_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_bloombox_licensure_RegulatoryLicense_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_licensure_RegulatoryLicense_descriptor,
new java.lang.String[] { "Key", "Type", "Authority", "Status", "Event", "Privilege", "Timestamps", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.db);
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.opencannabis.schema.geo.usa.USStateOuterClass.getDescriptor();
io.opencannabis.schema.geo.usa.California.getDescriptor();
io.opencannabis.schema.temporal.TemporalDate.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
io.opencannabis.schema.regulatory.usa.California.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy