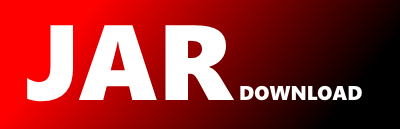
io.bloombox.schema.services.auth.v1beta1.AuthError Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: auth/v1beta1/AuthService_Beta1.proto
package io.bloombox.schema.services.auth.v1beta1;
/**
*
* Enumerates known errors that may be thrown by auth operations.
*
*
* Protobuf enum {@code bloombox.services.auth.v1beta1.AuthError}
*/
public enum AuthError
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* There was no error.
*
*
* NO_ERROR = 0;
*/
NO_ERROR(0),
/**
*
* Access was denied: the user's account is suspended.
*
*
* ACCOUNT_SUSPENDED = 1;
*/
ACCOUNT_SUSPENDED(1),
/**
*
* Profile could not be located.
*
*
* PROFILE_NOT_FOUND = 2;
*/
PROFILE_NOT_FOUND(2),
/**
*
* The provided user key was found to be invalid.
*
*
* INVALID_USER_KEY = 3;
*/
INVALID_USER_KEY(3),
/**
*
* The provided auth assertion was deemed to be invalid.
*
*
* INVALID_ASSERTION = 4;
*/
INVALID_ASSERTION(4),
/**
*
* The specified authentication type is not currently supported.
*
*
* UNSUPPORTED_LOGIN_TYPE = 5;
*/
UNSUPPORTED_LOGIN_TYPE(5),
/**
*
* Authentication token from Hydra was not valid.
*
*
* INVALID_AUTH_TOKEN = 6;
*/
INVALID_AUTH_TOKEN(6),
/**
*
* The ticket provided for consent was invalid.
*
*
* INVALID_TICKET = 7;
*/
INVALID_TICKET(7),
/**
*
* The client's profile could not be located.
*
*
* CLIENT_NOT_FOUND = 8;
*/
CLIENT_NOT_FOUND(8),
/**
*
* Consent ID is not valid.
*
*
* INVALID_CONSENT_ID = 9;
*/
INVALID_CONSENT_ID(9),
/**
*
* The provided ID token information was found to be invalid.
*
*
* INVALID_ID_TOKEN = 10;
*/
INVALID_ID_TOKEN(10),
/**
*
* The provided access token information was found to be invalid.
*
*
* INVALID_ACCESS_TOKEN = 11;
*/
INVALID_ACCESS_TOKEN(11),
/**
*
* The provided ID token was found to be expired.
*
*
* EXPIRED_ID_TOKEN = 12;
*/
EXPIRED_ID_TOKEN(12),
/**
*
* The provided access token was found to be expired.
*
*
* EXPIRED_ACCESS_TOKEN = 13;
*/
EXPIRED_ACCESS_TOKEN(13),
/**
*
* The specified client was invalid.
*
*
* INVALID_CLIENT = 14;
*/
INVALID_CLIENT(14),
/**
*
* The specified origin was invalid.
*
*
* INVALID_ORIGIN = 15;
*/
INVALID_ORIGIN(15),
/**
*
* Access was denied for unspecified reasons.
*
*
* ACCESS_DENIED = 16;
*/
ACCESS_DENIED(16),
/**
*
* The provided session value was invalid.
*
*
* INVALID_SESSION = 17;
*/
INVALID_SESSION(17),
/**
*
* The provided session has expired.
*
*
* EXPIRED_SESSION = 18;
*/
EXPIRED_SESSION(18),
/**
*
* The provided fingerprint value was invalid.
*
*
* INVALID_FINGERPRINT = 19;
*/
INVALID_FINGERPRINT(19),
/**
*
* Captcha verification value was invalid.
*
*
* INVALID_CAPTCHA = 20;
*/
INVALID_CAPTCHA(20),
/**
*
* The provided captcha veriication value was not correct.
*
*
* CAPTCHA_REJECTED = 21;
*/
CAPTCHA_REJECTED(21),
/**
*
* The specified consent ID could not be resolved.
*
*
* CONSENT_NOT_FOUND = 22;
*/
CONSENT_NOT_FOUND(22),
/**
*
* Consent operation or consent record has expired.
*
*
* EXPIRED_CONSENT = 23;
*/
EXPIRED_CONSENT(23),
/**
*
* The specified nonce was missing, invalid, or already used.
*
*
* INVALID_NONCE = 24;
*/
INVALID_NONCE(24),
/**
*
* A signature was required but none was found.
*
*
* SIGNATURE_REQUIRED = 25;
*/
SIGNATURE_REQUIRED(25),
/**
*
* The signature was invalid or could not be loaded/decoded.
*
*
* SIGNATURE_INVALID = 26;
*/
SIGNATURE_INVALID(26),
/**
*
* The signature did not pass verification.
*
*
* SIGNATURE_MISMATCH = 27;
*/
SIGNATURE_MISMATCH(27),
/**
*
* An internal error was encountered.
*
*
* INTERNAL_ERROR = 99;
*/
INTERNAL_ERROR(99),
UNRECOGNIZED(-1),
;
/**
*
* There was no error.
*
*
* NO_ERROR = 0;
*/
public static final int NO_ERROR_VALUE = 0;
/**
*
* Access was denied: the user's account is suspended.
*
*
* ACCOUNT_SUSPENDED = 1;
*/
public static final int ACCOUNT_SUSPENDED_VALUE = 1;
/**
*
* Profile could not be located.
*
*
* PROFILE_NOT_FOUND = 2;
*/
public static final int PROFILE_NOT_FOUND_VALUE = 2;
/**
*
* The provided user key was found to be invalid.
*
*
* INVALID_USER_KEY = 3;
*/
public static final int INVALID_USER_KEY_VALUE = 3;
/**
*
* The provided auth assertion was deemed to be invalid.
*
*
* INVALID_ASSERTION = 4;
*/
public static final int INVALID_ASSERTION_VALUE = 4;
/**
*
* The specified authentication type is not currently supported.
*
*
* UNSUPPORTED_LOGIN_TYPE = 5;
*/
public static final int UNSUPPORTED_LOGIN_TYPE_VALUE = 5;
/**
*
* Authentication token from Hydra was not valid.
*
*
* INVALID_AUTH_TOKEN = 6;
*/
public static final int INVALID_AUTH_TOKEN_VALUE = 6;
/**
*
* The ticket provided for consent was invalid.
*
*
* INVALID_TICKET = 7;
*/
public static final int INVALID_TICKET_VALUE = 7;
/**
*
* The client's profile could not be located.
*
*
* CLIENT_NOT_FOUND = 8;
*/
public static final int CLIENT_NOT_FOUND_VALUE = 8;
/**
*
* Consent ID is not valid.
*
*
* INVALID_CONSENT_ID = 9;
*/
public static final int INVALID_CONSENT_ID_VALUE = 9;
/**
*
* The provided ID token information was found to be invalid.
*
*
* INVALID_ID_TOKEN = 10;
*/
public static final int INVALID_ID_TOKEN_VALUE = 10;
/**
*
* The provided access token information was found to be invalid.
*
*
* INVALID_ACCESS_TOKEN = 11;
*/
public static final int INVALID_ACCESS_TOKEN_VALUE = 11;
/**
*
* The provided ID token was found to be expired.
*
*
* EXPIRED_ID_TOKEN = 12;
*/
public static final int EXPIRED_ID_TOKEN_VALUE = 12;
/**
*
* The provided access token was found to be expired.
*
*
* EXPIRED_ACCESS_TOKEN = 13;
*/
public static final int EXPIRED_ACCESS_TOKEN_VALUE = 13;
/**
*
* The specified client was invalid.
*
*
* INVALID_CLIENT = 14;
*/
public static final int INVALID_CLIENT_VALUE = 14;
/**
*
* The specified origin was invalid.
*
*
* INVALID_ORIGIN = 15;
*/
public static final int INVALID_ORIGIN_VALUE = 15;
/**
*
* Access was denied for unspecified reasons.
*
*
* ACCESS_DENIED = 16;
*/
public static final int ACCESS_DENIED_VALUE = 16;
/**
*
* The provided session value was invalid.
*
*
* INVALID_SESSION = 17;
*/
public static final int INVALID_SESSION_VALUE = 17;
/**
*
* The provided session has expired.
*
*
* EXPIRED_SESSION = 18;
*/
public static final int EXPIRED_SESSION_VALUE = 18;
/**
*
* The provided fingerprint value was invalid.
*
*
* INVALID_FINGERPRINT = 19;
*/
public static final int INVALID_FINGERPRINT_VALUE = 19;
/**
*
* Captcha verification value was invalid.
*
*
* INVALID_CAPTCHA = 20;
*/
public static final int INVALID_CAPTCHA_VALUE = 20;
/**
*
* The provided captcha veriication value was not correct.
*
*
* CAPTCHA_REJECTED = 21;
*/
public static final int CAPTCHA_REJECTED_VALUE = 21;
/**
*
* The specified consent ID could not be resolved.
*
*
* CONSENT_NOT_FOUND = 22;
*/
public static final int CONSENT_NOT_FOUND_VALUE = 22;
/**
*
* Consent operation or consent record has expired.
*
*
* EXPIRED_CONSENT = 23;
*/
public static final int EXPIRED_CONSENT_VALUE = 23;
/**
*
* The specified nonce was missing, invalid, or already used.
*
*
* INVALID_NONCE = 24;
*/
public static final int INVALID_NONCE_VALUE = 24;
/**
*
* A signature was required but none was found.
*
*
* SIGNATURE_REQUIRED = 25;
*/
public static final int SIGNATURE_REQUIRED_VALUE = 25;
/**
*
* The signature was invalid or could not be loaded/decoded.
*
*
* SIGNATURE_INVALID = 26;
*/
public static final int SIGNATURE_INVALID_VALUE = 26;
/**
*
* The signature did not pass verification.
*
*
* SIGNATURE_MISMATCH = 27;
*/
public static final int SIGNATURE_MISMATCH_VALUE = 27;
/**
*
* An internal error was encountered.
*
*
* INTERNAL_ERROR = 99;
*/
public static final int INTERNAL_ERROR_VALUE = 99;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AuthError valueOf(int value) {
return forNumber(value);
}
public static AuthError forNumber(int value) {
switch (value) {
case 0: return NO_ERROR;
case 1: return ACCOUNT_SUSPENDED;
case 2: return PROFILE_NOT_FOUND;
case 3: return INVALID_USER_KEY;
case 4: return INVALID_ASSERTION;
case 5: return UNSUPPORTED_LOGIN_TYPE;
case 6: return INVALID_AUTH_TOKEN;
case 7: return INVALID_TICKET;
case 8: return CLIENT_NOT_FOUND;
case 9: return INVALID_CONSENT_ID;
case 10: return INVALID_ID_TOKEN;
case 11: return INVALID_ACCESS_TOKEN;
case 12: return EXPIRED_ID_TOKEN;
case 13: return EXPIRED_ACCESS_TOKEN;
case 14: return INVALID_CLIENT;
case 15: return INVALID_ORIGIN;
case 16: return ACCESS_DENIED;
case 17: return INVALID_SESSION;
case 18: return EXPIRED_SESSION;
case 19: return INVALID_FINGERPRINT;
case 20: return INVALID_CAPTCHA;
case 21: return CAPTCHA_REJECTED;
case 22: return CONSENT_NOT_FOUND;
case 23: return EXPIRED_CONSENT;
case 24: return INVALID_NONCE;
case 25: return SIGNATURE_REQUIRED;
case 26: return SIGNATURE_INVALID;
case 27: return SIGNATURE_MISMATCH;
case 99: return INTERNAL_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AuthError> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AuthError findValueByNumber(int number) {
return AuthError.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.bloombox.schema.services.auth.v1beta1.AuthServiceBeta1.getDescriptor().getEnumTypes().get(0);
}
private static final AuthError[] VALUES = values();
public static AuthError valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AuthError(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.services.auth.v1beta1.AuthError)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy