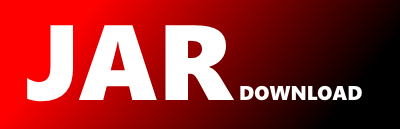
io.bloombox.schema.services.devices.v1beta1.DeviceActivation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas, Co. All rights reserved.
*
* Source and object computer code contained herein is the private intellectual
* property of Momentum Ideas Co., a Delaware Corporation. Use of this
* code in source form requires permission in writing before use or the
* assembly, distribution, or publishing of derivative works, for commercial
* purposes or any other purpose, from a duly authorized officer of Momentum
* Ideas Co.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: devices/v1beta1/DevicesService_Beta1.proto
package io.bloombox.schema.services.devices.v1beta1;
/**
*
* Describes the result of a device activation operation, which includes various pieces of cryptographic information,
* and the device's assignment and role information.
*
*
* Protobuf type {@code bloombox.services.devices.v1beta1.DeviceActivation}
*/
public final class DeviceActivation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.services.devices.v1beta1.DeviceActivation)
DeviceActivationOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeviceActivation.newBuilder() to construct.
private DeviceActivation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeviceActivation() {
uuid_ = "";
hostname_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeviceActivation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
hostname_ = s;
break;
}
case 26: {
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder subBuilder = null;
if (assignment_ != null) {
subBuilder = assignment_.toBuilder();
}
assignment_ = input.readMessage(io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(assignment_);
assignment_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder subBuilder = null;
if (ticket_ != null) {
subBuilder = ticket_.toBuilder();
}
ticket_ = input.readMessage(io.bloombox.schema.security.DeviceSecurity.DeviceTicket.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ticket_);
ticket_ = subBuilder.buildPartial();
}
break;
}
case 42: {
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder subBuilder = null;
if (endpoints_ != null) {
subBuilder = endpoints_.toBuilder();
}
endpoints_ = input.readMessage(io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(endpoints_);
endpoints_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.proximity.BluetoothBeacon.Builder subBuilder = null;
if (beacon_ != null) {
subBuilder = beacon_.toBuilder();
}
beacon_ = input.readMessage(io.opencannabis.schema.proximity.BluetoothBeacon.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(beacon_);
beacon_ = subBuilder.buildPartial();
}
break;
}
case 58: {
io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder subBuilder = null;
if (credentials_ != null) {
subBuilder = credentials_.toBuilder();
}
credentials_ = input.readMessage(io.bloombox.schema.services.devices.v1beta1.DeviceKeys.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(credentials_);
credentials_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.services.devices.v1beta1.DevicesServiceBeta1.internal_static_bloombox_services_devices_v1beta1_DeviceActivation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.services.devices.v1beta1.DevicesServiceBeta1.internal_static_bloombox_services_devices_v1beta1_DeviceActivation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.services.devices.v1beta1.DeviceActivation.class, io.bloombox.schema.services.devices.v1beta1.DeviceActivation.Builder.class);
}
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOSTNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object hostname_;
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostname_ = s;
return s;
}
}
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSIGNMENT_FIELD_NUMBER = 3;
private io.bloombox.schema.services.devices.v1beta1.DeviceAssignment assignment_;
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public boolean hasAssignment() {
return assignment_ != null;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceAssignment getAssignment() {
return assignment_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.getDefaultInstance() : assignment_;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceAssignmentOrBuilder getAssignmentOrBuilder() {
return getAssignment();
}
public static final int TICKET_FIELD_NUMBER = 4;
private io.bloombox.schema.security.DeviceSecurity.DeviceTicket ticket_;
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public boolean hasTicket() {
return ticket_ != null;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public io.bloombox.schema.security.DeviceSecurity.DeviceTicket getTicket() {
return ticket_ == null ? io.bloombox.schema.security.DeviceSecurity.DeviceTicket.getDefaultInstance() : ticket_;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public io.bloombox.schema.security.DeviceSecurity.DeviceTicketOrBuilder getTicketOrBuilder() {
return getTicket();
}
public static final int ENDPOINTS_FIELD_NUMBER = 5;
private io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints endpoints_;
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public boolean hasEndpoints() {
return endpoints_ != null;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints getEndpoints() {
return endpoints_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.getDefaultInstance() : endpoints_;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceEndpointsOrBuilder getEndpointsOrBuilder() {
return getEndpoints();
}
public static final int BEACON_FIELD_NUMBER = 6;
private io.opencannabis.schema.proximity.BluetoothBeacon beacon_;
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public boolean hasBeacon() {
return beacon_ != null;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public io.opencannabis.schema.proximity.BluetoothBeacon getBeacon() {
return beacon_ == null ? io.opencannabis.schema.proximity.BluetoothBeacon.getDefaultInstance() : beacon_;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public io.opencannabis.schema.proximity.BluetoothBeaconOrBuilder getBeaconOrBuilder() {
return getBeacon();
}
public static final int CREDENTIALS_FIELD_NUMBER = 7;
private io.bloombox.schema.services.devices.v1beta1.DeviceKeys credentials_;
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public boolean hasCredentials() {
return credentials_ != null;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceKeys getCredentials() {
return credentials_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceKeys.getDefaultInstance() : credentials_;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceKeysOrBuilder getCredentialsOrBuilder() {
return getCredentials();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
if (!getHostnameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, hostname_);
}
if (assignment_ != null) {
output.writeMessage(3, getAssignment());
}
if (ticket_ != null) {
output.writeMessage(4, getTicket());
}
if (endpoints_ != null) {
output.writeMessage(5, getEndpoints());
}
if (beacon_ != null) {
output.writeMessage(6, getBeacon());
}
if (credentials_ != null) {
output.writeMessage(7, getCredentials());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
if (!getHostnameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, hostname_);
}
if (assignment_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getAssignment());
}
if (ticket_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getTicket());
}
if (endpoints_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getEndpoints());
}
if (beacon_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getBeacon());
}
if (credentials_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCredentials());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.bloombox.schema.services.devices.v1beta1.DeviceActivation)) {
return super.equals(obj);
}
io.bloombox.schema.services.devices.v1beta1.DeviceActivation other = (io.bloombox.schema.services.devices.v1beta1.DeviceActivation) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (!getHostname()
.equals(other.getHostname())) return false;
if (hasAssignment() != other.hasAssignment()) return false;
if (hasAssignment()) {
if (!getAssignment()
.equals(other.getAssignment())) return false;
}
if (hasTicket() != other.hasTicket()) return false;
if (hasTicket()) {
if (!getTicket()
.equals(other.getTicket())) return false;
}
if (hasEndpoints() != other.hasEndpoints()) return false;
if (hasEndpoints()) {
if (!getEndpoints()
.equals(other.getEndpoints())) return false;
}
if (hasBeacon() != other.hasBeacon()) return false;
if (hasBeacon()) {
if (!getBeacon()
.equals(other.getBeacon())) return false;
}
if (hasCredentials() != other.hasCredentials()) return false;
if (hasCredentials()) {
if (!getCredentials()
.equals(other.getCredentials())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (37 * hash) + HOSTNAME_FIELD_NUMBER;
hash = (53 * hash) + getHostname().hashCode();
if (hasAssignment()) {
hash = (37 * hash) + ASSIGNMENT_FIELD_NUMBER;
hash = (53 * hash) + getAssignment().hashCode();
}
if (hasTicket()) {
hash = (37 * hash) + TICKET_FIELD_NUMBER;
hash = (53 * hash) + getTicket().hashCode();
}
if (hasEndpoints()) {
hash = (37 * hash) + ENDPOINTS_FIELD_NUMBER;
hash = (53 * hash) + getEndpoints().hashCode();
}
if (hasBeacon()) {
hash = (37 * hash) + BEACON_FIELD_NUMBER;
hash = (53 * hash) + getBeacon().hashCode();
}
if (hasCredentials()) {
hash = (37 * hash) + CREDENTIALS_FIELD_NUMBER;
hash = (53 * hash) + getCredentials().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.bloombox.schema.services.devices.v1beta1.DeviceActivation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes the result of a device activation operation, which includes various pieces of cryptographic information,
* and the device's assignment and role information.
*
*
* Protobuf type {@code bloombox.services.devices.v1beta1.DeviceActivation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.services.devices.v1beta1.DeviceActivation)
io.bloombox.schema.services.devices.v1beta1.DeviceActivationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.bloombox.schema.services.devices.v1beta1.DevicesServiceBeta1.internal_static_bloombox_services_devices_v1beta1_DeviceActivation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.bloombox.schema.services.devices.v1beta1.DevicesServiceBeta1.internal_static_bloombox_services_devices_v1beta1_DeviceActivation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.bloombox.schema.services.devices.v1beta1.DeviceActivation.class, io.bloombox.schema.services.devices.v1beta1.DeviceActivation.Builder.class);
}
// Construct using io.bloombox.schema.services.devices.v1beta1.DeviceActivation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
hostname_ = "";
if (assignmentBuilder_ == null) {
assignment_ = null;
} else {
assignment_ = null;
assignmentBuilder_ = null;
}
if (ticketBuilder_ == null) {
ticket_ = null;
} else {
ticket_ = null;
ticketBuilder_ = null;
}
if (endpointsBuilder_ == null) {
endpoints_ = null;
} else {
endpoints_ = null;
endpointsBuilder_ = null;
}
if (beaconBuilder_ == null) {
beacon_ = null;
} else {
beacon_ = null;
beaconBuilder_ = null;
}
if (credentialsBuilder_ == null) {
credentials_ = null;
} else {
credentials_ = null;
credentialsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.bloombox.schema.services.devices.v1beta1.DevicesServiceBeta1.internal_static_bloombox_services_devices_v1beta1_DeviceActivation_descriptor;
}
@java.lang.Override
public io.bloombox.schema.services.devices.v1beta1.DeviceActivation getDefaultInstanceForType() {
return io.bloombox.schema.services.devices.v1beta1.DeviceActivation.getDefaultInstance();
}
@java.lang.Override
public io.bloombox.schema.services.devices.v1beta1.DeviceActivation build() {
io.bloombox.schema.services.devices.v1beta1.DeviceActivation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.bloombox.schema.services.devices.v1beta1.DeviceActivation buildPartial() {
io.bloombox.schema.services.devices.v1beta1.DeviceActivation result = new io.bloombox.schema.services.devices.v1beta1.DeviceActivation(this);
result.uuid_ = uuid_;
result.hostname_ = hostname_;
if (assignmentBuilder_ == null) {
result.assignment_ = assignment_;
} else {
result.assignment_ = assignmentBuilder_.build();
}
if (ticketBuilder_ == null) {
result.ticket_ = ticket_;
} else {
result.ticket_ = ticketBuilder_.build();
}
if (endpointsBuilder_ == null) {
result.endpoints_ = endpoints_;
} else {
result.endpoints_ = endpointsBuilder_.build();
}
if (beaconBuilder_ == null) {
result.beacon_ = beacon_;
} else {
result.beacon_ = beaconBuilder_.build();
}
if (credentialsBuilder_ == null) {
result.credentials_ = credentials_;
} else {
result.credentials_ = credentialsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.bloombox.schema.services.devices.v1beta1.DeviceActivation) {
return mergeFrom((io.bloombox.schema.services.devices.v1beta1.DeviceActivation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.bloombox.schema.services.devices.v1beta1.DeviceActivation other) {
if (other == io.bloombox.schema.services.devices.v1beta1.DeviceActivation.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
if (!other.getHostname().isEmpty()) {
hostname_ = other.hostname_;
onChanged();
}
if (other.hasAssignment()) {
mergeAssignment(other.getAssignment());
}
if (other.hasTicket()) {
mergeTicket(other.getTicket());
}
if (other.hasEndpoints()) {
mergeEndpoints(other.getEndpoints());
}
if (other.hasBeacon()) {
mergeBeacon(other.getBeacon());
}
if (other.hasCredentials()) {
mergeCredentials(other.getCredentials());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.bloombox.schema.services.devices.v1beta1.DeviceActivation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.bloombox.schema.services.devices.v1beta1.DeviceActivation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object uuid_ = "";
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* Unique ID for the device.
*
*
* string uuid = 1;
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
private java.lang.Object hostname_ = "";
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public java.lang.String getHostname() {
java.lang.Object ref = hostname_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
hostname_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public com.google.protobuf.ByteString
getHostnameBytes() {
java.lang.Object ref = hostname_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostname_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public Builder setHostname(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
hostname_ = value;
onChanged();
return this;
}
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public Builder clearHostname() {
hostname_ = getDefaultInstance().getHostname();
onChanged();
return this;
}
/**
*
* Hostname for the device.
*
*
* string hostname = 2;
*/
public Builder setHostnameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
hostname_ = value;
onChanged();
return this;
}
private io.bloombox.schema.services.devices.v1beta1.DeviceAssignment assignment_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment, io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceAssignmentOrBuilder> assignmentBuilder_;
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public boolean hasAssignment() {
return assignmentBuilder_ != null || assignment_ != null;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceAssignment getAssignment() {
if (assignmentBuilder_ == null) {
return assignment_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.getDefaultInstance() : assignment_;
} else {
return assignmentBuilder_.getMessage();
}
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public Builder setAssignment(io.bloombox.schema.services.devices.v1beta1.DeviceAssignment value) {
if (assignmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
assignment_ = value;
onChanged();
} else {
assignmentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public Builder setAssignment(
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder builderForValue) {
if (assignmentBuilder_ == null) {
assignment_ = builderForValue.build();
onChanged();
} else {
assignmentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public Builder mergeAssignment(io.bloombox.schema.services.devices.v1beta1.DeviceAssignment value) {
if (assignmentBuilder_ == null) {
if (assignment_ != null) {
assignment_ =
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.newBuilder(assignment_).mergeFrom(value).buildPartial();
} else {
assignment_ = value;
}
onChanged();
} else {
assignmentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public Builder clearAssignment() {
if (assignmentBuilder_ == null) {
assignment_ = null;
onChanged();
} else {
assignment_ = null;
assignmentBuilder_ = null;
}
return this;
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder getAssignmentBuilder() {
onChanged();
return getAssignmentFieldBuilder().getBuilder();
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceAssignmentOrBuilder getAssignmentOrBuilder() {
if (assignmentBuilder_ != null) {
return assignmentBuilder_.getMessageOrBuilder();
} else {
return assignment_ == null ?
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.getDefaultInstance() : assignment_;
}
}
/**
*
* Assignment information for the device.
*
*
* .bloombox.services.devices.v1beta1.DeviceAssignment assignment = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment, io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceAssignmentOrBuilder>
getAssignmentFieldBuilder() {
if (assignmentBuilder_ == null) {
assignmentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceAssignment, io.bloombox.schema.services.devices.v1beta1.DeviceAssignment.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceAssignmentOrBuilder>(
getAssignment(),
getParentForChildren(),
isClean());
assignment_ = null;
}
return assignmentBuilder_;
}
private io.bloombox.schema.security.DeviceSecurity.DeviceTicket ticket_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.security.DeviceSecurity.DeviceTicket, io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder, io.bloombox.schema.security.DeviceSecurity.DeviceTicketOrBuilder> ticketBuilder_;
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public boolean hasTicket() {
return ticketBuilder_ != null || ticket_ != null;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public io.bloombox.schema.security.DeviceSecurity.DeviceTicket getTicket() {
if (ticketBuilder_ == null) {
return ticket_ == null ? io.bloombox.schema.security.DeviceSecurity.DeviceTicket.getDefaultInstance() : ticket_;
} else {
return ticketBuilder_.getMessage();
}
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public Builder setTicket(io.bloombox.schema.security.DeviceSecurity.DeviceTicket value) {
if (ticketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ticket_ = value;
onChanged();
} else {
ticketBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public Builder setTicket(
io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder builderForValue) {
if (ticketBuilder_ == null) {
ticket_ = builderForValue.build();
onChanged();
} else {
ticketBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public Builder mergeTicket(io.bloombox.schema.security.DeviceSecurity.DeviceTicket value) {
if (ticketBuilder_ == null) {
if (ticket_ != null) {
ticket_ =
io.bloombox.schema.security.DeviceSecurity.DeviceTicket.newBuilder(ticket_).mergeFrom(value).buildPartial();
} else {
ticket_ = value;
}
onChanged();
} else {
ticketBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public Builder clearTicket() {
if (ticketBuilder_ == null) {
ticket_ = null;
onChanged();
} else {
ticket_ = null;
ticketBuilder_ = null;
}
return this;
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder getTicketBuilder() {
onChanged();
return getTicketFieldBuilder().getBuilder();
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
public io.bloombox.schema.security.DeviceSecurity.DeviceTicketOrBuilder getTicketOrBuilder() {
if (ticketBuilder_ != null) {
return ticketBuilder_.getMessageOrBuilder();
} else {
return ticket_ == null ?
io.bloombox.schema.security.DeviceSecurity.DeviceTicket.getDefaultInstance() : ticket_;
}
}
/**
*
* Specifies an activation ticket for the subject device, including authorization information and a signed JWT that
* allows the device access to assigned information and credentials.
*
*
* .bloombox.security.DeviceTicket ticket = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.security.DeviceSecurity.DeviceTicket, io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder, io.bloombox.schema.security.DeviceSecurity.DeviceTicketOrBuilder>
getTicketFieldBuilder() {
if (ticketBuilder_ == null) {
ticketBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.security.DeviceSecurity.DeviceTicket, io.bloombox.schema.security.DeviceSecurity.DeviceTicket.Builder, io.bloombox.schema.security.DeviceSecurity.DeviceTicketOrBuilder>(
getTicket(),
getParentForChildren(),
isClean());
ticket_ = null;
}
return ticketBuilder_;
}
private io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints endpoints_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints, io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceEndpointsOrBuilder> endpointsBuilder_;
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public boolean hasEndpoints() {
return endpointsBuilder_ != null || endpoints_ != null;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints getEndpoints() {
if (endpointsBuilder_ == null) {
return endpoints_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.getDefaultInstance() : endpoints_;
} else {
return endpointsBuilder_.getMessage();
}
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public Builder setEndpoints(io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints value) {
if (endpointsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endpoints_ = value;
onChanged();
} else {
endpointsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public Builder setEndpoints(
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder builderForValue) {
if (endpointsBuilder_ == null) {
endpoints_ = builderForValue.build();
onChanged();
} else {
endpointsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public Builder mergeEndpoints(io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints value) {
if (endpointsBuilder_ == null) {
if (endpoints_ != null) {
endpoints_ =
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.newBuilder(endpoints_).mergeFrom(value).buildPartial();
} else {
endpoints_ = value;
}
onChanged();
} else {
endpointsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public Builder clearEndpoints() {
if (endpointsBuilder_ == null) {
endpoints_ = null;
onChanged();
} else {
endpoints_ = null;
endpointsBuilder_ = null;
}
return this;
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder getEndpointsBuilder() {
onChanged();
return getEndpointsFieldBuilder().getBuilder();
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceEndpointsOrBuilder getEndpointsOrBuilder() {
if (endpointsBuilder_ != null) {
return endpointsBuilder_.getMessageOrBuilder();
} else {
return endpoints_ == null ?
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.getDefaultInstance() : endpoints_;
}
}
/**
*
* Payload specifying endpoints the device should use under different circumstances, to include OAuth2, API use, and
* direct use of Firebase.
*
*
* .bloombox.services.devices.v1beta1.DeviceEndpoints endpoints = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints, io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceEndpointsOrBuilder>
getEndpointsFieldBuilder() {
if (endpointsBuilder_ == null) {
endpointsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints, io.bloombox.schema.services.devices.v1beta1.DeviceEndpoints.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceEndpointsOrBuilder>(
getEndpoints(),
getParentForChildren(),
isClean());
endpoints_ = null;
}
return endpointsBuilder_;
}
private io.opencannabis.schema.proximity.BluetoothBeacon beacon_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.proximity.BluetoothBeacon, io.opencannabis.schema.proximity.BluetoothBeacon.Builder, io.opencannabis.schema.proximity.BluetoothBeaconOrBuilder> beaconBuilder_;
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public boolean hasBeacon() {
return beaconBuilder_ != null || beacon_ != null;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public io.opencannabis.schema.proximity.BluetoothBeacon getBeacon() {
if (beaconBuilder_ == null) {
return beacon_ == null ? io.opencannabis.schema.proximity.BluetoothBeacon.getDefaultInstance() : beacon_;
} else {
return beaconBuilder_.getMessage();
}
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public Builder setBeacon(io.opencannabis.schema.proximity.BluetoothBeacon value) {
if (beaconBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
beacon_ = value;
onChanged();
} else {
beaconBuilder_.setMessage(value);
}
return this;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public Builder setBeacon(
io.opencannabis.schema.proximity.BluetoothBeacon.Builder builderForValue) {
if (beaconBuilder_ == null) {
beacon_ = builderForValue.build();
onChanged();
} else {
beaconBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public Builder mergeBeacon(io.opencannabis.schema.proximity.BluetoothBeacon value) {
if (beaconBuilder_ == null) {
if (beacon_ != null) {
beacon_ =
io.opencannabis.schema.proximity.BluetoothBeacon.newBuilder(beacon_).mergeFrom(value).buildPartial();
} else {
beacon_ = value;
}
onChanged();
} else {
beaconBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public Builder clearBeacon() {
if (beaconBuilder_ == null) {
beacon_ = null;
onChanged();
} else {
beacon_ = null;
beaconBuilder_ = null;
}
return this;
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public io.opencannabis.schema.proximity.BluetoothBeacon.Builder getBeaconBuilder() {
onChanged();
return getBeaconFieldBuilder().getBuilder();
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
public io.opencannabis.schema.proximity.BluetoothBeaconOrBuilder getBeaconOrBuilder() {
if (beaconBuilder_ != null) {
return beaconBuilder_.getMessageOrBuilder();
} else {
return beacon_ == null ?
io.opencannabis.schema.proximity.BluetoothBeacon.getDefaultInstance() : beacon_;
}
}
/**
*
* If this device is assigned to broadcast a BLE signal, its configuration parameters are specified here, including
* the minimum required for an iBeacon (UUID, major and minor values).
*
*
* .opencannabis.proximity.BluetoothBeacon beacon = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.proximity.BluetoothBeacon, io.opencannabis.schema.proximity.BluetoothBeacon.Builder, io.opencannabis.schema.proximity.BluetoothBeaconOrBuilder>
getBeaconFieldBuilder() {
if (beaconBuilder_ == null) {
beaconBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.proximity.BluetoothBeacon, io.opencannabis.schema.proximity.BluetoothBeacon.Builder, io.opencannabis.schema.proximity.BluetoothBeaconOrBuilder>(
getBeacon(),
getParentForChildren(),
isClean());
beacon_ = null;
}
return beaconBuilder_;
}
private io.bloombox.schema.services.devices.v1beta1.DeviceKeys credentials_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceKeys, io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceKeysOrBuilder> credentialsBuilder_;
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public boolean hasCredentials() {
return credentialsBuilder_ != null || credentials_ != null;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceKeys getCredentials() {
if (credentialsBuilder_ == null) {
return credentials_ == null ? io.bloombox.schema.services.devices.v1beta1.DeviceKeys.getDefaultInstance() : credentials_;
} else {
return credentialsBuilder_.getMessage();
}
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public Builder setCredentials(io.bloombox.schema.services.devices.v1beta1.DeviceKeys value) {
if (credentialsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
credentials_ = value;
onChanged();
} else {
credentialsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public Builder setCredentials(
io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder builderForValue) {
if (credentialsBuilder_ == null) {
credentials_ = builderForValue.build();
onChanged();
} else {
credentialsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public Builder mergeCredentials(io.bloombox.schema.services.devices.v1beta1.DeviceKeys value) {
if (credentialsBuilder_ == null) {
if (credentials_ != null) {
credentials_ =
io.bloombox.schema.services.devices.v1beta1.DeviceKeys.newBuilder(credentials_).mergeFrom(value).buildPartial();
} else {
credentials_ = value;
}
onChanged();
} else {
credentialsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public Builder clearCredentials() {
if (credentialsBuilder_ == null) {
credentials_ = null;
onChanged();
} else {
credentials_ = null;
credentialsBuilder_ = null;
}
return this;
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder getCredentialsBuilder() {
onChanged();
return getCredentialsFieldBuilder().getBuilder();
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
public io.bloombox.schema.services.devices.v1beta1.DeviceKeysOrBuilder getCredentialsOrBuilder() {
if (credentialsBuilder_ != null) {
return credentialsBuilder_.getMessageOrBuilder();
} else {
return credentials_ == null ?
io.bloombox.schema.services.devices.v1beta1.DeviceKeys.getDefaultInstance() : credentials_;
}
}
/**
*
* Specifies the cryptographic material this activated device should make use of when communicating with the Bloombox
* Platform server-side systems.
*
*
* .bloombox.services.devices.v1beta1.DeviceKeys credentials = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceKeys, io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceKeysOrBuilder>
getCredentialsFieldBuilder() {
if (credentialsBuilder_ == null) {
credentialsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.services.devices.v1beta1.DeviceKeys, io.bloombox.schema.services.devices.v1beta1.DeviceKeys.Builder, io.bloombox.schema.services.devices.v1beta1.DeviceKeysOrBuilder>(
getCredentials(),
getParentForChildren(),
isClean());
credentials_ = null;
}
return credentialsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.services.devices.v1beta1.DeviceActivation)
}
// @@protoc_insertion_point(class_scope:bloombox.services.devices.v1beta1.DeviceActivation)
private static final io.bloombox.schema.services.devices.v1beta1.DeviceActivation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.bloombox.schema.services.devices.v1beta1.DeviceActivation();
}
public static io.bloombox.schema.services.devices.v1beta1.DeviceActivation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeviceActivation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeviceActivation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.bloombox.schema.services.devices.v1beta1.DeviceActivation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy