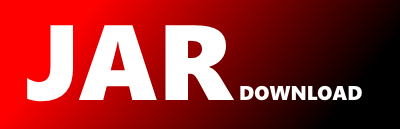
io.opencannabis.schema.commerce.CommercialOrder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas Co.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: commerce/Order.proto
package io.opencannabis.schema.commerce;
public final class CommercialOrder {
private CommercialOrder() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Specifies the type of order requested.
*
*
* Protobuf enum {@code opencannabis.commerce.OrderType}
*/
public enum OrderType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Express pickup order.
*
*
* PICKUP = 0;
*/
PICKUP(0),
/**
*
* Delivery order.
*
*
* DELIVERY = 1;
*/
DELIVERY(1),
UNRECOGNIZED(-1),
;
/**
*
* Express pickup order.
*
*
* PICKUP = 0;
*/
public static final int PICKUP_VALUE = 0;
/**
*
* Delivery order.
*
*
* DELIVERY = 1;
*/
public static final int DELIVERY_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OrderType valueOf(int value) {
return forNumber(value);
}
public static OrderType forNumber(int value) {
switch (value) {
case 0: return PICKUP;
case 1: return DELIVERY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OrderType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OrderType findValueByNumber(int number) {
return OrderType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.getDescriptor().getEnumTypes().get(0);
}
private static final OrderType[] VALUES = values();
public static OrderType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OrderType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.OrderType)
}
/**
*
* Specifies the types of delivery timing.
*
*
* Protobuf enum {@code opencannabis.commerce.SchedulingType}
*/
public enum SchedulingType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* As soon as possible.
*
*
* ASAP = 0;
*/
ASAP(0),
/**
*
* Desired time.
*
*
* TIMED = 1;
*/
TIMED(1),
UNRECOGNIZED(-1),
;
/**
*
* As soon as possible.
*
*
* ASAP = 0;
*/
public static final int ASAP_VALUE = 0;
/**
*
* Desired time.
*
*
* TIMED = 1;
*/
public static final int TIMED_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SchedulingType valueOf(int value) {
return forNumber(value);
}
public static SchedulingType forNumber(int value) {
switch (value) {
case 0: return ASAP;
case 1: return TIMED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SchedulingType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SchedulingType findValueByNumber(int number) {
return SchedulingType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.getDescriptor().getEnumTypes().get(1);
}
private static final SchedulingType[] VALUES = values();
public static SchedulingType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SchedulingType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.SchedulingType)
}
/**
*
* Enumeration for current status of order
*
*
* Protobuf enum {@code opencannabis.commerce.OrderStatus}
*/
public enum OrderStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Order has been submitted and is not yet approved.
*
*
* PENDING = 0;
*/
PENDING(0),
/**
*
* Order has been approved for fulfillment.
*
*
* APPROVED = 1;
*/
APPROVED(1),
/**
*
* Order could not be fulfilled for some reason.
*
*
* REJECTED = 2;
*/
REJECTED(2),
/**
*
* Order has been assigned.
*
*
* ASSIGNED = 3;
*/
ASSIGNED(3),
/**
*
* Order is en-route to the user (for delivery).
*
*
* EN_ROUTE = 4;
*/
EN_ROUTE(4),
/**
*
* Order has been fulfilled and is considered complete.
*
*
* FULFILLED = 5;
*/
FULFILLED(5),
UNRECOGNIZED(-1),
;
/**
*
* Order has been submitted and is not yet approved.
*
*
* PENDING = 0;
*/
public static final int PENDING_VALUE = 0;
/**
*
* Order has been approved for fulfillment.
*
*
* APPROVED = 1;
*/
public static final int APPROVED_VALUE = 1;
/**
*
* Order could not be fulfilled for some reason.
*
*
* REJECTED = 2;
*/
public static final int REJECTED_VALUE = 2;
/**
*
* Order has been assigned.
*
*
* ASSIGNED = 3;
*/
public static final int ASSIGNED_VALUE = 3;
/**
*
* Order is en-route to the user (for delivery).
*
*
* EN_ROUTE = 4;
*/
public static final int EN_ROUTE_VALUE = 4;
/**
*
* Order has been fulfilled and is considered complete.
*
*
* FULFILLED = 5;
*/
public static final int FULFILLED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OrderStatus valueOf(int value) {
return forNumber(value);
}
public static OrderStatus forNumber(int value) {
switch (value) {
case 0: return PENDING;
case 1: return APPROVED;
case 2: return REJECTED;
case 3: return ASSIGNED;
case 4: return EN_ROUTE;
case 5: return FULFILLED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OrderStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OrderStatus findValueByNumber(int number) {
return OrderStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.getDescriptor().getEnumTypes().get(2);
}
private static final OrderStatus[] VALUES = values();
public static OrderStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OrderStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.OrderStatus)
}
public interface OrderSchedulingOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.OrderScheduling)
com.google.protobuf.MessageOrBuilder {
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
int getSchedulingValue();
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
io.opencannabis.schema.commerce.CommercialOrder.SchedulingType getScheduling();
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
boolean hasDesiredTime();
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getDesiredTime();
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getDesiredTimeOrBuilder();
}
/**
*
* Specifies the desired timing of the delivery order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderScheduling}
*/
public static final class OrderScheduling extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.OrderScheduling)
OrderSchedulingOrBuilder {
private static final long serialVersionUID = 0L;
// Use OrderScheduling.newBuilder() to construct.
private OrderScheduling(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OrderScheduling() {
scheduling_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OrderScheduling(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
scheduling_ = rawValue;
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (desiredTime_ != null) {
subBuilder = desiredTime_.toBuilder();
}
desiredTime_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(desiredTime_);
desiredTime_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderScheduling_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderScheduling_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.class, io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder.class);
}
public static final int SCHEDULING_FIELD_NUMBER = 1;
private int scheduling_;
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public int getSchedulingValue() {
return scheduling_;
}
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.SchedulingType getScheduling() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.SchedulingType result = io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.valueOf(scheduling_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.UNRECOGNIZED : result;
}
public static final int DESIRED_TIME_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant desiredTime_;
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public boolean hasDesiredTime() {
return desiredTime_ != null;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getDesiredTime() {
return desiredTime_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : desiredTime_;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getDesiredTimeOrBuilder() {
return getDesiredTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (scheduling_ != io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.ASAP.getNumber()) {
output.writeEnum(1, scheduling_);
}
if (desiredTime_ != null) {
output.writeMessage(2, getDesiredTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (scheduling_ != io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.ASAP.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, scheduling_);
}
if (desiredTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDesiredTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling other = (io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling) obj;
if (scheduling_ != other.scheduling_) return false;
if (hasDesiredTime() != other.hasDesiredTime()) return false;
if (hasDesiredTime()) {
if (!getDesiredTime()
.equals(other.getDesiredTime())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SCHEDULING_FIELD_NUMBER;
hash = (53 * hash) + scheduling_;
if (hasDesiredTime()) {
hash = (37 * hash) + DESIRED_TIME_FIELD_NUMBER;
hash = (53 * hash) + getDesiredTime().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the desired timing of the delivery order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderScheduling}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.OrderScheduling)
io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderScheduling_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderScheduling_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.class, io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
scheduling_ = 0;
if (desiredTimeBuilder_ == null) {
desiredTime_ = null;
} else {
desiredTime_ = null;
desiredTimeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderScheduling_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling build() {
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling buildPartial() {
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling result = new io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling(this);
result.scheduling_ = scheduling_;
if (desiredTimeBuilder_ == null) {
result.desiredTime_ = desiredTime_;
} else {
result.desiredTime_ = desiredTimeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling other) {
if (other == io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.getDefaultInstance()) return this;
if (other.scheduling_ != 0) {
setSchedulingValue(other.getSchedulingValue());
}
if (other.hasDesiredTime()) {
mergeDesiredTime(other.getDesiredTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int scheduling_ = 0;
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public int getSchedulingValue() {
return scheduling_;
}
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public Builder setSchedulingValue(int value) {
scheduling_ = value;
onChanged();
return this;
}
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.SchedulingType getScheduling() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.SchedulingType result = io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.valueOf(scheduling_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.SchedulingType.UNRECOGNIZED : result;
}
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public Builder setScheduling(io.opencannabis.schema.commerce.CommercialOrder.SchedulingType value) {
if (value == null) {
throw new NullPointerException();
}
scheduling_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Scheduling type, either 'ASAP' or a target time.
*
*
* .opencannabis.commerce.SchedulingType scheduling = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling type, either \'ASAP\' or a target time."];
*/
public Builder clearScheduling() {
scheduling_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant desiredTime_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> desiredTimeBuilder_;
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public boolean hasDesiredTime() {
return desiredTimeBuilder_ != null || desiredTime_ != null;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getDesiredTime() {
if (desiredTimeBuilder_ == null) {
return desiredTime_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : desiredTime_;
} else {
return desiredTimeBuilder_.getMessage();
}
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public Builder setDesiredTime(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (desiredTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
desiredTime_ = value;
onChanged();
} else {
desiredTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public Builder setDesiredTime(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (desiredTimeBuilder_ == null) {
desiredTime_ = builderForValue.build();
onChanged();
} else {
desiredTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public Builder mergeDesiredTime(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (desiredTimeBuilder_ == null) {
if (desiredTime_ != null) {
desiredTime_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(desiredTime_).mergeFrom(value).buildPartial();
} else {
desiredTime_ = value;
}
onChanged();
} else {
desiredTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public Builder clearDesiredTime() {
if (desiredTimeBuilder_ == null) {
desiredTime_ = null;
onChanged();
} else {
desiredTime_ = null;
desiredTimeBuilder_ = null;
}
return this;
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getDesiredTimeBuilder() {
onChanged();
return getDesiredTimeFieldBuilder().getBuilder();
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getDesiredTimeOrBuilder() {
if (desiredTimeBuilder_ != null) {
return desiredTimeBuilder_.getMessageOrBuilder();
} else {
return desiredTime_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : desiredTime_;
}
}
/**
*
* Desired delivery time, if specified.
*
*
* .opencannabis.temporal.Instant desired_time = 2 [(.gen_bq_schema.description) = "Desired delivery time, if specified."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getDesiredTimeFieldBuilder() {
if (desiredTimeBuilder_ == null) {
desiredTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getDesiredTime(),
getParentForChildren(),
isClean());
desiredTime_ = null;
}
return desiredTimeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.OrderScheduling)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.OrderScheduling)
private static final io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling();
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OrderScheduling parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OrderScheduling(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.OrderPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
int getStatusValue();
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus();
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
int getMethodValue();
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod();
/**
*
* Amount of tax added to the subtotal.
*
*
* double tax = 3 [(.gen_bq_schema.description) = "Amount of tax added to the subtotal."];
*/
double getTax();
/**
*
* Amount the user has paid so far for this order.
*
*
* double paid = 4 [(.gen_bq_schema.description) = "Amount the user has paid so far for this order."];
*/
double getPaid();
}
/**
*
* Information about payment status and info for an order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderPayment}
*/
public static final class OrderPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.OrderPayment)
OrderPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use OrderPayment.newBuilder() to construct.
private OrderPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OrderPayment() {
status_ = 0;
method_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OrderPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 16: {
int rawValue = input.readEnum();
method_ = rawValue;
break;
}
case 25: {
tax_ = input.readDouble();
break;
}
case 33: {
paid_ = input.readDouble();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.class, io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentStatus result = io.opencannabis.schema.commerce.Payments.PaymentStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentStatus.UNRECOGNIZED : result;
}
public static final int METHOD_FIELD_NUMBER = 2;
private int method_;
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentMethod result = io.opencannabis.schema.commerce.Payments.PaymentMethod.valueOf(method_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentMethod.UNRECOGNIZED : result;
}
public static final int TAX_FIELD_NUMBER = 3;
private double tax_;
/**
*
* Amount of tax added to the subtotal.
*
*
* double tax = 3 [(.gen_bq_schema.description) = "Amount of tax added to the subtotal."];
*/
public double getTax() {
return tax_;
}
public static final int PAID_FIELD_NUMBER = 4;
private double paid_;
/**
*
* Amount the user has paid so far for this order.
*
*
* double paid = 4 [(.gen_bq_schema.description) = "Amount the user has paid so far for this order."];
*/
public double getPaid() {
return paid_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != io.opencannabis.schema.commerce.Payments.PaymentStatus.NOT_APPLICABLE.getNumber()) {
output.writeEnum(1, status_);
}
if (method_ != io.opencannabis.schema.commerce.Payments.PaymentMethod.CASH.getNumber()) {
output.writeEnum(2, method_);
}
if (tax_ != 0D) {
output.writeDouble(3, tax_);
}
if (paid_ != 0D) {
output.writeDouble(4, paid_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != io.opencannabis.schema.commerce.Payments.PaymentStatus.NOT_APPLICABLE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
if (method_ != io.opencannabis.schema.commerce.Payments.PaymentMethod.CASH.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, method_);
}
if (tax_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, tax_);
}
if (paid_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, paid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment other = (io.opencannabis.schema.commerce.CommercialOrder.OrderPayment) obj;
if (status_ != other.status_) return false;
if (method_ != other.method_) return false;
if (java.lang.Double.doubleToLongBits(getTax())
!= java.lang.Double.doubleToLongBits(
other.getTax())) return false;
if (java.lang.Double.doubleToLongBits(getPaid())
!= java.lang.Double.doubleToLongBits(
other.getPaid())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
hash = (37 * hash) + TAX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTax()));
hash = (37 * hash) + PAID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getPaid()));
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialOrder.OrderPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Information about payment status and info for an order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.OrderPayment)
io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.class, io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
method_ = 0;
tax_ = 0D;
paid_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment build() {
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment result = new io.opencannabis.schema.commerce.CommercialOrder.OrderPayment(this);
result.status_ = status_;
result.method_ = method_;
result.tax_ = tax_;
result.paid_ = paid_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialOrder.OrderPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialOrder.OrderPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.method_ != 0) {
setMethodValue(other.getMethodValue());
}
if (other.getTax() != 0D) {
setTax(other.getTax());
}
if (other.getPaid() != 0D) {
setPaid(other.getPaid());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialOrder.OrderPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int status_ = 0;
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentStatus result = io.opencannabis.schema.commerce.Payments.PaymentStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentStatus.UNRECOGNIZED : result;
}
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public Builder setStatus(io.opencannabis.schema.commerce.Payments.PaymentStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status of payment for this order.
*
*
* .opencannabis.commerce.PaymentStatus status = 1 [(.gen_bq_schema.description) = "Status of payment for this order."];
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private int method_ = 0;
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public Builder setMethodValue(int value) {
method_ = value;
onChanged();
return this;
}
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentMethod result = io.opencannabis.schema.commerce.Payments.PaymentMethod.valueOf(method_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentMethod.UNRECOGNIZED : result;
}
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public Builder setMethod(io.opencannabis.schema.commerce.Payments.PaymentMethod value) {
if (value == null) {
throw new NullPointerException();
}
method_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Method of payment used on this order.
*
*
* .opencannabis.commerce.PaymentMethod method = 2 [(.gen_bq_schema.description) = "Method of payment used on this order."];
*/
public Builder clearMethod() {
method_ = 0;
onChanged();
return this;
}
private double tax_ ;
/**
*
* Amount of tax added to the subtotal.
*
*
* double tax = 3 [(.gen_bq_schema.description) = "Amount of tax added to the subtotal."];
*/
public double getTax() {
return tax_;
}
/**
*
* Amount of tax added to the subtotal.
*
*
* double tax = 3 [(.gen_bq_schema.description) = "Amount of tax added to the subtotal."];
*/
public Builder setTax(double value) {
tax_ = value;
onChanged();
return this;
}
/**
*
* Amount of tax added to the subtotal.
*
*
* double tax = 3 [(.gen_bq_schema.description) = "Amount of tax added to the subtotal."];
*/
public Builder clearTax() {
tax_ = 0D;
onChanged();
return this;
}
private double paid_ ;
/**
*
* Amount the user has paid so far for this order.
*
*
* double paid = 4 [(.gen_bq_schema.description) = "Amount the user has paid so far for this order."];
*/
public double getPaid() {
return paid_;
}
/**
*
* Amount the user has paid so far for this order.
*
*
* double paid = 4 [(.gen_bq_schema.description) = "Amount the user has paid so far for this order."];
*/
public Builder setPaid(double value) {
paid_ = value;
onChanged();
return this;
}
/**
*
* Amount the user has paid so far for this order.
*
*
* double paid = 4 [(.gen_bq_schema.description) = "Amount the user has paid so far for this order."];
*/
public Builder clearPaid() {
paid_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.OrderPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.OrderPayment)
private static final io.opencannabis.schema.commerce.CommercialOrder.OrderPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialOrder.OrderPayment();
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OrderPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OrderPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StatusCheckinOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.StatusCheckin)
com.google.protobuf.MessageOrBuilder {
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
int getStatusValue();
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
boolean hasInstant();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder();
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
java.lang.String getMessage();
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
*
* Specifies a moment at which an order changed status, when it happened, and, optionally, why.
*
*
* Protobuf type {@code opencannabis.commerce.StatusCheckin}
*/
public static final class StatusCheckin extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.StatusCheckin)
StatusCheckinOrBuilder {
private static final long serialVersionUID = 0L;
// Use StatusCheckin.newBuilder() to construct.
private StatusCheckin(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StatusCheckin() {
status_ = 0;
message_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StatusCheckin(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (instant_ != null) {
subBuilder = instant_.toBuilder();
}
instant_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(instant_);
instant_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_StatusCheckin_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_StatusCheckin_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.class, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus result = io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.UNRECOGNIZED : result;
}
public static final int INSTANT_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant instant_;
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public boolean hasInstant() {
return instant_ != null;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant() {
return instant_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder() {
return getInstant();
}
public static final int MESSAGE_FIELD_NUMBER = 3;
private volatile java.lang.Object message_;
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.PENDING.getNumber()) {
output.writeEnum(1, status_);
}
if (instant_ != null) {
output.writeMessage(2, getInstant());
}
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, message_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.PENDING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
if (instant_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInstant());
}
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, message_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin other = (io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin) obj;
if (status_ != other.status_) return false;
if (hasInstant() != other.hasInstant()) return false;
if (hasInstant()) {
if (!getInstant()
.equals(other.getInstant())) return false;
}
if (!getMessage()
.equals(other.getMessage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasInstant()) {
hash = (37 * hash) + INSTANT_FIELD_NUMBER;
hash = (53 * hash) + getInstant().hashCode();
}
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a moment at which an order changed status, when it happened, and, optionally, why.
*
*
* Protobuf type {@code opencannabis.commerce.StatusCheckin}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.StatusCheckin)
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_StatusCheckin_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_StatusCheckin_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.class, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
if (instantBuilder_ == null) {
instant_ = null;
} else {
instant_ = null;
instantBuilder_ = null;
}
message_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_StatusCheckin_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin build() {
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin buildPartial() {
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin result = new io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin(this);
result.status_ = status_;
if (instantBuilder_ == null) {
result.instant_ = instant_;
} else {
result.instant_ = instantBuilder_.build();
}
result.message_ = message_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin other) {
if (other == io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasInstant()) {
mergeInstant(other.getInstant());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int status_ = 0;
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus result = io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.UNRECOGNIZED : result;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialOrder.OrderStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.OrderStatus status = 1;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant instant_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> instantBuilder_;
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public boolean hasInstant() {
return instantBuilder_ != null || instant_ != null;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant() {
if (instantBuilder_ == null) {
return instant_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
} else {
return instantBuilder_.getMessage();
}
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public Builder setInstant(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (instantBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
instant_ = value;
onChanged();
} else {
instantBuilder_.setMessage(value);
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public Builder setInstant(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (instantBuilder_ == null) {
instant_ = builderForValue.build();
onChanged();
} else {
instantBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public Builder mergeInstant(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (instantBuilder_ == null) {
if (instant_ != null) {
instant_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(instant_).mergeFrom(value).buildPartial();
} else {
instant_ = value;
}
onChanged();
} else {
instantBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public Builder clearInstant() {
if (instantBuilder_ == null) {
instant_ = null;
onChanged();
} else {
instant_ = null;
instantBuilder_ = null;
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getInstantBuilder() {
onChanged();
return getInstantFieldBuilder().getBuilder();
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder() {
if (instantBuilder_ != null) {
return instantBuilder_.getMessageOrBuilder();
} else {
return instant_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
}
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getInstantFieldBuilder() {
if (instantBuilder_ == null) {
instantBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getInstant(),
getParentForChildren(),
isClean());
instant_ = null;
}
return instantBuilder_;
}
private java.lang.Object message_ = "";
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 3;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.StatusCheckin)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.StatusCheckin)
private static final io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin();
}
public static io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StatusCheckin parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StatusCheckin(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.OrderKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
java.lang.String getId();
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
com.google.protobuf.ByteString
getIdBytes();
}
/**
*
* Specifies a unique key for a commercial order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderKey}
*/
public static final class OrderKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.OrderKey)
OrderKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use OrderKey.newBuilder() to construct.
private OrderKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OrderKey() {
id_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OrderKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderKey.class, io.opencannabis.schema.commerce.CommercialOrder.OrderKey.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderKey)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialOrder.OrderKey other = (io.opencannabis.schema.commerce.CommercialOrder.OrderKey) obj;
if (!getId()
.equals(other.getId())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialOrder.OrderKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a unique key for a commercial order.
*
*
* Protobuf type {@code opencannabis.commerce.OrderKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.OrderKey)
io.opencannabis.schema.commerce.CommercialOrder.OrderKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.OrderKey.class, io.opencannabis.schema.commerce.CommercialOrder.OrderKey.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialOrder.OrderKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_OrderKey_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderKey getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialOrder.OrderKey.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderKey build() {
io.opencannabis.schema.commerce.CommercialOrder.OrderKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderKey buildPartial() {
io.opencannabis.schema.commerce.CommercialOrder.OrderKey result = new io.opencannabis.schema.commerce.CommercialOrder.OrderKey(this);
result.id_ = id_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialOrder.OrderKey) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialOrder.OrderKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialOrder.OrderKey other) {
if (other == io.opencannabis.schema.commerce.CommercialOrder.OrderKey.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialOrder.OrderKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialOrder.OrderKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Order ID, assigned by the server upon creation.
*
*
* string id = 1 [(.gen_bq_schema.description) = "Order ID, assigned by the server upon creation."];
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.OrderKey)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.OrderKey)
private static final io.opencannabis.schema.commerce.CommercialOrder.OrderKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialOrder.OrderKey();
}
public static io.opencannabis.schema.commerce.CommercialOrder.OrderKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OrderKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OrderKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.OrderKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Order)
com.google.protobuf.MessageOrBuilder {
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
java.lang.String getId();
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
int getTypeValue();
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderType getType();
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
int getStatusValue();
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus();
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
boolean hasCustomer();
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
io.opencannabis.schema.commerce.OrderCustomer.Customer getCustomer();
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder getCustomerOrBuilder();
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
boolean hasScheduling();
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getScheduling();
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder getSchedulingOrBuilder();
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
boolean hasDestination();
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination getDestination();
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder getDestinationOrBuilder();
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
java.lang.String getNotes();
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
com.google.protobuf.ByteString
getNotesBytes();
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
java.util.List
getItemList();
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.commerce.OrderItem.Item getItem(int index);
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
int getItemCount();
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
java.util.List extends io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>
getItemOrBuilderList();
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder(
int index);
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
java.util.List
getActionLogList();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getActionLog(int index);
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
int getActionLogCount();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder>
getActionLogOrBuilderList();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder getActionLogOrBuilder(
int index);
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
boolean hasCreatedAt();
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getCreatedAt();
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedAtOrBuilder();
/**
*
* Order subtotal.
*
*
* double subtotal = 11 [(.gen_bq_schema.description) = "Order subtotal."];
*/
double getSubtotal();
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
boolean hasUpdatedAt();
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getUpdatedAt();
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getUpdatedAtOrBuilder();
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
java.lang.String getSid();
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
com.google.protobuf.ByteString
getSidBytes();
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
boolean hasPayment();
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getPayment();
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder getPaymentOrBuilder();
}
/**
*
* Represents a full order submitted to the server for fulfillment, from an end-user, for delivery or express pickup.
*
*
* Protobuf type {@code opencannabis.commerce.Order}
*/
public static final class Order extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Order)
OrderOrBuilder {
private static final long serialVersionUID = 0L;
// Use Order.newBuilder() to construct.
private Order(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Order() {
id_ = "";
type_ = 0;
status_ = 0;
notes_ = "";
item_ = java.util.Collections.emptyList();
actionLog_ = java.util.Collections.emptyList();
sid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Order(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 34: {
io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder subBuilder = null;
if (customer_ != null) {
subBuilder = customer_.toBuilder();
}
customer_ = input.readMessage(io.opencannabis.schema.commerce.OrderCustomer.Customer.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(customer_);
customer_ = subBuilder.buildPartial();
}
break;
}
case 42: {
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder subBuilder = null;
if (scheduling_ != null) {
subBuilder = scheduling_.toBuilder();
}
scheduling_ = input.readMessage(io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(scheduling_);
scheduling_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder subBuilder = null;
if (destination_ != null) {
subBuilder = destination_.toBuilder();
}
destination_ = input.readMessage(io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(destination_);
destination_ = subBuilder.buildPartial();
}
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
notes_ = s;
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) != 0)) {
item_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
item_.add(
input.readMessage(io.opencannabis.schema.commerce.OrderItem.Item.parser(), extensionRegistry));
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
actionLog_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
actionLog_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.parser(), extensionRegistry));
break;
}
case 82: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (createdAt_ != null) {
subBuilder = createdAt_.toBuilder();
}
createdAt_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(createdAt_);
createdAt_ = subBuilder.buildPartial();
}
break;
}
case 89: {
subtotal_ = input.readDouble();
break;
}
case 98: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (updatedAt_ != null) {
subBuilder = updatedAt_.toBuilder();
}
updatedAt_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(updatedAt_);
updatedAt_ = subBuilder.buildPartial();
}
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
sid_ = s;
break;
}
case 114: {
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder subBuilder = null;
if (payment_ != null) {
subBuilder = payment_.toBuilder();
}
payment_ = input.readMessage(io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(payment_);
payment_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
}
if (((mutable_bitField0_ & 0x00000100) != 0)) {
actionLog_ = java.util.Collections.unmodifiableList(actionLog_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_Order_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_Order_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.Order.class, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderType getType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderType result = io.opencannabis.schema.commerce.CommercialOrder.OrderType.valueOf(type_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderType.UNRECOGNIZED : result;
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public int getStatusValue() {
return status_;
}
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus result = io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.UNRECOGNIZED : result;
}
public static final int CUSTOMER_FIELD_NUMBER = 4;
private io.opencannabis.schema.commerce.OrderCustomer.Customer customer_;
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public boolean hasCustomer() {
return customer_ != null;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public io.opencannabis.schema.commerce.OrderCustomer.Customer getCustomer() {
return customer_ == null ? io.opencannabis.schema.commerce.OrderCustomer.Customer.getDefaultInstance() : customer_;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder getCustomerOrBuilder() {
return getCustomer();
}
public static final int SCHEDULING_FIELD_NUMBER = 5;
private io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling scheduling_;
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public boolean hasScheduling() {
return scheduling_ != null;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getScheduling() {
return scheduling_ == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.getDefaultInstance() : scheduling_;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder getSchedulingOrBuilder() {
return getScheduling();
}
public static final int DESTINATION_FIELD_NUMBER = 6;
private io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination destination_;
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public boolean hasDestination() {
return destination_ != null;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination getDestination() {
return destination_ == null ? io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.getDefaultInstance() : destination_;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder getDestinationOrBuilder() {
return getDestination();
}
public static final int NOTES_FIELD_NUMBER = 7;
private volatile java.lang.Object notes_;
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
}
}
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEM_FIELD_NUMBER = 8;
private java.util.List item_;
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List getItemList() {
return item_;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List extends io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public int getItemCount() {
return item_.size();
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.Item getItem(int index) {
return item_.get(index);
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int ACTION_LOG_FIELD_NUMBER = 9;
private java.util.List actionLog_;
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List getActionLogList() {
return actionLog_;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder>
getActionLogOrBuilderList() {
return actionLog_;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public int getActionLogCount() {
return actionLog_.size();
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getActionLog(int index) {
return actionLog_.get(index);
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder getActionLogOrBuilder(
int index) {
return actionLog_.get(index);
}
public static final int CREATED_AT_FIELD_NUMBER = 10;
private io.opencannabis.schema.temporal.TemporalInstant.Instant createdAt_;
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public boolean hasCreatedAt() {
return createdAt_ != null;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreatedAt() {
return createdAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : createdAt_;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedAtOrBuilder() {
return getCreatedAt();
}
public static final int SUBTOTAL_FIELD_NUMBER = 11;
private double subtotal_;
/**
*
* Order subtotal.
*
*
* double subtotal = 11 [(.gen_bq_schema.description) = "Order subtotal."];
*/
public double getSubtotal() {
return subtotal_;
}
public static final int UPDATED_AT_FIELD_NUMBER = 12;
private io.opencannabis.schema.temporal.TemporalInstant.Instant updatedAt_;
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public boolean hasUpdatedAt() {
return updatedAt_ != null;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getUpdatedAt() {
return updatedAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : updatedAt_;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getUpdatedAtOrBuilder() {
return getUpdatedAt();
}
public static final int SID_FIELD_NUMBER = 13;
private volatile java.lang.Object sid_;
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public java.lang.String getSid() {
java.lang.Object ref = sid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sid_ = s;
return s;
}
}
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public com.google.protobuf.ByteString
getSidBytes() {
java.lang.Object ref = sid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAYMENT_FIELD_NUMBER = 14;
private io.opencannabis.schema.commerce.CommercialOrder.OrderPayment payment_;
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public boolean hasPayment() {
return payment_ != null;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getPayment() {
return payment_ == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.getDefaultInstance() : payment_;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder getPaymentOrBuilder() {
return getPayment();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (type_ != io.opencannabis.schema.commerce.CommercialOrder.OrderType.PICKUP.getNumber()) {
output.writeEnum(2, type_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.PENDING.getNumber()) {
output.writeEnum(3, status_);
}
if (customer_ != null) {
output.writeMessage(4, getCustomer());
}
if (scheduling_ != null) {
output.writeMessage(5, getScheduling());
}
if (destination_ != null) {
output.writeMessage(6, getDestination());
}
if (!getNotesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, notes_);
}
for (int i = 0; i < item_.size(); i++) {
output.writeMessage(8, item_.get(i));
}
for (int i = 0; i < actionLog_.size(); i++) {
output.writeMessage(9, actionLog_.get(i));
}
if (createdAt_ != null) {
output.writeMessage(10, getCreatedAt());
}
if (subtotal_ != 0D) {
output.writeDouble(11, subtotal_);
}
if (updatedAt_ != null) {
output.writeMessage(12, getUpdatedAt());
}
if (!getSidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, sid_);
}
if (payment_ != null) {
output.writeMessage(14, getPayment());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (type_ != io.opencannabis.schema.commerce.CommercialOrder.OrderType.PICKUP.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.PENDING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (customer_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getCustomer());
}
if (scheduling_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getScheduling());
}
if (destination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getDestination());
}
if (!getNotesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, notes_);
}
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, item_.get(i));
}
for (int i = 0; i < actionLog_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, actionLog_.get(i));
}
if (createdAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getCreatedAt());
}
if (subtotal_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(11, subtotal_);
}
if (updatedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getUpdatedAt());
}
if (!getSidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, sid_);
}
if (payment_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getPayment());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialOrder.Order)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialOrder.Order other = (io.opencannabis.schema.commerce.CommercialOrder.Order) obj;
if (!getId()
.equals(other.getId())) return false;
if (type_ != other.type_) return false;
if (status_ != other.status_) return false;
if (hasCustomer() != other.hasCustomer()) return false;
if (hasCustomer()) {
if (!getCustomer()
.equals(other.getCustomer())) return false;
}
if (hasScheduling() != other.hasScheduling()) return false;
if (hasScheduling()) {
if (!getScheduling()
.equals(other.getScheduling())) return false;
}
if (hasDestination() != other.hasDestination()) return false;
if (hasDestination()) {
if (!getDestination()
.equals(other.getDestination())) return false;
}
if (!getNotes()
.equals(other.getNotes())) return false;
if (!getItemList()
.equals(other.getItemList())) return false;
if (!getActionLogList()
.equals(other.getActionLogList())) return false;
if (hasCreatedAt() != other.hasCreatedAt()) return false;
if (hasCreatedAt()) {
if (!getCreatedAt()
.equals(other.getCreatedAt())) return false;
}
if (java.lang.Double.doubleToLongBits(getSubtotal())
!= java.lang.Double.doubleToLongBits(
other.getSubtotal())) return false;
if (hasUpdatedAt() != other.hasUpdatedAt()) return false;
if (hasUpdatedAt()) {
if (!getUpdatedAt()
.equals(other.getUpdatedAt())) return false;
}
if (!getSid()
.equals(other.getSid())) return false;
if (hasPayment() != other.hasPayment()) return false;
if (hasPayment()) {
if (!getPayment()
.equals(other.getPayment())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasCustomer()) {
hash = (37 * hash) + CUSTOMER_FIELD_NUMBER;
hash = (53 * hash) + getCustomer().hashCode();
}
if (hasScheduling()) {
hash = (37 * hash) + SCHEDULING_FIELD_NUMBER;
hash = (53 * hash) + getScheduling().hashCode();
}
if (hasDestination()) {
hash = (37 * hash) + DESTINATION_FIELD_NUMBER;
hash = (53 * hash) + getDestination().hashCode();
}
hash = (37 * hash) + NOTES_FIELD_NUMBER;
hash = (53 * hash) + getNotes().hashCode();
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (getActionLogCount() > 0) {
hash = (37 * hash) + ACTION_LOG_FIELD_NUMBER;
hash = (53 * hash) + getActionLogList().hashCode();
}
if (hasCreatedAt()) {
hash = (37 * hash) + CREATED_AT_FIELD_NUMBER;
hash = (53 * hash) + getCreatedAt().hashCode();
}
hash = (37 * hash) + SUBTOTAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSubtotal()));
if (hasUpdatedAt()) {
hash = (37 * hash) + UPDATED_AT_FIELD_NUMBER;
hash = (53 * hash) + getUpdatedAt().hashCode();
}
hash = (37 * hash) + SID_FIELD_NUMBER;
hash = (53 * hash) + getSid().hashCode();
if (hasPayment()) {
hash = (37 * hash) + PAYMENT_FIELD_NUMBER;
hash = (53 * hash) + getPayment().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialOrder.Order prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents a full order submitted to the server for fulfillment, from an end-user, for delivery or express pickup.
*
*
* Protobuf type {@code opencannabis.commerce.Order}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Order)
io.opencannabis.schema.commerce.CommercialOrder.OrderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_Order_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_Order_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialOrder.Order.class, io.opencannabis.schema.commerce.CommercialOrder.Order.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialOrder.Order.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getItemFieldBuilder();
getActionLogFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
type_ = 0;
status_ = 0;
if (customerBuilder_ == null) {
customer_ = null;
} else {
customer_ = null;
customerBuilder_ = null;
}
if (schedulingBuilder_ == null) {
scheduling_ = null;
} else {
scheduling_ = null;
schedulingBuilder_ = null;
}
if (destinationBuilder_ == null) {
destination_ = null;
} else {
destination_ = null;
destinationBuilder_ = null;
}
notes_ = "";
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
itemBuilder_.clear();
}
if (actionLogBuilder_ == null) {
actionLog_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
} else {
actionLogBuilder_.clear();
}
if (createdAtBuilder_ == null) {
createdAt_ = null;
} else {
createdAt_ = null;
createdAtBuilder_ = null;
}
subtotal_ = 0D;
if (updatedAtBuilder_ == null) {
updatedAt_ = null;
} else {
updatedAt_ = null;
updatedAtBuilder_ = null;
}
sid_ = "";
if (paymentBuilder_ == null) {
payment_ = null;
} else {
payment_ = null;
paymentBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialOrder.internal_static_opencannabis_commerce_Order_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.Order getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.Order build() {
io.opencannabis.schema.commerce.CommercialOrder.Order result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.Order buildPartial() {
io.opencannabis.schema.commerce.CommercialOrder.Order result = new io.opencannabis.schema.commerce.CommercialOrder.Order(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.id_ = id_;
result.type_ = type_;
result.status_ = status_;
if (customerBuilder_ == null) {
result.customer_ = customer_;
} else {
result.customer_ = customerBuilder_.build();
}
if (schedulingBuilder_ == null) {
result.scheduling_ = scheduling_;
} else {
result.scheduling_ = schedulingBuilder_.build();
}
if (destinationBuilder_ == null) {
result.destination_ = destination_;
} else {
result.destination_ = destinationBuilder_.build();
}
result.notes_ = notes_;
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
if (actionLogBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
actionLog_ = java.util.Collections.unmodifiableList(actionLog_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.actionLog_ = actionLog_;
} else {
result.actionLog_ = actionLogBuilder_.build();
}
if (createdAtBuilder_ == null) {
result.createdAt_ = createdAt_;
} else {
result.createdAt_ = createdAtBuilder_.build();
}
result.subtotal_ = subtotal_;
if (updatedAtBuilder_ == null) {
result.updatedAt_ = updatedAt_;
} else {
result.updatedAt_ = updatedAtBuilder_.build();
}
result.sid_ = sid_;
if (paymentBuilder_ == null) {
result.payment_ = payment_;
} else {
result.payment_ = paymentBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialOrder.Order) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialOrder.Order)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialOrder.Order other) {
if (other == io.opencannabis.schema.commerce.CommercialOrder.Order.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasCustomer()) {
mergeCustomer(other.getCustomer());
}
if (other.hasScheduling()) {
mergeScheduling(other.getScheduling());
}
if (other.hasDestination()) {
mergeDestination(other.getDestination());
}
if (!other.getNotes().isEmpty()) {
notes_ = other.notes_;
onChanged();
}
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000080);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (actionLogBuilder_ == null) {
if (!other.actionLog_.isEmpty()) {
if (actionLog_.isEmpty()) {
actionLog_ = other.actionLog_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureActionLogIsMutable();
actionLog_.addAll(other.actionLog_);
}
onChanged();
}
} else {
if (!other.actionLog_.isEmpty()) {
if (actionLogBuilder_.isEmpty()) {
actionLogBuilder_.dispose();
actionLogBuilder_ = null;
actionLog_ = other.actionLog_;
bitField0_ = (bitField0_ & ~0x00000100);
actionLogBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getActionLogFieldBuilder() : null;
} else {
actionLogBuilder_.addAllMessages(other.actionLog_);
}
}
}
if (other.hasCreatedAt()) {
mergeCreatedAt(other.getCreatedAt());
}
if (other.getSubtotal() != 0D) {
setSubtotal(other.getSubtotal());
}
if (other.hasUpdatedAt()) {
mergeUpdatedAt(other.getUpdatedAt());
}
if (!other.getSid().isEmpty()) {
sid_ = other.sid_;
onChanged();
}
if (other.hasPayment()) {
mergePayment(other.getPayment());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialOrder.Order parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialOrder.Order) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* ID assigned to the order by the server, and potentially nominated by the client.
*
*
* string id = 1 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "ID assigned to the order by the server, and potentially nominated by the client."];
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public int getTypeValue() {
return type_;
}
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderType getType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderType result = io.opencannabis.schema.commerce.CommercialOrder.OrderType.valueOf(type_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderType.UNRECOGNIZED : result;
}
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public Builder setType(io.opencannabis.schema.commerce.CommercialOrder.OrderType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Type of order requested - either PICKUP or DELIVERY.
*
*
* .opencannabis.commerce.OrderType type = 2 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Type of order requested - either PICKUP or DELIVERY."];
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public int getStatusValue() {
return status_;
}
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialOrder.OrderStatus result = io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderStatus.UNRECOGNIZED : result;
}
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialOrder.OrderStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Current status of this order.
*
*
* .opencannabis.commerce.OrderStatus status = 3 [(.gen_bq_schema.ignore) = true];
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.OrderCustomer.Customer customer_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderCustomer.Customer, io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder, io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder> customerBuilder_;
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public boolean hasCustomer() {
return customerBuilder_ != null || customer_ != null;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public io.opencannabis.schema.commerce.OrderCustomer.Customer getCustomer() {
if (customerBuilder_ == null) {
return customer_ == null ? io.opencannabis.schema.commerce.OrderCustomer.Customer.getDefaultInstance() : customer_;
} else {
return customerBuilder_.getMessage();
}
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public Builder setCustomer(io.opencannabis.schema.commerce.OrderCustomer.Customer value) {
if (customerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
customer_ = value;
onChanged();
} else {
customerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public Builder setCustomer(
io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder builderForValue) {
if (customerBuilder_ == null) {
customer_ = builderForValue.build();
onChanged();
} else {
customerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public Builder mergeCustomer(io.opencannabis.schema.commerce.OrderCustomer.Customer value) {
if (customerBuilder_ == null) {
if (customer_ != null) {
customer_ =
io.opencannabis.schema.commerce.OrderCustomer.Customer.newBuilder(customer_).mergeFrom(value).buildPartial();
} else {
customer_ = value;
}
onChanged();
} else {
customerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public Builder clearCustomer() {
if (customerBuilder_ == null) {
customer_ = null;
onChanged();
} else {
customer_ = null;
customerBuilder_ = null;
}
return this;
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder getCustomerBuilder() {
onChanged();
return getCustomerFieldBuilder().getBuilder();
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
public io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder getCustomerOrBuilder() {
if (customerBuilder_ != null) {
return customerBuilder_.getMessageOrBuilder();
} else {
return customer_ == null ?
io.opencannabis.schema.commerce.OrderCustomer.Customer.getDefaultInstance() : customer_;
}
}
/**
*
* Customer that submitted this order.
*
*
* .opencannabis.commerce.Customer customer = 4 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Customer that submitted this order."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderCustomer.Customer, io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder, io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder>
getCustomerFieldBuilder() {
if (customerBuilder_ == null) {
customerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderCustomer.Customer, io.opencannabis.schema.commerce.OrderCustomer.Customer.Builder, io.opencannabis.schema.commerce.OrderCustomer.CustomerOrBuilder>(
getCustomer(),
getParentForChildren(),
isClean());
customer_ = null;
}
return customerBuilder_;
}
private io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling scheduling_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling, io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder> schedulingBuilder_;
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public boolean hasScheduling() {
return schedulingBuilder_ != null || scheduling_ != null;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling getScheduling() {
if (schedulingBuilder_ == null) {
return scheduling_ == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.getDefaultInstance() : scheduling_;
} else {
return schedulingBuilder_.getMessage();
}
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public Builder setScheduling(io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling value) {
if (schedulingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheduling_ = value;
onChanged();
} else {
schedulingBuilder_.setMessage(value);
}
return this;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public Builder setScheduling(
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder builderForValue) {
if (schedulingBuilder_ == null) {
scheduling_ = builderForValue.build();
onChanged();
} else {
schedulingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public Builder mergeScheduling(io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling value) {
if (schedulingBuilder_ == null) {
if (scheduling_ != null) {
scheduling_ =
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.newBuilder(scheduling_).mergeFrom(value).buildPartial();
} else {
scheduling_ = value;
}
onChanged();
} else {
schedulingBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public Builder clearScheduling() {
if (schedulingBuilder_ == null) {
scheduling_ = null;
onChanged();
} else {
scheduling_ = null;
schedulingBuilder_ = null;
}
return this;
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder getSchedulingBuilder() {
onChanged();
return getSchedulingFieldBuilder().getBuilder();
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder getSchedulingOrBuilder() {
if (schedulingBuilder_ != null) {
return schedulingBuilder_.getMessageOrBuilder();
} else {
return scheduling_ == null ?
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.getDefaultInstance() : scheduling_;
}
}
/**
*
* Scheduling spec for this order.
*
*
* .opencannabis.commerce.OrderScheduling scheduling = 5 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "Scheduling spec for this order."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling, io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder>
getSchedulingFieldBuilder() {
if (schedulingBuilder_ == null) {
schedulingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling, io.opencannabis.schema.commerce.CommercialOrder.OrderScheduling.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderSchedulingOrBuilder>(
getScheduling(),
getParentForChildren(),
isClean());
scheduling_ = null;
}
return schedulingBuilder_;
}
private io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination destination_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder> destinationBuilder_;
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public boolean hasDestination() {
return destinationBuilder_ != null || destination_ != null;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination getDestination() {
if (destinationBuilder_ == null) {
return destination_ == null ? io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.getDefaultInstance() : destination_;
} else {
return destinationBuilder_.getMessage();
}
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public Builder setDestination(io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination value) {
if (destinationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
destination_ = value;
onChanged();
} else {
destinationBuilder_.setMessage(value);
}
return this;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public Builder setDestination(
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder builderForValue) {
if (destinationBuilder_ == null) {
destination_ = builderForValue.build();
onChanged();
} else {
destinationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public Builder mergeDestination(io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination value) {
if (destinationBuilder_ == null) {
if (destination_ != null) {
destination_ =
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.newBuilder(destination_).mergeFrom(value).buildPartial();
} else {
destination_ = value;
}
onChanged();
} else {
destinationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public Builder clearDestination() {
if (destinationBuilder_ == null) {
destination_ = null;
onChanged();
} else {
destination_ = null;
destinationBuilder_ = null;
}
return this;
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder getDestinationBuilder() {
onChanged();
return getDestinationFieldBuilder().getBuilder();
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
public io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder getDestinationOrBuilder() {
if (destinationBuilder_ != null) {
return destinationBuilder_.getMessageOrBuilder();
} else {
return destination_ == null ?
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.getDefaultInstance() : destination_;
}
}
/**
*
* Location for delivery, if applicable.
*
*
* .opencannabis.commerce.DeliveryDestination destination = 6 [(.gen_bq_schema.description) = "Location for delivery, if applicable."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder>
getDestinationFieldBuilder() {
if (destinationBuilder_ == null) {
destinationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestination.Builder, io.opencannabis.schema.commerce.OrderDelivery.DeliveryDestinationOrBuilder>(
getDestination(),
getParentForChildren(),
isClean());
destination_ = null;
}
return destinationBuilder_;
}
private java.lang.Object notes_ = "";
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public Builder setNotes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
notes_ = value;
onChanged();
return this;
}
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public Builder clearNotes() {
notes_ = getDefaultInstance().getNotes();
onChanged();
return this;
}
/**
*
* User-provided notes or questions, if any.
*
*
* string notes = 7 [(.gen_bq_schema.ignore) = true];
*/
public Builder setNotesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
notes_ = value;
onChanged();
return this;
}
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder> itemBuilder_;
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.Item getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder setItem(
int index, io.opencannabis.schema.commerce.OrderItem.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder setItem(
int index, io.opencannabis.schema.commerce.OrderItem.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder addItem(io.opencannabis.schema.commerce.OrderItem.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder addItem(
int index, io.opencannabis.schema.commerce.OrderItem.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder addItem(
io.opencannabis.schema.commerce.OrderItem.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder addItem(
int index, io.opencannabis.schema.commerce.OrderItem.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder addAllItem(
java.lang.Iterable extends io.opencannabis.schema.commerce.OrderItem.Item> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.Item.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List extends io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.Item.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.OrderItem.Item.getDefaultInstance());
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.OrderItem.Item.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.OrderItem.Item.getDefaultInstance());
}
/**
*
* Items being ordered.
*
*
* repeated .opencannabis.commerce.Item item = 8 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>(
item_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private java.util.List actionLog_ =
java.util.Collections.emptyList();
private void ensureActionLogIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
actionLog_ = new java.util.ArrayList(actionLog_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder> actionLogBuilder_;
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List getActionLogList() {
if (actionLogBuilder_ == null) {
return java.util.Collections.unmodifiableList(actionLog_);
} else {
return actionLogBuilder_.getMessageList();
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public int getActionLogCount() {
if (actionLogBuilder_ == null) {
return actionLog_.size();
} else {
return actionLogBuilder_.getCount();
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin getActionLog(int index) {
if (actionLogBuilder_ == null) {
return actionLog_.get(index);
} else {
return actionLogBuilder_.getMessage(index);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder setActionLog(
int index, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin value) {
if (actionLogBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionLogIsMutable();
actionLog_.set(index, value);
onChanged();
} else {
actionLogBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder setActionLog(
int index, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder builderForValue) {
if (actionLogBuilder_ == null) {
ensureActionLogIsMutable();
actionLog_.set(index, builderForValue.build());
onChanged();
} else {
actionLogBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder addActionLog(io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin value) {
if (actionLogBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionLogIsMutable();
actionLog_.add(value);
onChanged();
} else {
actionLogBuilder_.addMessage(value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder addActionLog(
int index, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin value) {
if (actionLogBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionLogIsMutable();
actionLog_.add(index, value);
onChanged();
} else {
actionLogBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder addActionLog(
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder builderForValue) {
if (actionLogBuilder_ == null) {
ensureActionLogIsMutable();
actionLog_.add(builderForValue.build());
onChanged();
} else {
actionLogBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder addActionLog(
int index, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder builderForValue) {
if (actionLogBuilder_ == null) {
ensureActionLogIsMutable();
actionLog_.add(index, builderForValue.build());
onChanged();
} else {
actionLogBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder addAllActionLog(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin> values) {
if (actionLogBuilder_ == null) {
ensureActionLogIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, actionLog_);
onChanged();
} else {
actionLogBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder clearActionLog() {
if (actionLogBuilder_ == null) {
actionLog_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
actionLogBuilder_.clear();
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public Builder removeActionLog(int index) {
if (actionLogBuilder_ == null) {
ensureActionLogIsMutable();
actionLog_.remove(index);
onChanged();
} else {
actionLogBuilder_.remove(index);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder getActionLogBuilder(
int index) {
return getActionLogFieldBuilder().getBuilder(index);
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder getActionLogOrBuilder(
int index) {
if (actionLogBuilder_ == null) {
return actionLog_.get(index); } else {
return actionLogBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder>
getActionLogOrBuilderList() {
if (actionLogBuilder_ != null) {
return actionLogBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(actionLog_);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder addActionLogBuilder() {
return getActionLogFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.getDefaultInstance());
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder addActionLogBuilder(
int index) {
return getActionLogFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.getDefaultInstance());
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.StatusCheckin action_log = 9 [(.gen_bq_schema.ignore) = true];
*/
public java.util.List
getActionLogBuilderList() {
return getActionLogFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder>
getActionLogFieldBuilder() {
if (actionLogBuilder_ == null) {
actionLogBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckin.Builder, io.opencannabis.schema.commerce.CommercialOrder.StatusCheckinOrBuilder>(
actionLog_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
actionLog_ = null;
}
return actionLogBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant createdAt_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> createdAtBuilder_;
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public boolean hasCreatedAt() {
return createdAtBuilder_ != null || createdAt_ != null;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreatedAt() {
if (createdAtBuilder_ == null) {
return createdAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : createdAt_;
} else {
return createdAtBuilder_.getMessage();
}
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public Builder setCreatedAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createdAt_ = value;
onChanged();
} else {
createdAtBuilder_.setMessage(value);
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public Builder setCreatedAt(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (createdAtBuilder_ == null) {
createdAt_ = builderForValue.build();
onChanged();
} else {
createdAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public Builder mergeCreatedAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdAtBuilder_ == null) {
if (createdAt_ != null) {
createdAt_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(createdAt_).mergeFrom(value).buildPartial();
} else {
createdAt_ = value;
}
onChanged();
} else {
createdAtBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public Builder clearCreatedAt() {
if (createdAtBuilder_ == null) {
createdAt_ = null;
onChanged();
} else {
createdAt_ = null;
createdAtBuilder_ = null;
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getCreatedAtBuilder() {
onChanged();
return getCreatedAtFieldBuilder().getBuilder();
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedAtOrBuilder() {
if (createdAtBuilder_ != null) {
return createdAtBuilder_.getMessageOrBuilder();
} else {
return createdAt_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : createdAt_;
}
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant created_at = 10 [(.gen_bq_schema.require) = true, (.gen_bq_schema.description) = "When this order was created."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getCreatedAtFieldBuilder() {
if (createdAtBuilder_ == null) {
createdAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getCreatedAt(),
getParentForChildren(),
isClean());
createdAt_ = null;
}
return createdAtBuilder_;
}
private double subtotal_ ;
/**
*
* Order subtotal.
*
*
* double subtotal = 11 [(.gen_bq_schema.description) = "Order subtotal."];
*/
public double getSubtotal() {
return subtotal_;
}
/**
*
* Order subtotal.
*
*
* double subtotal = 11 [(.gen_bq_schema.description) = "Order subtotal."];
*/
public Builder setSubtotal(double value) {
subtotal_ = value;
onChanged();
return this;
}
/**
*
* Order subtotal.
*
*
* double subtotal = 11 [(.gen_bq_schema.description) = "Order subtotal."];
*/
public Builder clearSubtotal() {
subtotal_ = 0D;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant updatedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> updatedAtBuilder_;
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public boolean hasUpdatedAt() {
return updatedAtBuilder_ != null || updatedAt_ != null;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getUpdatedAt() {
if (updatedAtBuilder_ == null) {
return updatedAt_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : updatedAt_;
} else {
return updatedAtBuilder_.getMessage();
}
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public Builder setUpdatedAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (updatedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updatedAt_ = value;
onChanged();
} else {
updatedAtBuilder_.setMessage(value);
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public Builder setUpdatedAt(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (updatedAtBuilder_ == null) {
updatedAt_ = builderForValue.build();
onChanged();
} else {
updatedAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public Builder mergeUpdatedAt(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (updatedAtBuilder_ == null) {
if (updatedAt_ != null) {
updatedAt_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(updatedAt_).mergeFrom(value).buildPartial();
} else {
updatedAt_ = value;
}
onChanged();
} else {
updatedAtBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public Builder clearUpdatedAt() {
if (updatedAtBuilder_ == null) {
updatedAt_ = null;
onChanged();
} else {
updatedAt_ = null;
updatedAtBuilder_ = null;
}
return this;
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getUpdatedAtBuilder() {
onChanged();
return getUpdatedAtFieldBuilder().getBuilder();
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getUpdatedAtOrBuilder() {
if (updatedAtBuilder_ != null) {
return updatedAtBuilder_.getMessageOrBuilder();
} else {
return updatedAt_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : updatedAt_;
}
}
/**
*
* When this order was created.
*
*
* .opencannabis.temporal.Instant updated_at = 12 [(.gen_bq_schema.ignore) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getUpdatedAtFieldBuilder() {
if (updatedAtBuilder_ == null) {
updatedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getUpdatedAt(),
getParentForChildren(),
isClean());
updatedAt_ = null;
}
return updatedAtBuilder_;
}
private java.lang.Object sid_ = "";
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public java.lang.String getSid() {
java.lang.Object ref = sid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public com.google.protobuf.ByteString
getSidBytes() {
java.lang.Object ref = sid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public Builder setSid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sid_ = value;
onChanged();
return this;
}
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public Builder clearSid() {
sid_ = getDefaultInstance().getSid();
onChanged();
return this;
}
/**
*
* Session ID that was active when this order was submitted.
*
*
* string sid = 13 [(.gen_bq_schema.description) = "Session ID that was active when this order was submitted."];
*/
public Builder setSidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sid_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialOrder.OrderPayment payment_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment, io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder> paymentBuilder_;
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public boolean hasPayment() {
return paymentBuilder_ != null || payment_ != null;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment getPayment() {
if (paymentBuilder_ == null) {
return payment_ == null ? io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.getDefaultInstance() : payment_;
} else {
return paymentBuilder_.getMessage();
}
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public Builder setPayment(io.opencannabis.schema.commerce.CommercialOrder.OrderPayment value) {
if (paymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
payment_ = value;
onChanged();
} else {
paymentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public Builder setPayment(
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder builderForValue) {
if (paymentBuilder_ == null) {
payment_ = builderForValue.build();
onChanged();
} else {
paymentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public Builder mergePayment(io.opencannabis.schema.commerce.CommercialOrder.OrderPayment value) {
if (paymentBuilder_ == null) {
if (payment_ != null) {
payment_ =
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.newBuilder(payment_).mergeFrom(value).buildPartial();
} else {
payment_ = value;
}
onChanged();
} else {
paymentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public Builder clearPayment() {
if (paymentBuilder_ == null) {
payment_ = null;
onChanged();
} else {
payment_ = null;
paymentBuilder_ = null;
}
return this;
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder getPaymentBuilder() {
onChanged();
return getPaymentFieldBuilder().getBuilder();
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
public io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder getPaymentOrBuilder() {
if (paymentBuilder_ != null) {
return paymentBuilder_.getMessageOrBuilder();
} else {
return payment_ == null ?
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.getDefaultInstance() : payment_;
}
}
/**
*
* Payment information/metadata for this order, if applicable.
*
*
* .opencannabis.commerce.OrderPayment payment = 14 [(.gen_bq_schema.description) = "Payment information/metadata for this order, if applicable."];
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment, io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder>
getPaymentFieldBuilder() {
if (paymentBuilder_ == null) {
paymentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialOrder.OrderPayment, io.opencannabis.schema.commerce.CommercialOrder.OrderPayment.Builder, io.opencannabis.schema.commerce.CommercialOrder.OrderPaymentOrBuilder>(
getPayment(),
getParentForChildren(),
isClean());
payment_ = null;
}
return paymentBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Order)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Order)
private static final io.opencannabis.schema.commerce.CommercialOrder.Order DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialOrder.Order();
}
public static io.opencannabis.schema.commerce.CommercialOrder.Order getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Order parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Order(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialOrder.Order getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_OrderScheduling_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_OrderScheduling_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_OrderPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_OrderPayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_StatusCheckin_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_StatusCheckin_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_OrderKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_OrderKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Order_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Order_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024commerce/Order.proto\022\025opencannabis.com" +
"merce\032\016bq_field.proto\032\023commerce/Item.pro" +
"to\032\027commerce/Delivery.proto\032\027commerce/Cu" +
"stomer.proto\032\037commerce/payments/Payment." +
"proto\032\026temporal/Instant.proto\"\343\001\n\017OrderS" +
"cheduling\022q\n\nscheduling\030\001 \001(\0162%.opencann" +
"abis.commerce.SchedulingTypeB6\360?\001\212@0Sche" +
"duling type, either \'ASAP\' or a target t" +
"ime.\022]\n\014desired_time\030\002 \001(\0132\036.opencannabi" +
"s.temporal.InstantB\'\212@$Desired delivery " +
"time, if specified.\"\302\002\n\014OrderPayment\022Z\n\006" +
"status\030\001 \001(\0162$.opencannabis.commerce.Pay" +
"mentStatusB$\212@!Status of payment for thi" +
"s order.\022^\n\006method\030\002 \001(\0162$.opencannabis." +
"commerce.PaymentMethodB(\212@%Method of pay" +
"ment used on this order.\0224\n\003tax\030\003 \001(\001B\'\212" +
"@$Amount of tax added to the subtotal.\022@" +
"\n\004paid\030\004 \001(\001B2\212@/Amount the user has pai" +
"d so far for this order.\"\205\001\n\rStatusCheck" +
"in\0222\n\006status\030\001 \001(\0162\".opencannabis.commer" +
"ce.OrderStatus\022/\n\007instant\030\002 \001(\0132\036.openca" +
"nnabis.temporal.Instant\022\017\n\007message\030\003 \001(\t" +
"\"J\n\010OrderKey\022>\n\002id\030\001 \001(\tB2\212@/Order ID, a" +
"ssigned by the server upon creation.\"\267\010\n" +
"\005Order\022b\n\002id\030\001 \001(\tBV\360?\001\212@PID assigned to" +
" the order by the server, and potentiall" +
"y nominated by the client.\022j\n\004type\030\002 \001(\016" +
"2 .opencannabis.commerce.OrderTypeB:\360?\001\212" +
"@4Type of order requested - either PICKU" +
"P or DELIVERY.\0227\n\006status\030\003 \001(\0162\".opencan" +
"nabis.commerce.OrderStatusB\003\200@\001\022\\\n\010custo" +
"mer\030\004 \001(\0132\037.opencannabis.commerce.Custom" +
"erB)\360?\001\212@#Customer that submitted this o" +
"rder.\022a\n\nscheduling\030\005 \001(\0132&.opencannabis" +
".commerce.OrderSchedulingB%\360?\001\212@\037Schedul" +
"ing spec for this order.\022i\n\013destination\030" +
"\006 \001(\0132*.opencannabis.commerce.DeliveryDe" +
"stinationB(\212@%Location for delivery, if " +
"applicable.\022\022\n\005notes\030\007 \001(\tB\003\200@\001\022.\n\004item\030" +
"\010 \003(\0132\033.opencannabis.commerce.ItemB\003\200@\001\022" +
"=\n\naction_log\030\t \003(\0132$.opencannabis.comme" +
"rce.StatusCheckinB\003\200@\001\022V\n\ncreated_at\030\n \001" +
"(\0132\036.opencannabis.temporal.InstantB\"\360?\001\212" +
"@\034When this order was created.\022$\n\010subtot" +
"al\030\013 \001(\001B\022\212@\017Order subtotal.\0227\n\nupdated_" +
"at\030\014 \001(\0132\036.opencannabis.temporal.Instant" +
"B\003\200@\001\022I\n\003sid\030\r \001(\tB<\212@9Session ID that w" +
"as active when this order was submitted." +
"\022t\n\007payment\030\016 \001(\0132#.opencannabis.commerc" +
"e.OrderPaymentB>\212@;Payment information/m" +
"etadata for this order, if applicable.*%" +
"\n\tOrderType\022\n\n\006PICKUP\020\000\022\014\n\010DELIVERY\020\001*%\n" +
"\016SchedulingType\022\010\n\004ASAP\020\000\022\t\n\005TIMED\020\001*a\n\013" +
"OrderStatus\022\013\n\007PENDING\020\000\022\014\n\010APPROVED\020\001\022\014" +
"\n\010REJECTED\020\002\022\014\n\010ASSIGNED\020\003\022\014\n\010EN_ROUTE\020\004" +
"\022\r\n\tFULFILLED\020\005B<\n\037io.opencannabis.schem" +
"a.commerceB\017CommercialOrderH\001P\000\242\002\003OCSb\006p" +
"roto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
gen_bq_schema.BqField.getDescriptor(),
io.opencannabis.schema.commerce.OrderItem.getDescriptor(),
io.opencannabis.schema.commerce.OrderDelivery.getDescriptor(),
io.opencannabis.schema.commerce.OrderCustomer.getDescriptor(),
io.opencannabis.schema.commerce.Payments.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
}, assigner);
internal_static_opencannabis_commerce_OrderScheduling_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_opencannabis_commerce_OrderScheduling_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_OrderScheduling_descriptor,
new java.lang.String[] { "Scheduling", "DesiredTime", });
internal_static_opencannabis_commerce_OrderPayment_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_opencannabis_commerce_OrderPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_OrderPayment_descriptor,
new java.lang.String[] { "Status", "Method", "Tax", "Paid", });
internal_static_opencannabis_commerce_StatusCheckin_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_opencannabis_commerce_StatusCheckin_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_StatusCheckin_descriptor,
new java.lang.String[] { "Status", "Instant", "Message", });
internal_static_opencannabis_commerce_OrderKey_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_opencannabis_commerce_OrderKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_OrderKey_descriptor,
new java.lang.String[] { "Id", });
internal_static_opencannabis_commerce_Order_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_opencannabis_commerce_Order_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Order_descriptor,
new java.lang.String[] { "Id", "Type", "Status", "Customer", "Scheduling", "Destination", "Notes", "Item", "ActionLog", "CreatedAt", "Subtotal", "UpdatedAt", "Sid", "Payment", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(gen_bq_schema.BqField.description);
registry.add(gen_bq_schema.BqField.ignore);
registry.add(gen_bq_schema.BqField.require);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
gen_bq_schema.BqField.getDescriptor();
io.opencannabis.schema.commerce.OrderItem.getDescriptor();
io.opencannabis.schema.commerce.OrderDelivery.getDescriptor();
io.opencannabis.schema.commerce.OrderCustomer.getDescriptor();
io.opencannabis.schema.commerce.Payments.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy