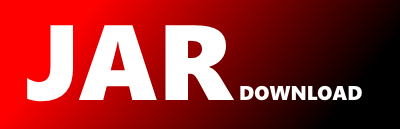
io.opencannabis.schema.commerce.CommercialPOS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas Co.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: pos/PointOfSale.proto
package io.opencannabis.schema.commerce;
public final class CommercialPOS {
private CommercialPOS() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Enumerates states that a point-of-sale session may take.
*
*
* Protobuf enum {@code bloombox.pos.SessionStatus}
*/
public enum SessionStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The session has been established, but no cash register session is open yet.
*
*
* ESTABLISHED = 0;
*/
ESTABLISHED(0),
/**
*
* This session is actively open.
*
*
* ACTIVE = 1;
*/
ACTIVE(1),
/**
*
* This session is paused temporarily.
*
*
* SUSPENDED = 2;
*/
SUSPENDED(2),
/**
*
* This session timed out/expired.
*
*
* EXPIRED = 3;
*/
EXPIRED(3),
/**
*
* This session was formally terminated.
*
*
* TERMINATED = 4;
*/
TERMINATED(4),
/**
*
* The connection has been lost for this session.
*
*
* LOST = 5;
*/
LOST(5),
UNRECOGNIZED(-1),
;
/**
*
* The session has been established, but no cash register session is open yet.
*
*
* ESTABLISHED = 0;
*/
public static final int ESTABLISHED_VALUE = 0;
/**
*
* This session is actively open.
*
*
* ACTIVE = 1;
*/
public static final int ACTIVE_VALUE = 1;
/**
*
* This session is paused temporarily.
*
*
* SUSPENDED = 2;
*/
public static final int SUSPENDED_VALUE = 2;
/**
*
* This session timed out/expired.
*
*
* EXPIRED = 3;
*/
public static final int EXPIRED_VALUE = 3;
/**
*
* This session was formally terminated.
*
*
* TERMINATED = 4;
*/
public static final int TERMINATED_VALUE = 4;
/**
*
* The connection has been lost for this session.
*
*
* LOST = 5;
*/
public static final int LOST_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SessionStatus valueOf(int value) {
return forNumber(value);
}
public static SessionStatus forNumber(int value) {
switch (value) {
case 0: return ESTABLISHED;
case 1: return ACTIVE;
case 2: return SUSPENDED;
case 3: return EXPIRED;
case 4: return TERMINATED;
case 5: return LOST;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SessionStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SessionStatus findValueByNumber(int number) {
return SessionStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.getDescriptor().getEnumTypes().get(0);
}
private static final SessionStatus[] VALUES = values();
public static SessionStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SessionStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.pos.SessionStatus)
}
/**
*
* Enumerates available point-of-sale device states.
*
*
* Protobuf enum {@code bloombox.pos.POSDeviceStatus}
*/
public enum POSDeviceStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Specifies a state where the POS device is not being used.
*
*
* IDLE = 0;
*/
IDLE(0),
/**
*
* Specifies a state where a session currently claims a POS device.
*
*
* CLAIMED = 1;
*/
CLAIMED(1),
UNRECOGNIZED(-1),
;
/**
*
* Specifies a state where the POS device is not being used.
*
*
* IDLE = 0;
*/
public static final int IDLE_VALUE = 0;
/**
*
* Specifies a state where a session currently claims a POS device.
*
*
* CLAIMED = 1;
*/
public static final int CLAIMED_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static POSDeviceStatus valueOf(int value) {
return forNumber(value);
}
public static POSDeviceStatus forNumber(int value) {
switch (value) {
case 0: return IDLE;
case 1: return CLAIMED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
POSDeviceStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public POSDeviceStatus findValueByNumber(int number) {
return POSDeviceStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.getDescriptor().getEnumTypes().get(1);
}
private static final POSDeviceStatus[] VALUES = values();
public static POSDeviceStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private POSDeviceStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.pos.POSDeviceStatus)
}
public interface POSHardwareOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.POSHardware)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
int getTypeValue();
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type getType();
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
boolean hasSupport();
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getSupport();
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder getSupportOrBuilder();
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
java.lang.String getVersion();
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
com.google.protobuf.ByteString
getVersionBytes();
}
/**
*
* Specifies information about the hardware powering a point-of-sale device.
*
*
* Protobuf type {@code bloombox.pos.POSHardware}
*/
public static final class POSHardware extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.POSHardware)
POSHardwareOrBuilder {
private static final long serialVersionUID = 0L;
// Use POSHardware.newBuilder() to construct.
private POSHardware(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private POSHardware() {
type_ = 0;
version_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private POSHardware(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 18: {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder subBuilder = null;
if (support_ != null) {
subBuilder = support_.toBuilder();
}
support_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(support_);
support_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
version_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.class, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder.class);
}
/**
*
* Enumerated, known types of point-of-sale hardware.
*
*
* Protobuf enum {@code bloombox.pos.POSHardware.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unspecified point-of-sale hardware.
*
*
* UNSPECIFIED = 0;
*/
UNSPECIFIED(0),
/**
*
* Bloombox Point-of-Sale.
*
*
* BLOOMBOX = 1;
*/
BLOOMBOX(1),
UNRECOGNIZED(-1),
;
/**
*
* Unspecified point-of-sale hardware.
*
*
* UNSPECIFIED = 0;
*/
public static final int UNSPECIFIED_VALUE = 0;
/**
*
* Bloombox Point-of-Sale.
*
*
* BLOOMBOX = 1;
*/
public static final int BLOOMBOX_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 0: return UNSPECIFIED;
case 1: return BLOOMBOX;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.pos.POSHardware.Type)
}
/**
*
* Specifies hardware features supported by the point-of-sale system.
*
*
* Protobuf enum {@code bloombox.pos.POSHardware.Feature}
*/
public enum Feature
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The system has a barcode printer.
*
*
* PRINTER = 0;
*/
PRINTER(0),
/**
*
* The system has a barcode scanner.
*
*
* BCS = 1;
*/
BCS(1),
/**
*
* The system has a magnetic stripe reader.
*
*
* MSR = 2;
*/
MSR(2),
/**
*
* The system has a Bluetooth Low Energy (BLE) antenna.
*
*
* BLE = 3;
*/
BLE(3),
/**
*
* The system has a scale attached.
*
*
* SCALE = 4;
*/
SCALE(4),
/**
*
* The system has a label printer attached.
*
*
* LABELLER = 5;
*/
LABELLER(5),
UNRECOGNIZED(-1),
;
/**
*
* The system has a barcode printer.
*
*
* PRINTER = 0;
*/
public static final int PRINTER_VALUE = 0;
/**
*
* The system has a barcode scanner.
*
*
* BCS = 1;
*/
public static final int BCS_VALUE = 1;
/**
*
* The system has a magnetic stripe reader.
*
*
* MSR = 2;
*/
public static final int MSR_VALUE = 2;
/**
*
* The system has a Bluetooth Low Energy (BLE) antenna.
*
*
* BLE = 3;
*/
public static final int BLE_VALUE = 3;
/**
*
* The system has a scale attached.
*
*
* SCALE = 4;
*/
public static final int SCALE_VALUE = 4;
/**
*
* The system has a label printer attached.
*
*
* LABELLER = 5;
*/
public static final int LABELLER_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Feature valueOf(int value) {
return forNumber(value);
}
public static Feature forNumber(int value) {
switch (value) {
case 0: return PRINTER;
case 1: return BCS;
case 2: return MSR;
case 3: return BLE;
case 4: return SCALE;
case 5: return LABELLER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Feature> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Feature findValueByNumber(int number) {
return Feature.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDescriptor().getEnumTypes().get(1);
}
private static final Feature[] VALUES = values();
public static Feature valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Feature(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bloombox.pos.POSHardware.Feature)
}
public interface FeaturesOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.POSHardware.Features)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
java.util.List getFeatureList();
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
int getFeatureCount();
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature getFeature(int index);
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
java.util.List
getFeatureValueList();
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
int getFeatureValue(int index);
/**
*
* Number of cash drawers supported by the hardware.
*
*
* uint32 drawers = 2;
*/
int getDrawers();
}
/**
*
* Specifies the features available on a POS device.
*
*
* Protobuf type {@code bloombox.pos.POSHardware.Features}
*/
public static final class Features extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.POSHardware.Features)
FeaturesOrBuilder {
private static final long serialVersionUID = 0L;
// Use Features.newBuilder() to construct.
private Features(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Features() {
feature_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Features(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
feature_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
feature_.add(rawValue);
break;
}
case 10: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
feature_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
feature_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 16: {
drawers_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
feature_ = java.util.Collections.unmodifiableList(feature_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_Features_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_Features_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.class, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder.class);
}
private int bitField0_;
public static final int FEATURE_FIELD_NUMBER = 1;
private java.util.List feature_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature> feature_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature>() {
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature result = io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature.valueOf(from);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature.UNRECOGNIZED : result;
}
};
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public java.util.List getFeatureList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature>(feature_, feature_converter_);
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public int getFeatureCount() {
return feature_.size();
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature getFeature(int index) {
return feature_converter_.convert(feature_.get(index));
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public java.util.List
getFeatureValueList() {
return feature_;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public int getFeatureValue(int index) {
return feature_.get(index);
}
private int featureMemoizedSerializedSize;
public static final int DRAWERS_FIELD_NUMBER = 2;
private int drawers_;
/**
*
* Number of cash drawers supported by the hardware.
*
*
* uint32 drawers = 2;
*/
public int getDrawers() {
return drawers_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getFeatureList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(featureMemoizedSerializedSize);
}
for (int i = 0; i < feature_.size(); i++) {
output.writeEnumNoTag(feature_.get(i));
}
if (drawers_ != 0) {
output.writeUInt32(2, drawers_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < feature_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(feature_.get(i));
}
size += dataSize;
if (!getFeatureList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}featureMemoizedSerializedSize = dataSize;
}
if (drawers_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, drawers_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features other = (io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features) obj;
if (!feature_.equals(other.feature_)) return false;
if (getDrawers()
!= other.getDrawers()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFeatureCount() > 0) {
hash = (37 * hash) + FEATURE_FIELD_NUMBER;
hash = (53 * hash) + feature_.hashCode();
}
hash = (37 * hash) + DRAWERS_FIELD_NUMBER;
hash = (53 * hash) + getDrawers();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the features available on a POS device.
*
*
* Protobuf type {@code bloombox.pos.POSHardware.Features}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.POSHardware.Features)
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_Features_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_Features_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.class, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
feature_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
drawers_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_Features_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features build() {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features result = new io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) != 0)) {
feature_ = java.util.Collections.unmodifiableList(feature_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.feature_ = feature_;
result.drawers_ = drawers_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.getDefaultInstance()) return this;
if (!other.feature_.isEmpty()) {
if (feature_.isEmpty()) {
feature_ = other.feature_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFeatureIsMutable();
feature_.addAll(other.feature_);
}
onChanged();
}
if (other.getDrawers() != 0) {
setDrawers(other.getDrawers());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List feature_ =
java.util.Collections.emptyList();
private void ensureFeatureIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
feature_ = new java.util.ArrayList(feature_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public java.util.List getFeatureList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature>(feature_, feature_converter_);
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public int getFeatureCount() {
return feature_.size();
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature getFeature(int index) {
return feature_converter_.convert(feature_.get(index));
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder setFeature(
int index, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature value) {
if (value == null) {
throw new NullPointerException();
}
ensureFeatureIsMutable();
feature_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder addFeature(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature value) {
if (value == null) {
throw new NullPointerException();
}
ensureFeatureIsMutable();
feature_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder addAllFeature(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature> values) {
ensureFeatureIsMutable();
for (io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Feature value : values) {
feature_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder clearFeature() {
feature_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public java.util.List
getFeatureValueList() {
return java.util.Collections.unmodifiableList(feature_);
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public int getFeatureValue(int index) {
return feature_.get(index);
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder setFeatureValue(
int index, int value) {
ensureFeatureIsMutable();
feature_.set(index, value);
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder addFeatureValue(int value) {
ensureFeatureIsMutable();
feature_.add(value);
onChanged();
return this;
}
/**
*
* Specifies features supported by the POS hardware.
*
*
* repeated .bloombox.pos.POSHardware.Feature feature = 1;
*/
public Builder addAllFeatureValue(
java.lang.Iterable values) {
ensureFeatureIsMutable();
for (int value : values) {
feature_.add(value);
}
onChanged();
return this;
}
private int drawers_ ;
/**
*
* Number of cash drawers supported by the hardware.
*
*
* uint32 drawers = 2;
*/
public int getDrawers() {
return drawers_;
}
/**
*
* Number of cash drawers supported by the hardware.
*
*
* uint32 drawers = 2;
*/
public Builder setDrawers(int value) {
drawers_ = value;
onChanged();
return this;
}
/**
*
* Number of cash drawers supported by the hardware.
*
*
* uint32 drawers = 2;
*/
public Builder clearDrawers() {
drawers_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.POSHardware.Features)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.POSHardware.Features)
private static final io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features();
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Features parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Features(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type getType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type result = io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.valueOf(type_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.UNRECOGNIZED : result;
}
public static final int SUPPORT_FIELD_NUMBER = 2;
private io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features support_;
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public boolean hasSupport() {
return support_ != null;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getSupport() {
return support_ == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.getDefaultInstance() : support_;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder getSupportOrBuilder() {
return getSupport();
}
public static final int VERSION_FIELD_NUMBER = 3;
private volatile java.lang.Object version_;
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.UNSPECIFIED.getNumber()) {
output.writeEnum(1, type_);
}
if (support_ != null) {
output.writeMessage(2, getSupport());
}
if (!getVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (support_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSupport());
}
if (!getVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.POSHardware)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.POSHardware other = (io.opencannabis.schema.commerce.CommercialPOS.POSHardware) obj;
if (type_ != other.type_) return false;
if (hasSupport() != other.hasSupport()) return false;
if (hasSupport()) {
if (!getSupport()
.equals(other.getSupport())) return false;
}
if (!getVersion()
.equals(other.getVersion())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasSupport()) {
hash = (37 * hash) + SUPPORT_FIELD_NUMBER;
hash = (53 * hash) + getSupport().hashCode();
}
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.POSHardware prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies information about the hardware powering a point-of-sale device.
*
*
* Protobuf type {@code bloombox.pos.POSHardware}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.POSHardware)
io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.class, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.POSHardware.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
if (supportBuilder_ == null) {
support_ = null;
} else {
support_ = null;
supportBuilder_ = null;
}
version_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_POSHardware_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware build() {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware result = new io.opencannabis.schema.commerce.CommercialPOS.POSHardware(this);
result.type_ = type_;
if (supportBuilder_ == null) {
result.support_ = support_;
} else {
result.support_ = supportBuilder_.build();
}
result.version_ = version_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.POSHardware) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.POSHardware)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.POSHardware other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasSupport()) {
mergeSupport(other.getSupport());
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.POSHardware) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int type_ = 0;
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public int getTypeValue() {
return type_;
}
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type getType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type result = io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.valueOf(type_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type.UNRECOGNIZED : result;
}
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public Builder setType(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Type value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the type of POS hardware.
*
*
* .bloombox.pos.POSHardware.Type type = 1;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features support_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder> supportBuilder_;
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public boolean hasSupport() {
return supportBuilder_ != null || support_ != null;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features getSupport() {
if (supportBuilder_ == null) {
return support_ == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.getDefaultInstance() : support_;
} else {
return supportBuilder_.getMessage();
}
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public Builder setSupport(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features value) {
if (supportBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
support_ = value;
onChanged();
} else {
supportBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public Builder setSupport(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder builderForValue) {
if (supportBuilder_ == null) {
support_ = builderForValue.build();
onChanged();
} else {
supportBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public Builder mergeSupport(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features value) {
if (supportBuilder_ == null) {
if (support_ != null) {
support_ =
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.newBuilder(support_).mergeFrom(value).buildPartial();
} else {
support_ = value;
}
onChanged();
} else {
supportBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public Builder clearSupport() {
if (supportBuilder_ == null) {
support_ = null;
onChanged();
} else {
support_ = null;
supportBuilder_ = null;
}
return this;
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder getSupportBuilder() {
onChanged();
return getSupportFieldBuilder().getBuilder();
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder getSupportOrBuilder() {
if (supportBuilder_ != null) {
return supportBuilder_.getMessageOrBuilder();
} else {
return support_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.getDefaultInstance() : support_;
}
}
/**
*
* Specifies the features supported by the POS hardware.
*
*
* .bloombox.pos.POSHardware.Features support = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder>
getSupportFieldBuilder() {
if (supportBuilder_ == null) {
supportBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Features.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.FeaturesOrBuilder>(
getSupport(),
getParentForChildren(),
isClean());
support_ = null;
}
return supportBuilder_;
}
private java.lang.Object version_ = "";
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
return this;
}
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
*
* Arbitrary version name string.
*
*
* string version = 3;
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
version_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.POSHardware)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.POSHardware)
private static final io.opencannabis.schema.commerce.CommercialPOS.POSHardware DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.POSHardware();
}
public static io.opencannabis.schema.commerce.CommercialPOS.POSHardware getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public POSHardware parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new POSHardware(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointOfSaleDeviceOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PointOfSaleDevice)
com.google.protobuf.MessageOrBuilder {
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
java.lang.String getUuid();
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUuidBytes();
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
java.lang.String getName();
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
int getStatusValue();
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus getStatus();
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
java.lang.String getClaim();
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
com.google.protobuf.ByteString
getClaimBytes();
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
boolean hasHardware();
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardware getHardware();
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder getHardwareOrBuilder();
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
boolean hasApp();
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication getApp();
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder getAppOrBuilder();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
java.util.List
getSessionList();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getSession(int index);
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
int getSessionCount();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder>
getSessionOrBuilderList();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder getSessionOrBuilder(
int index);
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
boolean hasState();
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState();
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder();
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
boolean hasSeen();
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
boolean hasCreated();
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated();
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder();
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
boolean hasModified();
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getModified();
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder();
}
/**
*
* Specifies a point of sale device, co-located at a retailer location, that is suitable for processing retail to
* consumer cannabis purchase transactions.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleDevice}
*/
public static final class PointOfSaleDevice extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PointOfSaleDevice)
PointOfSaleDeviceOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointOfSaleDevice.newBuilder() to construct.
private PointOfSaleDevice(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointOfSaleDevice() {
uuid_ = "";
name_ = "";
status_ = 0;
claim_ = "";
session_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PointOfSaleDevice(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
claim_ = s;
break;
}
case 42: {
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder subBuilder = null;
if (hardware_ != null) {
subBuilder = hardware_.toBuilder();
}
hardware_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.POSHardware.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(hardware_);
hardware_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder subBuilder = null;
if (app_ != null) {
subBuilder = app_.toBuilder();
}
app_ = input.readMessage(io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(app_);
app_ = subBuilder.buildPartial();
}
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) != 0)) {
session_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
session_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.parser(), extensionRegistry));
break;
}
case 66: {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder subBuilder = null;
if (state_ != null) {
subBuilder = state_.toBuilder();
}
state_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(state_);
state_ = subBuilder.buildPartial();
}
break;
}
case 74: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
case 786: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (created_ != null) {
subBuilder = created_.toBuilder();
}
created_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(created_);
created_ = subBuilder.buildPartial();
}
break;
}
case 794: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (modified_ != null) {
subBuilder = modified_.toBuilder();
}
modified_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(modified_);
modified_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) != 0)) {
session_ = java.util.Collections.unmodifiableList(session_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleDevice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleDevice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.Builder.class);
}
private int bitField0_;
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus result = io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.UNRECOGNIZED : result;
}
public static final int CLAIM_FIELD_NUMBER = 4;
private volatile java.lang.Object claim_;
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public java.lang.String getClaim() {
java.lang.Object ref = claim_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
claim_ = s;
return s;
}
}
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public com.google.protobuf.ByteString
getClaimBytes() {
java.lang.Object ref = claim_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
claim_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HARDWARE_FIELD_NUMBER = 5;
private io.opencannabis.schema.commerce.CommercialPOS.POSHardware hardware_;
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public boolean hasHardware() {
return hardware_ != null;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware getHardware() {
return hardware_ == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDefaultInstance() : hardware_;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder getHardwareOrBuilder() {
return getHardware();
}
public static final int APP_FIELD_NUMBER = 6;
private io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication app_;
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public boolean hasApp() {
return app_ != null;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication getApp() {
return app_ == null ? io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.getDefaultInstance() : app_;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder getAppOrBuilder() {
return getApp();
}
public static final int SESSION_FIELD_NUMBER = 7;
private java.util.List session_;
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public java.util.List getSessionList() {
return session_;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder>
getSessionOrBuilderList() {
return session_;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public int getSessionCount() {
return session_.size();
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getSession(int index) {
return session_.get(index);
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder getSessionOrBuilder(
int index) {
return session_.get(index);
}
public static final int STATE_FIELD_NUMBER = 8;
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState state_;
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public boolean hasState() {
return state_ != null;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState() {
return state_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder() {
return getState();
}
public static final int SEEN_FIELD_NUMBER = 9;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
public static final int CREATED_FIELD_NUMBER = 98;
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public boolean hasCreated() {
return created_ != null;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
return getCreated();
}
public static final int MODIFIED_FIELD_NUMBER = 99;
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public boolean hasModified() {
return modified_ != null;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
return getModified();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.IDLE.getNumber()) {
output.writeEnum(3, status_);
}
if (!getClaimBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, claim_);
}
if (hardware_ != null) {
output.writeMessage(5, getHardware());
}
if (app_ != null) {
output.writeMessage(6, getApp());
}
for (int i = 0; i < session_.size(); i++) {
output.writeMessage(7, session_.get(i));
}
if (state_ != null) {
output.writeMessage(8, getState());
}
if (seen_ != null) {
output.writeMessage(9, getSeen());
}
if (created_ != null) {
output.writeMessage(98, getCreated());
}
if (modified_ != null) {
output.writeMessage(99, getModified());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.IDLE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (!getClaimBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, claim_);
}
if (hardware_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getHardware());
}
if (app_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getApp());
}
for (int i = 0; i < session_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, session_.get(i));
}
if (state_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getState());
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getSeen());
}
if (created_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(98, getCreated());
}
if (modified_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(99, getModified());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice other = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (!getName()
.equals(other.getName())) return false;
if (status_ != other.status_) return false;
if (!getClaim()
.equals(other.getClaim())) return false;
if (hasHardware() != other.hasHardware()) return false;
if (hasHardware()) {
if (!getHardware()
.equals(other.getHardware())) return false;
}
if (hasApp() != other.hasApp()) return false;
if (hasApp()) {
if (!getApp()
.equals(other.getApp())) return false;
}
if (!getSessionList()
.equals(other.getSessionList())) return false;
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (!getState()
.equals(other.getState())) return false;
}
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (hasCreated() != other.hasCreated()) return false;
if (hasCreated()) {
if (!getCreated()
.equals(other.getCreated())) return false;
}
if (hasModified() != other.hasModified()) return false;
if (hasModified()) {
if (!getModified()
.equals(other.getModified())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + CLAIM_FIELD_NUMBER;
hash = (53 * hash) + getClaim().hashCode();
if (hasHardware()) {
hash = (37 * hash) + HARDWARE_FIELD_NUMBER;
hash = (53 * hash) + getHardware().hashCode();
}
if (hasApp()) {
hash = (37 * hash) + APP_FIELD_NUMBER;
hash = (53 * hash) + getApp().hashCode();
}
if (getSessionCount() > 0) {
hash = (37 * hash) + SESSION_FIELD_NUMBER;
hash = (53 * hash) + getSessionList().hashCode();
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
}
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
if (hasCreated()) {
hash = (37 * hash) + CREATED_FIELD_NUMBER;
hash = (53 * hash) + getCreated().hashCode();
}
if (hasModified()) {
hash = (37 * hash) + MODIFIED_FIELD_NUMBER;
hash = (53 * hash) + getModified().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a point of sale device, co-located at a retailer location, that is suitable for processing retail to
* consumer cannabis purchase transactions.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleDevice}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PointOfSaleDevice)
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDeviceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleDevice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleDevice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getSessionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
name_ = "";
status_ = 0;
claim_ = "";
if (hardwareBuilder_ == null) {
hardware_ = null;
} else {
hardware_ = null;
hardwareBuilder_ = null;
}
if (appBuilder_ == null) {
app_ = null;
} else {
app_ = null;
appBuilder_ = null;
}
if (sessionBuilder_ == null) {
session_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
sessionBuilder_.clear();
}
if (stateBuilder_ == null) {
state_ = null;
} else {
state_ = null;
stateBuilder_ = null;
}
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
if (createdBuilder_ == null) {
created_ = null;
} else {
created_ = null;
createdBuilder_ = null;
}
if (modifiedBuilder_ == null) {
modified_ = null;
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleDevice_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice build() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice result = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.uuid_ = uuid_;
result.name_ = name_;
result.status_ = status_;
result.claim_ = claim_;
if (hardwareBuilder_ == null) {
result.hardware_ = hardware_;
} else {
result.hardware_ = hardwareBuilder_.build();
}
if (appBuilder_ == null) {
result.app_ = app_;
} else {
result.app_ = appBuilder_.build();
}
if (sessionBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
session_ = java.util.Collections.unmodifiableList(session_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.session_ = session_;
} else {
result.session_ = sessionBuilder_.build();
}
if (stateBuilder_ == null) {
result.state_ = state_;
} else {
result.state_ = stateBuilder_.build();
}
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
if (createdBuilder_ == null) {
result.created_ = created_;
} else {
result.created_ = createdBuilder_.build();
}
if (modifiedBuilder_ == null) {
result.modified_ = modified_;
} else {
result.modified_ = modifiedBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.getClaim().isEmpty()) {
claim_ = other.claim_;
onChanged();
}
if (other.hasHardware()) {
mergeHardware(other.getHardware());
}
if (other.hasApp()) {
mergeApp(other.getApp());
}
if (sessionBuilder_ == null) {
if (!other.session_.isEmpty()) {
if (session_.isEmpty()) {
session_ = other.session_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureSessionIsMutable();
session_.addAll(other.session_);
}
onChanged();
}
} else {
if (!other.session_.isEmpty()) {
if (sessionBuilder_.isEmpty()) {
sessionBuilder_.dispose();
sessionBuilder_ = null;
session_ = other.session_;
bitField0_ = (bitField0_ & ~0x00000040);
sessionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSessionFieldBuilder() : null;
} else {
sessionBuilder_.addAllMessages(other.session_);
}
}
}
if (other.hasState()) {
mergeState(other.getState());
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
if (other.hasCreated()) {
mergeCreated(other.getCreated());
}
if (other.hasModified()) {
mergeModified(other.getModified());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uuid_ = "";
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* UUID assigned to, or gathered from, the device.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Label/human name for the point-of-sale device.
*
*
* string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus result = io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus.UNRECOGNIZED : result;
}
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialPOS.POSDeviceStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the current status of this POS device.
*
*
* .bloombox.pos.POSDeviceStatus status = 3;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object claim_ = "";
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public java.lang.String getClaim() {
java.lang.Object ref = claim_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
claim_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public com.google.protobuf.ByteString
getClaimBytes() {
java.lang.Object ref = claim_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
claim_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public Builder setClaim(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
claim_ = value;
onChanged();
return this;
}
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public Builder clearClaim() {
claim_ = getDefaultInstance().getClaim();
onChanged();
return this;
}
/**
*
* Specifies the string session ID currently claiming (or that was last to claim) this register.
*
*
* string claim = 4;
*/
public Builder setClaimBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
claim_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPOS.POSHardware hardware_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder> hardwareBuilder_;
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public boolean hasHardware() {
return hardwareBuilder_ != null || hardware_ != null;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware getHardware() {
if (hardwareBuilder_ == null) {
return hardware_ == null ? io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDefaultInstance() : hardware_;
} else {
return hardwareBuilder_.getMessage();
}
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public Builder setHardware(io.opencannabis.schema.commerce.CommercialPOS.POSHardware value) {
if (hardwareBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hardware_ = value;
onChanged();
} else {
hardwareBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public Builder setHardware(
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder builderForValue) {
if (hardwareBuilder_ == null) {
hardware_ = builderForValue.build();
onChanged();
} else {
hardwareBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public Builder mergeHardware(io.opencannabis.schema.commerce.CommercialPOS.POSHardware value) {
if (hardwareBuilder_ == null) {
if (hardware_ != null) {
hardware_ =
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.newBuilder(hardware_).mergeFrom(value).buildPartial();
} else {
hardware_ = value;
}
onChanged();
} else {
hardwareBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public Builder clearHardware() {
if (hardwareBuilder_ == null) {
hardware_ = null;
onChanged();
} else {
hardware_ = null;
hardwareBuilder_ = null;
}
return this;
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder getHardwareBuilder() {
onChanged();
return getHardwareFieldBuilder().getBuilder();
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder getHardwareOrBuilder() {
if (hardwareBuilder_ != null) {
return hardwareBuilder_.getMessageOrBuilder();
} else {
return hardware_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.POSHardware.getDefaultInstance() : hardware_;
}
}
/**
*
* Specifies information about the point-of-sale hardware in use.
*
*
* .bloombox.pos.POSHardware hardware = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder>
getHardwareFieldBuilder() {
if (hardwareBuilder_ == null) {
hardwareBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.POSHardware, io.opencannabis.schema.commerce.CommercialPOS.POSHardware.Builder, io.opencannabis.schema.commerce.CommercialPOS.POSHardwareOrBuilder>(
getHardware(),
getParentForChildren(),
isClean());
hardware_ = null;
}
return hardwareBuilder_;
}
private io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication app_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder> appBuilder_;
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public boolean hasApp() {
return appBuilder_ != null || app_ != null;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication getApp() {
if (appBuilder_ == null) {
return app_ == null ? io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.getDefaultInstance() : app_;
} else {
return appBuilder_.getMessage();
}
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public Builder setApp(io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication value) {
if (appBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
app_ = value;
onChanged();
} else {
appBuilder_.setMessage(value);
}
return this;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public Builder setApp(
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder builderForValue) {
if (appBuilder_ == null) {
app_ = builderForValue.build();
onChanged();
} else {
appBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public Builder mergeApp(io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication value) {
if (appBuilder_ == null) {
if (app_ != null) {
app_ =
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.newBuilder(app_).mergeFrom(value).buildPartial();
} else {
app_ = value;
}
onChanged();
} else {
appBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public Builder clearApp() {
if (appBuilder_ == null) {
app_ = null;
onChanged();
} else {
app_ = null;
appBuilder_ = null;
}
return this;
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder getAppBuilder() {
onChanged();
return getAppFieldBuilder().getBuilder();
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
public io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder getAppOrBuilder() {
if (appBuilder_ != null) {
return appBuilder_.getMessageOrBuilder();
} else {
return app_ == null ?
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.getDefaultInstance() : app_;
}
}
/**
*
* Information about the last-seen POS app in use.
*
*
* .bloombox.analytics.context.DeviceApplication app = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder>
getAppFieldBuilder() {
if (appBuilder_ == null) {
appBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplication.Builder, io.bloombox.schema.telemetry.context.ApplicationContext.DeviceApplicationOrBuilder>(
getApp(),
getParentForChildren(),
isClean());
app_ = null;
}
return appBuilder_;
}
private java.util.List session_ =
java.util.Collections.emptyList();
private void ensureSessionIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
session_ = new java.util.ArrayList(session_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder> sessionBuilder_;
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public java.util.List getSessionList() {
if (sessionBuilder_ == null) {
return java.util.Collections.unmodifiableList(session_);
} else {
return sessionBuilder_.getMessageList();
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public int getSessionCount() {
if (sessionBuilder_ == null) {
return session_.size();
} else {
return sessionBuilder_.getCount();
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getSession(int index) {
if (sessionBuilder_ == null) {
return session_.get(index);
} else {
return sessionBuilder_.getMessage(index);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder setSession(
int index, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession value) {
if (sessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIsMutable();
session_.set(index, value);
onChanged();
} else {
sessionBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder setSession(
int index, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder builderForValue) {
if (sessionBuilder_ == null) {
ensureSessionIsMutable();
session_.set(index, builderForValue.build());
onChanged();
} else {
sessionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder addSession(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession value) {
if (sessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIsMutable();
session_.add(value);
onChanged();
} else {
sessionBuilder_.addMessage(value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder addSession(
int index, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession value) {
if (sessionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionIsMutable();
session_.add(index, value);
onChanged();
} else {
sessionBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder addSession(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder builderForValue) {
if (sessionBuilder_ == null) {
ensureSessionIsMutable();
session_.add(builderForValue.build());
onChanged();
} else {
sessionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder addSession(
int index, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder builderForValue) {
if (sessionBuilder_ == null) {
ensureSessionIsMutable();
session_.add(index, builderForValue.build());
onChanged();
} else {
sessionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder addAllSession(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession> values) {
if (sessionBuilder_ == null) {
ensureSessionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, session_);
onChanged();
} else {
sessionBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder clearSession() {
if (sessionBuilder_ == null) {
session_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
sessionBuilder_.clear();
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public Builder removeSession(int index) {
if (sessionBuilder_ == null) {
ensureSessionIsMutable();
session_.remove(index);
onChanged();
} else {
sessionBuilder_.remove(index);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder getSessionBuilder(
int index) {
return getSessionFieldBuilder().getBuilder(index);
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder getSessionOrBuilder(
int index) {
if (sessionBuilder_ == null) {
return session_.get(index); } else {
return sessionBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder>
getSessionOrBuilderList() {
if (sessionBuilder_ != null) {
return sessionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(session_);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder addSessionBuilder() {
return getSessionFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.getDefaultInstance());
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder addSessionBuilder(
int index) {
return getSessionFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.getDefaultInstance());
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PointOfSaleSession session = 7 [(.core.collection) = { ... }
*/
public java.util.List
getSessionBuilderList() {
return getSessionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder>
getSessionFieldBuilder() {
if (sessionBuilder_ == null) {
sessionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder>(
session_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
session_ = null;
}
return sessionBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState state_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder> stateBuilder_;
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public boolean hasState() {
return stateBuilder_ != null || state_ != null;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState() {
if (stateBuilder_ == null) {
return state_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
} else {
return stateBuilder_.getMessage();
}
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public Builder setState(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState value) {
if (stateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
} else {
stateBuilder_.setMessage(value);
}
return this;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public Builder setState(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder builderForValue) {
if (stateBuilder_ == null) {
state_ = builderForValue.build();
onChanged();
} else {
stateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public Builder mergeState(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState value) {
if (stateBuilder_ == null) {
if (state_ != null) {
state_ =
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.newBuilder(state_).mergeFrom(value).buildPartial();
} else {
state_ = value;
}
onChanged();
} else {
stateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public Builder clearState() {
if (stateBuilder_ == null) {
state_ = null;
onChanged();
} else {
state_ = null;
stateBuilder_ = null;
}
return this;
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder getStateBuilder() {
onChanged();
return getStateFieldBuilder().getBuilder();
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder() {
if (stateBuilder_ != null) {
return stateBuilder_.getMessageOrBuilder();
} else {
return state_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
}
}
/**
*
* Indicates the current state of the point-of-sale device, including the current till value.
*
*
* .bloombox.pos.PointOfSaleState state = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder>
getStateFieldBuilder() {
if (stateBuilder_ == null) {
stateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder>(
getState(),
getParentForChildren(),
isClean());
state_ = null;
}
return stateBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Last time this point-of-sale device was seen.
*
*
* .opencannabis.temporal.Instant seen = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> createdBuilder_;
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public boolean hasCreated() {
return createdBuilder_ != null || created_ != null;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
if (createdBuilder_ == null) {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
} else {
return createdBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public Builder setCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
created_ = value;
onChanged();
} else {
createdBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public Builder setCreated(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (createdBuilder_ == null) {
created_ = builderForValue.build();
onChanged();
} else {
createdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public Builder mergeCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (created_ != null) {
created_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(created_).mergeFrom(value).buildPartial();
} else {
created_ = value;
}
onChanged();
} else {
createdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public Builder clearCreated() {
if (createdBuilder_ == null) {
created_ = null;
onChanged();
} else {
created_ = null;
createdBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getCreatedBuilder() {
onChanged();
return getCreatedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
if (createdBuilder_ != null) {
return createdBuilder_.getMessageOrBuilder();
} else {
return created_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
}
/**
*
* Timestamp for when this device was created.
*
*
* .opencannabis.temporal.Instant created = 98;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getCreatedFieldBuilder() {
if (createdBuilder_ == null) {
createdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getCreated(),
getParentForChildren(),
isClean());
created_ = null;
}
return createdBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> modifiedBuilder_;
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public boolean hasModified() {
return modifiedBuilder_ != null || modified_ != null;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
if (modifiedBuilder_ == null) {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
} else {
return modifiedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public Builder setModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
modified_ = value;
onChanged();
} else {
modifiedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public Builder setModified(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (modifiedBuilder_ == null) {
modified_ = builderForValue.build();
onChanged();
} else {
modifiedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public Builder mergeModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (modified_ != null) {
modified_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(modified_).mergeFrom(value).buildPartial();
} else {
modified_ = value;
}
onChanged();
} else {
modifiedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public Builder clearModified() {
if (modifiedBuilder_ == null) {
modified_ = null;
onChanged();
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getModifiedBuilder() {
onChanged();
return getModifiedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
if (modifiedBuilder_ != null) {
return modifiedBuilder_.getMessageOrBuilder();
} else {
return modified_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
}
/**
*
* Timestamp for when this device was last modified.
*
*
* .opencannabis.temporal.Instant modified = 99;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getModifiedFieldBuilder() {
if (modifiedBuilder_ == null) {
modifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getModified(),
getParentForChildren(),
isClean());
modified_ = null;
}
return modifiedBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PointOfSaleDevice)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PointOfSaleDevice)
private static final io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointOfSaleDevice parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PointOfSaleDevice(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleDevice getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointOfSaleStateOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PointOfSaleState)
com.google.protobuf.MessageOrBuilder {
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
boolean hasOpen();
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getOpen();
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder getOpenOrBuilder();
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
java.util.List
getTransactionList();
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getTransaction(int index);
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
int getTransactionCount();
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>
getTransactionOrBuilderList();
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getTransactionOrBuilder(
int index);
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
boolean hasCurrent();
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getCurrent();
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getCurrentOrBuilder();
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
boolean hasClose();
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getClose();
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder getCloseOrBuilder();
}
/**
*
* Specifies a combined state payload, provided to the point-of-sale unit once a session changes status.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState}
*/
public static final class PointOfSaleState extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PointOfSaleState)
PointOfSaleStateOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointOfSaleState.newBuilder() to construct.
private PointOfSaleState(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointOfSaleState() {
transaction_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PointOfSaleState(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder subBuilder = null;
if (open_ != null) {
subBuilder = open_.toBuilder();
}
open_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(open_);
open_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
transaction_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
transaction_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.parser(), extensionRegistry));
break;
}
case 26: {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder subBuilder = null;
if (current_ != null) {
subBuilder = current_.toBuilder();
}
current_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(current_);
current_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder subBuilder = null;
if (close_ != null) {
subBuilder = close_.toBuilder();
}
close_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(close_);
close_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
transaction_ = java.util.Collections.unmodifiableList(transaction_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder.class);
}
public interface SessionOpenOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PointOfSaleState.SessionOpen)
com.google.protobuf.MessageOrBuilder {
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
boolean hasOpeningFloat();
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getOpeningFloat();
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getOpeningFloatOrBuilder();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
boolean hasOccurred();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder();
}
/**
*
* Message payload when a session has been opened.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState.SessionOpen}
*/
public static final class SessionOpen extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PointOfSaleState.SessionOpen)
SessionOpenOrBuilder {
private static final long serialVersionUID = 0L;
// Use SessionOpen.newBuilder() to construct.
private SessionOpen(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SessionOpen() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionOpen(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (openingFloat_ != null) {
subBuilder = openingFloat_.toBuilder();
}
openingFloat_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(openingFloat_);
openingFloat_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (occurred_ != null) {
subBuilder = occurred_.toBuilder();
}
occurred_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(occurred_);
occurred_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionOpen_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder.class);
}
public static final int OPENING_FLOAT_FIELD_NUMBER = 1;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue openingFloat_;
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public boolean hasOpeningFloat() {
return openingFloat_ != null;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getOpeningFloat() {
return openingFloat_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : openingFloat_;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getOpeningFloatOrBuilder() {
return getOpeningFloat();
}
public static final int OCCURRED_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public boolean hasOccurred() {
return occurred_ != null;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
return getOccurred();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (openingFloat_ != null) {
output.writeMessage(1, getOpeningFloat());
}
if (occurred_ != null) {
output.writeMessage(2, getOccurred());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (openingFloat_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getOpeningFloat());
}
if (occurred_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getOccurred());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen other = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen) obj;
if (hasOpeningFloat() != other.hasOpeningFloat()) return false;
if (hasOpeningFloat()) {
if (!getOpeningFloat()
.equals(other.getOpeningFloat())) return false;
}
if (hasOccurred() != other.hasOccurred()) return false;
if (hasOccurred()) {
if (!getOccurred()
.equals(other.getOccurred())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasOpeningFloat()) {
hash = (37 * hash) + OPENING_FLOAT_FIELD_NUMBER;
hash = (53 * hash) + getOpeningFloat().hashCode();
}
if (hasOccurred()) {
hash = (37 * hash) + OCCURRED_FIELD_NUMBER;
hash = (53 * hash) + getOccurred().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Message payload when a session has been opened.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState.SessionOpen}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PointOfSaleState.SessionOpen)
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionOpen_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (openingFloatBuilder_ == null) {
openingFloat_ = null;
} else {
openingFloat_ = null;
openingFloatBuilder_ = null;
}
if (occurredBuilder_ == null) {
occurred_ = null;
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen build() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen result = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen(this);
if (openingFloatBuilder_ == null) {
result.openingFloat_ = openingFloat_;
} else {
result.openingFloat_ = openingFloatBuilder_.build();
}
if (occurredBuilder_ == null) {
result.occurred_ = occurred_;
} else {
result.occurred_ = occurredBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.getDefaultInstance()) return this;
if (other.hasOpeningFloat()) {
mergeOpeningFloat(other.getOpeningFloat());
}
if (other.hasOccurred()) {
mergeOccurred(other.getOccurred());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue openingFloat_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> openingFloatBuilder_;
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public boolean hasOpeningFloat() {
return openingFloatBuilder_ != null || openingFloat_ != null;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getOpeningFloat() {
if (openingFloatBuilder_ == null) {
return openingFloat_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : openingFloat_;
} else {
return openingFloatBuilder_.getMessage();
}
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public Builder setOpeningFloat(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (openingFloatBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
openingFloat_ = value;
onChanged();
} else {
openingFloatBuilder_.setMessage(value);
}
return this;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public Builder setOpeningFloat(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (openingFloatBuilder_ == null) {
openingFloat_ = builderForValue.build();
onChanged();
} else {
openingFloatBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public Builder mergeOpeningFloat(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (openingFloatBuilder_ == null) {
if (openingFloat_ != null) {
openingFloat_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(openingFloat_).mergeFrom(value).buildPartial();
} else {
openingFloat_ = value;
}
onChanged();
} else {
openingFloatBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public Builder clearOpeningFloat() {
if (openingFloatBuilder_ == null) {
openingFloat_ = null;
onChanged();
} else {
openingFloat_ = null;
openingFloatBuilder_ = null;
}
return this;
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getOpeningFloatBuilder() {
onChanged();
return getOpeningFloatFieldBuilder().getBuilder();
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getOpeningFloatOrBuilder() {
if (openingFloatBuilder_ != null) {
return openingFloatBuilder_.getMessageOrBuilder();
} else {
return openingFloat_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : openingFloat_;
}
}
/**
*
* Opening float value for the cash register. This is the initial cash amount used for change, etc.
*
*
* .opencannabis.commerce.CurrencyValue opening_float = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getOpeningFloatFieldBuilder() {
if (openingFloatBuilder_ == null) {
openingFloatBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getOpeningFloat(),
getParentForChildren(),
isClean());
openingFloat_ = null;
}
return openingFloatBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> occurredBuilder_;
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public boolean hasOccurred() {
return occurredBuilder_ != null || occurred_ != null;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
if (occurredBuilder_ == null) {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
} else {
return occurredBuilder_.getMessage();
}
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder setOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
occurred_ = value;
onChanged();
} else {
occurredBuilder_.setMessage(value);
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder setOccurred(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (occurredBuilder_ == null) {
occurred_ = builderForValue.build();
onChanged();
} else {
occurredBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder mergeOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (occurred_ != null) {
occurred_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(occurred_).mergeFrom(value).buildPartial();
} else {
occurred_ = value;
}
onChanged();
} else {
occurredBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder clearOccurred() {
if (occurredBuilder_ == null) {
occurred_ = null;
onChanged();
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getOccurredBuilder() {
onChanged();
return getOccurredFieldBuilder().getBuilder();
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
if (occurredBuilder_ != null) {
return occurredBuilder_.getMessageOrBuilder();
} else {
return occurred_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getOccurredFieldBuilder() {
if (occurredBuilder_ == null) {
occurredBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getOccurred(),
getParentForChildren(),
isClean());
occurred_ = null;
}
return occurredBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PointOfSaleState.SessionOpen)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PointOfSaleState.SessionOpen)
private static final io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SessionOpen parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionOpen(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SessionCloseOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PointOfSaleState.SessionClose)
com.google.protobuf.MessageOrBuilder {
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
boolean hasClosingCount();
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getClosingCount();
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getClosingCountOrBuilder();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
boolean hasOccurred();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred();
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder();
}
/**
*
* Message payload when a session has been closed.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState.SessionClose}
*/
public static final class SessionClose extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PointOfSaleState.SessionClose)
SessionCloseOrBuilder {
private static final long serialVersionUID = 0L;
// Use SessionClose.newBuilder() to construct.
private SessionClose(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SessionClose() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionClose(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (closingCount_ != null) {
subBuilder = closingCount_.toBuilder();
}
closingCount_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(closingCount_);
closingCount_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (occurred_ != null) {
subBuilder = occurred_.toBuilder();
}
occurred_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(occurred_);
occurred_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionClose_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder.class);
}
public static final int CLOSING_COUNT_FIELD_NUMBER = 1;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue closingCount_;
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public boolean hasClosingCount() {
return closingCount_ != null;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getClosingCount() {
return closingCount_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : closingCount_;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getClosingCountOrBuilder() {
return getClosingCount();
}
public static final int OCCURRED_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public boolean hasOccurred() {
return occurred_ != null;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
return getOccurred();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (closingCount_ != null) {
output.writeMessage(1, getClosingCount());
}
if (occurred_ != null) {
output.writeMessage(2, getOccurred());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (closingCount_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getClosingCount());
}
if (occurred_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getOccurred());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose other = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose) obj;
if (hasClosingCount() != other.hasClosingCount()) return false;
if (hasClosingCount()) {
if (!getClosingCount()
.equals(other.getClosingCount())) return false;
}
if (hasOccurred() != other.hasOccurred()) return false;
if (hasOccurred()) {
if (!getOccurred()
.equals(other.getOccurred())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasClosingCount()) {
hash = (37 * hash) + CLOSING_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getClosingCount().hashCode();
}
if (hasOccurred()) {
hash = (37 * hash) + OCCURRED_FIELD_NUMBER;
hash = (53 * hash) + getOccurred().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Message payload when a session has been closed.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState.SessionClose}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PointOfSaleState.SessionClose)
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionClose_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (closingCountBuilder_ == null) {
closingCount_ = null;
} else {
closingCount_ = null;
closingCountBuilder_ = null;
}
if (occurredBuilder_ == null) {
occurred_ = null;
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose build() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose result = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose(this);
if (closingCountBuilder_ == null) {
result.closingCount_ = closingCount_;
} else {
result.closingCount_ = closingCountBuilder_.build();
}
if (occurredBuilder_ == null) {
result.occurred_ = occurred_;
} else {
result.occurred_ = occurredBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.getDefaultInstance()) return this;
if (other.hasClosingCount()) {
mergeClosingCount(other.getClosingCount());
}
if (other.hasOccurred()) {
mergeOccurred(other.getOccurred());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue closingCount_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> closingCountBuilder_;
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public boolean hasClosingCount() {
return closingCountBuilder_ != null || closingCount_ != null;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getClosingCount() {
if (closingCountBuilder_ == null) {
return closingCount_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : closingCount_;
} else {
return closingCountBuilder_.getMessage();
}
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public Builder setClosingCount(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (closingCountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
closingCount_ = value;
onChanged();
} else {
closingCountBuilder_.setMessage(value);
}
return this;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public Builder setClosingCount(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (closingCountBuilder_ == null) {
closingCount_ = builderForValue.build();
onChanged();
} else {
closingCountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public Builder mergeClosingCount(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (closingCountBuilder_ == null) {
if (closingCount_ != null) {
closingCount_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(closingCount_).mergeFrom(value).buildPartial();
} else {
closingCount_ = value;
}
onChanged();
} else {
closingCountBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public Builder clearClosingCount() {
if (closingCountBuilder_ == null) {
closingCount_ = null;
onChanged();
} else {
closingCount_ = null;
closingCountBuilder_ = null;
}
return this;
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getClosingCountBuilder() {
onChanged();
return getClosingCountFieldBuilder().getBuilder();
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getClosingCountOrBuilder() {
if (closingCountBuilder_ != null) {
return closingCountBuilder_.getMessageOrBuilder();
} else {
return closingCount_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : closingCount_;
}
}
/**
*
* Closing cash value for the session. This is the total cash amount in the register upon closing.
*
*
* .opencannabis.commerce.CurrencyValue closing_count = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getClosingCountFieldBuilder() {
if (closingCountBuilder_ == null) {
closingCountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getClosingCount(),
getParentForChildren(),
isClean());
closingCount_ = null;
}
return closingCountBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant occurred_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> occurredBuilder_;
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public boolean hasOccurred() {
return occurredBuilder_ != null || occurred_ != null;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getOccurred() {
if (occurredBuilder_ == null) {
return occurred_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
} else {
return occurredBuilder_.getMessage();
}
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder setOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
occurred_ = value;
onChanged();
} else {
occurredBuilder_.setMessage(value);
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder setOccurred(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (occurredBuilder_ == null) {
occurred_ = builderForValue.build();
onChanged();
} else {
occurredBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder mergeOccurred(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (occurredBuilder_ == null) {
if (occurred_ != null) {
occurred_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(occurred_).mergeFrom(value).buildPartial();
} else {
occurred_ = value;
}
onChanged();
} else {
occurredBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public Builder clearOccurred() {
if (occurredBuilder_ == null) {
occurred_ = null;
onChanged();
} else {
occurred_ = null;
occurredBuilder_ = null;
}
return this;
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getOccurredBuilder() {
onChanged();
return getOccurredFieldBuilder().getBuilder();
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getOccurredOrBuilder() {
if (occurredBuilder_ != null) {
return occurredBuilder_.getMessageOrBuilder();
} else {
return occurred_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : occurred_;
}
}
/**
*
* Describes the moment the session was actually opened.
*
*
* .opencannabis.temporal.Instant occurred = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getOccurredFieldBuilder() {
if (occurredBuilder_ == null) {
occurredBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getOccurred(),
getParentForChildren(),
isClean());
occurred_ = null;
}
return occurredBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PointOfSaleState.SessionClose)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PointOfSaleState.SessionClose)
private static final io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SessionClose parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionClose(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int OPEN_FIELD_NUMBER = 1;
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen open_;
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public boolean hasOpen() {
return open_ != null;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getOpen() {
return open_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.getDefaultInstance() : open_;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder getOpenOrBuilder() {
return getOpen();
}
public static final int TRANSACTION_FIELD_NUMBER = 2;
private java.util.List transaction_;
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public java.util.List getTransactionList() {
return transaction_;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>
getTransactionOrBuilderList() {
return transaction_;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public int getTransactionCount() {
return transaction_.size();
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getTransaction(int index) {
return transaction_.get(index);
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getTransactionOrBuilder(
int index) {
return transaction_.get(index);
}
public static final int CURRENT_FIELD_NUMBER = 3;
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges current_;
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public boolean hasCurrent() {
return current_ != null;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getCurrent() {
return current_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : current_;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getCurrentOrBuilder() {
return getCurrent();
}
public static final int CLOSE_FIELD_NUMBER = 4;
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose close_;
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public boolean hasClose() {
return close_ != null;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getClose() {
return close_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.getDefaultInstance() : close_;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder getCloseOrBuilder() {
return getClose();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (open_ != null) {
output.writeMessage(1, getOpen());
}
for (int i = 0; i < transaction_.size(); i++) {
output.writeMessage(2, transaction_.get(i));
}
if (current_ != null) {
output.writeMessage(3, getCurrent());
}
if (close_ != null) {
output.writeMessage(4, getClose());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (open_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getOpen());
}
for (int i = 0; i < transaction_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, transaction_.get(i));
}
if (current_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCurrent());
}
if (close_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getClose());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState other = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState) obj;
if (hasOpen() != other.hasOpen()) return false;
if (hasOpen()) {
if (!getOpen()
.equals(other.getOpen())) return false;
}
if (!getTransactionList()
.equals(other.getTransactionList())) return false;
if (hasCurrent() != other.hasCurrent()) return false;
if (hasCurrent()) {
if (!getCurrent()
.equals(other.getCurrent())) return false;
}
if (hasClose() != other.hasClose()) return false;
if (hasClose()) {
if (!getClose()
.equals(other.getClose())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasOpen()) {
hash = (37 * hash) + OPEN_FIELD_NUMBER;
hash = (53 * hash) + getOpen().hashCode();
}
if (getTransactionCount() > 0) {
hash = (37 * hash) + TRANSACTION_FIELD_NUMBER;
hash = (53 * hash) + getTransactionList().hashCode();
}
if (hasCurrent()) {
hash = (37 * hash) + CURRENT_FIELD_NUMBER;
hash = (53 * hash) + getCurrent().hashCode();
}
if (hasClose()) {
hash = (37 * hash) + CLOSE_FIELD_NUMBER;
hash = (53 * hash) + getClose().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a combined state payload, provided to the point-of-sale unit once a session changes status.
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleState}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PointOfSaleState)
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTransactionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (openBuilder_ == null) {
open_ = null;
} else {
open_ = null;
openBuilder_ = null;
}
if (transactionBuilder_ == null) {
transaction_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
transactionBuilder_.clear();
}
if (currentBuilder_ == null) {
current_ = null;
} else {
current_ = null;
currentBuilder_ = null;
}
if (closeBuilder_ == null) {
close_ = null;
} else {
close_ = null;
closeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleState_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState build() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState result = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (openBuilder_ == null) {
result.open_ = open_;
} else {
result.open_ = openBuilder_.build();
}
if (transactionBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
transaction_ = java.util.Collections.unmodifiableList(transaction_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.transaction_ = transaction_;
} else {
result.transaction_ = transactionBuilder_.build();
}
if (currentBuilder_ == null) {
result.current_ = current_;
} else {
result.current_ = currentBuilder_.build();
}
if (closeBuilder_ == null) {
result.close_ = close_;
} else {
result.close_ = closeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance()) return this;
if (other.hasOpen()) {
mergeOpen(other.getOpen());
}
if (transactionBuilder_ == null) {
if (!other.transaction_.isEmpty()) {
if (transaction_.isEmpty()) {
transaction_ = other.transaction_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTransactionIsMutable();
transaction_.addAll(other.transaction_);
}
onChanged();
}
} else {
if (!other.transaction_.isEmpty()) {
if (transactionBuilder_.isEmpty()) {
transactionBuilder_.dispose();
transactionBuilder_ = null;
transaction_ = other.transaction_;
bitField0_ = (bitField0_ & ~0x00000002);
transactionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTransactionFieldBuilder() : null;
} else {
transactionBuilder_.addAllMessages(other.transaction_);
}
}
}
if (other.hasCurrent()) {
mergeCurrent(other.getCurrent());
}
if (other.hasClose()) {
mergeClose(other.getClose());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen open_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder> openBuilder_;
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public boolean hasOpen() {
return openBuilder_ != null || open_ != null;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen getOpen() {
if (openBuilder_ == null) {
return open_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.getDefaultInstance() : open_;
} else {
return openBuilder_.getMessage();
}
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public Builder setOpen(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen value) {
if (openBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
open_ = value;
onChanged();
} else {
openBuilder_.setMessage(value);
}
return this;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public Builder setOpen(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder builderForValue) {
if (openBuilder_ == null) {
open_ = builderForValue.build();
onChanged();
} else {
openBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public Builder mergeOpen(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen value) {
if (openBuilder_ == null) {
if (open_ != null) {
open_ =
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.newBuilder(open_).mergeFrom(value).buildPartial();
} else {
open_ = value;
}
onChanged();
} else {
openBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public Builder clearOpen() {
if (openBuilder_ == null) {
open_ = null;
onChanged();
} else {
open_ = null;
openBuilder_ = null;
}
return this;
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder getOpenBuilder() {
onChanged();
return getOpenFieldBuilder().getBuilder();
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder getOpenOrBuilder() {
if (openBuilder_ != null) {
return openBuilder_.getMessageOrBuilder();
} else {
return open_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.getDefaultInstance() : open_;
}
}
/**
*
* Describes the opening state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionOpen open = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder>
getOpenFieldBuilder() {
if (openBuilder_ == null) {
openBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpen.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionOpenOrBuilder>(
getOpen(),
getParentForChildren(),
isClean());
open_ = null;
}
return openBuilder_;
}
private java.util.List transaction_ =
java.util.Collections.emptyList();
private void ensureTransactionIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
transaction_ = new java.util.ArrayList(transaction_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder> transactionBuilder_;
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public java.util.List getTransactionList() {
if (transactionBuilder_ == null) {
return java.util.Collections.unmodifiableList(transaction_);
} else {
return transactionBuilder_.getMessageList();
}
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public int getTransactionCount() {
if (transactionBuilder_ == null) {
return transaction_.size();
} else {
return transactionBuilder_.getCount();
}
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getTransaction(int index) {
if (transactionBuilder_ == null) {
return transaction_.get(index);
} else {
return transactionBuilder_.getMessage(index);
}
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder setTransaction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey value) {
if (transactionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransactionIsMutable();
transaction_.set(index, value);
onChanged();
} else {
transactionBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder setTransaction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder builderForValue) {
if (transactionBuilder_ == null) {
ensureTransactionIsMutable();
transaction_.set(index, builderForValue.build());
onChanged();
} else {
transactionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder addTransaction(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey value) {
if (transactionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransactionIsMutable();
transaction_.add(value);
onChanged();
} else {
transactionBuilder_.addMessage(value);
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder addTransaction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey value) {
if (transactionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransactionIsMutable();
transaction_.add(index, value);
onChanged();
} else {
transactionBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder addTransaction(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder builderForValue) {
if (transactionBuilder_ == null) {
ensureTransactionIsMutable();
transaction_.add(builderForValue.build());
onChanged();
} else {
transactionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder addTransaction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder builderForValue) {
if (transactionBuilder_ == null) {
ensureTransactionIsMutable();
transaction_.add(index, builderForValue.build());
onChanged();
} else {
transactionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder addAllTransaction(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey> values) {
if (transactionBuilder_ == null) {
ensureTransactionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, transaction_);
onChanged();
} else {
transactionBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder clearTransaction() {
if (transactionBuilder_ == null) {
transaction_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
transactionBuilder_.clear();
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public Builder removeTransaction(int index) {
if (transactionBuilder_ == null) {
ensureTransactionIsMutable();
transaction_.remove(index);
onChanged();
} else {
transactionBuilder_.remove(index);
}
return this;
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder getTransactionBuilder(
int index) {
return getTransactionFieldBuilder().getBuilder(index);
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getTransactionOrBuilder(
int index) {
if (transactionBuilder_ == null) {
return transaction_.get(index); } else {
return transactionBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>
getTransactionOrBuilderList() {
if (transactionBuilder_ != null) {
return transactionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(transaction_);
}
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder addTransactionBuilder() {
return getTransactionFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance());
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder addTransactionBuilder(
int index) {
return getTransactionFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance());
}
/**
*
* Describes all transactions that occurred during a point of sale session.
*
*
* repeated .opencannabis.commerce.PurchaseKey transaction = 2;
*/
public java.util.List
getTransactionBuilderList() {
return getTransactionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>
getTransactionFieldBuilder() {
if (transactionBuilder_ == null) {
transactionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>(
transaction_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
transaction_ = null;
}
return transactionBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges current_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder> currentBuilder_;
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public boolean hasCurrent() {
return currentBuilder_ != null || current_ != null;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getCurrent() {
if (currentBuilder_ == null) {
return current_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : current_;
} else {
return currentBuilder_.getMessage();
}
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public Builder setCurrent(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (currentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
current_ = value;
onChanged();
} else {
currentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public Builder setCurrent(
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder builderForValue) {
if (currentBuilder_ == null) {
current_ = builderForValue.build();
onChanged();
} else {
currentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public Builder mergeCurrent(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (currentBuilder_ == null) {
if (current_ != null) {
current_ =
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.newBuilder(current_).mergeFrom(value).buildPartial();
} else {
current_ = value;
}
onChanged();
} else {
currentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public Builder clearCurrent() {
if (currentBuilder_ == null) {
current_ = null;
onChanged();
} else {
current_ = null;
currentBuilder_ = null;
}
return this;
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder getCurrentBuilder() {
onChanged();
return getCurrentFieldBuilder().getBuilder();
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getCurrentOrBuilder() {
if (currentBuilder_ != null) {
return currentBuilder_.getMessageOrBuilder();
} else {
return current_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : current_;
}
}
/**
*
* Specifies the current, complete, aggregated bill-of-charges state. Computed by the backend.
*
*
* .opencannabis.commerce.BillOfCharges current = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>
getCurrentFieldBuilder() {
if (currentBuilder_ == null) {
currentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>(
getCurrent(),
getParentForChildren(),
isClean());
current_ = null;
}
return currentBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose close_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder> closeBuilder_;
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public boolean hasClose() {
return closeBuilder_ != null || close_ != null;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose getClose() {
if (closeBuilder_ == null) {
return close_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.getDefaultInstance() : close_;
} else {
return closeBuilder_.getMessage();
}
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public Builder setClose(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose value) {
if (closeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
close_ = value;
onChanged();
} else {
closeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public Builder setClose(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder builderForValue) {
if (closeBuilder_ == null) {
close_ = builderForValue.build();
onChanged();
} else {
closeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public Builder mergeClose(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose value) {
if (closeBuilder_ == null) {
if (close_ != null) {
close_ =
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.newBuilder(close_).mergeFrom(value).buildPartial();
} else {
close_ = value;
}
onChanged();
} else {
closeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public Builder clearClose() {
if (closeBuilder_ == null) {
close_ = null;
onChanged();
} else {
close_ = null;
closeBuilder_ = null;
}
return this;
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder getCloseBuilder() {
onChanged();
return getCloseFieldBuilder().getBuilder();
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder getCloseOrBuilder() {
if (closeBuilder_ != null) {
return closeBuilder_.getMessageOrBuilder();
} else {
return close_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.getDefaultInstance() : close_;
}
}
/**
*
* Describes the closing state of a point of sale session.
*
*
* .bloombox.pos.PointOfSaleState.SessionClose close = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder>
getCloseFieldBuilder() {
if (closeBuilder_ == null) {
closeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionClose.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.SessionCloseOrBuilder>(
getClose(),
getParentForChildren(),
isClean());
close_ = null;
}
return closeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PointOfSaleState)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PointOfSaleState)
private static final io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointOfSaleState parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PointOfSaleState(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseTicketOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PurchaseTicket)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
boolean hasKey();
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getKey();
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getKeyOrBuilder();
/**
*
* Version or revision number for this purchase ticket.
*
*
* uint32 version = 2;
*/
int getVersion();
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
int getStatusValue();
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus();
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
java.lang.String getClaim();
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
com.google.protobuf.ByteString
getClaimBytes();
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
boolean hasFacilitator();
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getFacilitator();
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder getFacilitatorOrBuilder();
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
boolean hasCustomer();
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getCustomer();
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder getCustomerOrBuilder();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
boolean hasBill();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getBill();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getBillOrBuilder();
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
java.util.List
getItemList();
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getItem(int index);
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
int getItemCount();
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder>
getItemOrBuilderList();
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder getItemOrBuilder(
int index);
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
java.util.List
getPaymentList();
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment getPayment(int index);
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
int getPaymentCount();
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder>
getPaymentOrBuilderList();
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder getPaymentOrBuilder(
int index);
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
java.util.List
getActionList();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getAction(int index);
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
int getActionCount();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder>
getActionOrBuilderList();
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder getActionOrBuilder(
int index);
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
boolean hasTs();
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getTs();
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder getTsOrBuilder();
}
/**
*
* Specifies a record of a purchase made by a consumer at a retail cannabis location. Purchases are like orders, in that
* they are both consumer interactions with retailers in a commercial setting, but purchases are always made in-person
* using a point-of-sale device, and never online or from remote.
*
*
* Protobuf type {@code bloombox.pos.PurchaseTicket}
*/
public static final class PurchaseTicket extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PurchaseTicket)
PurchaseTicketOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseTicket.newBuilder() to construct.
private PurchaseTicket(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseTicket() {
status_ = 0;
claim_ = "";
item_ = java.util.Collections.emptyList();
payment_ = java.util.Collections.emptyList();
action_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseTicket(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 16: {
version_ = input.readUInt32();
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
claim_ = s;
break;
}
case 42: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder subBuilder = null;
if (facilitator_ != null) {
subBuilder = facilitator_.toBuilder();
}
facilitator_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(facilitator_);
facilitator_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder subBuilder = null;
if (customer_ != null) {
subBuilder = customer_.toBuilder();
}
customer_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(customer_);
customer_ = subBuilder.buildPartial();
}
break;
}
case 58: {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder subBuilder = null;
if (bill_ != null) {
subBuilder = bill_.toBuilder();
}
bill_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(bill_);
bill_ = subBuilder.buildPartial();
}
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) != 0)) {
item_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
item_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.parser(), extensionRegistry));
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) != 0)) {
payment_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
payment_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.parser(), extensionRegistry));
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000200) != 0)) {
action_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
action_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.parser(), extensionRegistry));
break;
}
case 90: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder subBuilder = null;
if (ts_ != null) {
subBuilder = ts_.toBuilder();
}
ts_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ts_);
ts_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
}
if (((mutable_bitField0_ & 0x00000100) != 0)) {
payment_ = java.util.Collections.unmodifiableList(payment_);
}
if (((mutable_bitField0_ & 0x00000200) != 0)) {
action_ = java.util.Collections.unmodifiableList(action_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PurchaseTicket_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PurchaseTicket_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.class, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey key_;
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getKey() {
return key_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance() : key_;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int VERSION_FIELD_NUMBER = 2;
private int version_;
/**
*
* Version or revision number for this purchase ticket.
*
*
* uint32 version = 2;
*/
public int getVersion() {
return version_;
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.UNRECOGNIZED : result;
}
public static final int CLAIM_FIELD_NUMBER = 4;
private volatile java.lang.Object claim_;
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public java.lang.String getClaim() {
java.lang.Object ref = claim_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
claim_ = s;
return s;
}
}
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public com.google.protobuf.ByteString
getClaimBytes() {
java.lang.Object ref = claim_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
claim_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FACILITATOR_FIELD_NUMBER = 5;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator facilitator_;
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public boolean hasFacilitator() {
return facilitator_ != null;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getFacilitator() {
return facilitator_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.getDefaultInstance() : facilitator_;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder getFacilitatorOrBuilder() {
return getFacilitator();
}
public static final int CUSTOMER_FIELD_NUMBER = 6;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer customer_;
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public boolean hasCustomer() {
return customer_ != null;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getCustomer() {
return customer_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.getDefaultInstance() : customer_;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder getCustomerOrBuilder() {
return getCustomer();
}
public static final int BILL_FIELD_NUMBER = 7;
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges bill_;
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public boolean hasBill() {
return bill_ != null;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getBill() {
return bill_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : bill_;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getBillOrBuilder() {
return getBill();
}
public static final int ITEM_FIELD_NUMBER = 8;
private java.util.List item_;
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public java.util.List getItemList() {
return item_;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public int getItemCount() {
return item_.size();
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getItem(int index) {
return item_.get(index);
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int PAYMENT_FIELD_NUMBER = 9;
private java.util.List payment_;
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public java.util.List getPaymentList() {
return payment_;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder>
getPaymentOrBuilderList() {
return payment_;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public int getPaymentCount() {
return payment_.size();
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment getPayment(int index) {
return payment_.get(index);
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder getPaymentOrBuilder(
int index) {
return payment_.get(index);
}
public static final int ACTION_FIELD_NUMBER = 10;
private java.util.List action_;
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public java.util.List getActionList() {
return action_;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder>
getActionOrBuilderList() {
return action_;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public int getActionCount() {
return action_.size();
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getAction(int index) {
return action_.get(index);
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder getActionOrBuilder(
int index) {
return action_.get(index);
}
public static final int TS_FIELD_NUMBER = 11;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps ts_;
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public boolean hasTs() {
return ts_ != null;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getTs() {
return ts_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.getDefaultInstance() : ts_;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder getTsOrBuilder() {
return getTs();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (version_ != 0) {
output.writeUInt32(2, version_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.FRESH.getNumber()) {
output.writeEnum(3, status_);
}
if (!getClaimBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, claim_);
}
if (facilitator_ != null) {
output.writeMessage(5, getFacilitator());
}
if (customer_ != null) {
output.writeMessage(6, getCustomer());
}
if (bill_ != null) {
output.writeMessage(7, getBill());
}
for (int i = 0; i < item_.size(); i++) {
output.writeMessage(8, item_.get(i));
}
for (int i = 0; i < payment_.size(); i++) {
output.writeMessage(9, payment_.get(i));
}
for (int i = 0; i < action_.size(); i++) {
output.writeMessage(10, action_.get(i));
}
if (ts_ != null) {
output.writeMessage(11, getTs());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, version_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.FRESH.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (!getClaimBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, claim_);
}
if (facilitator_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getFacilitator());
}
if (customer_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getCustomer());
}
if (bill_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getBill());
}
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, item_.get(i));
}
for (int i = 0; i < payment_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, payment_.get(i));
}
for (int i = 0; i < action_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, action_.get(i));
}
if (ts_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getTs());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket other = (io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (getVersion()
!= other.getVersion()) return false;
if (status_ != other.status_) return false;
if (!getClaim()
.equals(other.getClaim())) return false;
if (hasFacilitator() != other.hasFacilitator()) return false;
if (hasFacilitator()) {
if (!getFacilitator()
.equals(other.getFacilitator())) return false;
}
if (hasCustomer() != other.hasCustomer()) return false;
if (hasCustomer()) {
if (!getCustomer()
.equals(other.getCustomer())) return false;
}
if (hasBill() != other.hasBill()) return false;
if (hasBill()) {
if (!getBill()
.equals(other.getBill())) return false;
}
if (!getItemList()
.equals(other.getItemList())) return false;
if (!getPaymentList()
.equals(other.getPaymentList())) return false;
if (!getActionList()
.equals(other.getActionList())) return false;
if (hasTs() != other.hasTs()) return false;
if (hasTs()) {
if (!getTs()
.equals(other.getTs())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + CLAIM_FIELD_NUMBER;
hash = (53 * hash) + getClaim().hashCode();
if (hasFacilitator()) {
hash = (37 * hash) + FACILITATOR_FIELD_NUMBER;
hash = (53 * hash) + getFacilitator().hashCode();
}
if (hasCustomer()) {
hash = (37 * hash) + CUSTOMER_FIELD_NUMBER;
hash = (53 * hash) + getCustomer().hashCode();
}
if (hasBill()) {
hash = (37 * hash) + BILL_FIELD_NUMBER;
hash = (53 * hash) + getBill().hashCode();
}
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (getPaymentCount() > 0) {
hash = (37 * hash) + PAYMENT_FIELD_NUMBER;
hash = (53 * hash) + getPaymentList().hashCode();
}
if (getActionCount() > 0) {
hash = (37 * hash) + ACTION_FIELD_NUMBER;
hash = (53 * hash) + getActionList().hashCode();
}
if (hasTs()) {
hash = (37 * hash) + TS_FIELD_NUMBER;
hash = (53 * hash) + getTs().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a record of a purchase made by a consumer at a retail cannabis location. Purchases are like orders, in that
* they are both consumer interactions with retailers in a commercial setting, but purchases are always made in-person
* using a point-of-sale device, and never online or from remote.
*
*
* Protobuf type {@code bloombox.pos.PurchaseTicket}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PurchaseTicket)
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PurchaseTicket_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PurchaseTicket_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.class, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getItemFieldBuilder();
getPaymentFieldBuilder();
getActionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
version_ = 0;
status_ = 0;
claim_ = "";
if (facilitatorBuilder_ == null) {
facilitator_ = null;
} else {
facilitator_ = null;
facilitatorBuilder_ = null;
}
if (customerBuilder_ == null) {
customer_ = null;
} else {
customer_ = null;
customerBuilder_ = null;
}
if (billBuilder_ == null) {
bill_ = null;
} else {
bill_ = null;
billBuilder_ = null;
}
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
itemBuilder_.clear();
}
if (paymentBuilder_ == null) {
payment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
} else {
paymentBuilder_.clear();
}
if (actionBuilder_ == null) {
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
} else {
actionBuilder_.clear();
}
if (tsBuilder_ == null) {
ts_ = null;
} else {
ts_ = null;
tsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PurchaseTicket_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket build() {
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket result = new io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
result.version_ = version_;
result.status_ = status_;
result.claim_ = claim_;
if (facilitatorBuilder_ == null) {
result.facilitator_ = facilitator_;
} else {
result.facilitator_ = facilitatorBuilder_.build();
}
if (customerBuilder_ == null) {
result.customer_ = customer_;
} else {
result.customer_ = customerBuilder_.build();
}
if (billBuilder_ == null) {
result.bill_ = bill_;
} else {
result.bill_ = billBuilder_.build();
}
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
if (paymentBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
payment_ = java.util.Collections.unmodifiableList(payment_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.payment_ = payment_;
} else {
result.payment_ = paymentBuilder_.build();
}
if (actionBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
action_ = java.util.Collections.unmodifiableList(action_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.action_ = action_;
} else {
result.action_ = actionBuilder_.build();
}
if (tsBuilder_ == null) {
result.ts_ = ts_;
} else {
result.ts_ = tsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.getClaim().isEmpty()) {
claim_ = other.claim_;
onChanged();
}
if (other.hasFacilitator()) {
mergeFacilitator(other.getFacilitator());
}
if (other.hasCustomer()) {
mergeCustomer(other.getCustomer());
}
if (other.hasBill()) {
mergeBill(other.getBill());
}
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000080);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (paymentBuilder_ == null) {
if (!other.payment_.isEmpty()) {
if (payment_.isEmpty()) {
payment_ = other.payment_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensurePaymentIsMutable();
payment_.addAll(other.payment_);
}
onChanged();
}
} else {
if (!other.payment_.isEmpty()) {
if (paymentBuilder_.isEmpty()) {
paymentBuilder_.dispose();
paymentBuilder_ = null;
payment_ = other.payment_;
bitField0_ = (bitField0_ & ~0x00000100);
paymentBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPaymentFieldBuilder() : null;
} else {
paymentBuilder_.addAllMessages(other.payment_);
}
}
}
if (actionBuilder_ == null) {
if (!other.action_.isEmpty()) {
if (action_.isEmpty()) {
action_ = other.action_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureActionIsMutable();
action_.addAll(other.action_);
}
onChanged();
}
} else {
if (!other.action_.isEmpty()) {
if (actionBuilder_.isEmpty()) {
actionBuilder_.dispose();
actionBuilder_ = null;
action_ = other.action_;
bitField0_ = (bitField0_ & ~0x00000200);
actionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getActionFieldBuilder() : null;
} else {
actionBuilder_.addAllMessages(other.action_);
}
}
}
if (other.hasTs()) {
mergeTs(other.getTs());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder> keyBuilder_;
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public Builder mergeKey(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance() : key_;
}
}
/**
*
* Unique key generated to address this purchase. Usually consists of a string UUID.
*
*
* .opencannabis.commerce.PurchaseKey key = 1 [(.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private int version_ ;
/**
*
* Version or revision number for this purchase ticket.
*
*
* uint32 version = 2;
*/
public int getVersion() {
return version_;
}
/**
*
* Version or revision number for this purchase ticket.
*
*
* uint32 version = 2;
*/
public Builder setVersion(int value) {
version_ = value;
onChanged();
return this;
}
/**
*
* Version or revision number for this purchase ticket.
*
*
* uint32 version = 2;
*/
public Builder clearVersion() {
version_ = 0;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.UNRECOGNIZED : result;
}
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the current status of this individual purchase transaction.
*
*
* .opencannabis.commerce.PurchaseStatus status = 3;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object claim_ = "";
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public java.lang.String getClaim() {
java.lang.Object ref = claim_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
claim_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public com.google.protobuf.ByteString
getClaimBytes() {
java.lang.Object ref = claim_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
claim_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public Builder setClaim(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
claim_ = value;
onChanged();
return this;
}
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public Builder clearClaim() {
claim_ = getDefaultInstance().getClaim();
onChanged();
return this;
}
/**
*
* Point-of-sale session that is currently claiming this ticket, or last claimed this ticket.
*
*
* string claim = 4;
*/
public Builder setClaimBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
claim_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator facilitator_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder> facilitatorBuilder_;
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public boolean hasFacilitator() {
return facilitatorBuilder_ != null || facilitator_ != null;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getFacilitator() {
if (facilitatorBuilder_ == null) {
return facilitator_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.getDefaultInstance() : facilitator_;
} else {
return facilitatorBuilder_.getMessage();
}
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public Builder setFacilitator(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator value) {
if (facilitatorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
facilitator_ = value;
onChanged();
} else {
facilitatorBuilder_.setMessage(value);
}
return this;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public Builder setFacilitator(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder builderForValue) {
if (facilitatorBuilder_ == null) {
facilitator_ = builderForValue.build();
onChanged();
} else {
facilitatorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public Builder mergeFacilitator(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator value) {
if (facilitatorBuilder_ == null) {
if (facilitator_ != null) {
facilitator_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.newBuilder(facilitator_).mergeFrom(value).buildPartial();
} else {
facilitator_ = value;
}
onChanged();
} else {
facilitatorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public Builder clearFacilitator() {
if (facilitatorBuilder_ == null) {
facilitator_ = null;
onChanged();
} else {
facilitator_ = null;
facilitatorBuilder_ = null;
}
return this;
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder getFacilitatorBuilder() {
onChanged();
return getFacilitatorFieldBuilder().getBuilder();
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder getFacilitatorOrBuilder() {
if (facilitatorBuilder_ != null) {
return facilitatorBuilder_.getMessageOrBuilder();
} else {
return facilitator_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.getDefaultInstance() : facilitator_;
}
}
/**
*
* Partner organization, location, device, and staff member that facilitated this transaction.
*
*
* .opencannabis.commerce.PurchaseFacilitator facilitator = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder>
getFacilitatorFieldBuilder() {
if (facilitatorBuilder_ == null) {
facilitatorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder>(
getFacilitator(),
getParentForChildren(),
isClean());
facilitator_ = null;
}
return facilitatorBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer customer_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder> customerBuilder_;
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public boolean hasCustomer() {
return customerBuilder_ != null || customer_ != null;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getCustomer() {
if (customerBuilder_ == null) {
return customer_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.getDefaultInstance() : customer_;
} else {
return customerBuilder_.getMessage();
}
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public Builder setCustomer(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer value) {
if (customerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
customer_ = value;
onChanged();
} else {
customerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public Builder setCustomer(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder builderForValue) {
if (customerBuilder_ == null) {
customer_ = builderForValue.build();
onChanged();
} else {
customerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public Builder mergeCustomer(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer value) {
if (customerBuilder_ == null) {
if (customer_ != null) {
customer_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.newBuilder(customer_).mergeFrom(value).buildPartial();
} else {
customer_ = value;
}
onChanged();
} else {
customerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public Builder clearCustomer() {
if (customerBuilder_ == null) {
customer_ = null;
onChanged();
} else {
customer_ = null;
customerBuilder_ = null;
}
return this;
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder getCustomerBuilder() {
onChanged();
return getCustomerFieldBuilder().getBuilder();
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder getCustomerOrBuilder() {
if (customerBuilder_ != null) {
return customerBuilder_.getMessageOrBuilder();
} else {
return customer_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.getDefaultInstance() : customer_;
}
}
/**
*
* Specifies information regarding the customer that made this purchase.
*
*
* .opencannabis.commerce.PurchaseCustomer customer = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder>
getCustomerFieldBuilder() {
if (customerBuilder_ == null) {
customerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder>(
getCustomer(),
getParentForChildren(),
isClean());
customer_ = null;
}
return customerBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges bill_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder> billBuilder_;
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public boolean hasBill() {
return billBuilder_ != null || bill_ != null;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getBill() {
if (billBuilder_ == null) {
return bill_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : bill_;
} else {
return billBuilder_.getMessage();
}
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public Builder setBill(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (billBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
bill_ = value;
onChanged();
} else {
billBuilder_.setMessage(value);
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public Builder setBill(
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder builderForValue) {
if (billBuilder_ == null) {
bill_ = builderForValue.build();
onChanged();
} else {
billBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public Builder mergeBill(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (billBuilder_ == null) {
if (bill_ != null) {
bill_ =
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.newBuilder(bill_).mergeFrom(value).buildPartial();
} else {
bill_ = value;
}
onChanged();
} else {
billBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public Builder clearBill() {
if (billBuilder_ == null) {
bill_ = null;
onChanged();
} else {
bill_ = null;
billBuilder_ = null;
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder getBillBuilder() {
onChanged();
return getBillFieldBuilder().getBuilder();
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getBillOrBuilder() {
if (billBuilder_ != null) {
return billBuilder_.getMessageOrBuilder();
} else {
return bill_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : bill_;
}
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges bill = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>
getBillFieldBuilder() {
if (billBuilder_ == null) {
billBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>(
getBill(),
getParentForChildren(),
isClean());
bill_ = null;
}
return billBuilder_;
}
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder, io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder> itemBuilder_;
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder setItem(
int index, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder setItem(
int index, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder addItem(io.opencannabis.schema.commerce.CommercialPurchase.TicketItem value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder addItem(
int index, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder addItem(
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder addItem(
int index, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder addAllItem(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPurchase.TicketItem> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.getDefaultInstance());
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.getDefaultInstance());
}
/**
*
* Constituent items purchased as part of this commercial purchase ticket.
*
*
* repeated .opencannabis.commerce.TicketItem item = 8;
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder, io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder, io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder>(
item_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private java.util.List payment_ =
java.util.Collections.emptyList();
private void ensurePaymentIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
payment_ = new java.util.ArrayList(payment_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder> paymentBuilder_;
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public java.util.List getPaymentList() {
if (paymentBuilder_ == null) {
return java.util.Collections.unmodifiableList(payment_);
} else {
return paymentBuilder_.getMessageList();
}
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public int getPaymentCount() {
if (paymentBuilder_ == null) {
return payment_.size();
} else {
return paymentBuilder_.getCount();
}
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment getPayment(int index) {
if (paymentBuilder_ == null) {
return payment_.get(index);
} else {
return paymentBuilder_.getMessage(index);
}
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder setPayment(
int index, io.opencannabis.schema.commerce.CommercialPurchase.Payment value) {
if (paymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePaymentIsMutable();
payment_.set(index, value);
onChanged();
} else {
paymentBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder setPayment(
int index, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder builderForValue) {
if (paymentBuilder_ == null) {
ensurePaymentIsMutable();
payment_.set(index, builderForValue.build());
onChanged();
} else {
paymentBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder addPayment(io.opencannabis.schema.commerce.CommercialPurchase.Payment value) {
if (paymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePaymentIsMutable();
payment_.add(value);
onChanged();
} else {
paymentBuilder_.addMessage(value);
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder addPayment(
int index, io.opencannabis.schema.commerce.CommercialPurchase.Payment value) {
if (paymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePaymentIsMutable();
payment_.add(index, value);
onChanged();
} else {
paymentBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder addPayment(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder builderForValue) {
if (paymentBuilder_ == null) {
ensurePaymentIsMutable();
payment_.add(builderForValue.build());
onChanged();
} else {
paymentBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder addPayment(
int index, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder builderForValue) {
if (paymentBuilder_ == null) {
ensurePaymentIsMutable();
payment_.add(index, builderForValue.build());
onChanged();
} else {
paymentBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder addAllPayment(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPurchase.Payment> values) {
if (paymentBuilder_ == null) {
ensurePaymentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, payment_);
onChanged();
} else {
paymentBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder clearPayment() {
if (paymentBuilder_ == null) {
payment_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
paymentBuilder_.clear();
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public Builder removePayment(int index) {
if (paymentBuilder_ == null) {
ensurePaymentIsMutable();
payment_.remove(index);
onChanged();
} else {
paymentBuilder_.remove(index);
}
return this;
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder getPaymentBuilder(
int index) {
return getPaymentFieldBuilder().getBuilder(index);
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder getPaymentOrBuilder(
int index) {
if (paymentBuilder_ == null) {
return payment_.get(index); } else {
return paymentBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder>
getPaymentOrBuilderList() {
if (paymentBuilder_ != null) {
return paymentBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(payment_);
}
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder addPaymentBuilder() {
return getPaymentFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.getDefaultInstance());
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder addPaymentBuilder(
int index) {
return getPaymentFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPurchase.Payment.getDefaultInstance());
}
/**
*
* Specifies how this order was paid for, if applicable at this point in the ticket lifecycle.
*
*
* repeated .opencannabis.commerce.Payment payment = 9;
*/
public java.util.List
getPaymentBuilderList() {
return getPaymentFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder>
getPaymentFieldBuilder() {
if (paymentBuilder_ == null) {
paymentBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder>(
payment_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
payment_ = null;
}
return paymentBuilder_;
}
private java.util.List action_ =
java.util.Collections.emptyList();
private void ensureActionIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
action_ = new java.util.ArrayList(action_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder> actionBuilder_;
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public java.util.List getActionList() {
if (actionBuilder_ == null) {
return java.util.Collections.unmodifiableList(action_);
} else {
return actionBuilder_.getMessageList();
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public int getActionCount() {
if (actionBuilder_ == null) {
return action_.size();
} else {
return actionBuilder_.getCount();
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getAction(int index) {
if (actionBuilder_ == null) {
return action_.get(index);
} else {
return actionBuilder_.getMessage(index);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder setAction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry value) {
if (actionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.set(index, value);
onChanged();
} else {
actionBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder setAction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder builderForValue) {
if (actionBuilder_ == null) {
ensureActionIsMutable();
action_.set(index, builderForValue.build());
onChanged();
} else {
actionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder addAction(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry value) {
if (actionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.add(value);
onChanged();
} else {
actionBuilder_.addMessage(value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder addAction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry value) {
if (actionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.add(index, value);
onChanged();
} else {
actionBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder addAction(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder builderForValue) {
if (actionBuilder_ == null) {
ensureActionIsMutable();
action_.add(builderForValue.build());
onChanged();
} else {
actionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder addAction(
int index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder builderForValue) {
if (actionBuilder_ == null) {
ensureActionIsMutable();
action_.add(index, builderForValue.build());
onChanged();
} else {
actionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder addAllAction(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry> values) {
if (actionBuilder_ == null) {
ensureActionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, action_);
onChanged();
} else {
actionBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder clearAction() {
if (actionBuilder_ == null) {
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
actionBuilder_.clear();
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public Builder removeAction(int index) {
if (actionBuilder_ == null) {
ensureActionIsMutable();
action_.remove(index);
onChanged();
} else {
actionBuilder_.remove(index);
}
return this;
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder getActionBuilder(
int index) {
return getActionFieldBuilder().getBuilder(index);
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder getActionOrBuilder(
int index) {
if (actionBuilder_ == null) {
return action_.get(index); } else {
return actionBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder>
getActionOrBuilderList() {
if (actionBuilder_ != null) {
return actionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(action_);
}
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder addActionBuilder() {
return getActionFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.getDefaultInstance());
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder addActionBuilder(
int index) {
return getActionFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.getDefaultInstance());
}
/**
*
* Actions taken on this order.
*
*
* repeated .opencannabis.commerce.PurchaseLogEntry action = 10;
*/
public java.util.List
getActionBuilderList() {
return getActionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder>
getActionFieldBuilder() {
if (actionBuilder_ == null) {
actionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder>(
action_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
action_ = null;
}
return actionBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps ts_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder> tsBuilder_;
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public boolean hasTs() {
return tsBuilder_ != null || ts_ != null;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getTs() {
if (tsBuilder_ == null) {
return ts_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.getDefaultInstance() : ts_;
} else {
return tsBuilder_.getMessage();
}
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public Builder setTs(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps value) {
if (tsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ts_ = value;
onChanged();
} else {
tsBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public Builder setTs(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder builderForValue) {
if (tsBuilder_ == null) {
ts_ = builderForValue.build();
onChanged();
} else {
tsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public Builder mergeTs(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps value) {
if (tsBuilder_ == null) {
if (ts_ != null) {
ts_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.newBuilder(ts_).mergeFrom(value).buildPartial();
} else {
ts_ = value;
}
onChanged();
} else {
tsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public Builder clearTs() {
if (tsBuilder_ == null) {
ts_ = null;
onChanged();
} else {
ts_ = null;
tsBuilder_ = null;
}
return this;
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder getTsBuilder() {
onChanged();
return getTsFieldBuilder().getBuilder();
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder getTsOrBuilder() {
if (tsBuilder_ != null) {
return tsBuilder_.getMessageOrBuilder();
} else {
return ts_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.getDefaultInstance() : ts_;
}
}
/**
*
* Timestamps that record the temporal position of individual purchase lifecycle events.
*
*
* .opencannabis.commerce.PurchaseTimestamps ts = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder>
getTsFieldBuilder() {
if (tsBuilder_ == null) {
tsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder>(
getTs(),
getParentForChildren(),
isClean());
ts_ = null;
}
return tsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PurchaseTicket)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PurchaseTicket)
private static final io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseTicket parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseTicket(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointOfSaleSessionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bloombox.pos.PointOfSaleSession)
com.google.protobuf.MessageOrBuilder {
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
java.lang.String getUuid();
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUuidBytes();
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
int getStatusValue();
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
io.opencannabis.schema.commerce.CommercialPOS.SessionStatus getStatus();
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
boolean hasUser();
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
io.bloombox.schema.identity.AppUserKey.UserKey getUser();
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
java.util.List
getTicketList();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getTicket(int index);
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
int getTicketCount();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder>
getTicketOrBuilderList();
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder getTicketOrBuilder(
int index);
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
boolean hasState();
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState();
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder();
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
boolean hasSeen();
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen();
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder();
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
boolean hasCreated();
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated();
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder();
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
boolean hasModified();
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getModified();
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder();
}
/**
*
* Specifies the notion of a cash register session. All transactions must be conducted under a valid point of sale
* session, previously established and then opened by an authorized user on an authorized device. Tickets may only be
* part of one POS session, and POS sessions may contain many transactions (or 'purchases').
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleSession}
*/
public static final class PointOfSaleSession extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:bloombox.pos.PointOfSaleSession)
PointOfSaleSessionOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointOfSaleSession.newBuilder() to construct.
private PointOfSaleSession(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointOfSaleSession() {
uuid_ = "";
status_ = 0;
ticket_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PointOfSaleSession(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 26: {
io.bloombox.schema.identity.AppUserKey.UserKey.Builder subBuilder = null;
if (user_ != null) {
subBuilder = user_.toBuilder();
}
user_ = input.readMessage(io.bloombox.schema.identity.AppUserKey.UserKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(user_);
user_ = subBuilder.buildPartial();
}
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
ticket_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
ticket_.add(
input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.parser(), extensionRegistry));
break;
}
case 42: {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder subBuilder = null;
if (state_ != null) {
subBuilder = state_.toBuilder();
}
state_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(state_);
state_ = subBuilder.buildPartial();
}
break;
}
case 50: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (seen_ != null) {
subBuilder = seen_.toBuilder();
}
seen_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seen_);
seen_ = subBuilder.buildPartial();
}
break;
}
case 58: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (created_ != null) {
subBuilder = created_.toBuilder();
}
created_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(created_);
created_ = subBuilder.buildPartial();
}
break;
}
case 66: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (modified_ != null) {
subBuilder = modified_.toBuilder();
}
modified_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(modified_);
modified_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) != 0)) {
ticket_ = java.util.Collections.unmodifiableList(ticket_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleSession_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleSession_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder.class);
}
private int bitField0_;
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_;
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.SessionStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.SessionStatus result = io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.UNRECOGNIZED : result;
}
public static final int USER_FIELD_NUMBER = 3;
private io.bloombox.schema.identity.AppUserKey.UserKey user_;
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public boolean hasUser() {
return user_ != null;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
return user_ == null ? io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
return getUser();
}
public static final int TICKET_FIELD_NUMBER = 4;
private java.util.List ticket_;
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public java.util.List getTicketList() {
return ticket_;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder>
getTicketOrBuilderList() {
return ticket_;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public int getTicketCount() {
return ticket_.size();
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getTicket(int index) {
return ticket_.get(index);
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder getTicketOrBuilder(
int index) {
return ticket_.get(index);
}
public static final int STATE_FIELD_NUMBER = 5;
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState state_;
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public boolean hasState() {
return state_ != null;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState() {
return state_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder() {
return getState();
}
public static final int SEEN_FIELD_NUMBER = 6;
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public boolean hasSeen() {
return seen_ != null;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
return getSeen();
}
public static final int CREATED_FIELD_NUMBER = 7;
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public boolean hasCreated() {
return created_ != null;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
return getCreated();
}
public static final int MODIFIED_FIELD_NUMBER = 8;
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public boolean hasModified() {
return modified_ != null;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
return getModified();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.ESTABLISHED.getNumber()) {
output.writeEnum(2, status_);
}
if (user_ != null) {
output.writeMessage(3, getUser());
}
for (int i = 0; i < ticket_.size(); i++) {
output.writeMessage(4, ticket_.get(i));
}
if (state_ != null) {
output.writeMessage(5, getState());
}
if (seen_ != null) {
output.writeMessage(6, getSeen());
}
if (created_ != null) {
output.writeMessage(7, getCreated());
}
if (modified_ != null) {
output.writeMessage(8, getModified());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
if (status_ != io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.ESTABLISHED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (user_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getUser());
}
for (int i = 0; i < ticket_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, ticket_.get(i));
}
if (state_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getState());
}
if (seen_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSeen());
}
if (created_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCreated());
}
if (modified_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getModified());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession other = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (status_ != other.status_) return false;
if (hasUser() != other.hasUser()) return false;
if (hasUser()) {
if (!getUser()
.equals(other.getUser())) return false;
}
if (!getTicketList()
.equals(other.getTicketList())) return false;
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (!getState()
.equals(other.getState())) return false;
}
if (hasSeen() != other.hasSeen()) return false;
if (hasSeen()) {
if (!getSeen()
.equals(other.getSeen())) return false;
}
if (hasCreated() != other.hasCreated()) return false;
if (hasCreated()) {
if (!getCreated()
.equals(other.getCreated())) return false;
}
if (hasModified() != other.hasModified()) return false;
if (hasModified()) {
if (!getModified()
.equals(other.getModified())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
if (getTicketCount() > 0) {
hash = (37 * hash) + TICKET_FIELD_NUMBER;
hash = (53 * hash) + getTicketList().hashCode();
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
}
if (hasSeen()) {
hash = (37 * hash) + SEEN_FIELD_NUMBER;
hash = (53 * hash) + getSeen().hashCode();
}
if (hasCreated()) {
hash = (37 * hash) + CREATED_FIELD_NUMBER;
hash = (53 * hash) + getCreated().hashCode();
}
if (hasModified()) {
hash = (37 * hash) + MODIFIED_FIELD_NUMBER;
hash = (53 * hash) + getModified().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the notion of a cash register session. All transactions must be conducted under a valid point of sale
* session, previously established and then opened by an authorized user on an authorized device. Tickets may only be
* part of one POS session, and POS sessions may contain many transactions (or 'purchases').
*
*
* Protobuf type {@code bloombox.pos.PointOfSaleSession}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:bloombox.pos.PointOfSaleSession)
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSessionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleSession_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleSession_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.class, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTicketFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
status_ = 0;
if (userBuilder_ == null) {
user_ = null;
} else {
user_ = null;
userBuilder_ = null;
}
if (ticketBuilder_ == null) {
ticket_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ticketBuilder_.clear();
}
if (stateBuilder_ == null) {
state_ = null;
} else {
state_ = null;
stateBuilder_ = null;
}
if (seenBuilder_ == null) {
seen_ = null;
} else {
seen_ = null;
seenBuilder_ = null;
}
if (createdBuilder_ == null) {
created_ = null;
} else {
created_ = null;
createdBuilder_ = null;
}
if (modifiedBuilder_ == null) {
modified_ = null;
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPOS.internal_static_bloombox_pos_PointOfSaleSession_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession build() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession buildPartial() {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession result = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.uuid_ = uuid_;
result.status_ = status_;
if (userBuilder_ == null) {
result.user_ = user_;
} else {
result.user_ = userBuilder_.build();
}
if (ticketBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
ticket_ = java.util.Collections.unmodifiableList(ticket_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.ticket_ = ticket_;
} else {
result.ticket_ = ticketBuilder_.build();
}
if (stateBuilder_ == null) {
result.state_ = state_;
} else {
result.state_ = stateBuilder_.build();
}
if (seenBuilder_ == null) {
result.seen_ = seen_;
} else {
result.seen_ = seenBuilder_.build();
}
if (createdBuilder_ == null) {
result.created_ = created_;
} else {
result.created_ = createdBuilder_.build();
}
if (modifiedBuilder_ == null) {
result.modified_ = modified_;
} else {
result.modified_ = modifiedBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession other) {
if (other == io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasUser()) {
mergeUser(other.getUser());
}
if (ticketBuilder_ == null) {
if (!other.ticket_.isEmpty()) {
if (ticket_.isEmpty()) {
ticket_ = other.ticket_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureTicketIsMutable();
ticket_.addAll(other.ticket_);
}
onChanged();
}
} else {
if (!other.ticket_.isEmpty()) {
if (ticketBuilder_.isEmpty()) {
ticketBuilder_.dispose();
ticketBuilder_ = null;
ticket_ = other.ticket_;
bitField0_ = (bitField0_ & ~0x00000008);
ticketBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTicketFieldBuilder() : null;
} else {
ticketBuilder_.addAllMessages(other.ticket_);
}
}
}
if (other.hasState()) {
mergeState(other.getState());
}
if (other.hasSeen()) {
mergeSeen(other.getSeen());
}
if (other.hasCreated()) {
mergeCreated(other.getCreated());
}
if (other.hasModified()) {
mergeModified(other.getModified());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object uuid_ = "";
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* UUID generated to address this session.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public io.opencannabis.schema.commerce.CommercialPOS.SessionStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPOS.SessionStatus result = io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPOS.SessionStatus.UNRECOGNIZED : result;
}
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialPOS.SessionStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the current status of this POS session.
*
*
* .bloombox.pos.SessionStatus status = 2;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private io.bloombox.schema.identity.AppUserKey.UserKey user_;
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder> userBuilder_;
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public boolean hasUser() {
return userBuilder_ != null || user_ != null;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey getUser() {
if (userBuilder_ == null) {
return user_ == null ? io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
} else {
return userBuilder_.getMessage();
}
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public Builder setUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
user_ = value;
onChanged();
} else {
userBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public Builder setUser(
io.bloombox.schema.identity.AppUserKey.UserKey.Builder builderForValue) {
if (userBuilder_ == null) {
user_ = builderForValue.build();
onChanged();
} else {
userBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public Builder mergeUser(io.bloombox.schema.identity.AppUserKey.UserKey value) {
if (userBuilder_ == null) {
if (user_ != null) {
user_ =
io.bloombox.schema.identity.AppUserKey.UserKey.newBuilder(user_).mergeFrom(value).buildPartial();
} else {
user_ = value;
}
onChanged();
} else {
userBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public Builder clearUser() {
if (userBuilder_ == null) {
user_ = null;
onChanged();
} else {
user_ = null;
userBuilder_ = null;
}
return this;
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public io.bloombox.schema.identity.AppUserKey.UserKey.Builder getUserBuilder() {
onChanged();
return getUserFieldBuilder().getBuilder();
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
public io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder getUserOrBuilder() {
if (userBuilder_ != null) {
return userBuilder_.getMessageOrBuilder();
} else {
return user_ == null ?
io.bloombox.schema.identity.AppUserKey.UserKey.getDefaultInstance() : user_;
}
}
/**
*
* Specifies the authorized user account bound to this POS session.
*
*
* .bloombox.identity.UserKey user = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>
getUserFieldBuilder() {
if (userBuilder_ == null) {
userBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.bloombox.schema.identity.AppUserKey.UserKey, io.bloombox.schema.identity.AppUserKey.UserKey.Builder, io.bloombox.schema.identity.AppUserKey.UserKeyOrBuilder>(
getUser(),
getParentForChildren(),
isClean());
user_ = null;
}
return userBuilder_;
}
private java.util.List ticket_ =
java.util.Collections.emptyList();
private void ensureTicketIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
ticket_ = new java.util.ArrayList(ticket_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder> ticketBuilder_;
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public java.util.List getTicketList() {
if (ticketBuilder_ == null) {
return java.util.Collections.unmodifiableList(ticket_);
} else {
return ticketBuilder_.getMessageList();
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public int getTicketCount() {
if (ticketBuilder_ == null) {
return ticket_.size();
} else {
return ticketBuilder_.getCount();
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket getTicket(int index) {
if (ticketBuilder_ == null) {
return ticket_.get(index);
} else {
return ticketBuilder_.getMessage(index);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder setTicket(
int index, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket value) {
if (ticketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTicketIsMutable();
ticket_.set(index, value);
onChanged();
} else {
ticketBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder setTicket(
int index, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder builderForValue) {
if (ticketBuilder_ == null) {
ensureTicketIsMutable();
ticket_.set(index, builderForValue.build());
onChanged();
} else {
ticketBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder addTicket(io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket value) {
if (ticketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTicketIsMutable();
ticket_.add(value);
onChanged();
} else {
ticketBuilder_.addMessage(value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder addTicket(
int index, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket value) {
if (ticketBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTicketIsMutable();
ticket_.add(index, value);
onChanged();
} else {
ticketBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder addTicket(
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder builderForValue) {
if (ticketBuilder_ == null) {
ensureTicketIsMutable();
ticket_.add(builderForValue.build());
onChanged();
} else {
ticketBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder addTicket(
int index, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder builderForValue) {
if (ticketBuilder_ == null) {
ensureTicketIsMutable();
ticket_.add(index, builderForValue.build());
onChanged();
} else {
ticketBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder addAllTicket(
java.lang.Iterable extends io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket> values) {
if (ticketBuilder_ == null) {
ensureTicketIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ticket_);
onChanged();
} else {
ticketBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder clearTicket() {
if (ticketBuilder_ == null) {
ticket_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
ticketBuilder_.clear();
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public Builder removeTicket(int index) {
if (ticketBuilder_ == null) {
ensureTicketIsMutable();
ticket_.remove(index);
onChanged();
} else {
ticketBuilder_.remove(index);
}
return this;
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder getTicketBuilder(
int index) {
return getTicketFieldBuilder().getBuilder(index);
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder getTicketOrBuilder(
int index) {
if (ticketBuilder_ == null) {
return ticket_.get(index); } else {
return ticketBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public java.util.List extends io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder>
getTicketOrBuilderList() {
if (ticketBuilder_ != null) {
return ticketBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(ticket_);
}
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder addTicketBuilder() {
return getTicketFieldBuilder().addBuilder(
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.getDefaultInstance());
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder addTicketBuilder(
int index) {
return getTicketFieldBuilder().addBuilder(
index, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.getDefaultInstance());
}
/**
*
* Specifies sessions attached to this point of sale device.
*
*
* repeated .bloombox.pos.PurchaseTicket ticket = 4 [(.core.collection) = { ... }
*/
public java.util.List
getTicketBuilderList() {
return getTicketFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder>
getTicketFieldBuilder() {
if (ticketBuilder_ == null) {
ticketBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicket.Builder, io.opencannabis.schema.commerce.CommercialPOS.PurchaseTicketOrBuilder>(
ticket_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
ticket_ = null;
}
return ticketBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState state_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder> stateBuilder_;
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public boolean hasState() {
return stateBuilder_ != null || state_ != null;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState getState() {
if (stateBuilder_ == null) {
return state_ == null ? io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
} else {
return stateBuilder_.getMessage();
}
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public Builder setState(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState value) {
if (stateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
} else {
stateBuilder_.setMessage(value);
}
return this;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public Builder setState(
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder builderForValue) {
if (stateBuilder_ == null) {
state_ = builderForValue.build();
onChanged();
} else {
stateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public Builder mergeState(io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState value) {
if (stateBuilder_ == null) {
if (state_ != null) {
state_ =
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.newBuilder(state_).mergeFrom(value).buildPartial();
} else {
state_ = value;
}
onChanged();
} else {
stateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public Builder clearState() {
if (stateBuilder_ == null) {
state_ = null;
onChanged();
} else {
state_ = null;
stateBuilder_ = null;
}
return this;
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder getStateBuilder() {
onChanged();
return getStateFieldBuilder().getBuilder();
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder getStateOrBuilder() {
if (stateBuilder_ != null) {
return stateBuilder_.getMessageOrBuilder();
} else {
return state_ == null ?
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.getDefaultInstance() : state_;
}
}
/**
*
* Opening and closing state of the cash register.
*
*
* .bloombox.pos.PointOfSaleState state = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder>
getStateFieldBuilder() {
if (stateBuilder_ == null) {
stateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleState.Builder, io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleStateOrBuilder>(
getState(),
getParentForChildren(),
isClean());
state_ = null;
}
return stateBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant seen_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> seenBuilder_;
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public boolean hasSeen() {
return seenBuilder_ != null || seen_ != null;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getSeen() {
if (seenBuilder_ == null) {
return seen_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
} else {
return seenBuilder_.getMessage();
}
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public Builder setSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seen_ = value;
onChanged();
} else {
seenBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public Builder setSeen(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (seenBuilder_ == null) {
seen_ = builderForValue.build();
onChanged();
} else {
seenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public Builder mergeSeen(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (seenBuilder_ == null) {
if (seen_ != null) {
seen_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(seen_).mergeFrom(value).buildPartial();
} else {
seen_ = value;
}
onChanged();
} else {
seenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public Builder clearSeen() {
if (seenBuilder_ == null) {
seen_ = null;
onChanged();
} else {
seen_ = null;
seenBuilder_ = null;
}
return this;
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getSeenBuilder() {
onChanged();
return getSeenFieldBuilder().getBuilder();
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getSeenOrBuilder() {
if (seenBuilder_ != null) {
return seenBuilder_.getMessageOrBuilder();
} else {
return seen_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : seen_;
}
}
/**
*
* Specifies the last moment this session was witnessed on the API.
*
*
* .opencannabis.temporal.Instant seen = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getSeenFieldBuilder() {
if (seenBuilder_ == null) {
seenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getSeen(),
getParentForChildren(),
isClean());
seen_ = null;
}
return seenBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> createdBuilder_;
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public boolean hasCreated() {
return createdBuilder_ != null || created_ != null;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
if (createdBuilder_ == null) {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
} else {
return createdBuilder_.getMessage();
}
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public Builder setCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
created_ = value;
onChanged();
} else {
createdBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public Builder setCreated(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (createdBuilder_ == null) {
created_ = builderForValue.build();
onChanged();
} else {
createdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public Builder mergeCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (created_ != null) {
created_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(created_).mergeFrom(value).buildPartial();
} else {
created_ = value;
}
onChanged();
} else {
createdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public Builder clearCreated() {
if (createdBuilder_ == null) {
created_ = null;
onChanged();
} else {
created_ = null;
createdBuilder_ = null;
}
return this;
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getCreatedBuilder() {
onChanged();
return getCreatedFieldBuilder().getBuilder();
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
if (createdBuilder_ != null) {
return createdBuilder_.getMessageOrBuilder();
} else {
return created_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
}
/**
*
* Specifies the moment this session was created on the API.
*
*
* .opencannabis.temporal.Instant created = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getCreatedFieldBuilder() {
if (createdBuilder_ == null) {
createdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getCreated(),
getParentForChildren(),
isClean());
created_ = null;
}
return createdBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> modifiedBuilder_;
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public boolean hasModified() {
return modifiedBuilder_ != null || modified_ != null;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
if (modifiedBuilder_ == null) {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
} else {
return modifiedBuilder_.getMessage();
}
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public Builder setModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
modified_ = value;
onChanged();
} else {
modifiedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public Builder setModified(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (modifiedBuilder_ == null) {
modified_ = builderForValue.build();
onChanged();
} else {
modifiedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public Builder mergeModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (modified_ != null) {
modified_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(modified_).mergeFrom(value).buildPartial();
} else {
modified_ = value;
}
onChanged();
} else {
modifiedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public Builder clearModified() {
if (modifiedBuilder_ == null) {
modified_ = null;
onChanged();
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getModifiedBuilder() {
onChanged();
return getModifiedFieldBuilder().getBuilder();
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
if (modifiedBuilder_ != null) {
return modifiedBuilder_.getMessageOrBuilder();
} else {
return modified_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
}
/**
*
* Specifies the last time this session was changed, except for `seen` updates.
*
*
* .opencannabis.temporal.Instant modified = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getModifiedFieldBuilder() {
if (modifiedBuilder_ == null) {
modifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getModified(),
getParentForChildren(),
isClean());
modified_ = null;
}
return modifiedBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:bloombox.pos.PointOfSaleSession)
}
// @@protoc_insertion_point(class_scope:bloombox.pos.PointOfSaleSession)
private static final io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession();
}
public static io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointOfSaleSession parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PointOfSaleSession(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPOS.PointOfSaleSession getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_POSHardware_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_POSHardware_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_POSHardware_Features_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_POSHardware_Features_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PointOfSaleDevice_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PointOfSaleDevice_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PointOfSaleState_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PointOfSaleState_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PointOfSaleState_SessionOpen_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PointOfSaleState_SessionClose_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PurchaseTicket_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PurchaseTicket_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_bloombox_pos_PointOfSaleSession_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_bloombox_pos_PointOfSaleSession_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\025pos/PointOfSale.proto\022\014bloombox.pos\032\024c" +
"ore/Datamodel.proto\032\026identity/UserKey.pr" +
"oto\032\027commerce/Currency.proto\032\027commerce/P" +
"urchase.proto\032#analytics/context/Applica" +
"tion.proto\032\026temporal/Instant.proto\"\305\002\n\013P" +
"OSHardware\022,\n\004type\030\001 \001(\0162\036.bloombox.pos." +
"POSHardware.Type\0223\n\007support\030\002 \001(\0132\".bloo" +
"mbox.pos.POSHardware.Features\022\017\n\007version" +
"\030\003 \001(\t\032O\n\010Features\0222\n\007feature\030\001 \003(\0162!.bl" +
"oombox.pos.POSHardware.Feature\022\017\n\007drawer" +
"s\030\002 \001(\r\"%\n\004Type\022\017\n\013UNSPECIFIED\020\000\022\014\n\010BLOO" +
"MBOX\020\001\"J\n\007Feature\022\013\n\007PRINTER\020\000\022\007\n\003BCS\020\001\022" +
"\007\n\003MSR\020\002\022\007\n\003BLE\020\003\022\t\n\005SCALE\020\004\022\014\n\010LABELLER" +
"\020\005\"\354\003\n\021PointOfSaleDevice\022\024\n\004uuid\030\001 \001(\tB\006" +
"\302\265\003\002\010\002\022\014\n\004name\030\002 \001(\t\022-\n\006status\030\003 \001(\0162\035.b" +
"loombox.pos.POSDeviceStatus\022\r\n\005claim\030\004 \001" +
"(\t\022+\n\010hardware\030\005 \001(\0132\031.bloombox.pos.POSH" +
"ardware\022:\n\003app\030\006 \001(\0132-.bloombox.analytic" +
"s.context.DeviceApplication\0229\n\007session\030\007" +
" \003(\0132 .bloombox.pos.PointOfSaleSessionB\006" +
"\322\265\003\002\010\001\022-\n\005state\030\010 \001(\0132\036.bloombox.pos.Poi" +
"ntOfSaleState\022,\n\004seen\030\t \001(\0132\036.opencannab" +
"is.temporal.Instant\022/\n\007created\030b \001(\0132\036.o" +
"pencannabis.temporal.Instant\0220\n\010modified" +
"\030c \001(\0132\036.opencannabis.temporal.Instant:\021" +
"\202\367\002\r\010\002\022\tregisters\"\365\003\n\020PointOfSaleState\0228" +
"\n\004open\030\001 \001(\0132*.bloombox.pos.PointOfSaleS" +
"tate.SessionOpen\0227\n\013transaction\030\002 \003(\0132\"." +
"opencannabis.commerce.PurchaseKey\0225\n\007cur" +
"rent\030\003 \001(\0132$.opencannabis.commerce.BillO" +
"fCharges\022:\n\005close\030\004 \001(\0132+.bloombox.pos.P" +
"ointOfSaleState.SessionClose\032|\n\013SessionO" +
"pen\022;\n\ropening_float\030\001 \001(\0132$.opencannabi" +
"s.commerce.CurrencyValue\0220\n\010occurred\030\002 \001" +
"(\0132\036.opencannabis.temporal.Instant\032}\n\014Se" +
"ssionClose\022;\n\rclosing_count\030\001 \001(\0132$.open" +
"cannabis.commerce.CurrencyValue\0220\n\010occur" +
"red\030\002 \001(\0132\036.opencannabis.temporal.Instan" +
"t\"\265\004\n\016PurchaseTicket\0227\n\003key\030\001 \001(\0132\".open" +
"cannabis.commerce.PurchaseKeyB\006\302\265\003\002\010\001\022\017\n" +
"\007version\030\002 \001(\r\0225\n\006status\030\003 \001(\0162%.opencan" +
"nabis.commerce.PurchaseStatus\022\r\n\005claim\030\004" +
" \001(\t\022?\n\013facilitator\030\005 \001(\0132*.opencannabis" +
".commerce.PurchaseFacilitator\0229\n\010custome" +
"r\030\006 \001(\0132\'.opencannabis.commerce.Purchase" +
"Customer\0222\n\004bill\030\007 \001(\0132$.opencannabis.co" +
"mmerce.BillOfCharges\022/\n\004item\030\010 \003(\0132!.ope" +
"ncannabis.commerce.TicketItem\022/\n\007payment" +
"\030\t \003(\0132\036.opencannabis.commerce.Payment\0227" +
"\n\006action\030\n \003(\0132\'.opencannabis.commerce.P" +
"urchaseLogEntry\0225\n\002ts\030\013 \001(\0132).opencannab" +
"is.commerce.PurchaseTimestamps:\021\202\367\002\r\010\002\022\t" +
"purchases\"\233\003\n\022PointOfSaleSession\022\024\n\004uuid" +
"\030\001 \001(\tB\006\302\265\003\002\010\002\022+\n\006status\030\002 \001(\0162\033.bloombo" +
"x.pos.SessionStatus\022(\n\004user\030\003 \001(\0132\032.bloo" +
"mbox.identity.UserKey\022F\n\006ticket\030\004 \003(\0132\034." +
"bloombox.pos.PurchaseTicketB\030\322\265\003\002\010\001\322\265\003\016\032" +
"\014transactions\022-\n\005state\030\005 \001(\0132\036.bloombox." +
"pos.PointOfSaleState\022,\n\004seen\030\006 \001(\0132\036.ope" +
"ncannabis.temporal.Instant\022/\n\007created\030\007 " +
"\001(\0132\036.opencannabis.temporal.Instant\0220\n\010m" +
"odified\030\010 \001(\0132\036.opencannabis.temporal.In" +
"stant:\020\202\367\002\014\010\001\022\010sessions*b\n\rSessionStatus" +
"\022\017\n\013ESTABLISHED\020\000\022\n\n\006ACTIVE\020\001\022\r\n\tSUSPEND" +
"ED\020\002\022\013\n\007EXPIRED\020\003\022\016\n\nTERMINATED\020\004\022\010\n\004LOS" +
"T\020\005*(\n\017POSDeviceStatus\022\010\n\004IDLE\020\000\022\013\n\007CLAI" +
"MED\020\001B:\n\037io.opencannabis.schema.commerce" +
"B\rCommercialPOSH\001P\000\242\002\003OCSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.bloombox.schema.identity.AppUserKey.getDescriptor(),
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor(),
io.opencannabis.schema.commerce.CommercialPurchase.getDescriptor(),
io.bloombox.schema.telemetry.context.ApplicationContext.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
}, assigner);
internal_static_bloombox_pos_POSHardware_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_bloombox_pos_POSHardware_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_POSHardware_descriptor,
new java.lang.String[] { "Type", "Support", "Version", });
internal_static_bloombox_pos_POSHardware_Features_descriptor =
internal_static_bloombox_pos_POSHardware_descriptor.getNestedTypes().get(0);
internal_static_bloombox_pos_POSHardware_Features_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_POSHardware_Features_descriptor,
new java.lang.String[] { "Feature", "Drawers", });
internal_static_bloombox_pos_PointOfSaleDevice_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_bloombox_pos_PointOfSaleDevice_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PointOfSaleDevice_descriptor,
new java.lang.String[] { "Uuid", "Name", "Status", "Claim", "Hardware", "App", "Session", "State", "Seen", "Created", "Modified", });
internal_static_bloombox_pos_PointOfSaleState_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_bloombox_pos_PointOfSaleState_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PointOfSaleState_descriptor,
new java.lang.String[] { "Open", "Transaction", "Current", "Close", });
internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor =
internal_static_bloombox_pos_PointOfSaleState_descriptor.getNestedTypes().get(0);
internal_static_bloombox_pos_PointOfSaleState_SessionOpen_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PointOfSaleState_SessionOpen_descriptor,
new java.lang.String[] { "OpeningFloat", "Occurred", });
internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor =
internal_static_bloombox_pos_PointOfSaleState_descriptor.getNestedTypes().get(1);
internal_static_bloombox_pos_PointOfSaleState_SessionClose_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PointOfSaleState_SessionClose_descriptor,
new java.lang.String[] { "ClosingCount", "Occurred", });
internal_static_bloombox_pos_PurchaseTicket_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_bloombox_pos_PurchaseTicket_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PurchaseTicket_descriptor,
new java.lang.String[] { "Key", "Version", "Status", "Claim", "Facilitator", "Customer", "Bill", "Item", "Payment", "Action", "Ts", });
internal_static_bloombox_pos_PointOfSaleSession_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_bloombox_pos_PointOfSaleSession_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_bloombox_pos_PointOfSaleSession_descriptor,
new java.lang.String[] { "Uuid", "Status", "User", "Ticket", "State", "Seen", "Created", "Modified", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.collection);
registry.add(core.Datamodel.db);
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.bloombox.schema.identity.AppUserKey.getDescriptor();
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor();
io.opencannabis.schema.commerce.CommercialPurchase.getDescriptor();
io.bloombox.schema.telemetry.context.ApplicationContext.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy