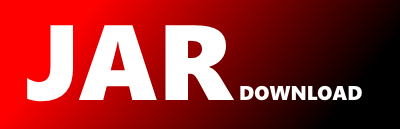
io.opencannabis.schema.commerce.CommercialPurchase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Java RPCAPI client for the Bloombox Cloud.
The newest version!
/*
* Copyright 2019, Momentum Ideas Co.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: commerce/Purchase.proto
package io.opencannabis.schema.commerce;
public final class CommercialPurchase {
private CommercialPurchase() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Enumerates statuses that a purchase transaction may take.
*
*
* Protobuf enum {@code opencannabis.commerce.PurchaseStatus}
*/
public enum PurchaseStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The purchase has been allocated and has not yet begun filling with data.
*
*
* FRESH = 0;
*/
FRESH(0),
/**
*
* The purchase is actively being collaborated on.
*
*
* OPEN = 1;
*/
OPEN(1),
/**
*
* The purchase is saved and closed for later recall.
*
*
* CLOSED = 2;
*/
CLOSED(2),
/**
*
* The purchase was cancelled. This status is terminal.
*
*
* VOIDED = 3;
*/
VOIDED(3),
/**
*
* The purchase was completed and is now closed. This status prevents edits.
*
*
* FINALIZED = 4;
*/
FINALIZED(4),
/**
*
* The purchase record has been reconciled with accounting systems. This status is terminal.
*
*
* RECONCILED = 5;
*/
RECONCILED(5),
UNRECOGNIZED(-1),
;
/**
*
* The purchase has been allocated and has not yet begun filling with data.
*
*
* FRESH = 0;
*/
public static final int FRESH_VALUE = 0;
/**
*
* The purchase is actively being collaborated on.
*
*
* OPEN = 1;
*/
public static final int OPEN_VALUE = 1;
/**
*
* The purchase is saved and closed for later recall.
*
*
* CLOSED = 2;
*/
public static final int CLOSED_VALUE = 2;
/**
*
* The purchase was cancelled. This status is terminal.
*
*
* VOIDED = 3;
*/
public static final int VOIDED_VALUE = 3;
/**
*
* The purchase was completed and is now closed. This status prevents edits.
*
*
* FINALIZED = 4;
*/
public static final int FINALIZED_VALUE = 4;
/**
*
* The purchase record has been reconciled with accounting systems. This status is terminal.
*
*
* RECONCILED = 5;
*/
public static final int RECONCILED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PurchaseStatus valueOf(int value) {
return forNumber(value);
}
public static PurchaseStatus forNumber(int value) {
switch (value) {
case 0: return FRESH;
case 1: return OPEN;
case 2: return CLOSED;
case 3: return VOIDED;
case 4: return FINALIZED;
case 5: return RECONCILED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PurchaseStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PurchaseStatus findValueByNumber(int number) {
return PurchaseStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.getDescriptor().getEnumTypes().get(0);
}
private static final PurchaseStatus[] VALUES = values();
public static PurchaseStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PurchaseStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.PurchaseStatus)
}
/**
*
* Specifies the authority under which a particular purchase occurs.
*
*
* Protobuf enum {@code opencannabis.commerce.PurchaseAuthority}
*/
public enum PurchaseAuthority
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Standard or unspecified authority.
*
*
* STANDARD = 0;
*/
STANDARD(0),
/**
*
* Medical cannabis authority.
*
*
* MEDICAL = 1;
*/
MEDICAL(1),
/**
*
* Adult-use, or recreational, cannabis authority.
*
*
* ADULT_USE = 2;
*/
ADULT_USE(2),
UNRECOGNIZED(-1),
;
/**
*
* Standard or unspecified authority.
*
*
* STANDARD = 0;
*/
public static final int STANDARD_VALUE = 0;
/**
*
* Medical cannabis authority.
*
*
* MEDICAL = 1;
*/
public static final int MEDICAL_VALUE = 1;
/**
*
* Adult-use, or recreational, cannabis authority.
*
*
* ADULT_USE = 2;
*/
public static final int ADULT_USE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PurchaseAuthority valueOf(int value) {
return forNumber(value);
}
public static PurchaseAuthority forNumber(int value) {
switch (value) {
case 0: return STANDARD;
case 1: return MEDICAL;
case 2: return ADULT_USE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PurchaseAuthority> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PurchaseAuthority findValueByNumber(int number) {
return PurchaseAuthority.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.getDescriptor().getEnumTypes().get(1);
}
private static final PurchaseAuthority[] VALUES = values();
public static PurchaseAuthority valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PurchaseAuthority(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.PurchaseAuthority)
}
/**
*
* Specifies a purchase event logged as part of a greater purchase transaction.
*
*
* Protobuf enum {@code opencannabis.commerce.PurchaseEvent}
*/
public enum PurchaseEvent
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The status of the ticket changed.
*
*
* STATUS = 0;
*/
STATUS(0),
/**
*
* The purchase was saved to the cloud for later use.
*
*
* SAVE = 1;
*/
SAVE(1),
/**
*
* The purchase was loaded from the cloud.
*
*
* LOAD = 2;
*/
LOAD(2),
/**
*
* An item was added to the ticket.
*
*
* ITEM_ADDED = 10;
*/
ITEM_ADDED(10),
/**
*
* An item was entirely removed from the ticket.
*
*
* ITEM_REMOVED = 11;
*/
ITEM_REMOVED(11),
/**
*
* An item's desired quantity was changed.
*
*
* ITEM_QUANTITY_CHANGED = 12;
*/
ITEM_QUANTITY_CHANGED(12),
/**
*
* A discount was added to a ticket item.
*
*
* ITEM_DISCOUNT_ADDED = 13;
*/
ITEM_DISCOUNT_ADDED(13),
/**
*
* A discount was removed from a ticket item.
*
*
* ITEM_DISCOUNT_REMOVED = 14;
*/
ITEM_DISCOUNT_REMOVED(14),
/**
*
* The purchase was voided entirely.
*
*
* PURCHASE_VOID = 20;
*/
PURCHASE_VOID(20),
/**
*
* The purchase was completed and finalized.
*
*
* PURCHASE_FINALIZE = 21;
*/
PURCHASE_FINALIZE(21),
UNRECOGNIZED(-1),
;
/**
*
* The status of the ticket changed.
*
*
* STATUS = 0;
*/
public static final int STATUS_VALUE = 0;
/**
*
* The purchase was saved to the cloud for later use.
*
*
* SAVE = 1;
*/
public static final int SAVE_VALUE = 1;
/**
*
* The purchase was loaded from the cloud.
*
*
* LOAD = 2;
*/
public static final int LOAD_VALUE = 2;
/**
*
* An item was added to the ticket.
*
*
* ITEM_ADDED = 10;
*/
public static final int ITEM_ADDED_VALUE = 10;
/**
*
* An item was entirely removed from the ticket.
*
*
* ITEM_REMOVED = 11;
*/
public static final int ITEM_REMOVED_VALUE = 11;
/**
*
* An item's desired quantity was changed.
*
*
* ITEM_QUANTITY_CHANGED = 12;
*/
public static final int ITEM_QUANTITY_CHANGED_VALUE = 12;
/**
*
* A discount was added to a ticket item.
*
*
* ITEM_DISCOUNT_ADDED = 13;
*/
public static final int ITEM_DISCOUNT_ADDED_VALUE = 13;
/**
*
* A discount was removed from a ticket item.
*
*
* ITEM_DISCOUNT_REMOVED = 14;
*/
public static final int ITEM_DISCOUNT_REMOVED_VALUE = 14;
/**
*
* The purchase was voided entirely.
*
*
* PURCHASE_VOID = 20;
*/
public static final int PURCHASE_VOID_VALUE = 20;
/**
*
* The purchase was completed and finalized.
*
*
* PURCHASE_FINALIZE = 21;
*/
public static final int PURCHASE_FINALIZE_VALUE = 21;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PurchaseEvent valueOf(int value) {
return forNumber(value);
}
public static PurchaseEvent forNumber(int value) {
switch (value) {
case 0: return STATUS;
case 1: return SAVE;
case 2: return LOAD;
case 10: return ITEM_ADDED;
case 11: return ITEM_REMOVED;
case 12: return ITEM_QUANTITY_CHANGED;
case 13: return ITEM_DISCOUNT_ADDED;
case 14: return ITEM_DISCOUNT_REMOVED;
case 20: return PURCHASE_VOID;
case 21: return PURCHASE_FINALIZE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PurchaseEvent> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PurchaseEvent findValueByNumber(int number) {
return PurchaseEvent.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.getDescriptor().getEnumTypes().get(2);
}
private static final PurchaseEvent[] VALUES = values();
public static PurchaseEvent valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PurchaseEvent(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:opencannabis.commerce.PurchaseEvent)
}
public interface PurchaseLogEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseLogEntry)
com.google.protobuf.MessageOrBuilder {
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
int getStatusValue();
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus();
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
int getEventValue();
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent getEvent();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
boolean hasInstant();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant();
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder();
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
java.lang.String getSku();
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
com.google.protobuf.ByteString
getSkuBytes();
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
java.lang.String getMessage();
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
*
* Specifies an event that takes place against a Purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseLogEntry}
*/
public static final class PurchaseLogEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseLogEntry)
PurchaseLogEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseLogEntry.newBuilder() to construct.
private PurchaseLogEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseLogEntry() {
status_ = 0;
event_ = 0;
sku_ = "";
message_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseLogEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 16: {
int rawValue = input.readEnum();
event_ = rawValue;
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (instant_ != null) {
subBuilder = instant_.toBuilder();
}
instant_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(instant_);
instant_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
sku_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseLogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.UNRECOGNIZED : result;
}
public static final int EVENT_FIELD_NUMBER = 2;
private int event_;
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public int getEventValue() {
return event_;
}
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent getEvent() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.valueOf(event_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.UNRECOGNIZED : result;
}
public static final int INSTANT_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalInstant.Instant instant_;
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public boolean hasInstant() {
return instant_ != null;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant() {
return instant_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder() {
return getInstant();
}
public static final int SKU_FIELD_NUMBER = 4;
private volatile java.lang.Object sku_;
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public java.lang.String getSku() {
java.lang.Object ref = sku_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sku_ = s;
return s;
}
}
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public com.google.protobuf.ByteString
getSkuBytes() {
java.lang.Object ref = sku_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sku_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MESSAGE_FIELD_NUMBER = 5;
private volatile java.lang.Object message_;
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.FRESH.getNumber()) {
output.writeEnum(1, status_);
}
if (event_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.STATUS.getNumber()) {
output.writeEnum(2, event_);
}
if (instant_ != null) {
output.writeMessage(3, getInstant());
}
if (!getSkuBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, sku_);
}
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, message_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.FRESH.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
if (event_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.STATUS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, event_);
}
if (instant_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getInstant());
}
if (!getSkuBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, sku_);
}
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, message_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry) obj;
if (status_ != other.status_) return false;
if (event_ != other.event_) return false;
if (hasInstant() != other.hasInstant()) return false;
if (hasInstant()) {
if (!getInstant()
.equals(other.getInstant())) return false;
}
if (!getSku()
.equals(other.getSku())) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + EVENT_FIELD_NUMBER;
hash = (53 * hash) + event_;
if (hasInstant()) {
hash = (37 * hash) + INSTANT_FIELD_NUMBER;
hash = (53 * hash) + getInstant().hashCode();
}
hash = (37 * hash) + SKU_FIELD_NUMBER;
hash = (53 * hash) + getSku().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies an event that takes place against a Purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseLogEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseLogEntry)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseLogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
event_ = 0;
if (instantBuilder_ == null) {
instant_ = null;
} else {
instant_ = null;
instantBuilder_ = null;
}
sku_ = "";
message_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry(this);
result.status_ = status_;
result.event_ = event_;
if (instantBuilder_ == null) {
result.instant_ = instant_;
} else {
result.instant_ = instantBuilder_.build();
}
result.sku_ = sku_;
result.message_ = message_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.event_ != 0) {
setEventValue(other.getEventValue());
}
if (other.hasInstant()) {
mergeInstant(other.getInstant());
}
if (!other.getSku().isEmpty()) {
sku_ = other.sku_;
onChanged();
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int status_ = 0;
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus.UNRECOGNIZED : result;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public Builder setStatus(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status the order moved to.
*
*
* .opencannabis.commerce.PurchaseStatus status = 1;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private int event_ = 0;
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public int getEventValue() {
return event_;
}
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public Builder setEventValue(int value) {
event_ = value;
onChanged();
return this;
}
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent getEvent() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.valueOf(event_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent.UNRECOGNIZED : result;
}
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public Builder setEvent(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseEvent value) {
if (value == null) {
throw new NullPointerException();
}
event_ = value.getNumber();
onChanged();
return this;
}
/**
* .opencannabis.commerce.PurchaseEvent event = 2;
*/
public Builder clearEvent() {
event_ = 0;
onChanged();
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant instant_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> instantBuilder_;
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public boolean hasInstant() {
return instantBuilder_ != null || instant_ != null;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getInstant() {
if (instantBuilder_ == null) {
return instant_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
} else {
return instantBuilder_.getMessage();
}
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public Builder setInstant(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (instantBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
instant_ = value;
onChanged();
} else {
instantBuilder_.setMessage(value);
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public Builder setInstant(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (instantBuilder_ == null) {
instant_ = builderForValue.build();
onChanged();
} else {
instantBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public Builder mergeInstant(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (instantBuilder_ == null) {
if (instant_ != null) {
instant_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(instant_).mergeFrom(value).buildPartial();
} else {
instant_ = value;
}
onChanged();
} else {
instantBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public Builder clearInstant() {
if (instantBuilder_ == null) {
instant_ = null;
onChanged();
} else {
instant_ = null;
instantBuilder_ = null;
}
return this;
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getInstantBuilder() {
onChanged();
return getInstantFieldBuilder().getBuilder();
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getInstantOrBuilder() {
if (instantBuilder_ != null) {
return instantBuilder_.getMessageOrBuilder();
} else {
return instant_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : instant_;
}
}
/**
*
* Instant the order was moved to this status.
*
*
* .opencannabis.temporal.Instant instant = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getInstantFieldBuilder() {
if (instantBuilder_ == null) {
instantBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getInstant(),
getParentForChildren(),
isClean());
instant_ = null;
}
return instantBuilder_;
}
private java.lang.Object sku_ = "";
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public java.lang.String getSku() {
java.lang.Object ref = sku_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sku_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public com.google.protobuf.ByteString
getSkuBytes() {
java.lang.Object ref = sku_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sku_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public Builder setSku(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sku_ = value;
onChanged();
return this;
}
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public Builder clearSku() {
sku_ = getDefaultInstance().getSku();
onChanged();
return this;
}
/**
*
* Stock-Keeping-Unit that was related to this purchase event, if applicable.
*
*
* string sku = 4;
*/
public Builder setSkuBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sku_ = value;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* Message or reason given for this status change, if any.
*
*
* string message = 5;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseLogEntry)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseLogEntry)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseLogEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseLogEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseLogEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BillOfChargesOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.BillOfCharges)
com.google.protobuf.MessageOrBuilder {
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
int getStatusValue();
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
io.opencannabis.schema.commerce.Payments.BillStatus getStatus();
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
java.util.List
getTaxList();
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
io.opencannabis.schema.accounting.AccountingTaxes.Tax getTax(int index);
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
int getTaxCount();
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
java.util.List extends io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder>
getTaxOrBuilderList();
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder getTaxOrBuilder(
int index);
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
java.util.List
getDiscountList();
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
io.opencannabis.schema.accounting.CommercialDiscounts.Discount getDiscount(int index);
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
int getDiscountCount();
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
java.util.List extends io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder>
getDiscountOrBuilderList();
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder getDiscountOrBuilder(
int index);
/**
*
* Price paid for this item or transaction at the time of purchase.
*
*
* double price = 4;
*/
double getPrice();
/**
*
* Computed tax amount for this receipt line-item or purchase ticket.
*
*
* double taxes = 5;
*/
double getTaxes();
/**
*
* Computed discount amount for this receipt line-item or purchase ticket.
*
*
* double discounts = 6;
*/
double getDiscounts();
/**
*
* Computed item or ticket subtotal amount (equal to count times price less discounts).
*
*
* double subtotal = 7;
*/
double getSubtotal();
/**
*
* Computed item or ticket total amount (equal to subtotal plus taxes).
*
*
* double total = 8;
*/
double getTotal();
}
/**
*
* Specifies a bill of charges for a given purchase ticket or line item.
*
*
* Protobuf type {@code opencannabis.commerce.BillOfCharges}
*/
public static final class BillOfCharges extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.BillOfCharges)
BillOfChargesOrBuilder {
private static final long serialVersionUID = 0L;
// Use BillOfCharges.newBuilder() to construct.
private BillOfCharges(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BillOfCharges() {
status_ = 0;
tax_ = java.util.Collections.emptyList();
discount_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BillOfCharges(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
tax_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
tax_.add(
input.readMessage(io.opencannabis.schema.accounting.AccountingTaxes.Tax.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
discount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
discount_.add(
input.readMessage(io.opencannabis.schema.accounting.CommercialDiscounts.Discount.parser(), extensionRegistry));
break;
}
case 33: {
price_ = input.readDouble();
break;
}
case 41: {
taxes_ = input.readDouble();
break;
}
case 49: {
discounts_ = input.readDouble();
break;
}
case 57: {
subtotal_ = input.readDouble();
break;
}
case 65: {
total_ = input.readDouble();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
tax_ = java.util.Collections.unmodifiableList(tax_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
discount_ = java.util.Collections.unmodifiableList(discount_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_BillOfCharges_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_BillOfCharges_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.class, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public io.opencannabis.schema.commerce.Payments.BillStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.BillStatus result = io.opencannabis.schema.commerce.Payments.BillStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.BillStatus.UNRECOGNIZED : result;
}
public static final int TAX_FIELD_NUMBER = 2;
private java.util.List tax_;
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public java.util.List getTaxList() {
return tax_;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public java.util.List extends io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder>
getTaxOrBuilderList() {
return tax_;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public int getTaxCount() {
return tax_.size();
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.Tax getTax(int index) {
return tax_.get(index);
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder getTaxOrBuilder(
int index) {
return tax_.get(index);
}
public static final int DISCOUNT_FIELD_NUMBER = 3;
private java.util.List discount_;
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public java.util.List getDiscountList() {
return discount_;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public java.util.List extends io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder>
getDiscountOrBuilderList() {
return discount_;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public int getDiscountCount() {
return discount_.size();
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.Discount getDiscount(int index) {
return discount_.get(index);
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder getDiscountOrBuilder(
int index) {
return discount_.get(index);
}
public static final int PRICE_FIELD_NUMBER = 4;
private double price_;
/**
*
* Price paid for this item or transaction at the time of purchase.
*
*
* double price = 4;
*/
public double getPrice() {
return price_;
}
public static final int TAXES_FIELD_NUMBER = 5;
private double taxes_;
/**
*
* Computed tax amount for this receipt line-item or purchase ticket.
*
*
* double taxes = 5;
*/
public double getTaxes() {
return taxes_;
}
public static final int DISCOUNTS_FIELD_NUMBER = 6;
private double discounts_;
/**
*
* Computed discount amount for this receipt line-item or purchase ticket.
*
*
* double discounts = 6;
*/
public double getDiscounts() {
return discounts_;
}
public static final int SUBTOTAL_FIELD_NUMBER = 7;
private double subtotal_;
/**
*
* Computed item or ticket subtotal amount (equal to count times price less discounts).
*
*
* double subtotal = 7;
*/
public double getSubtotal() {
return subtotal_;
}
public static final int TOTAL_FIELD_NUMBER = 8;
private double total_;
/**
*
* Computed item or ticket total amount (equal to subtotal plus taxes).
*
*
* double total = 8;
*/
public double getTotal() {
return total_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != io.opencannabis.schema.commerce.Payments.BillStatus.SUSPENSE.getNumber()) {
output.writeEnum(1, status_);
}
for (int i = 0; i < tax_.size(); i++) {
output.writeMessage(2, tax_.get(i));
}
for (int i = 0; i < discount_.size(); i++) {
output.writeMessage(3, discount_.get(i));
}
if (price_ != 0D) {
output.writeDouble(4, price_);
}
if (taxes_ != 0D) {
output.writeDouble(5, taxes_);
}
if (discounts_ != 0D) {
output.writeDouble(6, discounts_);
}
if (subtotal_ != 0D) {
output.writeDouble(7, subtotal_);
}
if (total_ != 0D) {
output.writeDouble(8, total_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != io.opencannabis.schema.commerce.Payments.BillStatus.SUSPENSE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
for (int i = 0; i < tax_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, tax_.get(i));
}
for (int i = 0; i < discount_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, discount_.get(i));
}
if (price_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, price_);
}
if (taxes_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, taxes_);
}
if (discounts_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, discounts_);
}
if (subtotal_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(7, subtotal_);
}
if (total_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(8, total_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges other = (io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges) obj;
if (status_ != other.status_) return false;
if (!getTaxList()
.equals(other.getTaxList())) return false;
if (!getDiscountList()
.equals(other.getDiscountList())) return false;
if (java.lang.Double.doubleToLongBits(getPrice())
!= java.lang.Double.doubleToLongBits(
other.getPrice())) return false;
if (java.lang.Double.doubleToLongBits(getTaxes())
!= java.lang.Double.doubleToLongBits(
other.getTaxes())) return false;
if (java.lang.Double.doubleToLongBits(getDiscounts())
!= java.lang.Double.doubleToLongBits(
other.getDiscounts())) return false;
if (java.lang.Double.doubleToLongBits(getSubtotal())
!= java.lang.Double.doubleToLongBits(
other.getSubtotal())) return false;
if (java.lang.Double.doubleToLongBits(getTotal())
!= java.lang.Double.doubleToLongBits(
other.getTotal())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (getTaxCount() > 0) {
hash = (37 * hash) + TAX_FIELD_NUMBER;
hash = (53 * hash) + getTaxList().hashCode();
}
if (getDiscountCount() > 0) {
hash = (37 * hash) + DISCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getDiscountList().hashCode();
}
hash = (37 * hash) + PRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getPrice()));
hash = (37 * hash) + TAXES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTaxes()));
hash = (37 * hash) + DISCOUNTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDiscounts()));
hash = (37 * hash) + SUBTOTAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSubtotal()));
hash = (37 * hash) + TOTAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTotal()));
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a bill of charges for a given purchase ticket or line item.
*
*
* Protobuf type {@code opencannabis.commerce.BillOfCharges}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.BillOfCharges)
io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_BillOfCharges_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_BillOfCharges_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.class, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTaxFieldBuilder();
getDiscountFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
if (taxBuilder_ == null) {
tax_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
taxBuilder_.clear();
}
if (discountBuilder_ == null) {
discount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
discountBuilder_.clear();
}
price_ = 0D;
taxes_ = 0D;
discounts_ = 0D;
subtotal_ = 0D;
total_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_BillOfCharges_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges build() {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges result = new io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.status_ = status_;
if (taxBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
tax_ = java.util.Collections.unmodifiableList(tax_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.tax_ = tax_;
} else {
result.tax_ = taxBuilder_.build();
}
if (discountBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
discount_ = java.util.Collections.unmodifiableList(discount_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.discount_ = discount_;
} else {
result.discount_ = discountBuilder_.build();
}
result.price_ = price_;
result.taxes_ = taxes_;
result.discounts_ = discounts_;
result.subtotal_ = subtotal_;
result.total_ = total_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (taxBuilder_ == null) {
if (!other.tax_.isEmpty()) {
if (tax_.isEmpty()) {
tax_ = other.tax_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTaxIsMutable();
tax_.addAll(other.tax_);
}
onChanged();
}
} else {
if (!other.tax_.isEmpty()) {
if (taxBuilder_.isEmpty()) {
taxBuilder_.dispose();
taxBuilder_ = null;
tax_ = other.tax_;
bitField0_ = (bitField0_ & ~0x00000002);
taxBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTaxFieldBuilder() : null;
} else {
taxBuilder_.addAllMessages(other.tax_);
}
}
}
if (discountBuilder_ == null) {
if (!other.discount_.isEmpty()) {
if (discount_.isEmpty()) {
discount_ = other.discount_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureDiscountIsMutable();
discount_.addAll(other.discount_);
}
onChanged();
}
} else {
if (!other.discount_.isEmpty()) {
if (discountBuilder_.isEmpty()) {
discountBuilder_.dispose();
discountBuilder_ = null;
discount_ = other.discount_;
bitField0_ = (bitField0_ & ~0x00000004);
discountBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDiscountFieldBuilder() : null;
} else {
discountBuilder_.addAllMessages(other.discount_);
}
}
}
if (other.getPrice() != 0D) {
setPrice(other.getPrice());
}
if (other.getTaxes() != 0D) {
setTaxes(other.getTaxes());
}
if (other.getDiscounts() != 0D) {
setDiscounts(other.getDiscounts());
}
if (other.getSubtotal() != 0D) {
setSubtotal(other.getSubtotal());
}
if (other.getTotal() != 0D) {
setTotal(other.getTotal());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int status_ = 0;
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public io.opencannabis.schema.commerce.Payments.BillStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.BillStatus result = io.opencannabis.schema.commerce.Payments.BillStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.BillStatus.UNRECOGNIZED : result;
}
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public Builder setStatus(io.opencannabis.schema.commerce.Payments.BillStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Settlement status for this bill. I.e, whether it has been paid for partially, in full, or not at all.
*
*
* .opencannabis.commerce.BillStatus status = 1;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.util.List tax_ =
java.util.Collections.emptyList();
private void ensureTaxIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
tax_ = new java.util.ArrayList(tax_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.AccountingTaxes.Tax, io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder, io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder> taxBuilder_;
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public java.util.List getTaxList() {
if (taxBuilder_ == null) {
return java.util.Collections.unmodifiableList(tax_);
} else {
return taxBuilder_.getMessageList();
}
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public int getTaxCount() {
if (taxBuilder_ == null) {
return tax_.size();
} else {
return taxBuilder_.getCount();
}
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.Tax getTax(int index) {
if (taxBuilder_ == null) {
return tax_.get(index);
} else {
return taxBuilder_.getMessage(index);
}
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder setTax(
int index, io.opencannabis.schema.accounting.AccountingTaxes.Tax value) {
if (taxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTaxIsMutable();
tax_.set(index, value);
onChanged();
} else {
taxBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder setTax(
int index, io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder builderForValue) {
if (taxBuilder_ == null) {
ensureTaxIsMutable();
tax_.set(index, builderForValue.build());
onChanged();
} else {
taxBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder addTax(io.opencannabis.schema.accounting.AccountingTaxes.Tax value) {
if (taxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTaxIsMutable();
tax_.add(value);
onChanged();
} else {
taxBuilder_.addMessage(value);
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder addTax(
int index, io.opencannabis.schema.accounting.AccountingTaxes.Tax value) {
if (taxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTaxIsMutable();
tax_.add(index, value);
onChanged();
} else {
taxBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder addTax(
io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder builderForValue) {
if (taxBuilder_ == null) {
ensureTaxIsMutable();
tax_.add(builderForValue.build());
onChanged();
} else {
taxBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder addTax(
int index, io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder builderForValue) {
if (taxBuilder_ == null) {
ensureTaxIsMutable();
tax_.add(index, builderForValue.build());
onChanged();
} else {
taxBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder addAllTax(
java.lang.Iterable extends io.opencannabis.schema.accounting.AccountingTaxes.Tax> values) {
if (taxBuilder_ == null) {
ensureTaxIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tax_);
onChanged();
} else {
taxBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder clearTax() {
if (taxBuilder_ == null) {
tax_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
taxBuilder_.clear();
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public Builder removeTax(int index) {
if (taxBuilder_ == null) {
ensureTaxIsMutable();
tax_.remove(index);
onChanged();
} else {
taxBuilder_.remove(index);
}
return this;
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder getTaxBuilder(
int index) {
return getTaxFieldBuilder().getBuilder(index);
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder getTaxOrBuilder(
int index) {
if (taxBuilder_ == null) {
return tax_.get(index); } else {
return taxBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public java.util.List extends io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder>
getTaxOrBuilderList() {
if (taxBuilder_ != null) {
return taxBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tax_);
}
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder addTaxBuilder() {
return getTaxFieldBuilder().addBuilder(
io.opencannabis.schema.accounting.AccountingTaxes.Tax.getDefaultInstance());
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder addTaxBuilder(
int index) {
return getTaxFieldBuilder().addBuilder(
index, io.opencannabis.schema.accounting.AccountingTaxes.Tax.getDefaultInstance());
}
/**
*
* Tax entries applied to this item or ticket.
*
*
* repeated .opencannabis.taxes.Tax tax = 2;
*/
public java.util.List
getTaxBuilderList() {
return getTaxFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.AccountingTaxes.Tax, io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder, io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder>
getTaxFieldBuilder() {
if (taxBuilder_ == null) {
taxBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.AccountingTaxes.Tax, io.opencannabis.schema.accounting.AccountingTaxes.Tax.Builder, io.opencannabis.schema.accounting.AccountingTaxes.TaxOrBuilder>(
tax_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
tax_ = null;
}
return taxBuilder_;
}
private java.util.List discount_ =
java.util.Collections.emptyList();
private void ensureDiscountIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
discount_ = new java.util.ArrayList(discount_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.CommercialDiscounts.Discount, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder, io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder> discountBuilder_;
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public java.util.List getDiscountList() {
if (discountBuilder_ == null) {
return java.util.Collections.unmodifiableList(discount_);
} else {
return discountBuilder_.getMessageList();
}
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public int getDiscountCount() {
if (discountBuilder_ == null) {
return discount_.size();
} else {
return discountBuilder_.getCount();
}
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.Discount getDiscount(int index) {
if (discountBuilder_ == null) {
return discount_.get(index);
} else {
return discountBuilder_.getMessage(index);
}
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder setDiscount(
int index, io.opencannabis.schema.accounting.CommercialDiscounts.Discount value) {
if (discountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiscountIsMutable();
discount_.set(index, value);
onChanged();
} else {
discountBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder setDiscount(
int index, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder builderForValue) {
if (discountBuilder_ == null) {
ensureDiscountIsMutable();
discount_.set(index, builderForValue.build());
onChanged();
} else {
discountBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder addDiscount(io.opencannabis.schema.accounting.CommercialDiscounts.Discount value) {
if (discountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiscountIsMutable();
discount_.add(value);
onChanged();
} else {
discountBuilder_.addMessage(value);
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder addDiscount(
int index, io.opencannabis.schema.accounting.CommercialDiscounts.Discount value) {
if (discountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiscountIsMutable();
discount_.add(index, value);
onChanged();
} else {
discountBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder addDiscount(
io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder builderForValue) {
if (discountBuilder_ == null) {
ensureDiscountIsMutable();
discount_.add(builderForValue.build());
onChanged();
} else {
discountBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder addDiscount(
int index, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder builderForValue) {
if (discountBuilder_ == null) {
ensureDiscountIsMutable();
discount_.add(index, builderForValue.build());
onChanged();
} else {
discountBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder addAllDiscount(
java.lang.Iterable extends io.opencannabis.schema.accounting.CommercialDiscounts.Discount> values) {
if (discountBuilder_ == null) {
ensureDiscountIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, discount_);
onChanged();
} else {
discountBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder clearDiscount() {
if (discountBuilder_ == null) {
discount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
discountBuilder_.clear();
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public Builder removeDiscount(int index) {
if (discountBuilder_ == null) {
ensureDiscountIsMutable();
discount_.remove(index);
onChanged();
} else {
discountBuilder_.remove(index);
}
return this;
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder getDiscountBuilder(
int index) {
return getDiscountFieldBuilder().getBuilder(index);
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder getDiscountOrBuilder(
int index) {
if (discountBuilder_ == null) {
return discount_.get(index); } else {
return discountBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public java.util.List extends io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder>
getDiscountOrBuilderList() {
if (discountBuilder_ != null) {
return discountBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(discount_);
}
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder addDiscountBuilder() {
return getDiscountFieldBuilder().addBuilder(
io.opencannabis.schema.accounting.CommercialDiscounts.Discount.getDefaultInstance());
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder addDiscountBuilder(
int index) {
return getDiscountFieldBuilder().addBuilder(
index, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.getDefaultInstance());
}
/**
*
* Discount entries applied to this item or ticket.
*
*
* repeated .opencannabis.commerce.Discount discount = 3;
*/
public java.util.List
getDiscountBuilderList() {
return getDiscountFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.CommercialDiscounts.Discount, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder, io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder>
getDiscountFieldBuilder() {
if (discountBuilder_ == null) {
discountBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.opencannabis.schema.accounting.CommercialDiscounts.Discount, io.opencannabis.schema.accounting.CommercialDiscounts.Discount.Builder, io.opencannabis.schema.accounting.CommercialDiscounts.DiscountOrBuilder>(
discount_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
discount_ = null;
}
return discountBuilder_;
}
private double price_ ;
/**
*
* Price paid for this item or transaction at the time of purchase.
*
*
* double price = 4;
*/
public double getPrice() {
return price_;
}
/**
*
* Price paid for this item or transaction at the time of purchase.
*
*
* double price = 4;
*/
public Builder setPrice(double value) {
price_ = value;
onChanged();
return this;
}
/**
*
* Price paid for this item or transaction at the time of purchase.
*
*
* double price = 4;
*/
public Builder clearPrice() {
price_ = 0D;
onChanged();
return this;
}
private double taxes_ ;
/**
*
* Computed tax amount for this receipt line-item or purchase ticket.
*
*
* double taxes = 5;
*/
public double getTaxes() {
return taxes_;
}
/**
*
* Computed tax amount for this receipt line-item or purchase ticket.
*
*
* double taxes = 5;
*/
public Builder setTaxes(double value) {
taxes_ = value;
onChanged();
return this;
}
/**
*
* Computed tax amount for this receipt line-item or purchase ticket.
*
*
* double taxes = 5;
*/
public Builder clearTaxes() {
taxes_ = 0D;
onChanged();
return this;
}
private double discounts_ ;
/**
*
* Computed discount amount for this receipt line-item or purchase ticket.
*
*
* double discounts = 6;
*/
public double getDiscounts() {
return discounts_;
}
/**
*
* Computed discount amount for this receipt line-item or purchase ticket.
*
*
* double discounts = 6;
*/
public Builder setDiscounts(double value) {
discounts_ = value;
onChanged();
return this;
}
/**
*
* Computed discount amount for this receipt line-item or purchase ticket.
*
*
* double discounts = 6;
*/
public Builder clearDiscounts() {
discounts_ = 0D;
onChanged();
return this;
}
private double subtotal_ ;
/**
*
* Computed item or ticket subtotal amount (equal to count times price less discounts).
*
*
* double subtotal = 7;
*/
public double getSubtotal() {
return subtotal_;
}
/**
*
* Computed item or ticket subtotal amount (equal to count times price less discounts).
*
*
* double subtotal = 7;
*/
public Builder setSubtotal(double value) {
subtotal_ = value;
onChanged();
return this;
}
/**
*
* Computed item or ticket subtotal amount (equal to count times price less discounts).
*
*
* double subtotal = 7;
*/
public Builder clearSubtotal() {
subtotal_ = 0D;
onChanged();
return this;
}
private double total_ ;
/**
*
* Computed item or ticket total amount (equal to subtotal plus taxes).
*
*
* double total = 8;
*/
public double getTotal() {
return total_;
}
/**
*
* Computed item or ticket total amount (equal to subtotal plus taxes).
*
*
* double total = 8;
*/
public Builder setTotal(double value) {
total_ = value;
onChanged();
return this;
}
/**
*
* Computed item or ticket total amount (equal to subtotal plus taxes).
*
*
* double total = 8;
*/
public Builder clearTotal() {
total_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.BillOfCharges)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.BillOfCharges)
private static final io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BillOfCharges parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BillOfCharges(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TicketItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.TicketItem)
com.google.protobuf.MessageOrBuilder {
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
boolean hasKey();
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
io.opencannabis.schema.inventory.InventoryKey getKey();
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
io.opencannabis.schema.inventory.InventoryKeyOrBuilder getKeyOrBuilder();
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
java.lang.String getSku();
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
com.google.protobuf.ByteString
getSkuBytes();
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
boolean hasItem();
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
io.opencannabis.schema.commerce.OrderItem.Item getItem();
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
boolean hasLine();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getLine();
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getLineOrBuilder();
}
/**
*
* Specifies an individual item purchased as part of a larger commercial purchase ticket, including any variance values
* required to fully specify the item.
*
*
* Protobuf type {@code opencannabis.commerce.TicketItem}
*/
public static final class TicketItem extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.TicketItem)
TicketItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use TicketItem.newBuilder() to construct.
private TicketItem(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TicketItem() {
sku_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TicketItem(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.inventory.InventoryKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.opencannabis.schema.inventory.InventoryKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
sku_ = s;
break;
}
case 26: {
io.opencannabis.schema.commerce.OrderItem.Item.Builder subBuilder = null;
if (item_ != null) {
subBuilder = item_.toBuilder();
}
item_ = input.readMessage(io.opencannabis.schema.commerce.OrderItem.Item.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(item_);
item_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder subBuilder = null;
if (line_ != null) {
subBuilder = line_.toBuilder();
}
line_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(line_);
line_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_TicketItem_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_TicketItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.class, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private io.opencannabis.schema.inventory.InventoryKey key_;
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public io.opencannabis.schema.inventory.InventoryKey getKey() {
return key_ == null ? io.opencannabis.schema.inventory.InventoryKey.getDefaultInstance() : key_;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public io.opencannabis.schema.inventory.InventoryKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int SKU_FIELD_NUMBER = 2;
private volatile java.lang.Object sku_;
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public java.lang.String getSku() {
java.lang.Object ref = sku_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sku_ = s;
return s;
}
}
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public com.google.protobuf.ByteString
getSkuBytes() {
java.lang.Object ref = sku_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sku_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEM_FIELD_NUMBER = 3;
private io.opencannabis.schema.commerce.OrderItem.Item item_;
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public boolean hasItem() {
return item_ != null;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public io.opencannabis.schema.commerce.OrderItem.Item getItem() {
return item_ == null ? io.opencannabis.schema.commerce.OrderItem.Item.getDefaultInstance() : item_;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder() {
return getItem();
}
public static final int LINE_FIELD_NUMBER = 4;
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges line_;
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public boolean hasLine() {
return line_ != null;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getLine() {
return line_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : line_;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getLineOrBuilder() {
return getLine();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (!getSkuBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, sku_);
}
if (item_ != null) {
output.writeMessage(3, getItem());
}
if (line_ != null) {
output.writeMessage(4, getLine());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (!getSkuBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, sku_);
}
if (item_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getItem());
}
if (line_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getLine());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.TicketItem)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem other = (io.opencannabis.schema.commerce.CommercialPurchase.TicketItem) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (!getSku()
.equals(other.getSku())) return false;
if (hasItem() != other.hasItem()) return false;
if (hasItem()) {
if (!getItem()
.equals(other.getItem())) return false;
}
if (hasLine() != other.hasLine()) return false;
if (hasLine()) {
if (!getLine()
.equals(other.getLine())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (37 * hash) + SKU_FIELD_NUMBER;
hash = (53 * hash) + getSku().hashCode();
if (hasItem()) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItem().hashCode();
}
if (hasLine()) {
hash = (37 * hash) + LINE_FIELD_NUMBER;
hash = (53 * hash) + getLine().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.TicketItem prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies an individual item purchased as part of a larger commercial purchase ticket, including any variance values
* required to fully specify the item.
*
*
* Protobuf type {@code opencannabis.commerce.TicketItem}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.TicketItem)
io.opencannabis.schema.commerce.CommercialPurchase.TicketItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_TicketItem_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_TicketItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.class, io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
sku_ = "";
if (itemBuilder_ == null) {
item_ = null;
} else {
item_ = null;
itemBuilder_ = null;
}
if (lineBuilder_ == null) {
line_ = null;
} else {
line_ = null;
lineBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_TicketItem_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem build() {
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem result = new io.opencannabis.schema.commerce.CommercialPurchase.TicketItem(this);
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
result.sku_ = sku_;
if (itemBuilder_ == null) {
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
if (lineBuilder_ == null) {
result.line_ = line_;
} else {
result.line_ = lineBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.TicketItem) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.TicketItem)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.TicketItem other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.TicketItem.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (!other.getSku().isEmpty()) {
sku_ = other.sku_;
onChanged();
}
if (other.hasItem()) {
mergeItem(other.getItem());
}
if (other.hasLine()) {
mergeLine(other.getLine());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.TicketItem parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.TicketItem) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.inventory.InventoryKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.inventory.InventoryKey, io.opencannabis.schema.inventory.InventoryKey.Builder, io.opencannabis.schema.inventory.InventoryKeyOrBuilder> keyBuilder_;
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public io.opencannabis.schema.inventory.InventoryKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.opencannabis.schema.inventory.InventoryKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public Builder setKey(io.opencannabis.schema.inventory.InventoryKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public Builder setKey(
io.opencannabis.schema.inventory.InventoryKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public Builder mergeKey(io.opencannabis.schema.inventory.InventoryKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.opencannabis.schema.inventory.InventoryKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public io.opencannabis.schema.inventory.InventoryKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
public io.opencannabis.schema.inventory.InventoryKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.opencannabis.schema.inventory.InventoryKey.getDefaultInstance() : key_;
}
}
/**
*
* Key specifying the address of a specific inventory item.
*
*
* .opencannabis.inventory.InventoryKey key = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.inventory.InventoryKey, io.opencannabis.schema.inventory.InventoryKey.Builder, io.opencannabis.schema.inventory.InventoryKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.inventory.InventoryKey, io.opencannabis.schema.inventory.InventoryKey.Builder, io.opencannabis.schema.inventory.InventoryKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private java.lang.Object sku_ = "";
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public java.lang.String getSku() {
java.lang.Object ref = sku_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sku_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public com.google.protobuf.ByteString
getSkuBytes() {
java.lang.Object ref = sku_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sku_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public Builder setSku(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sku_ = value;
onChanged();
return this;
}
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public Builder clearSku() {
sku_ = getDefaultInstance().getSku();
onChanged();
return this;
}
/**
*
* Stock-Keeping-Unit that was scanned as part of this item being onboarded onto a commercial purchase ticket.
*
*
* string sku = 2;
*/
public Builder setSkuBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sku_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.OrderItem.Item item_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder> itemBuilder_;
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public boolean hasItem() {
return itemBuilder_ != null || item_ != null;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public io.opencannabis.schema.commerce.OrderItem.Item getItem() {
if (itemBuilder_ == null) {
return item_ == null ? io.opencannabis.schema.commerce.OrderItem.Item.getDefaultInstance() : item_;
} else {
return itemBuilder_.getMessage();
}
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public Builder setItem(io.opencannabis.schema.commerce.OrderItem.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
item_ = value;
onChanged();
} else {
itemBuilder_.setMessage(value);
}
return this;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public Builder setItem(
io.opencannabis.schema.commerce.OrderItem.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
item_ = builderForValue.build();
onChanged();
} else {
itemBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public Builder mergeItem(io.opencannabis.schema.commerce.OrderItem.Item value) {
if (itemBuilder_ == null) {
if (item_ != null) {
item_ =
io.opencannabis.schema.commerce.OrderItem.Item.newBuilder(item_).mergeFrom(value).buildPartial();
} else {
item_ = value;
}
onChanged();
} else {
itemBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = null;
onChanged();
} else {
item_ = null;
itemBuilder_ = null;
}
return this;
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public io.opencannabis.schema.commerce.OrderItem.Item.Builder getItemBuilder() {
onChanged();
return getItemFieldBuilder().getBuilder();
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
public io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder getItemOrBuilder() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilder();
} else {
return item_ == null ?
io.opencannabis.schema.commerce.OrderItem.Item.getDefaultInstance() : item_;
}
}
/**
*
* Specifies the commercial item
*
*
* .opencannabis.commerce.Item item = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.OrderItem.Item, io.opencannabis.schema.commerce.OrderItem.Item.Builder, io.opencannabis.schema.commerce.OrderItem.ItemOrBuilder>(
getItem(),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges line_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder> lineBuilder_;
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public boolean hasLine() {
return lineBuilder_ != null || line_ != null;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges getLine() {
if (lineBuilder_ == null) {
return line_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : line_;
} else {
return lineBuilder_.getMessage();
}
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public Builder setLine(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (lineBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
line_ = value;
onChanged();
} else {
lineBuilder_.setMessage(value);
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public Builder setLine(
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder builderForValue) {
if (lineBuilder_ == null) {
line_ = builderForValue.build();
onChanged();
} else {
lineBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public Builder mergeLine(io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges value) {
if (lineBuilder_ == null) {
if (line_ != null) {
line_ =
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.newBuilder(line_).mergeFrom(value).buildPartial();
} else {
line_ = value;
}
onChanged();
} else {
lineBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public Builder clearLine() {
if (lineBuilder_ == null) {
line_ = null;
onChanged();
} else {
line_ = null;
lineBuilder_ = null;
}
return this;
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder getLineBuilder() {
onChanged();
return getLineFieldBuilder().getBuilder();
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder getLineOrBuilder() {
if (lineBuilder_ != null) {
return lineBuilder_.getMessageOrBuilder();
} else {
return line_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.getDefaultInstance() : line_;
}
}
/**
*
* Line-item bill of charges, or purchase sums. Includes taxes and discounts.
*
*
* .opencannabis.commerce.BillOfCharges line = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>
getLineFieldBuilder() {
if (lineBuilder_ == null) {
lineBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges, io.opencannabis.schema.commerce.CommercialPurchase.BillOfCharges.Builder, io.opencannabis.schema.commerce.CommercialPurchase.BillOfChargesOrBuilder>(
getLine(),
getParentForChildren(),
isClean());
line_ = null;
}
return lineBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.TicketItem)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.TicketItem)
private static final io.opencannabis.schema.commerce.CommercialPurchase.TicketItem DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.TicketItem();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TicketItem parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TicketItem(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.TicketItem getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseTimestampsOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseTimestamps)
com.google.protobuf.MessageOrBuilder {
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
boolean hasEstablished();
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getEstablished();
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstablishedOrBuilder();
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
boolean hasCreated();
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated();
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder();
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
boolean hasModified();
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getModified();
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder();
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
boolean hasExecuted();
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getExecuted();
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getExecutedOrBuilder();
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
boolean hasFinalized();
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.Instant getFinalized();
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getFinalizedOrBuilder();
}
/**
*
* Specifies a set of timestamps recorded for each purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseTimestamps}
*/
public static final class PurchaseTimestamps extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseTimestamps)
PurchaseTimestampsOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseTimestamps.newBuilder() to construct.
private PurchaseTimestamps(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseTimestamps() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseTimestamps(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (established_ != null) {
subBuilder = established_.toBuilder();
}
established_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(established_);
established_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (created_ != null) {
subBuilder = created_.toBuilder();
}
created_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(created_);
created_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (modified_ != null) {
subBuilder = modified_.toBuilder();
}
modified_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(modified_);
modified_ = subBuilder.buildPartial();
}
break;
}
case 34: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (executed_ != null) {
subBuilder = executed_.toBuilder();
}
executed_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(executed_);
executed_ = subBuilder.buildPartial();
}
break;
}
case 42: {
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder subBuilder = null;
if (finalized_ != null) {
subBuilder = finalized_.toBuilder();
}
finalized_ = input.readMessage(io.opencannabis.schema.temporal.TemporalInstant.Instant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(finalized_);
finalized_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder.class);
}
public static final int ESTABLISHED_FIELD_NUMBER = 1;
private io.opencannabis.schema.temporal.TemporalInstant.Instant established_;
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public boolean hasEstablished() {
return established_ != null;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstablished() {
return established_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : established_;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstablishedOrBuilder() {
return getEstablished();
}
public static final int CREATED_FIELD_NUMBER = 2;
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public boolean hasCreated() {
return created_ != null;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
return getCreated();
}
public static final int MODIFIED_FIELD_NUMBER = 3;
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public boolean hasModified() {
return modified_ != null;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
return getModified();
}
public static final int EXECUTED_FIELD_NUMBER = 4;
private io.opencannabis.schema.temporal.TemporalInstant.Instant executed_;
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public boolean hasExecuted() {
return executed_ != null;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getExecuted() {
return executed_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : executed_;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getExecutedOrBuilder() {
return getExecuted();
}
public static final int FINALIZED_FIELD_NUMBER = 5;
private io.opencannabis.schema.temporal.TemporalInstant.Instant finalized_;
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public boolean hasFinalized() {
return finalized_ != null;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getFinalized() {
return finalized_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : finalized_;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getFinalizedOrBuilder() {
return getFinalized();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (established_ != null) {
output.writeMessage(1, getEstablished());
}
if (created_ != null) {
output.writeMessage(2, getCreated());
}
if (modified_ != null) {
output.writeMessage(3, getModified());
}
if (executed_ != null) {
output.writeMessage(4, getExecuted());
}
if (finalized_ != null) {
output.writeMessage(5, getFinalized());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (established_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getEstablished());
}
if (created_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCreated());
}
if (modified_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getModified());
}
if (executed_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getExecuted());
}
if (finalized_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getFinalized());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps) obj;
if (hasEstablished() != other.hasEstablished()) return false;
if (hasEstablished()) {
if (!getEstablished()
.equals(other.getEstablished())) return false;
}
if (hasCreated() != other.hasCreated()) return false;
if (hasCreated()) {
if (!getCreated()
.equals(other.getCreated())) return false;
}
if (hasModified() != other.hasModified()) return false;
if (hasModified()) {
if (!getModified()
.equals(other.getModified())) return false;
}
if (hasExecuted() != other.hasExecuted()) return false;
if (hasExecuted()) {
if (!getExecuted()
.equals(other.getExecuted())) return false;
}
if (hasFinalized() != other.hasFinalized()) return false;
if (hasFinalized()) {
if (!getFinalized()
.equals(other.getFinalized())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEstablished()) {
hash = (37 * hash) + ESTABLISHED_FIELD_NUMBER;
hash = (53 * hash) + getEstablished().hashCode();
}
if (hasCreated()) {
hash = (37 * hash) + CREATED_FIELD_NUMBER;
hash = (53 * hash) + getCreated().hashCode();
}
if (hasModified()) {
hash = (37 * hash) + MODIFIED_FIELD_NUMBER;
hash = (53 * hash) + getModified().hashCode();
}
if (hasExecuted()) {
hash = (37 * hash) + EXECUTED_FIELD_NUMBER;
hash = (53 * hash) + getExecuted().hashCode();
}
if (hasFinalized()) {
hash = (37 * hash) + FINALIZED_FIELD_NUMBER;
hash = (53 * hash) + getFinalized().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a set of timestamps recorded for each purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseTimestamps}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseTimestamps)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestampsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (establishedBuilder_ == null) {
established_ = null;
} else {
established_ = null;
establishedBuilder_ = null;
}
if (createdBuilder_ == null) {
created_ = null;
} else {
created_ = null;
createdBuilder_ = null;
}
if (modifiedBuilder_ == null) {
modified_ = null;
} else {
modified_ = null;
modifiedBuilder_ = null;
}
if (executedBuilder_ == null) {
executed_ = null;
} else {
executed_ = null;
executedBuilder_ = null;
}
if (finalizedBuilder_ == null) {
finalized_ = null;
} else {
finalized_ = null;
finalizedBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps(this);
if (establishedBuilder_ == null) {
result.established_ = established_;
} else {
result.established_ = establishedBuilder_.build();
}
if (createdBuilder_ == null) {
result.created_ = created_;
} else {
result.created_ = createdBuilder_.build();
}
if (modifiedBuilder_ == null) {
result.modified_ = modified_;
} else {
result.modified_ = modifiedBuilder_.build();
}
if (executedBuilder_ == null) {
result.executed_ = executed_;
} else {
result.executed_ = executedBuilder_.build();
}
if (finalizedBuilder_ == null) {
result.finalized_ = finalized_;
} else {
result.finalized_ = finalizedBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps.getDefaultInstance()) return this;
if (other.hasEstablished()) {
mergeEstablished(other.getEstablished());
}
if (other.hasCreated()) {
mergeCreated(other.getCreated());
}
if (other.hasModified()) {
mergeModified(other.getModified());
}
if (other.hasExecuted()) {
mergeExecuted(other.getExecuted());
}
if (other.hasFinalized()) {
mergeFinalized(other.getFinalized());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant established_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> establishedBuilder_;
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public boolean hasEstablished() {
return establishedBuilder_ != null || established_ != null;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getEstablished() {
if (establishedBuilder_ == null) {
return established_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : established_;
} else {
return establishedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public Builder setEstablished(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (establishedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
established_ = value;
onChanged();
} else {
establishedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public Builder setEstablished(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (establishedBuilder_ == null) {
established_ = builderForValue.build();
onChanged();
} else {
establishedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public Builder mergeEstablished(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (establishedBuilder_ == null) {
if (established_ != null) {
established_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(established_).mergeFrom(value).buildPartial();
} else {
established_ = value;
}
onChanged();
} else {
establishedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public Builder clearEstablished() {
if (establishedBuilder_ == null) {
established_ = null;
onChanged();
} else {
established_ = null;
establishedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getEstablishedBuilder() {
onChanged();
return getEstablishedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getEstablishedOrBuilder() {
if (establishedBuilder_ != null) {
return establishedBuilder_.getMessageOrBuilder();
} else {
return established_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : established_;
}
}
/**
*
* Timestamp for when a particular purchase was first allocated.
*
*
* .opencannabis.temporal.Instant established = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getEstablishedFieldBuilder() {
if (establishedBuilder_ == null) {
establishedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getEstablished(),
getParentForChildren(),
isClean());
established_ = null;
}
return establishedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant created_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> createdBuilder_;
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public boolean hasCreated() {
return createdBuilder_ != null || created_ != null;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getCreated() {
if (createdBuilder_ == null) {
return created_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
} else {
return createdBuilder_.getMessage();
}
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public Builder setCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
created_ = value;
onChanged();
} else {
createdBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public Builder setCreated(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (createdBuilder_ == null) {
created_ = builderForValue.build();
onChanged();
} else {
createdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public Builder mergeCreated(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (createdBuilder_ == null) {
if (created_ != null) {
created_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(created_).mergeFrom(value).buildPartial();
} else {
created_ = value;
}
onChanged();
} else {
createdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public Builder clearCreated() {
if (createdBuilder_ == null) {
created_ = null;
onChanged();
} else {
created_ = null;
createdBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getCreatedBuilder() {
onChanged();
return getCreatedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getCreatedOrBuilder() {
if (createdBuilder_ != null) {
return createdBuilder_.getMessageOrBuilder();
} else {
return created_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : created_;
}
}
/**
*
* Timestamp for when a particular purchase was first saved.
*
*
* .opencannabis.temporal.Instant created = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getCreatedFieldBuilder() {
if (createdBuilder_ == null) {
createdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getCreated(),
getParentForChildren(),
isClean());
created_ = null;
}
return createdBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant modified_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> modifiedBuilder_;
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public boolean hasModified() {
return modifiedBuilder_ != null || modified_ != null;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getModified() {
if (modifiedBuilder_ == null) {
return modified_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
} else {
return modifiedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public Builder setModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
modified_ = value;
onChanged();
} else {
modifiedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public Builder setModified(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (modifiedBuilder_ == null) {
modified_ = builderForValue.build();
onChanged();
} else {
modifiedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public Builder mergeModified(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (modifiedBuilder_ == null) {
if (modified_ != null) {
modified_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(modified_).mergeFrom(value).buildPartial();
} else {
modified_ = value;
}
onChanged();
} else {
modifiedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public Builder clearModified() {
if (modifiedBuilder_ == null) {
modified_ = null;
onChanged();
} else {
modified_ = null;
modifiedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getModifiedBuilder() {
onChanged();
return getModifiedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getModifiedOrBuilder() {
if (modifiedBuilder_ != null) {
return modifiedBuilder_.getMessageOrBuilder();
} else {
return modified_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : modified_;
}
}
/**
*
* Timestamp for when a particular purchase was last modified.
*
*
* .opencannabis.temporal.Instant modified = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getModifiedFieldBuilder() {
if (modifiedBuilder_ == null) {
modifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getModified(),
getParentForChildren(),
isClean());
modified_ = null;
}
return modifiedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant executed_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> executedBuilder_;
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public boolean hasExecuted() {
return executedBuilder_ != null || executed_ != null;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getExecuted() {
if (executedBuilder_ == null) {
return executed_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : executed_;
} else {
return executedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public Builder setExecuted(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (executedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
executed_ = value;
onChanged();
} else {
executedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public Builder setExecuted(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (executedBuilder_ == null) {
executed_ = builderForValue.build();
onChanged();
} else {
executedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public Builder mergeExecuted(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (executedBuilder_ == null) {
if (executed_ != null) {
executed_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(executed_).mergeFrom(value).buildPartial();
} else {
executed_ = value;
}
onChanged();
} else {
executedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public Builder clearExecuted() {
if (executedBuilder_ == null) {
executed_ = null;
onChanged();
} else {
executed_ = null;
executedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getExecutedBuilder() {
onChanged();
return getExecutedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getExecutedOrBuilder() {
if (executedBuilder_ != null) {
return executedBuilder_.getMessageOrBuilder();
} else {
return executed_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : executed_;
}
}
/**
*
* Timestamp for when a purchase was conducted and paid for.
*
*
* .opencannabis.temporal.Instant executed = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getExecutedFieldBuilder() {
if (executedBuilder_ == null) {
executedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getExecuted(),
getParentForChildren(),
isClean());
executed_ = null;
}
return executedBuilder_;
}
private io.opencannabis.schema.temporal.TemporalInstant.Instant finalized_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder> finalizedBuilder_;
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public boolean hasFinalized() {
return finalizedBuilder_ != null || finalized_ != null;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant getFinalized() {
if (finalizedBuilder_ == null) {
return finalized_ == null ? io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : finalized_;
} else {
return finalizedBuilder_.getMessage();
}
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public Builder setFinalized(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (finalizedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
finalized_ = value;
onChanged();
} else {
finalizedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public Builder setFinalized(
io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder builderForValue) {
if (finalizedBuilder_ == null) {
finalized_ = builderForValue.build();
onChanged();
} else {
finalizedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public Builder mergeFinalized(io.opencannabis.schema.temporal.TemporalInstant.Instant value) {
if (finalizedBuilder_ == null) {
if (finalized_ != null) {
finalized_ =
io.opencannabis.schema.temporal.TemporalInstant.Instant.newBuilder(finalized_).mergeFrom(value).buildPartial();
} else {
finalized_ = value;
}
onChanged();
} else {
finalizedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public Builder clearFinalized() {
if (finalizedBuilder_ == null) {
finalized_ = null;
onChanged();
} else {
finalized_ = null;
finalizedBuilder_ = null;
}
return this;
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder getFinalizedBuilder() {
onChanged();
return getFinalizedFieldBuilder().getBuilder();
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
public io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder getFinalizedOrBuilder() {
if (finalizedBuilder_ != null) {
return finalizedBuilder_.getMessageOrBuilder();
} else {
return finalized_ == null ?
io.opencannabis.schema.temporal.TemporalInstant.Instant.getDefaultInstance() : finalized_;
}
}
/**
*
* Timestamp for when a purchase was finalized after any post-purchase edits.
*
*
* .opencannabis.temporal.Instant finalized = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>
getFinalizedFieldBuilder() {
if (finalizedBuilder_ == null) {
finalizedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.temporal.TemporalInstant.Instant, io.opencannabis.schema.temporal.TemporalInstant.Instant.Builder, io.opencannabis.schema.temporal.TemporalInstant.InstantOrBuilder>(
getFinalized(),
getParentForChildren(),
isClean());
finalized_ = null;
}
return finalizedBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseTimestamps)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseTimestamps)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseTimestamps parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseTimestamps(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseTimestamps getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
java.lang.String getUuid();
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUuidBytes();
}
/**
*
* Specifies a key that uniquely addresses a purchase made by a consumer.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseKey}
*/
public static final class PurchaseKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseKey)
PurchaseKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseKey.newBuilder() to construct.
private PurchaseKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseKey() {
uuid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder.class);
}
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a key that uniquely addresses a purchase made by a consumer.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseKey)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseKey_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey(this);
result.uuid_ = uuid_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object uuid_ = "";
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* String UUID generated to address this purchase. Generally allocated client-side.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseKey)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseKey)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseSignatureOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseSignature)
com.google.protobuf.MessageOrBuilder {
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
java.lang.String getNonce();
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
com.google.protobuf.ByteString
getNonceBytes();
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
boolean hasFacilitator();
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
io.opencannabis.schema.crypto.Signature getFacilitator();
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
io.opencannabis.schema.crypto.SignatureOrBuilder getFacilitatorOrBuilder();
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
boolean hasCustomer();
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
io.opencannabis.schema.crypto.Signature getCustomer();
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
io.opencannabis.schema.crypto.SignatureOrBuilder getCustomerOrBuilder();
}
/**
*
* Specifies a cryptographic signature attached to a commercial/retail purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseSignature}
*/
public static final class PurchaseSignature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseSignature)
PurchaseSignatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseSignature.newBuilder() to construct.
private PurchaseSignature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseSignature() {
nonce_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseSignature(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
nonce_ = s;
break;
}
case 18: {
io.opencannabis.schema.crypto.Signature.Builder subBuilder = null;
if (facilitator_ != null) {
subBuilder = facilitator_.toBuilder();
}
facilitator_ = input.readMessage(io.opencannabis.schema.crypto.Signature.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(facilitator_);
facilitator_ = subBuilder.buildPartial();
}
break;
}
case 26: {
io.opencannabis.schema.crypto.Signature.Builder subBuilder = null;
if (customer_ != null) {
subBuilder = customer_.toBuilder();
}
customer_ = input.readMessage(io.opencannabis.schema.crypto.Signature.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(customer_);
customer_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseSignature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseSignature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder.class);
}
public static final int NONCE_FIELD_NUMBER = 1;
private volatile java.lang.Object nonce_;
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public java.lang.String getNonce() {
java.lang.Object ref = nonce_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nonce_ = s;
return s;
}
}
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public com.google.protobuf.ByteString
getNonceBytes() {
java.lang.Object ref = nonce_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nonce_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FACILITATOR_FIELD_NUMBER = 2;
private io.opencannabis.schema.crypto.Signature facilitator_;
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public boolean hasFacilitator() {
return facilitator_ != null;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public io.opencannabis.schema.crypto.Signature getFacilitator() {
return facilitator_ == null ? io.opencannabis.schema.crypto.Signature.getDefaultInstance() : facilitator_;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public io.opencannabis.schema.crypto.SignatureOrBuilder getFacilitatorOrBuilder() {
return getFacilitator();
}
public static final int CUSTOMER_FIELD_NUMBER = 3;
private io.opencannabis.schema.crypto.Signature customer_;
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public boolean hasCustomer() {
return customer_ != null;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public io.opencannabis.schema.crypto.Signature getCustomer() {
return customer_ == null ? io.opencannabis.schema.crypto.Signature.getDefaultInstance() : customer_;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public io.opencannabis.schema.crypto.SignatureOrBuilder getCustomerOrBuilder() {
return getCustomer();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNonceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nonce_);
}
if (facilitator_ != null) {
output.writeMessage(2, getFacilitator());
}
if (customer_ != null) {
output.writeMessage(3, getCustomer());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNonceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nonce_);
}
if (facilitator_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getFacilitator());
}
if (customer_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCustomer());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature) obj;
if (!getNonce()
.equals(other.getNonce())) return false;
if (hasFacilitator() != other.hasFacilitator()) return false;
if (hasFacilitator()) {
if (!getFacilitator()
.equals(other.getFacilitator())) return false;
}
if (hasCustomer() != other.hasCustomer()) return false;
if (hasCustomer()) {
if (!getCustomer()
.equals(other.getCustomer())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NONCE_FIELD_NUMBER;
hash = (53 * hash) + getNonce().hashCode();
if (hasFacilitator()) {
hash = (37 * hash) + FACILITATOR_FIELD_NUMBER;
hash = (53 * hash) + getFacilitator().hashCode();
}
if (hasCustomer()) {
hash = (37 * hash) + CUSTOMER_FIELD_NUMBER;
hash = (53 * hash) + getCustomer().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a cryptographic signature attached to a commercial/retail purchase.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseSignature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseSignature)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseSignature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseSignature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
nonce_ = "";
if (facilitatorBuilder_ == null) {
facilitator_ = null;
} else {
facilitator_ = null;
facilitatorBuilder_ = null;
}
if (customerBuilder_ == null) {
customer_ = null;
} else {
customer_ = null;
customerBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseSignature_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature(this);
result.nonce_ = nonce_;
if (facilitatorBuilder_ == null) {
result.facilitator_ = facilitator_;
} else {
result.facilitator_ = facilitatorBuilder_.build();
}
if (customerBuilder_ == null) {
result.customer_ = customer_;
} else {
result.customer_ = customerBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance()) return this;
if (!other.getNonce().isEmpty()) {
nonce_ = other.nonce_;
onChanged();
}
if (other.hasFacilitator()) {
mergeFacilitator(other.getFacilitator());
}
if (other.hasCustomer()) {
mergeCustomer(other.getCustomer());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object nonce_ = "";
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public java.lang.String getNonce() {
java.lang.Object ref = nonce_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nonce_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public com.google.protobuf.ByteString
getNonceBytes() {
java.lang.Object ref = nonce_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nonce_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public Builder setNonce(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nonce_ = value;
onChanged();
return this;
}
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public Builder clearNonce() {
nonce_ = getDefaultInstance().getNonce();
onChanged();
return this;
}
/**
*
* Verification number allocated for this transaction from a device under the customer's control.
*
*
* string nonce = 1;
*/
public Builder setNonceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nonce_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.crypto.Signature facilitator_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder> facilitatorBuilder_;
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public boolean hasFacilitator() {
return facilitatorBuilder_ != null || facilitator_ != null;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public io.opencannabis.schema.crypto.Signature getFacilitator() {
if (facilitatorBuilder_ == null) {
return facilitator_ == null ? io.opencannabis.schema.crypto.Signature.getDefaultInstance() : facilitator_;
} else {
return facilitatorBuilder_.getMessage();
}
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public Builder setFacilitator(io.opencannabis.schema.crypto.Signature value) {
if (facilitatorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
facilitator_ = value;
onChanged();
} else {
facilitatorBuilder_.setMessage(value);
}
return this;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public Builder setFacilitator(
io.opencannabis.schema.crypto.Signature.Builder builderForValue) {
if (facilitatorBuilder_ == null) {
facilitator_ = builderForValue.build();
onChanged();
} else {
facilitatorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public Builder mergeFacilitator(io.opencannabis.schema.crypto.Signature value) {
if (facilitatorBuilder_ == null) {
if (facilitator_ != null) {
facilitator_ =
io.opencannabis.schema.crypto.Signature.newBuilder(facilitator_).mergeFrom(value).buildPartial();
} else {
facilitator_ = value;
}
onChanged();
} else {
facilitatorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public Builder clearFacilitator() {
if (facilitatorBuilder_ == null) {
facilitator_ = null;
onChanged();
} else {
facilitator_ = null;
facilitatorBuilder_ = null;
}
return this;
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public io.opencannabis.schema.crypto.Signature.Builder getFacilitatorBuilder() {
onChanged();
return getFacilitatorFieldBuilder().getBuilder();
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
public io.opencannabis.schema.crypto.SignatureOrBuilder getFacilitatorOrBuilder() {
if (facilitatorBuilder_ != null) {
return facilitatorBuilder_.getMessageOrBuilder();
} else {
return facilitator_ == null ?
io.opencannabis.schema.crypto.Signature.getDefaultInstance() : facilitator_;
}
}
/**
*
* Cryptographic signature issued by the point-of-sale device for this transaction.
*
*
* .opencannabis.crypto.Signature facilitator = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder>
getFacilitatorFieldBuilder() {
if (facilitatorBuilder_ == null) {
facilitatorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder>(
getFacilitator(),
getParentForChildren(),
isClean());
facilitator_ = null;
}
return facilitatorBuilder_;
}
private io.opencannabis.schema.crypto.Signature customer_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder> customerBuilder_;
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public boolean hasCustomer() {
return customerBuilder_ != null || customer_ != null;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public io.opencannabis.schema.crypto.Signature getCustomer() {
if (customerBuilder_ == null) {
return customer_ == null ? io.opencannabis.schema.crypto.Signature.getDefaultInstance() : customer_;
} else {
return customerBuilder_.getMessage();
}
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public Builder setCustomer(io.opencannabis.schema.crypto.Signature value) {
if (customerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
customer_ = value;
onChanged();
} else {
customerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public Builder setCustomer(
io.opencannabis.schema.crypto.Signature.Builder builderForValue) {
if (customerBuilder_ == null) {
customer_ = builderForValue.build();
onChanged();
} else {
customerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public Builder mergeCustomer(io.opencannabis.schema.crypto.Signature value) {
if (customerBuilder_ == null) {
if (customer_ != null) {
customer_ =
io.opencannabis.schema.crypto.Signature.newBuilder(customer_).mergeFrom(value).buildPartial();
} else {
customer_ = value;
}
onChanged();
} else {
customerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public Builder clearCustomer() {
if (customerBuilder_ == null) {
customer_ = null;
onChanged();
} else {
customer_ = null;
customerBuilder_ = null;
}
return this;
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public io.opencannabis.schema.crypto.Signature.Builder getCustomerBuilder() {
onChanged();
return getCustomerFieldBuilder().getBuilder();
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
public io.opencannabis.schema.crypto.SignatureOrBuilder getCustomerOrBuilder() {
if (customerBuilder_ != null) {
return customerBuilder_.getMessageOrBuilder();
} else {
return customer_ == null ?
io.opencannabis.schema.crypto.Signature.getDefaultInstance() : customer_;
}
}
/**
*
* Cryptographic signature issued by the customer for this transaction.
*
*
* .opencannabis.crypto.Signature customer = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder>
getCustomerFieldBuilder() {
if (customerBuilder_ == null) {
customerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.crypto.Signature, io.opencannabis.schema.crypto.Signature.Builder, io.opencannabis.schema.crypto.SignatureOrBuilder>(
getCustomer(),
getParentForChildren(),
isClean());
customer_ = null;
}
return customerBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseSignature)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseSignature)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseSignature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseSignature(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseCustomerOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseCustomer)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
java.lang.String getUniqueId();
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
com.google.protobuf.ByteString
getUniqueIdBytes();
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
boolean hasSignature();
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature();
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder();
}
/**
*
* Specifies combined information regarding the customer who made a particular purchase. This includes their digitally
* identifying information, their legal identification, and the authority under which the purchase occurred.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseCustomer}
*/
public static final class PurchaseCustomer extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseCustomer)
PurchaseCustomerOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseCustomer.newBuilder() to construct.
private PurchaseCustomer(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseCustomer() {
uniqueId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseCustomer(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uniqueId_ = s;
break;
}
case 18: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder subBuilder = null;
if (signature_ != null) {
subBuilder = signature_.toBuilder();
}
signature_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signature_);
signature_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseCustomer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseCustomer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder.class);
}
public static final int UNIQUE_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object uniqueId_;
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public java.lang.String getUniqueId() {
java.lang.Object ref = uniqueId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uniqueId_ = s;
return s;
}
}
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public com.google.protobuf.ByteString
getUniqueIdBytes() {
java.lang.Object ref = uniqueId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uniqueId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIGNATURE_FIELD_NUMBER = 2;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature signature_;
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public boolean hasSignature() {
return signature_ != null;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature() {
return signature_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder() {
return getSignature();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUniqueIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uniqueId_);
}
if (signature_ != null) {
output.writeMessage(2, getSignature());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUniqueIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uniqueId_);
}
if (signature_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSignature());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer) obj;
if (!getUniqueId()
.equals(other.getUniqueId())) return false;
if (hasSignature() != other.hasSignature()) return false;
if (hasSignature()) {
if (!getSignature()
.equals(other.getSignature())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UNIQUE_ID_FIELD_NUMBER;
hash = (53 * hash) + getUniqueId().hashCode();
if (hasSignature()) {
hash = (37 * hash) + SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getSignature().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies combined information regarding the customer who made a particular purchase. This includes their digitally
* identifying information, their legal identification, and the authority under which the purchase occurred.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseCustomer}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseCustomer)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseCustomer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseCustomer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uniqueId_ = "";
if (signatureBuilder_ == null) {
signature_ = null;
} else {
signature_ = null;
signatureBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseCustomer_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer(this);
result.uniqueId_ = uniqueId_;
if (signatureBuilder_ == null) {
result.signature_ = signature_;
} else {
result.signature_ = signatureBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer.getDefaultInstance()) return this;
if (!other.getUniqueId().isEmpty()) {
uniqueId_ = other.uniqueId_;
onChanged();
}
if (other.hasSignature()) {
mergeSignature(other.getSignature());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object uniqueId_ = "";
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public java.lang.String getUniqueId() {
java.lang.Object ref = uniqueId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uniqueId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public com.google.protobuf.ByteString
getUniqueIdBytes() {
java.lang.Object ref = uniqueId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uniqueId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public Builder setUniqueId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uniqueId_ = value;
onChanged();
return this;
}
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public Builder clearUniqueId() {
uniqueId_ = getDefaultInstance().getUniqueId();
onChanged();
return this;
}
/**
*
* Unique and opaque ID, representing this customer's accounting of interactions.
*
*
* string unique_id = 1;
*/
public Builder setUniqueIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uniqueId_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature signature_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder> signatureBuilder_;
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public boolean hasSignature() {
return signatureBuilder_ != null || signature_ != null;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature() {
if (signatureBuilder_ == null) {
return signature_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
} else {
return signatureBuilder_.getMessage();
}
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public Builder setSignature(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature value) {
if (signatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signature_ = value;
onChanged();
} else {
signatureBuilder_.setMessage(value);
}
return this;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public Builder setSignature(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder builderForValue) {
if (signatureBuilder_ == null) {
signature_ = builderForValue.build();
onChanged();
} else {
signatureBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public Builder mergeSignature(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature value) {
if (signatureBuilder_ == null) {
if (signature_ != null) {
signature_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.newBuilder(signature_).mergeFrom(value).buildPartial();
} else {
signature_ = value;
}
onChanged();
} else {
signatureBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public Builder clearSignature() {
if (signatureBuilder_ == null) {
signature_ = null;
onChanged();
} else {
signature_ = null;
signatureBuilder_ = null;
}
return this;
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder getSignatureBuilder() {
onChanged();
return getSignatureFieldBuilder().getBuilder();
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder() {
if (signatureBuilder_ != null) {
return signatureBuilder_.getMessageOrBuilder();
} else {
return signature_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
}
}
/**
*
* Digital signature provided by the customer, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder>
getSignatureFieldBuilder() {
if (signatureBuilder_ == null) {
signatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder>(
getSignature(),
getParentForChildren(),
isClean());
signature_ = null;
}
return signatureBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseCustomer)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseCustomer)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseCustomer parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseCustomer(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseCustomer getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PurchaseFacilitatorOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PurchaseFacilitator)
com.google.protobuf.MessageOrBuilder {
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
int getAuthorityValue();
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority getAuthority();
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
java.lang.String getAgent();
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
com.google.protobuf.ByteString
getAgentBytes();
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
java.lang.String getDevice();
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
com.google.protobuf.ByteString
getDeviceBytes();
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
boolean hasSignature();
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature();
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder();
}
/**
*
* Specifies information about the facilitator of a retail/commercial purchase, i.e., the retailer's side of a retail
* transaction with a consumer or patient.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseFacilitator}
*/
public static final class PurchaseFacilitator extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PurchaseFacilitator)
PurchaseFacilitatorOrBuilder {
private static final long serialVersionUID = 0L;
// Use PurchaseFacilitator.newBuilder() to construct.
private PurchaseFacilitator(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PurchaseFacilitator() {
authority_ = 0;
agent_ = "";
device_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PurchaseFacilitator(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
authority_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
agent_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
device_ = s;
break;
}
case 34: {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder subBuilder = null;
if (signature_ != null) {
subBuilder = signature_.toBuilder();
}
signature_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(signature_);
signature_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseFacilitator_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder.class);
}
public static final int AUTHORITY_FIELD_NUMBER = 1;
private int authority_;
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public int getAuthorityValue() {
return authority_;
}
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority getAuthority() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.valueOf(authority_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.UNRECOGNIZED : result;
}
public static final int AGENT_FIELD_NUMBER = 2;
private volatile java.lang.Object agent_;
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
agent_ = s;
return s;
}
}
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICE_FIELD_NUMBER = 3;
private volatile java.lang.Object device_;
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
device_ = s;
return s;
}
}
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIGNATURE_FIELD_NUMBER = 4;
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature signature_;
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public boolean hasSignature() {
return signature_ != null;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature() {
return signature_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder() {
return getSignature();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (authority_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.STANDARD.getNumber()) {
output.writeEnum(1, authority_);
}
if (!getAgentBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, agent_);
}
if (!getDeviceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, device_);
}
if (signature_ != null) {
output.writeMessage(4, getSignature());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (authority_ != io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.STANDARD.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, authority_);
}
if (!getAgentBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, agent_);
}
if (!getDeviceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, device_);
}
if (signature_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSignature());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator other = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator) obj;
if (authority_ != other.authority_) return false;
if (!getAgent()
.equals(other.getAgent())) return false;
if (!getDevice()
.equals(other.getDevice())) return false;
if (hasSignature() != other.hasSignature()) return false;
if (hasSignature()) {
if (!getSignature()
.equals(other.getSignature())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + AUTHORITY_FIELD_NUMBER;
hash = (53 * hash) + authority_;
hash = (37 * hash) + AGENT_FIELD_NUMBER;
hash = (53 * hash) + getAgent().hashCode();
hash = (37 * hash) + DEVICE_FIELD_NUMBER;
hash = (53 * hash) + getDevice().hashCode();
if (hasSignature()) {
hash = (37 * hash) + SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getSignature().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies information about the facilitator of a retail/commercial purchase, i.e., the retailer's side of a retail
* transaction with a consumer or patient.
*
*
* Protobuf type {@code opencannabis.commerce.PurchaseFacilitator}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PurchaseFacilitator)
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitatorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseFacilitator_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.class, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
authority_ = 0;
agent_ = "";
device_ = "";
if (signatureBuilder_ == null) {
signature_ = null;
} else {
signature_ = null;
signatureBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator build() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator result = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator(this);
result.authority_ = authority_;
result.agent_ = agent_;
result.device_ = device_;
if (signatureBuilder_ == null) {
result.signature_ = signature_;
} else {
result.signature_ = signatureBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator.getDefaultInstance()) return this;
if (other.authority_ != 0) {
setAuthorityValue(other.getAuthorityValue());
}
if (!other.getAgent().isEmpty()) {
agent_ = other.agent_;
onChanged();
}
if (!other.getDevice().isEmpty()) {
device_ = other.device_;
onChanged();
}
if (other.hasSignature()) {
mergeSignature(other.getSignature());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int authority_ = 0;
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public int getAuthorityValue() {
return authority_;
}
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public Builder setAuthorityValue(int value) {
authority_ = value;
onChanged();
return this;
}
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority getAuthority() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority result = io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.valueOf(authority_);
return result == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority.UNRECOGNIZED : result;
}
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public Builder setAuthority(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseAuthority value) {
if (value == null) {
throw new NullPointerException();
}
authority_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies the authority under which this purchase occurred, if applicable (i.e. medical, vs. adult-use/recreational
* cannabis purchases, depending on jurisdiction).
*
*
* .opencannabis.commerce.PurchaseAuthority authority = 1;
*/
public Builder clearAuthority() {
authority_ = 0;
onChanged();
return this;
}
private java.lang.Object agent_ = "";
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
agent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public Builder setAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
agent_ = value;
onChanged();
return this;
}
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public Builder clearAgent() {
agent_ = getDefaultInstance().getAgent();
onChanged();
return this;
}
/**
*
* Specifies the opaque ID for the user that conducted the purchase (i.e. the sales agent or budtender).
*
*
* string agent = 2;
*/
public Builder setAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
agent_ = value;
onChanged();
return this;
}
private java.lang.Object device_ = "";
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
device_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public Builder setDevice(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
device_ = value;
onChanged();
return this;
}
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public Builder clearDevice() {
device_ = getDefaultInstance().getDevice();
onChanged();
return this;
}
/**
*
* Unique/opaque reference to the partner co-located point-of-sale device that facilitated this transaction.
*
*
* string device = 3;
*/
public Builder setDeviceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
device_ = value;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature signature_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder> signatureBuilder_;
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public boolean hasSignature() {
return signatureBuilder_ != null || signature_ != null;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature getSignature() {
if (signatureBuilder_ == null) {
return signature_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
} else {
return signatureBuilder_.getMessage();
}
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public Builder setSignature(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature value) {
if (signatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
signature_ = value;
onChanged();
} else {
signatureBuilder_.setMessage(value);
}
return this;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public Builder setSignature(
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder builderForValue) {
if (signatureBuilder_ == null) {
signature_ = builderForValue.build();
onChanged();
} else {
signatureBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public Builder mergeSignature(io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature value) {
if (signatureBuilder_ == null) {
if (signature_ != null) {
signature_ =
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.newBuilder(signature_).mergeFrom(value).buildPartial();
} else {
signature_ = value;
}
onChanged();
} else {
signatureBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public Builder clearSignature() {
if (signatureBuilder_ == null) {
signature_ = null;
onChanged();
} else {
signature_ = null;
signatureBuilder_ = null;
}
return this;
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder getSignatureBuilder() {
onChanged();
return getSignatureFieldBuilder().getBuilder();
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder getSignatureOrBuilder() {
if (signatureBuilder_ != null) {
return signatureBuilder_.getMessageOrBuilder();
} else {
return signature_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.getDefaultInstance() : signature_;
}
}
/**
*
* Digital signature provided by the facilitator, if applicable and supported.
*
*
* .opencannabis.commerce.PurchaseSignature signature = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder>
getSignatureFieldBuilder() {
if (signatureBuilder_ == null) {
signatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignature.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PurchaseSignatureOrBuilder>(
getSignature(),
getParentForChildren(),
isClean());
signature_ = null;
}
return signatureBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PurchaseFacilitator)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PurchaseFacilitator)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PurchaseFacilitator parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PurchaseFacilitator(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PurchaseFacilitator getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PaymentKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.PaymentKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
java.lang.String getUuid();
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
com.google.protobuf.ByteString
getUuidBytes();
}
/**
*
* Specifies the structure of an individual payment's key.
*
*
* Protobuf type {@code opencannabis.commerce.PaymentKey}
*/
public static final class PaymentKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.PaymentKey)
PaymentKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use PaymentKey.newBuilder() to construct.
private PaymentKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PaymentKey() {
uuid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PaymentKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
uuid_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PaymentKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PaymentKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.class, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder.class);
}
public static final int UUID_FIELD_NUMBER = 1;
private volatile java.lang.Object uuid_;
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
}
}
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUuidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, uuid_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUuidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, uuid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey other = (io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey) obj;
if (!getUuid()
.equals(other.getUuid())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + UUID_FIELD_NUMBER;
hash = (53 * hash) + getUuid().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the structure of an individual payment's key.
*
*
* Protobuf type {@code opencannabis.commerce.PaymentKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.PaymentKey)
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PaymentKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PaymentKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.class, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
uuid_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_PaymentKey_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey build() {
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey result = new io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey(this);
result.uuid_ = uuid_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.getDefaultInstance()) return this;
if (!other.getUuid().isEmpty()) {
uuid_ = other.uuid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object uuid_ = "";
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public java.lang.String getUuid() {
java.lang.Object ref = uuid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uuid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public com.google.protobuf.ByteString
getUuidBytes() {
java.lang.Object ref = uuid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uuid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uuid_ = value;
onChanged();
return this;
}
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder clearUuid() {
uuid_ = getDefaultInstance().getUuid();
onChanged();
return this;
}
/**
*
* Unique ID provisioned for this payment.
*
*
* string uuid = 1 [(.core.field) = { ... }
*/
public Builder setUuidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uuid_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.PaymentKey)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.PaymentKey)
private static final io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PaymentKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PaymentKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
boolean hasKey();
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getKey();
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder getKeyOrBuilder();
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
int getMethodValue();
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod();
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
int getStatusValue();
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus();
/**
*
* Amount for this payment.
*
*
* double amount = 4;
*/
double getAmount();
/**
*
* Whether this fully satisfies the order, or not.
*
*
* bool full = 5;
*/
boolean getFull();
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
boolean hasCash();
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getCash();
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder getCashOrBuilder();
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
boolean hasCheck();
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getCheck();
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder getCheckOrBuilder();
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
boolean hasCard();
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getCard();
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder getCardOrBuilder();
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
boolean hasBank();
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getBank();
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder getBankOrBuilder();
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
boolean hasDigital();
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDigital();
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder getDigitalOrBuilder();
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.SpecCase getSpecCase();
}
/**
*
* Specifies the style, amount, and parameters regarding how this purchase was paid for.
*
*
* Protobuf type {@code opencannabis.commerce.Payment}
*/
public static final class Payment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment)
PaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use Payment.newBuilder() to construct.
private Payment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Payment() {
method_ = 0;
status_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Payment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
method_ = rawValue;
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 33: {
amount_ = input.readDouble();
break;
}
case 40: {
full_ = input.readBool();
break;
}
case 82: {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder subBuilder = null;
if (specCase_ == 10) {
subBuilder = ((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_).toBuilder();
}
spec_ =
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_);
spec_ = subBuilder.buildPartial();
}
specCase_ = 10;
break;
}
case 90: {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder subBuilder = null;
if (specCase_ == 11) {
subBuilder = ((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_).toBuilder();
}
spec_ =
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_);
spec_ = subBuilder.buildPartial();
}
specCase_ = 11;
break;
}
case 98: {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder subBuilder = null;
if (specCase_ == 12) {
subBuilder = ((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_).toBuilder();
}
spec_ =
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_);
spec_ = subBuilder.buildPartial();
}
specCase_ = 12;
break;
}
case 106: {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder subBuilder = null;
if (specCase_ == 13) {
subBuilder = ((io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_).toBuilder();
}
spec_ =
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_);
spec_ = subBuilder.buildPartial();
}
specCase_ = 13;
break;
}
case 114: {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder subBuilder = null;
if (specCase_ == 14) {
subBuilder = ((io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_).toBuilder();
}
spec_ =
input.readMessage(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_);
spec_ = subBuilder.buildPartial();
}
specCase_ = 14;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder.class);
}
public interface CashPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment.CashPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
boolean hasTendered();
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getTendered();
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getTenderedOrBuilder();
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
boolean hasChange();
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getChange();
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getChangeOrBuilder();
}
/**
*
* Specifies details regarding a cash payment.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CashPayment}
*/
public static final class CashPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment.CashPayment)
CashPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use CashPayment.newBuilder() to construct.
private CashPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CashPayment() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CashPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (tendered_ != null) {
subBuilder = tendered_.toBuilder();
}
tendered_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tendered_);
tendered_ = subBuilder.buildPartial();
}
break;
}
case 18: {
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder subBuilder = null;
if (change_ != null) {
subBuilder = change_.toBuilder();
}
change_ = input.readMessage(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(change_);
change_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CashPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CashPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder.class);
}
public static final int TENDERED_FIELD_NUMBER = 1;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue tendered_;
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public boolean hasTendered() {
return tendered_ != null;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getTendered() {
return tendered_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : tendered_;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getTenderedOrBuilder() {
return getTendered();
}
public static final int CHANGE_FIELD_NUMBER = 2;
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue change_;
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public boolean hasChange() {
return change_ != null;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getChange() {
return change_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : change_;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getChangeOrBuilder() {
return getChange();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tendered_ != null) {
output.writeMessage(1, getTendered());
}
if (change_ != null) {
output.writeMessage(2, getChange());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tendered_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTendered());
}
if (change_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getChange());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) obj;
if (hasTendered() != other.hasTendered()) return false;
if (hasTendered()) {
if (!getTendered()
.equals(other.getTendered())) return false;
}
if (hasChange() != other.hasChange()) return false;
if (hasChange()) {
if (!getChange()
.equals(other.getChange())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTendered()) {
hash = (37 * hash) + TENDERED_FIELD_NUMBER;
hash = (53 * hash) + getTendered().hashCode();
}
if (hasChange()) {
hash = (37 * hash) + CHANGE_FIELD_NUMBER;
hash = (53 * hash) + getChange().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies details regarding a cash payment.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CashPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment.CashPayment)
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CashPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CashPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tenderedBuilder_ == null) {
tendered_ = null;
} else {
tendered_ = null;
tenderedBuilder_ = null;
}
if (changeBuilder_ == null) {
change_ = null;
} else {
change_ = null;
changeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CashPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment(this);
if (tenderedBuilder_ == null) {
result.tendered_ = tendered_;
} else {
result.tendered_ = tenderedBuilder_.build();
}
if (changeBuilder_ == null) {
result.change_ = change_;
} else {
result.change_ = changeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance()) return this;
if (other.hasTendered()) {
mergeTendered(other.getTendered());
}
if (other.hasChange()) {
mergeChange(other.getChange());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue tendered_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> tenderedBuilder_;
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public boolean hasTendered() {
return tenderedBuilder_ != null || tendered_ != null;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getTendered() {
if (tenderedBuilder_ == null) {
return tendered_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : tendered_;
} else {
return tenderedBuilder_.getMessage();
}
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public Builder setTendered(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (tenderedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tendered_ = value;
onChanged();
} else {
tenderedBuilder_.setMessage(value);
}
return this;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public Builder setTendered(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (tenderedBuilder_ == null) {
tendered_ = builderForValue.build();
onChanged();
} else {
tenderedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public Builder mergeTendered(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (tenderedBuilder_ == null) {
if (tendered_ != null) {
tendered_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(tendered_).mergeFrom(value).buildPartial();
} else {
tendered_ = value;
}
onChanged();
} else {
tenderedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public Builder clearTendered() {
if (tenderedBuilder_ == null) {
tendered_ = null;
onChanged();
} else {
tendered_ = null;
tenderedBuilder_ = null;
}
return this;
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getTenderedBuilder() {
onChanged();
return getTenderedFieldBuilder().getBuilder();
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getTenderedOrBuilder() {
if (tenderedBuilder_ != null) {
return tenderedBuilder_.getMessageOrBuilder();
} else {
return tendered_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : tendered_;
}
}
/**
*
* The amount of cash tendered by the customer for payment.
*
*
* .opencannabis.commerce.CurrencyValue tendered = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getTenderedFieldBuilder() {
if (tenderedBuilder_ == null) {
tenderedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getTendered(),
getParentForChildren(),
isClean());
tendered_ = null;
}
return tenderedBuilder_;
}
private io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue change_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder> changeBuilder_;
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public boolean hasChange() {
return changeBuilder_ != null || change_ != null;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue getChange() {
if (changeBuilder_ == null) {
return change_ == null ? io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : change_;
} else {
return changeBuilder_.getMessage();
}
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public Builder setChange(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (changeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
change_ = value;
onChanged();
} else {
changeBuilder_.setMessage(value);
}
return this;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public Builder setChange(
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder builderForValue) {
if (changeBuilder_ == null) {
change_ = builderForValue.build();
onChanged();
} else {
changeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public Builder mergeChange(io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue value) {
if (changeBuilder_ == null) {
if (change_ != null) {
change_ =
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.newBuilder(change_).mergeFrom(value).buildPartial();
} else {
change_ = value;
}
onChanged();
} else {
changeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public Builder clearChange() {
if (changeBuilder_ == null) {
change_ = null;
onChanged();
} else {
change_ = null;
changeBuilder_ = null;
}
return this;
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder getChangeBuilder() {
onChanged();
return getChangeFieldBuilder().getBuilder();
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
public io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder getChangeOrBuilder() {
if (changeBuilder_ != null) {
return changeBuilder_.getMessageOrBuilder();
} else {
return change_ == null ?
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.getDefaultInstance() : change_;
}
}
/**
*
* The amount of change given back to the customer after payment.
*
*
* .opencannabis.commerce.CurrencyValue change = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>
getChangeFieldBuilder() {
if (changeBuilder_ == null) {
changeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValue.Builder, io.opencannabis.schema.currency.CommerceCurrency.CurrencyValueOrBuilder>(
getChange(),
getParentForChildren(),
isClean());
change_ = null;
}
return changeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment.CashPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment.CashPayment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CashPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CashPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment.CheckPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
java.lang.String getCheckNumber();
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
com.google.protobuf.ByteString
getCheckNumberBytes();
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
java.lang.String getRoutingNumber();
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
com.google.protobuf.ByteString
getRoutingNumberBytes();
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
java.lang.String getAccountNumber();
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
com.google.protobuf.ByteString
getAccountNumberBytes();
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
java.lang.String getInstitution();
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
com.google.protobuf.ByteString
getInstitutionBytes();
/**
*
* Whether the check is certified or not. Certified checks are issued by institutions, usually banks.
*
*
* bool certified = 5;
*/
boolean getCertified();
}
/**
*
* Check payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CheckPayment}
*/
public static final class CheckPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment.CheckPayment)
CheckPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckPayment.newBuilder() to construct.
private CheckPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckPayment() {
checkNumber_ = "";
routingNumber_ = "";
accountNumber_ = "";
institution_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
checkNumber_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
routingNumber_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
accountNumber_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
institution_ = s;
break;
}
case 40: {
certified_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CheckPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder.class);
}
public static final int CHECK_NUMBER_FIELD_NUMBER = 1;
private volatile java.lang.Object checkNumber_;
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public java.lang.String getCheckNumber() {
java.lang.Object ref = checkNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
checkNumber_ = s;
return s;
}
}
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public com.google.protobuf.ByteString
getCheckNumberBytes() {
java.lang.Object ref = checkNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checkNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROUTING_NUMBER_FIELD_NUMBER = 2;
private volatile java.lang.Object routingNumber_;
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public java.lang.String getRoutingNumber() {
java.lang.Object ref = routingNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routingNumber_ = s;
return s;
}
}
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public com.google.protobuf.ByteString
getRoutingNumberBytes() {
java.lang.Object ref = routingNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routingNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_NUMBER_FIELD_NUMBER = 3;
private volatile java.lang.Object accountNumber_;
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public java.lang.String getAccountNumber() {
java.lang.Object ref = accountNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountNumber_ = s;
return s;
}
}
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public com.google.protobuf.ByteString
getAccountNumberBytes() {
java.lang.Object ref = accountNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTITUTION_FIELD_NUMBER = 4;
private volatile java.lang.Object institution_;
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public java.lang.String getInstitution() {
java.lang.Object ref = institution_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
institution_ = s;
return s;
}
}
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public com.google.protobuf.ByteString
getInstitutionBytes() {
java.lang.Object ref = institution_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
institution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CERTIFIED_FIELD_NUMBER = 5;
private boolean certified_;
/**
*
* Whether the check is certified or not. Certified checks are issued by institutions, usually banks.
*
*
* bool certified = 5;
*/
public boolean getCertified() {
return certified_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCheckNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, checkNumber_);
}
if (!getRoutingNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, routingNumber_);
}
if (!getAccountNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, accountNumber_);
}
if (!getInstitutionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, institution_);
}
if (certified_ != false) {
output.writeBool(5, certified_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCheckNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, checkNumber_);
}
if (!getRoutingNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, routingNumber_);
}
if (!getAccountNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, accountNumber_);
}
if (!getInstitutionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, institution_);
}
if (certified_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, certified_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) obj;
if (!getCheckNumber()
.equals(other.getCheckNumber())) return false;
if (!getRoutingNumber()
.equals(other.getRoutingNumber())) return false;
if (!getAccountNumber()
.equals(other.getAccountNumber())) return false;
if (!getInstitution()
.equals(other.getInstitution())) return false;
if (getCertified()
!= other.getCertified()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHECK_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getCheckNumber().hashCode();
hash = (37 * hash) + ROUTING_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getRoutingNumber().hashCode();
hash = (37 * hash) + ACCOUNT_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getAccountNumber().hashCode();
hash = (37 * hash) + INSTITUTION_FIELD_NUMBER;
hash = (53 * hash) + getInstitution().hashCode();
hash = (37 * hash) + CERTIFIED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCertified());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Check payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CheckPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment.CheckPayment)
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CheckPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
checkNumber_ = "";
routingNumber_ = "";
accountNumber_ = "";
institution_ = "";
certified_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment(this);
result.checkNumber_ = checkNumber_;
result.routingNumber_ = routingNumber_;
result.accountNumber_ = accountNumber_;
result.institution_ = institution_;
result.certified_ = certified_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance()) return this;
if (!other.getCheckNumber().isEmpty()) {
checkNumber_ = other.checkNumber_;
onChanged();
}
if (!other.getRoutingNumber().isEmpty()) {
routingNumber_ = other.routingNumber_;
onChanged();
}
if (!other.getAccountNumber().isEmpty()) {
accountNumber_ = other.accountNumber_;
onChanged();
}
if (!other.getInstitution().isEmpty()) {
institution_ = other.institution_;
onChanged();
}
if (other.getCertified() != false) {
setCertified(other.getCertified());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object checkNumber_ = "";
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public java.lang.String getCheckNumber() {
java.lang.Object ref = checkNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
checkNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public com.google.protobuf.ByteString
getCheckNumberBytes() {
java.lang.Object ref = checkNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checkNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public Builder setCheckNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
checkNumber_ = value;
onChanged();
return this;
}
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public Builder clearCheckNumber() {
checkNumber_ = getDefaultInstance().getCheckNumber();
onChanged();
return this;
}
/**
*
* Sequence number from the check for payment.
*
*
* string check_number = 1;
*/
public Builder setCheckNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
checkNumber_ = value;
onChanged();
return this;
}
private java.lang.Object routingNumber_ = "";
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public java.lang.String getRoutingNumber() {
java.lang.Object ref = routingNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routingNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public com.google.protobuf.ByteString
getRoutingNumberBytes() {
java.lang.Object ref = routingNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routingNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public Builder setRoutingNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
routingNumber_ = value;
onChanged();
return this;
}
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public Builder clearRoutingNumber() {
routingNumber_ = getDefaultInstance().getRoutingNumber();
onChanged();
return this;
}
/**
*
* Routing number from the check for payment. Private and encrypted.
*
*
* string routing_number = 2;
*/
public Builder setRoutingNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
routingNumber_ = value;
onChanged();
return this;
}
private java.lang.Object accountNumber_ = "";
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public java.lang.String getAccountNumber() {
java.lang.Object ref = accountNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public com.google.protobuf.ByteString
getAccountNumberBytes() {
java.lang.Object ref = accountNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public Builder setAccountNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accountNumber_ = value;
onChanged();
return this;
}
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public Builder clearAccountNumber() {
accountNumber_ = getDefaultInstance().getAccountNumber();
onChanged();
return this;
}
/**
*
* Account number from the check for payment. Private and encrypted.
*
*
* string account_number = 3;
*/
public Builder setAccountNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accountNumber_ = value;
onChanged();
return this;
}
private java.lang.Object institution_ = "";
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public java.lang.String getInstitution() {
java.lang.Object ref = institution_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
institution_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public com.google.protobuf.ByteString
getInstitutionBytes() {
java.lang.Object ref = institution_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
institution_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public Builder setInstitution(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
institution_ = value;
onChanged();
return this;
}
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public Builder clearInstitution() {
institution_ = getDefaultInstance().getInstitution();
onChanged();
return this;
}
/**
*
* Name of the bank or issuing institution. Required if the check is certified.
*
*
* string institution = 4;
*/
public Builder setInstitutionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
institution_ = value;
onChanged();
return this;
}
private boolean certified_ ;
/**
*
* Whether the check is certified or not. Certified checks are issued by institutions, usually banks.
*
*
* bool certified = 5;
*/
public boolean getCertified() {
return certified_;
}
/**
*
* Whether the check is certified or not. Certified checks are issued by institutions, usually banks.
*
*
* bool certified = 5;
*/
public Builder setCertified(boolean value) {
certified_ = value;
onChanged();
return this;
}
/**
*
* Whether the check is certified or not. Certified checks are issued by institutions, usually banks.
*
*
* bool certified = 5;
*/
public Builder clearCertified() {
certified_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment.CheckPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment.CheckPayment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CardPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment.CardPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
int getCardTypeValue();
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
io.opencannabis.schema.commerce.Payments.PaymentCardType getCardType();
}
/**
*
* Card-based payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CardPayment}
*/
public static final class CardPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment.CardPayment)
CardPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use CardPayment.newBuilder() to construct.
private CardPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CardPayment() {
cardType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CardPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
cardType_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CardPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CardPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder.class);
}
public static final int CARD_TYPE_FIELD_NUMBER = 1;
private int cardType_;
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public int getCardTypeValue() {
return cardType_;
}
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public io.opencannabis.schema.commerce.Payments.PaymentCardType getCardType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentCardType result = io.opencannabis.schema.commerce.Payments.PaymentCardType.valueOf(cardType_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentCardType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (cardType_ != io.opencannabis.schema.commerce.Payments.PaymentCardType.NO_CARD_TYPE.getNumber()) {
output.writeEnum(1, cardType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (cardType_ != io.opencannabis.schema.commerce.Payments.PaymentCardType.NO_CARD_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, cardType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) obj;
if (cardType_ != other.cardType_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CARD_TYPE_FIELD_NUMBER;
hash = (53 * hash) + cardType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Card-based payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.CardPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment.CardPayment)
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CardPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CardPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
cardType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_CardPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment(this);
result.cardType_ = cardType_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance()) return this;
if (other.cardType_ != 0) {
setCardTypeValue(other.getCardTypeValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int cardType_ = 0;
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public int getCardTypeValue() {
return cardType_;
}
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public Builder setCardTypeValue(int value) {
cardType_ = value;
onChanged();
return this;
}
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public io.opencannabis.schema.commerce.Payments.PaymentCardType getCardType() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentCardType result = io.opencannabis.schema.commerce.Payments.PaymentCardType.valueOf(cardType_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentCardType.UNRECOGNIZED : result;
}
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public Builder setCardType(io.opencannabis.schema.commerce.Payments.PaymentCardType value) {
if (value == null) {
throw new NullPointerException();
}
cardType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Card type used by the customer to pay.
*
*
* .opencannabis.commerce.PaymentCardType card_type = 1;
*/
public Builder clearCardType() {
cardType_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment.CardPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment.CardPayment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CardPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CardPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BankPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment.BankPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
java.lang.String getRoutingNumber();
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
com.google.protobuf.ByteString
getRoutingNumberBytes();
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
java.lang.String getAccountNumber();
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
com.google.protobuf.ByteString
getAccountNumberBytes();
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
java.lang.String getReference();
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
com.google.protobuf.ByteString
getReferenceBytes();
}
/**
*
* Bank-based payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.BankPayment}
*/
public static final class BankPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment.BankPayment)
BankPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use BankPayment.newBuilder() to construct.
private BankPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BankPayment() {
routingNumber_ = "";
accountNumber_ = "";
reference_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BankPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
routingNumber_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
accountNumber_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
reference_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_BankPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_BankPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder.class);
}
public static final int ROUTING_NUMBER_FIELD_NUMBER = 1;
private volatile java.lang.Object routingNumber_;
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public java.lang.String getRoutingNumber() {
java.lang.Object ref = routingNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routingNumber_ = s;
return s;
}
}
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public com.google.protobuf.ByteString
getRoutingNumberBytes() {
java.lang.Object ref = routingNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routingNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_NUMBER_FIELD_NUMBER = 2;
private volatile java.lang.Object accountNumber_;
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public java.lang.String getAccountNumber() {
java.lang.Object ref = accountNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountNumber_ = s;
return s;
}
}
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public com.google.protobuf.ByteString
getAccountNumberBytes() {
java.lang.Object ref = accountNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REFERENCE_FIELD_NUMBER = 3;
private volatile java.lang.Object reference_;
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRoutingNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, routingNumber_);
}
if (!getAccountNumberBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, accountNumber_);
}
if (!getReferenceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, reference_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRoutingNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, routingNumber_);
}
if (!getAccountNumberBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, accountNumber_);
}
if (!getReferenceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, reference_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) obj;
if (!getRoutingNumber()
.equals(other.getRoutingNumber())) return false;
if (!getAccountNumber()
.equals(other.getAccountNumber())) return false;
if (!getReference()
.equals(other.getReference())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ROUTING_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getRoutingNumber().hashCode();
hash = (37 * hash) + ACCOUNT_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getAccountNumber().hashCode();
hash = (37 * hash) + REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getReference().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Bank-based payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.BankPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment.BankPayment)
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_BankPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_BankPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
routingNumber_ = "";
accountNumber_ = "";
reference_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_BankPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment(this);
result.routingNumber_ = routingNumber_;
result.accountNumber_ = accountNumber_;
result.reference_ = reference_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance()) return this;
if (!other.getRoutingNumber().isEmpty()) {
routingNumber_ = other.routingNumber_;
onChanged();
}
if (!other.getAccountNumber().isEmpty()) {
accountNumber_ = other.accountNumber_;
onChanged();
}
if (!other.getReference().isEmpty()) {
reference_ = other.reference_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object routingNumber_ = "";
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public java.lang.String getRoutingNumber() {
java.lang.Object ref = routingNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
routingNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public com.google.protobuf.ByteString
getRoutingNumberBytes() {
java.lang.Object ref = routingNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routingNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public Builder setRoutingNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
routingNumber_ = value;
onChanged();
return this;
}
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public Builder clearRoutingNumber() {
routingNumber_ = getDefaultInstance().getRoutingNumber();
onChanged();
return this;
}
/**
*
* Routing number for the bank account to pay with.
*
*
* string routing_number = 1;
*/
public Builder setRoutingNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
routingNumber_ = value;
onChanged();
return this;
}
private java.lang.Object accountNumber_ = "";
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public java.lang.String getAccountNumber() {
java.lang.Object ref = accountNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public com.google.protobuf.ByteString
getAccountNumberBytes() {
java.lang.Object ref = accountNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public Builder setAccountNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accountNumber_ = value;
onChanged();
return this;
}
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public Builder clearAccountNumber() {
accountNumber_ = getDefaultInstance().getAccountNumber();
onChanged();
return this;
}
/**
*
* Account number for the bank to pay with.
*
*
* string account_number = 2;
*/
public Builder setAccountNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accountNumber_ = value;
onChanged();
return this;
}
private java.lang.Object reference_ = "";
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder setReference(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
reference_ = value;
onChanged();
return this;
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder clearReference() {
reference_ = getDefaultInstance().getReference();
onChanged();
return this;
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder setReferenceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
reference_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment.BankPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment.BankPayment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BankPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BankPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DigitalPaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:opencannabis.commerce.Payment.DigitalPayment)
com.google.protobuf.MessageOrBuilder {
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
int getNetworkValue();
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork getNetwork();
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
java.lang.String getUsername();
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
com.google.protobuf.ByteString
getUsernameBytes();
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
java.lang.String getReference();
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
com.google.protobuf.ByteString
getReferenceBytes();
}
/**
*
* Digital payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.DigitalPayment}
*/
public static final class DigitalPayment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:opencannabis.commerce.Payment.DigitalPayment)
DigitalPaymentOrBuilder {
private static final long serialVersionUID = 0L;
// Use DigitalPayment.newBuilder() to construct.
private DigitalPayment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DigitalPayment() {
network_ = 0;
username_ = "";
reference_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DigitalPayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
network_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
username_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
reference_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_DigitalPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder.class);
}
public static final int NETWORK_FIELD_NUMBER = 1;
private int network_;
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public int getNetworkValue() {
return network_;
}
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork getNetwork() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork result = io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.valueOf(network_);
return result == null ? io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.UNRECOGNIZED : result;
}
public static final int USERNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object username_;
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
username_ = s;
return s;
}
}
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REFERENCE_FIELD_NUMBER = 3;
private volatile java.lang.Object reference_;
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (network_ != io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.UNSPECIFIED_NETWORK.getNumber()) {
output.writeEnum(1, network_);
}
if (!getUsernameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, username_);
}
if (!getReferenceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, reference_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (network_ != io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.UNSPECIFIED_NETWORK.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, network_);
}
if (!getUsernameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, username_);
}
if (!getReferenceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, reference_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) obj;
if (network_ != other.network_) return false;
if (!getUsername()
.equals(other.getUsername())) return false;
if (!getReference()
.equals(other.getReference())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + network_;
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUsername().hashCode();
hash = (37 * hash) + REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getReference().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Digital payment made as part of a greater purchase payment set.
*
*
* Protobuf type {@code opencannabis.commerce.Payment.DigitalPayment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment.DigitalPayment)
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_DigitalPayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
network_ = 0;
username_ = "";
reference_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment(this);
result.network_ = network_;
result.username_ = username_;
result.reference_ = reference_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance()) return this;
if (other.network_ != 0) {
setNetworkValue(other.getNetworkValue());
}
if (!other.getUsername().isEmpty()) {
username_ = other.username_;
onChanged();
}
if (!other.getReference().isEmpty()) {
reference_ = other.reference_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int network_ = 0;
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public int getNetworkValue() {
return network_;
}
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public Builder setNetworkValue(int value) {
network_ = value;
onChanged();
return this;
}
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork getNetwork() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork result = io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.valueOf(network_);
return result == null ? io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork.UNRECOGNIZED : result;
}
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public Builder setNetwork(io.opencannabis.schema.commerce.Payments.DigitalPaymentNetwork value) {
if (value == null) {
throw new NullPointerException();
}
network_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Digital payment network used by the customer.
*
*
* .opencannabis.commerce.DigitalPaymentNetwork network = 1;
*/
public Builder clearNetwork() {
network_ = 0;
onChanged();
return this;
}
private java.lang.Object username_ = "";
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
username_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public Builder setUsername(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
username_ = value;
onChanged();
return this;
}
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public Builder clearUsername() {
username_ = getDefaultInstance().getUsername();
onChanged();
return this;
}
/**
*
* Username on the digital payment network.
*
*
* string username = 2;
*/
public Builder setUsernameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
username_ = value;
onChanged();
return this;
}
private java.lang.Object reference_ = "";
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public java.lang.String getReference() {
java.lang.Object ref = reference_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
reference_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public com.google.protobuf.ByteString
getReferenceBytes() {
java.lang.Object ref = reference_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder setReference(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
reference_ = value;
onChanged();
return this;
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder clearReference() {
reference_ = getDefaultInstance().getReference();
onChanged();
return this;
}
/**
*
* Reference code or ID for the transaction.
*
*
* string reference = 3;
*/
public Builder setReferenceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
reference_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment.DigitalPayment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment.DigitalPayment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DigitalPayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DigitalPayment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int specCase_ = 0;
private java.lang.Object spec_;
public enum SpecCase
implements com.google.protobuf.Internal.EnumLite {
CASH(10),
CHECK(11),
CARD(12),
BANK(13),
DIGITAL(14),
SPEC_NOT_SET(0);
private final int value;
private SpecCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SpecCase valueOf(int value) {
return forNumber(value);
}
public static SpecCase forNumber(int value) {
switch (value) {
case 10: return CASH;
case 11: return CHECK;
case 12: return CARD;
case 13: return BANK;
case 14: return DIGITAL;
case 0: return SPEC_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public SpecCase
getSpecCase() {
return SpecCase.forNumber(
specCase_);
}
public static final int KEY_FIELD_NUMBER = 1;
private io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey key_;
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getKey() {
return key_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.getDefaultInstance() : key_;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int METHOD_FIELD_NUMBER = 2;
private int method_;
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentMethod result = io.opencannabis.schema.commerce.Payments.PaymentMethod.valueOf(method_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentMethod.UNRECOGNIZED : result;
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentStatus result = io.opencannabis.schema.commerce.Payments.PaymentStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentStatus.UNRECOGNIZED : result;
}
public static final int AMOUNT_FIELD_NUMBER = 4;
private double amount_;
/**
*
* Amount for this payment.
*
*
* double amount = 4;
*/
public double getAmount() {
return amount_;
}
public static final int FULL_FIELD_NUMBER = 5;
private boolean full_;
/**
*
* Whether this fully satisfies the order, or not.
*
*
* bool full = 5;
*/
public boolean getFull() {
return full_;
}
public static final int CASH_FIELD_NUMBER = 10;
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public boolean hasCash() {
return specCase_ == 10;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getCash() {
if (specCase_ == 10) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder getCashOrBuilder() {
if (specCase_ == 10) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
public static final int CHECK_FIELD_NUMBER = 11;
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public boolean hasCheck() {
return specCase_ == 11;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getCheck() {
if (specCase_ == 11) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder getCheckOrBuilder() {
if (specCase_ == 11) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
public static final int CARD_FIELD_NUMBER = 12;
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public boolean hasCard() {
return specCase_ == 12;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getCard() {
if (specCase_ == 12) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder getCardOrBuilder() {
if (specCase_ == 12) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
public static final int BANK_FIELD_NUMBER = 13;
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public boolean hasBank() {
return specCase_ == 13;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getBank() {
if (specCase_ == 13) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder getBankOrBuilder() {
if (specCase_ == 13) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
public static final int DIGITAL_FIELD_NUMBER = 14;
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public boolean hasDigital() {
return specCase_ == 14;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDigital() {
if (specCase_ == 14) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder getDigitalOrBuilder() {
if (specCase_ == 14) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (key_ != null) {
output.writeMessage(1, getKey());
}
if (method_ != io.opencannabis.schema.commerce.Payments.PaymentMethod.CASH.getNumber()) {
output.writeEnum(2, method_);
}
if (status_ != io.opencannabis.schema.commerce.Payments.PaymentStatus.NOT_APPLICABLE.getNumber()) {
output.writeEnum(3, status_);
}
if (amount_ != 0D) {
output.writeDouble(4, amount_);
}
if (full_ != false) {
output.writeBool(5, full_);
}
if (specCase_ == 10) {
output.writeMessage(10, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_);
}
if (specCase_ == 11) {
output.writeMessage(11, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_);
}
if (specCase_ == 12) {
output.writeMessage(12, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_);
}
if (specCase_ == 13) {
output.writeMessage(13, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_);
}
if (specCase_ == 14) {
output.writeMessage(14, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getKey());
}
if (method_ != io.opencannabis.schema.commerce.Payments.PaymentMethod.CASH.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, method_);
}
if (status_ != io.opencannabis.schema.commerce.Payments.PaymentStatus.NOT_APPLICABLE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (amount_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, amount_);
}
if (full_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, full_);
}
if (specCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_);
}
if (specCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_);
}
if (specCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_);
}
if (specCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_);
}
if (specCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment)) {
return super.equals(obj);
}
io.opencannabis.schema.commerce.CommercialPurchase.Payment other = (io.opencannabis.schema.commerce.CommercialPurchase.Payment) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (method_ != other.method_) return false;
if (status_ != other.status_) return false;
if (java.lang.Double.doubleToLongBits(getAmount())
!= java.lang.Double.doubleToLongBits(
other.getAmount())) return false;
if (getFull()
!= other.getFull()) return false;
if (!getSpecCase().equals(other.getSpecCase())) return false;
switch (specCase_) {
case 10:
if (!getCash()
.equals(other.getCash())) return false;
break;
case 11:
if (!getCheck()
.equals(other.getCheck())) return false;
break;
case 12:
if (!getCard()
.equals(other.getCard())) return false;
break;
case 13:
if (!getBank()
.equals(other.getBank())) return false;
break;
case 14:
if (!getDigital()
.equals(other.getDigital())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAmount()));
hash = (37 * hash) + FULL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFull());
switch (specCase_) {
case 10:
hash = (37 * hash) + CASH_FIELD_NUMBER;
hash = (53 * hash) + getCash().hashCode();
break;
case 11:
hash = (37 * hash) + CHECK_FIELD_NUMBER;
hash = (53 * hash) + getCheck().hashCode();
break;
case 12:
hash = (37 * hash) + CARD_FIELD_NUMBER;
hash = (53 * hash) + getCard().hashCode();
break;
case 13:
hash = (37 * hash) + BANK_FIELD_NUMBER;
hash = (53 * hash) + getBank().hashCode();
break;
case 14:
hash = (37 * hash) + DIGITAL_FIELD_NUMBER;
hash = (53 * hash) + getDigital().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.opencannabis.schema.commerce.CommercialPurchase.Payment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies the style, amount, and parameters regarding how this purchase was paid for.
*
*
* Protobuf type {@code opencannabis.commerce.Payment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:opencannabis.commerce.Payment)
io.opencannabis.schema.commerce.CommercialPurchase.PaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.class, io.opencannabis.schema.commerce.CommercialPurchase.Payment.Builder.class);
}
// Construct using io.opencannabis.schema.commerce.CommercialPurchase.Payment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
method_ = 0;
status_ = 0;
amount_ = 0D;
full_ = false;
specCase_ = 0;
spec_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.internal_static_opencannabis_commerce_Payment_descriptor;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment getDefaultInstanceForType() {
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.getDefaultInstance();
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment build() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment buildPartial() {
io.opencannabis.schema.commerce.CommercialPurchase.Payment result = new io.opencannabis.schema.commerce.CommercialPurchase.Payment(this);
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
result.method_ = method_;
result.status_ = status_;
result.amount_ = amount_;
result.full_ = full_;
if (specCase_ == 10) {
if (cashBuilder_ == null) {
result.spec_ = spec_;
} else {
result.spec_ = cashBuilder_.build();
}
}
if (specCase_ == 11) {
if (checkBuilder_ == null) {
result.spec_ = spec_;
} else {
result.spec_ = checkBuilder_.build();
}
}
if (specCase_ == 12) {
if (cardBuilder_ == null) {
result.spec_ = spec_;
} else {
result.spec_ = cardBuilder_.build();
}
}
if (specCase_ == 13) {
if (bankBuilder_ == null) {
result.spec_ = spec_;
} else {
result.spec_ = bankBuilder_.build();
}
}
if (specCase_ == 14) {
if (digitalBuilder_ == null) {
result.spec_ = spec_;
} else {
result.spec_ = digitalBuilder_.build();
}
}
result.specCase_ = specCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.opencannabis.schema.commerce.CommercialPurchase.Payment) {
return mergeFrom((io.opencannabis.schema.commerce.CommercialPurchase.Payment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.opencannabis.schema.commerce.CommercialPurchase.Payment other) {
if (other == io.opencannabis.schema.commerce.CommercialPurchase.Payment.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.method_ != 0) {
setMethodValue(other.getMethodValue());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getAmount() != 0D) {
setAmount(other.getAmount());
}
if (other.getFull() != false) {
setFull(other.getFull());
}
switch (other.getSpecCase()) {
case CASH: {
mergeCash(other.getCash());
break;
}
case CHECK: {
mergeCheck(other.getCheck());
break;
}
case CARD: {
mergeCard(other.getCard());
break;
}
case BANK: {
mergeBank(other.getBank());
break;
}
case DIGITAL: {
mergeDigital(other.getDigital());
break;
}
case SPEC_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.opencannabis.schema.commerce.CommercialPurchase.Payment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.opencannabis.schema.commerce.CommercialPurchase.Payment) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int specCase_ = 0;
private java.lang.Object spec_;
public SpecCase
getSpecCase() {
return SpecCase.forNumber(
specCase_);
}
public Builder clearSpec() {
specCase_ = 0;
spec_ = null;
onChanged();
return this;
}
private io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey key_;
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder> keyBuilder_;
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey getKey() {
if (keyBuilder_ == null) {
return key_ == null ? io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public Builder setKey(
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public Builder mergeKey(io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
public io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.getDefaultInstance() : key_;
}
}
/**
*
* Unique key provisioned to address this payment, if applicable.
*
*
* .opencannabis.commerce.PaymentKey key = 1 [(.core.field) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKey.Builder, io.opencannabis.schema.commerce.CommercialPurchase.PaymentKeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private int method_ = 0;
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public int getMethodValue() {
return method_;
}
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public Builder setMethodValue(int value) {
method_ = value;
onChanged();
return this;
}
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public io.opencannabis.schema.commerce.Payments.PaymentMethod getMethod() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentMethod result = io.opencannabis.schema.commerce.Payments.PaymentMethod.valueOf(method_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentMethod.UNRECOGNIZED : result;
}
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public Builder setMethod(io.opencannabis.schema.commerce.Payments.PaymentMethod value) {
if (value == null) {
throw new NullPointerException();
}
method_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Method of payment used by the customer.
*
*
* .opencannabis.commerce.PaymentMethod method = 2;
*/
public Builder clearMethod() {
method_ = 0;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public int getStatusValue() {
return status_;
}
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public io.opencannabis.schema.commerce.Payments.PaymentStatus getStatus() {
@SuppressWarnings("deprecation")
io.opencannabis.schema.commerce.Payments.PaymentStatus result = io.opencannabis.schema.commerce.Payments.PaymentStatus.valueOf(status_);
return result == null ? io.opencannabis.schema.commerce.Payments.PaymentStatus.UNRECOGNIZED : result;
}
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public Builder setStatus(io.opencannabis.schema.commerce.Payments.PaymentStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Status for this payment.
*
*
* .opencannabis.commerce.PaymentStatus status = 3;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private double amount_ ;
/**
*
* Amount for this payment.
*
*
* double amount = 4;
*/
public double getAmount() {
return amount_;
}
/**
*
* Amount for this payment.
*
*
* double amount = 4;
*/
public Builder setAmount(double value) {
amount_ = value;
onChanged();
return this;
}
/**
*
* Amount for this payment.
*
*
* double amount = 4;
*/
public Builder clearAmount() {
amount_ = 0D;
onChanged();
return this;
}
private boolean full_ ;
/**
*
* Whether this fully satisfies the order, or not.
*
*
* bool full = 5;
*/
public boolean getFull() {
return full_;
}
/**
*
* Whether this fully satisfies the order, or not.
*
*
* bool full = 5;
*/
public Builder setFull(boolean value) {
full_ = value;
onChanged();
return this;
}
/**
*
* Whether this fully satisfies the order, or not.
*
*
* bool full = 5;
*/
public Builder clearFull() {
full_ = false;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder> cashBuilder_;
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public boolean hasCash() {
return specCase_ == 10;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment getCash() {
if (cashBuilder_ == null) {
if (specCase_ == 10) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
} else {
if (specCase_ == 10) {
return cashBuilder_.getMessage();
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public Builder setCash(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment value) {
if (cashBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
spec_ = value;
onChanged();
} else {
cashBuilder_.setMessage(value);
}
specCase_ = 10;
return this;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public Builder setCash(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder builderForValue) {
if (cashBuilder_ == null) {
spec_ = builderForValue.build();
onChanged();
} else {
cashBuilder_.setMessage(builderForValue.build());
}
specCase_ = 10;
return this;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public Builder mergeCash(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment value) {
if (cashBuilder_ == null) {
if (specCase_ == 10 &&
spec_ != io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance()) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.newBuilder((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_)
.mergeFrom(value).buildPartial();
} else {
spec_ = value;
}
onChanged();
} else {
if (specCase_ == 10) {
cashBuilder_.mergeFrom(value);
}
cashBuilder_.setMessage(value);
}
specCase_ = 10;
return this;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public Builder clearCash() {
if (cashBuilder_ == null) {
if (specCase_ == 10) {
specCase_ = 0;
spec_ = null;
onChanged();
}
} else {
if (specCase_ == 10) {
specCase_ = 0;
spec_ = null;
}
cashBuilder_.clear();
}
return this;
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder getCashBuilder() {
return getCashFieldBuilder().getBuilder();
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder getCashOrBuilder() {
if ((specCase_ == 10) && (cashBuilder_ != null)) {
return cashBuilder_.getMessageOrBuilder();
} else {
if (specCase_ == 10) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
}
/**
*
* Payment made with hard cash.
*
*
* .opencannabis.commerce.Payment.CashPayment cash = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder>
getCashFieldBuilder() {
if (cashBuilder_ == null) {
if (!(specCase_ == 10)) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.getDefaultInstance();
}
cashBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPaymentOrBuilder>(
(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CashPayment) spec_,
getParentForChildren(),
isClean());
spec_ = null;
}
specCase_ = 10;
onChanged();;
return cashBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder> checkBuilder_;
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public boolean hasCheck() {
return specCase_ == 11;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment getCheck() {
if (checkBuilder_ == null) {
if (specCase_ == 11) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
} else {
if (specCase_ == 11) {
return checkBuilder_.getMessage();
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public Builder setCheck(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment value) {
if (checkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
spec_ = value;
onChanged();
} else {
checkBuilder_.setMessage(value);
}
specCase_ = 11;
return this;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public Builder setCheck(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder builderForValue) {
if (checkBuilder_ == null) {
spec_ = builderForValue.build();
onChanged();
} else {
checkBuilder_.setMessage(builderForValue.build());
}
specCase_ = 11;
return this;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public Builder mergeCheck(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment value) {
if (checkBuilder_ == null) {
if (specCase_ == 11 &&
spec_ != io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance()) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.newBuilder((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_)
.mergeFrom(value).buildPartial();
} else {
spec_ = value;
}
onChanged();
} else {
if (specCase_ == 11) {
checkBuilder_.mergeFrom(value);
}
checkBuilder_.setMessage(value);
}
specCase_ = 11;
return this;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public Builder clearCheck() {
if (checkBuilder_ == null) {
if (specCase_ == 11) {
specCase_ = 0;
spec_ = null;
onChanged();
}
} else {
if (specCase_ == 11) {
specCase_ = 0;
spec_ = null;
}
checkBuilder_.clear();
}
return this;
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder getCheckBuilder() {
return getCheckFieldBuilder().getBuilder();
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder getCheckOrBuilder() {
if ((specCase_ == 11) && (checkBuilder_ != null)) {
return checkBuilder_.getMessageOrBuilder();
} else {
if (specCase_ == 11) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
}
/**
*
* Payment made by check.
*
*
* .opencannabis.commerce.Payment.CheckPayment check = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder>
getCheckFieldBuilder() {
if (checkBuilder_ == null) {
if (!(specCase_ == 11)) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.getDefaultInstance();
}
checkBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPaymentOrBuilder>(
(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CheckPayment) spec_,
getParentForChildren(),
isClean());
spec_ = null;
}
specCase_ = 11;
onChanged();;
return checkBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder> cardBuilder_;
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public boolean hasCard() {
return specCase_ == 12;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment getCard() {
if (cardBuilder_ == null) {
if (specCase_ == 12) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
} else {
if (specCase_ == 12) {
return cardBuilder_.getMessage();
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public Builder setCard(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment value) {
if (cardBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
spec_ = value;
onChanged();
} else {
cardBuilder_.setMessage(value);
}
specCase_ = 12;
return this;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public Builder setCard(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder builderForValue) {
if (cardBuilder_ == null) {
spec_ = builderForValue.build();
onChanged();
} else {
cardBuilder_.setMessage(builderForValue.build());
}
specCase_ = 12;
return this;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public Builder mergeCard(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment value) {
if (cardBuilder_ == null) {
if (specCase_ == 12 &&
spec_ != io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance()) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.newBuilder((io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_)
.mergeFrom(value).buildPartial();
} else {
spec_ = value;
}
onChanged();
} else {
if (specCase_ == 12) {
cardBuilder_.mergeFrom(value);
}
cardBuilder_.setMessage(value);
}
specCase_ = 12;
return this;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public Builder clearCard() {
if (cardBuilder_ == null) {
if (specCase_ == 12) {
specCase_ = 0;
spec_ = null;
onChanged();
}
} else {
if (specCase_ == 12) {
specCase_ = 0;
spec_ = null;
}
cardBuilder_.clear();
}
return this;
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder getCardBuilder() {
return getCardFieldBuilder().getBuilder();
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder getCardOrBuilder() {
if ((specCase_ == 12) && (cardBuilder_ != null)) {
return cardBuilder_.getMessageOrBuilder();
} else {
if (specCase_ == 12) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
}
/**
*
* Payment made by debit or credit card.
*
*
* .opencannabis.commerce.Payment.CardPayment card = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder>
getCardFieldBuilder() {
if (cardBuilder_ == null) {
if (!(specCase_ == 12)) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.getDefaultInstance();
}
cardBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPaymentOrBuilder>(
(io.opencannabis.schema.commerce.CommercialPurchase.Payment.CardPayment) spec_,
getParentForChildren(),
isClean());
spec_ = null;
}
specCase_ = 12;
onChanged();;
return cardBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder> bankBuilder_;
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public boolean hasBank() {
return specCase_ == 13;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment getBank() {
if (bankBuilder_ == null) {
if (specCase_ == 13) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
} else {
if (specCase_ == 13) {
return bankBuilder_.getMessage();
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public Builder setBank(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment value) {
if (bankBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
spec_ = value;
onChanged();
} else {
bankBuilder_.setMessage(value);
}
specCase_ = 13;
return this;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public Builder setBank(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder builderForValue) {
if (bankBuilder_ == null) {
spec_ = builderForValue.build();
onChanged();
} else {
bankBuilder_.setMessage(builderForValue.build());
}
specCase_ = 13;
return this;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public Builder mergeBank(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment value) {
if (bankBuilder_ == null) {
if (specCase_ == 13 &&
spec_ != io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance()) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.newBuilder((io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_)
.mergeFrom(value).buildPartial();
} else {
spec_ = value;
}
onChanged();
} else {
if (specCase_ == 13) {
bankBuilder_.mergeFrom(value);
}
bankBuilder_.setMessage(value);
}
specCase_ = 13;
return this;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public Builder clearBank() {
if (bankBuilder_ == null) {
if (specCase_ == 13) {
specCase_ = 0;
spec_ = null;
onChanged();
}
} else {
if (specCase_ == 13) {
specCase_ = 0;
spec_ = null;
}
bankBuilder_.clear();
}
return this;
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder getBankBuilder() {
return getBankFieldBuilder().getBuilder();
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder getBankOrBuilder() {
if ((specCase_ == 13) && (bankBuilder_ != null)) {
return bankBuilder_.getMessageOrBuilder();
} else {
if (specCase_ == 13) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
}
/**
*
* Payment made by bank transfer.
*
*
* .opencannabis.commerce.Payment.BankPayment bank = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder>
getBankFieldBuilder() {
if (bankBuilder_ == null) {
if (!(specCase_ == 13)) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.getDefaultInstance();
}
bankBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPaymentOrBuilder>(
(io.opencannabis.schema.commerce.CommercialPurchase.Payment.BankPayment) spec_,
getParentForChildren(),
isClean());
spec_ = null;
}
specCase_ = 13;
onChanged();;
return bankBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder> digitalBuilder_;
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public boolean hasDigital() {
return specCase_ == 14;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment getDigital() {
if (digitalBuilder_ == null) {
if (specCase_ == 14) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
} else {
if (specCase_ == 14) {
return digitalBuilder_.getMessage();
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public Builder setDigital(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment value) {
if (digitalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
spec_ = value;
onChanged();
} else {
digitalBuilder_.setMessage(value);
}
specCase_ = 14;
return this;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public Builder setDigital(
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder builderForValue) {
if (digitalBuilder_ == null) {
spec_ = builderForValue.build();
onChanged();
} else {
digitalBuilder_.setMessage(builderForValue.build());
}
specCase_ = 14;
return this;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public Builder mergeDigital(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment value) {
if (digitalBuilder_ == null) {
if (specCase_ == 14 &&
spec_ != io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance()) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.newBuilder((io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_)
.mergeFrom(value).buildPartial();
} else {
spec_ = value;
}
onChanged();
} else {
if (specCase_ == 14) {
digitalBuilder_.mergeFrom(value);
}
digitalBuilder_.setMessage(value);
}
specCase_ = 14;
return this;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public Builder clearDigital() {
if (digitalBuilder_ == null) {
if (specCase_ == 14) {
specCase_ = 0;
spec_ = null;
onChanged();
}
} else {
if (specCase_ == 14) {
specCase_ = 0;
spec_ = null;
}
digitalBuilder_.clear();
}
return this;
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder getDigitalBuilder() {
return getDigitalFieldBuilder().getBuilder();
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
public io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder getDigitalOrBuilder() {
if ((specCase_ == 14) && (digitalBuilder_ != null)) {
return digitalBuilder_.getMessageOrBuilder();
} else {
if (specCase_ == 14) {
return (io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_;
}
return io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
}
/**
*
* Payment made via digital payment networks.
*
*
* .opencannabis.commerce.Payment.DigitalPayment digital = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder>
getDigitalFieldBuilder() {
if (digitalBuilder_ == null) {
if (!(specCase_ == 14)) {
spec_ = io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.getDefaultInstance();
}
digitalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment.Builder, io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPaymentOrBuilder>(
(io.opencannabis.schema.commerce.CommercialPurchase.Payment.DigitalPayment) spec_,
getParentForChildren(),
isClean());
spec_ = null;
}
specCase_ = 14;
onChanged();;
return digitalBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:opencannabis.commerce.Payment)
}
// @@protoc_insertion_point(class_scope:opencannabis.commerce.Payment)
private static final io.opencannabis.schema.commerce.CommercialPurchase.Payment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.opencannabis.schema.commerce.CommercialPurchase.Payment();
}
public static io.opencannabis.schema.commerce.CommercialPurchase.Payment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Payment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Payment(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.opencannabis.schema.commerce.CommercialPurchase.Payment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseLogEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_BillOfCharges_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_BillOfCharges_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_TicketItem_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_TicketItem_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseTimestamps_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseSignature_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseSignature_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseCustomer_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseCustomer_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PurchaseFacilitator_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_PaymentKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_PaymentKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_CashPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_CashPayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_CheckPayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_CardPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_CardPayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_BankPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_BankPayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_opencannabis_commerce_Payment_DigitalPayment_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\027commerce/Purchase.proto\022\025opencannabis." +
"commerce\032\024core/Datamodel.proto\032\026accounti" +
"ng/Taxes.proto\032\023commerce/Item.proto\032\027com" +
"merce/Currency.proto\032\030commerce/Discounts" +
".proto\032\037commerce/payments/Payment.proto\032" +
" inventory/InventoryProduct.proto\032\026crypt" +
"o/Signature.proto\032\026temporal/Instant.prot" +
"o\"\315\001\n\020PurchaseLogEntry\0225\n\006status\030\001 \001(\0162%" +
".opencannabis.commerce.PurchaseStatus\0223\n" +
"\005event\030\002 \001(\0162$.opencannabis.commerce.Pur" +
"chaseEvent\022/\n\007instant\030\003 \001(\0132\036.opencannab" +
"is.temporal.Instant\022\013\n\003sku\030\004 \001(\t\022\017\n\007mess" +
"age\030\005 \001(\t\"\355\001\n\rBillOfCharges\0221\n\006status\030\001 " +
"\001(\0162!.opencannabis.commerce.BillStatus\022$" +
"\n\003tax\030\002 \003(\0132\027.opencannabis.taxes.Tax\0221\n\010" +
"discount\030\003 \003(\0132\037.opencannabis.commerce.D" +
"iscount\022\r\n\005price\030\004 \001(\001\022\r\n\005taxes\030\005 \001(\001\022\021\n" +
"\tdiscounts\030\006 \001(\001\022\020\n\010subtotal\030\007 \001(\001\022\r\n\005to" +
"tal\030\010 \001(\001\"\253\001\n\nTicketItem\0221\n\003key\030\001 \001(\0132$." +
"opencannabis.inventory.InventoryKey\022\013\n\003s" +
"ku\030\002 \001(\t\022)\n\004item\030\003 \001(\0132\033.opencannabis.co" +
"mmerce.Item\0222\n\004line\030\004 \001(\0132$.opencannabis" +
".commerce.BillOfCharges\"\221\002\n\022PurchaseTime" +
"stamps\0223\n\013established\030\001 \001(\0132\036.opencannab" +
"is.temporal.Instant\022/\n\007created\030\002 \001(\0132\036.o" +
"pencannabis.temporal.Instant\0220\n\010modified" +
"\030\003 \001(\0132\036.opencannabis.temporal.Instant\0220" +
"\n\010executed\030\004 \001(\0132\036.opencannabis.temporal" +
".Instant\0221\n\tfinalized\030\005 \001(\0132\036.opencannab" +
"is.temporal.Instant\"#\n\013PurchaseKey\022\024\n\004uu" +
"id\030\001 \001(\tB\006\302\265\003\002\010\002\"\211\001\n\021PurchaseSignature\022\r" +
"\n\005nonce\030\001 \001(\t\0223\n\013facilitator\030\002 \001(\0132\036.ope" +
"ncannabis.crypto.Signature\0220\n\010customer\030\003" +
" \001(\0132\036.opencannabis.crypto.Signature\"b\n\020" +
"PurchaseCustomer\022\021\n\tunique_id\030\001 \001(\t\022;\n\ts" +
"ignature\030\002 \001(\0132(.opencannabis.commerce.P" +
"urchaseSignature\"\256\001\n\023PurchaseFacilitator" +
"\022;\n\tauthority\030\001 \001(\0162(.opencannabis.comme" +
"rce.PurchaseAuthority\022\r\n\005agent\030\002 \001(\t\022\016\n\006" +
"device\030\003 \001(\t\022;\n\tsignature\030\004 \001(\0132(.openca" +
"nnabis.commerce.PurchaseSignature\"\"\n\nPay" +
"mentKey\022\024\n\004uuid\030\001 \001(\tB\006\302\265\003\002\010\002\"\224\010\n\007Paymen" +
"t\0226\n\003key\030\001 \001(\0132!.opencannabis.commerce.P" +
"aymentKeyB\006\302\265\003\002\010\001\0224\n\006method\030\002 \001(\0162$.open" +
"cannabis.commerce.PaymentMethod\0224\n\006statu" +
"s\030\003 \001(\0162$.opencannabis.commerce.PaymentS" +
"tatus\022\016\n\006amount\030\004 \001(\001\022\014\n\004full\030\005 \001(\010\022:\n\004c" +
"ash\030\n \001(\0132*.opencannabis.commerce.Paymen" +
"t.CashPaymentH\000\022<\n\005check\030\013 \001(\0132+.opencan" +
"nabis.commerce.Payment.CheckPaymentH\000\022:\n" +
"\004card\030\014 \001(\0132*.opencannabis.commerce.Paym" +
"ent.CardPaymentH\000\022:\n\004bank\030\r \001(\0132*.openca" +
"nnabis.commerce.Payment.BankPaymentH\000\022@\n" +
"\007digital\030\016 \001(\0132-.opencannabis.commerce.P" +
"ayment.DigitalPaymentH\000\032{\n\013CashPayment\0226" +
"\n\010tendered\030\001 \001(\0132$.opencannabis.commerce" +
".CurrencyValue\0224\n\006change\030\002 \001(\0132$.opencan" +
"nabis.commerce.CurrencyValue\032|\n\014CheckPay" +
"ment\022\024\n\014check_number\030\001 \001(\t\022\026\n\016routing_nu" +
"mber\030\002 \001(\t\022\026\n\016account_number\030\003 \001(\t\022\023\n\013in" +
"stitution\030\004 \001(\t\022\021\n\tcertified\030\005 \001(\010\032H\n\013Ca" +
"rdPayment\0229\n\tcard_type\030\001 \001(\0162&.opencanna" +
"bis.commerce.PaymentCardType\032P\n\013BankPaym" +
"ent\022\026\n\016routing_number\030\001 \001(\t\022\026\n\016account_n" +
"umber\030\002 \001(\t\022\021\n\treference\030\003 \001(\t\032t\n\016Digita" +
"lPayment\022=\n\007network\030\001 \001(\0162,.opencannabis" +
".commerce.DigitalPaymentNetwork\022\020\n\010usern" +
"ame\030\002 \001(\t\022\021\n\treference\030\003 \001(\tB\006\n\004spec*\\\n\016" +
"PurchaseStatus\022\t\n\005FRESH\020\000\022\010\n\004OPEN\020\001\022\n\n\006C" +
"LOSED\020\002\022\n\n\006VOIDED\020\003\022\r\n\tFINALIZED\020\004\022\016\n\nRE" +
"CONCILED\020\005*=\n\021PurchaseAuthority\022\014\n\010STAND" +
"ARD\020\000\022\013\n\007MEDICAL\020\001\022\r\n\tADULT_USE\020\002*\312\001\n\rPu" +
"rchaseEvent\022\n\n\006STATUS\020\000\022\010\n\004SAVE\020\001\022\010\n\004LOA" +
"D\020\002\022\016\n\nITEM_ADDED\020\n\022\020\n\014ITEM_REMOVED\020\013\022\031\n" +
"\025ITEM_QUANTITY_CHANGED\020\014\022\027\n\023ITEM_DISCOUN" +
"T_ADDED\020\r\022\031\n\025ITEM_DISCOUNT_REMOVED\020\016\022\021\n\r" +
"PURCHASE_VOID\020\024\022\025\n\021PURCHASE_FINALIZE\020\025B?" +
"\n\037io.opencannabis.schema.commerceB\022Comme" +
"rcialPurchaseH\001P\000\242\002\003OCSb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
core.Datamodel.getDescriptor(),
io.opencannabis.schema.accounting.AccountingTaxes.getDescriptor(),
io.opencannabis.schema.commerce.OrderItem.getDescriptor(),
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor(),
io.opencannabis.schema.accounting.CommercialDiscounts.getDescriptor(),
io.opencannabis.schema.commerce.Payments.getDescriptor(),
io.opencannabis.schema.inventory.InventoryProductOuterClass.getDescriptor(),
io.opencannabis.schema.crypto.SignatureOuterClass.getDescriptor(),
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor(),
}, assigner);
internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_opencannabis_commerce_PurchaseLogEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseLogEntry_descriptor,
new java.lang.String[] { "Status", "Event", "Instant", "Sku", "Message", });
internal_static_opencannabis_commerce_BillOfCharges_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_opencannabis_commerce_BillOfCharges_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_BillOfCharges_descriptor,
new java.lang.String[] { "Status", "Tax", "Discount", "Price", "Taxes", "Discounts", "Subtotal", "Total", });
internal_static_opencannabis_commerce_TicketItem_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_opencannabis_commerce_TicketItem_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_TicketItem_descriptor,
new java.lang.String[] { "Key", "Sku", "Item", "Line", });
internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_opencannabis_commerce_PurchaseTimestamps_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseTimestamps_descriptor,
new java.lang.String[] { "Established", "Created", "Modified", "Executed", "Finalized", });
internal_static_opencannabis_commerce_PurchaseKey_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_opencannabis_commerce_PurchaseKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseKey_descriptor,
new java.lang.String[] { "Uuid", });
internal_static_opencannabis_commerce_PurchaseSignature_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_opencannabis_commerce_PurchaseSignature_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseSignature_descriptor,
new java.lang.String[] { "Nonce", "Facilitator", "Customer", });
internal_static_opencannabis_commerce_PurchaseCustomer_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_opencannabis_commerce_PurchaseCustomer_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseCustomer_descriptor,
new java.lang.String[] { "UniqueId", "Signature", });
internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_opencannabis_commerce_PurchaseFacilitator_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PurchaseFacilitator_descriptor,
new java.lang.String[] { "Authority", "Agent", "Device", "Signature", });
internal_static_opencannabis_commerce_PaymentKey_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_opencannabis_commerce_PaymentKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_PaymentKey_descriptor,
new java.lang.String[] { "Uuid", });
internal_static_opencannabis_commerce_Payment_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_opencannabis_commerce_Payment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_descriptor,
new java.lang.String[] { "Key", "Method", "Status", "Amount", "Full", "Cash", "Check", "Card", "Bank", "Digital", "Spec", });
internal_static_opencannabis_commerce_Payment_CashPayment_descriptor =
internal_static_opencannabis_commerce_Payment_descriptor.getNestedTypes().get(0);
internal_static_opencannabis_commerce_Payment_CashPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_CashPayment_descriptor,
new java.lang.String[] { "Tendered", "Change", });
internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor =
internal_static_opencannabis_commerce_Payment_descriptor.getNestedTypes().get(1);
internal_static_opencannabis_commerce_Payment_CheckPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_CheckPayment_descriptor,
new java.lang.String[] { "CheckNumber", "RoutingNumber", "AccountNumber", "Institution", "Certified", });
internal_static_opencannabis_commerce_Payment_CardPayment_descriptor =
internal_static_opencannabis_commerce_Payment_descriptor.getNestedTypes().get(2);
internal_static_opencannabis_commerce_Payment_CardPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_CardPayment_descriptor,
new java.lang.String[] { "CardType", });
internal_static_opencannabis_commerce_Payment_BankPayment_descriptor =
internal_static_opencannabis_commerce_Payment_descriptor.getNestedTypes().get(3);
internal_static_opencannabis_commerce_Payment_BankPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_BankPayment_descriptor,
new java.lang.String[] { "RoutingNumber", "AccountNumber", "Reference", });
internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor =
internal_static_opencannabis_commerce_Payment_descriptor.getNestedTypes().get(4);
internal_static_opencannabis_commerce_Payment_DigitalPayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_opencannabis_commerce_Payment_DigitalPayment_descriptor,
new java.lang.String[] { "Network", "Username", "Reference", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(core.Datamodel.field);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
core.Datamodel.getDescriptor();
io.opencannabis.schema.accounting.AccountingTaxes.getDescriptor();
io.opencannabis.schema.commerce.OrderItem.getDescriptor();
io.opencannabis.schema.currency.CommerceCurrency.getDescriptor();
io.opencannabis.schema.accounting.CommercialDiscounts.getDescriptor();
io.opencannabis.schema.commerce.Payments.getDescriptor();
io.opencannabis.schema.inventory.InventoryProductOuterClass.getDescriptor();
io.opencannabis.schema.crypto.SignatureOuterClass.getDescriptor();
io.opencannabis.schema.temporal.TemporalInstant.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy