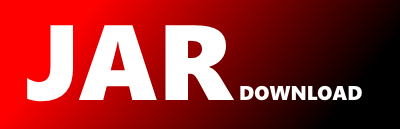
io.bloombox.schema.pass.PassID Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: identity/pass/Pass.proto
package io.bloombox.schema.pass;
public final class PassID {
private PassID() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Enumerates statuses that a digital pass record may be in.
*
*
* Protobuf enum {@code bloombox.identity.pass.PassStatus}
*/
public enum PassStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The pass has been provisioned, but not issued.
*
*
* PROVISIONED = 0;
*/
PROVISIONED(0),
/**
*
* The pass has been issued, meaning it has been sent to the user via some means.
*
*
* ISSUED = 1;
*/
ISSUED(1),
/**
*
* The pass has been activated and is ready for use.
*
*
* ACTIVE = 2;
*/
ACTIVE(2),
/**
*
* The pass has been decommissioned by the user. This status is terminal.
*
*
* DECOMMISSIONED = 3;
*/
DECOMMISSIONED(3),
/**
*
* The pass has been suspended by the partner or location. It is temporarily not usable.
*
*
* SUSPENDED = 4;
*/
SUSPENDED(4),
/**
*
* The pass is fully banned by the partner or location. This status is terminal and the pass is no longer usable.
*
*
* BANNED = 5;
*/
BANNED(5),
UNRECOGNIZED(-1),
;
/**
*
* The pass has been provisioned, but not issued.
*
*
* PROVISIONED = 0;
*/
public static final int PROVISIONED_VALUE = 0;
/**
*
* The pass has been issued, meaning it has been sent to the user via some means.
*
*
* ISSUED = 1;
*/
public static final int ISSUED_VALUE = 1;
/**
*
* The pass has been activated and is ready for use.
*
*
* ACTIVE = 2;
*/
public static final int ACTIVE_VALUE = 2;
/**
*
* The pass has been decommissioned by the user. This status is terminal.
*
*
* DECOMMISSIONED = 3;
*/
public static final int DECOMMISSIONED_VALUE = 3;
/**
*
* The pass has been suspended by the partner or location. It is temporarily not usable.
*
*
* SUSPENDED = 4;
*/
public static final int SUSPENDED_VALUE = 4;
/**
*
* The pass is fully banned by the partner or location. This status is terminal and the pass is no longer usable.
*
*
* BANNED = 5;
*/
public static final int BANNED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PassStatus valueOf(int value) {
return forNumber(value);
}
public static PassStatus forNumber(int value) {
switch (value) {
case 0: return PROVISIONED;
case 1: return ISSUED;
case 2: return ACTIVE;
case 3: return DECOMMISSIONED;
case 4: return SUSPENDED;
case 5: return BANNED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PassStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PassStatus findValueByNumber(int number) {
return PassStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.pass.PassID.getDescriptor().getEnumTypes().get(0);
}
private static final PassStatus[] VALUES = values();
public static PassStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PassStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.identity.pass.PassStatus)
}
public interface PassOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.identity.pass.Pass)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
boolean hasKey();
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.pass.PassIDKey.PassKey getKey();
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder getKeyOrBuilder();
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
java.lang.String getToken();
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
com.google.protobuf.ByteString
getTokenBytes();
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
java.lang.String getUri();
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
com.google.protobuf.ByteString
getUriBytes();
/**
*
* Specifies whether this pass is active.
*
*
* bool active = 4;
*/
boolean getActive();
/**
*
* Whether this pass is personalizable.
*
*
* bool personalizable = 5;
*/
boolean getPersonalizable();
/**
*
* Whether this pass is personalizable, and has been personalized.
*
*
* bool personalized = 6;
*/
boolean getPersonalized();
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
int getStatusValue();
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
io.bloombox.schema.pass.PassID.PassStatus getStatus();
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
boolean hasProvisioned();
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getProvisioned();
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getProvisionedOrBuilder();
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
boolean hasIssued();
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued();
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder();
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
boolean hasActivated();
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getActivated();
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getActivatedOrBuilder();
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
boolean hasSuspended();
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSuspended();
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSuspendedOrBuilder();
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
boolean hasBanned();
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getBanned();
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getBannedOrBuilder();
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
boolean hasSeen();
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
boolean hasCheckin();
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getCheckin();
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCheckinOrBuilder();
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
boolean hasEnroll();
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getEnroll();
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEnrollOrBuilder();
}
/**
*
* Specifies the structure of a digital pass record.
*
*
* Protobuf type {@code bloombox.identity.pass.Pass}
*/
public static final class Pass extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.identity.pass.Pass)
PassOrBuilder {
private static final long serialVersionUID = 0L;
// Use Pass.newBuilder() to construct.
private Pass(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Pass() {
token_ = "";
uri_ = "";
status_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Pass(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.bloombox.schema.pass.PassIDKey.PassKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.bloombox.schema.pass.PassIDKey.PassKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
token_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
uri_ = s;
break;
}
case 32: {
active_ = input.readBool();
break;
}
case 40: {
personalizable_ = input.readBool();
break;
}
case 48: {
personalized_ = input.readBool();
break;
}
case 56: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 66: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (provisioned_ != null) {
subBuilder = provisioned_.toBuilder();
}
provisioned_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(provisioned_);
provisioned_ = subBuilder.buildPartial();
}
break;
}
case 74: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (issued_ != null) {
subBuilder = issued_.toBuilder();
}
issued_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(issued_);
issued_ = subBuilder.buildPartial();
}
break;
}
case 82: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (activated_ != null) {
subBuilder = activated_.toBuilder();
}
activated_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(activated_);
activated_ = subBuilder.buildPartial();
}
break;
}
case 90: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (suspended_ != null) {
subBuilder = suspended_.toBuilder();
}
suspended_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(suspended_);
suspended_ = subBuilder.buildPartial();
}
break;
}
case 98: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (banned_ != null) {
subBuilder = banned_.toBuilder();
}
banned_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(banned_);
banned_ = subBuilder.buildPartial();
}
break;
}
case 106: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
case 114: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (checkin_ != null) {
subBuilder = checkin_.toBuilder();
}
checkin_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(checkin_);
checkin_ = subBuilder.buildPartial();
}
break;
}
case 122: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (enroll_ != null) {
subBuilder = enroll_.toBuilder();
}
enroll_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(enroll_);
enroll_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.pass.PassID.internal_static_bloombox_identity_pass_Pass_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.pass.PassID.internal_static_bloombox_identity_pass_Pass_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.pass.PassID.Pass.class, io.bloombox.schema.pass.PassID.Pass.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private io.bloombox.schema.pass.PassIDKey.PassKey key_;
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.pass.PassIDKey.PassKey getKey() {
return key_ == null ? io.bloombox.schema.pass.PassIDKey.PassKey.getDefaultInstance() : key_;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int TOKEN_FIELD_NUMBER = 2;
private volatile java.lang.Object token_;
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
}
}
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int URI_FIELD_NUMBER = 3;
private volatile java.lang.Object uri_;
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uri_ = s;
return s;
}
}
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public com.google.protobuf.ByteString
getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACTIVE_FIELD_NUMBER = 4;
private boolean active_;
/**
*
* Specifies whether this pass is active.
*
*
* bool active = 4;
*/
public boolean getActive() {
return active_;
}
public static final int PERSONALIZABLE_FIELD_NUMBER = 5;
private boolean personalizable_;
/**
*
* Whether this pass is personalizable.
*
*
* bool personalizable = 5;
*/
public boolean getPersonalizable() {
return personalizable_;
}
public static final int PERSONALIZED_FIELD_NUMBER = 6;
private boolean personalized_;
/**
*
* Whether this pass is personalizable, and has been personalized.
*
*
* bool personalized = 6;
*/
public boolean getPersonalized() {
return personalized_;
}
public static final int STATUS_FIELD_NUMBER = 7;
private int status_;
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public io.bloombox.schema.pass.PassID.PassStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.pass.PassID.PassStatus result = io.bloombox.schema.pass.PassID.PassStatus.valueOf(status_);
return result == null ? io.bloombox.schema.pass.PassID.PassStatus.UNRECOGNIZED : result;
}
public static final int PROVISIONED_FIELD_NUMBER = 8;
private io.opencannabis.schema.temporal.TemporalInstant.Instant provisioned_;
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public boolean hasProvisioned() {
return provisioned_ != null;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getProvisioned() {
return provisioned_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : provisioned_;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getProvisionedOrBuilder() {
return getProvisioned();
}
public static final int ISSUED_FIELD_NUMBER = 9;
private io.opencannabis.schema.temporal.TemporalInstant.Instant issued_;
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public boolean hasIssued() {
return issued_ != null;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued() {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder() {
return getIssued();
}
public static final int ACTIVATED_FIELD_NUMBER = 10;
private io.opencannabis.schema.temporal.TemporalInstant.Instant activated_;
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public boolean hasActivated() {
return activated_ != null;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getActivated() {
return activated_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : activated_;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getActivatedOrBuilder() {
return getActivated();
}
public static final int SUSPENDED_FIELD_NUMBER = 11;
private io.opencannabis.schema.temporal.TemporalInstant.Instant suspended_;
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public boolean hasSuspended() {
return suspended_ != null;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSuspended() {
return suspended_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : suspended_;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSuspendedOrBuilder() {
return getSuspended();
}
public static final int BANNED_FIELD_NUMBER = 12;
private io.opencannabis.schema.temporal.TemporalInstant.Instant banned_;
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public boolean hasBanned() {
return banned_ != null;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getBanned() {
return banned_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : banned_;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getBannedOrBuilder() {
return getBanned();
}
public static final int SEEN_FIELD_NUMBER = 13;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
public static final int CHECKIN_FIELD_NUMBER = 14;
private io.opencannabis.schema.temporal.TemporalInstant.Instant checkin_;
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public boolean hasCheckin() {
return checkin_ != null;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCheckin() {
return checkin_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : checkin_;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCheckinOrBuilder() {
return getCheckin();
}
public static final int ENROLL_FIELD_NUMBER = 15;
private io.opencannabis.schema.temporal.TemporalInstant.Instant enroll_;
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public boolean hasEnroll() {
return enroll_ != null;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEnroll() {
return enroll_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : enroll_;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEnrollOrBuilder() {
return getEnroll();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (!getTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, token_);
}
if (!getUriBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, uri_);
}
if (active_ != false) {
output.writeBool(4, active_);
}
if (personalizable_ != false) {
output.writeBool(5, personalizable_);
}
if (personalized_ != false) {
output.writeBool(6, personalized_);
}
if (status_ != io.bloombox.schema.pass.PassID.PassStatus.PROVISIONED.getNumber()) {
output.writeEnum(7, status_);
}
if (provisioned_ != null) {
output.writeMessage(8, getProvisioned());
}
if (issued_ != null) {
output.writeMessage(9, getIssued());
}
if (activated_ != null) {
output.writeMessage(10, getActivated());
}
if (suspended_ != null) {
output.writeMessage(11, getSuspended());
}
if (banned_ != null) {
output.writeMessage(12, getBanned());
}
if (seen_ != null) {
output.writeMessage(13, getSeen());
}
if (checkin_ != null) {
output.writeMessage(14, getCheckin());
}
if (enroll_ != null) {
output.writeMessage(15, getEnroll());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (!getTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, token_);
}
if (!getUriBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, uri_);
}
if (active_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, active_);
}
if (personalizable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, personalizable_);
}
if (personalized_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, personalized_);
}
if (status_ != io.bloombox.schema.pass.PassID.PassStatus.PROVISIONED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, status_);
}
if (provisioned_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getProvisioned());
}
if (issued_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getIssued());
}
if (activated_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getActivated());
}
if (suspended_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getSuspended());
}
if (banned_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getBanned());
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getSeen());
}
if (checkin_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getCheckin());
}
if (enroll_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getEnroll());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.pass.PassID.Pass)) {
return super.equals(obj);
}
io.bloombox.schema.pass.PassID.Pass other = (io.bloombox.schema.pass.PassID.Pass) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (!getToken()
.equals(other.getToken())) return false;
if (!getUri()
.equals(other.getUri())) return false;
if (getActive()
!= other.getActive()) return false;
if (getPersonalizable()
!= other.getPersonalizable()) return false;
if (getPersonalized()
!= other.getPersonalized()) return false;
if (status_ != other.status_) return false;
if (hasProvisioned() != other.hasProvisioned()) return false;
if (hasProvisioned()) {
if (!getProvisioned()
.equals(other.getProvisioned())) return false;
}
if (hasIssued() != other.hasIssued()) return false;
if (hasIssued()) {
if (!getIssued()
.equals(other.getIssued())) return false;
}
if (hasActivated() != other.hasActivated()) return false;
if (hasActivated()) {
if (!getActivated()
.equals(other.getActivated())) return false;
}
if (hasSuspended() != other.hasSuspended()) return false;
if (hasSuspended()) {
if (!getSuspended()
.equals(other.getSuspended())) return false;
}
if (hasBanned() != other.hasBanned()) return false;
if (hasBanned()) {
if (!getBanned()
.equals(other.getBanned())) return false;
}
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (hasCheckin() != other.hasCheckin()) return false;
if (hasCheckin()) {
if (!getCheckin()
.equals(other.getCheckin())) return false;
}
if (hasEnroll() != other.hasEnroll()) return false;
if (hasEnroll()) {
if (!getEnroll()
.equals(other.getEnroll())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
hash = (37 * hash) + URI_FIELD_NUMBER;
hash = (53 * hash) + getUri().hashCode();
hash = (37 * hash) + ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getActive());
hash = (37 * hash) + PERSONALIZABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPersonalizable());
hash = (37 * hash) + PERSONALIZED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPersonalized());
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasProvisioned()) {
hash = (37 * hash) + PROVISIONED_FIELD_NUMBER;
hash = (53 * hash) + getProvisioned().hashCode();
}
if (hasIssued()) {
hash = (37 * hash) + ISSUED_FIELD_NUMBER;
hash = (53 * hash) + getIssued().hashCode();
}
if (hasActivated()) {
hash = (37 * hash) + ACTIVATED_FIELD_NUMBER;
hash = (53 * hash) + getActivated().hashCode();
}
if (hasSuspended()) {
hash = (37 * hash) + SUSPENDED_FIELD_NUMBER;
hash = (53 * hash) + getSuspended().hashCode();
}
if (hasBanned()) {
hash = (37 * hash) + BANNED_FIELD_NUMBER;
hash = (53 * hash) + getBanned().hashCode();
}
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
if (hasCheckin()) {
hash = (37 * hash) + CHECKIN_FIELD_NUMBER;
hash = (53 * hash) + getCheckin().hashCode();
}
if (hasEnroll()) {
hash = (37 * hash) + ENROLL_FIELD_NUMBER;
hash = (53 * hash) + getEnroll().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.pass.PassID.Pass parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.pass.PassID.Pass parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.pass.PassID.Pass parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.pass.PassID.Pass prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of a digital pass record.
*
*
* Protobuf type {@code bloombox.identity.pass.Pass}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.identity.pass.Pass)
io.bloombox.schema.pass.PassID.PassOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.pass.PassID.internal_static_bloombox_identity_pass_Pass_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.pass.PassID.internal_static_bloombox_identity_pass_Pass_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.pass.PassID.Pass.class, io.bloombox.schema.pass.PassID.Pass.Builder.class);
}
// Construct using io.bloombox.schema.pass.PassID.Pass.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
token_ = "";
uri_ = "";
active_ = false;
personalizable_ = false;
personalized_ = false;
status_ = 0;
if (provisionedBuilder_ == null) {
provisioned_ = null;
} else {
provisioned_ = null;
provisionedBuilder_ = null;
}
if (issuedBuilder_ == null) {
issued_ = null;
} else {
issued_ = null;
issuedBuilder_ = null;
}
if (activatedBuilder_ == null) {
activated_ = null;
} else {
activated_ = null;
activatedBuilder_ = null;
}
if (suspendedBuilder_ == null) {
suspended_ = null;
} else {
suspended_ = null;
suspendedBuilder_ = null;
}
if (bannedBuilder_ == null) {
banned_ = null;
} else {
banned_ = null;
bannedBuilder_ = null;
}
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
if (checkinBuilder_ == null) {
checkin_ = null;
} else {
checkin_ = null;
checkinBuilder_ = null;
}
if (enrollBuilder_ == null) {
enroll_ = null;
} else {
enroll_ = null;
enrollBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.pass.PassID.internal_static_bloombox_identity_pass_Pass_descriptor;
}
@java.lang.Override
public io.bloombox.schema.pass.PassID.Pass getDefaultInstanceForType() {
return io.bloombox.schema.pass.PassID.Pass.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.pass.PassID.Pass build() {
io.bloombox.schema.pass.PassID.Pass result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.pass.PassID.Pass buildPartial() {
io.bloombox.schema.pass.PassID.Pass result = new io.bloombox.schema.pass.PassID.Pass(this);
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
result.token_ = token_;
result.uri_ = uri_;
result.active_ = active_;
result.personalizable_ = personalizable_;
result.personalized_ = personalized_;
result.status_ = status_;
if (provisionedBuilder_ == null) {
result.provisioned_ = provisioned_;
} else {
result.provisioned_ = provisionedBuilder_.build();
}
if (issuedBuilder_ == null) {
result.issued_ = issued_;
} else {
result.issued_ = issuedBuilder_.build();
}
if (activatedBuilder_ == null) {
result.activated_ = activated_;
} else {
result.activated_ = activatedBuilder_.build();
}
if (suspendedBuilder_ == null) {
result.suspended_ = suspended_;
} else {
result.suspended_ = suspendedBuilder_.build();
}
if (bannedBuilder_ == null) {
result.banned_ = banned_;
} else {
result.banned_ = bannedBuilder_.build();
}
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
if (checkinBuilder_ == null) {
result.checkin_ = checkin_;
} else {
result.checkin_ = checkinBuilder_.build();
}
if (enrollBuilder_ == null) {
result.enroll_ = enroll_;
} else {
result.enroll_ = enrollBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.pass.PassID.Pass) {
return mergeFrom((io.bloombox.schema.pass.PassID.Pass)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.pass.PassID.Pass other) {
if (other == io.bloombox.schema.pass.PassID.Pass.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (!other.getToken().isEmpty()) {
token_ = other.token_;
onChanged();
}
if (!other.getUri().isEmpty()) {
uri_ = other.uri_;
onChanged();
}
if (other.getActive() != false) {
setActive(other.getActive());
}
if (other.getPersonalizable() != false) {
setPersonalizable(other.getPersonalizable());
}
if (other.getPersonalized() != false) {
setPersonalized(other.getPersonalized());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasProvisioned()) {
mergeProvisioned(other.getProvisioned());
}
if (other.hasIssued()) {
mergeIssued(other.getIssued());
}
if (other.hasActivated()) {
mergeActivated(other.getActivated());
}
if (other.hasSuspended()) {
mergeSuspended(other.getSuspended());
}
if (other.hasBanned()) {
mergeBanned(other.getBanned());
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
if (other.hasCheckin()) {
mergeCheckin(other.getCheckin());
}
if (other.hasEnroll()) {
mergeEnroll(other.getEnroll());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.pass.PassID.Pass parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.pass.PassID.Pass) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.bloombox.schema.pass.PassIDKey.PassKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.pass.PassIDKey.PassKey, io.bloombox.schema.pass.PassIDKey.PassKey.Builder, io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder> keyBuilder_;
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.pass.PassIDKey.PassKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.bloombox.schema.pass.PassIDKey.PassKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(io.bloombox.schema.pass.PassIDKey.PassKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(
io.bloombox.schema.pass.PassIDKey.PassKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public Builder mergeKey(io.bloombox.schema.pass.PassIDKey.PassKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.bloombox.schema.pass.PassIDKey.PassKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.pass.PassIDKey.PassKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
public io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.bloombox.schema.pass.PassIDKey.PassKey.getDefaultInstance() : key_;
}
}
/**
*
* Specifies the key for a digital pass record.
*
*
* .bloombox.identity.pass.PassKey key = 1 [(.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.pass.PassIDKey.PassKey, io.bloombox.schema.pass.PassIDKey.PassKey.Builder, io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.pass.PassIDKey.PassKey, io.bloombox.schema.pass.PassIDKey.PassKey.Builder, io.bloombox.schema.pass.PassIDKey.PassKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private java.lang.Object token_ = "";
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public Builder setToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
return this;
}
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public Builder clearToken() {
token_ = getDefaultInstance().getToken();
onChanged();
return this;
}
/**
*
* Specifies the auth token value for a given digital pass.
*
*
* string token = 2;
*/
public Builder setTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
token_ = value;
onChanged();
return this;
}
private java.lang.Object uri_ = "";
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public com.google.protobuf.ByteString
getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public Builder setUri(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uri_ = value;
onChanged();
return this;
}
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public Builder clearUri() {
uri_ = getDefaultInstance().getUri();
onChanged();
return this;
}
/**
*
* Specifes a URI where the subject digital pass may be downloaded.
*
*
* string uri = 3;
*/
public Builder setUriBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uri_ = value;
onChanged();
return this;
}
private boolean active_ ;
/**
*
* Specifies whether this pass is active.
*
*
* bool active = 4;
*/
public boolean getActive() {
return active_;
}
/**
*
* Specifies whether this pass is active.
*
*
* bool active = 4;
*/
public Builder setActive(boolean value) {
active_ = value;
onChanged();
return this;
}
/**
*
* Specifies whether this pass is active.
*
*
* bool active = 4;
*/
public Builder clearActive() {
active_ = false;
onChanged();
return this;
}
private boolean personalizable_ ;
/**
*
* Whether this pass is personalizable.
*
*
* bool personalizable = 5;
*/
public boolean getPersonalizable() {
return personalizable_;
}
/**
*
* Whether this pass is personalizable.
*
*
* bool personalizable = 5;
*/
public Builder setPersonalizable(boolean value) {
personalizable_ = value;
onChanged();
return this;
}
/**
*
* Whether this pass is personalizable.
*
*
* bool personalizable = 5;
*/
public Builder clearPersonalizable() {
personalizable_ = false;
onChanged();
return this;
}
private boolean personalized_ ;
/**
*
* Whether this pass is personalizable, and has been personalized.
*
*
* bool personalized = 6;
*/
public boolean getPersonalized() {
return personalized_;
}
/**
*
* Whether this pass is personalizable, and has been personalized.
*
*
* bool personalized = 6;
*/
public Builder setPersonalized(boolean value) {
personalized_ = value;
onChanged();
return this;
}
/**
*
* Whether this pass is personalizable, and has been personalized.
*
*
* bool personalized = 6;
*/
public Builder clearPersonalized() {
personalized_ = false;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public io.bloombox.schema.pass.PassID.PassStatus getStatus() {
@SuppressWarnings("deprecation")
io.bloombox.schema.pass.PassID.PassStatus result = io.bloombox.schema.pass.PassID.PassStatus.valueOf(status_);
return result == null ? io.bloombox.schema.pass.PassID.PassStatus.UNRECOGNIZED : result;
}
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public Builder setStatus(io.bloombox.schema.pass.PassID.PassStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Current status of this pass.
*
*
* .bloombox.identity.pass.PassStatus status = 7;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant provisioned_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> provisionedBuilder_;
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public boolean hasProvisioned() {
return provisionedBuilder_ != null || provisioned_ != null;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getProvisioned() {
if (provisionedBuilder_ == null) {
return provisioned_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : provisioned_;
} else {
return provisionedBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public Builder setProvisioned(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (provisionedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
provisioned_ = value;
onChanged();
} else {
provisionedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public Builder setProvisioned(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (provisionedBuilder_ == null) {
provisioned_ = builderForValue.build();
onChanged();
} else {
provisionedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public Builder mergeProvisioned(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (provisionedBuilder_ == null) {
if (provisioned_ != null) {
provisioned_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(provisioned_).mergeFrom(value).buildPartial();
} else {
provisioned_ = value;
}
onChanged();
} else {
provisionedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public Builder clearProvisioned() {
if (provisionedBuilder_ == null) {
provisioned_ = null;
onChanged();
} else {
provisioned_ = null;
provisionedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getProvisionedBuilder() {
onChanged();
return getProvisionedFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getProvisionedOrBuilder() {
if (provisionedBuilder_ != null) {
return provisionedBuilder_.getMessageOrBuilder();
} else {
return provisioned_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : provisioned_;
}
}
/**
*
* Timestamp indicating when this pass was originally provisioned.
*
*
* .opencannabis.temporal.Instant provisioned = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getProvisionedFieldBuilder() {
if (provisionedBuilder_ == null) {
provisionedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getProvisioned(),
getParentForChildren(),
isClean());
provisioned_ = null;
}
return provisionedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant issued_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> issuedBuilder_;
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public boolean hasIssued() {
return issuedBuilder_ != null || issued_ != null;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getIssued() {
if (issuedBuilder_ == null) {
return issued_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
} else {
return issuedBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public Builder setIssued(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (issuedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
issued_ = value;
onChanged();
} else {
issuedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public Builder setIssued(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (issuedBuilder_ == null) {
issued_ = builderForValue.build();
onChanged();
} else {
issuedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public Builder mergeIssued(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (issuedBuilder_ == null) {
if (issued_ != null) {
issued_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(issued_).mergeFrom(value).buildPartial();
} else {
issued_ = value;
}
onChanged();
} else {
issuedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public Builder clearIssued() {
if (issuedBuilder_ == null) {
issued_ = null;
onChanged();
} else {
issued_ = null;
issuedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getIssuedBuilder() {
onChanged();
return getIssuedFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getIssuedOrBuilder() {
if (issuedBuilder_ != null) {
return issuedBuilder_.getMessageOrBuilder();
} else {
return issued_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : issued_;
}
}
/**
*
* Timestamp indicating when this pass was originally issued.
*
*
* .opencannabis.temporal.Instant issued = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getIssuedFieldBuilder() {
if (issuedBuilder_ == null) {
issuedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getIssued(),
getParentForChildren(),
isClean());
issued_ = null;
}
return issuedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant activated_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> activatedBuilder_;
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public boolean hasActivated() {
return activatedBuilder_ != null || activated_ != null;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getActivated() {
if (activatedBuilder_ == null) {
return activated_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : activated_;
} else {
return activatedBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public Builder setActivated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (activatedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
activated_ = value;
onChanged();
} else {
activatedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public Builder setActivated(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (activatedBuilder_ == null) {
activated_ = builderForValue.build();
onChanged();
} else {
activatedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public Builder mergeActivated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (activatedBuilder_ == null) {
if (activated_ != null) {
activated_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(activated_).mergeFrom(value).buildPartial();
} else {
activated_ = value;
}
onChanged();
} else {
activatedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public Builder clearActivated() {
if (activatedBuilder_ == null) {
activated_ = null;
onChanged();
} else {
activated_ = null;
activatedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getActivatedBuilder() {
onChanged();
return getActivatedFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getActivatedOrBuilder() {
if (activatedBuilder_ != null) {
return activatedBuilder_.getMessageOrBuilder();
} else {
return activated_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : activated_;
}
}
/**
*
* Timestamp indicating when this pass was activated for use.
*
*
* .opencannabis.temporal.Instant activated = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getActivatedFieldBuilder() {
if (activatedBuilder_ == null) {
activatedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getActivated(),
getParentForChildren(),
isClean());
activated_ = null;
}
return activatedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant suspended_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> suspendedBuilder_;
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public boolean hasSuspended() {
return suspendedBuilder_ != null || suspended_ != null;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSuspended() {
if (suspendedBuilder_ == null) {
return suspended_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : suspended_;
} else {
return suspendedBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public Builder setSuspended(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (suspendedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
suspended_ = value;
onChanged();
} else {
suspendedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public Builder setSuspended(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (suspendedBuilder_ == null) {
suspended_ = builderForValue.build();
onChanged();
} else {
suspendedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public Builder mergeSuspended(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (suspendedBuilder_ == null) {
if (suspended_ != null) {
suspended_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(suspended_).mergeFrom(value).buildPartial();
} else {
suspended_ = value;
}
onChanged();
} else {
suspendedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public Builder clearSuspended() {
if (suspendedBuilder_ == null) {
suspended_ = null;
onChanged();
} else {
suspended_ = null;
suspendedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSuspendedBuilder() {
onChanged();
return getSuspendedFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSuspendedOrBuilder() {
if (suspendedBuilder_ != null) {
return suspendedBuilder_.getMessageOrBuilder();
} else {
return suspended_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : suspended_;
}
}
/**
*
* Timestamp indicating when this pass was suspended, if applicable.
*
*
* .opencannabis.temporal.Instant suspended = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSuspendedFieldBuilder() {
if (suspendedBuilder_ == null) {
suspendedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSuspended(),
getParentForChildren(),
isClean());
suspended_ = null;
}
return suspendedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant banned_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> bannedBuilder_;
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public boolean hasBanned() {
return bannedBuilder_ != null || banned_ != null;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getBanned() {
if (bannedBuilder_ == null) {
return banned_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : banned_;
} else {
return bannedBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public Builder setBanned(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (bannedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
banned_ = value;
onChanged();
} else {
bannedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public Builder setBanned(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (bannedBuilder_ == null) {
banned_ = builderForValue.build();
onChanged();
} else {
bannedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public Builder mergeBanned(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (bannedBuilder_ == null) {
if (banned_ != null) {
banned_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(banned_).mergeFrom(value).buildPartial();
} else {
banned_ = value;
}
onChanged();
} else {
bannedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public Builder clearBanned() {
if (bannedBuilder_ == null) {
banned_ = null;
onChanged();
} else {
banned_ = null;
bannedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getBannedBuilder() {
onChanged();
return getBannedFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getBannedOrBuilder() {
if (bannedBuilder_ != null) {
return bannedBuilder_.getMessageOrBuilder();
} else {
return banned_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : banned_;
}
}
/**
*
* Timestamp indicating when this pass was banned, if applicable.
*
*
* .opencannabis.temporal.Instant banned = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getBannedFieldBuilder() {
if (bannedBuilder_ == null) {
bannedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getBanned(),
getParentForChildren(),
isClean());
banned_ = null;
}
return bannedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Timestamp indicating when this pass was last seen.
*
*
* .opencannabis.temporal.Instant seen = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant checkin_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> checkinBuilder_;
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public boolean hasCheckin() {
return checkinBuilder_ != null || checkin_ != null;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCheckin() {
if (checkinBuilder_ == null) {
return checkin_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : checkin_;
} else {
return checkinBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public Builder setCheckin(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (checkinBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
checkin_ = value;
onChanged();
} else {
checkinBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public Builder setCheckin(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (checkinBuilder_ == null) {
checkin_ = builderForValue.build();
onChanged();
} else {
checkinBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public Builder mergeCheckin(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (checkinBuilder_ == null) {
if (checkin_ != null) {
checkin_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(checkin_).mergeFrom(value).buildPartial();
} else {
checkin_ = value;
}
onChanged();
} else {
checkinBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public Builder clearCheckin() {
if (checkinBuilder_ == null) {
checkin_ = null;
onChanged();
} else {
checkin_ = null;
checkinBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getCheckinBuilder() {
onChanged();
return getCheckinFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCheckinOrBuilder() {
if (checkinBuilder_ != null) {
return checkinBuilder_.getMessageOrBuilder();
} else {
return checkin_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : checkin_;
}
}
/**
*
* Timestamp indicating when this pass was last used to check-in, if applicable.
*
*
* .opencannabis.temporal.Instant checkin = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getCheckinFieldBuilder() {
if (checkinBuilder_ == null) {
checkinBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getCheckin(),
getParentForChildren(),
isClean());
checkin_ = null;
}
return checkinBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant enroll_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> enrollBuilder_;
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public boolean hasEnroll() {
return enrollBuilder_ != null || enroll_ != null;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEnroll() {
if (enrollBuilder_ == null) {
return enroll_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : enroll_;
} else {
return enrollBuilder_.getMessage();
}
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public Builder setEnroll(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (enrollBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enroll_ = value;
onChanged();
} else {
enrollBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public Builder setEnroll(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (enrollBuilder_ == null) {
enroll_ = builderForValue.build();
onChanged();
} else {
enrollBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public Builder mergeEnroll(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (enrollBuilder_ == null) {
if (enroll_ != null) {
enroll_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(enroll_).mergeFrom(value).buildPartial();
} else {
enroll_ = value;
}
onChanged();
} else {
enrollBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public Builder clearEnroll() {
if (enrollBuilder_ == null) {
enroll_ = null;
onChanged();
} else {
enroll_ = null;
enrollBuilder_ = null;
}
return this;
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getEnrollBuilder() {
onChanged();
return getEnrollFieldBuilder().getBuilder();
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEnrollOrBuilder() {
if (enrollBuilder_ != null) {
return enrollBuilder_.getMessageOrBuilder();
} else {
return enroll_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : enroll_;
}
}
/**
*
* Timestamp indicating when this pass was enrolled, if it's a personalizable pass.
*
*
* .opencannabis.temporal.Instant enroll = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getEnrollFieldBuilder() {
if (enrollBuilder_ == null) {
enrollBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getEnroll(),
getParentForChildren(),
isClean());
enroll_ = null;
}
return enrollBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.identity.pass.Pass)
}
// @@protoc_insertion_point(class_scope:bloombox.identity.pass.Pass)
private static final io.bloombox.schema.pass.PassID.Pass DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.pass.PassID.Pass();
}
public static io.bloombox.schema.pass.PassID.Pass getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Pass parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Pass(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.pass.PassID.Pass getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_identity_pass_Pass_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_identity_pass_Pass_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\030identity/pass/Pass.proto\022\026bloombox.ide" +
"ntity.pass\032\024core/Datamodel.proto\032\033identi" +
"ty/pass/PassKey.proto\032\026temporal/Instant." +
"proto\"\324\004\n\004Pass\0224\n\003key\030\001 \001(\0132\037.bloombox.i" +
"dentity.pass.PassKeyB\006\302\265\003\002\010\001\022\r\n\005token\030\002 " +
"\001(\t\022\013\n\003uri\030\003 \001(\t\022\016\n\006active\030\004 \001(\010\022\026\n\016pers" +
"onalizable\030\005 \001(\010\022\024\n\014personalized\030\006 \001(\010\0222" +
"\n\006status\030\007 \001(\0162\".bloombox.identity.pass." +
"PassStatus\0223\n\013provisioned\030\010 \001(\0132\036.openca" +
"nnabis.temporal.Instant\022.\n\006issued\030\t \001(\0132" +
"\036.opencannabis.temporal.Instant\0221\n\tactiv" +
"ated\030\n \001(\0132\036.opencannabis.temporal.Insta" +
"nt\0221\n\tsuspended\030\013 \001(\0132\036.opencannabis.tem" +
"poral.Instant\022.\n\006banned\030\014 \001(\0132\036.opencann" +
"abis.temporal.Instant\022,\n\004seen\030\r \001(\0132\036.op" +
"encannabis.temporal.Instant\022/\n\007checkin\030\016" +
" \001(\0132\036.opencannabis.temporal.Instant\022.\n\006" +
"enroll\030\017 \001(\0132\036.opencannabis.temporal.Ins" +
"tant*d\n\nPassStatus\022\017\n\013PROVISIONED\020\000\022\n\n\006I" +
"SSUED\020\001\022\n\n\006ACTIVE\020\002\022\022\n\016DECOMMISSIONED\020\003\022" +
"\r\n\tSUSPENDED\020\004\022\n\n\006BANNED\020\005B+\n\027io.bloombo" +
"x.schema.passB\006PassIDH\001P\000\242\002\003BBSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.bloombox.schema.pass.PassIDKey.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
}, assigner);
internal_static_bloombox_identity_pass_Pass_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_identity_pass_Pass_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_identity_pass_Pass_descriptor,
new java.lang.String[] { "Key", "Token", "Uri", "Active", "Personalizable", "Personalized", "Status", "Provisioned", "Issued", "Activated", "Suspended", "Banned", "Seen", "Checkin", "Enroll", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.bloombox.schema.pass.PassIDKey.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy